# -*- coding:utf-8 -*-
import random
import config
import json
import os
geojson_suffix = '.geojson'
json_suffix = '.json'
def parse_geojson_to_json(input, output):
data = config.read_config(input, '')
result = []
features = data["features"]
for node in features:
result_node = {}
properties = node["properties"]
result_node["name"] = properties["name"]
result_node["value"] = properties["cp"]
result.append(result_node)
config.write_config(output, result)
def parse_geojson_to_json_1(input, output, startValue, endValue):
data = config.read_config(input, '')
result = []
features = data["features"]
for node in features:
result_node = {}
properties = node["properties"]
result_node["name"] = properties["name"]
result_node["value"] = random.randint(startValue, endValue)
result.append(result_node)
config.write_config(output, result)
def parse_geojson_to_json_2(input, output, startValue, endValue):
data = config.read_config(input, '')
result = []
try:
features = data["features"]
for node in features:
result_node = {}
properties = node["properties"]
try:
result_node["name"] = properties["name"]
result_node["value"] = [properties["cp"][0], properties["cp"][1], random.randint(startValue, endValue)]
result.append(result_node)
except Exception as e:
pass
config.write_config(output, result)
except Exception as e:
print("exception: %s %s" % input, e.args)
def parse_geojson_to_json_3(input, output, startValue, endValue):
data = config.read_config(input, '')
result = []
try:
features = data["features"]
for node in features:
result_node = {}
properties = node["properties"]
try:
result_node["name"] = properties["name"]
result_node["value"] = [properties["cp"][0], properties["cp"][1], random.randint(startValue, endValue)]
except Exception as e:
coordinatesValue = node["geometry"]["coordinates"][0][0]
coordLen = len(coordinatesValue)
randomKey = random.randint(0, coordLen)
result_node["value"] = [coordinatesValue[randomKey][0], coordinatesValue[randomKey][1], random.randint(startValue, endValue)]
result.append(result_node)
config.write_config(output, result)
except Exception as e:
print("exception: %s" % input)
def traverse_dir():
input_path = './geojson/shape-with-internal-borders'
output_path = 'json/'
file_list = os.listdir(input_path)
for node in file_list:
filename = node.split(".")[0] + json_suffix
inputfile = os.path.join(input_path, node)
outfile = os.path.join(output_path, filename)
parse_geojson_to_json_2(inputfile, outfile, 100, 500)
if __name__ == "__main__":
input_path = 'geojson/shape-with-internal-borders/'
output_path = 'json/neimenggu/'
filename = 'nei4_meng2_gu3_xi2_lin2_guo1_le4_meng2'
inputfile = input_path + filename + '.geojson'
outputfile = output_path + filename + '.json'
# parse_geojson_to_json(input, output)
# parse_geojson_to_json_1(input, output_1, 100, 500)
parse_geojson_to_json_2(inputfile, outputfile, 100, 500)
# traverse_dir()
没有合适的资源?快使用搜索试试~ 我知道了~
【源码】数据可视化:基于Echarts + GeoJson实现的地图视觉映射散点(气泡)组件【17】 - 内蒙古省.zip
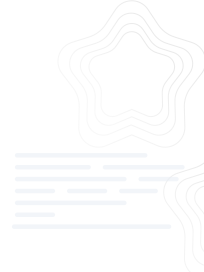
共46个文件
js:15个
json:12个
geojson:12个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
1、Echarts ,Python 实现的地图 视觉映射图,散点(气泡)图。 2、包括地图坐标经纬度geoJSON数据。 3、详细手册参考我的博文: https://blog.csdn.net/lildkdkdkjf/article/details/120165810 4、技术支持QQ微信同号: 6550523,欢迎来撩~
资源推荐
资源详情
资源评论
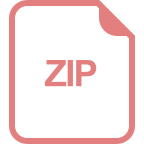
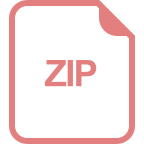
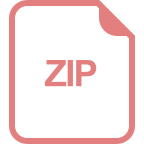
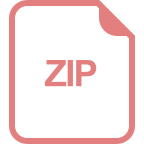
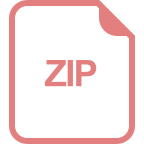
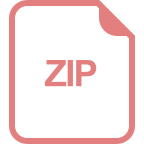
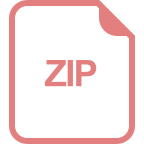
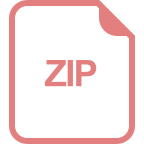
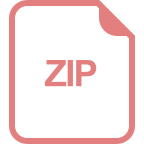
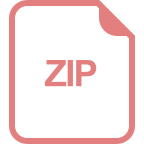
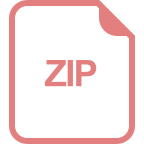
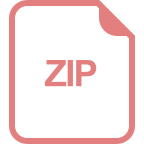
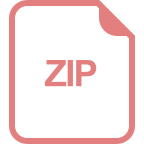
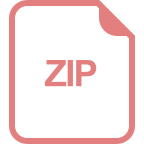
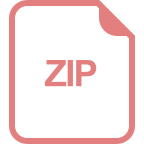
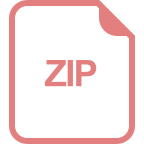
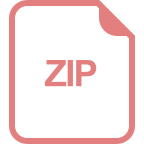
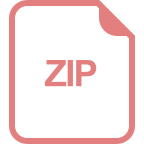
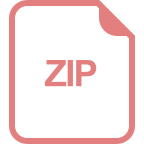
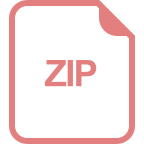
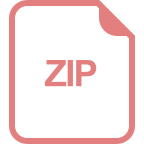
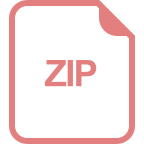
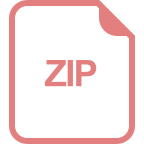
收起资源包目录


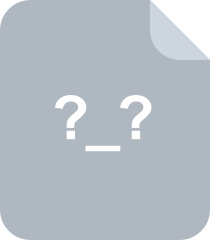
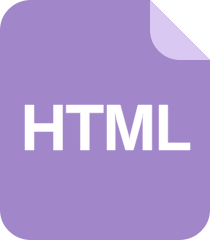

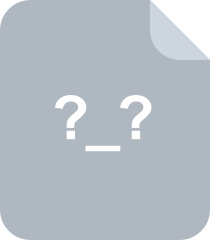


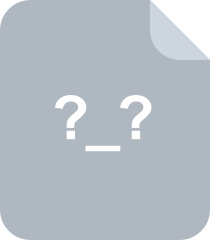
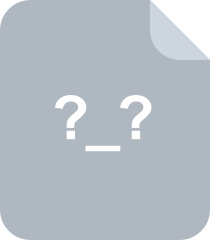
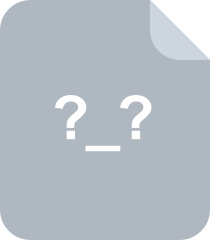
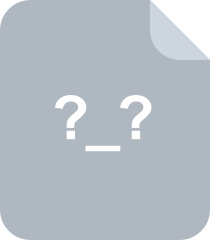
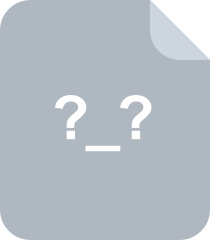
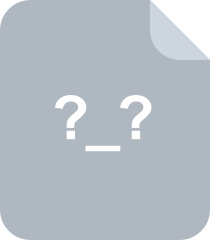
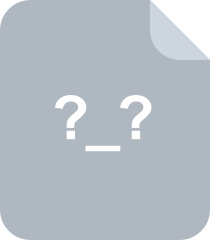
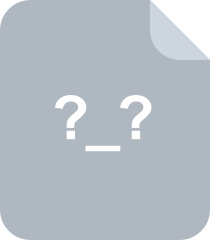
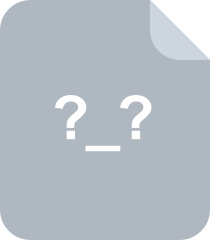
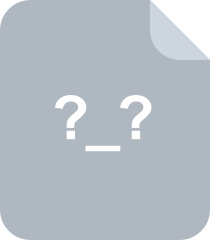
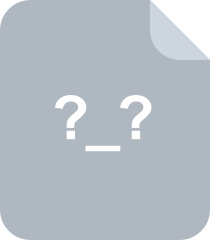
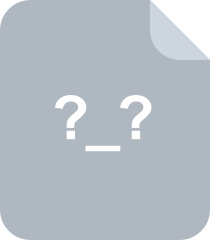

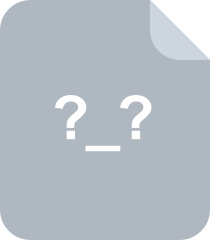


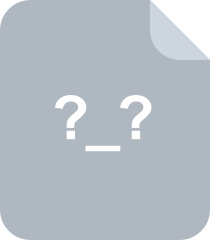
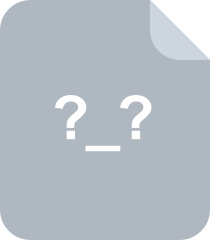
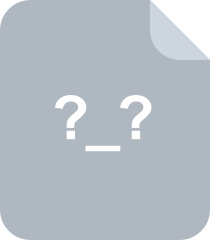
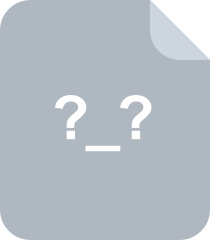
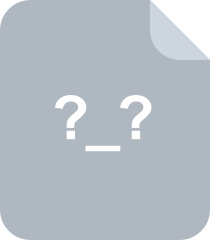
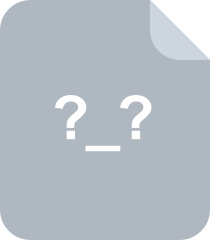
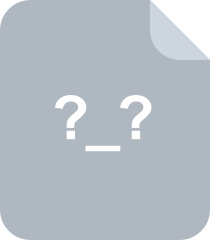
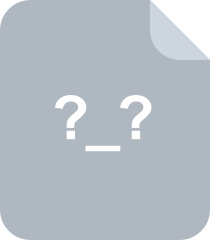
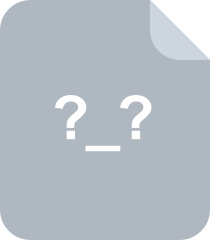
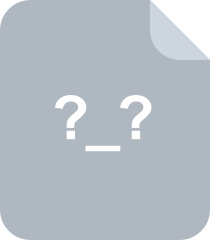
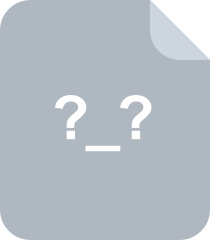
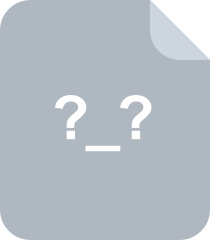
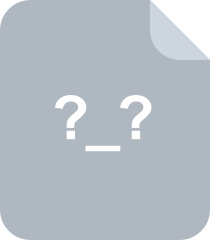
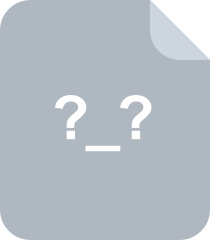
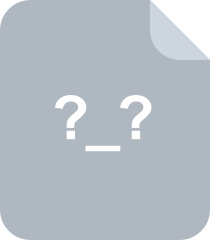
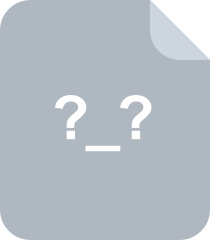

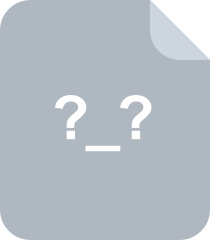
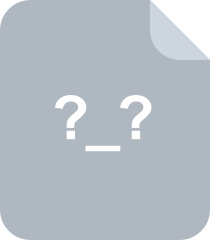
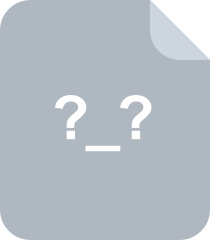
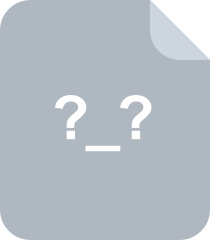
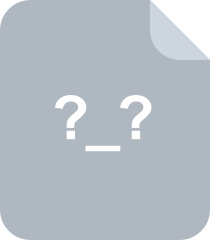
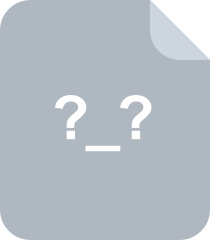
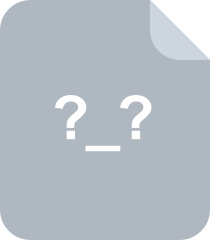
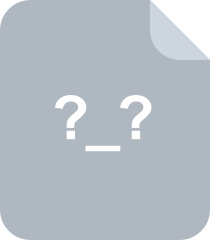
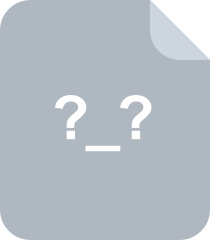
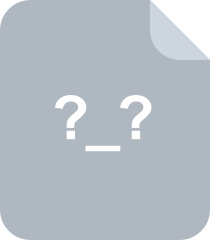
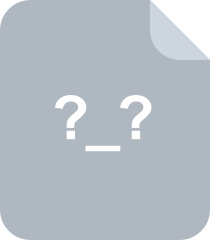
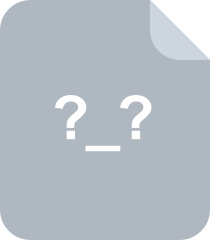

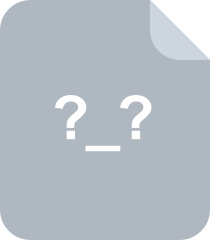
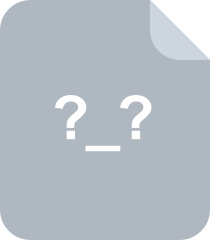
共 46 条
- 1
资源评论
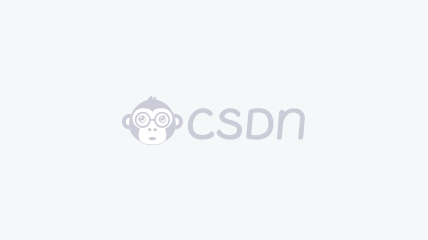
- js君2022-05-04用户下载后在一定时间内未进行评价,系统默认好评。

YYDataV软件开发
- 粉丝: 4w+
- 资源: 126

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

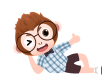
最新资源
- 【岗位说明】电工岗位说明书.doc
- 【岗位说明】电焊工岗位说明书.doc
- 【岗位说明】冬旺门窗岗位职责.doc
- 【岗位说明】各类气体押运工职务说明书.doc
- 【岗位说明】锅炉司炉岗位说明书.doc
- 【岗位说明】锅炉班长、司炉工、维修工岗位职责及任职条件.doc
- 【岗位说明】行车工岗位说明书.doc
- 【岗位说明】机械部岗位职责01.doc
- 【岗位说明】机械技术员岗位职责.doc
- 【岗位说明】金属门窗安全生产岗位职责制度.doc
- 【岗位说明】门窗厂班组长岗位职责.doc
- 【岗位说明】门窗厂车间主任岗位职责.doc
- 【岗位说明】铝合金门窗厂组织结构部门职能.doc
- 【岗位说明】门窗公司技术部部门职责.doc
- 【岗位说明】某五金厂生产部岗位说明书.doc
- 【岗位说明】某机械公司部门岗位职责说明书.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


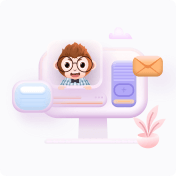
安全验证
文档复制为VIP权益,开通VIP直接复制
