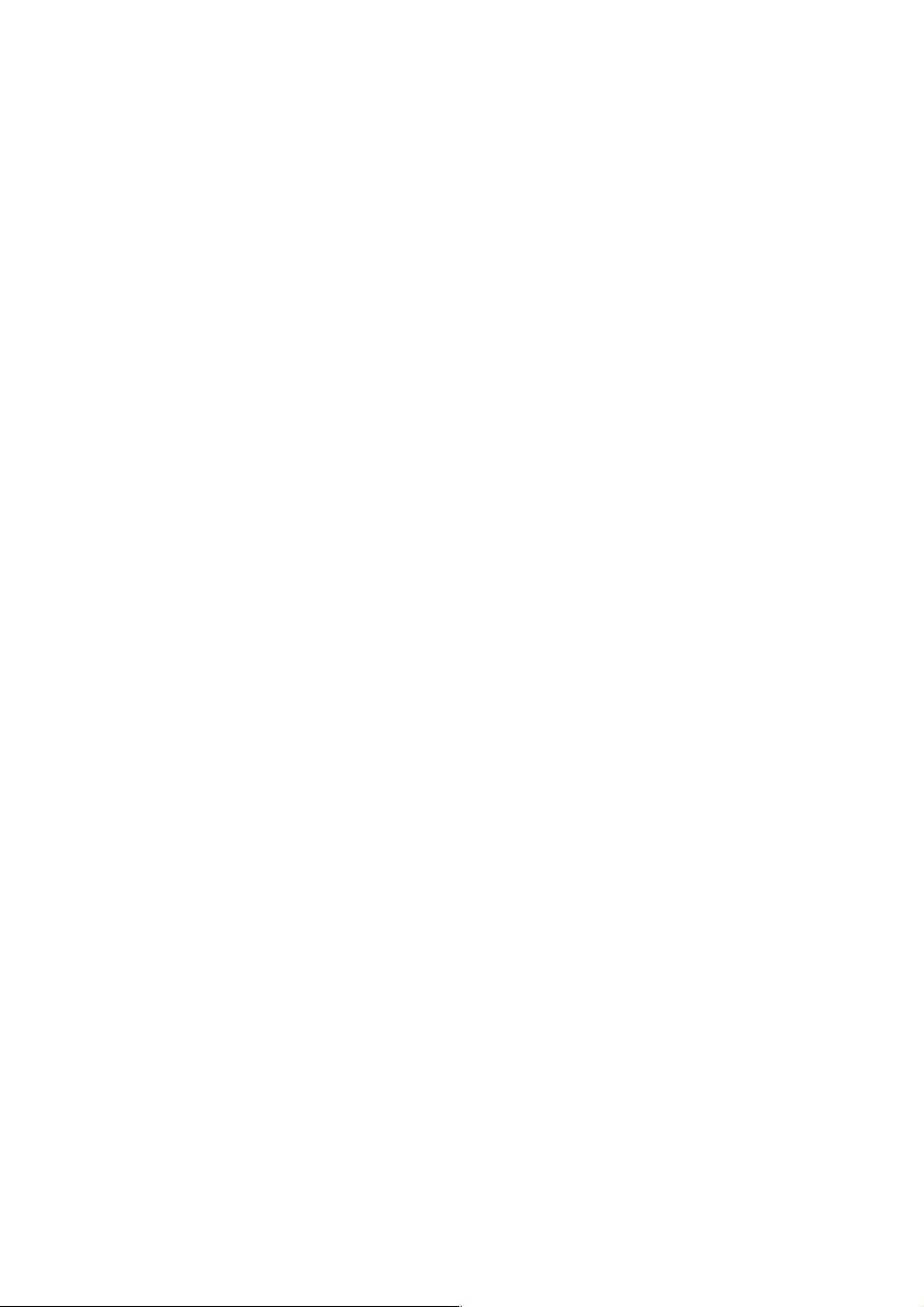
1.3.%Conceptual%Overview
A%debugger%is%a%program%that%lets%you%run%a%second%program,%which%we%will%call%the%debuggee.%The%debugger%lets%you%examine%and%change%the%
state%of%the%debuggee,%and%control%its%execution.%In%particular,%you%can%single-step%the%program,%executing%one%statement%or%instruction%at%a%
time,%in%order%to%watch%the%program's%behavior.
Debuggers%come%in%two%flavors:%instruction-level%debuggers,%which%work%at%the%level%of%machine%instructions,%and%source-level%debuggers,%
which%operate%in%terms%of%your%program's%source%code%and%programming%language.%The%latter%are%considerably%easier%to%use,%and%usually%can%
do%machine-level%debugging%if%necessary.%GDB%is%a%source-level%debugger;%it%is%probably%the%most%widely%applicable%(portable%to%the%largest%
number%of%architectures)%of%any%current%debugger.
GDB%itself%provides%two%user%interfaces:%the%traditional%command-line%interface%(CLI)%and%a%text%user%interface%(TUI).%The%latter%is%meant%for%
regular%terminals%or%terminal%emulators,%dividing%the%screen%into%separate%"windows"%for%the%display%of%source%code,%register%values,%and%so%on.
GDB%provides%support%for%debugging%programs%written%in%C,%C++,%Objective%C,%Java™,
[1]
%and%Fortran.%It%provides%partial%support%for%Modula-2%
programs%compiled%with%the%GNU%Modula-2%compiler%and%for%Ada%programs%compiled%with%the%GNU%Ada%Translator,%GNAT.%GDB%provides%
some%minimal%support%for%debugging%Pascal%programs.%The%Chill%language%is%no%longer%supported.
[1]
%
*
%GDB%can%only%debug%Java%programs%that%have%been%compiled%to%native%machine%code%with%GJC,%the%GNU%Java%compiler%
(part%of%GCC,%the%GNU%Compiler%Collection).
When%working%with%C++%and%Objective%C,%GDB%provides%name%demangling.%C++%and%Objective%C%encode%overloaded%procedure%names%into%a%
unique%"mangled"%name%that%represents%the%procedure's%return%type,%argument%types,%and%class%membership.%This%ensures%so-called%type-safe%
linkage.%There%are%different%methods%for%name%mangling,%thus%GDB%allows%you%to%select%among%a%set%of%supported%methods,%besides%just%
automatically%demangling%names%in%displays.
If%your%program%is%compiled%with%the%GNU%Compiler%Collection%(GCC),%using%the%-g3%and%-gdwarf-2%options,%GDB%understands%references%to%C%
preprocessor%macros.%This%is%particularly%helpful%for%code%using%macros%to%simplify%complicated%struct%and%union%members.%GDB%itself%also%
has%partial%support%for%expanding%preprocessor%macros,%with%more%support%planned.
GDB%allows%you%to%specify%several%different%kinds%of%files%when%doing%debugging:
•
The%exec%file%is%the%executable%program%to%be%debugged—i.e.,%your%program.
•
The%optional%core%file%is%a%memory%dump%generated%by%the%program%when%it%dies;%this%is%used,%together%with%the%exec%file,%for%
postmortem%debugging.%Core%files%are%usually%named%core%on%commercial%Unix%systems.%On%BSD%systems,%they%are%named%
program.core.%On%GNU/Linux%systems,%they%are%named%core.PID,%where%PID%represents%the%process%ID%number.%This%lets%you%
keep%multiple%core%dumps,%if%necessary.
•
The%symbol%file%is%a%separate%file%from%which%GDB%can%read%symbol%information:%information%describing%variable%names,%types,%sizes,%
and%locations%in%the%executable%file.%GDB,%not%the%compiler,%creates%these%files%if%necessary.%Symbol%files%are%rather%esoteric;%they're%
not%necessary%for%run-of-the-mill%debugging.
There%are%different%ways%to%stop%your%program:
•
A%breakpoint%specifies%that%execution%should%stop%at%a%particular%source%code%location.
•
A%watchpoint%indicates%that%execution%should%stop%when%a%particular%memory%location%changes%value.%The%location%can%be%specified%
either%as%a%regular%variable%name%or%via%an%expression%(such%as%one%involving%pointers).%If%hardware%assistance%for%watchpoints%is%
available,%GDB%uses%it,%making%the%cost%of%using%watchpoints%small.%If%it%is%not%available,%GDB%uses%virtual%memory%techniques,%if%
possible,%to%implement%watchpoints.%This%also%keeps%the%cost%down.%Otherwise,%GDB%implements%watchpoints%in%software%by%single-
stepping%the%program%(executing%one%instruction%at%a%time).
•
A%catchpoint%specifies%that%execution%should%stop%when%a%particular%event%occurs.
The%GDB%documentation%and%command%set%often%use%the%word%breakpoint%as%a%generic%term%to%mean%all%three%kinds%of%program%stoppers.%In%
particular,%use%the%same%commands%to%enable,%disable,%and%remove%all%three.
GDB%applies%different%statuses%to%breakpoints%(and%watchpoints%and%catchpoints).%They%may%be%enabled,%which%means%that%the%program%stops%
when%the%breakpoint%is%hit%(or%fires);%disabled,%which%means%that%GDB%keeps%track%of%them%but%that%they%don't%affect%execution;%or%deleted,%which%
means%that%GDB%forgets%about%them%completely.%As%a%special%case,%breakpoints%can%be%enabled%only%once.%Such%a%breakpoint%stops%execution%
when%it%is%encountered,%then%becomes%disabled%(but%not%forgotten).
Breakpoints%may%have%conditions%associated%with%them.%When%execution%reaches%the%breakpoint,%GDB%checks%the%condition,%stopping%the%
program%only%if%the%condition%is%true.
Breakpoints%may%also%have%an%ignore%count,%which%is%a%count%of%how%many%times%GDB%should%ignore%the%breakpoint%when%it's%reached.%As%long%
as%a%breakpoint's%ignore%count%is%nonzero,%GDB%does%not%bother%checking%any%condition%associated%with%the%breakpoint.