# sql-util 实体SQL工具
[](https://jitpack.io/#com.gitee.wb04307201/sql-util)
[](https://gitee.com/wb04307201/sql-util)
[](https://gitee.com/wb04307201/sql-util)
[](https://github.com/wb04307201/sql-util)
[](https://github.com/wb04307201/sql-util)
> 提供一套高效、便捷的数据库操作工具集,
> 包括多数据源连接池、SQL语句执行工具类、SQL构造工具类、从实体类构造SQL工具类,
> 亦可以通过实体快速构造web页面,
> 帮助开发者简化数据库操作,提高开发效率和代码质量。
## 代码示例
1. 使用[实体SQL工具](https://gitee.com/wb04307201/sql-util)实现的[实体SQL工具Demo](https://gitee.com/wb04307201/sql-util-demo)
2. 使用[文档在线预览](https://gitee.com/wb04307201/file-preview-spring-boot-starter)、[多平台文件存储](https://gitee.com/wb04307201/file-storage-spring-boot-starter)、[实体SQL工具](https://gitee.com/wb04307201/sql-util)实现的[文件预览Demo](https://gitee.com/wb04307201/file-preview-demo)
3. 使用[多平台文件存储](https://gitee.com/wb04307201/file-storage-spring-boot-starter)、[实体SQL工具](https://gitee.com/wb04307201/sql-util)实现的[文件存储Demo](https://gitee.com/wb04307201/file-storage-demo)
4. 使用[消息中间件](https://gitee.com/wb04307201/message-spring-boot-starter)、[实体SQL工具](https://gitee.com/wb04307201/sql-util)实现的[消息发送代码示例](https://gitee.com/wb04307201/message-demo)
5. 使用[动态调度](https://gitee.com/wb04307201/dynamic-schedule-spring-boot-starter)、[消息中间件](https://gitee.com/wb04307201/message-spring-boot-starter)、[动态编译加载执行工具](https://gitee.com/wb04307201/loader-util)、[实体SQL工具](https://gitee.com/wb04307201/sql-util)实现的[在线编码、动态调度、发送钉钉群消息、快速构造web页面Demo](https://gitee.com/wb04307201/dynamic-schedule-demo)
| 序号 | 工具类 | 描述 |
|----|---------------------|----------------|
| 1 | MutilConnectionPool | 一个多数据源连接池 |
| 2 | ExecuteSqlUtils | sql语句执行工具类 |
| 3 | SQL | SQL构造工具,执行工具 |
| 4 | ModelSqlUtils | 从实体类构造SQL,执行工具 |
| 5 | EntityWeb | 从实体类快速构造web页面 |
## 第一步 增加 JitPack 仓库
```xml
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
```
## 第二步 引入jar
1.3.0版本后升级到jdk17 SpringBoot3+
继续使用jdk 8请查看jdk8分支
```xml
<dependency>
<groupId>com.gitee.wb04307201</groupId>
<artifactId>sql-util</artifactId>
<version>1.3.6</version>
</dependency>
```
## 第三步 使用工具
#### MutilConnectionPool使用示例
```java
// 判断数据源是否加载
if (Boolean.FALSE.equals(MutilConnectionPool.check("test"))) {
// 加载数据源
MutilConnectionPool.init("test", "jdbc:h2:file:./data/demo;AUTO_SERVER=TRUE", "sa", "");
}
// 获取数据源,注意使用完之后释放连接
Connection connection = MutilConnectionPool.getConnection("test");
connection.close();
// 通过传入函数式接口执行方法,内部会自动创建连接并在使用之后释放
MutilConnectionPool.run("test", conn -> null);
```
#### ExecuteSqlUtils使用示例
```java
// 判断数据源是否加载
if (Boolean.FALSE.equals(MutilConnectionPool.check("test"))) {
// 加载数据源
MutilConnectionPool.init("test", "jdbc:h2:file:./data/demo;AUTO_SERVER=TRUE", "sa", "");
}
Connection connection = MutilConnectionPool.getConnection("test");
// 判断表是否存在
Boolean check = ExecuteSqlUtils.isTableExists(connection, "test_user");
// 通过MutilConnectionPool.run检查表是否存在
check = MutilConnectionPool.run("test", conn -> ExecuteSqlUtils.isTableExists(conn, "test_user"));
Map<Integer, Object> params = new HashMap<>();
params.put(1, "123123");
// 执行插入、更新的sql语句
int count = ExecuteSqlUtils.executeUpdate(connection, "update test_user set user_name = ?", params);
count = MutilConnectionPool.run("test", conn -> ExecuteSqlUtils.executeUpdate(conn, "update test_user set user_name = ?", params));
// 执行查询的sql语句
List<Map<String, Object>> list = ExecuteSqlUtils.executeQuery(connection, "select * from test_user where user_name = ?", params, new TypeReference<Map<String, Object>>() {
});
list = MutilConnectionPool.run("test", conn -> ExecuteSqlUtils.executeQuery(conn, "select * from test_user where user_name = ?", params, new TypeReference<Map<String, Object>>() {
}));
// 执行删除的sql语句
MutilConnectionPool.run("test", conn -> ExecuteSqlUtils.executeUpdate(conn, "delete from test_user where user_name = ?", params));
connection.close();
```
#### 在测试SQL、ModelSqlUtils前,请先创建一个测试实体类,例如
```java
package cn.wubo.sql.util.test;
import cn.wubo.sql.util.annotations.Column;
import cn.wubo.sql.util.annotations.Key;
import cn.wubo.sql.util.annotations.Table;
import cn.wubo.sql.util.enums.ColumnType;
import lombok.Data;
import java.util.Date;
@Data
@Table(value = "test_user", desc = "用户")
public class User {
@Key
@Column(value = "id")
private String id;
@Column(value = "user_name",desc = "用户名", type = ColumnType.VARCHAR, length = 20)
private String userName;
@Column(value = "department")
private String department;
@Column(value = "birth", type = ColumnType.DATE)
private Date birth;
@Column(value = "birth1")
private Date birth1;
@Column(value = "age", type = ColumnType.NUMBER, precision = 10, scale = 0)
private Integer age;
@Column(value = "age1")
private Integer age1;
}
```
#### SQL使用示例
```java
// 判断数据源是否加载
if (Boolean.FALSE.equals(MutilConnectionPool.check("test"))) {
// 加载数据源
MutilConnectionPool.init("test", "jdbc:h2:file:./data/demo;AUTO_SERVER=TRUE", "sa", "");
}
SQL<User> userSQL = new SQL<User>(){};
// 判断表是否存在
if(Boolean.TRUE.equals(MutilConnectionPool.run("test",conn -> userSQL.isTableExists(conn)))){
// 删除表
MutilConnectionPool.run("test",conn -> userSQL.drop().dropTable(conn));
}
// 创建表
MutilConnectionPool.run("test",conn -> userSQL.create().createTable(conn));
// 插入数据
MutilConnectionPool.run("test",conn -> userSQL.insert().addSet("user_name","11111").executeUpdate(conn));
// 更新数据
MutilConnectionPool.run("test",conn -> userSQL.update().addSet("user_name","22222").addWhereEQ("user_name","11111").executeUpdate(conn));
// 查询数据
List<User> userList = MutilConnectionPool.run("test",conn -> userSQL.select().addWhereEQ("user_name","22222").executeQuery(conn));
// 删除数据
MutilConnectionPool.run("test",conn -> userSQL.delete().addWhereEQ("user_name","22222").executeUpdate(conn));
```
#### ModelSqlUtils使用示例
```java
// 判断数据源是否加载
if (Boolean.FALSE.equals(MutilConnectionPool.check("test"))) {
// 加载数据源
MutilConnectionPool.init("test", "jdbc:h2:file:./data/demo;AUTO_SERVER=TRUE", "sa", "");
}

忘却的纪念
- 粉丝: 1959
- 资源: 435
最新资源
- 基于Springboot的大学生就业服务平台。Javaee项目,springboot项目。
- 基于STM32与FreeRTOS的智能家居设计实践:从代码敲定到秋招应对的全过程详解,STM32与FreeRTOS结合的智能家居设计实战:从代码敲打到秋招面试准备全解析,基于stm32和freerto
- 基于SSM的校园交易平台。Javaee项目。ssm项目。
- 光储并网直流微电网Simulink仿真模型:混合储能与MPPT最大功率输出及二阶低通滤波法应用,光储并网直流微电网Simulink仿真模型:混合储能与MPPT最大功率输出及二阶低通滤波法应用,光储并网
- 使用C语言编程设计的MP3音乐播放器项目的源代码
- DPF转html的Java代码jar包
- 天然气水合物降压开采技术:基于COMSOL热-流-固耦合模拟与多场动态演化分析,天然气水合物降压开采模拟:基于COMSOL热-流-固耦合及储层参数演化研究,包括水平井筒环空高压充填石英砂层的多维模型探
- Fluent金属熔凝学习宝典:涵盖流动传热、激光热源、金属相变、偏析现象及UDF代码详解,Fluent金属熔凝学习资料大全:涵盖流动传热、激光热源、熔化凝固、宏观偏析、激光熔覆及UDF代码详解,flu
- stata18相关数据集.txt
- Pem电解槽等温与非等温阳极流道模型参数化建模:融合多物理场仿真分析,Pem电解槽等温与非等温阳极流道模型参数化建模:结合多物理场仿真优化研究,Pem电解槽等温阳极单侧流道模型,水电解槽模块与自由与多
- 经历BAT面试后总结的【高级Java后台开发面试指南】,纯净干货无废话,针对高频面试点.zip
- 基于Springboot的协同过滤算法商品推荐系统。Javaee项目,springboot项目。
- 基于开源数据集SMART-DS的计及负荷异常增长的空间负荷预测与配电网规划研究:数据清洗、异常增长诊断、集成学习预测、模糊综合评价与选线定容优化技术探讨,基于开源数据集SMART-DS的计及负荷异常增
- 基于SSM的餐厅点菜管理系统(有报告)。Javaee项目。ssm项目。
- 基于Halcon 64位与Qt 5.8的机器视觉缺陷检测C++源码包,支持VS2015编译环境,全源码解析与实现 ,基于Halcon 64位与Qt 5.8的机器视觉缺陷检测C++源码包,支持VS201
- Springboot+vue的企业OA管理系统(有报告),Javaee项目,springboot vue前后端分离项目。
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


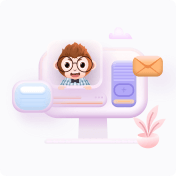