<h1 align="center">OkRedis</h1>
<p align="center">
<a href="LICENSE"><img src="https://badgen.net/github/license/kristoff-it/zig-okredis" /></a>
<a href="https://twitter.com/croloris"><img src="https://badgen.net/badge/twitter/@croloris/1DA1F2?icon&label" /></a>
</p>
<p align="center">
OkRedis is a zero-allocation client for Redis 6+
</p>
## Handy and Efficient
OkRedis aims to offer an interface with great ergonomics without
compromising on performance or flexibility.
## Project status
OkRedis is currently in alpha. The main features are mostly complete,
but a lot of polishing is still required. Zig version `0.10.1` is
supported. Once `async` is available in a stable release, OkRedis will
pivot to a new Zig version.
Everything mentioned in the docs is already implemented and you can just
`zig run example.zig` to quickly see what it can do. Remember OkRedis only
supports Redis 6 and above.
## Zero dynamic allocations, unless explicitly wanted
The client has two main interfaces to send commands: `send` and `sendAlloc`.
Following Zig's mantra of making dynamic allocations explicit, only `sendAlloc`
can allocate dynamic memory, and only does so by using a user-provided allocator.
The way this is achieved is by making good use of RESP3's typed responses and
Zig's metaprogramming facilities.
The library uses compile-time reflection to specialize down to the parser level,
allowing OkRedis to decode whenever possible a reply directly into a function
frame, **without any intermediate dynamic allocation**. If you want more
information about Zig's comptime:
- [Official documentation](https://ziglang.org/documentation/master/#comptime)
- [What is Zig's Comptime?](https://kristoff.it/blog/what-is-zig-comptime) (blog post written by me)
By using `sendAlloc` you can decode replies with arbrirary shape at the cost of
occasionally performing dynamic allocations. The interface takes an allocator
as input, so the user can setup custom allocation schemes such as
[arenas](https://en.wikipedia.org/wiki/Region-based_memory_management).
## Quickstart
```zig
const std = @import("std");
const okredis = @import("./src/okredis.zig");
const SET = okredis.commands.strings.SET;
const OrErr = okredis.types.OrErr;
const Client = okredis.Client;
pub fn main() !void {
const addr = try std.net.Address.parseIp4("127.0.0.1", 6379);
var connection = try std.net.tcpConnectToAddress(addr);
var client: Client = undefined;
try client.init(connection);
defer client.close();
// Basic interface
try client.send(void, .{ "SET", "key", "42" });
const reply = try client.send(i64, .{ "GET", "key" });
if (reply != 42) @panic("out of towels");
// Command builder interface
const cmd = SET.init("key", "43", .NoExpire, .IfAlreadyExisting);
const otherReply = try client.send(OrErr(void), cmd);
switch (otherReply) {
.Nil => @panic("command should not have returned nil"),
.Err => @panic("command should not have returned an error"),
.Ok => std.debug.print("success!", .{}),
}
}
```
## Available Documentation
The reference documentation [is available here](https://kristoff.it/zig-okredis#root).
* [Using the OkRedis client](CLIENT.md#using-the-okredis-client)
* [Connecting](CLIENT.md#connecting)
* [Buffering](CLIENT.md#buffering)
* [Evented vs blocking I/O](CLIENT.md#evented-vs-blocking-io)
* [Pipelining](CLIENT.md#pipelining)
* [Transactions](CLIENT.md#transactions)
* [Pub/Sub](CLIENT.md#pubsub)
* [Sending commands](COMMANDS.md#sending-commands)
* [Base interface](COMMANDS.md#base-interface)
* [Command builder interface](COMMANDS.md#command-builder-interface)
* [Validating command syntax](COMMANDS.md#validating-command-syntax)
* [Optimized command builders](COMMANDS.md#optimized-command-builders)
* [Creating new command builders](COMMANDS.md#creating-new-command-builders)
* [An afterword on command builders vs methods](COMMANDS.md#an-afterword-on-command-builders-vs-methods)
* [Decoding Redis Replies](REPLIES.md#decoding-redis-replies)
* [Introduction](REPLIES.md#introduction)
* [The first and second rule of decoding replies](REPLIES.md#the-first-and-second-rule-of-decoding-replies)
* [Decoding Zig types](REPLIES.md#decoding-zig-types)
* [Void](REPLIES.md#void)
* [Numbers](REPLIES.md#numbers)
* [Optionals](REPLIES.md#optionals)
* [Strings](REPLIES.md#strings)
* [Structs](REPLIES.md#structs)
* [Decoding Redis errors and nil replies as values](REPLIES.md#decoding-redis-errors-and-nil-replies-as-values)
* [Redis OK replies](REPLIES.md#redis-ok-replies)
* [Allocating memory dynamically](REPLIES.md#allocating-memory-dynamically)
* [Allocating strings](REPLIES.md#allocating-strings)
* [Freeing complex replies](REPLIES.md#freeing-complex-replies)
* [Allocating Redis Error messages](REPLIES.md#allocating-redis-error-messages)
* [Allocating structured types](REPLIES.md#allocating-structured-types)
* [Parsing dynamic replies](REPLIES.md#parsing-dynamic-replies)
* [Bundled types](REPLIES.md#bundled-types)
* [Decoding types in the standard library](REPLIES.md#decoding-types-in-the-standard-library)
* [Implementing decodable types](REPLIES.md#implementing-decodable-types)
* [Adding types for custom commands (Lua scripts or Redis modules)](REPLIES.md#adding-types-for-custom-commands-lua-scripts-or-redis-modules)
* [Adding types used by a higher-level language](REPLIES.md#adding-types-used-by-a-higher-level-language)
## Extending OkRedis
If you are a Lua script or Redis module author, you might be interested in
reading the final sections of `COMMANDS.md` and `REPLIES.md`.
## Embedding OkRedis in a higher level language
Take a look at the final section of `REPLIES.md`.
## TODOS
- Implement remaining command builders
- Better connection management (ipv6, unixsockets, ...)
- Streamline design of Zig errors
- Refine support for async/await and think about connection pooling
- Refine the Redis traits
- Pub/Sub
没有合适的资源?快使用搜索试试~ 我知道了~
Redis 6+ 的零分配客户端.zip
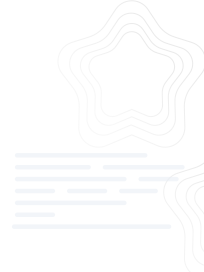
共107个文件
zig:91个
md:6个
txt:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 26 浏览量
2024-12-04
14:23:04
上传
评论
收藏 429KB ZIP 举报
温馨提示
奥克雷迪斯 OkRedis 是 Redis 6+ 的零分配客户端便捷高效OkRedis 的目标是提供符合人体工程学的界面,同时不影响性能或灵活性。项目状态OkRedis 目前处于 alpha 阶段。主要功能基本完成,但仍需大量完善。0.10.1支持 Zig 版本。一旦async稳定版本推出,OkRedis 将转向新的 Zig 版本。文档中提到的所有内容都已实现,您可以 zig run example.zig快速了解它的功能。请记住,OkRedis 仅支持 Redis 6 及更高版本。零动态分配,除非明确需要客户端有两个主要接口来发送命令send和sendAlloc。遵循 Zig 明确动态分配的原则,只能sendAlloc 分配动态内存,并且只能通过使用用户提供的分配器来实现。实现这一点的方法是充分利用 RESP3 的类型化响应和 Zig 的元编程功能。该库使用编译时反射来专门化到解析器级别,允许 OkRedis 尽可能将回复直接解码为函数框架,而无需任何中间动态分配。如果你想要有关 Zig 的 comptime 的更多信息官方文档Zig 的
资源推荐
资源详情
资源评论
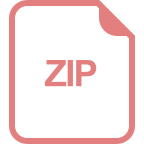
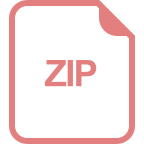
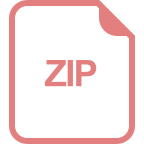
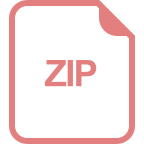
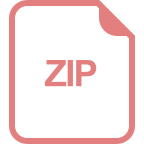
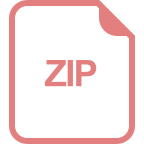
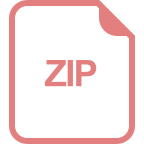
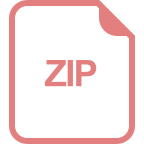
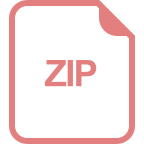
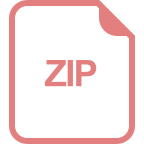
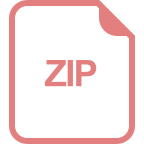
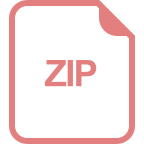
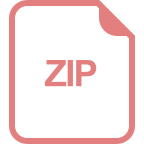
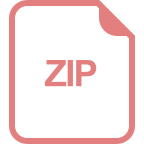
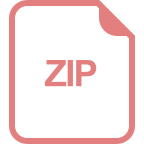
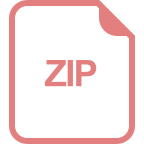
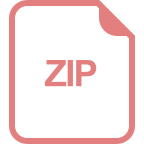
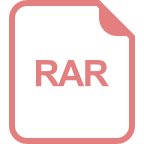
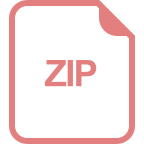
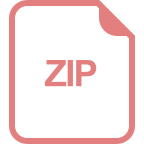
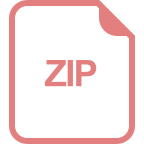
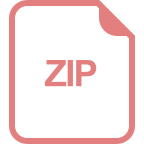
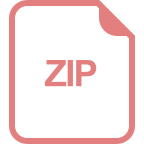
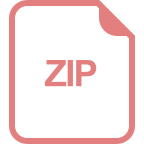
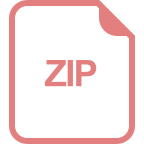
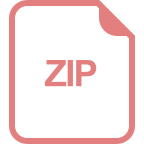
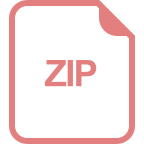
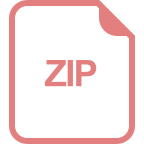
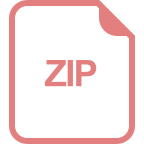
收起资源包目录

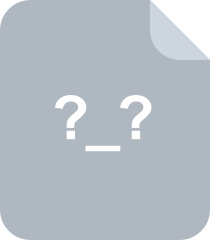
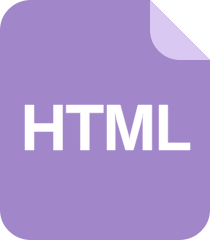
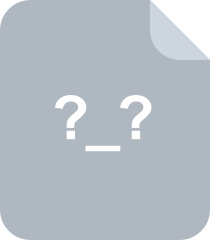
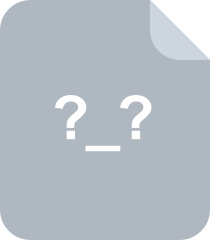
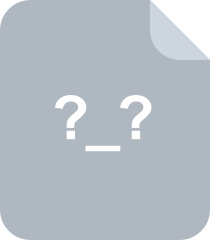
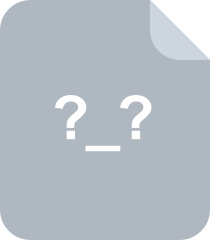
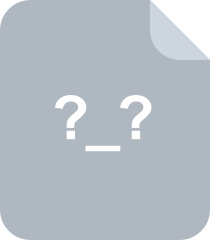
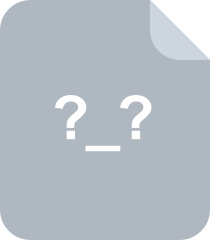
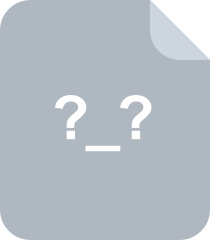
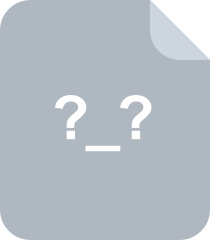
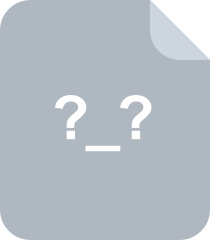
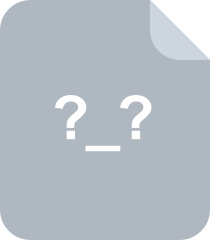
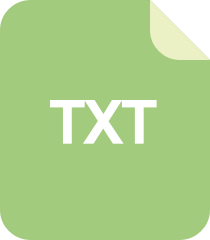
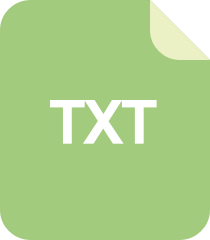
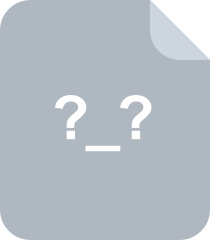
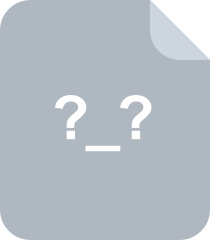
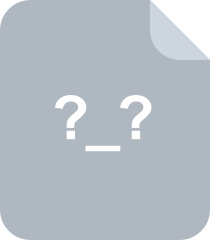
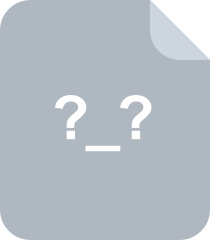
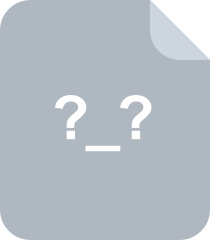
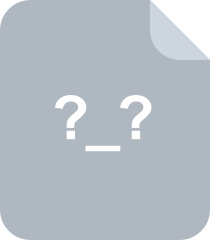
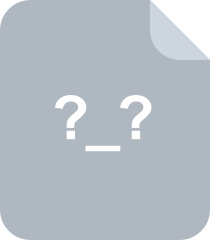
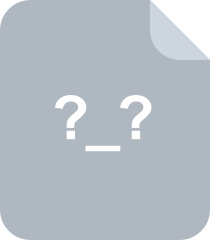
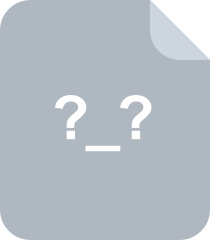
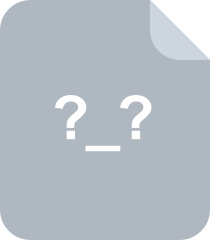
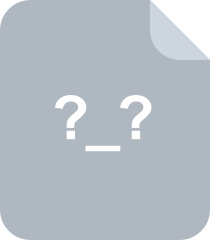
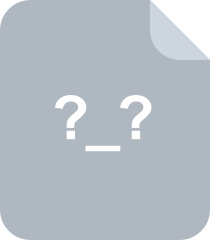
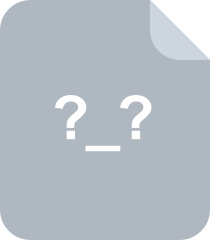
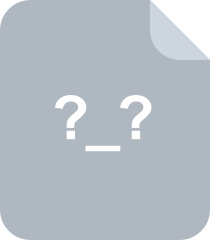
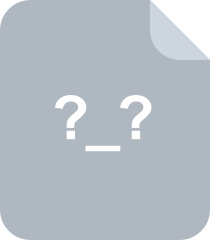
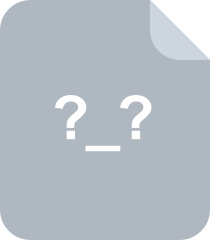
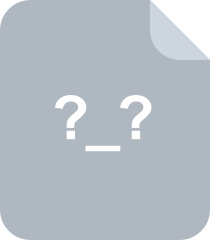
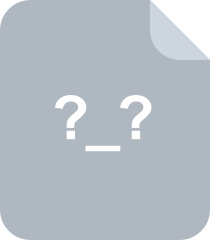
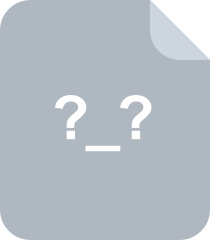
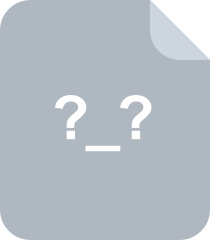
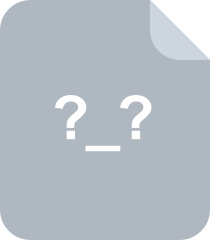
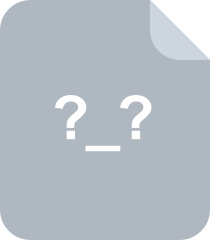
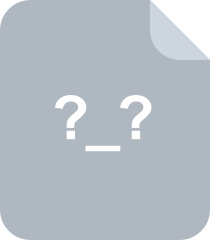
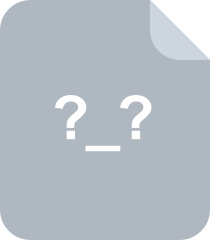
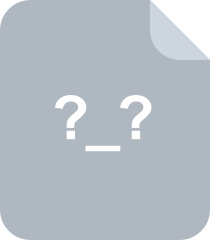
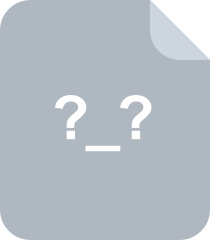
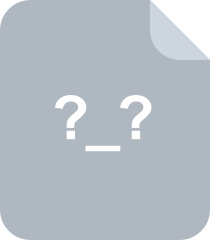
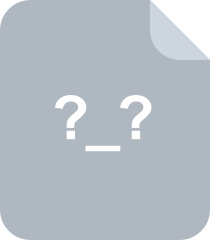
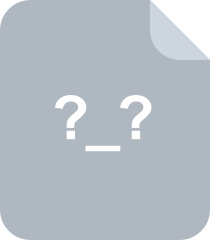
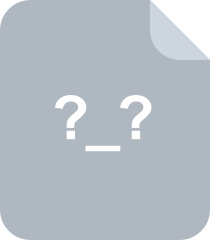
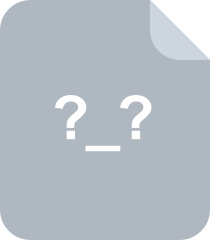
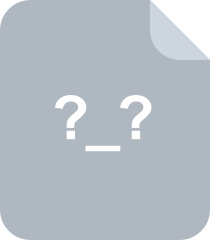
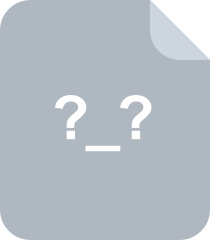
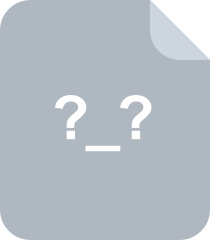
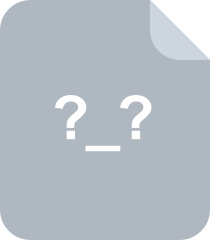
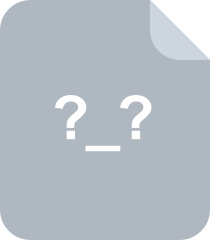
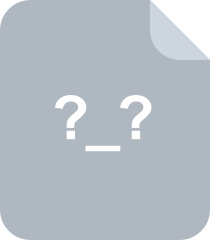
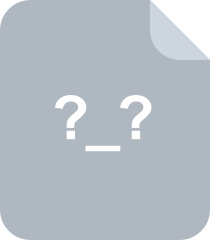
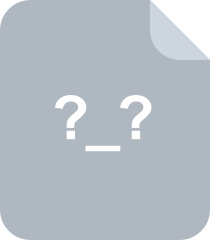
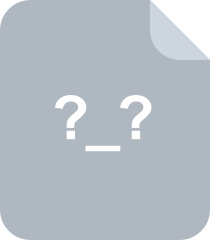
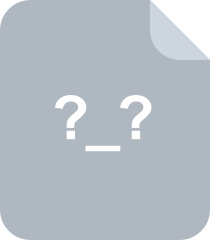
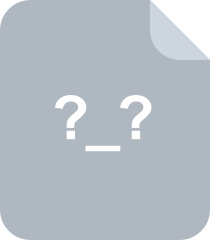
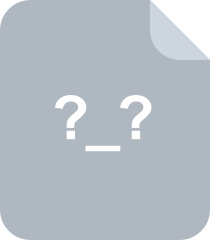
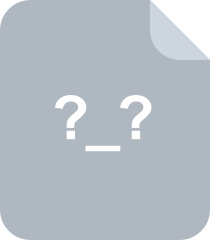
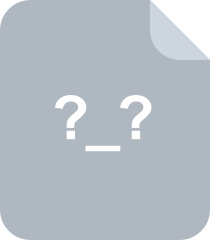
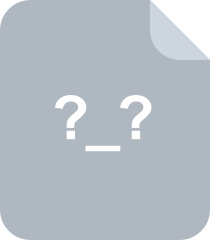
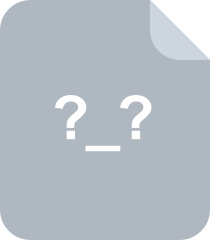
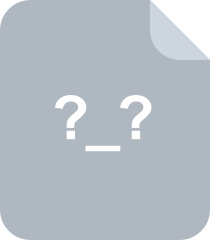
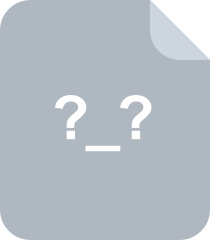
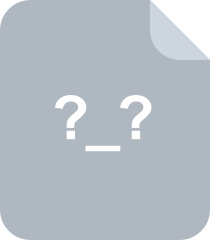
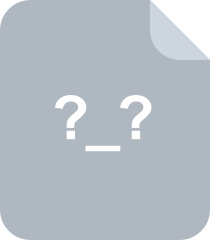
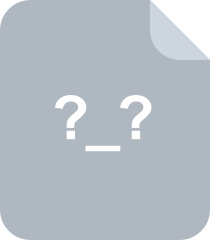
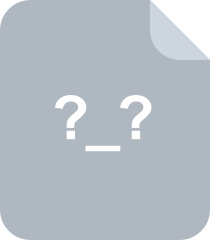
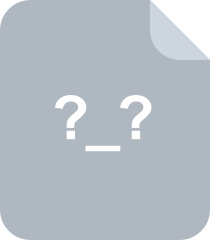
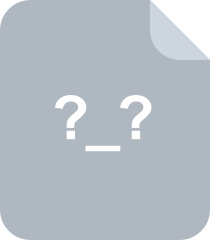
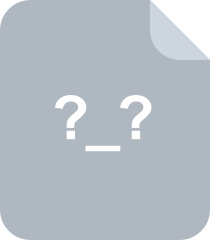
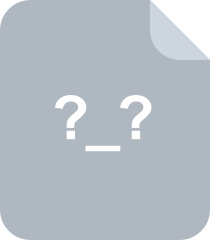
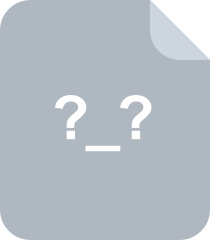
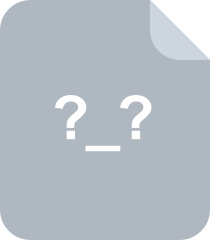
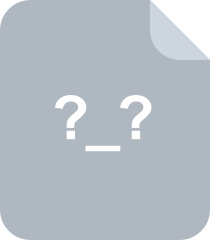
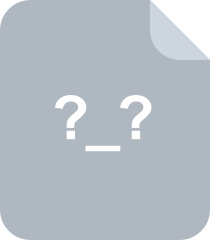
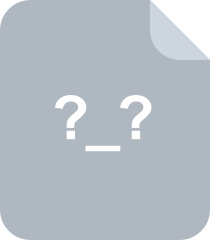
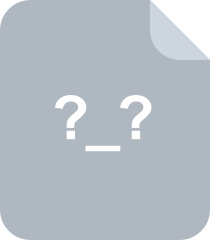
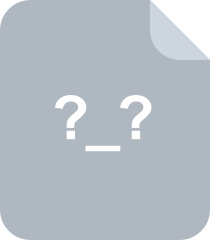
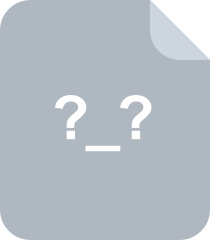
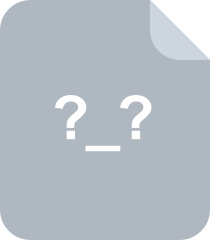
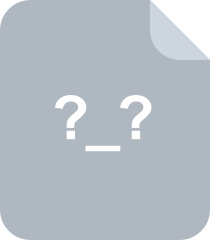
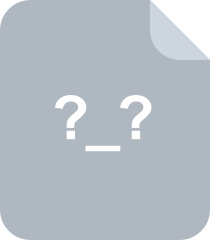
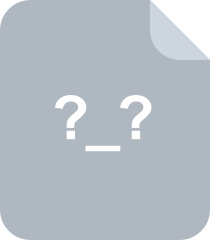
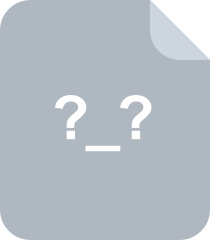
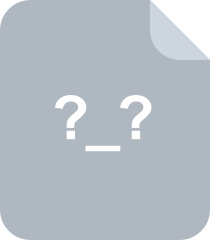
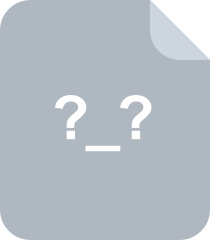
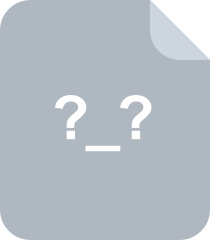
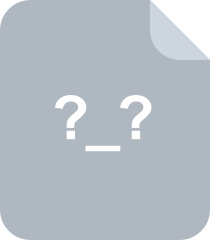
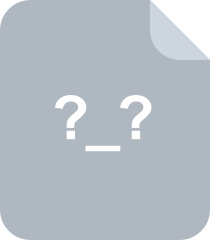
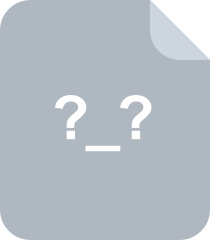
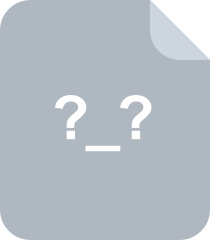
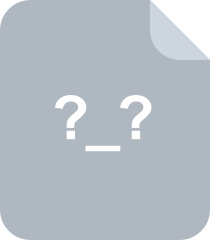
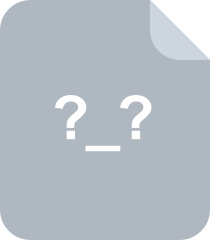
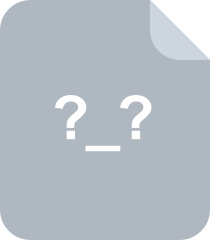
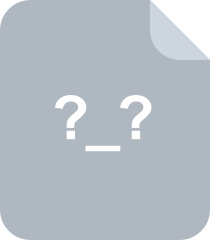
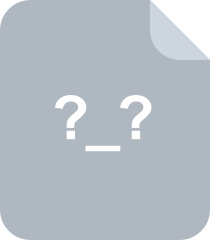
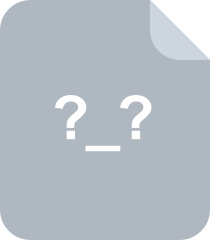
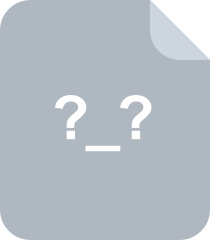
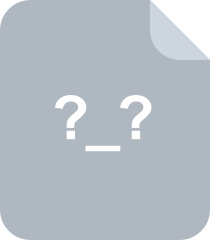
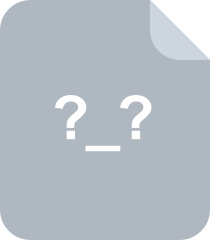
共 107 条
- 1
- 2
资源评论
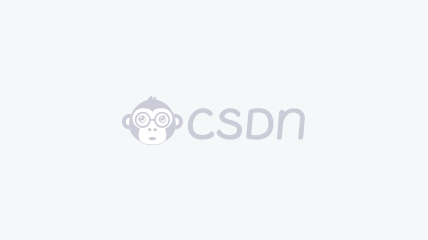

徐浪老师
- 粉丝: 8317
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

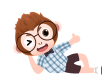
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


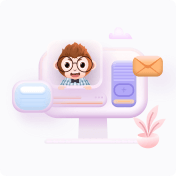
安全验证
文档复制为VIP权益,开通VIP直接复制
