#include "gamescene.h"
GameScene::GameScene(QWidget *parent) : QWidget(parent) {
setWindowTitle("超级玛丽");//设置标题
setFixedSize(800, 545);//设置窗口大小
Game_Init();
Pause_Init();
QTimer::singleShot(1500, this, [=]() {
timer1 = startTimer(15);
timer3 = startTimer(40);
game_start = true;
});
}
void GameScene::Pause_Init() {
Pause = new GamePause();//初始化暂停窗口
MyPushButton *btn_continue = new MyPushButton(":/photo/continueGame.png");//添加继续按钮
btn_continue->setParent(Pause);
btn_continue->setFixedSize(150, 75);
btn_continue->setIconSize(QSize(150, 75));
btn_continue->move(20, 10);
connect(btn_continue, &QPushButton::clicked, this, [=]() {
btn_continue->zoom1();//加载动画
btn_continue->zoom2();
QTimer::singleShot(500, this, [=]() {
timer1 = startTimer(15);
timer3 = startTimer(40);
mary->walk_state = 0;//初始化mary的行走状态
key = "null";
Pause->close();
});
});
MyPushButton *initGame = new MyPushButton(":/photo/initGame.png");//添加初始化窗口
initGame->setParent(Pause);
initGame->setFixedSize(150, 75);
initGame->setIconSize(QSize(150, 75));
initGame->move(20, 90);
connect(initGame, &QPushButton::clicked, this, [=]() {
initGame->zoom1();
initGame->zoom2();
QTimer::singleShot(500, this, [=]() {
Pause_Game_Init();//游戏初始化
Pause->close();
QTimer::singleShot(1500, this, [=]() {
timer1 = startTimer(15);//开启定时器
timer3 = startTimer(40);
game_start = true;
});
});
});
MyPushButton *btn_exit = new MyPushButton(":/photo/start.png");//添加离开按钮
btn_exit->setParent(Pause);
btn_exit->setFixedSize(150, 75);
btn_exit->setIconSize(QSize(150, 75));
btn_exit->move(20, 170);
connect(btn_exit, &QPushButton::clicked, this, [=]() {
btn_exit->zoom1();
btn_exit->zoom2();
QTimer::singleShot(500, this, [=]() {
this->close();
});
});
}
void GameScene::timerEvent(QTimerEvent *event) {
if (event->timerId() == timer1 && mary->is_die) {
mary->Mary_die();
Die_Init();
update();
return;
}
if (event->timerId() == timer1) {
mary->Mary_Move(key);
mary->Jump_And_Down();
Jump_Collision();
Move_Collision();
brick->ShatterState();
mushroom->Move_state();
master->Master_Move();
Die_Init();
Fall_Down(mary->y);
fire->Fire_state();
update();
}
if (event->timerId() == timer2) {
mary->Mary_Move(key);
}
if (event->timerId() == timer3) {
time -= 0.04;
unknown->Unknown_State();
unknown->Crash_state();
}
}
void GameScene::keyPressEvent(QKeyEvent *event) {
if (!mary->is_die) {
switch (event->key()) {
case Qt::Key_Right:
mary->direction = key = "right";
break;
case Qt::Key_Left:
mary->direction = key = "left";
break;
case Qt::Key_Z:
timer2 = startTimer(25);
is_kill_timer2 = false;
break;
case Qt::Key_Space:
mary->is_jump = true;
break;
case Qt::Key_B:
if (game_start) {
killTimer(timer1);
if (is_kill_timer2) {
killTimer(timer2);
}
killTimer(timer3);
Pause->setParent(this);
Pause->open();
}
break;
case Qt::Key_C:
if (mary->life < 8) {
mary->life++;
}
case Qt::Key_X:
if (!is_press_x && !mary->is_jump && mary->is_jump_end && mary->colour == 3) {
is_press_x = true;
fire->Fire_xy();
}
break;
}
}
}
void GameScene::keyReleaseEvent(QKeyEvent *event) {
if (!mary->is_die) {
switch (event->key()) {
case Qt::Key_Right:
mary->walk_state = 0;
key = "null";
break;
case Qt::Key_Left:
mary->walk_state = 0;
key = "null";
break;
case Qt::Key_Z:
is_kill_timer2 = true;
killTimer(timer2);
break;
case Qt::Key_Space:
mary->is_jump = false;
mary->is_space_release = true;
break;
case Qt::Key_X:
is_press_x = false;
break;
}
}
}
void GameScene::paintEvent(QPaintEvent *) {
QPainter painter(this);
if (!game_start) {
painter.drawPixmap(0, 0, 800, 550, QPixmap(":/photo/blackground2.png"));
painter.drawPixmap(300, 250, 40, 40, QPixmap(":/photo/life.png"));
painter.setPen(QColor(255, 255, 255));
QFont font;
font.setPointSize(35);
painter.setFont(font);
painter.drawText(360, 280, "x");
painter.drawText(110, 30, "times:");
painter.drawText(220, 32, QString::number(time, 'f', 1));
painter.drawText(600, 30, "coin:");
painter.drawText(680, 32, QString::number(unknown->coin));
font.setPointSize(45);
painter.setFont(font);
painter.drawText(400, 287, QString::number(mary->life));
return;
}
painter.drawPixmap(0, 0, 800, 550, QPixmap(":/photo/sky.png"));//画背景
painter.drawPixmap(230, 10, QPixmap(":/photo/coin.png"), 0, 0, 30, 30);
painter.drawPixmap(380, 10, 30, 30, QPixmap(":/photo/score.png"));
painter.setFont(QFont("Times", 45, QFont::Bold));
painter.drawText(280, 38, QString::number(unknown->coin));
painter.drawText(430, 38, QString::number(score));
for (int i = 1; i <= mary->life; i++) {
painter.drawPixmap(800 - i * 35, 10, 30, 30, QPixmap(":/photo/life.png"));
}
painter.drawPixmap(10, 10, 30, 30, QPixmap(":/photo/time.png"));
painter.drawText(50, 38, QString::number(time, 'f', 1));
painter.drawPixmap(0, 500, QPixmap(":/photo/ground.png"), mary->ground_state, 0, 800, 45);//画地板
if (mary->x > 7800) {
QVector < QVector < int >> ::iterator
it = castle->m.begin()->begin();
painter.drawPixmap(*it->begin() - mary->x, *(it->begin() + 1), 200, 200, QPixmap(":/photo/castle.png"));
}
if (mushroom->mushroom_state != 0) {
painter.drawPixmap(mushroom->mushroom_x - mary->x, mushroom->mushroom_y, 40, 40,
QPixmap(":/photo/mushroom" + QString::number(mary->colour) + ".png"));
}
for (QVector < QVector < int >> ::iterator it = brick->m.begin()->begin(); it != brick->m.begin()->end();
it++)
{
if (*(it->begin()) - mary->x > -50 && *(it->begin()) - mary->x < 800 && *(it->begin() + 2) == 1) {
painter.drawPixmap(*(it->begin()) - mary->x, *(it->begin() + 1), 50, 40, QPixmap(":/photo/brick1.png"));
}
}
for (QVector < QVector < int >> ::iterator it = unknown->m.begin()->begin(); it != unknown->m.begin()->end();
it++)
{
if (*(it->begin()) - mary->x > -50 && *(it->begin()) - mary->x < 800 && *(it->begin() + 2) != 0) {
painter.drawPixmap(*(it->begin()) - mary->x, *(it->begin() + 1), QPixmap(":/photo/unknown.png"),
unknown->unknown_state, 0, 50, 40);
} else if (*(it->begin()) - mary->x > -50 && *(it->begin()) - mary->x < 800 && *(it->begin() + 2) == 0) {
painter.drawPixmap(*(it->begin()) - mary->x, *(it->begin() + 1), 50, 40,
没有合适的资源?快使用搜索试试~ 我知道了~
大学课程设计作业-基于QT和C++开发实现的超级玛丽游戏(简易版)源码+项目说明.zip
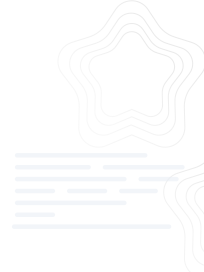
共78个文件
png:43个
cpp:14个
h:13个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 141 浏览量
2023-06-02
14:01:19
上传
评论 3
收藏 8.42MB ZIP 举报
温馨提示
大学课程设计作业-基于QT和C++开发实现的超级玛丽游戏(简易版)源码+项目说明.zip 大学课程设计作业-基于QT和C++开发实现的超级玛丽游戏(简易版)源码+项目说明.zip 大学课程设计作业-基于QT和C++开发实现的超级玛丽游戏(简易版)源码+项目说明.zip 一个简单的超级玛丽游戏,很早之前学习Qt写的,逻辑现在不是很清楚了,需要优化的地方有很多,代码写的也不太严谨,实现了第一关, 后面的关卡需要在Vector中存储地图信息。 【开发环境】 基于QT ,使用C++编写 开发环境为:MacOs + QtCreator,请使用QtCreator打开super_mary.pro文件 注意!:使用低分辨率屏幕或windows打开游戏画面可能出现一些小问题,类似下面的图片,
资源推荐
资源详情
资源评论
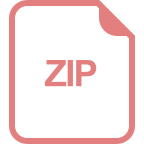
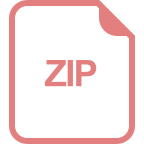
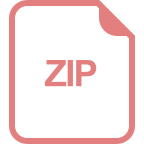
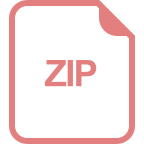
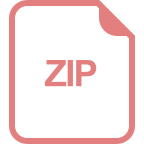
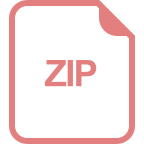
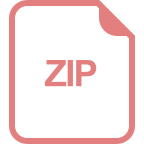
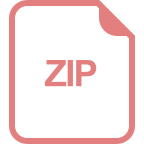
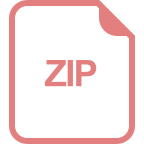
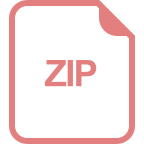
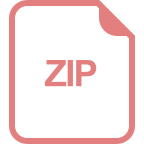
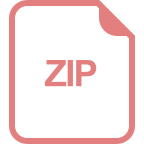
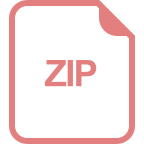
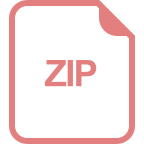
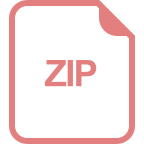
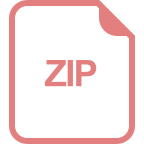
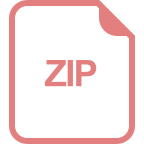
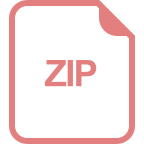
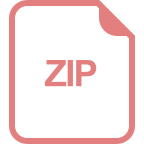
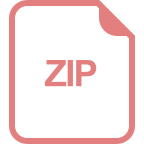
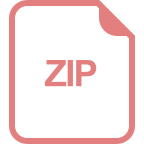
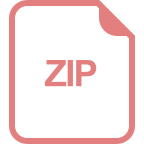
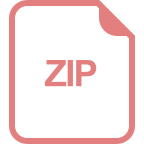
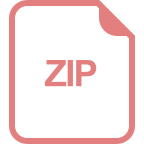
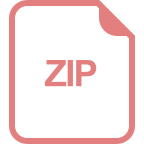
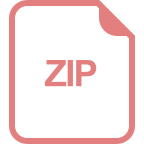
收起资源包目录

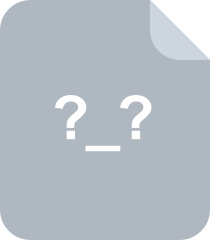
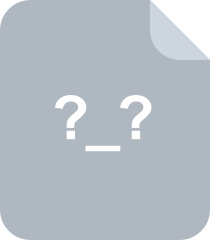
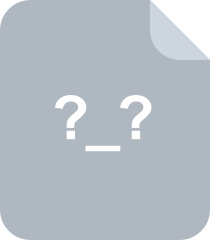
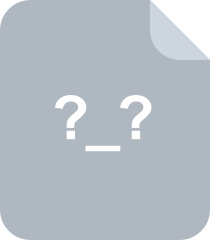
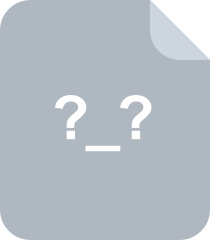
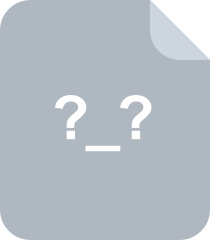
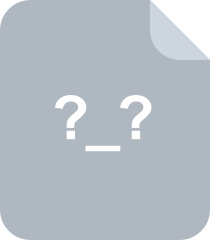
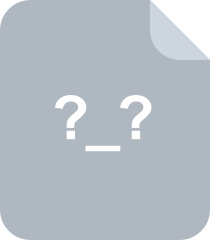
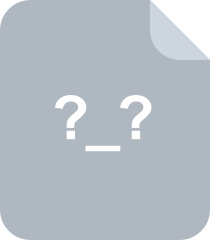
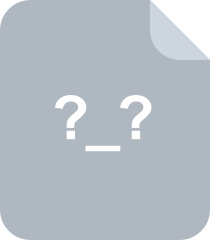
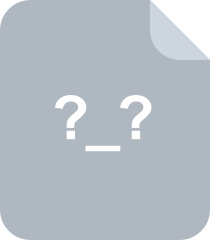
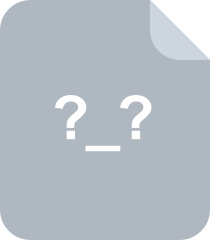
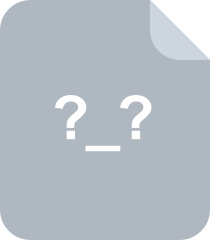
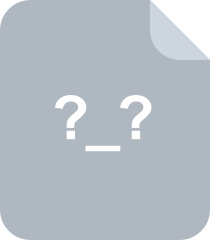
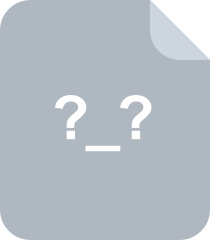
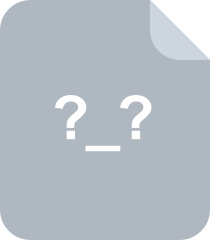
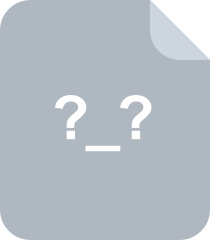
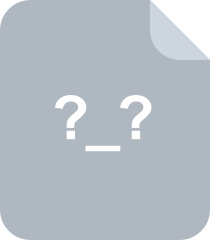
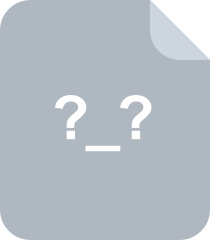
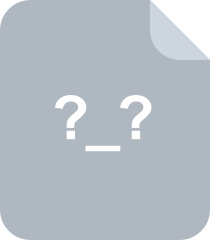
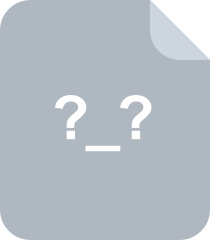
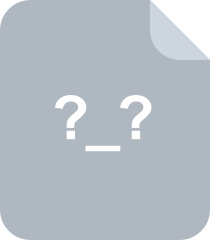
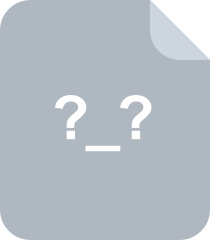
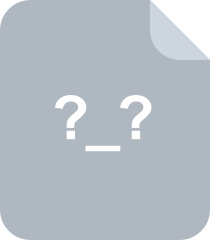
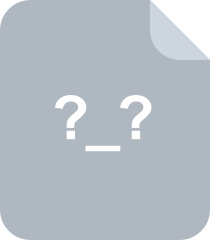
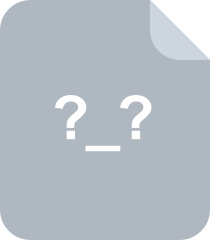
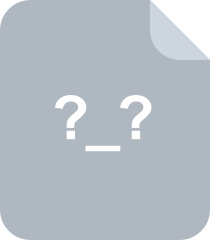
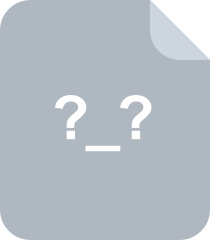
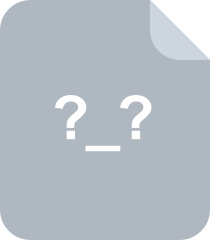
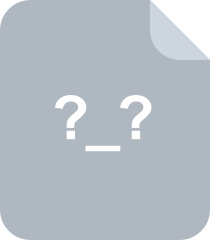

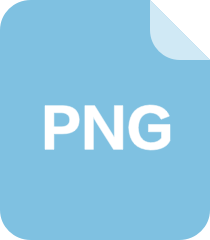
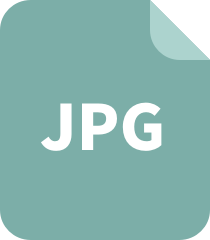
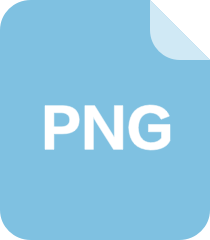
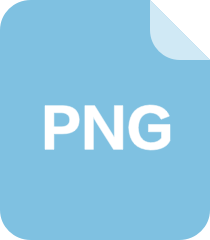
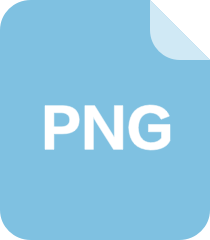
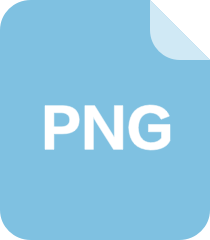
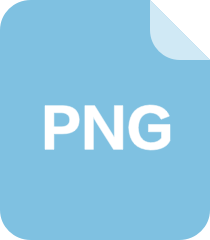
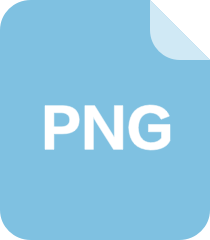
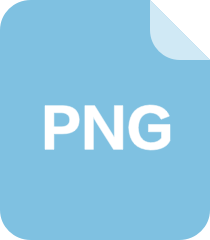
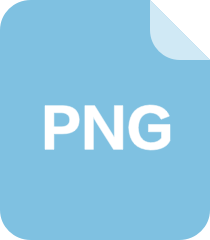
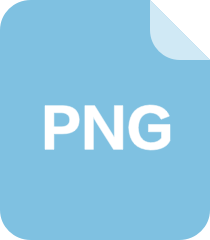
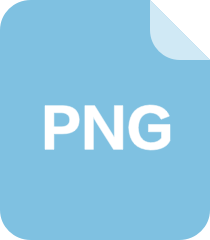
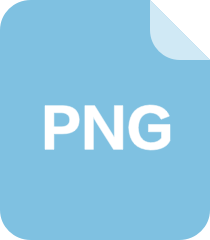
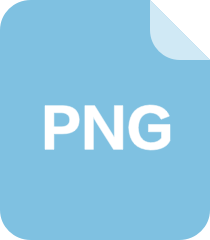
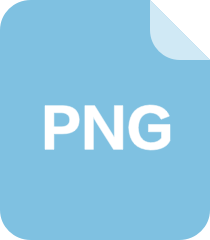
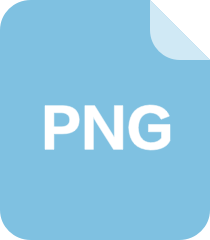
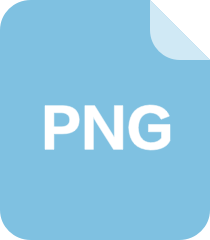
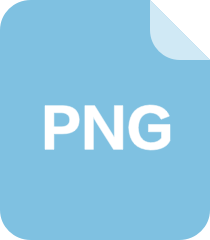
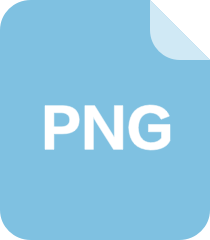
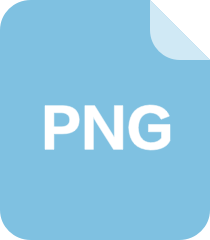
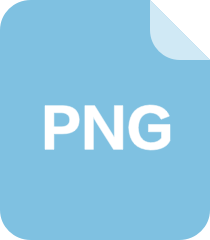
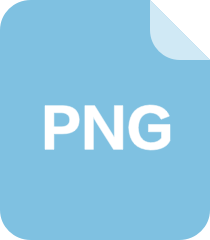
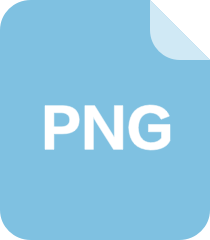
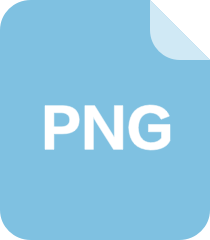
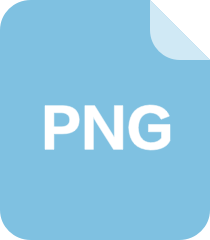
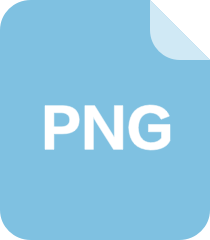
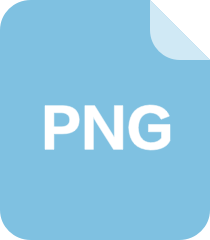
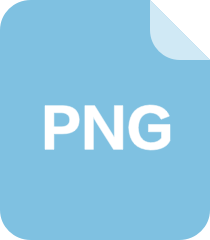
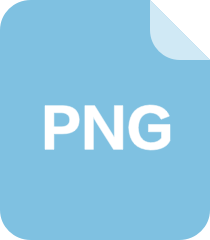
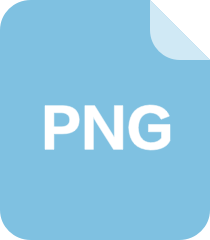
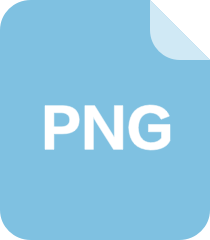
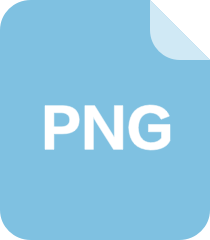
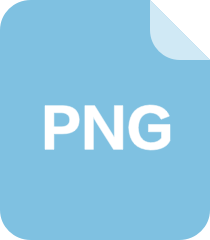
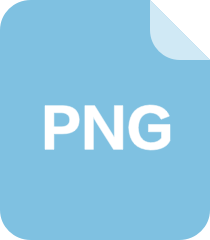
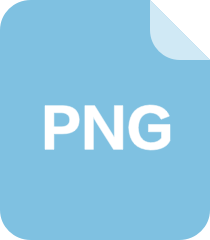
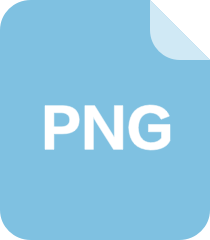
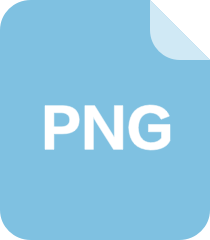
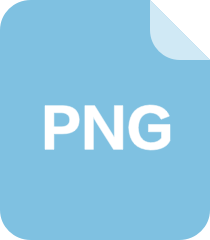
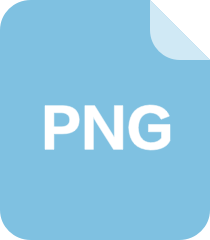
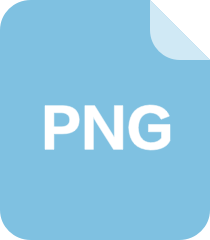
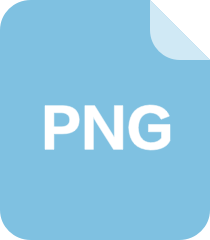
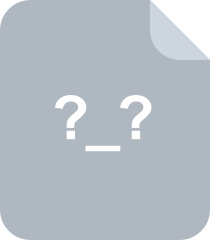
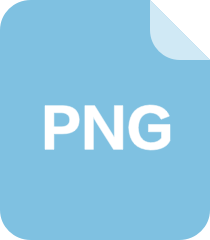
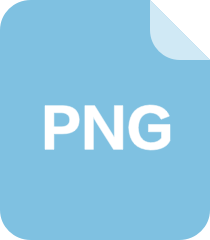
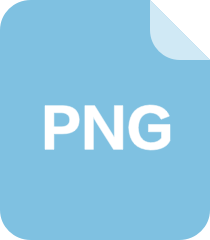
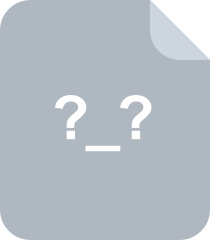
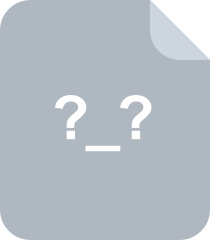
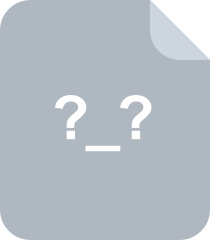
共 78 条
- 1
资源评论
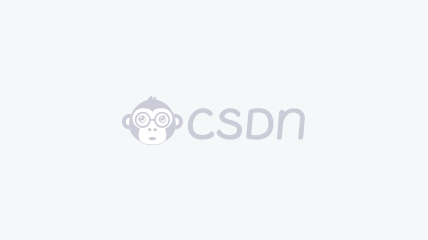

manylinux
- 粉丝: 4535
- 资源: 2485

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

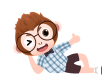
最新资源
- 基于动态窗口算法的AGV仿真避障 可设置起点目标点,设置地图,设置移动障碍物起始点目标点,未知静态障碍物 动态窗口方法(DynamicWindowApproach) 是一种可以实现实时避障的局部规划算
- Power Quality Disturbance:基于MATLAB Simulink的各种电能质量扰动仿真模型,包括配电线路故障、感应电机启动、变压器励磁、单相 三相非线性负载等模型,可用于模拟各种
- 数据爬虫项目全套技术资料100%好用.zip
- 聊天系统项目全套技术资料100%好用.zip
- putty,linux客户端工具
- 丹佛丝堆垛机变频器参数配置起升、运行、货叉
- redhat-lsb-core,安装磐维数据库,安装oracle数据库等常用的依赖包
- lsb-release,安装磐维数据库,安装oracle数据库等常用的依赖包
- glibc-devel,安装磐维数据库,安装oracle数据库等常用的依赖包
- redhat-lsb-submit-security,安装磐维数据库,安装oracle数据库等常用的依赖包
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


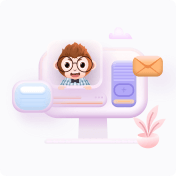
安全验证
文档复制为VIP权益,开通VIP直接复制
