超级网络入门与实践相关的实例
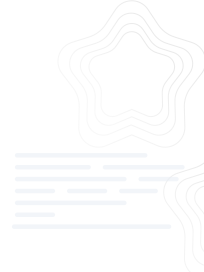


《超级网络入门与实践》系列教程是一套针对网络技术初学者和爱好者的宝贵资源,它涵盖了网络编程的基础以及网络协议的深入理解。本压缩包文件包含的实例将帮助你通过实践来巩固理论知识,增强对网络操作的直观认识。下面我们将详细探讨这个系列的四个部分及其相关知识点。 (一) 网络脚本语言基础 这部分内容主要讲解如何使用脚本语言进行网络编程。脚本语言如Python、JavaScript和Perl在处理网络任务时非常灵活和高效。它们可以用来创建网络爬虫、自动化测试、数据抓取等。学习网络脚本语言基础包括了解基本语法、HTTP请求方法(GET和POST)、解析响应数据以及错误处理。此外,还会涉及网络库的使用,如Python的requests库和BeautifulSoup库,这些库简化了网络交互和数据解析的过程。 (二) 学习TCP、IP、Ethernet协作的原理 TCP/IP协议栈是互联网通信的核心,而以太网(Ethernet)是局域网中最常见的通信标准。在这一部分,你会了解到TCP(传输控制协议)和IP(互联网协议)是如何确保数据可靠传输的,包括TCP的三次握手、四次挥手、滑动窗口机制以及IP的分片和重组。同时,以太网协议的工作原理,如MAC地址、CSMA/CD(载波监听多路访问/冲突检测)和帧结构也会被详细阐述。 (三) 完全理解ICMP Internet Control Message Protocol(ICMP)是网络层协议,用于报告错误和传递控制信息。通过学习ICMP,你可以理解如何处理网络中的问题,如目标不可达、超时和回显请求(ping)。ICMP报文的类型和代码,以及它们在网络故障诊断中的应用,是这部分的重点。 (四) 深入学习DHCP Dynamic Host Configuration Protocol(DHCP)负责自动分配IP地址、子网掩码、默认网关等网络配置信息。深入学习DHCP包括了解其工作流程,如DORA(Discover、Offer、Request、Acknowledgement)过程,以及服务器和客户端的角色。此外,你还将学习到如何配置DHCP服务器,以及在不同网络环境中考虑的策略和安全问题。 在压缩包内的"sample"文件中,可能包含了与以上各部分对应的实践代码、实验说明或者测试用例。通过实际运行和分析这些例子,你将能够更好地理解和应用所学知识,提升网络技术技能。无论是对于网络管理员、开发人员还是普通用户,这个系列的学习都将大大增进对网络运作机制的理解,从而在日常工作中更加游刃有余。
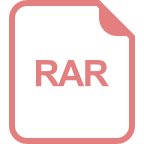
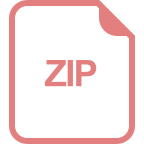
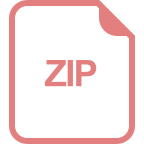
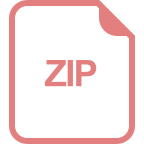
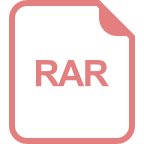
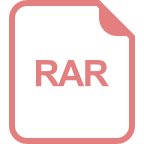
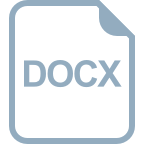
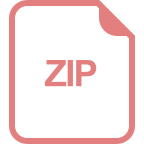
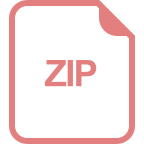
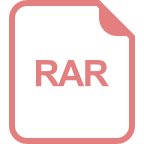
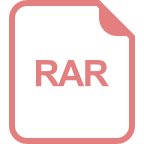
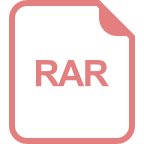
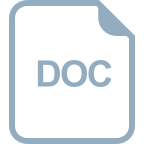
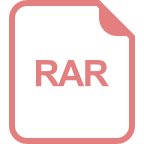
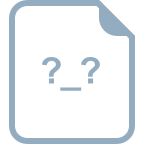
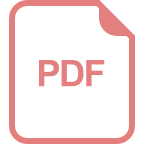
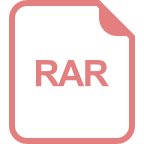
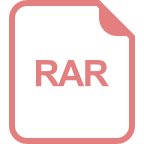
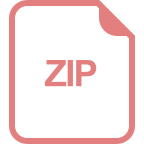
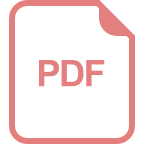
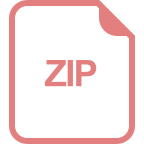
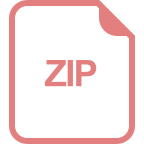
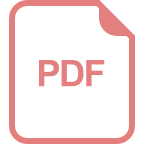
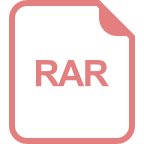
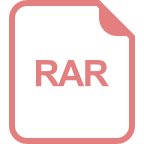
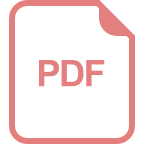


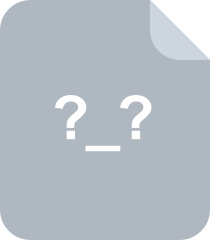
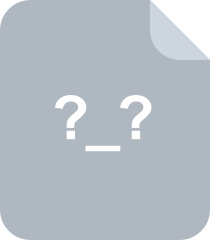
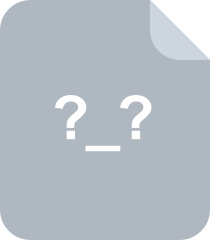
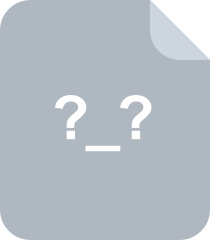
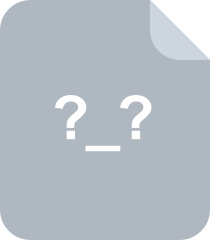
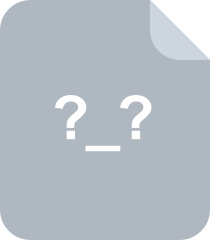
- 1
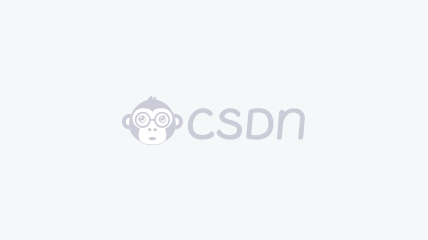
- millons2012-07-31是.py程序,不知道用什么工具打开。
- liuzhijingquan2012-06-21对于学习网络协议很有用,可以收藏学习

- 粉丝: 0
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

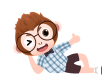
最新资源
- 2024年江西省职业院校技能大赛:GZ015-机器人系统集成应用技术(学生赛)赛项(高职组)样题
- 适用Centos7/8/9的libpcap、libpcap-devel离线安装包rpm
- MPC模型预测控制,风电调频,风储调频 在风储调频基础上加了MPC控制,复现的EI文献 MPC控制预测频率变化,进而改变风电出力 实时改变风电出力调频 创新就是, 仿真对比了实际仿真和在MP
- 圆管自动上料机sw16可编辑全套技术资料100%好用.zip
- NSFileNotFoundError如何解决.md
- TabError.md
- GlobalMixinError解决办法.md
- SystemError.md
- MalformedURLException(解决方案).md
- SystemExit.md
- FilterError解决办法.md
- UnknownHostException(解决方案).md
- NSFileAlreadyExistsError如何解决.md
- ConnectException(解决方案).md
- NSOutOfMemoryError如何解决.md
- DependencyInjectionError解决办法.md

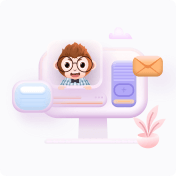
