package com.wisely.ch9_2.batch;
import javax.sql.DataSource;
import org.springframework.batch.core.Job;
import org.springframework.batch.core.Step;
import org.springframework.batch.core.configuration.annotation.EnableBatchProcessing;
import org.springframework.batch.core.configuration.annotation.JobBuilderFactory;
import org.springframework.batch.core.configuration.annotation.StepBuilderFactory;
import org.springframework.batch.core.configuration.annotation.StepScope;
import org.springframework.batch.core.launch.support.RunIdIncrementer;
import org.springframework.batch.core.launch.support.SimpleJobLauncher;
import org.springframework.batch.core.repository.JobRepository;
import org.springframework.batch.core.repository.support.JobRepositoryFactoryBean;
import org.springframework.batch.item.ItemProcessor;
import org.springframework.batch.item.ItemReader;
import org.springframework.batch.item.ItemWriter;
import org.springframework.batch.item.database.BeanPropertyItemSqlParameterSourceProvider;
import org.springframework.batch.item.database.JdbcBatchItemWriter;
import org.springframework.batch.item.file.FlatFileItemReader;
import org.springframework.batch.item.file.mapping.BeanWrapperFieldSetMapper;
import org.springframework.batch.item.file.mapping.DefaultLineMapper;
import org.springframework.batch.item.file.transform.DelimitedLineTokenizer;
import org.springframework.batch.item.validator.Validator;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.io.ClassPathResource;
import org.springframework.transaction.PlatformTransactionManager;
import com.wisely.ch9_2.domain.Person;
@Configuration
@EnableBatchProcessing
public class TriggerBatchConfig {
@Bean
@StepScope
public FlatFileItemReader<Person> reader(@Value("#{jobParameters['input.file.name']}") String pathToFile) throws Exception {
FlatFileItemReader<Person> reader = new FlatFileItemReader<Person>(); //1
reader.setResource(new ClassPathResource(pathToFile)); //2
reader.setLineMapper(new DefaultLineMapper<Person>() {{ //3
setLineTokenizer(new DelimitedLineTokenizer() {{
setNames(new String[] { "name","age", "nation" ,"address"});
}});
setFieldSetMapper(new BeanWrapperFieldSetMapper<Person>() {{
setTargetType(Person.class);
}});
}});
return reader;
}
@Bean
public ItemProcessor<Person, Person> processor() {
CsvItemProcessor processor = new CsvItemProcessor(); //1
processor.setValidator(csvBeanValidator()); //2
return processor;
}
@Bean
public ItemWriter<Person> writer(DataSource dataSource) {//1
JdbcBatchItemWriter<Person> writer = new JdbcBatchItemWriter<Person>(); //2
writer.setItemSqlParameterSourceProvider(new BeanPropertyItemSqlParameterSourceProvider<Person>());
String sql = "insert into person " + "(id,name,age,nation,address) "
+ "values(hibernate_sequence.nextval, :name, :age, :nation,:address)";
writer.setSql(sql); //3
writer.setDataSource(dataSource);
return writer;
}
@Bean
public JobRepository jobRepository(DataSource dataSource, PlatformTransactionManager transactionManager)
throws Exception {
JobRepositoryFactoryBean jobRepositoryFactoryBean = new JobRepositoryFactoryBean();
jobRepositoryFactoryBean.setDataSource(dataSource);
jobRepositoryFactoryBean.setTransactionManager(transactionManager);
jobRepositoryFactoryBean.setDatabaseType("oracle");
return jobRepositoryFactoryBean.getObject();
}
@Bean
public SimpleJobLauncher jobLauncher(DataSource dataSource, PlatformTransactionManager transactionManager)
throws Exception {
SimpleJobLauncher jobLauncher = new SimpleJobLauncher();
jobLauncher.setJobRepository(jobRepository(dataSource, transactionManager));
return jobLauncher;
}
@Bean
public Job importJob(JobBuilderFactory jobs, Step s1) {
return jobs.get("importJob")
.incrementer(new RunIdIncrementer())
.flow(s1) //1
.end()
.listener(csvJobListener()) //2
.build();
}
@Bean
public Step step1(StepBuilderFactory stepBuilderFactory, ItemReader<Person> reader, ItemWriter<Person> writer,
ItemProcessor<Person,Person> processor) {
return stepBuilderFactory
.get("step1")
.<Person, Person>chunk(65000) //1
.reader(reader) //2
.processor(processor) //3
.writer(writer) //4
.build();
}
@Bean
public CsvJobListener csvJobListener() {
return new CsvJobListener();
}
@Bean
public Validator<Person> csvBeanValidator() {
return new CsvBeanValidator<Person>();
}
}

StrugglingBean
- 粉丝: 22
- 资源: 9
最新资源
- java毕设项目之ssm基于Vue.js的在线购物系统的设计与实现+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm汽车养护管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm简易版营业厅宽带系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm绿色农产品推广应用网站+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm人事管理信息系统+jsp(完整前后端+说明文档+mysql+lw).zip
- 自考04741《计算机网络原理》试题及答案2016-2018
- java毕设项目之ssm社区管理与服务的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm社区文化宣传网站+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm实验室耗材管理系统设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网络游戏公司官方平台设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm蜀都天香酒楼的网站设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网上医院预约挂号系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网上花店设计+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm网上服装销售系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm小型企业办公自动化系统的设计和开发+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm物流管理系统设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


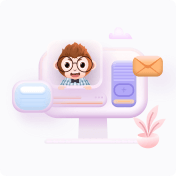