import React from 'react';
import {Row, Col, Icon, Layout, Button, Tabs, Table, Tree, Card,Tag ,Timeline} from 'antd';
import {Map, Markers, InfoWindow} from 'react-amap';
import fetch from 'dva/fetch';
import PropTypes from 'prop-types';
import bpimg from '../assets/BP.png';
// import { routerRedux } from 'dva/router';
// function reducers(state = 1, action) {
// switch (action.type) {
// case 'Done':
// return state + action.payload;
// default:
// return state;
// }
// };
const TreeNode = Tree.TreeNode;
const {Sider, Content} = Layout;
const {Column} = Table;
const TabPane = Tabs.TabPane;
const ReactHighcharts = require('react-highcharts');
ReactHighcharts.Highcharts.setOptions({
global:{
useUTC:false
}
});
const Highcharts = require('highcharts');
// const chartconfig = {
// chart: {
// zoomType: 'x',
// },
// title: {
// text: '洪涝事件探测结果阶段划分图',
// },
// subtitle: {
// text: '异常事件',
// },
// xAxis: {
// type: 'datetime',
// labels: {
// format: '{value:%y-%m-%d %H:%M}',
// align: 'right',
// rotation: -30,
// },
// },
// series: null,
// };
const divStyle = {
color: 'red',
backgroundImage: 'url(../assets/BP.png)',
// 或者 background: `url${require("1.jpg")}`
};
export class EventShowComponent extends React.Component {
static contextTypes = {
router: PropTypes.object,
}
state = {
eventID: '',
floodResult:null,
propertys: [],
sensors: null,
markers: {
position: {
longitude: 115,
latitude: 30,
},
},
currentMarker: null,
visible: true,
selectedRowKeys: [],
center: {longitude: 120.1, latitude: 30.1},
activeServiceStatus: '无事件',
chartconfig: {
chart: {
zoomType: 'x',
},
title: {
text: '洪涝事件探测结果阶段划分图',
},
subtitle: {
text: '异常事件',
},
xAxis: {
type: 'datetime',
labels: {
format: '{value:%y-%m-%d %H:%M}',
align: 'right',
rotation: -30,
},
},
series: null,
},
currentMessage:null,
predictConfig:null,
statisticMessage:null
};
constructor(props, context) {
super(props, context);
// alert(this.props.eventID);
// 根据eventID获取事件的名称,事件的传感器信息,事件的基本参数信息
// 获取当前页面的基本信息
// alert(this.props.eventID);
// this.showChart();
const _this = this;
// 随机生成 10 个标记点的原始数据
this.markers = null;
this.markersEvents = {
click(e, marker) {
// 通过高德原生提供的 getExtData 方法获取原始数据
const extData = marker.getExtData();
const index = extData.myIndex;
// alert(extData.position.longitude);
const visi = _this.state.visible;
_this.setState({currentMarker: extData, visible: !visi});
},
};
this.setState({
eventID: this.props.eventID,
});
}
componentDidMount() {
// this.interval = setInterval(() => fetch('http://www.myflood.com/getEventStatus', {
// method: 'POST',
// credentials: 'include',
// headers:
// {
// Accept: 'application/json',
// 'Content-Type': 'application/json; charset=utf-8',
// },
// body: this.props.eventID,
// }).then((response) => {
// if (response.status >= 200 && response.status < 300) {
// return response.json();
// } else {
// const error = new Error(response.statusText);
// error.response = response;
// throw error;
// }
// },
// ).then((data) => {
// if (data.flag) {
// this.setState({
// activeServiceStatus: data.object,
// });
// } else {
// const path = {
// pathname: '/error',
// query: data.message,
// };
// this.context.router.history.push(path);
// }
// }), 1000);
// this.showChart();
// 获取传感器的基本信息
fetch('http://www.myflood.com/getEventBaseData', {
method: 'POST',
credentials: 'include',
headers:
{
Accept: 'application/json',
'Content-Type': 'application/json; charset=utf-8',
},
body: this.props.eventID,
}).then((response) => {
if (response.status >= 200 && response.status < 300) {
return response.json();
} else {
const error = new Error(response.statusText);
error.response = response;
throw error;
}
},
).then((data) => {
if (data.flag) {
// 构建一个数组,用于存储所有的传感器数据
var predictSensors = data.object.dataset.sensors;
var statisticSensors=data.object.sensors;
var sensors = new Array();
for(var i=0;i<predictSensors.length;i++) {
sensors.push(predictSensors[i]);
}
for (var i=0;i<statisticSensors.length;i++) {
sensors.push(statisticSensors[i]);
}
console.log(sensors);
this.setState({
floodResult: data.object,
sensors: sensors,
propertys: data.object.propertys,
center: {
longitude: data.object.dataset.diagnosisSensor.lon,
latitude: data.object.dataset.diagnosisSensor.lat
},
});
} else {
const path = {
pathname: '/error',
query: data.message,
};
this.context.router.history.push(path);
}
}).catch((error) => {
alert(error.toString());
});
// 获取当前流
// this.runPolling();
}
// *pollForWeatherInfo() {
// while (true) {
// yield fetch('http://www.myflood.com/sadJson', {
// method: 'get',
// }).then((d) => {
// const json = d.json();
// return json;
// });
// }
// }
// runPolling=(generator) => {
// if (!generator) {
// generator = this.pollForWeatherInfo();
// }
//
// const p = generator.next();
// p.value.then((d) => {
// if (true) {
// this.setState({
// chartconfig: {
// chart: {
// zoomType: 'x',
// },
// title: {
// text: '洪涝事件探测结果阶段划分图',
// },
// subtitle: {
// text: '异常事件',
// },
// xAxis: {
// type: 'datetime',
// labels: {
// format: '{value:%y-%m-%d %H:%M}',
// align: 'right',
// rotation: -30,
// },
// },
// series: d,
// },
// });
// this.runPolling(generator);
// } else {
// console.log(d);
// }
// });
// }
showChart = () => {
// clearInterval(this.interval);
fetch('http://www.myflood.com/getEventDataJson', {
method: 'POST',
credentials: 'include',
headers:
{
Accept: 'application/json',
// 'Access-Control-Allow-Origin': 'http://127.0.0.1',
// 'Access-Control-Allow-Credentials': 'true',
'Content-Type': 'application/json; charset=utf-8',
},
body: JSON.stringify(this.props.eventID),
}).then((response) => {
if (response.status >= 200 && response.status < 300) {
return response.json();
} else {
const error = new Error(response.statusText);
error.response = response;
throw error;
}
},
).then((data) => {
if (data.flag) {
this.setState({
chartconfig: {
chart: {
zoomType: 'x',
},
title: {
text: '洪涝事件探测结果阶段划分图',
},
s
没有合适的资源?快使用搜索试试~ 我知道了~
基于JavaScript的洪涝监测系统源码.zip
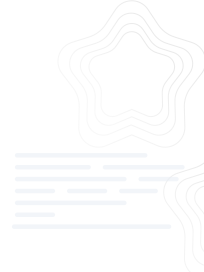
共63个文件
js:37个
png:7个
xml:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
基于JavaScript的洪涝监测系统源码.zip 使用ant desgin完成项目前端页面 其中,将mock服务器关闭,使用fetch实现跨域session请求等, 后台使用springmvc接收数据 链接跳转以window.location.href方式前端跳转
资源推荐
资源详情
资源评论
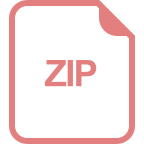
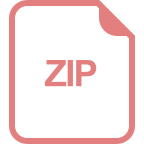
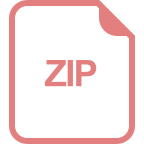
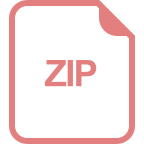
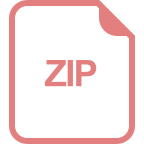
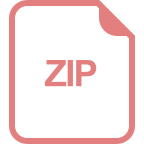
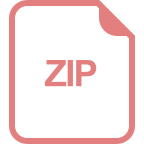
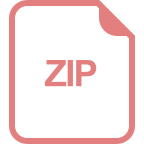
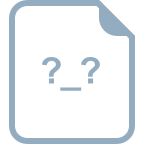
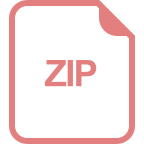
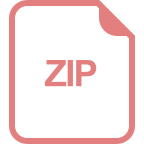
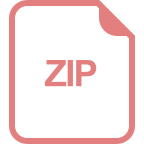
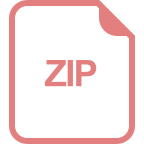
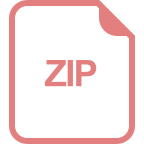
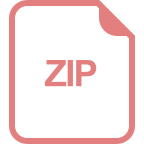
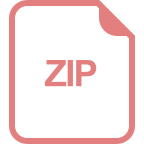
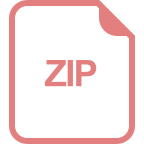
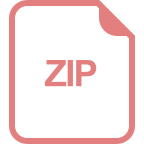
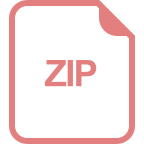
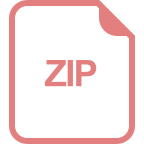
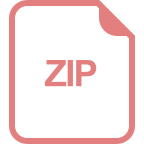
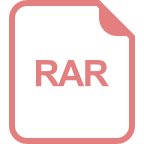
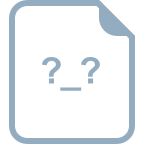
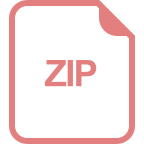
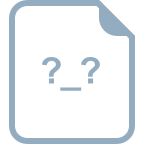
收起资源包目录

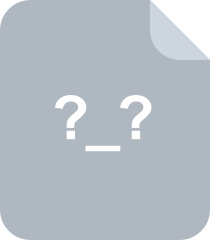

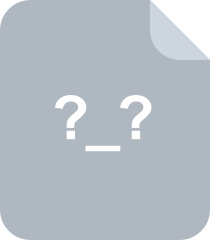
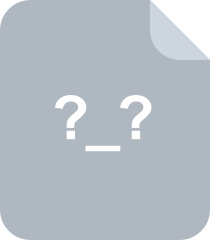
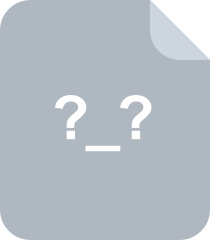


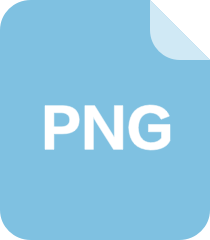
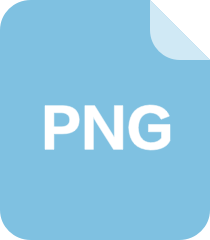
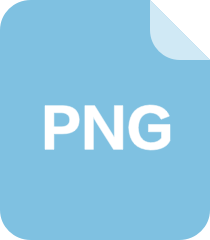
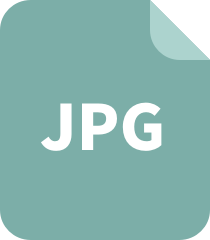
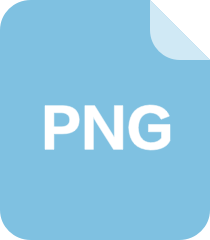
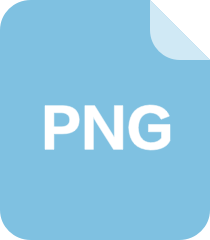
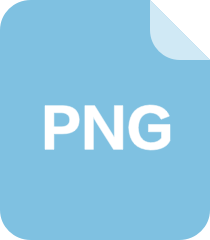
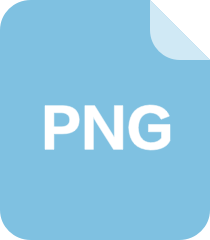

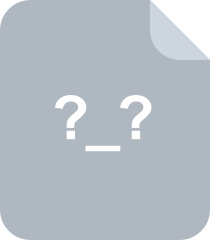
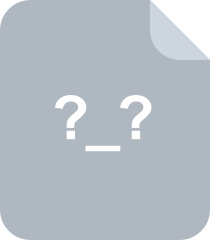
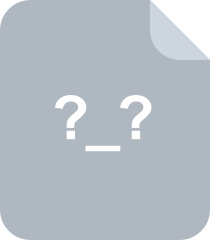

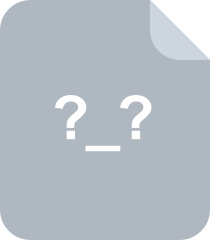

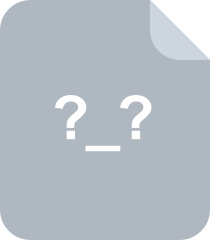
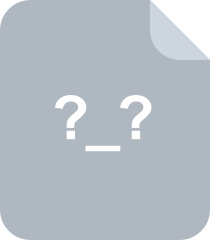
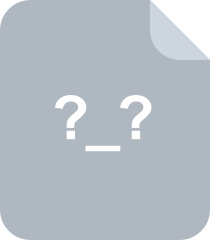
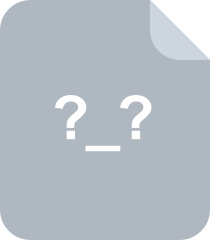
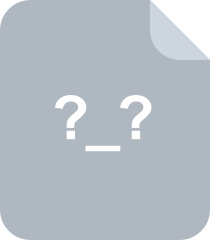
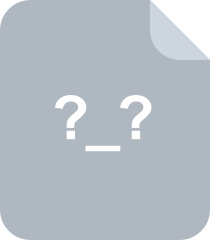
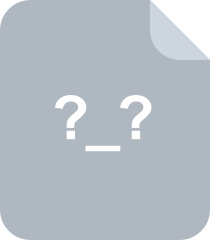
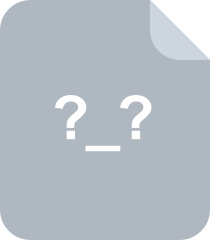
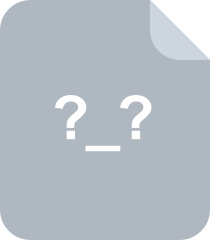
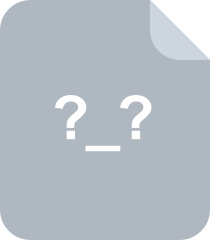
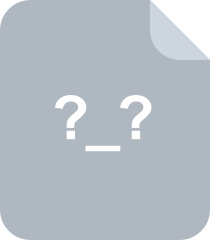
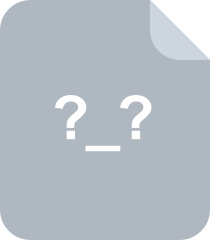
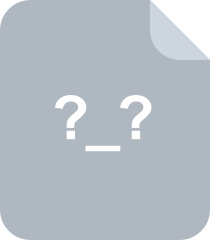
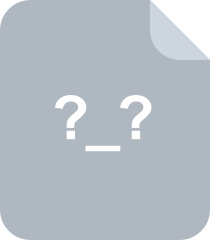
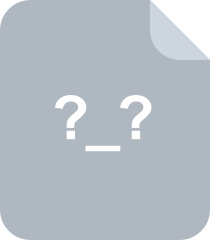
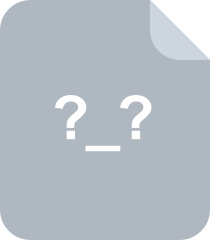

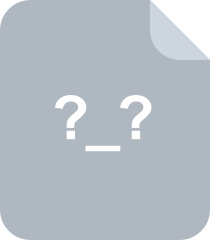
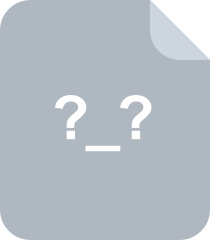
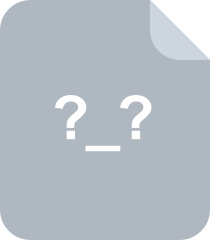
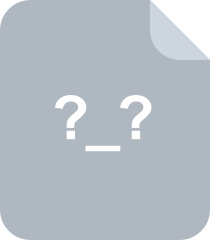
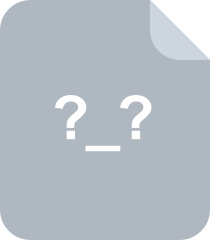
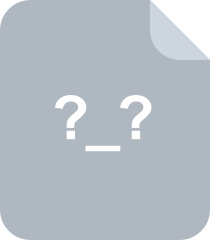
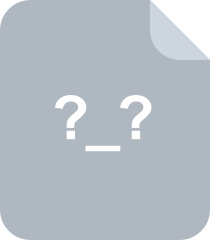
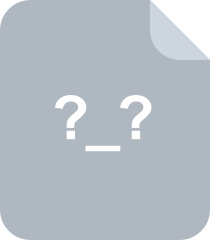
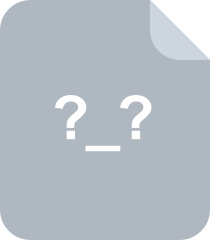
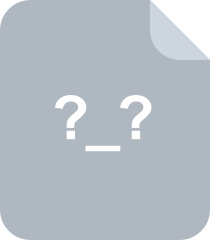
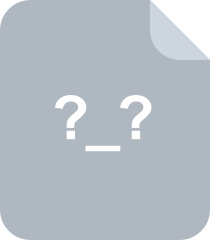
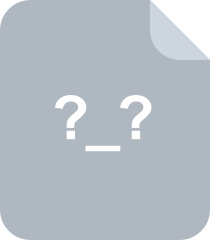
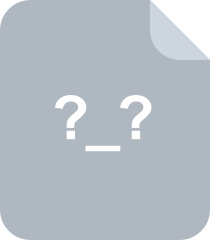
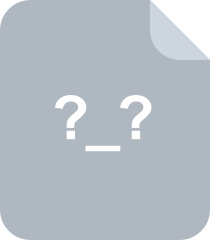
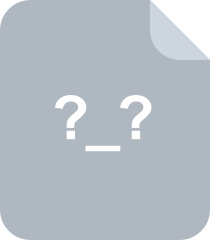

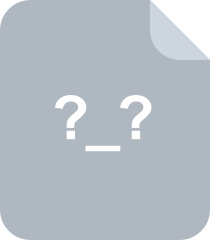
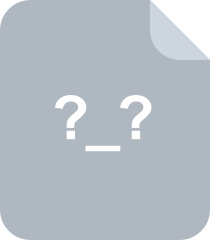
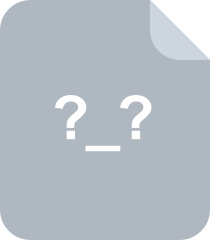
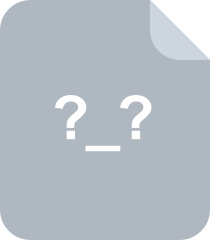
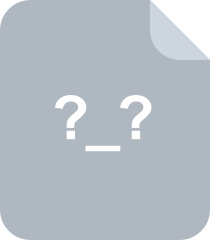

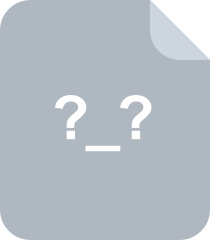
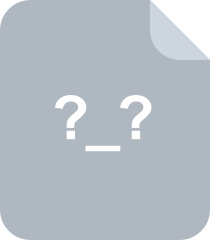
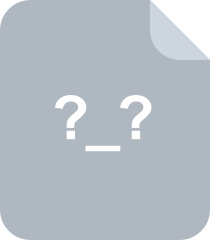

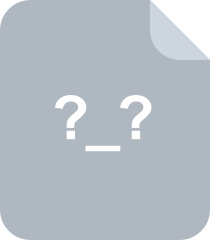
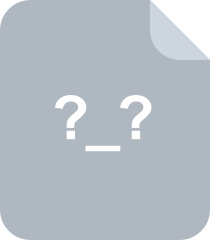
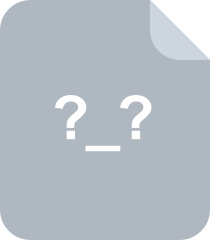
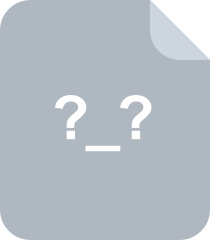

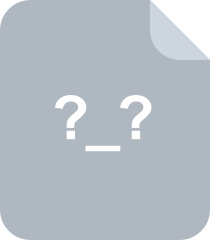
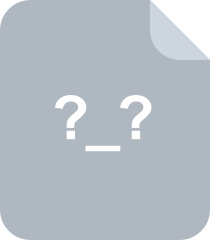
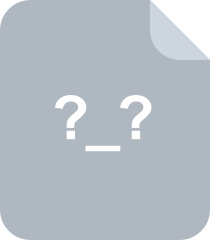
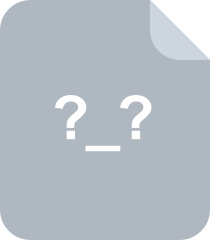
共 63 条
- 1
资源评论
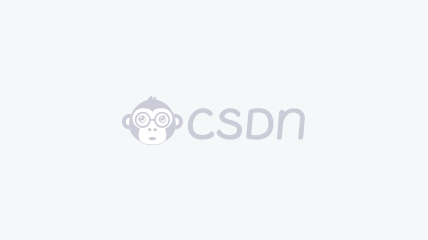
- 八月的八2024-05-08这个资源内容超赞,对我来说很有价值,很实用,感谢大佬分享~

manylinux
- 粉丝: 4524
- 资源: 2517
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

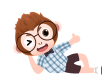
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


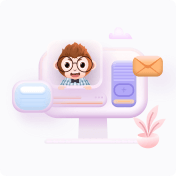
安全验证
文档复制为VIP权益,开通VIP直接复制
