#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#include <signal.h>
#include "hi_common.h"
#include "hi_comm_video.h"
#include "hi_comm_sys.h"
#include "hi_comm_svp.h"
#include "mpi_sys.h"
#include "mpi_vb.h"
//#include "mpi_dsp.h"
#include "sample_comm_svp.h"
static HI_BOOL s_bSampleSvpInit = HI_FALSE;
#if 0
/*
*System init
*/
static HI_S32 SAMPLE_COMM_SVP_SysInit(HI_VOID)
{
HI_S32 s32Ret = HI_FAILURE;
VB_CONFIG_S struVbConf;
HI_MPI_SYS_Exit();
HI_MPI_VB_Exit();
memset(&struVbConf,0,sizeof(VB_CONFIG_S));
struVbConf.u32MaxPoolCnt = 2;
struVbConf.astCommPool[1].u64BlkSize = 768*576*2;
struVbConf.astCommPool[1].u32BlkCnt = 1;
s32Ret = HI_MPI_VB_SetConfig((const VB_CONFIG_S *)&struVbConf);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_VB_SetConf failed!\n", s32Ret);
s32Ret = HI_MPI_VB_Init();
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_VB_Init failed!\n", s32Ret);
s32Ret = HI_MPI_SYS_Init();
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SYS_Init failed!\n", s32Ret);
return s32Ret;
}
/*
*System exit
*/
static HI_S32 SAMPLE_COMM_SVP_SysExit(HI_VOID)
{
HI_S32 s32Ret = HI_FAILURE;
s32Ret = HI_MPI_SYS_Exit();
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SYS_Exit failed!\n", s32Ret);
s32Ret = HI_MPI_VB_Exit();
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_VB_Exit failed!\n", s32Ret);
return HI_SUCCESS;
}
/*
*System init
*/
HI_VOID SAMPLE_COMM_SVP_CheckSysInit(HI_VOID)
{
if(HI_FALSE == s_bSampleSvpInit)
{
if (SAMPLE_COMM_SVP_SysInit())
{
SAMPLE_SVP_TRACE(SAMPLE_SVP_ERR_LEVEL_ERROR,"Svp mpi init failed!\n");
exit(-1);
}
s_bSampleSvpInit = HI_TRUE;
}
SAMPLE_SVP_TRACE(SAMPLE_SVP_ERR_LEVEL_DEBUG,"Svp mpi init ok!\n");
}
/*
*System exit
*/
HI_VOID SAMPLE_COMM_SVP_CheckSysExit(HI_VOID)
{
if ( s_bSampleSvpInit)
{
SAMPLE_COMM_SVP_SysExit();
s_bSampleSvpInit = HI_FALSE;
}
SAMPLE_SVP_TRACE(SAMPLE_SVP_ERR_LEVEL_DEBUG,"Svp mpi exit ok!\n");
}
#endif
/*
*Load bin
*/
/*
HI_S32 SAMPLE_COMM_SVP_LoadCoreBinary(SVP_DSP_ID_E enCoreId)
{
HI_S32 s32Ret = HI_FAILURE;
HI_CHAR * aszBin[4][4] = {
{"./dsp_bin/dsp0/hi_sram.bin","./dsp_bin/dsp0/hi_iram0.bin","./dsp_bin/dsp0/hi_dram0.bin","./dsp_bin/dsp0/hi_dram1.bin"},
{"./dsp_bin/dsp1/hi_sram.bin","./dsp_bin/dsp1/hi_iram0.bin","./dsp_bin/dsp1/hi_dram0.bin","./dsp_bin/dsp1/hi_dram1.bin"},
{"./dsp_bin/dsp2/hi_sram.bin","./dsp_bin/dsp2/hi_iram0.bin","./dsp_bin/dsp2/hi_dram0.bin","./dsp_bin/dsp2/hi_dram1.bin"},
{"./dsp_bin/dsp3/hi_sram.bin","./dsp_bin/dsp3/hi_iram0.bin","./dsp_bin/dsp3/hi_dram0.bin","./dsp_bin/dsp3/hi_dram1.bin"}};
s32Ret = HI_MPI_SVP_DSP_PowerOn(enCoreId);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_PowerUp failed!\n", s32Ret);
s32Ret = HI_MPI_SVP_DSP_LoadBin(aszBin[enCoreId][1], enCoreId * 4 + 1);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_LoadBin failed!\n", s32Ret);
s32Ret = HI_MPI_SVP_DSP_LoadBin(aszBin[enCoreId][0], enCoreId * 4 + 0);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_LoadBin failed!\n", s32Ret);
s32Ret = HI_MPI_SVP_DSP_LoadBin(aszBin[enCoreId][2], enCoreId * 4 + 2);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_LoadBin failed!\n", s32Ret);
s32Ret = HI_MPI_SVP_DSP_LoadBin(aszBin[enCoreId][3], enCoreId * 4 + 3);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_LoadBin failed!\n", s32Ret);
s32Ret = HI_MPI_SVP_DSP_EnableCore(enCoreId);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_EnableCore failed!\n", s32Ret);
return s32Ret;
}
*/
/*
*UnLoad bin
*/
/*
void SAMPLE_COMM_SVP_UnLoadCoreBinary(SVP_DSP_ID_E enCoreId)
{
HI_S32 s32Ret = HI_FAILURE;
s32Ret = HI_MPI_SVP_DSP_DisableCore(enCoreId);
SAMPLE_SVP_CHECK_EXPR_RET_VOID(HI_SUCCESS != s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_DisableCore failed!\n", s32Ret);
s32Ret = HI_MPI_SVP_DSP_PowerOff(enCoreId);
SAMPLE_SVP_CHECK_EXPR_RET_VOID(HI_SUCCESS != s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SVP_DSP_PowerDown failed!\n", s32Ret);
}
*/
/*
*Align
*/
HI_U32 SAMPLE_COMM_SVP_Align(HI_U32 u32Size, HI_U16 u16Align)
{
HI_U32 u32Stride = u32Size + (u16Align - u32Size%u16Align)%u16Align;
return u32Stride;
}
/*
*Create Image memory
*/
HI_S32 SAMPLE_COMM_SVP_CreateImage(SVP_IMAGE_S *pstImg,SVP_IMAGE_TYPE_E enType,HI_U32 u32Width,
HI_U32 u32Height,HI_U32 u32AddrOffset)
{
HI_U32 u32Size = 0;
HI_S32 s32Ret;
pstImg->enType = enType;
pstImg->u32Width = u32Width;
pstImg->u32Height = u32Height;
pstImg->au32Stride[0] = SAMPLE_COMM_SVP_Align(pstImg->u32Width,SAMPLE_SVP_ALIGN_16);
switch(enType)
{
case SVP_IMAGE_TYPE_U8C1:
case SVP_IMAGE_TYPE_S8C1:
{
u32Size = pstImg->au32Stride[0] * pstImg->u32Height + u32AddrOffset;
s32Ret = HI_MPI_SYS_MmzAlloc(&pstImg->au64PhyAddr[0], (void**)&pstImg->au64VirAddr[0], NULL, HI_NULL, u32Size);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SYS_MmzAlloc failed!\n", s32Ret);
pstImg->au64PhyAddr[0] += u32AddrOffset;
pstImg->au64VirAddr[0] += u32AddrOffset;
}
break;
case SVP_IMAGE_TYPE_YUV420SP:
{
u32Size = pstImg->au32Stride[0] * pstImg->u32Height * 3 / 2 + u32AddrOffset;
s32Ret = HI_MPI_SYS_MmzAlloc(&pstImg->au64PhyAddr[0], (void**)&pstImg->au64VirAddr[0], NULL, HI_NULL, u32Size);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SYS_MmzAlloc failed!\n", s32Ret);
pstImg->au64PhyAddr[0] += u32AddrOffset;
pstImg->au64VirAddr[0] += u32AddrOffset;
pstImg->au32Stride[1] = pstImg->au32Stride[0];
pstImg->au64PhyAddr[1] = pstImg->au64PhyAddr[0] + pstImg->au32Stride[0] * pstImg->u32Height;
pstImg->au64VirAddr[1] = pstImg->au64VirAddr[0] + pstImg->au32Stride[0] * pstImg->u32Height;
}
break;
case SVP_IMAGE_TYPE_YUV422SP:
{
u32Size = pstImg->au32Stride[0] * pstImg->u32Height * 2 + u32AddrOffset;
s32Ret = HI_MPI_SYS_MmzAlloc(&pstImg->au64PhyAddr[0], (void**)&pstImg->au64VirAddr[0], NULL, HI_NULL, u32Size);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SYS_MmzAlloc failed!\n", s32Ret);
pstImg->au64PhyAddr[0] += u32AddrOffset;
pstImg->au64VirAddr[0] += u32AddrOffset;
pstImg->au32Stride[1] = pstImg->au32Stride[0];
pstImg->au64PhyAddr[1] = pstImg->au64PhyAddr[0] + pstImg->au32Stride[0] * pstImg->u32Height;
pstImg->au64VirAddr[1] = pstImg->au64VirAddr[0] + pstImg->au32Stride[0] * pstImg->u32Height;
}
break;
case SVP_IMAGE_TYPE_YUV420P:
{
pstImg->au32Stride[1] = SAMPLE_COMM_SVP_Align(pstImg->u32Width/2,SAMPLE_SVP_ALIGN_16);
pstImg->au32Stride[2] = pstImg->au32Stride[1];
u32Size = pstImg->au32Stride[0] * pstImg->u32Height + pstImg->au32Stride[1] * pstImg->u32Height + u32AddrOffset;
s32Ret = HI_MPI_SYS_MmzAlloc(&pstImg->au64PhyAddr[0], (void**)&pstImg->au64VirAddr[0], NULL, HI_NULL, u32Size);
SAMPLE_SVP_CHECK_EXPR_RET(HI_SUCCESS != s32Ret, s32Ret, SAMPLE_SVP_ERR_LEVEL_ERROR, "Error(%#x):HI_MPI_SYS_MmzAlloc failed!\n", s32Ret);
pstImg->au64PhyAd
没有合适的资源?快使用搜索试试~ 我知道了~
YOLOv3移植部署海思3516DV300板子c++源码(含wk模型+CV库+项目使用说明).zip
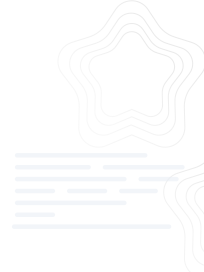
共440个文件
hpp:219个
h:157个
a:30个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
YOLOv3移植部署海思3516DV300板子c++源码(含wk模型+CV库+项目使用说明).zip 海思Hi3516DV300移植YOLOv3 在海思Hisilicon的Hi3516dv300芯片上,利用nnie和opencv库,简洁了官方yolov3用例中各种复杂的嵌套调用/复杂编译,提供了交叉编译后可成功上板部署运行的demo。 同时,对在海思Hisilicon的其他AI芯片上利用nnie部署CNN模型提供参考和借鉴。 以下为demo工程说明: 1.libopencv 交叉编译时所依赖的opencv库,已经打包好了; 亦可根据自己项目需要选择不同版本/不同阉割程度的opencv依赖库,需自行编译。 2.libhisi 交叉编译时所依赖的nnie库,海思官方提供,未作处理全部堆上,lib静态库,亦可换为动态库。 3.image demo输入原始图像dog_bike_car.jpg;dog_bike_car_416x416.jpg; 官方输入原始图像dog_bike_car_416x416.bgr; 项目使用原始图像dog_bike_car_416x416.yuv。
资源推荐
资源详情
资源评论
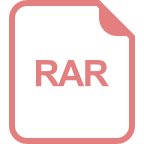
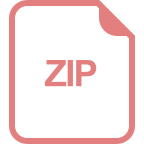
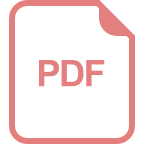
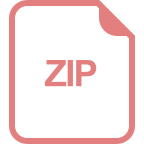
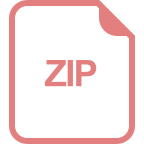
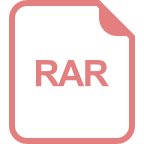
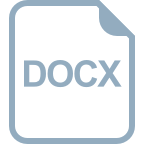
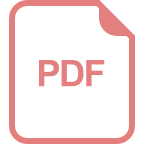
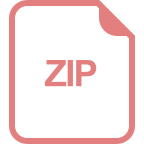
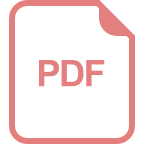
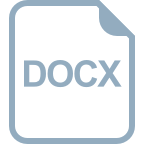
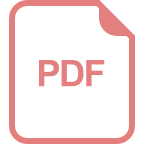
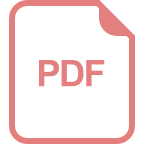
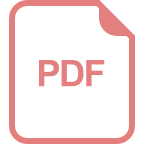
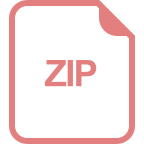
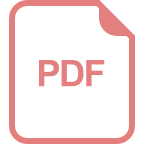
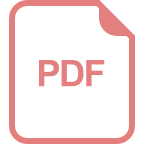
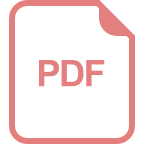
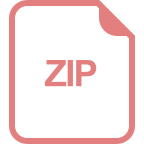
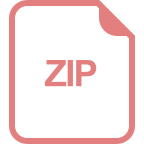
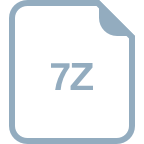
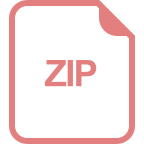
收起资源包目录

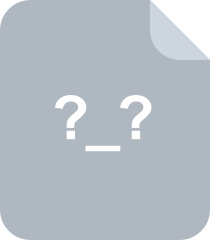
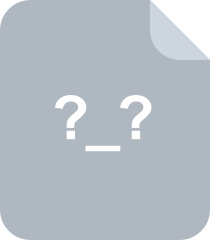
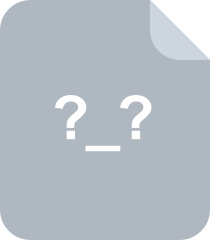
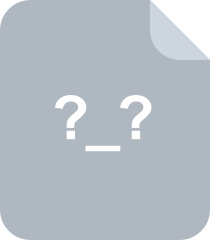
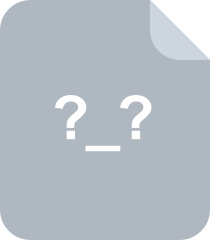
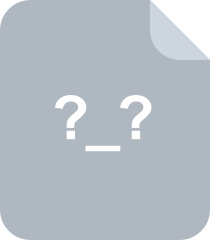
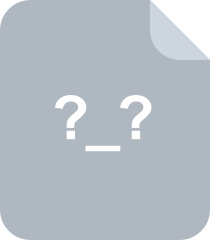
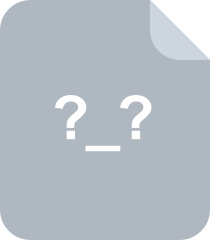
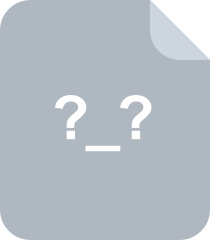
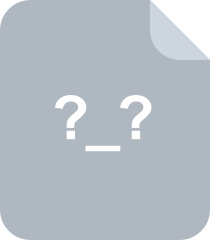
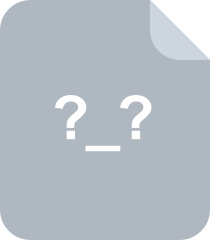
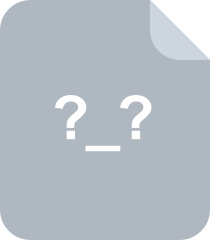
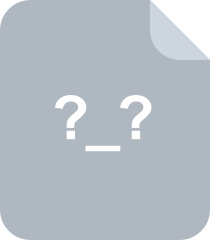
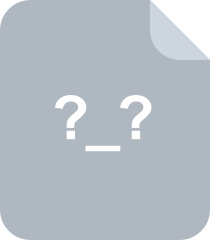
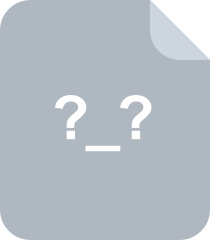
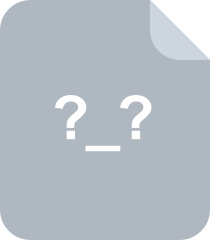
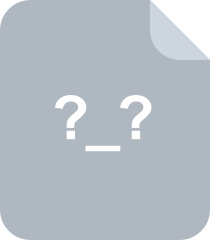
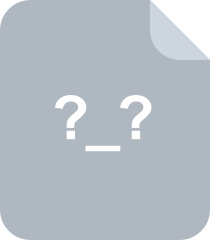
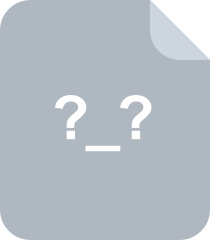
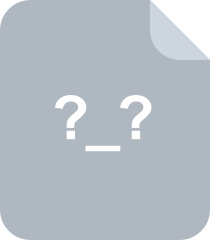
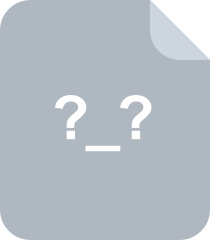
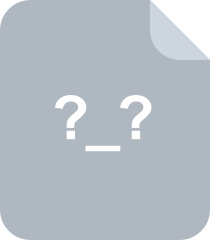
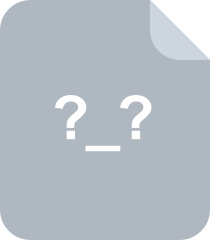
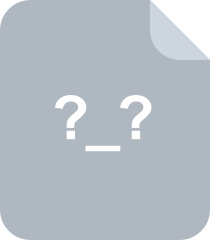
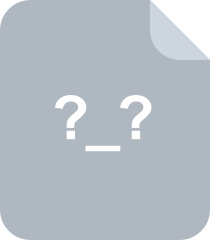
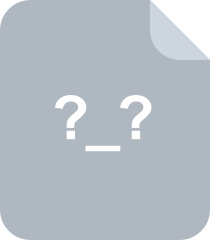
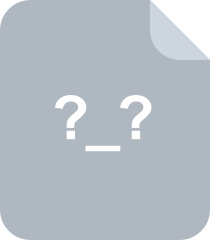
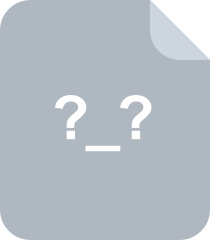
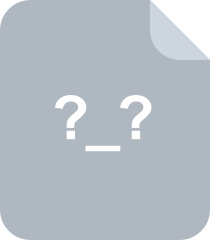
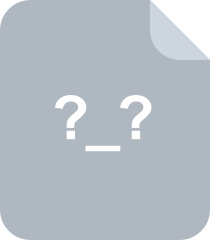
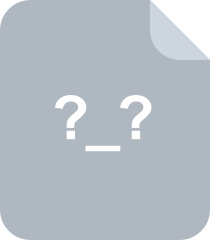
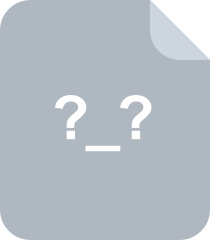
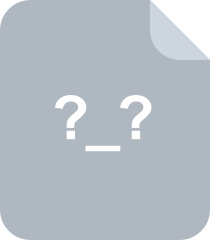
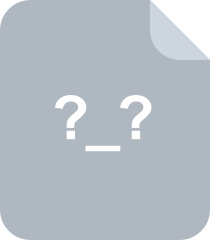
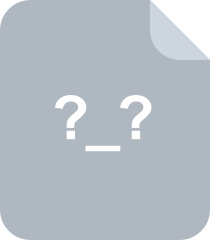
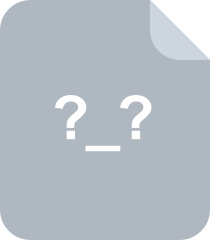
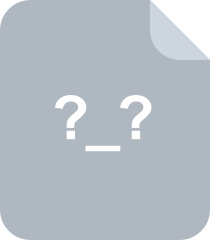
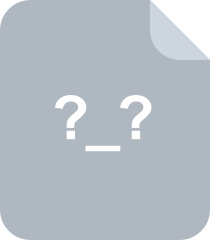
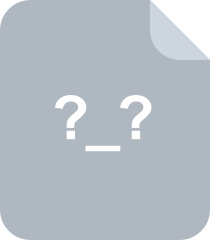
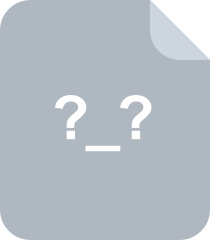
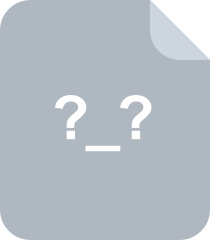
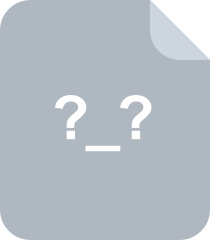
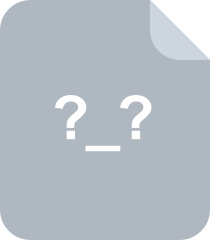
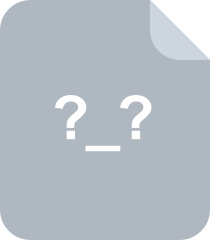
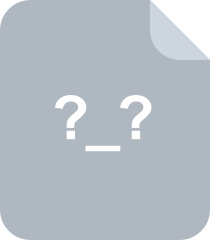
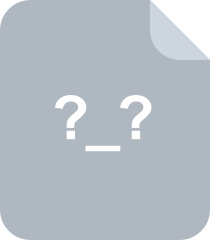
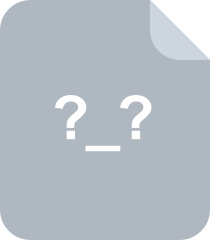
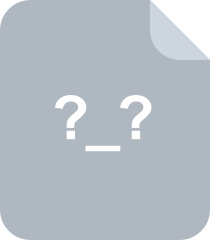
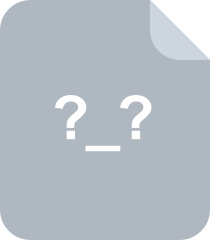
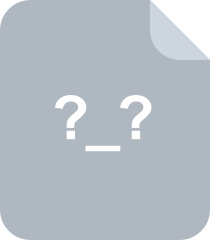
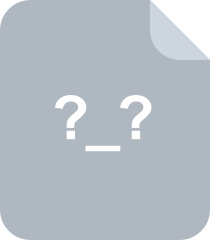
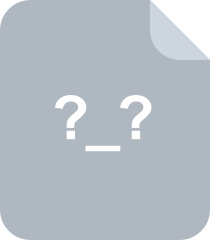
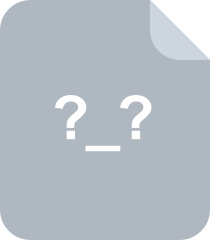
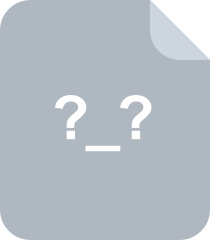
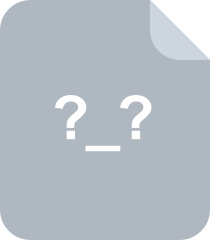
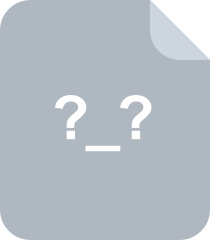
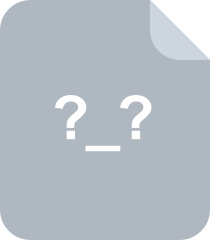
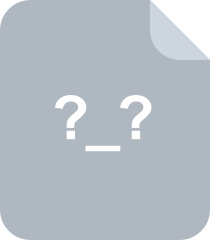
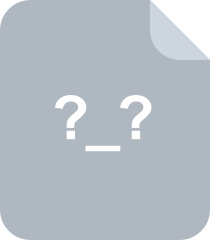
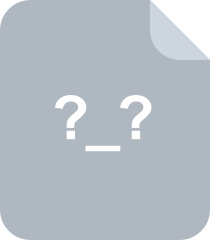
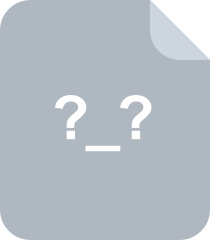
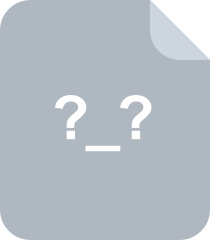
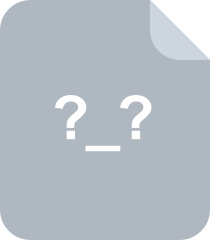
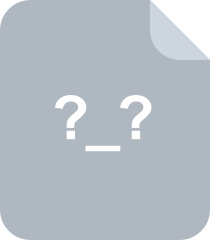
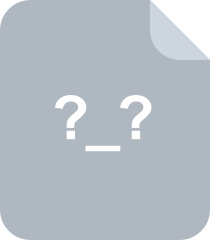
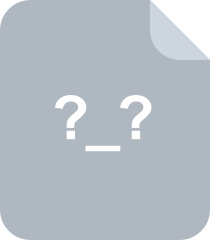
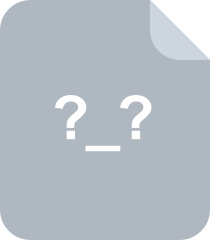
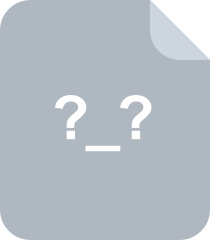
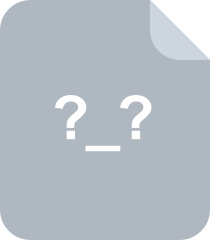
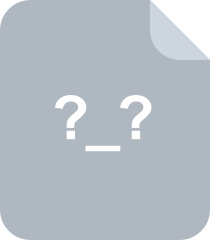
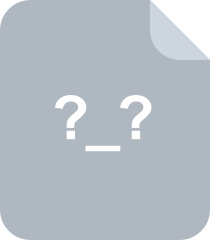
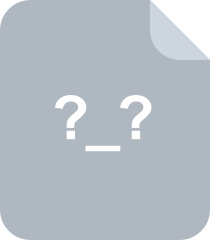
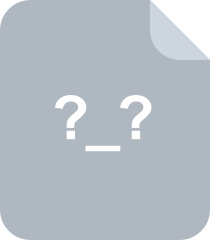
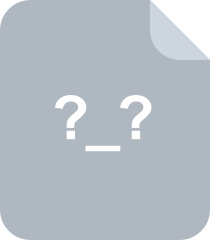
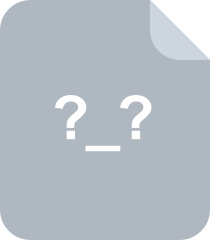
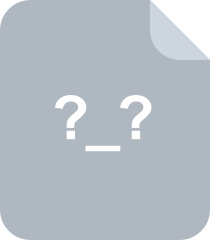
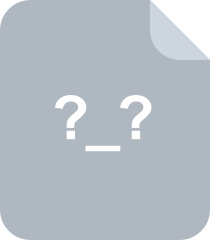
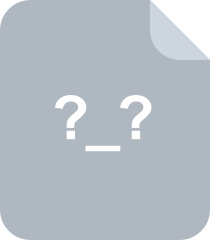
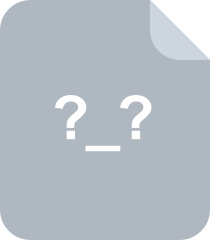
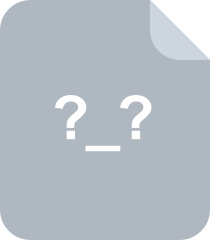
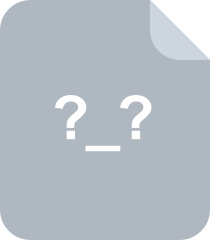
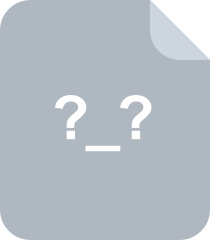
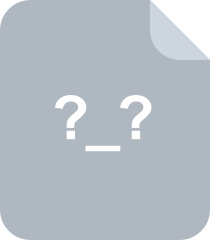
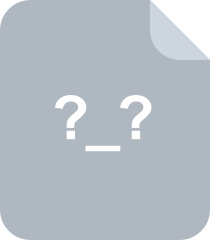
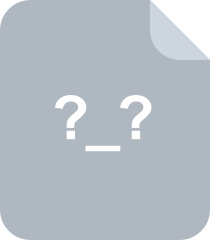
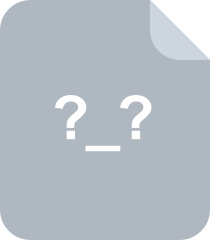
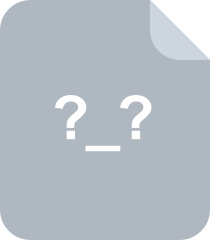
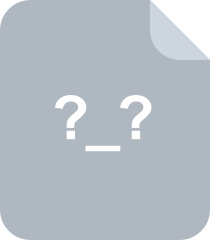
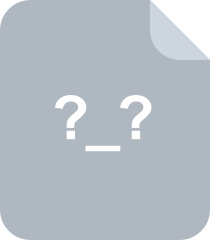
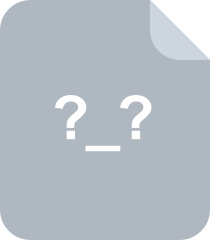
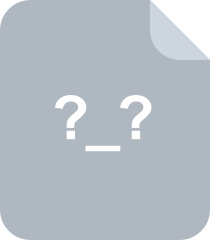
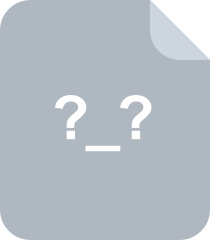
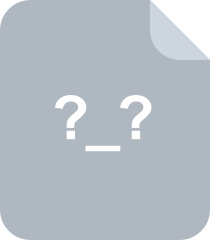
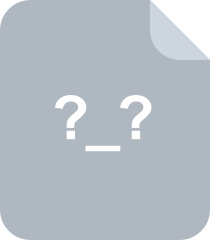
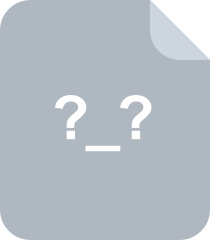
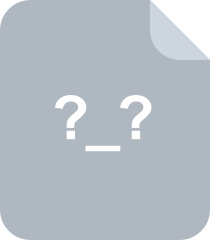
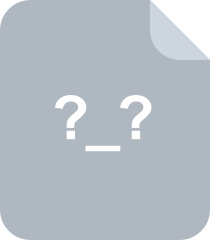
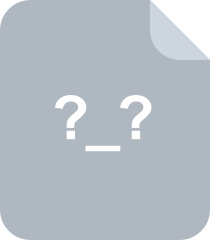
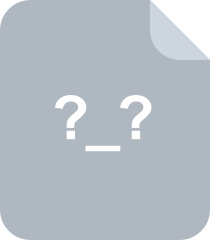
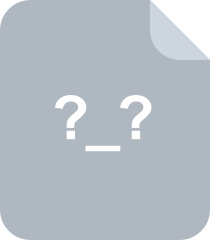
共 440 条
- 1
- 2
- 3
- 4
- 5
资源评论
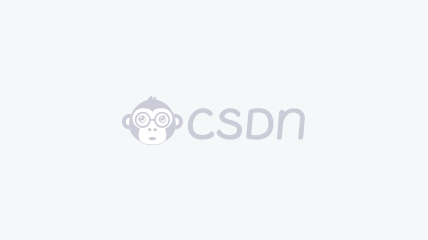
- sksksnss2024-06-14非常有用的资源,可以直接使用,对我很有用,果断支持!
- 普通网友2024-01-29资源太好了,解决了我当下遇到的难题,抱紧大佬的大腿~manylinux2024-06-07感谢支持!!
- 2301_767428182023-09-22总算找到了自己想要的资源,对自己的启发很大,感谢分享~manylinux2024-01-22欢迎交流学习

manylinux
- 粉丝: 4426
- 资源: 2491
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

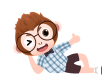
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


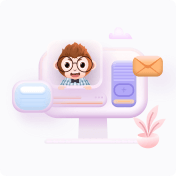
安全验证
文档复制为VIP权益,开通VIP直接复制
