import json
from functools import wraps
from . import *
from flask import render_template, request, flash, redirect, url_for, make_response, session,escape
from .forms import RegisterForm,ArticleForm
from passlib.hash import sha256_crypt
from .models import Users,Artciles
@app.route('/register',methods=['GET','POST'])
def register():
form = RegisterForm(request.form)
if form.validate_on_submit():
# 接收表单数据
email = form.email.data
username = form.username.data
password = sha256_crypt.encrypt(str(form.password.data))
# 存入数据库
user = Users()
user.email =email
user.username = username
user.password = password
db.session.add(user)
db.session.commit()
flash('您已注册成功,请登录','success')
return redirect(url_for('login'))
return render_template('register.html',form=form)
@app.route('/login',methods=['GET','POST'])
def login():
# 查看是否已经登录,若已登录,直接跳转
if 'login_in' in request.cookies:
return redirect(url_for('dashboard'))
else:
if 'login_in' in session:
session['login_in'] = True
session['username'] = request.cookies.get('username')
return redirect(url_for('dashboard'))
if request.method == 'POST':
# 获取表单数据
username = request.form['username']
password_candicate = request.form['password']
res = Users.query.filter_by(username=username).first()
if res:
password = res.password
if sha256_crypt.verify(password_candicate,password):
# 调用verify方法验证如果为真,验证通过
# 写入cookie
response = make_response(
'<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 3.2 Final//EN">\n'
"<title>Redirecting...</title>\n"
"<h1>Redirecting...</h1>\n"
"<p>You should be redirected automatically to target URL: "
'<a href="%s">%s</a>. If not click the link.'
% (escape(url_for('dashboard')),
escape(url_for('dashboard'))), 302)
response.set_cookie('login_in','True',max_age=60*60*24*38)
response.set_cookie('username',username,max_age=60*60*24*38)
# 存入session
session['login_in'] = True
session['username']=username
response.location = escape(url_for('dashboard'))
return response
else:
error = '用户名和密码不匹配'
return render_template('login.html',error=error)
else:
error = '用户名不存在'
return render_template('login.html',error=error)
return render_template('login.html')
def is_login_in(f):
@wraps(f)
def wrap(*args,**kwargs):
if 'login_in' in session:
return f(*args,**kwargs)
else:
flash('无权访问,请先登录','danger')
return redirect(url_for('login'))
return wrap
@app.route('/logout')
@is_login_in
def logout():
if 'login_in' in request.cookies:
response = make_response(
'<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 3.2 Final//EN">\n'
"<title>Redirecting...</title>\n"
"<h1>Redirecting...</h1>\n"
"<p>You should be redirected automatically to target URL: "
'<a href="%s">%s</a>. If not click the link.'
% (escape(url_for('index')),
escape(url_for('index'))), 302)
response.delete_cookie('login_in')
response.location = escape(url_for('index'))
return response
else:
session.clear()
flash('您已成功退出', 'success')
return redirect(url_for('index'))
@app.route('/dashboard')
@is_login_in
def dashboard():
notes = Artciles.query.order_by(Artciles.timestamp.desc()).all()
if notes:
return render_template('dashboard.html',notes=notes)
else:
msg = '暂无笔记信息'
return render_template('dashboard.html',msg=msg)
@app.route('/artcile/<string:id>')
@is_login_in
def artcile(id):
note = Artciles.query.get(id)
return render_template('article.html',note=note)
@app.route('/add_article',methods=['GET','POST'])
@is_login_in
def add_article():
form = ArticleForm()
if form.validate_on_submit():
note = Artciles()
note.title = form.title.data
note.author = session['username']
note.body = form.body.data
db.session.add(note)
db.session.commit()
flash('提交成功','success')
return redirect(url_for('dashboard'))
return render_template('add_article.html', form=form) # 渲染模板
@app.route('/delete_article/<int:id>',methods=['POST'])
@is_login_in
def delete_article(id):
note = Artciles.query.filter_by(id=id).first()
print(note.body)
db.session.delete(note)
db.session.commit()
flash('删除成功','success')
return redirect(url_for('dashboard'))
@app.route('/edit_article/<int:id>',methods=['GET','POST'])
@is_login_in
def edit_article(id):
note = Artciles.query.filter_by(id=id).first()
if not note:
flash('ID错误','danger')
return redirect(url_for('dashboard'))
form = ArticleForm(request.form)
if form.validate_on_submit():
note.title = form.title.data
note.body = form.body.data
db.session.add(note)
db.session.commit()
flash('更改成功','success')
return redirect(url_for('dashboard'))
form.title.data = note.title
form.body.data = note.body
return render_template('edit_article.html',form=form)
@app.route('/')
def index():
return render_template('home.html')
# 如果表单提交且字段验证通过,查询数据库是否存在相同数据
# 如果存在,返回错误提示,如果不存在,则存入数据库
@app.route('/check_info')
def check_info(data):
# 接收数据
email = data
if Users.object.filter(email=email):
dic = {
'status':0,
'msg':'邮箱已被注册'
}
else:
dic = {
'status': 1,
'msg': '邮箱可以使用'
}
return json.dumps(dic)
@app.route('/checkLogged')
def checkLogged():
if 'login_in' in session:
dic = {
'loginStatus':1,
}
else:
dic = {
'loginStatus':0,
}
print(dic['loginStatus'])
return json.dumps(dic)
if __name__ == '__main__':
app.run(debug=True)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
CSDN IT狂飙上传的代码均可运行,功能ok的情况下才上传的,直接替换数据即可使用,小白也能轻松上手 【资源说明】 Python优秀项目 基于Flask+MySQL实现的在线笔记源码+部署文档+数据资料.zip 1、代码压缩包内容 代码的项目文件 部署文档文件 2、代码运行版本 python3.7或者3.7以上的版本;若运行有误,根据提示GPT修改;若不会,私信博主(问题描述要详细) 3、运行操作步骤 步骤一:将代码的项目目录使用IDEA打开(IDEA要配置好python环境) 步骤二:根据部署文档或运行提示安装项目所需的库 步骤三:IDEA点击运行,等待程序服务启动完成 4、python资讯 如需要其他python项目的定制服务,可后台私信博主(注明你的项目需求) 4.1 python或人工智能项目辅导 4.2 python或人工智能程序定制 4.3 python科研合作 Django、Flask、Pytorch、Scrapy、PyQt、爬虫、可视化、大数据、推荐系统、人工智能、大模型
资源推荐
资源详情
资源评论
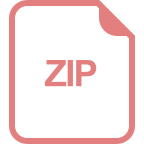
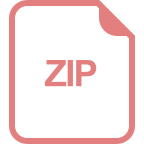
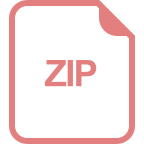
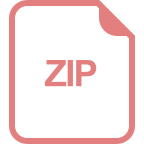
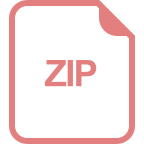
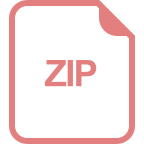
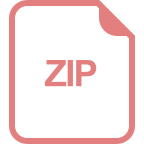
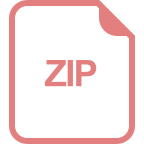
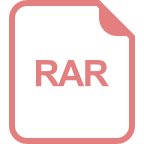
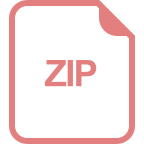
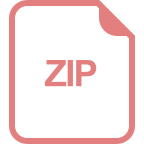
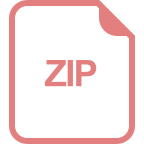
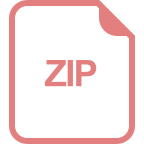
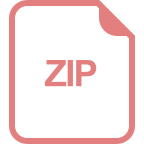
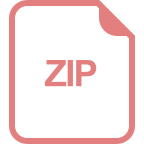
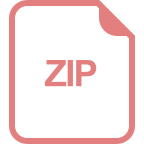
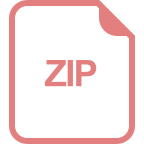
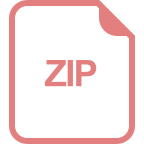
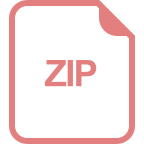
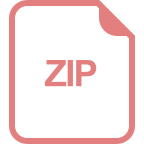
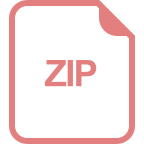
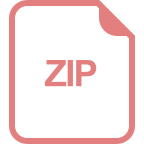
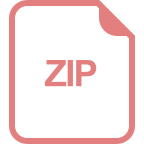
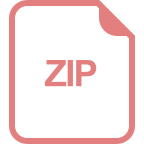
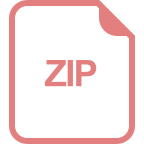
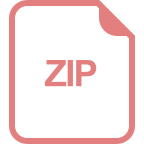
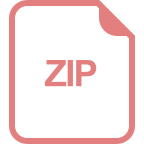
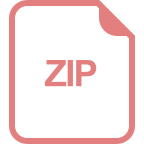
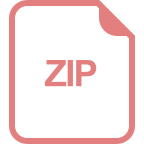
收起资源包目录

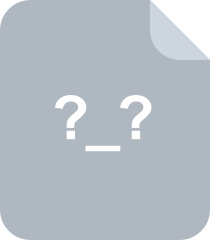
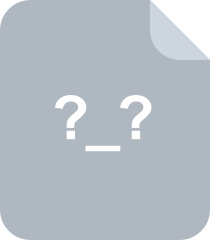


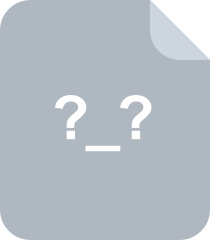

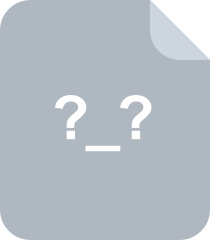

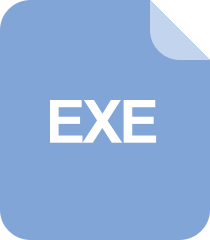
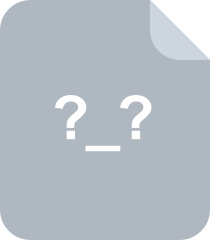
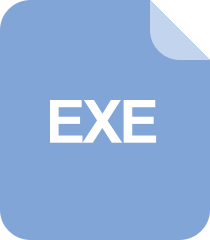
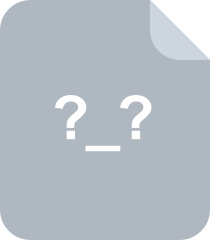
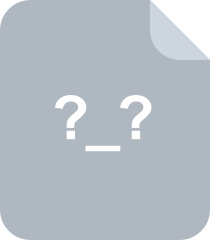
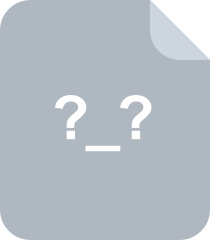
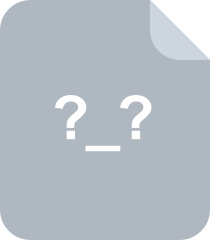
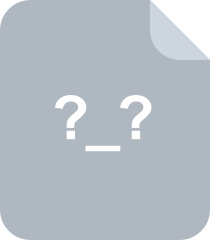

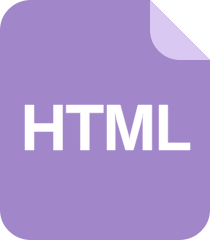

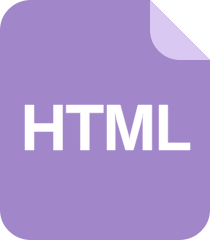
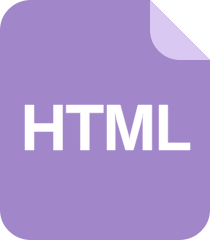
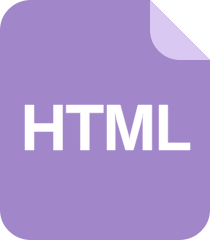
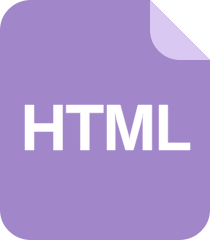
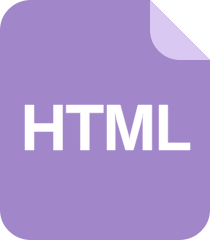
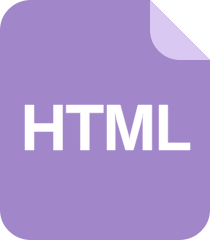
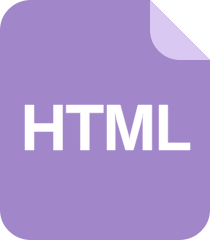
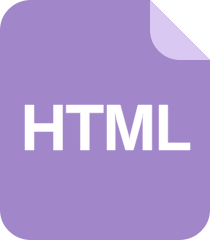
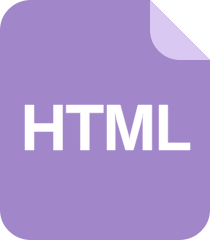
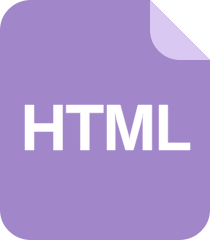
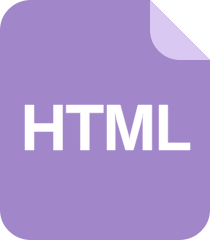
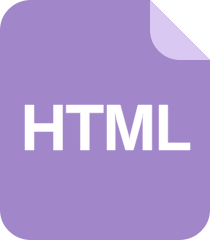
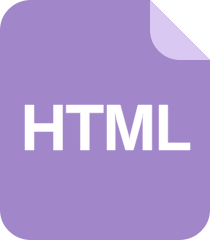

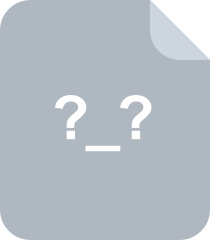
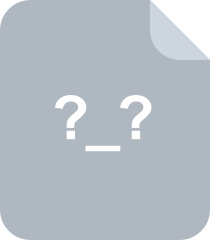
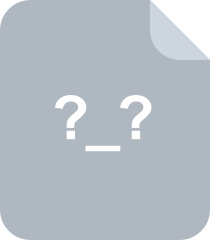

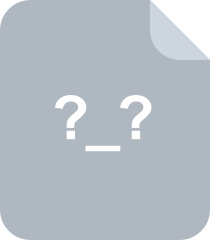
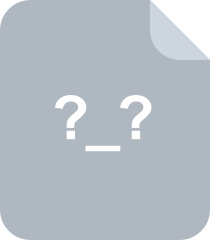

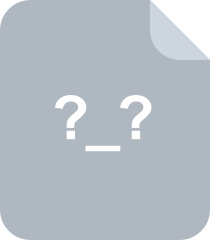
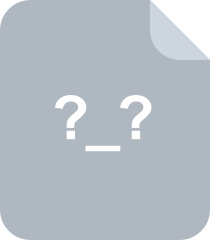
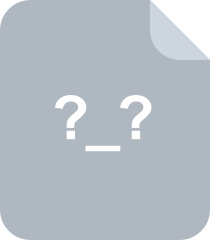
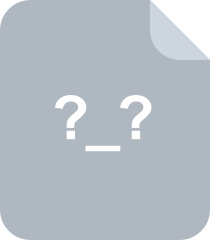
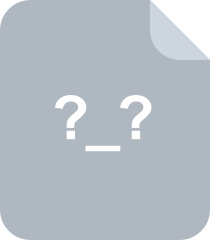

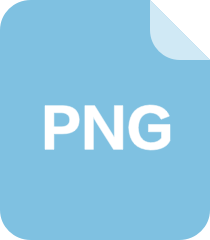
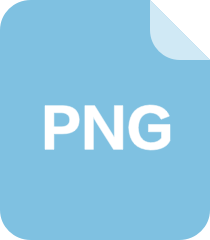

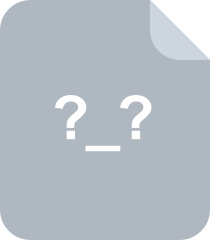
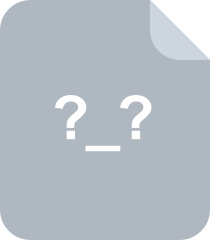
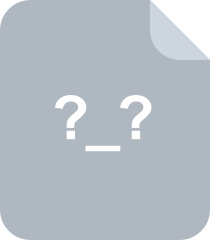
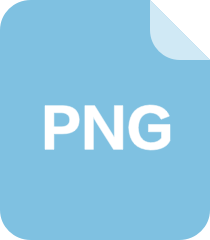
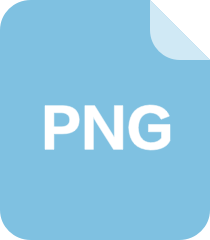
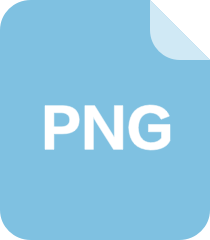

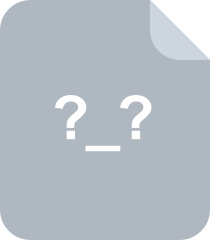
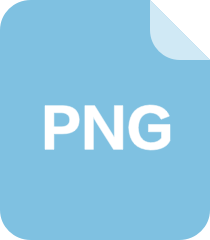
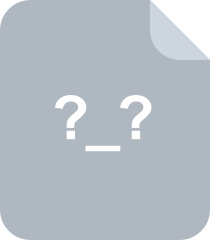
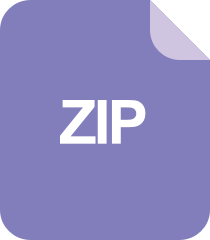
共 48 条
- 1
资源评论
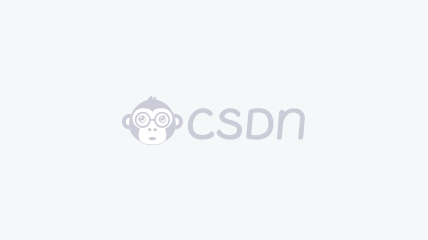

IT狂飙
- 粉丝: 4824
- 资源: 2654
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

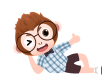
最新资源
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
- (源码)基于计算机系统原理与Arduino技术的学习平台.zip
- (源码)基于SSM框架的大学消息通知系统服务端.zip
- (源码)基于Java Servlet的学生信息管理系统.zip
- (源码)基于Qt和AVR的FestosMechatronics系统终端.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


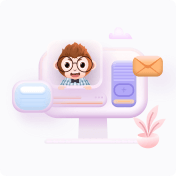
安全验证
文档复制为VIP权益,开通VIP直接复制
