package cn.itcast.hotel.service.impl;
import cn.itcast.hotel.mapper.HotelMapper;
import cn.itcast.hotel.pojo.Hotel;
import cn.itcast.hotel.pojo.HotelDoc;
import cn.itcast.hotel.pojo.PageResult;
import cn.itcast.hotel.pojo.RequestParams;
import cn.itcast.hotel.service.IHotelService;
import com.alibaba.fastjson.JSON;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.elasticsearch.action.delete.DeleteRequest;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.geo.GeoPoint;
import org.elasticsearch.common.unit.DistanceUnit;
import org.elasticsearch.common.xcontent.XContentType;
import org.elasticsearch.index.query.BoolQueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.index.query.functionscore.FunctionScoreQueryBuilder;
import org.elasticsearch.index.query.functionscore.ScoreFunctionBuilders;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.SearchHits;
import org.elasticsearch.search.aggregations.AggregationBuilders;
import org.elasticsearch.search.aggregations.Aggregations;
import org.elasticsearch.search.aggregations.bucket.terms.Terms;
import org.elasticsearch.search.fetch.subphase.highlight.HighlightField;
import org.elasticsearch.search.sort.SortBuilders;
import org.elasticsearch.search.sort.SortOrder;
import org.elasticsearch.search.suggest.Suggest;
import org.elasticsearch.search.suggest.SuggestBuilder;
import org.elasticsearch.search.suggest.SuggestBuilders;
import org.elasticsearch.search.suggest.completion.CompletionSuggestion;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Slf4j
@Service
public class HotelService extends ServiceImpl<HotelMapper, Hotel> implements IHotelService {
@Autowired
private RestHighLevelClient restHighLevelClient;
@Override
public PageResult search(RequestParams params) {
try {
// 1.准备Request
SearchRequest request = new SearchRequest("hotel");
// 2.准备请求参数
// 2.1.query
buildBasicQuery(params, request);
// 2.2.分页
int page = params.getPage();
int size = params.getSize();
request.source().from((page - 1) * size).size(size);
// 2.3.距离排序
String location = params.getLocation();
if (StringUtils.isNotBlank(location)) {
request.source().sort(SortBuilders
.geoDistanceSort("location", new GeoPoint(location))
.order(SortOrder.ASC)
.unit(DistanceUnit.KILOMETERS)
);
}
// 3.发送请求
SearchResponse response = restHighLevelClient.search(request, RequestOptions.DEFAULT);
// 4.解析响应
return handleResponse(response);
} catch (IOException e) {
throw new RuntimeException("搜索数据失败", e);
}
}
@Override
public Map<String, List<String>> getFilters(RequestParams params) {
try {
// 1.准备请求
SearchRequest request = new SearchRequest("hotel");
// 2.请求参数
// 2.1.query
buildBasicQuery(params, request);
// 2.2.size
request.source().size(0);
// 2.3.聚合
buildAggregations(request);
// 3.发出请求
SearchResponse response = restHighLevelClient.search(request, RequestOptions.DEFAULT);
// 4.解析结果
Aggregations aggregations = response.getAggregations();
Map<String, List<String>> filters = new HashMap<>(3);
// 4.1.解析品牌
List<String> brandList = getAggregationByName(aggregations, "brandAgg");
filters.put("brand", brandList);
// 4.1.解析品牌
List<String> cityList = getAggregationByName(aggregations, "cityAgg");
filters.put("city", cityList);
// 4.1.解析品牌
List<String> starList = getAggregationByName(aggregations, "starAgg");
filters.put("starName", starList);
return filters;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public List<String> getSuggestion(String key) {
try {
// 1.准备请求
SearchRequest request = new SearchRequest("hotel");
// 2.请求参数
request.source().suggest(new SuggestBuilder()
.addSuggestion(
"hotelSuggest",
SuggestBuilders
.completionSuggestion("suggestion")
.size(10)
.skipDuplicates(true)
.prefix(key)
));
// 3.发出请求
SearchResponse response = restHighLevelClient.search(request, RequestOptions.DEFAULT);
// 4.解析
Suggest suggest = response.getSuggest();
// 4.1.根据名称获取结果
CompletionSuggestion suggestion = suggest.getSuggestion("hotelSuggest");
// 4.2.获取options
List<String> list = new ArrayList<>();
for (CompletionSuggestion.Entry.Option option : suggestion.getOptions()) {
// 4.3.获取补全的结果
String str = option.getText().toString();
// 4.4.放入集合
list.add(str);
}
return list;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void deleteById(Long hotelId) {
try {
// 1.创建request
DeleteRequest request = new DeleteRequest("hotel", hotelId.toString());
// 2.发送请求
restHighLevelClient.delete(request, RequestOptions.DEFAULT);
} catch (IOException e) {
throw new RuntimeException("删除酒店数据失败", e);
}
}
@Override
public void saveById(Long hotelId) {
try {
// 查询酒店数据,应该基于Feign远程调用hotel-admin,根据id查询酒店数据(现在直接去数据库查)
Hotel hotel = getById(hotelId);
// 转换
HotelDoc hotelDoc = new HotelDoc(hotel);
// 1.创建Request
IndexRequest request = new IndexRequest("hotel").id(hotelId.toString());
// 2.准备参数
request.source(JSON.toJSONString(hotelDoc), XContentType.JSON);
// 3.发送请求
restHighLevelClient.index(request, RequestOptions.DEFAULT);
} catch (IOException e) {
throw new RuntimeException("新增酒店数据失败", e);
}
}
private List<String> getAggregationByName(Aggregations aggregations, String aggName) {
// 4.1.根据聚合名称,获取聚合结果
Terms terms = aggregations.get(aggName);
// 4.2.获取buckets
List<? extends Terms.Bucket> buckets = terms.getBuckets();
// 4.3.遍历
List<String> list = new ArrayList<>(buckets.size());
for (Terms.B
没有合适的资源?快使用搜索试试~ 我知道了~
Docker基于ElasticSearch全文搜索引擎的旅游景点搜索网设计+sql数据库(毕设源码).zip
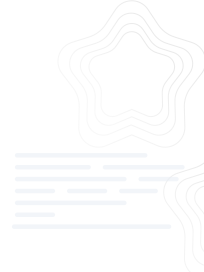
共303个文件
xml:219个
java:25个
class:25个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
【资源说明】 该项目是个人毕设项目源码,评审分达到95分,都经过严格调试,确保可以运行!放心下载使用。 该项目资源主要针对计算机、自动化等相关专业的学生或从业者下载使用,也可作为期末课程设计、课程大作业、毕业设计等。 具有较高的学习借鉴价值!基础能力强的可以在此基础上修改调整,以实现类似其他功能。 开发工具:Eclipse/Idea + 微信开发者工具 + mysql数据库 这里设计了一个微信小程序模式的失物招领平台,现在人人都有微信,用微信的就能使用咱们的小程序,及时发布和寻找失物招领信息,让同学们真正的找到属于自己的平台!学生用户在app端可以注册登录后发布自己的失物招领物品,也可以搜索自己感兴趣的失物招领信息,还可以给管理员留言,查询新闻公告!管理员可以在后台维护注册用户信息,管理所有失物招领信息,回复用户留言和发布新闻公告等等! 用户信息: 手机用户名,登陆密码,姓名,性别,出生日期,用户qq,家庭地址,用户邮箱,用户头像,附加信息,微信openid 物品分类: 类别编号,类别名称 物品信息: 物品编号,物品类别,物品名称,价格区域,物品价格,成色,区域,物品图片,联系人,联系电话,物品描述,发布人,发布时间 价格区间: 记录编号,价格区间 新旧程度: 记录编号,新旧程度 区域信息: 记录编号,区域名称 公告信息: 公告编号,公告标题,公告内容,发布日期 留言: 留言id,留言标题,留言内容,留言人,留言时间,管理回复,回复时间
资源推荐
资源详情
资源评论
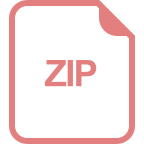
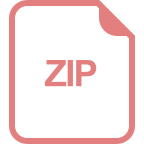
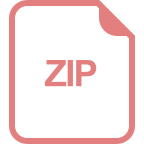
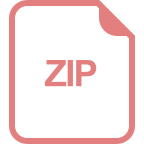
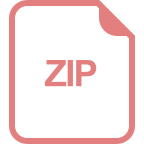
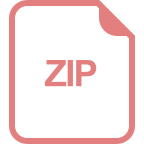
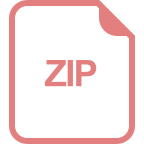
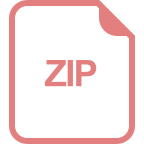
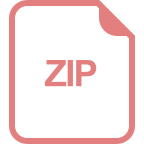
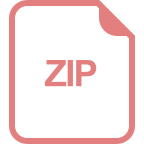
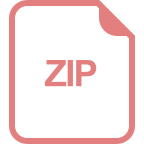
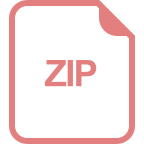
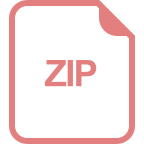
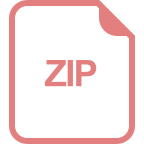
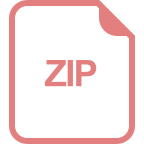
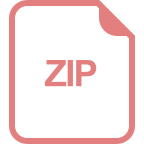
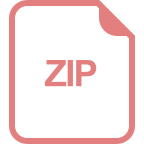
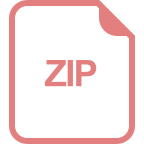
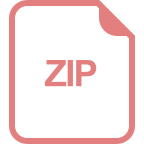
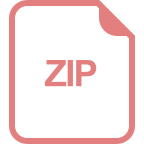
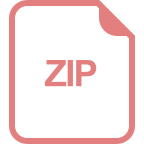
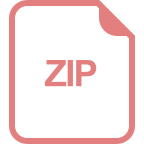
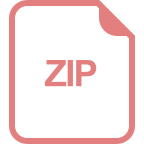
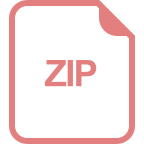
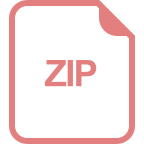
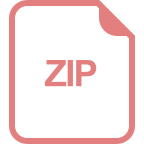
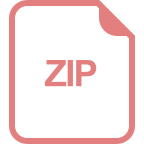
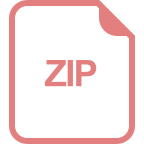
收起资源包目录

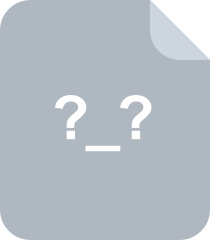
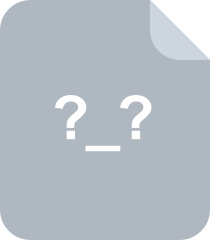
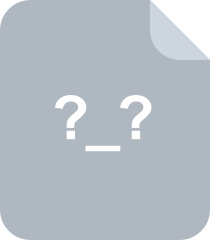
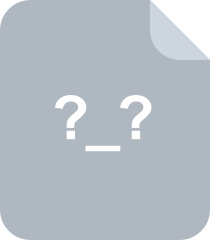
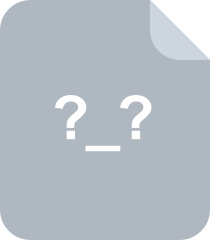
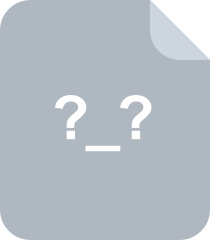
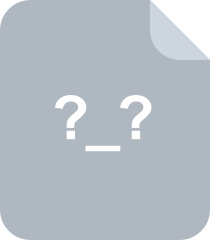
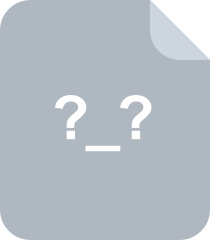
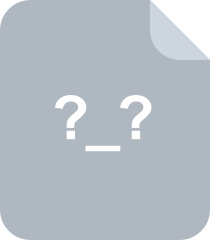
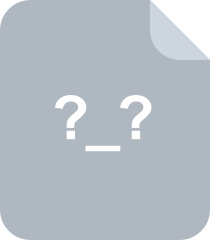
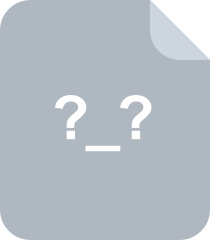
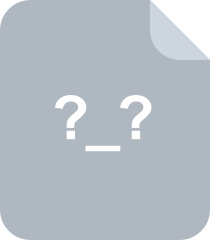
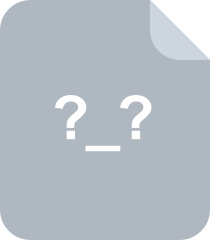
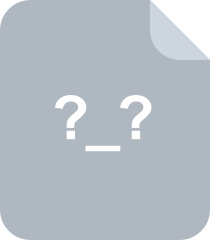
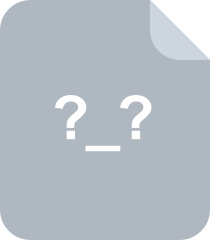
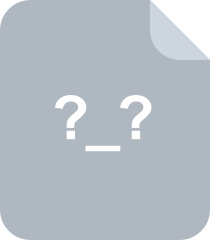
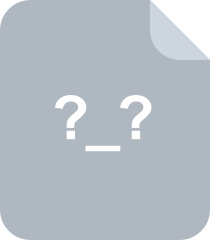
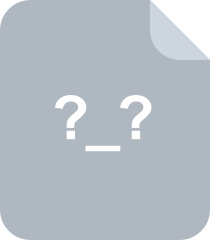
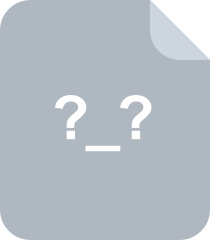
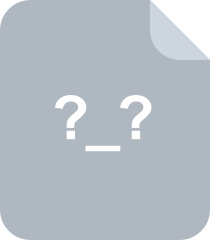
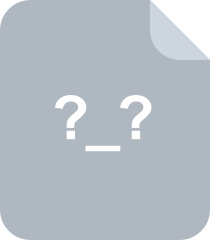
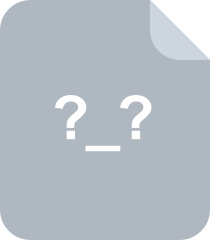
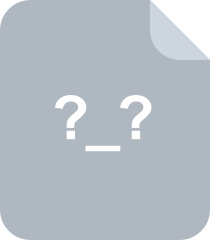
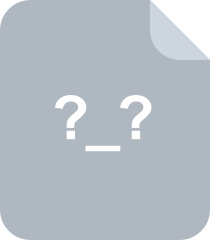
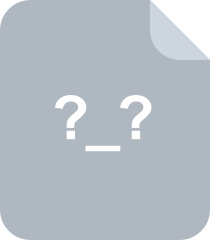
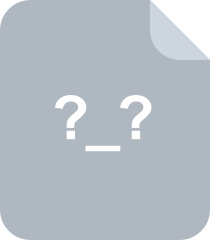
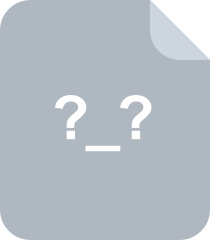
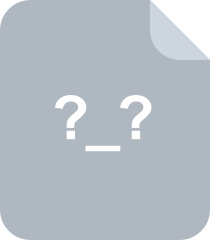
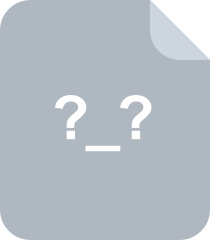
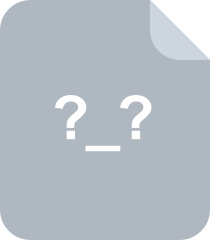
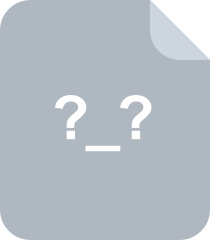
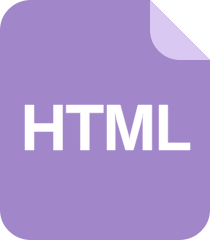
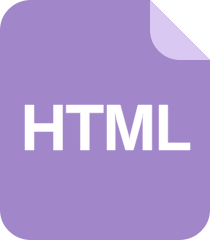
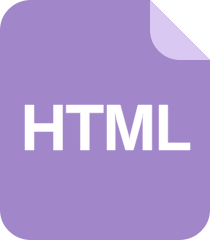
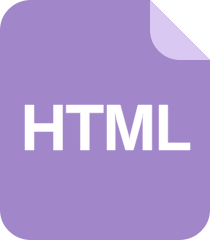
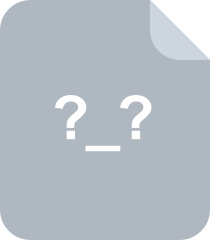
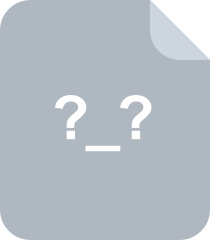
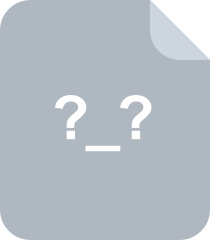
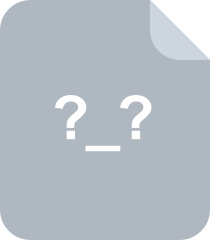
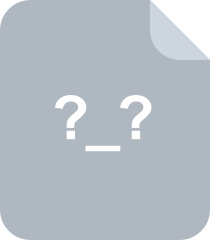
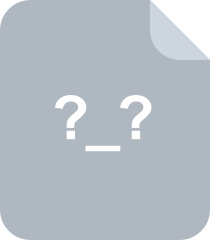
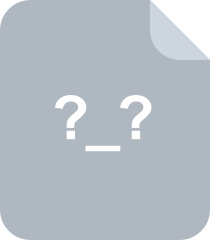
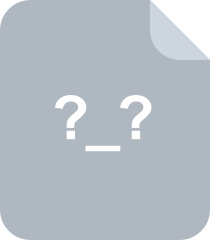
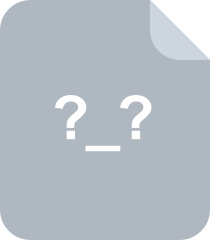
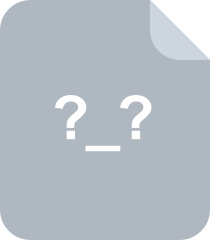
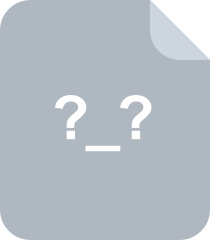
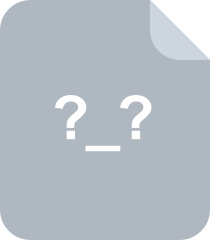
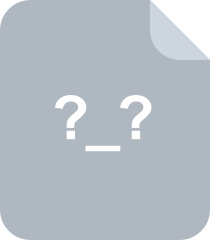
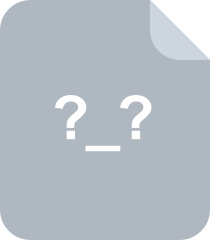
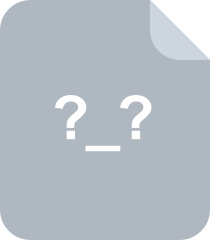
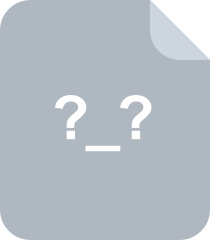
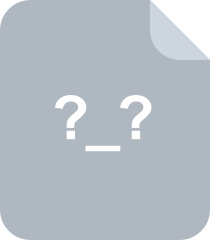
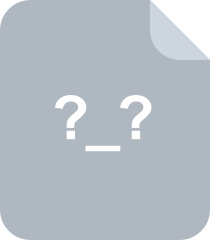
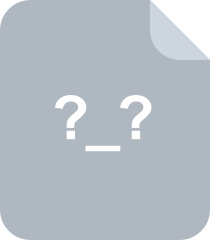
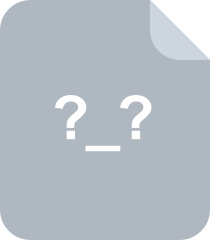
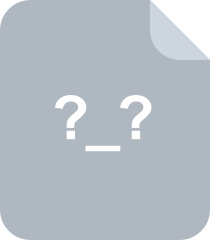
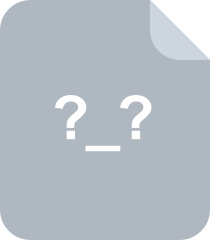
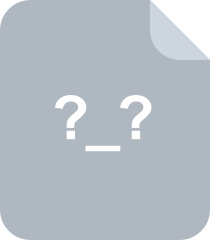
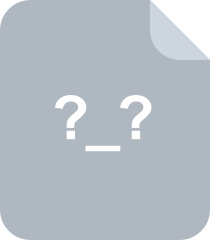
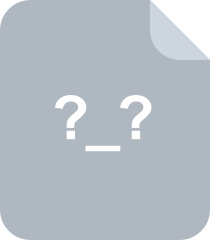
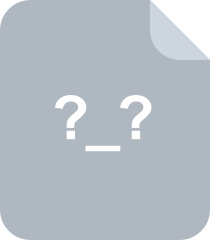
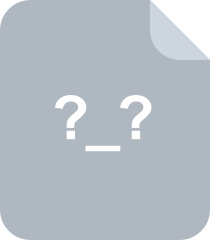
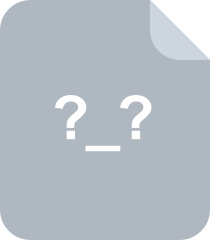
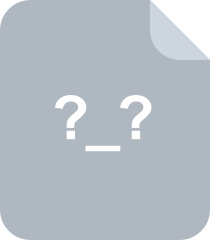
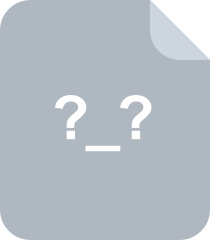
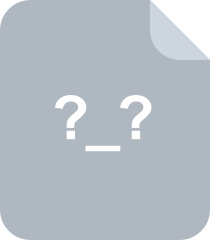
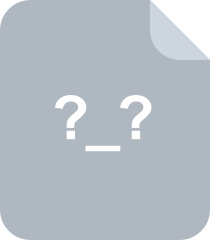
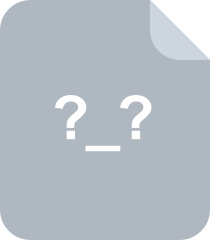
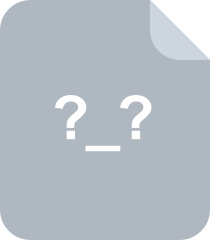
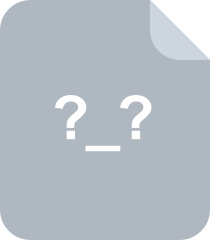
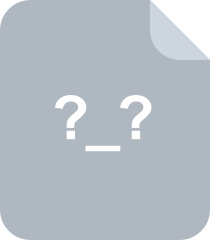
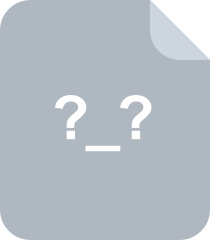
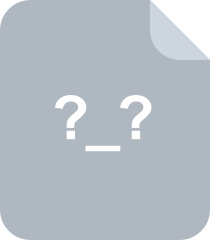
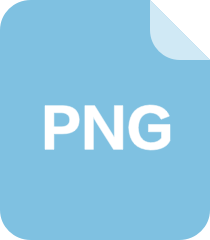
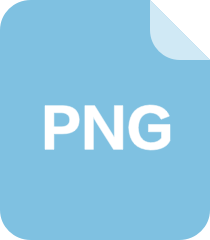
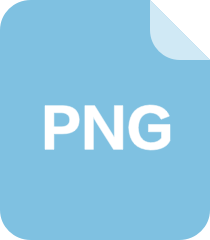
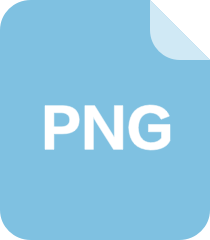
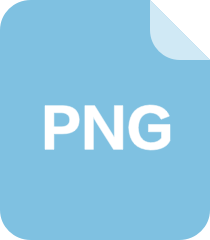
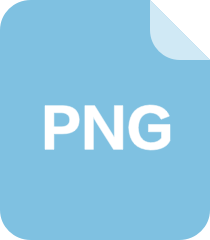
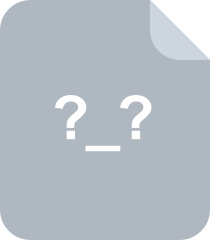
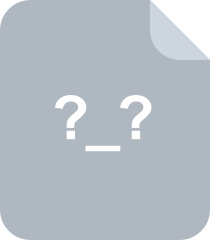
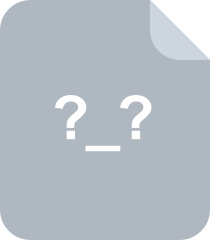
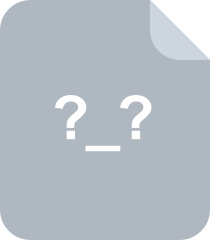
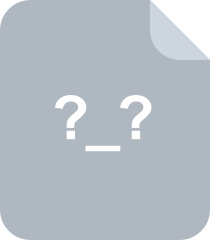
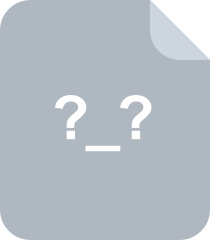
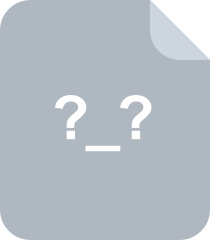
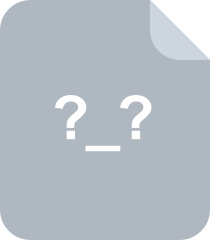
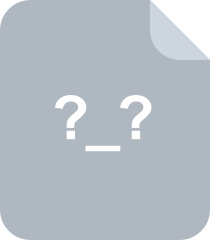
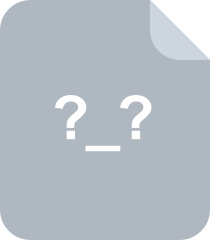
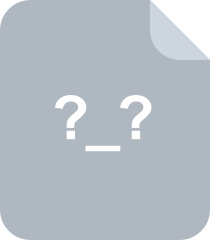
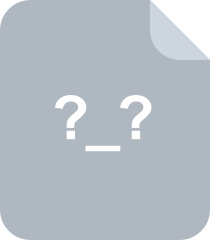
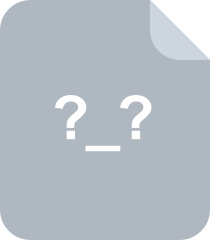
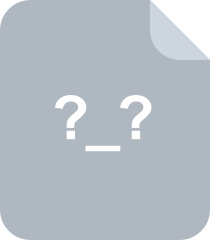
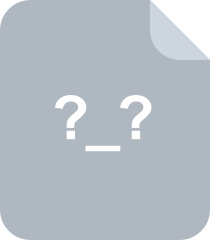
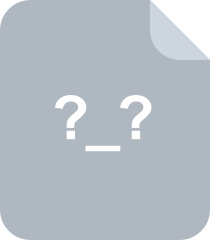
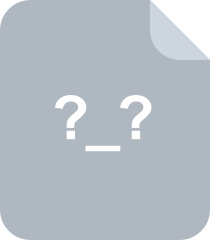
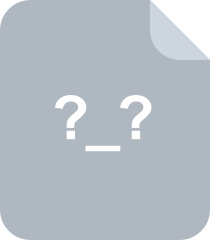
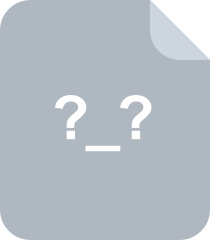
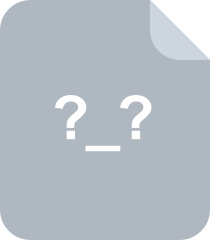
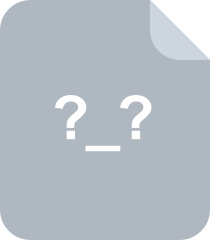
共 303 条
- 1
- 2
- 3
- 4
资源评论
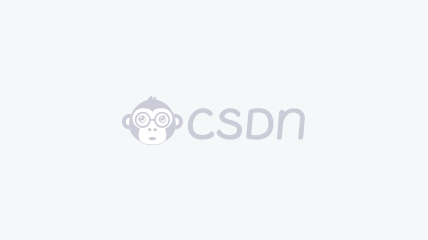
- 11年第一顺位2024-04-28怎么能有这么好的资源!只能用感激涕零来形容TAT...

manylinux
- 粉丝: 4417
- 资源: 2491
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

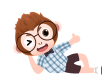
安全验证
文档复制为VIP权益,开通VIP直接复制
