<html>
<head>
<title>弹碰小游戏</title>
<script src="jscex.jscexRequire.min.js" type="text/javascript"></script>
<style type="text/css">
#main{border-radius:10px;width:300px;height:500px;border: 10px solid #8B8989;margin-left:auto;margin-right:auto;margin-top:50px;}
</style>
</head>
<body>
<div id="main">
<!--将画布嵌在div块里面,使其可以居中-->
<canvas id="liuming_canvas" width="300px" height="500px">
</canvas>
</div>
<!--主要的javascript代码--->
<script type="text/javascript">
var canvas = document.getElementById('liuming_canvas');
var cxt = canvas.getContext('2d');
//初始化画布的颜色
function init_canvas_background(){
//设置画布背景颜色
cxt.fillStyle = "#000000";
cxt.fillRect(0, 0, canvas.width, canvas.height);
}
//定义一个“方块” 主要用于击打小球和反弹
var diamond = {
x : 100,
y : 485,
width : 100,
height : 15,
move : 10
}
//定义一个小球 用于击打 “小方块”
var ball_impact = {
x : 150,
y : 465,
r : 10,
vx : 200,
vy : 200
}
//先定义一组被击打的小方块
var diamond_impact = [];
diamond_impact.length = 0;
var width_span = 25; // 任意两个小方块的横向间隔
var height_span = 25; //任意两个小方块的水平间隔
for(var i =1 ; i <=10 ; i++){//控制每行输出的小方块
for(var j = 1 ; j < 10 ; j++){//输出每列的小方块 只有x轴和y轴的坐标不一样而已
var diamond_impact_children = {
x : width_span,
y : height_span,
width : 10,
height : 10
};
width_span += 30;
diamond_impact.push(diamond_impact_children); //将得到的小方块放在 diamond_impact 中,已被以后使用
}
height_span += 25;
width_span = 25;
}
//初始化所有被击打的小方块
function init_diamond_impact(){
for(var i in diamond_impact){
cxt.fillStyle = "#EAFA03";
cxt.fillRect(diamond_impact[i].x,diamond_impact[i].y,diamond_impact[i].width,diamond_impact[i].height);
}
}
//初始化小球
function init_ball_impact(){
cxt.fillStyle = "#FFFFFF";
cxt.arc(ball_impact.x,ball_impact.y,ball_impact.r,0,Math.PI * 2,true);
cxt.fill();
}
//初始化方块
function init_diamond(){
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}
//擦除方框
function clear_diamond(){
cxt.clearRect(diamond.x,diamond.y,diamond.width,diamond.height);
}
//定义四个方法来重绘制方块的位置 分别有 左、右、上、下
function move_right_diamond(){
clear_diamond();//清除以前的方块
init_canvas_background();//再次初始化画布 下同
//重新绘制小方块的位置
if(diamond.x + diamond.width >= canvas.width){ //判断方块是否已经到达最右端
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}else{
diamond.x += diamond.move;
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}
}
function move_left_diamond(){
clear_diamond();
init_canvas_background();
//重新绘制小方块的位置
if(diamond.x <= 0){ //判断方块是否已经到达最右端
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}else{
diamond.x -= diamond.move;
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}
}
function move_up_diamond(){
clear_diamond();
init_canvas_background();
//重新绘制小方块的位置
if(diamond.y <= 300){ //判断方块是否已经到达最右端
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}else{
diamond.y -= diamond.move;
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}
}
function move_down_diamond(){
clear_diamond();
init_canvas_background();
//重新绘制小方块的位置
if(diamond.y + diamond.height >= 500){ //判断方块是否已经到达最右端
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}else{
diamond.y += diamond.move;
cxt.fillStyle = "#17F705";
cxt.fillRect(diamond.x,diamond.y,diamond.width,diamond.height);
}
}
//键盘事件,获取当前在那个方向运动
var direction = "";
document.onkeydown = function (e) {
if (e.keyCode == 37 ) direction = "left" ;
if (e.keyCode == 39 ) direction = "right";
if (e.keyCode == 38 ) direction = "up";
if (e.keyCode == 40 ) direction = "down";
}
//让小球移动起来 动态绘制移动的路径
var cyc = 10;
var somethingAsync = eval(Jscex.compile("async", function () {
while (true) {
cxt.fillStyle = "rgba(0,0,0,1)";
cxt.fillRect(0,0,canvas.width,canvas.height);
cxt.fillStyle = "#fff";
cxt.fill();
cxt.beginPath();
cxt.arc(ball_impact.x,ball_impact.y,ball_impact.r,0,Math.PI * 2,true);
cxt.closePath();
cxt.fill();
ball_impact.x += ball_impact.vx * cyc /1000;
ball_impact.y += ball_impact.vy * cyc /1000;
//显示一组用来被击打的小方块
init_diamond_impact();
//判断小球碰壁状态
if(ball_impact.x + ball_impact.r >= canvas.width || ball_impact.x <= ball_impact.r) ball_impact.vx *= -1;
if(ball_impact.y <= ball_impact.r) ball_impact.vy *= -1;
//判断游戏是否失败
if(ball_impact.y + ball_impact.r >= canvas.height){
cxt.fillStyle = "#FC0000";
cxt.font = "bold 50px 微软雅黑";
cxt.fillText("FAILURE!",30,250);
diamond.move = 0;//不能移动板块
}
init_diamond();
//判断方块的移动
if (direction == "right") {
move_right_diamond();
direction = "";//清除当前的默认位置
}
if (direction == "left") {
move_left_diamond();
direction = "";//清除当前的默认位置
}
if (direction == "up") {
move_up_diamond();
direction = "";//清除当前的默认位置
}
if (direction == "down") {
move_down_diamond();
direction = "";//清除当前的默认位置
}
//判断小球与方块接触碰撞的方法
//(注意:x轴与y轴都要判断,在于小球必须在方框上才能够反弹运动)
if(ball_impact.y + ball_impact.r == diamond.y){
if(ball_impact.x + ball_impact.r >= diamond.x && ball_impact.x - ball_impact.r <= diamond.x + diamond.width){//当球在左边
ball_impact.vy *= -1;
}
}
//判断小方块和小球的位置,以判断他们是否被击中
// 注意:要判断小球和小方块的x轴和y轴的坐标位置 (循环判断所有的小方块和当前的小球的位置关系)
for(var i in diamond_impact){
if(ball_impact.x + ball_impact.r >= diamond_impact[i].x && ball_impact.x - ball_impact.r <= diamond_impact[i].x + diamond_impact[i].width){//先判断x轴的位置
if(ball_impact.y - ball_impact.r <= diamond_impact[i].y + diamond_impact[i].height && ball_impact.y + ball_impact.r >= diamond_impact[i].y){
diamond_impact[i].width = 0;
diamond_impact[i].height = 0;
cxt.clearRect(diamond_impact[i].x,diamond_impact[i].y,diamond_impact[i].width,diamond_impact[i].height);
}
}
}
//判断是否已经消灭了所有的小球
var count_sum = 0;
for(var i in diamond_impact){
if(diamond_impact[i].width == 0){
count_sum ++;
}
}
//判断是否与所有的小方块数相等
if(count_sum == 90){
cxt.fillStyle = "#FCF205";
cxt.font = "bold 50px 微软雅黑";
cxt.fillText("SUCCESS!",20,250);
diamond.move = 0;//不能移动板块

我有一个小毛驴
- 粉丝: 84
- 资源: 9
最新资源
- Keil C51 插件 检测所有if语句
- 各种排序算法java实现的源代码.zip
- 金山PDF教育版编辑器
- 基于springboot+element的校园服务平台源代码项目包含全套技术资料.zip
- 自动化应用驱动的容器弹性管理平台解决方案
- 各种排序算法 Python 实现的源代码
- BlurAdmin 是一款使用 AngularJs + Bootstrap实现的单页管理端模版,视觉冲击极强的管理后台,各种动画效果
- 基于JSP+Servlet的网上书店系统源代码项目包含全套技术资料.zip
- GGJGJGJGGDGGDGG
- 基于SpringBoot的毕业设计选题系统源代码项目包含全套技术资料.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


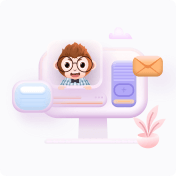