/*******************************************************************************
* Copyright (c) 2009-2011 Luaj.org. All rights reserved.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
package org.luaj.vm2;
/**
* Base class for all concrete lua type values.
* <p>
* Establishes base implementations for all the operations on lua types.
* This allows Java clients to deal essentially with one type for all Java values, namely {@link LuaValue}.
* <p>
* Constructors are provided as static methods for common Java types, such as
* {@link LuaValue#valueOf(int)} or {@link LuaValue#valueOf(String)}
* to allow for instance pooling.
* <p>
* Constants are defined for the lua values
* {@link #NIL}, {@link #TRUE}, and {@link #FALSE}.
* A constant {@link #NONE} is defined which is a {@link Varargs} list having no values.
* <p>
* Operations are performed on values directly via their Java methods.
* For example, the following code divides two numbers:
* <pre> {@code
* LuaValue a = LuaValue.valueOf( 5 );
* LuaValue b = LuaValue.valueOf( 4 );
* LuaValue c = a.div(b);
* } </pre>
* Note that in this example, c will be a {@link LuaDouble}, but would be a {@link LuaInteger}
* if the value of a were changed to 8, say.
* In general the value of c in practice will vary depending on both the types and values of a and b
* as well as any metatable/metatag processing that occurs.
* <p>
* Field access and function calls are similar, with common overloads to simplify Java usage:
* <pre> {@code
* LuaValue globals = JsePlatform.standardGlobals();
* LuaValue sqrt = globals.get("math").get("sqrt");
* LuaValue print = globals.get("print");
* LuaValue d = sqrt.call( a );
* print.call( LuaValue.valueOf("sqrt(5):"), a );
* } </pre>
* <p>
* To supply variable arguments or get multiple return values, use
* {@link invoke( Varargs )} or {@link invokemethod( LuaValue , Varargs )} methods:
* <pre> {@code
* LuaValue modf = globals.get("math").get("modf");
* Varargs r = modf.invoke( d );
* print.call( r.arg(1), r.arg(2) );
* } </pre>
* <p>
* To load and run a script, {@link LoadState} is used:
* <pre> {@code
* LoadState.load( new FileInputStream("main.lua"), "main.lua", globals ).call();
* } </pre>
* <p>
* although {@code require} could also be used:
* <pre> {@code
* globals.get("require").call(LuaValue.valueOf("main"));
* } </pre>
* For this to work the file must be in the current directory, or in the class path,
* dependening on the platform.
* See {@link JsePlatform} and {@link JmePlatform} for details.
* <p>
* In general a {@link LuaError} may be thrown on any operation when the
* types supplied to any operation are illegal from a lua perspective.
* Examples could be attempting to concatenate a NIL value, or attempting arithmetic
* on values that are not number.
* <p>
* There are several methods for preinitializing tables, such as:
* <ul>
* <li>{@link #listOf(LuaValue[])} for unnamed elements</li>
* <li>{@link #tableOf(LuaValue[])} for named elements</li>
* <li>{@link #tableOf(LuaValue[], LuaValue[], Varargs)} for mixtures</li>
* </ul>
* <p>
* Predefined constants exist for the standard lua type constants
* {@link TNIL}, {@link TBOOLEAN}, {@link TLIGHTUSERDATA}, {@link TNUMBER}, {@link TSTRING},
* {@link TTABLE}, {@link TFUNCTION}, {@link TUSERDATA}, {@link TTHREAD},
* and extended lua type constants
* {@link TINT}, {@link TNONE}, {@link TVALUE}
* <p>
* Predefined constants exist for all strings used as metatags:
* {@link INDEX}, {@link NEWINDEX}, {@link CALL}, {@link MODE}, {@link METATABLE},
* {@link ADD}, {@link SUB}, {@link DIV}, {@link MUL}, {@link POW},
* {@link MOD}, {@link UNM}, {@link LEN}, {@link EQ}, {@link LT},
* {@link LE}, {@link TOSTRING}, and {@link CONCAT}.
*
* @see JsePlatform
* @see JmePlatform
* @see LoadState
* @see Varargs
*/
abstract
public class LuaValue extends Varargs {
/**
* Type enumeration constant for lua numbers that are ints, for compatibility with lua 5.1 number patch only
*/
public static final int TINT = (-2);
/**
* Type enumeration constant for lua values that have no type, for example weak table entries
*/
public static final int TNONE = (-1);
/**
* Type enumeration constant for lua nil
*/
public static final int TNIL = 0;
/**
* Type enumeration constant for lua booleans
*/
public static final int TBOOLEAN = 1;
/**
* Type enumeration constant for lua light userdata, for compatibility with C-based lua only
*/
static final int TLIGHTUSERDATA = 2;
/**
* Type enumeration constant for lua numbers
*/
public static final int TNUMBER = 3;
/**
* Type enumeration constant for lua strings
*/
public static final int TSTRING = 4;
/**
* Type enumeration constant for lua tables
*/
public static final int TTABLE = 5;
/**
* Type enumeration constant for lua functions
*/
public static final int TFUNCTION = 6;
/**
* Type enumeration constant for lua userdatas
*/
public static final int TUSERDATA = 7;
/**
* Type enumeration constant for lua threads
*/
public static final int TTHREAD = 8;
/**
* Type enumeration constant for unknown values, for compatibility with C-based lua only
*/
public static final int TVALUE = 9;
/** String array constant containing names of each of the lua value types
* @see #type()
* @see #typename()
*/
// public static final String[] TYPE_NAMES = {
// "nil",
// "boolean",
// "lightuserdata",
// "number",
// "string",
// "table",
// "function",
// "userdata",
// "thread",
// "value",
// };
/**
* LuaValue constant corresponding to lua {@code nil}
*/
public static final LuaValue NIL = LuaNil._NIL;
/**
* LuaBoolean constant corresponding to lua {@code true}
*/
public static final LuaBoolean TRUE = LuaBoolean._TRUE;
/**
* LuaBoolean constant corresponding to lua {@code false}
*/
public static final LuaBoolean FALSE = LuaBoolean._FALSE;
/**
* LuaValue constant corresponding to a {@link Varargs} list of no values
*/
public static final LuaValue NONE = None._NONE;
/**
* LuaValue number constant equal to 0
*/
public static final LuaNumber ZERO = LuaInteger.valueOf(0);
/**
* LuaValue number constant equal to 1
*/
public static final LuaNumber ONE = LuaInteger.valueOf(1);
/**
* LuaValue number constant equal to -1
*/
public static final LuaNumber MINUSONE = LuaInteger.valueOf(-1);
/**
* LuaValue array constant with no values
*/
public static final LuaValue[] NOVALS = {};
/**
* The variable name of the environment.
*/
public static LuaString ENV = valueOf("_ENV");
/**
没有合适的资源?快使用搜索试试~ 我知道了~
VideoOS 安卓端 SDK.zip
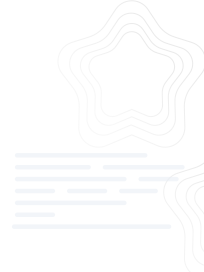
共1056个文件
java:806个
xml:88个
png:82个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 36 浏览量
2024-12-05
13:28:10
上传
评论
收藏 37.69MB ZIP 举报
温馨提示
VideoOS Android SDK本项目是VideoOS的Android SDK对接文档对接文档请移至这里项目概述VideoOS为视频流量主要提供基于视频场景生态下的小程序解决方案。紧贴视频内容运营结合视频内容,选择紧贴视频内容的运营方案,在播放视频时,自然的出现小程序。提高观看的互动性丰富多样的小程序,主题9大行业,激发用户观看视频的互动性,带来更多流量的转化。可实现快速改变小程序提供电商、广告等多种变现形式,流量主使用后可快速体验到流量的变现价值。AI助力Video++有成熟的视频AI算法加持,自动识别视频内容中的场景点位,降低人工运营成本。项目介绍VideoOS采用了Lua脚本语言来完成动态化方案,具有可高效扩展性强、简单、占用体积小、启动速度快等优势。VideoOS-Android-SDK结构app_demo : Android相关环境配置好后,可直接运行的模块,演示演示模块。venvy_pub : SDK核心模块,处理lua加载,lua脚本文件下载等逻辑。VenvyLibrary : 提供本地相关
资源推荐
资源详情
资源评论
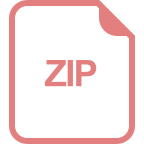
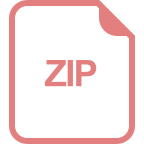
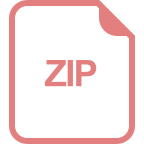
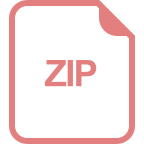
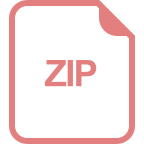
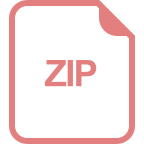
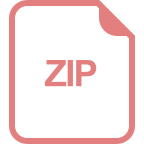
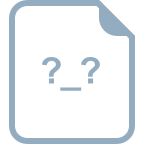
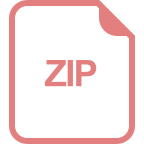
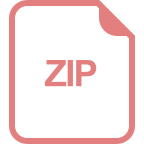
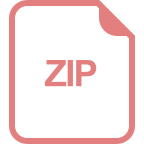
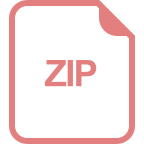
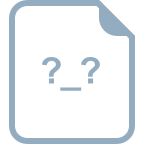
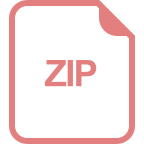
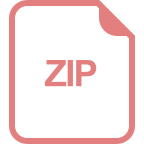
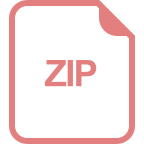
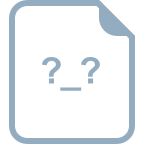
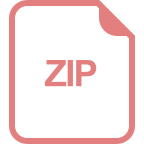
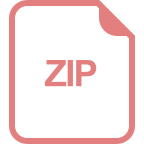
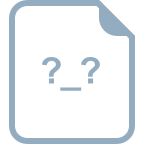
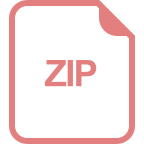
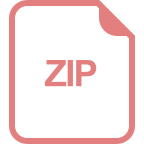
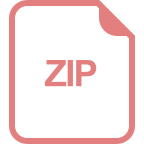
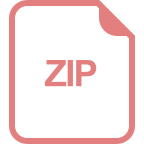
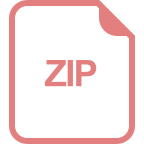
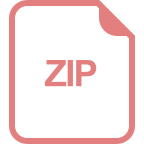
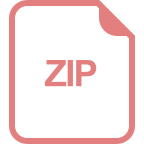
收起资源包目录

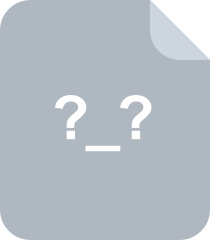
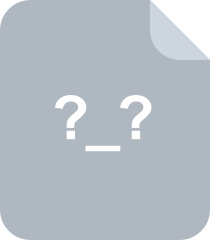
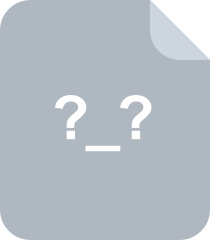
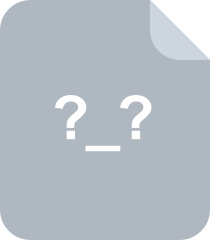
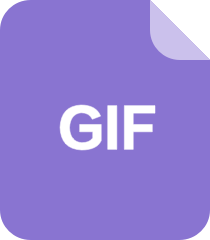
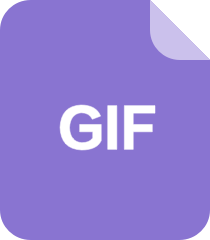
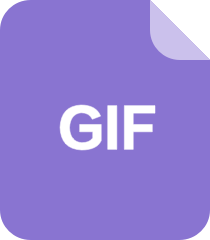
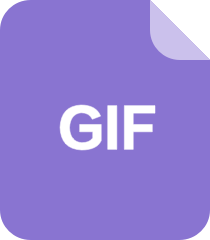
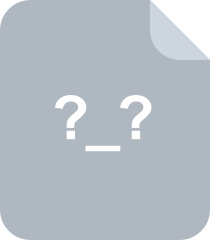
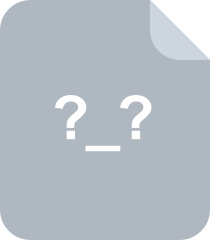
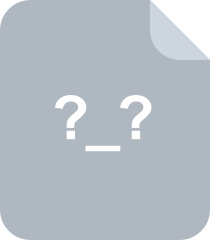
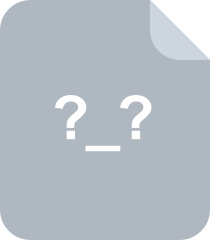
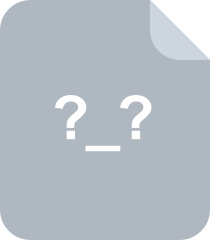
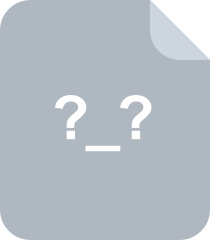
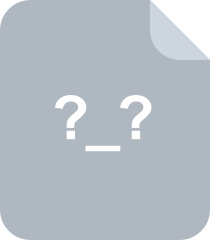
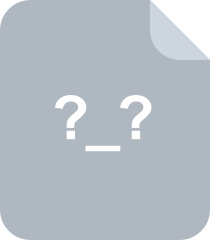
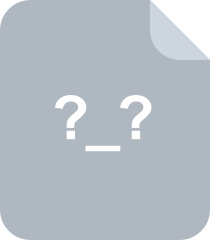
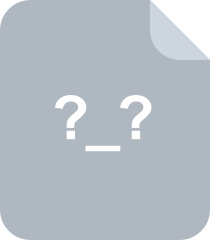
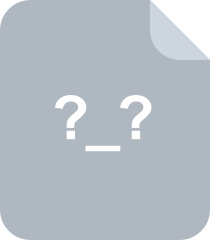
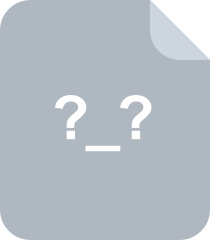
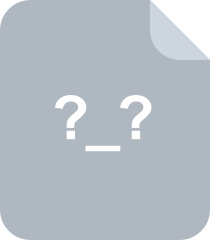
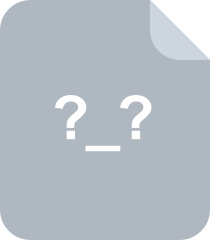
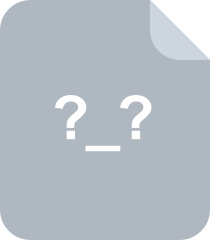
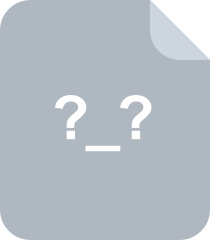
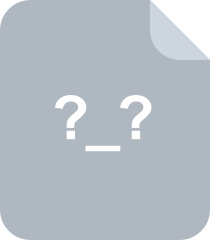
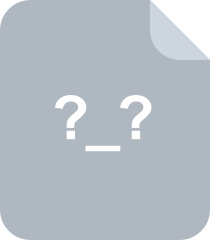
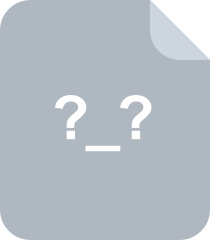
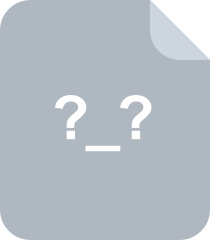
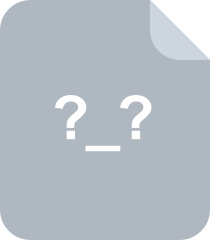
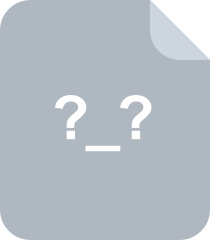
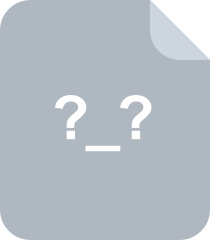
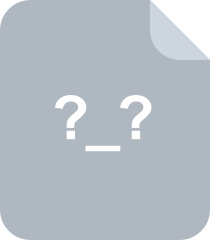
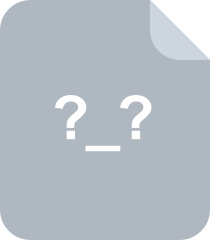
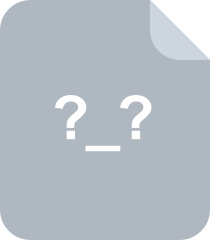
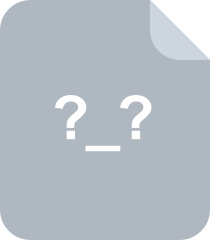
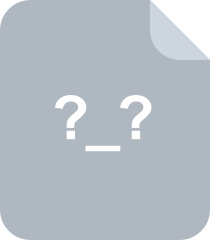
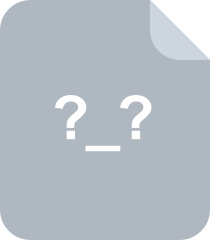
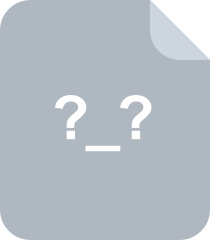
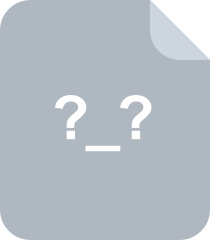
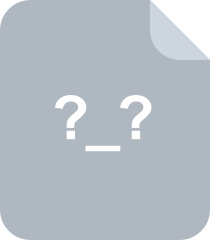
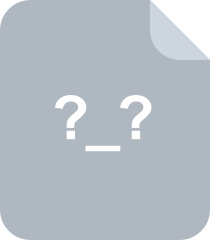
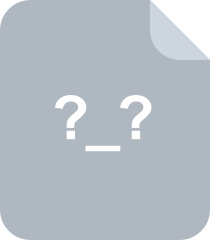
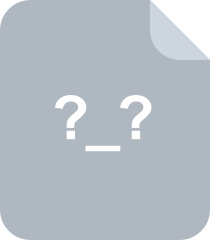
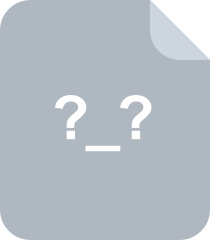
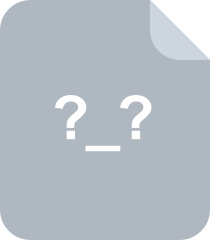
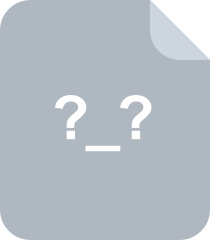
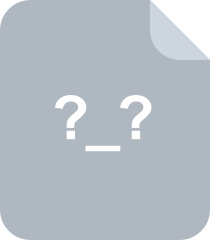
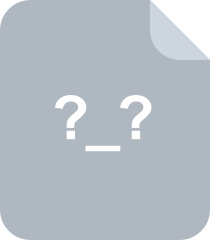
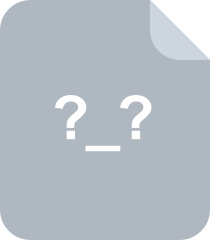
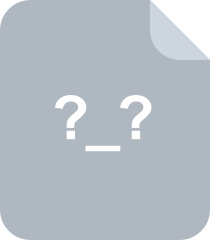
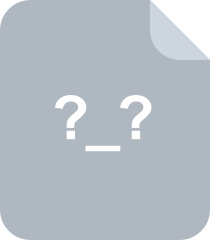
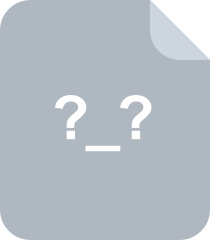
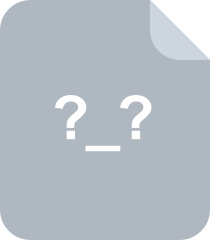
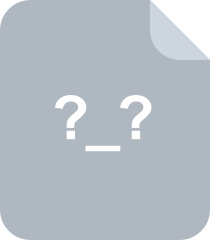
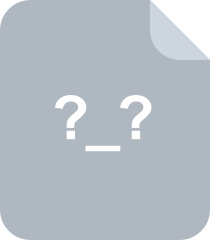
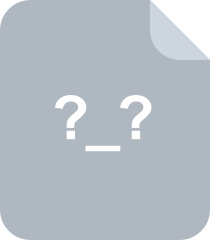
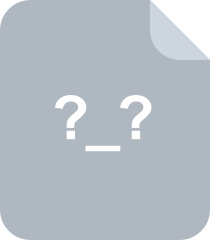
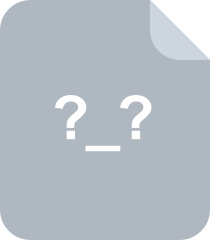
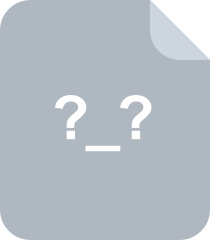
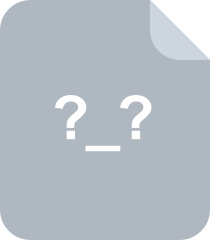
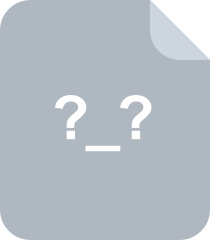
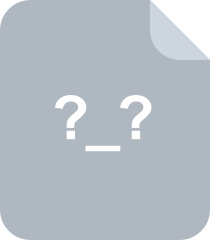
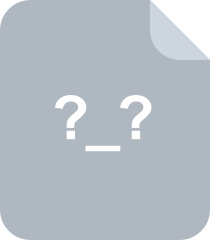
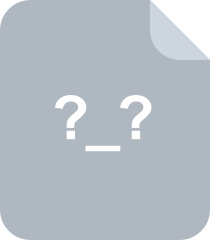
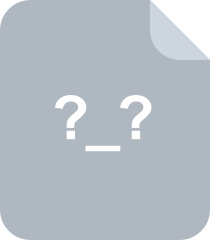
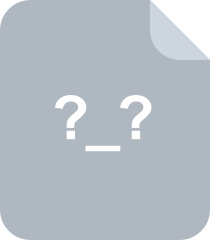
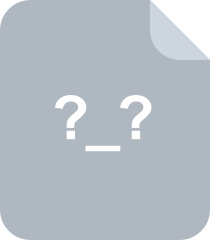
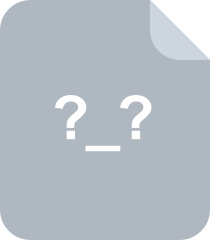
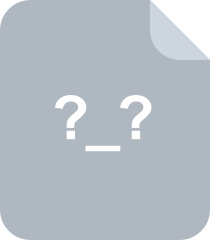
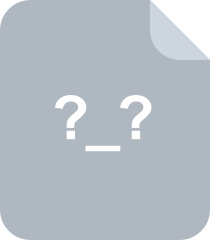
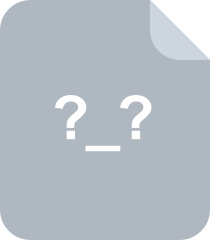
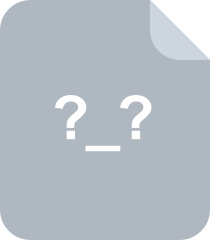
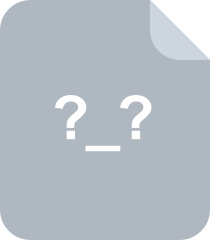
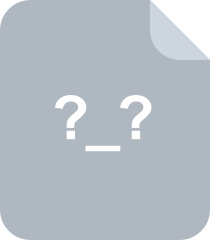
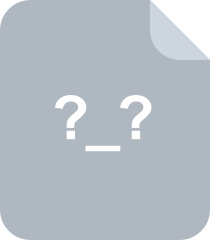
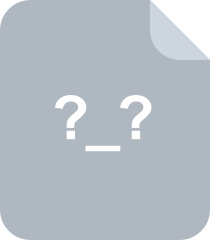
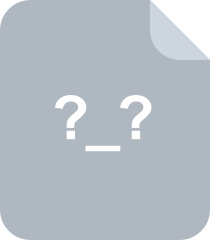
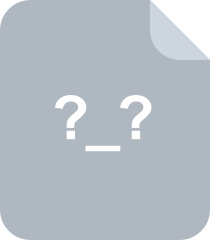
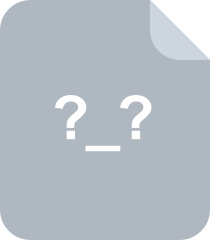
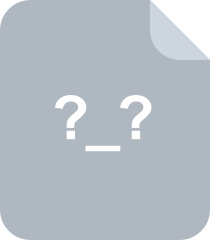
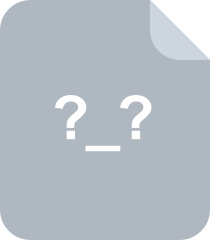
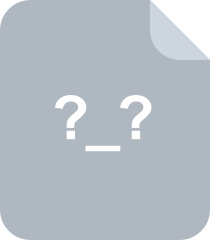
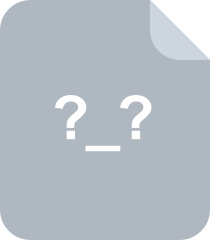
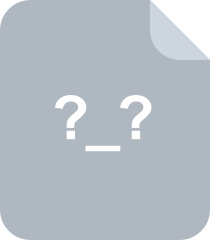
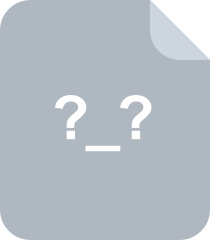
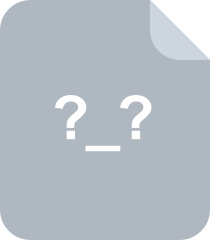
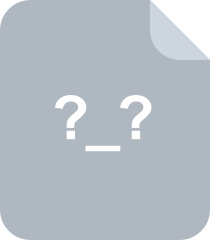
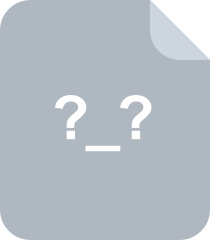
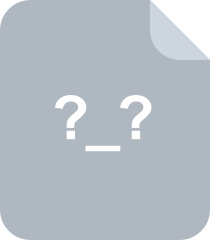
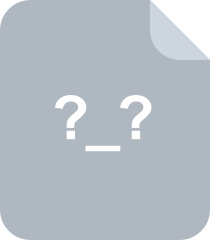
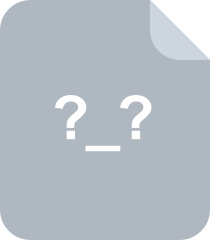
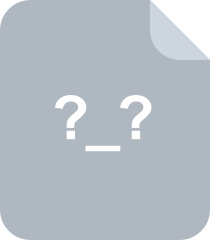
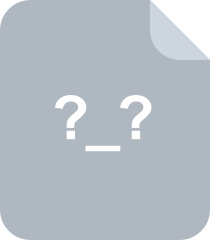
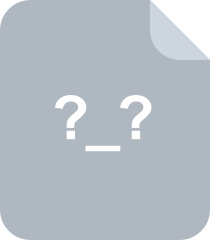
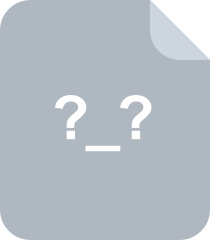
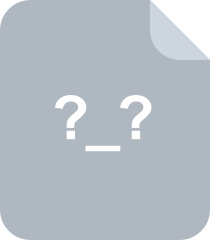
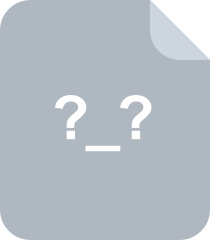
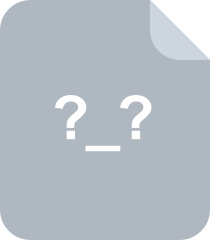
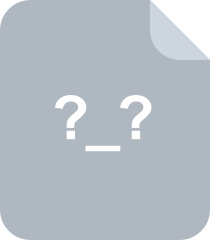
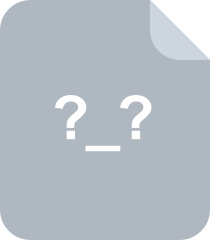
共 1056 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
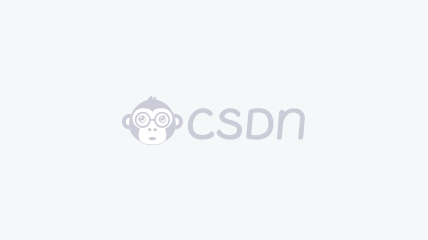

徐浪老师
- 粉丝: 8545
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

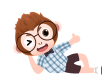
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


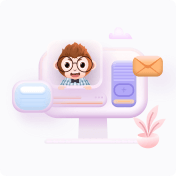
安全验证
文档复制为VIP权益,开通VIP直接复制
