在C#编程环境中,实现画线和简单的画图功能是一项基本任务,这通常涉及到Windows Forms或WPF(Windows Presentation Foundation)的应用开发。本教程将基于C# 4.0框架,教你如何创建一个用户界面,允许用户通过鼠标拖动来绘制线条。 你需要创建一个新的Windows Forms应用程序项目。在Visual Studio中,选择"File" -> "New" -> "Project",然后在模板列表中找到"C# Windows Forms Application",并为其命名。 **创建控件与事件处理** 在设计视图中,将一个`PictureBox`控件拖放到窗体上,用于显示绘图区域。设置其`SizeMode`属性为`StretchImage`,以便自适应大小。此外,还需要添加两个按钮,一个用于清除画布,另一个用于保存图片。 接下来,我们需要监听鼠标的事件。在代码编辑器中,为`PictureBox`控件添加以下事件处理程序: 1. `MouseDown`:当用户按下鼠标时,记录起始坐标。 2. `MouseMove`:当鼠标移动时,如果鼠标被按下,就在`PictureBox`上画线。 3. `MouseUp`:当用户释放鼠标时,停止画线。 **绘图逻辑** 在`MouseMove`事件处理程序中,你可以使用`Graphics`对象来绘制线条。获取`PictureBox`的`CreateGraphics()`实例,然后使用`DrawLine`方法,传入起始点和当前鼠标位置作为参数。为了保证线条的平滑,可以使用`GraphicsPath`和`DrawPath`。 ```csharp private Point startPoint; private GraphicsPath graphicsPath; private void pictureBox_MouseDown(object sender, MouseEventArgs e) { startPoint = e.Location; graphicsPath = new GraphicsPath(); graphicsPath.AddLine(startPoint, startPoint); } private void pictureBox_MouseMove(object sender, MouseEventArgs e) { if (e.Button == MouseButtons.Left) { graphicsPath.AddLine(startPoint, e.Location); pictureBox.Invalidate(); startPoint = e.Location; } } private void pictureBox_Paint(object sender, PaintEventArgs e) { using (Pen pen = new Pen(Color.Black, 3)) { e.Graphics.DrawPath(pen, graphicsPath); } } ``` **清除和保存功能** 对于清除画布,可以重置`graphicsPath`并在`pictureBox_Paint`事件中清除图像。保存图片则可以使用`pictureBox.Image.Save`方法。 ```csharp private void clearButton_Click(object sender, EventArgs e) { graphicsPath = new GraphicsPath(); pictureBox.Invalidate(); } private void saveButton_Click(object sender, EventArgs e) { if (pictureBox.Image != null) { SaveFileDialog saveFileDialog = new SaveFileDialog(); saveFileDialog.Filter = "JPEG Image|*.jpg|Bitmap Image|*.bmp|PNG Image|*.png"; if (saveFileDialog.ShowDialog() == DialogResult.OK) { pictureBox.Image.Save(saveFileDialog.FileName); } } } ``` 以上代码实现了一个基本的画线应用,用户可以通过拖动鼠标在`PictureBox`上画线,并能清除画布或保存图片。为了完善这个应用,可以考虑增加颜色选择、线型选择、橡皮擦功能等。同时,为了提高性能,避免频繁调用`pictureBox.Invalidate()`,可以考虑使用双缓冲技术,减少闪烁现象。 请注意,这个例子假设你对C#和Windows Forms有一定的了解。如果你是初学者,建议先学习基础语法和控件使用。如果你想要了解更多关于C#图形编程的知识,可以查阅.NET Framework的图形系统文档,包括GDI+和Direct2D等高级话题。
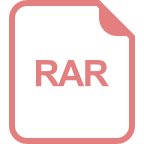
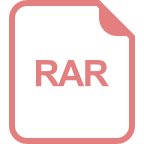
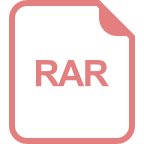
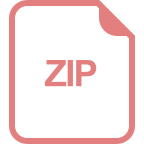
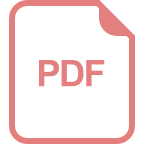
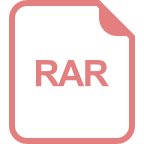
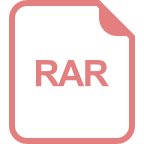





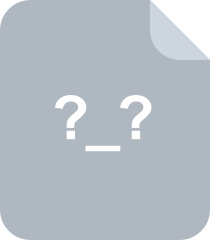
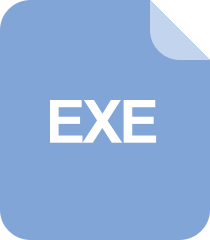
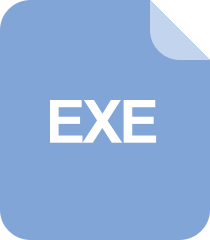
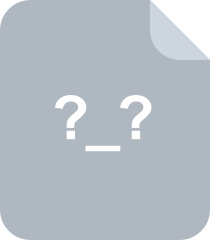
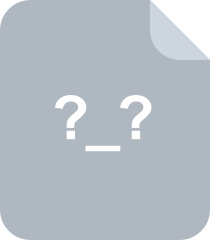
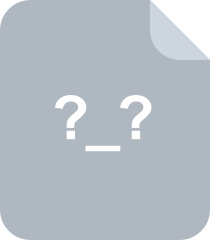
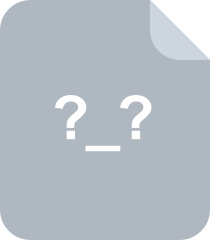



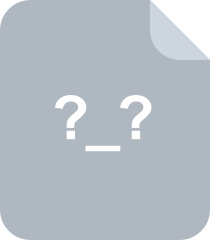
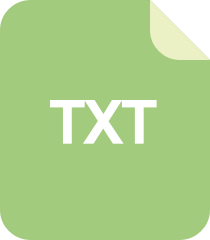
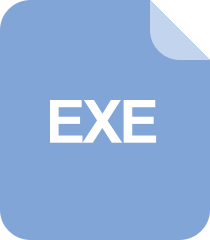
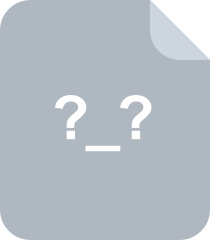
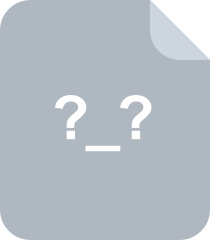
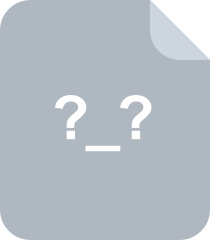
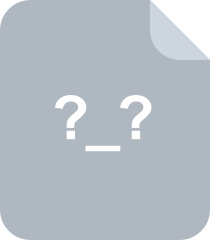

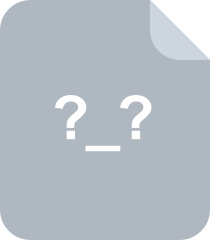
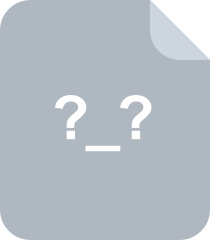
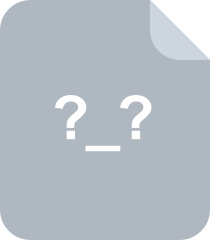
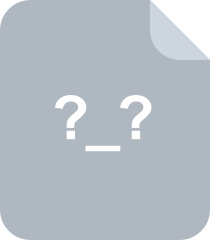
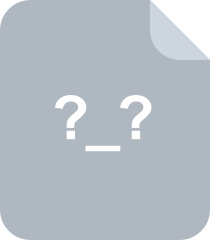
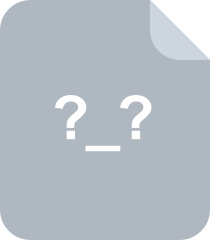
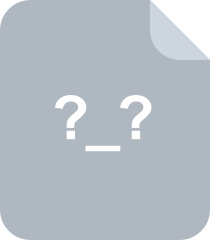
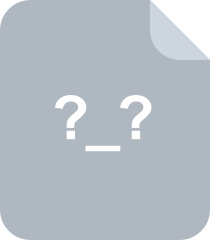

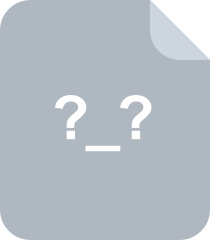
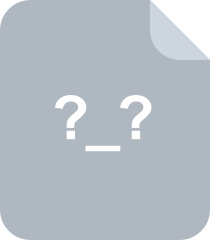
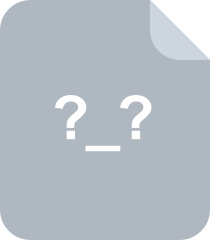
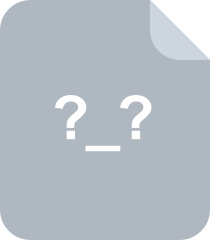
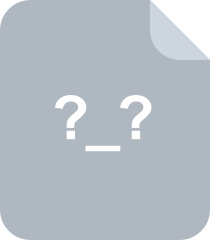
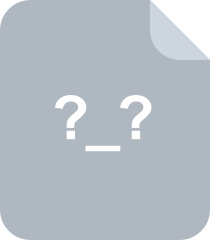
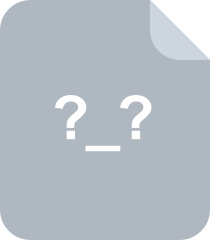
- 1
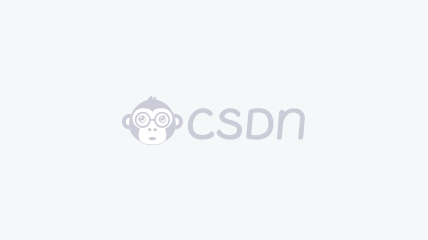

- 粉丝: 35
- 资源: 8
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

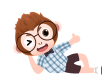
