/*
* compiler/back_ends/c++_gen/gen_code.c - routines for printing C++ code from type trees
*
* assumes that the type tree has already been run through the
* c++ type generator (c++_gen/types.c).
*
* This was hastily written - it has some huge routines in it.
* Needs a lot of cleaning up and modularization...
*
* Mike Sample
* 92
* Copyright (C) 1991, 1992 Michael Sample
* and the University of British Columbia
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* $Header: /usr/app/odstb/CVS/snacc/compiler/back-ends/c++-gen/gen-code.c,v 1.12 1997/02/28 13:39:53 wan Exp $
* $Log: gen-code.c,v $
*
* Revision 1.4.1 2004/03/02
* Daniel Ferenci - Siemens
* added BASICTYPE_CHOICE
* added automatic distinguishig of ASN ANY type (what is behind)
*
* Revision 1.12 1997/02/28 13:39:53 wan
* Modifications collected for new version 1.3: Bug fixes, tk4.2.
*
* Revision 1.11 1997/02/16 15:14:06 rj
* made return *this after calling abort()'' a compile time option.
*
* Revision 1.10 1997/02/16 12:46:31 rj
* use the TIME_WITH_SYS_TIME flag (checked and generated by configure).
* return *this after calling abort() for compilers that don't know about this volatile function.
* comment out unused parameters, the compiler otherwise may complain.
*
* Revision 1.9 1995/09/07 20:47:32 rj
* deep copying assingment operators added.
*
* Revision 1.8 1995/09/07 19:25:27 rj
* PrintCxxCode(): boolean genMeta changed to enum type MetaNameStyle. used globally in printMetaG.
*
* set Tcl's errorCode variable.
*
* Revision 1.7 1995/08/17 15:00:06 rj
* the PDU flag belongs to the metacode, not only to the tcl interface. (type and variable named adjusted)
*
* Revision 1.6 1995/07/27 10:52:28 rj
* include config.h before using its #define's :-)
*
* file name has been shortened for redundant part: c++-gen/gen-c++-code -> c++-gen/gen-code.
*
* functions used only locally made static.
*
* #if TCL ... #endif wrapped into #if META ... #endif, both here and in generated files.
*
* code changes to allow for more than one PDU (meta code), e.g. generate -create() functions.
*
* generate additional TclUnsetVal() function to delete OPTIONAL members and SEQUENCE OF and SET OF list elements.
*
* _getref() gets an additional optional argument to faciliate the different member access semantics of TclGetVal() and TclSetVal().
*
* the list functions Append(), Prepend(), InsertBefore() and InsertAfter() now set the current element to the element just inserted.
*
* changed `_' to `-' in file names.
*
* Revision 1.5 1995/02/18 14:45:16 rj
* tried to make the print function's output a little more readable. [kho]
*
* Revision 1.4 1994/10/08 03:19:24 rj
* since i was still irritated by cpp standing for c++ and not the C preprocessor, i renamed them to cxx (which is one known suffix for C++ source files). since the standard #define is __cplusplus, cplusplus would have been the more obvious choice, but it is a little too long.
*
* turned the functions order upside down to get rid of those annoying declarations.
*
* turned character pointers into constant character arrays.
*
* code for meta structures added (provides information about the generated code itself).
*
* code for Tcl interface added (makes use of the above mentioned meta code).
*
* instead of being a no-op, the no-arg-constructors (that get used by Clone()) do something useful now, namely:
* - initialize the pointer in a choice union. (the destruktor may try to free the bogus pointer).
* - for the same reason: initialize pointers in sequences and sets.
*
* to complement the destructors, T::T (const T&) and T &T::operator = (const T &) have been added to override the defaults supplied by the compiler.
* reason: simple pointer duplication may lead to unreferenced objects and to objects referenced more than once (on which the destructors delete may choke).
*
* virtual inline functions (the destructor and the Clone() function) moved from inc/*.h to src/*.C because g++ turns every one of them into a static non-inline function in every file where the .h file gets included.
*
* made Print() const (and some other, mainly comparison functions).
*
* Revision 1.3 1994/09/01 00:16:29 rj
* change of IBM ENC integrated: large inlines turned into normal functions.
* more portable .h file inclusion.
*
* Revision 1.2 1994/08/31 09:49:05 rj
* for the C++ code generated: turned TRUE/FALSE into true/false;
* the keyword `struct' had to be removed before AsnListElmt, or gcc 2.6 wouldn't compile the generated code.
*
* Revision 1.1 1994/08/28 09:48:01 rj
* first check-in. for a list of changes to the snacc-1.1 distribution please refer to the ChangeLog.
*
*/
#include "snacc.h"
#if TIME_WITH_SYS_TIME
# include <sys/time.h>
# include <time.h>
#else
# if HAVE_SYS_TIME_H
# include <sys/time.h>
# else
# include <time.h>
# endif
#endif
#if STDC_HEADERS || HAVE_STRING_H
#include <string.h>
#else
#include <strings.h>
#endif
#include <stdio.h>
#include "asn-incl.h"
#include "asn1module.h"
#include "define.h"
#include "mem.h"
#include "lib-types.h"
#include "rules.h"
#include "types.h"
#include "cond.h"
#include "str-util.h"
#include "snacc-util.h"
#include "print.h"
#include "tag-util.h" /* get GetTags/FreeTags/CountTags/TagByteLen */
#if META
#include "meta.h"
#endif
#include "gen-vals.h"
#include "gen-any.h"
#include "gen-code.h"
static const char bufTypeNameG[] = "BUF_TYPE";
static const char lenTypeNameG[] = "AsnLen";
static const char tagTypeNameG[] = "AsnTag";
static const char envTypeNameG[] = "ENV_TYPE";
static long int longJmpValG = -100;
static const char baseClassesG[] = ": public AsnType";
static int printTypesG;
static int printEncodersG;
static int printDecodersG;
static int printPrintersG;
static int printFreeG;
#if META
static MetaNameStyle printMetaG;
static MetaPDU *meta_pdus_G;
#if TCL
static int printTclG;
#endif
#endif /* META */
static void
PrintHdrComment PARAMS ((hdr, m),
FILE *hdr _AND_
Module *m)
{
time_t now = time (NULL);
fprintf (hdr, "// NOTE: this is a machine generated file--editing not recommended\n");
fprintf (hdr, "//\n");
fprintf (hdr, "// %s - class definitions for ASN.1 module %s\n", m->cxxHdrFileName, m->modId->name);
fprintf (hdr, "//\n");
fprintf (hdr, "// This file was generated by snacc on %s", ctime (&now));
fprintf (hdr, "// UBC snacc by Mike Sample\n");
fprintf (hdr, "// A couple of enhancements made by IBM European Networking Center\n"); /* 20.8.93 Thomas Meyer */
fprintf (hdr, "\n");
} /* PrintHdrComment */
static void
PrintSrcComment PARAMS ((src, m),
FILE *src _AND_
Module *m)
{
time_t now = time (NULL);
fprintf (src, "// NOTE: this is a machine generated file--editing not recommended\n");
fprintf (src, "//\n");
fprintf (src, "// %s - class member functions for ASN.1 module %s\n", m->cxxSrcFileName, m->modId->name);
fprintf (src, "//\n");
fprintf (src, "// This file was generated by snacc on %s", ctime (&now));
fprintf (src, "// UBC snacc written by Mike Sample\n");
fprintf (src, "// A couple of enhancements made by IBM European Networking Center\n"); /* 20.8.93 Thomas Meyer */
fprintf (src, "\n");
} /* PrintSrcComment */
static void
PrintSrcIncludes PARAMS ((src, if_IBM_ENC (srcdb COMMA) mods, m),
FILE *src _AND_
if_IBM_ENC (FILE *srcdb _AND_)
ModuleList *mods _AND_
Module *m)
{
void *tmp;
Module *currMod;
#ifdef _IBM_ENC_
size_t length;
char *inclstring;
#endif /* _IBM_ENC_ */
fprintf (src, "#include \"asn-incl.h\"\n");
/* dafe
by now source will include just itself header and common.h
tmp = (void *

情意绵绵的胸毛
- 粉丝: 2
- 资源: 2
最新资源
- 毕设和企业适用springboot企业知识管理平台类及机器学习平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及酒店管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及技术文档管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及企业IT解决方案平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及客户管理系统源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及企业数字化转型平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及全流程管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及企业项目管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及全球电商管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及全生命周期管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及视频监控系统源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及人工智能医疗平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及团队协作平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及线上广告平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及网络营销平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及职业技能培训平台源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


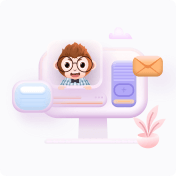