# Redis PHP Mock [](https://github.com/BedrockStreaming/RedisMock/actions/workflows/ci.yml) [](https://packagist.org/packages/m6web/redis-mock)
PHP 7.1 library providing a Redis PHP mock for your tests.
**Work for now only with predis**
## Installation
```bash
$ composer require --dev m6web/redis-mock
```
## Functions
It currently mocks these Redis commands :
Redis command | Description
-------------------------------------------------|------------
**DBSIZE** | Returns the number of keys in the selected database
**DEL** *key* *[key ...]* | Deletes one or more keys
**DECR** *key* | Decrements the integer value of a key by one
**DECRBY** *key* *decrement* | Decrements the integer value of a key by `decrement` value
**DECRBYFLOAT** *key* *decrement* | Decrements the float value of a key by `decrement` value
**EXISTS** *key* | Determines if a key exists
**EXPIRE** *key* *seconds* | Sets a key's time to live in seconds
**FLUSHDB** | Flushes the database
**GET** *key* | Gets the value of a key
**HDEL** *key* *array\<fields\>* | Delete hash fields
**HEXISTS** *key* *field* | Determines if a hash field exists
**HMGET** *key* *array\<field\>* | Gets the values of multiple hash fields
**HGET** *key* *field* | Gets the value of a hash field
**HGETALL** *key* | Gets all the fields and values in a hash
**HKEYS** *key* | Gets all the fields in a hash
**HLEN** *key* | Gets the number of fields in a hash
**HMSET** *key* *array\<field, value\>* | Sets each value in the corresponding field
**HSET** *key* *field* *value* | Sets the string value of a hash field
**HSETNX** *key* *field* *value* | Sets field in the hash stored at key to value, only if field does not yet exist
**HINCRBY** *key* *field* *increment* | Increments the integer stored at `field` in the hash stored at `key` by `increment`.
**INCR** *key* | Increments the integer value of a key by one
**INCRBY** *key* *increment* | Increments the integer value of a key by `increment` value
**INCRBYFLOAT** *key* *increment* | Increments the float value of a key by `increment` value
**KEYS** *pattern* | Finds all keys matching the given pattern
**LINDEX** *key* *index* | Returns the element at index *index* in the list stored at *key*
**LLEN** *key* | Returns the length of the list stored at *key*
**LPUSH** *key* *value* *[value ...]* | Pushs values at the head of a list
**LPOP** *key* | Pops values at the head of a list
**LREM** *key* *value* *count* | Removes `count` instances of `value` from the head of a list (follows the [`predis` parameters order](https://github.com/phpredis/phpredis#lrem-lremove))
**LTRIM** *key* *start* *stop* | Removes the values of the `key` list which are outside the range `start`...`stop`
**LRANGE** *key* *start* *stop* | Gets a range of elements from a list
**MGET** *array\<field\>* | Gets the values of multiple keys
**MSET** *array\<field, value\>* | Sets the string values of multiple keys
**QUIT** | Quit the REDIS
**RPUSH** *key* *value* | Pushs values at the tail of a list
**RPOP** *key* | Pops values at the tail of a list
**SCAN** | Iterates the set of keys in the currently selected Redis database.
**SSCAN** | Iterates elements of Sets types.
**SET** *key* *value* | Sets the string value of a key
**SETEX** *key* *seconds* *value* | Sets the value and expiration of a key
**SETNX** *key* *value* | Sets key to hold value if key does not exist
**SADD** *key* *member* *[member ...]* | Adds one or more members to a set
**SDIFF** *key* *key* *[key ...]* | Returns the members of the set resulting from the difference between the first set and all the successive sets.
**SISMEMBER** *key* *member* | Determines if a member is in a set
**SMEMBERS** *key* | Gets all the members in a set
**SUNION** *key* *[key ...]* | Returns the members of the set resulting from the union of all the given sets.
**SINTER** *key* *[key ...]* | Returns the members of the set resulting from the intersection of all the given sets.
**SCARD** *key* | Get cardinality of set (count of members)
**SREM** *key* *member* *[member ...]* | Removes one or more members from a set
**TTL** *key* | Gets the time to live for a key
**TYPE** *key* | Returns the string representation of the type of the value stored at key.
**ZADD** *key* *score* *member* | Adds one member to a sorted set, or update its score if it already exists
**ZINCRBY** *key* *increment* *member* | Increments the score of *member* in the sorted set stored at *key* by *increment*
**ZCARD** *key* | Returns the sorted set cardinality (number of elements) of the sorted set stored at *key*
**ZCOUNT** **key** **min** **max** | Returns the number of elements in the stored set at *key* with a score between *min* and *max*.
**ZRANGE** *key* *start* *stop* *[withscores]* | Returns the specified range of members in a sorted set
**ZRANGEBYSCORE** *key* *min* *max* *options* | Returns a range of members in a sorted set, by score
**ZRANK** *key* *member* | Returns the rank of *member* in the sorted set stored at *key*, with the scores ordered from low to high
**ZREVRANK** *key* *member* | Returns the rank of *member* in the sorted set stored at *key*, with the scores ordered from high to low
**ZREM** *key* *member* | Removes one membner from a sorted set
**ZREMRANGEBYSCORE** *key* *min* *max* | Removes all members in a sorted set within the given scores
**ZREVRANGE** *key* *start* *stop* *[withscores]*| Returns the specified range of members in a sorted set, with scores ordered from high to low
**ZREVRANGEBYSCORE** *key* *min* *max* *options* | Returns a range of members in a sorted set, by score, with scores ordered from high to low
**ZSCAN** | Iterates elements of Sorted Sets types.
**ZSCORE** *key* *member* | Returns the score of *member* in the sorted set at *key*
**ZUNIONSTORE** *dest* *numkeys* *key* ... *[weights ...]* *[aggregate SUM/MIN/MAX]* | Computes the union of the stored sets given by the specified keys, store the result in the destination key, and returns the number of elements of the new sorted set.
It mocks **MULTI**, **DISCARD** and **EXEC** commands but without any transaction behaviors, they just make the interface fluent and return each command results.
**PIPELINE** and **EXECUTE** pseudo commands (client pipelining) are also mocked.
**EVAL**, **EVALSHA**, **WATCH** and **UNWATCH** are just s
没有合适的资源?快使用搜索试试~ 我知道了~
一个简单的 PHP Redis 模拟.zip
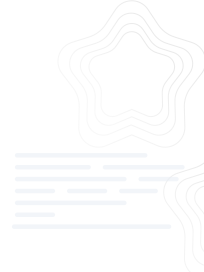
共16个文件
php:7个
txt:2个
md:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 106 浏览量
2024-12-03
12:52:00
上传
评论
收藏 25KB ZIP 举报
温馨提示
一个简单的 PHP Redis 模拟Redis PHP 模拟 PHP 7.1 库为您的测试提供 Redis PHP 模拟。目前仅适用于 predis安装$ composer require --dev m6web/redis-mock功能它目前模拟这些 Redis 命令Redis 命令 描述数据库大小 返回所选数据库中的键数DEL 键 [键...] 删除一个或多个键DECR 键 将键的整数值减一DECRBY 密钥 减量 将键的整数值减去decrement值DECRBYFLOAT 键 减量 decrement按值减少键的浮点值EXISTS 键 确定键是否存在EXPIRE 关键 秒数 设置密钥的存活时间(以秒为单位)刷新数据库 刷新数据库获取 密钥 获取键的值HDEL 键 数组<字段> 删除哈希字段HEXISTS 关键字 段 确定哈希字段是否存在HMGET 键 数组<field> 获取多个哈希字段的值HGET 关键字 段 获取哈希字段的值HGETALL 键 获取哈希中的所有字段和值HKEYS 键 获取哈希表中的所有字段HLEN 键
资源推荐
资源详情
资源评论
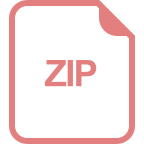
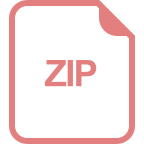
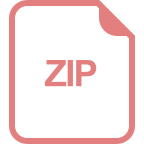
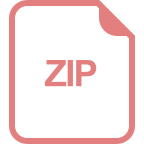
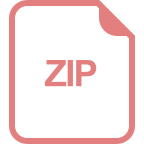
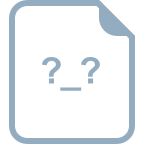
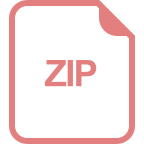
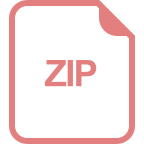
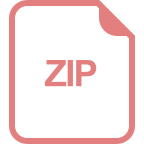
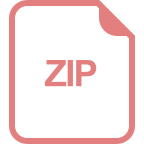
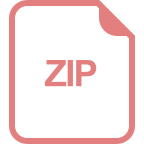
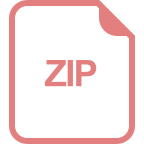
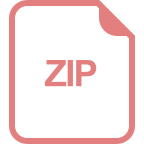
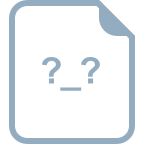
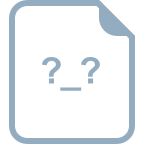
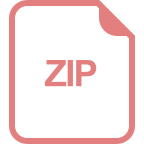
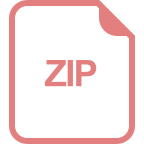
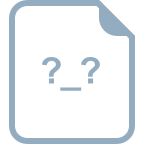
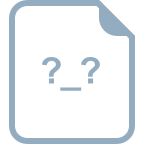
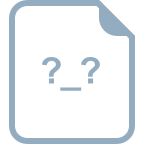
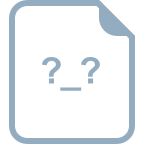
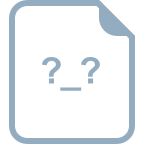
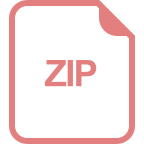
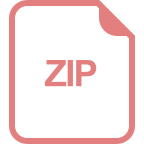
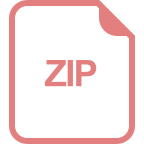
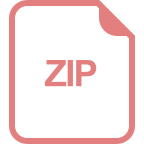
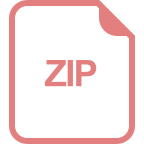
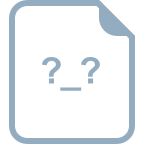
收起资源包目录

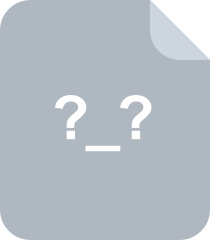

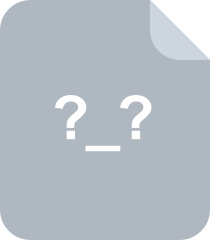

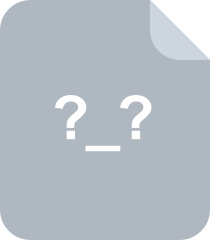
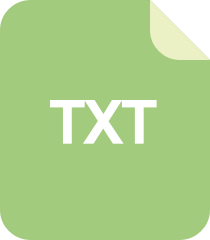




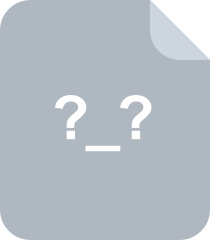
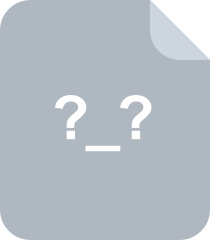
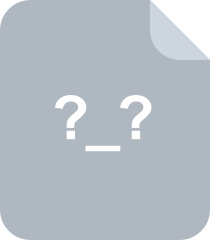
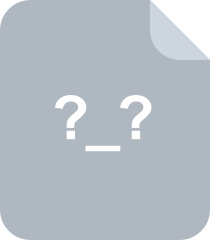
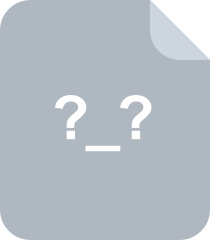


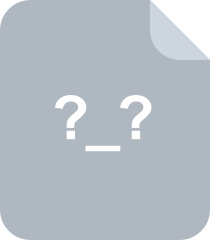
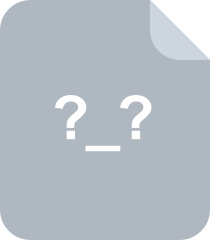
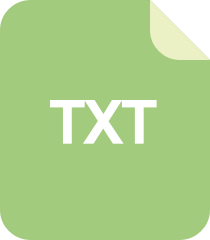
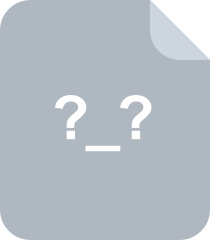
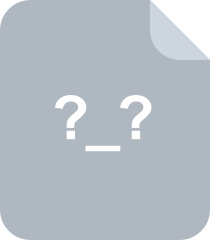
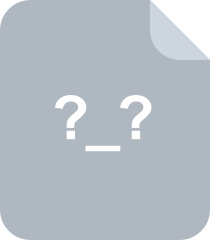
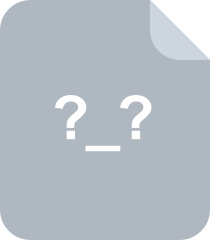
共 16 条
- 1
资源评论
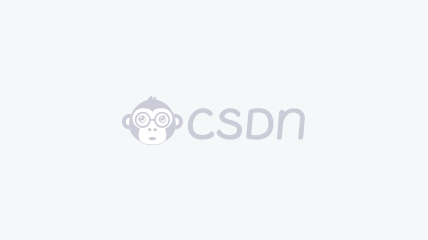

徐浪老师
- 粉丝: 8455
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

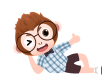
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


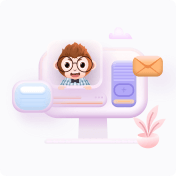
安全验证
文档复制为VIP权益,开通VIP直接复制
