package com.gr.geias.controller;
import com.gr.geias.dto.ClassGradeAndSpecialty;
import com.gr.geias.dto.CollegeAndPerson;
import com.gr.geias.dto.SpecialtyAndCollege;
import com.gr.geias.entity.ClassGrade;
import com.gr.geias.entity.College;
import com.gr.geias.entity.PersonInfo;
import com.gr.geias.entity.Specialty;
import com.gr.geias.enums.EnableStatusEnums;
import com.gr.geias.service.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.dao.DataIntegrityViolationException;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest;
import java.util.*;
/**
* @author maitentai
* @version 1.0
* @date 2020-03-08 19:52
*/
@RestController
@RequestMapping("/organizationcontroller")
public class OrganizationController {
@Autowired
CollegeService collegeService;
@Autowired
PersonInfoService personInfoService;
@Autowired
OrganizationNumService organizationNumService;
@Autowired
SpecialtyService specialtyService;
@Autowired
ClassGradeService classGradeService;
/**
* 返回学院"详细"信息 (2级权限)
*
* @param name
* @return
*/
@RequestMapping(value = "/getcollegelist", method = RequestMethod.GET)
public Map<String, Object> getCollegeList(@RequestParam(value = "name", required = false) String name) {
Map<String, Object> map = new HashMap<>(3);
List<College> college = collegeService.getCollege(null);
List<CollegeAndPerson> list = new ArrayList<>();
for (int i = 0; i < college.size(); i++) {
CollegeAndPerson collegeAndPerson = new CollegeAndPerson();
College college1 = college.get(i);
collegeAndPerson.setCollege(college1);
PersonInfo personById = personInfoService.getPersonById(college1.getAdminId());
collegeAndPerson.setPersonInfo(personById);
Integer integer = organizationNumService.getcollegeCount(college1.getCollegeId());
collegeAndPerson.setSum(integer);
list.add(collegeAndPerson);
}
map.put("success", true);
map.put("List", list);
return map;
}
/**
* 查询所有空闲的学院管理员 enable_Status=1 and college_id = null 权限 2
*
* @param collegeadminId
* @return
*/
@RequestMapping(value = "/getcollegeadmin", method = RequestMethod.GET)
public Map<String, Object> getCollegeAdmin(@RequestParam(value = "collegeadminId", required = false) Integer collegeadminId) {
Map<String, Object> map = new HashMap<>(3);
List<PersonInfo> collegePerson = personInfoService.getCollegePerson();
if (collegePerson.size() > 0) {
map.put("success", true);
map.put("collegePerson", collegePerson);
return map;
} else {
map.put("success", false);
map.put("errMsg", "请保证至少有一名学院管理身份的闲置用户存在");
return map;
}
}
/**
* 添加学院 权限 2
*
* @param collegeName
* @param personId
* @return
*/
@RequestMapping(value = "/addcollege", method = RequestMethod.GET)
public Map<String, Object> addCollege(@RequestParam("collegeName") String collegeName,
@RequestParam("personId") Integer personId) {
Map<String, Object> map = new HashMap<>(3);
if (collegeName == null || collegeName.equals("") || personId == null || personId == 0) {
map.put("success", false);
map.put("errMsg", "输入信息错误");
}
College college = new College();
college.setAdminId(personId);
college.setCollegeName(collegeName);
college.setCreateTime(new Date());
try {
Boolean aBoolean = collegeService.addCollege(college);
map.put("success", true);
} catch (Exception e) {
map.put("success", false);
map.put("errMsg", e.getMessage());
}
return map;
}
/**
* 更新学院 权限2
*
* @param collegeName
* @param personId
* @param collegeId
* @return
*/
@RequestMapping(value = "/updatecollege", method = RequestMethod.GET)
public Map<String, Object> updateCollege(@RequestParam(value = "collegeName", required = false) String collegeName,
@RequestParam(value = "personId", required = false) Integer personId,
@RequestParam("collegeId") Integer collegeId) {
Map<String, Object> map = new HashMap<>(3);
if (personId == null || personId == 0) {
personId = null;
}
College college = new College();
college.setCollegeId(collegeId);
college.setCollegeName(collegeName);
college.setAdminId(personId);
try {
Boolean aBoolean = collegeService.updateCollege(college);
if (aBoolean) {
map.put("success", true);
}
} catch (RuntimeException e) {
map.put("success", false);
map.put("errMsg", e.getMessage());
}
return map;
}
/**
* 删除学院 权限2
*
* @param collegeId
* @return
*/
@RequestMapping(value = "/delcollege", method = RequestMethod.GET)
public Map<String, Object> delCollege(@RequestParam("collegeId") Integer collegeId) {
Map<String, Object> map = new HashMap<>(3);
if (collegeId != null && collegeId != null) {
try {
collegeService.delCollege(collegeId);
map.put("success", true);
} catch (RuntimeException e) {
map.put("success", false);
map.put("errMsg", e.getMessage());
}
} else {
map.put("success", false);
map.put("errMsg", "信息错误");
}
return map;
}
/**
* 根据用户权限获取学院列表(简化信息) 权限 1,2
*
* @param request
* @return
*/
@RequestMapping(value = "/getcollegeinit", method = RequestMethod.GET)
public Map<String, Object> getCollegeinit(HttpServletRequest request) {
Map<String, Object> map = new HashMap<>(3);
PersonInfo person = (PersonInfo) request.getSession().getAttribute("person");
List<College> college = null;
if (person.getEnableStatus() == EnableStatusEnums.schoolmaster.getState()) {
college = collegeService.getCollege(null);
}
if (person.getEnableStatus() == EnableStatusEnums.PREXY.getState()) {
college = collegeService.getCollege(person.getPersonId());
}
if (college != null) {
map.put("success", true);
map.put("collegeList", college);
} else {
map.put("success", false);
map.put("errMsg", "查找学院信息错误");
}
return map;
}
/**
* 获取专业详细列表 权限 1,2
*
* @param collegeId
* @param request
* @return
*/
@RequestMapping(value = "/getspecialty", method = RequestMethod.GET)
public Map<String, Object> getSpecialty(@RequestParam(value = "collegeId", required = false) Integer collegeId,
HttpServletRequest request) {
Map<String, Object> map = new HashMap<>(3);
PersonInfo person = (PersonInfo) request.getSession().getAttribute("person");
List<Specialty> specialtyList = null;
College college = null;
if (person.getEnableStatus() == EnableStatusEnums.PREXY.getState()) {
List<College> college
没有合适的资源?快使用搜索试试~ 我知道了~
基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统.zip
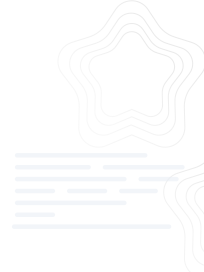
共251个文件
gif:75个
java:75个
html:29个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 60 浏览量
2023-10-16
13:11:12
上传
评论 1
收藏 1.29MB ZIP 举报
温馨提示
基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统 基于SpringBoot+Mybatis+mysql的毕业生就业信息分析系统
资源推荐
资源详情
资源评论
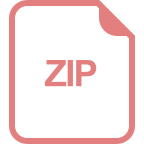
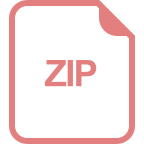
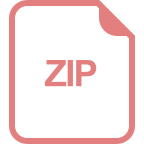
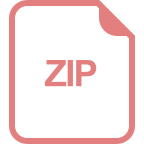
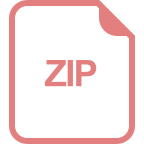
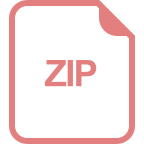
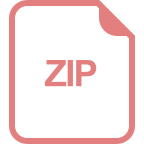
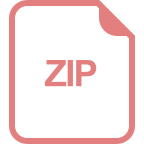
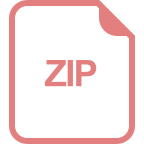
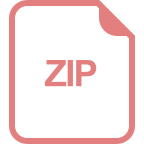
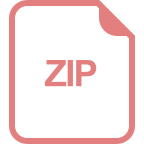
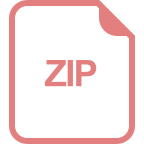
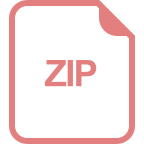
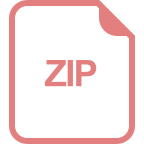
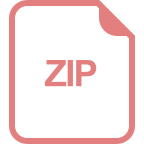
收起资源包目录

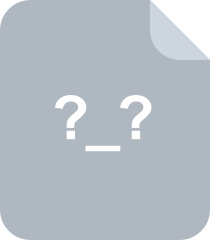
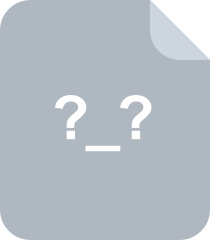
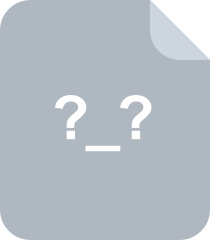
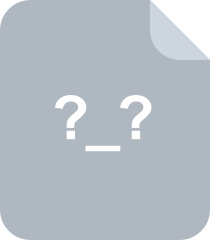
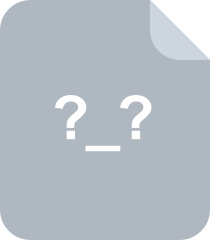
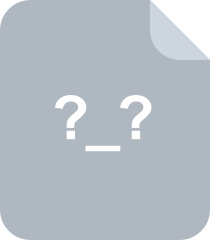
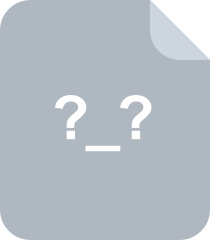
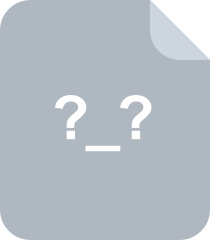
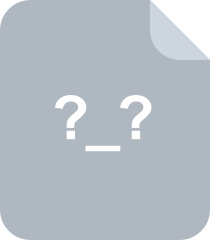
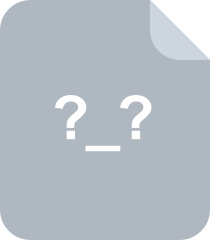
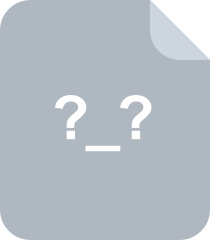
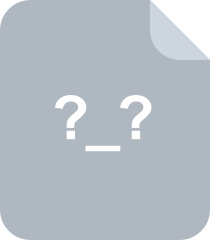
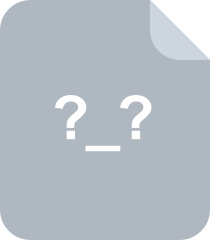
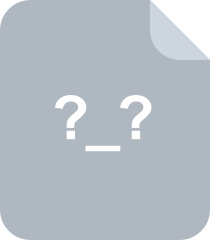
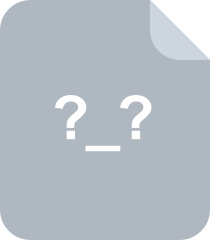
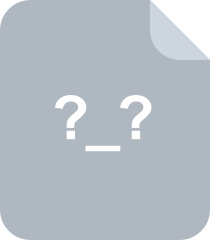
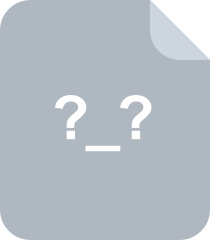
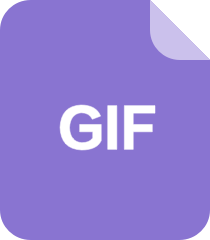
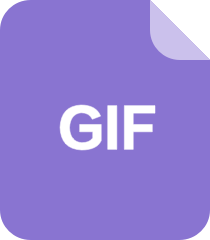
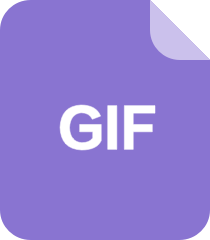
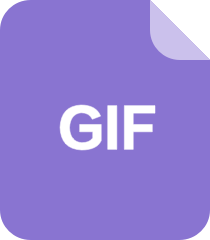
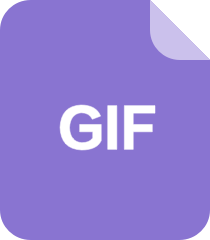
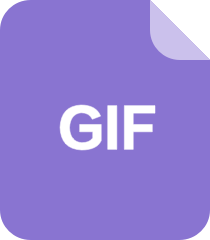
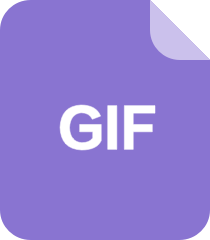
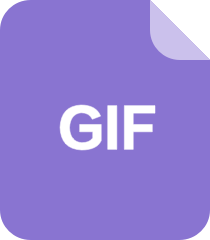
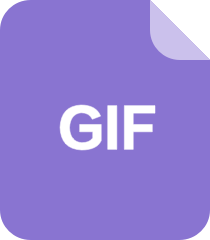
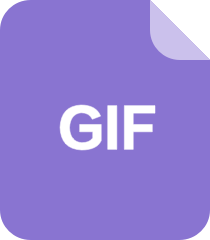
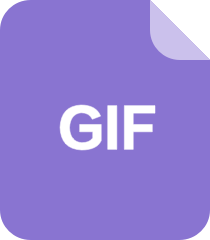
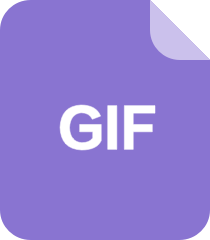
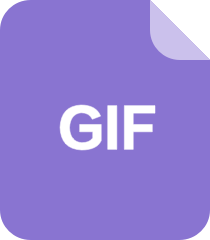
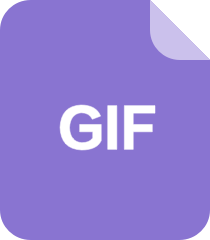
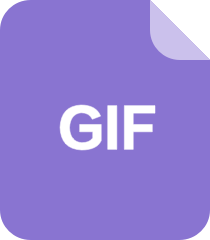
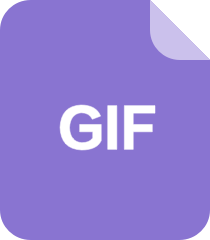
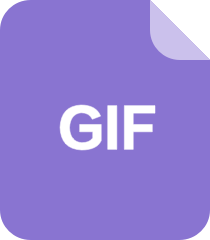
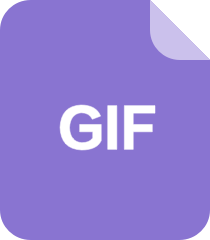
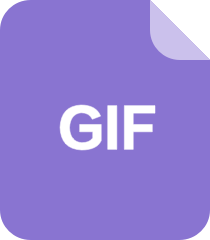
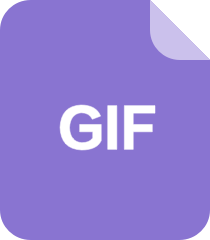
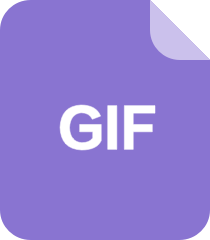
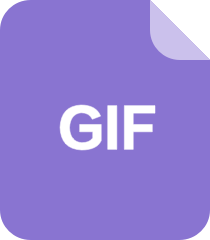
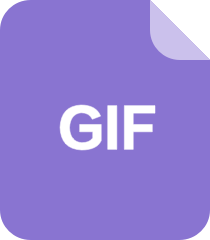
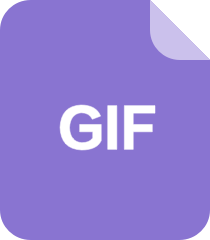
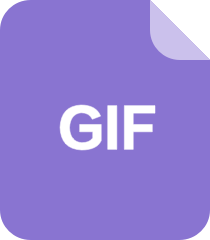
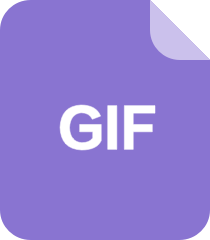
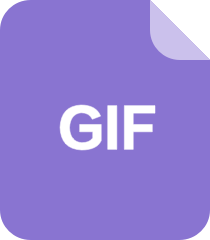
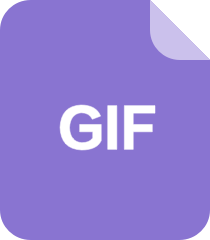
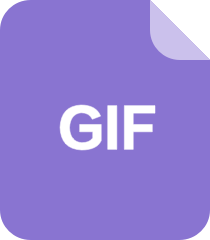
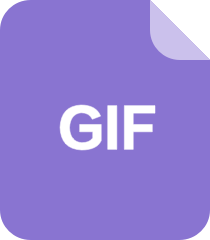
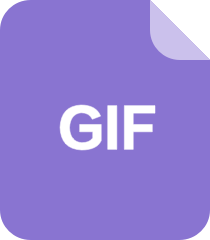
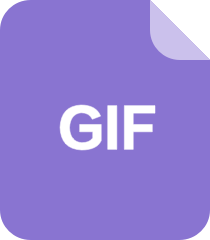
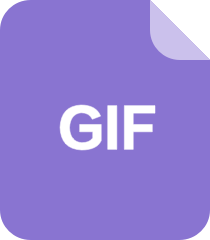
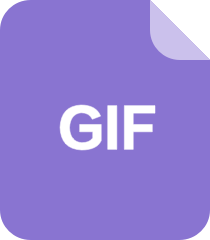
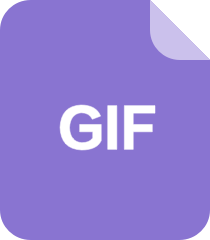
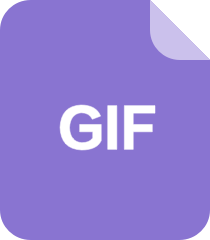
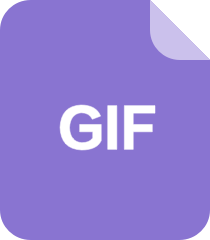
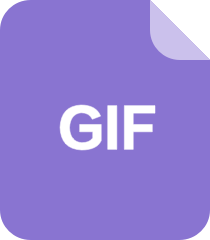
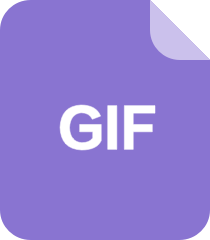
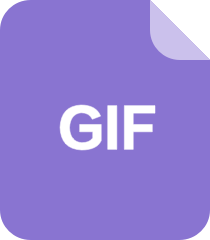
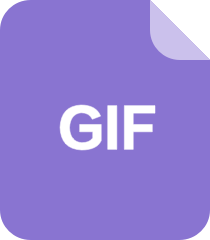
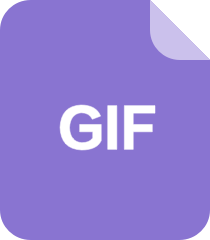
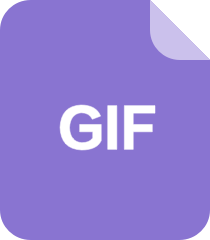
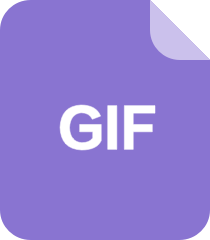
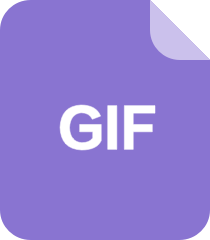
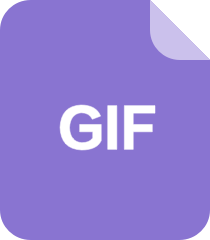
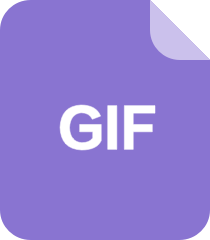
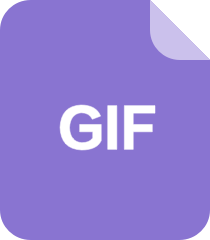
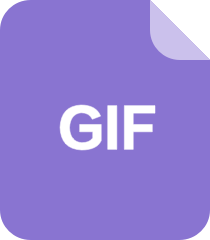
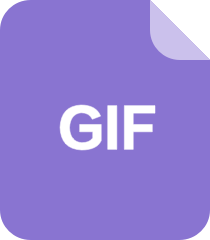
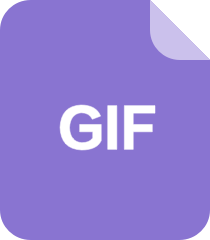
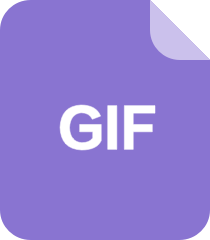
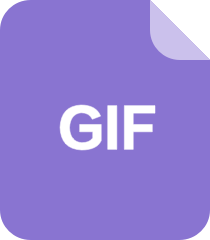
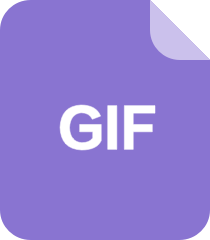
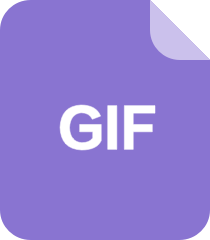
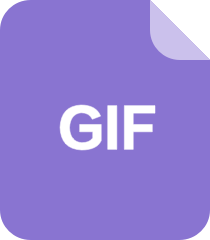
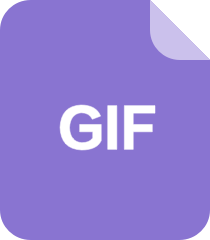
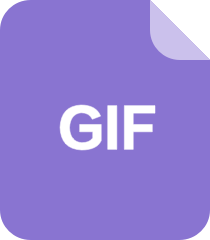
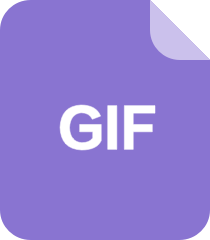
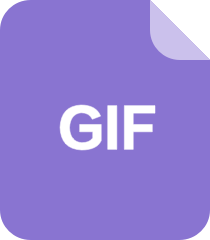
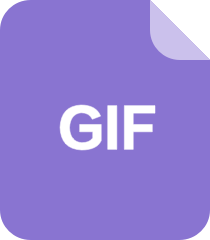
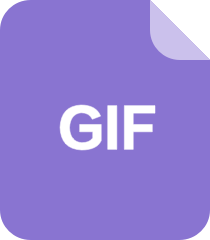
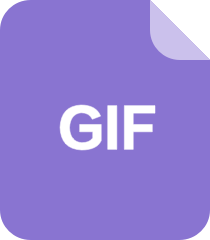
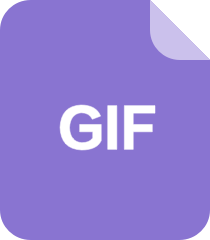
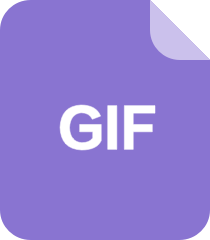
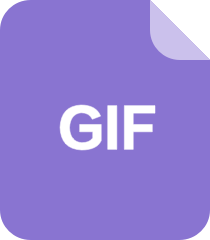
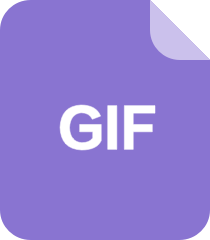
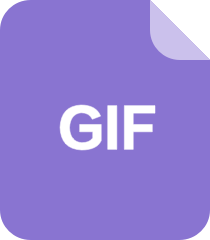
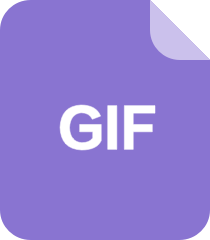
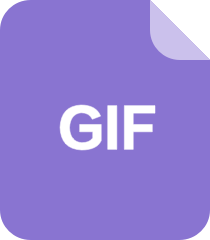
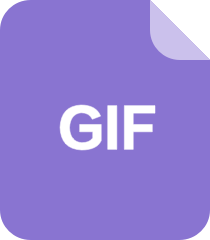
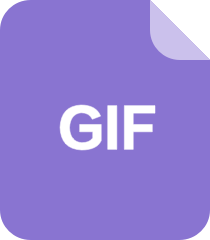
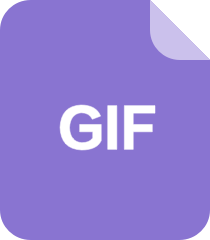
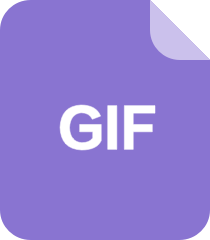
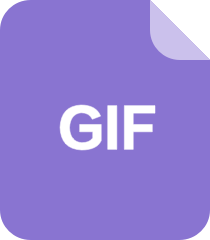
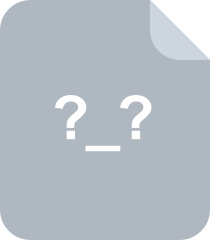
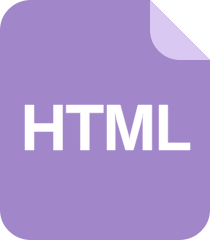
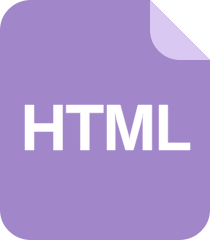
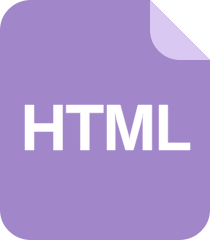
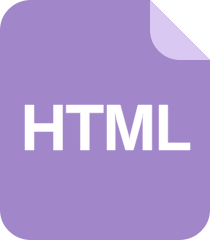
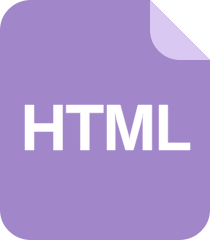
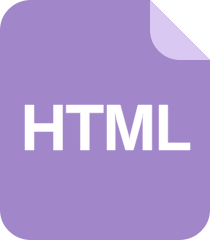
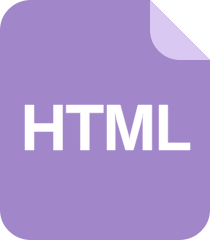
共 251 条
- 1
- 2
- 3
资源评论
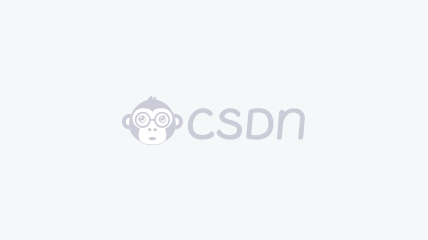


辣椒种子
- 粉丝: 4135
- 资源: 5738

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

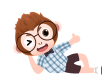
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


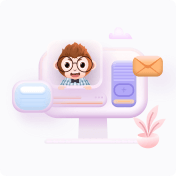
安全验证
文档复制为VIP权益,开通VIP直接复制
