# vue-ethereum-ipfs
Distributed Application Starter: Vue front-end, Ethereum / IPFS Backend
Ethereum is a distributed virtual machine that pays eth in return for miners running
your smart contracts. IPFS is a kind of distributed content distribution network. Vue
is a javascript framework for building client-side webapps. By keeping state inside
ethereum and using IPFS to deliver HTML, webapps can become nearly indestructible!
## How do I use this to make indestructible Vue apps that speak Ethereum?
### Before you start
Install IPFS: [https://ipfs.io/docs/install/](https://ipfs.io/docs/install/) <br/>
Install the MetaMask Ethereum wallet (and register an account): [https://metamask.io/](https://metamask.io/) <br/>
Install: `npm i -g ganache-cli` (local Ethereum test network) <br/>
Install: `npm i -g truffle` (Solidity toolkit!) <br/>
### Obtain your IPFS repo key and set an environment variable
To obtain your key: `ipfs key list -l` <br/>
Set: `export IPFS_PUBKEY=QmQozMTQHW9g6fKmHerVHoKQNQo4zhfXQMsWMTuJ6D1sJd` (Example key)
### Start the local Ethereum test net <br/>
Run: `ganache-cli --accounts=4`
### Connect Metamask to the test net <br/>
Select **Localhost 8545** as your RPC form the MetaMask UI
Use the generated passphrase to log into MetaMask eg:
```
HD Wallet
==================
Mnemonic: shoe panic long movie sponsor clarify casino stable calm scene enforce federal
```
Import the other accounts in to MetaMask for testing using the generated private key eg:
```
Private Keys
==================
(0) 2f3a3521d79a5e5c58972224d80a678c993a1a50b7cf8a2ee51e255e55fb041d <- the passphrase unlocks this account
(1) 557d2bd6ab422edda5d57a0c20e0908c31c94a3c7c8af40c923925a3403bd214
(2) 76e98c90b7168242fd523b718a76b95966ab09904129c011582369e7339327a8
(3) 683746dee343d96dd792130b01febc0d75dd5a540fae79350db6ed9f597d
```
### Install the Vue packages
```
$ npm install
```
### Vue Build and deploy commands
```
"scripts": {
"dev":
"webpack-dev-server --inline --progress --config build/webpack.dev.conf.js",
"start": "npm run dev",
"unit": "jest --config test/unit/jest.conf.js --coverage",
"e2e": "node test/e2e/runner.js",
"test": "npm run unit && npm run e2e",
"lint": "eslint --ext .js,.vue src test/unit test/e2e/specs",
"build": "export IPFS_PUBKEY= && node build/build.js",
"publish:ipfs": "npm run test && node build/build.js && node build/ipfs-publish.js"
},
```
```
$ npm start
Your application is running here: http://localhost:8081
```
### Create your own Smart Contracts
The easiest way to start developing Smart Contracts: <br/>
#### [https://remix.ethereum.org/](https://remix.ethereum.org/)
### Add contracts to the Vue App
- Add all of your contracts (.sol files) to the `/contracts` directory
- Run: `truffle compile && truffle migrate --network development`
### Use your Contracts in the App!
Example `web3Service.js`. This code demonstrates a contract factory pattern. For the full code see the `web3Service.js` file in the project.
```js
import contract from 'truffle-contract'
import contractJSON from '../build/contracts/WitnessContract.json'
const Contract = contract(contractJSON)
const createContractInstance = async c => {
try {
const newContract = await Contract.new(c.name, c.terms, {
from: c.witness,
gasPrice: 2000000000,
gas: '2000000'
})
return newContract
} catch (e) {
console.log(e, 'Error creating contract...')
}
}
export { createContractInstance }
```
Tested with:
* Node (>=)9.0.0
* go version go1.9.4 darwin/amd64
* ipfs version 0.4.11
* Ganache CLI v6.0.3 (ganache-core: 2.0.2)
* Google Chrome 64.0.3282.167 (Official Build) (64-bit)
---
### Links
Teach and learn JavaScript with us at RED Academy: https://redacademy.com/
没有合适的资源?快使用搜索试试~ 我知道了~
分布式应用程序启动器Vue 前端、以太坊 , IPFS 后端.zip
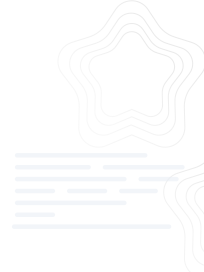
共58个文件
js:30个
vue:6个
json:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 174 浏览量
2024-12-02
02:22:18
上传
评论
收藏 207KB ZIP 举报
温馨提示
vue-ethereum-ipfs分布式应用程序启动器Vue 前端、以太坊 / IPFS 后端以太坊是一个分布式虚拟机,它向运行智能合约的矿工支付 eth 作为回报。IPFS 是一种分布式内容分发网络。Vue 是一个用于构建客户端 Web 应用程序的 JavaScript 框架。通过在以太坊内部保持状态并使用 IPFS 传递 HTML,Web 应用程序几乎可以变得坚不可摧!我如何使用它来制作使用以太坊的坚不可摧的 Vue 应用程序?开始之前安装 IPFShttps://ipfs.io/docs/install/安装 MetaMask 以太坊钱包(并注册账号)https://metamask.io/ 安装(npm i -g ganache-cli本地以太坊测试网络)安装npm i -g truffle(Solidity 工具包!)获取你的 IPFS 存储库密钥并设置环境变量要获取您的密钥ipfs key list -l设置(export IPFS_PUBKEY=QmQozMTQHW9g6fKmHerVHoKQNQo4zhfXQMsWMTuJ6D1sJd
资源推荐
资源详情
资源评论
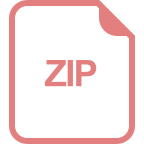
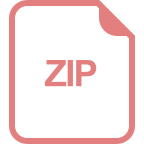
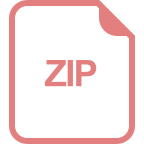
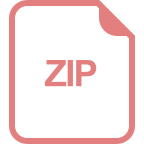
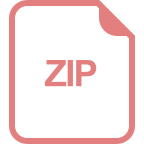
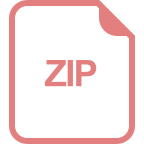
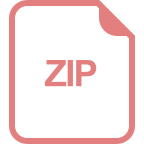
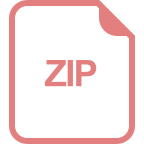
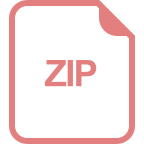
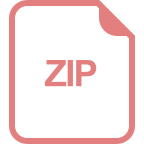
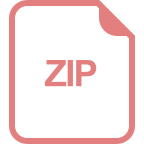
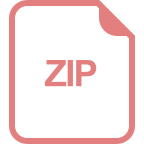
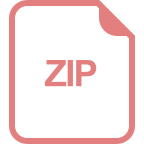
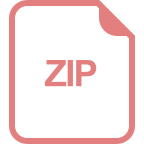
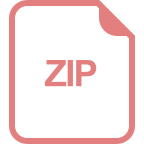
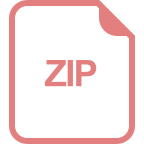
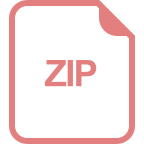
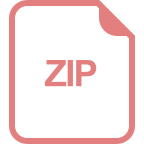
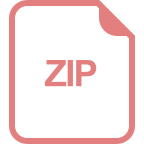
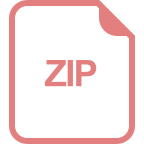
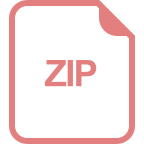
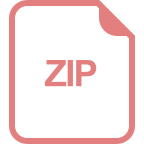
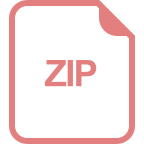
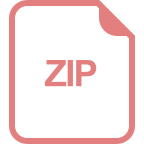
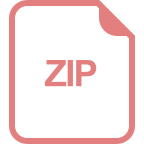
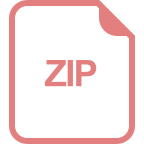
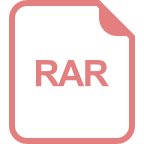
收起资源包目录

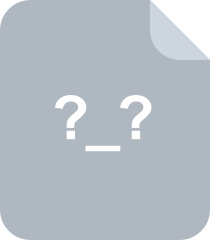
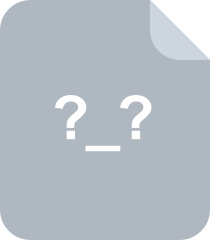
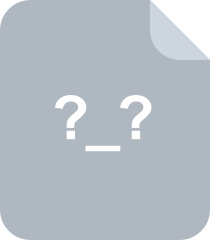
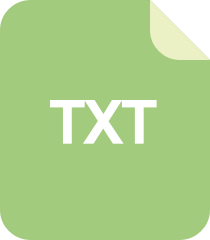

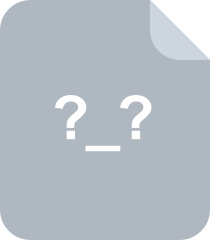
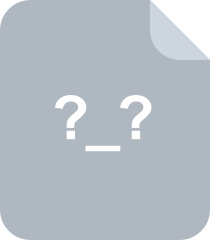
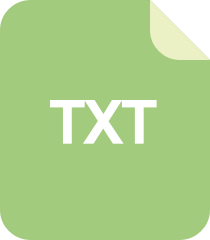

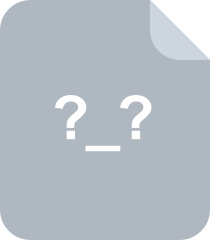
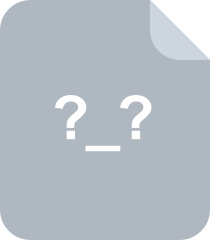

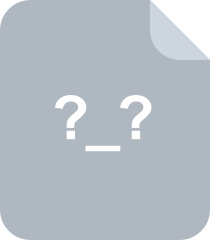


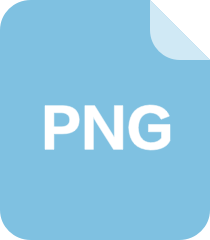
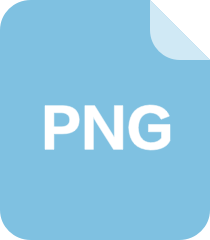
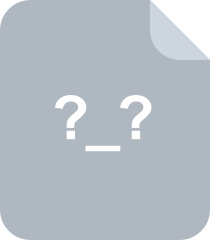

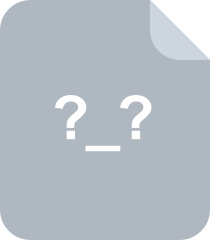
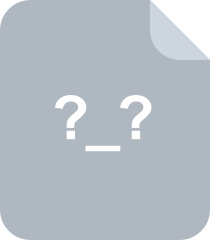
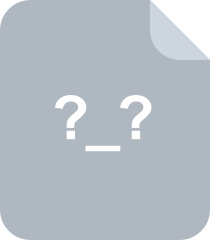
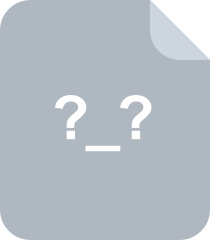
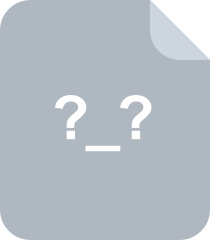
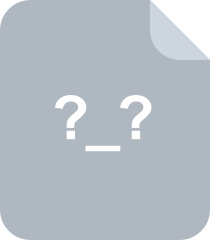

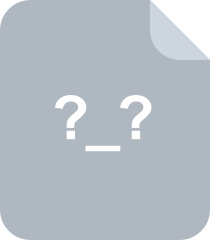
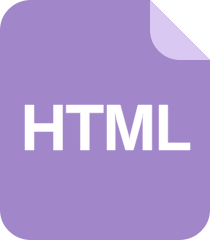
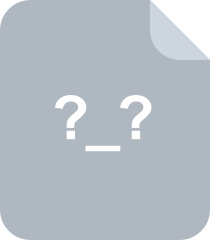
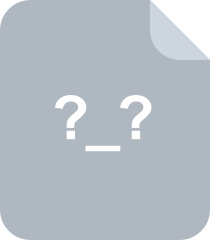

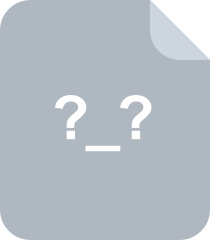
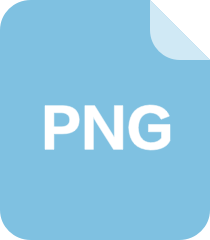
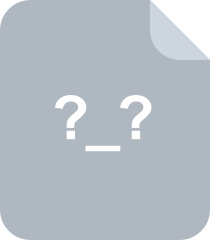
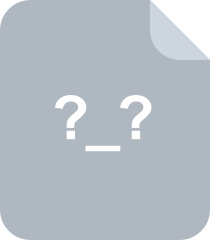
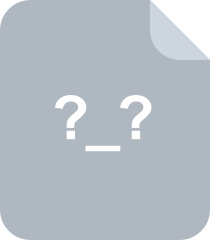
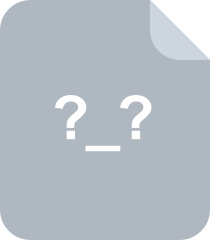
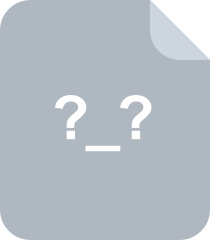

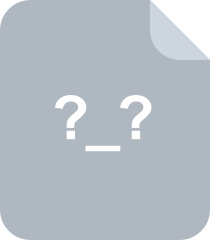
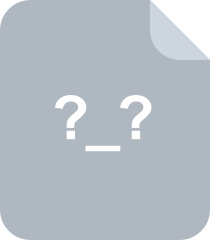
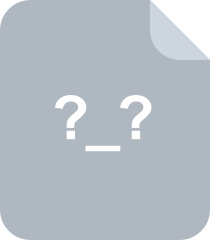
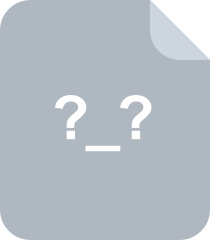
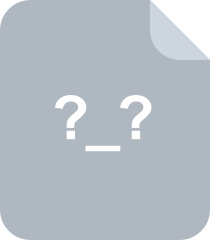
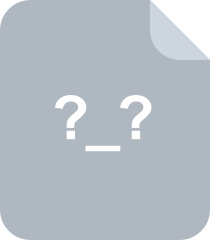
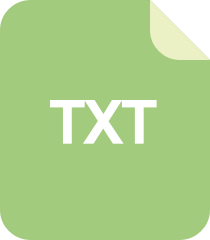
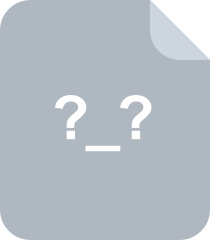
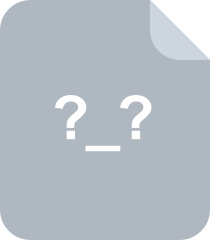
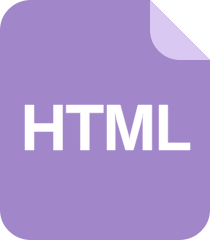



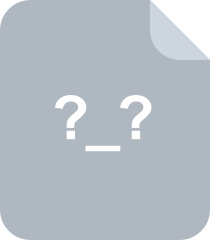
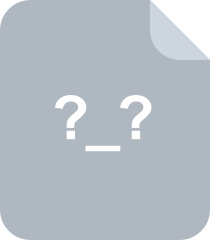
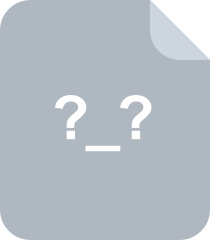

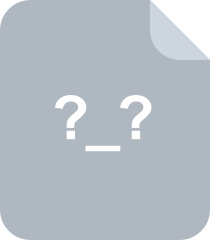

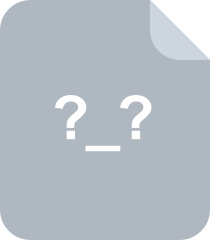
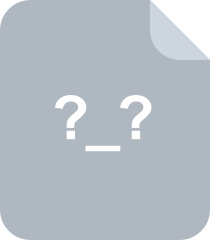

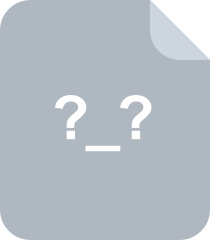
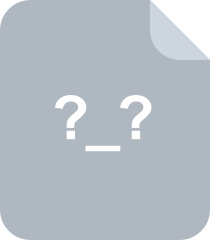
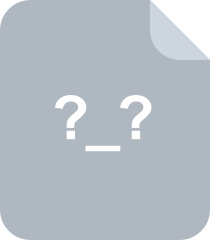
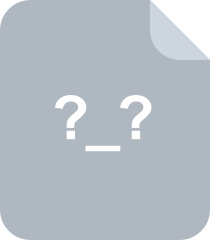

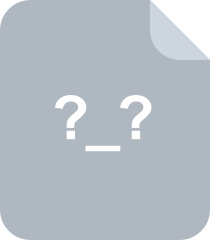
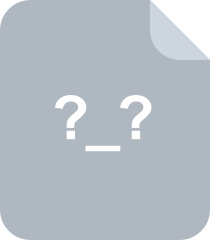

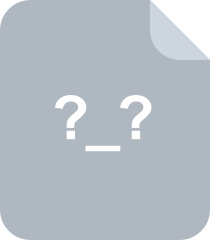
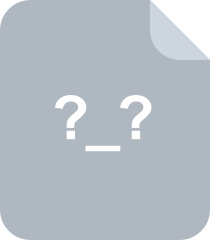

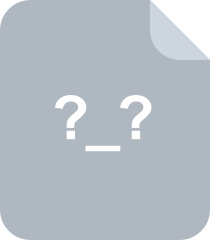
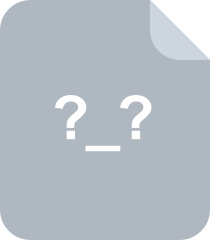
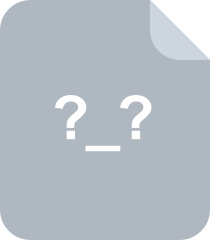
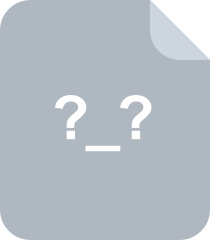
共 58 条
- 1
资源评论
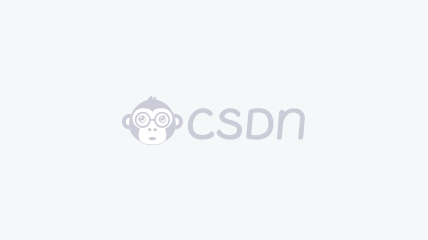

徐浪老师
- 粉丝: 8285
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

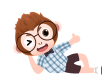
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


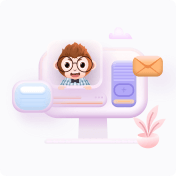
安全验证
文档复制为VIP权益,开通VIP直接复制
