用struts上传多个文件的方法
### 使用Struts框架进行多文件上传的技术解析 在Web应用开发中,文件上传是一个非常常见的需求。Apache Struts作为一款成熟的MVC框架,在处理文件上传方面有着独特的优势。本文将详细解析如何使用Struts框架实现多文件上传的功能,并对相关的代码进行深入分析。 #### 一、Struts框架简介 Struts是一款开源的Java MVC(Model-View-Controller)框架,它简化了Web应用程序的开发过程,提供了一种结构化的方式来组织代码。Struts的核心是ActionServlet,它是整个框架的控制中心,负责接收客户端请求并将其分发到对应的Action对象上执行。此外,Struts还提供了ActionForm类来封装表单数据,以及一系列用于验证、国际化等功能的支持。 #### 二、文件上传的需求分析 在Web应用中,文件上传通常涉及以下几个步骤: 1. **前端表单设计**:用户界面需要设计一个包含文件输入框的表单。 2. **后端处理逻辑**:服务器端需要能够解析这些文件,并进行相应的处理,如存储到数据库或文件系统中。 3. **异常处理**:需要处理可能出现的各种异常情况,比如文件大小超过限制等。 #### 三、实现多文件上传的关键技术点 ##### 3.1 配置Struts 在Struts框架中实现多文件上传功能之前,首先需要确保Struts配置正确。这包括但不限于`struts-config.xml`和`web.xml`的设置。 - **`struts-config.xml`**: 需要在配置文件中定义上传Action及其映射关系。 - **`web.xml`**: 在`web.xml`中配置MultipartRequestHandler过滤器,以便处理多部分请求。 ##### 3.2 设计ActionForm 为了封装上传表单的数据,我们需要创建一个继承自`ActionForm`的类,这里我们以`UpLoadForm`为例: ```java public class UpLoadForm extends ActionForm { protected FormFile theFile; protected FormFile theFile2; // Getter和Setter方法 public FormFile getTheFile() { return theFile; } public void setTheFile(FormFile theFile) { this.theFile = theFile; } public FormFile getTheFile2() { return theFile2; } public void setTheFile2(FormFile theFile2) { this.theFile2 = theFile2; } // 验证方法 public ActionErrors validate(ActionMapping mapping, HttpServletRequest request) { ActionErrors errors = null; Boolean maxLengthExceeded = (Boolean) request.getAttribute(MultipartRequestHandler.ATTRIBUTE_MAX_LENGTH_EXCEEDED); if ((maxLengthExceeded != null) && (maxLengthExceeded.booleanValue())) { errors = new ActionErrors(); errors.add("maxLengthExceeded", new ActionError("maxLengthExceeded")); } return errors; } } ``` 这里定义了两个文件上传字段`theFile`和`theFile2`,并通过`validate`方法检查文件大小是否超过了最大限制。 ##### 3.3 实现Action 接下来需要创建一个Action类来处理文件上传的具体逻辑。以`UpLoadAction`为例: ```java public class UpLoadAction extends Action { public ActionForward execute(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception { String encoding = request.getCharacterEncoding(); if (encoding == null) { encoding = "UTF-8"; } UpLoadForm uploadForm = (UpLoadForm) form; FormFile file = uploadForm.getTheFile(); FormFile file2 = uploadForm.getTheFile2(); // 进行文件保存等逻辑处理 // ... // 返回结果页面 return mapping.findForward("success"); } } ``` 在`execute`方法中,通过`UpLoadForm`对象获取上传的文件,并进行后续处理。 #### 四、总结 通过上述步骤,我们可以成功地在Struts框架中实现多文件上传功能。需要注意的是,实际开发过程中还需要考虑安全性、用户体验等方面的问题。例如,可以增加文件类型的限制、使用异步上传提高用户体验等。此外,随着技术的发展,目前更多项目可能会选择Spring MVC等更现代的框架来实现类似功能,但Struts作为一种经典框架仍然具有一定的参考价值。
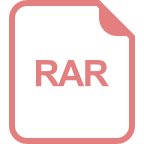
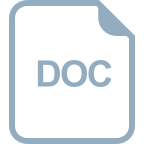
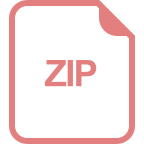
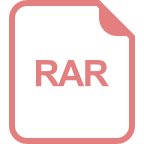
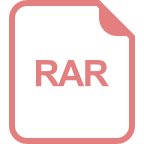
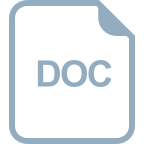
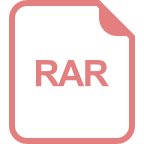
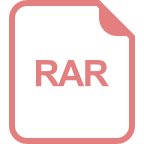
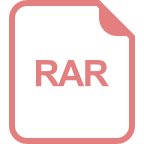
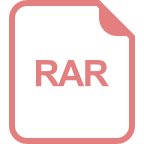
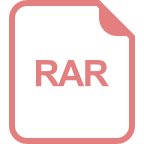
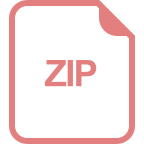
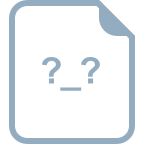
package com.cnehu.struts.form;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts.action.ActionError;
import org.apache.struts.action.ActionErrors;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.upload.FormFile;
import org.apache.struts.upload.MultipartRequestHandler;
/**
*
* Title:UpLoadForm
*
*
* Copyright: Copyright (c) 2005 techyang ;http://blog.csdn.net/techyang
*
* @author techyang
* @version 1.0
*/
public class UpLoadForm extends ActionForm
{
public static final String ERROR_PROPERTY_MAX_LENGTH_EXCEEDED = "org.apache.struts.webapp.upload.MaxLengthExceeded";
protected FormFile theFile;
protected FormFile theFile2;
public FormFile getTheFile()
{
return theFile;
}
public void setTheFile(FormFile theFile)
{
this.theFile = theFile;
}
public ActionErrors validate(ActionMapping mapping,
HttpServletRequest request)
{
ActionErrors errors = null;
//has the maximum length been exceeded?
Boolean maxLengthExceeded = (Boolean) request
.getAttribute(MultipartRequestHandler.ATTRIBUTE_MAX_LENGTH_EXCEEDED);
if ((maxLengthExceeded != null) && (maxLengthExceeded.booleanValue()))
{
errors = new ActionErrors();
errors.add(ERROR_PROPERTY_MAX_LENGTH_EXCEEDED, new ActionError(
"maxLengthExceeded"));
}
剩余8页未读,继续阅读
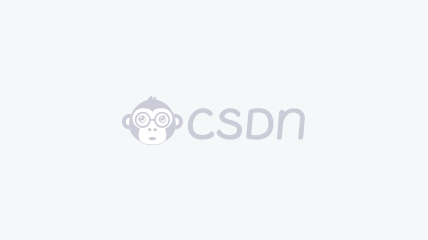

- 粉丝: 43
- 资源: 8
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

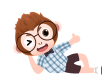
最新资源
- asm-西电微机原理实验
- Arduino-arduino
- C语言-leetcode题解之70-climbing-stairs.c
- C语言-leetcode题解之68-text-justification.c
- C语言-leetcode题解之66-plus-one.c
- C语言-leetcode题解之64-minimum-path-sum.c
- C语言-leetcode题解之63-unique-paths-ii.c
- C语言-leetcode题解之62-unique-paths.c
- C语言-leetcode题解之61-rotate-list.c
- C语言-leetcode题解之59-spiral-matrix-ii.c

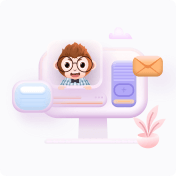
