//---------------------------------------------------------
// OPC Custom 2.0 Sample
// Asynchronous IO with MFC and ATL
// (c) Siemens AG 2000
//---------------------------------------------------------
//
// OPCDA_AsyncDlg.cpp : implementation file of the dialog class
// all OPC calls are located here
//
#include "stdafx.h"
#include "pre_opc.h" // for OPC: include MFC, ATL and OPC header files
#include "OPCDA_Async.h" // include class declaration for application class
#include "OPCDA_AsyncDlg.h" // include class declaration of dialog class
#include "Callback.h" // for OPC: include class declaration fpr this callback class
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
//// Used by GetErrorString; Language code ////
#define LOCALE_ID 0x409 // Code 0x409 = ENGLISH
const LPWSTR szItemID0 = L"S7:[DEMO]MB1"; // this item must be readable and writeablein this sample
const LPWSTR szItemID1 = L"S7:[DEMO]MW3"; // this item must be readable in this sample
const DWORD vtDataTypeItem0 = VT_EMPTY; // canonical datatype
const DWORD vtDataTypeItem1 = VT_UI2; // unsigned integer 2 Bytes
/////////////////////////////////////////////////////////////////////////////
// CAboutDlg dialog used for App About
class CAboutDlg : public CDialog
{
public:
CAboutDlg();
// Dialog Data
//{{AFX_DATA(CAboutDlg)
enum { IDD = IDD_ABOUTBOX };
//}}AFX_DATA
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(CAboutDlg)
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
//}}AFX_VIRTUAL
// Implementation
protected:
//{{AFX_MSG(CAboutDlg)
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD)
{
//{{AFX_DATA_INIT(CAboutDlg)
//}}AFX_DATA_INIT
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CAboutDlg)
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)
//{{AFX_MSG_MAP(CAboutDlg)
// No message handlers
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// COPCDA_AsyncDlg dialog
//
// class constructor: initialisation of dialog variables
//
COPCDA_AsyncDlg::COPCDA_AsyncDlg(CWnd* pParent /*=NULL*/)
: CDialog(COPCDA_AsyncDlg::IDD, pParent)
{
//{{AFX_DATA_INIT(COPCDA_AsyncDlg)
m_ReadValue = 0;
m_QualityRead = _T("");
m_TimeStampRead = _T("");
m_WriteResult = _T("");
m_WriteVal = 0;
m_bChkActivateGroup = FALSE;
m_Quality0 = _T("");
m_TimeStamp0 = _T("");
m_Value0 = _T("");
m_Quality1 = _T("");
m_TimeStamp1 = _T("");
m_Value1 = _T("");
m_szItemID0 = szItemID0; //_T("");
m_szItemID1 = szItemID1; // _T("");
//}}AFX_DATA_INIT
m_hIcon = AfxGetApp()->LoadIcon(IDI_ICONOPC);
}
//
// MFC Dialog Data Exchange handler: Maps control IDs to variables
//
void COPCDA_AsyncDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(COPCDA_AsyncDlg)
DDX_Control(pDX, IDC_CHECK_ACTIVATEGROUP, m_CtrlChkActive);
DDX_Control(pDX, IDC_BUTTON_WRITE, m_CtrlWrite);
DDX_Control(pDX, IDC_BUTTON_STOP, m_CtrlStop);
DDX_Control(pDX, IDC_BUTTON_START, m_CtrlStart);
DDX_Control(pDX, IDC_BUTTON_READ, m_CtrlRead);
DDX_Text(pDX, IDC_EDIT_READVAL, m_ReadValue);
DDX_Text(pDX, IDC_EDIT_QUALITY, m_QualityRead);
DDX_Text(pDX, IDC_EDIT_TIMESTAMP, m_TimeStampRead);
DDX_Text(pDX, IDC_EDIT_WRITERESULT, m_WriteResult);
DDX_Text(pDX, IDC_EDIT_WRITEVAL, m_WriteVal);
DDX_Check(pDX, IDC_CHECK_ACTIVATEGROUP, m_bChkActivateGroup);
DDX_Text(pDX, IDC_EDIT_ONDATA_QU1, m_Quality0);
DDX_Text(pDX, IDC_EDIT_ONDATA_TIMEST1, m_TimeStamp0);
DDX_Text(pDX, IDC_EDIT_ONDATA_VAL1, m_Value0);
DDX_Text(pDX, IDC_EDIT_ONDATA_QU2, m_Quality1);
DDX_Text(pDX, IDC_EDIT_ONDATA_TIMEST2, m_TimeStamp1);
DDX_Text(pDX, IDC_EDIT_ONDATA_VAL2, m_Value1);
DDX_Text(pDX, IDC_STATIC_ITEMID0, m_szItemID0);
DDX_Text(pDX, IDC_STATIC_ITEMID1, m_szItemID1);
//}}AFX_DATA_MAP
}
//
// MFC Message map: maps control ID to handler routines
//
BEGIN_MESSAGE_MAP(COPCDA_AsyncDlg, CDialog)
//{{AFX_MSG_MAP(COPCDA_AsyncDlg)
ON_WM_SYSCOMMAND()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_BN_CLICKED(IDC_BUTTON_START, OnButtonStart)
ON_BN_CLICKED(IDC_BUTTON_READ, OnButtonRead)
ON_BN_CLICKED(IDC_BUTTON_WRITE, OnButtonWrite)
ON_BN_CLICKED(IDC_BUTTON_STOP, OnButtonStop)
ON_BN_CLICKED(IDC_CHECK_ACTIVATEGROUP, OnCheckActivategroup)
ON_WM_DESTROY()
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// COPCDA_AsyncDlg message handlers
//
//
// OnInitDialog: being called, when dialog is opened the first time
//
BOOL COPCDA_AsyncDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Add "About..." menu item to system menu.
// IDM_ABOUTBOX must be in the system command range.
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// for OPC: remainder of this method
// initialise interface pointer
m_pIOPCServer = NULL;
m_pIOPCItemMgt = NULL;
m_pIOPCGroupStateMgt = NULL;
m_dwAdvise = 0;
// Disable Buttons until Sample is started to avoid operation mistakes
m_CtrlStop.EnableWindow(FALSE);
m_CtrlRead.EnableWindow(FALSE);
m_CtrlWrite.EnableWindow(FALSE);
m_CtrlChkActive.EnableWindow(FALSE);
return TRUE;
}
//
// OnSysCommand: being called, when system command "about" has been invoked
//
void COPCDA_AsyncDlg::OnSysCommand(UINT nID, LPARAM lParam)
{
if ((nID & 0xFFF0) == IDM_ABOUTBOX)
{
CAboutDlg dlgAbout;
dlgAbout.DoModal();
}
else
{
CDialog::OnSysCommand(nID, lParam);
}
}
//
// OnPaint: MFC handler, being called, when Windows or an application makes a
// request to repaint a portion of an application�s window
//
void COPCDA_AsyncDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, (WPARAM) dc.GetSafeHdc(), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
HCURSOR COPCDA_AsyncDlg::OnQueryDragIcon()
{
return (HCURSOR) m_hIcon;
}
//
// OnButtonStart: handler is being called, when button "Start Sample" has been pressed
//
// This handler is for OPC:
// - initializes COM
// - creates an OPC server object
// - adds an OPC group
// - adds 2 items to this group
// - establishes the callback
void COPCDA_AsyncDlg::OnButtonStart()
{
HRESULT r1;
CLSID clsid;
LONG TimeBias = 0;
FLOAT PercentDeadband = 0.0;
DWORD RevisedUpdateRate;
LPWSTR ErrorStr1,ErrorStr2;
CString szErrorText;
m_pItemResult = NULL;
// Initialize the COM library
r1 = CoInitialize(NULL);
if (r1 != S_OK)
{ if (r1 == S_FALSE)
{ MessageBox("COM Library already initialized",
"Error CoInitialize()", MB_OK+MB_ICONEXCLAMATION);
}
else
{ szErrorText.Format("Initialisation of COM Library failed. ErrorCode= %4x", r1);
MessageBox(szErrorText,"Error CoInitialize()", MB_OK+MB_ICONERROR);
SendMessage(WM_CLOSE);
return;
}
}
// Given a ProgID, this looks up the associated CLSID in the re
没有合适的资源?快使用搜索试试~ 我知道了~
VC编写的OPC客户端程序源代码(异步通讯)
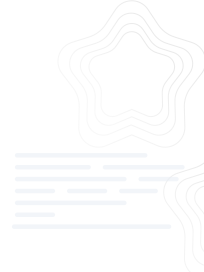
共42个文件
h:6个
cpp:5个
obj:5个


温馨提示
PC是Object Linking and Embedding(OLE)for Process Control的缩写,它是微软公司的对象链接和嵌入技术在过程控制方面的应用。OPC以OLE/COM/DCOM技术为基础,采用客户/服务器模式,为工业自动化软件面向对象的开发提供了统一的标准,这个标准定义了应用Microsoft操作系统在基于PC的客户机之间交换自动化实时数据的方法。采用这项标准后,硬件开发商将取代软件开发商为自己的硬件产品开发统一的OPC接口程序,而软件开发者可免除开发驱动程序的工作,充分发挥自己的特长,把更多的精力投入到其核心产品的开发上。这样不但可避免开发的重复性,也提高了系统的开放性和可互操作性。
资源推荐
资源详情
资源评论
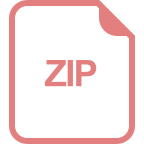
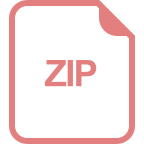
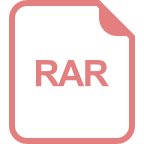
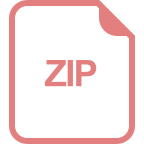
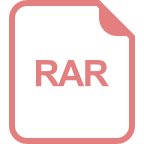
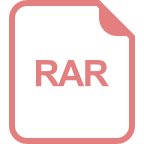
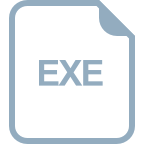
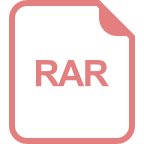
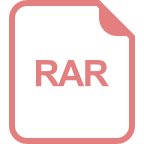
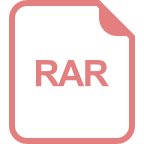
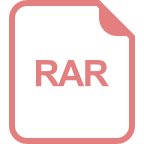
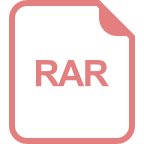
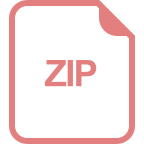
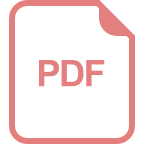
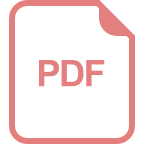
收起资源包目录


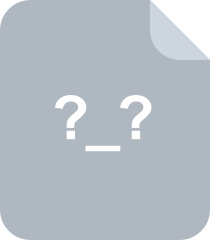
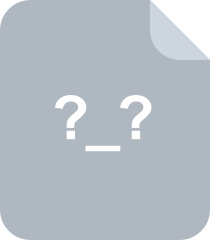
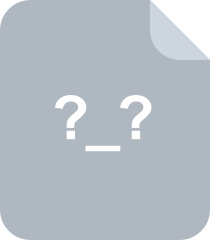
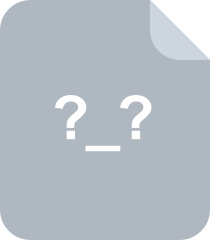
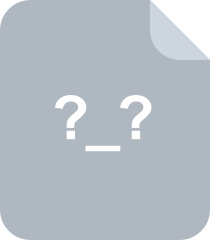
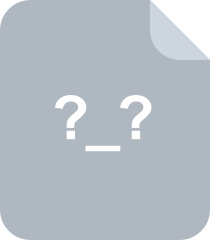
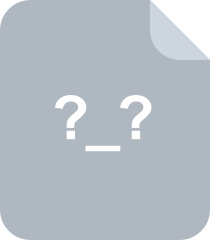
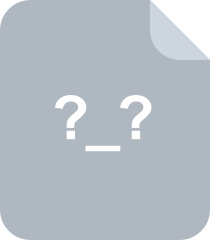
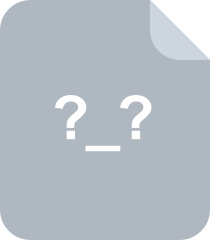
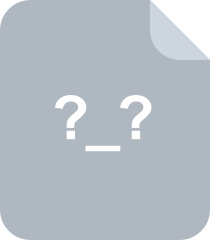
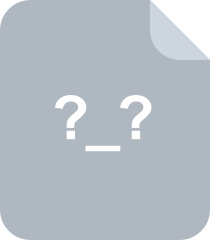
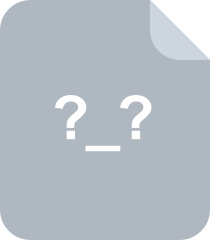
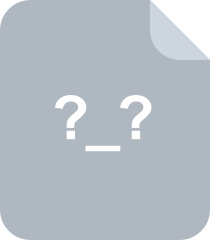

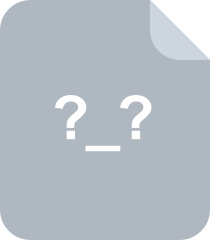
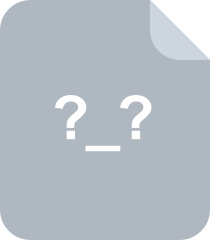
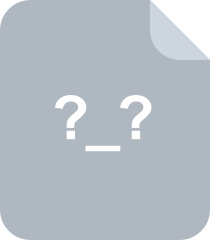
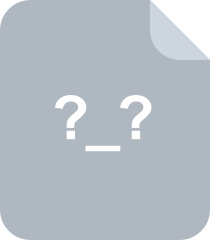
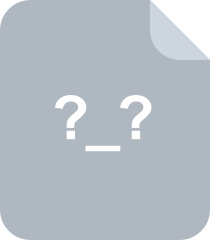
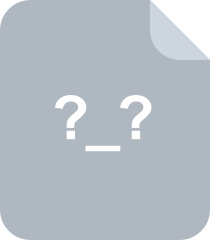
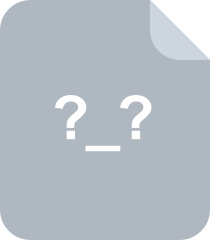
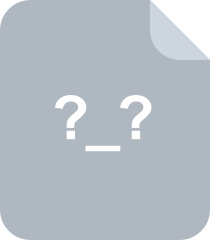
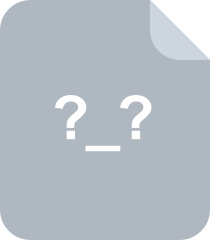
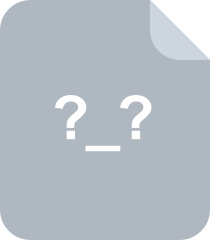
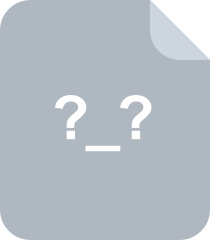
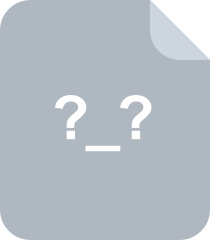
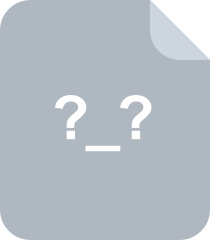
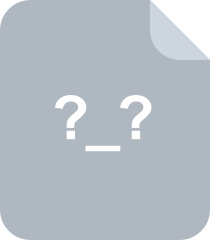
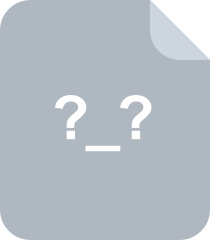
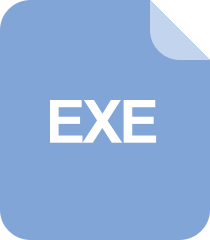
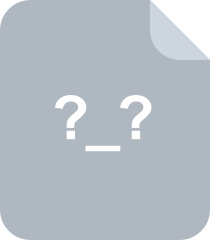
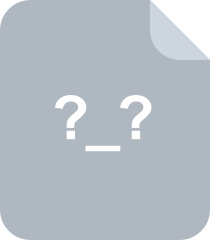
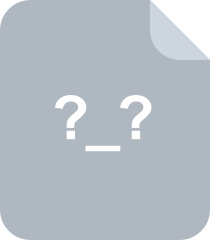
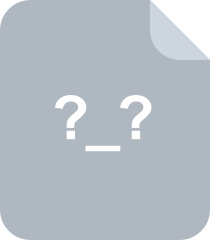
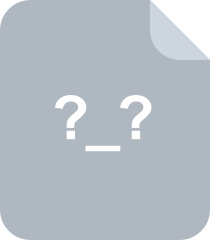

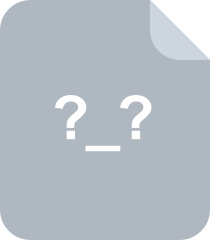
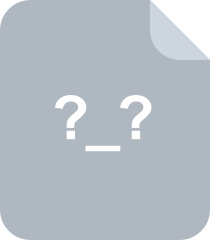
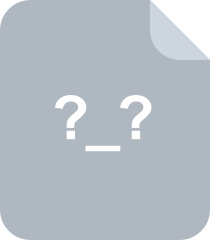
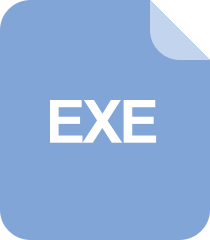
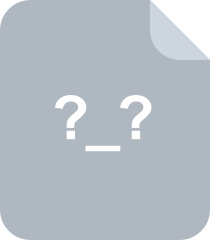
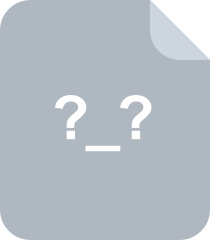
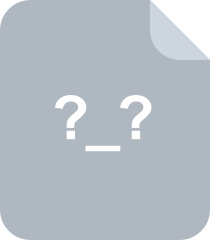
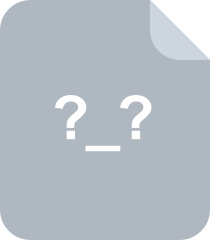
共 42 条
- 1

libo_jeason
- 粉丝: 0
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

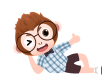
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


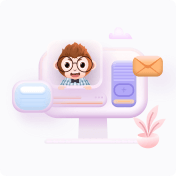
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页