# vue
[](https://godoc.org/github.com/norunners/vue)
Package `vue` is the progressive framework for [WebAssembly](https://github.com/golang/go/wiki/WebAssembly) applications.
## Install
```bash
GOARCH=wasm GOOS=js go get github.com/norunners/vue
```
*Requires Go 1.12 or higher.*
## Goals
* Provide a unified solution for a framework, state manager and router in the frontend space.
* Leverage [templating](https://github.com/norunners/vueg) to separate application logic from frontend rendering.
* Simplify data binding to ease the relation of state management to rendering.
* Encourage component reuse to promote development productivity.
* Follow an idiomatic Go translation of the familiar Vue API.
## Hello World!
The `main.go` file is compiled to a `.wasm` WebAssembly file.
```go
package main
import "github.com/norunners/vue"
type Data struct {
Message string
}
func main() {
vue.New(
vue.El("#app"),
vue.Template("<p>{{ Message }}</p>"),
vue.Data(Data{Message: "Hello WebAssembly!"}),
)
select {}
}
```
The `index.wasmgo.html` file fetches and runs a `.wasm` WebAssembly file.
```html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<script src="{{ .Script }}"></script>
</head>
<body>
<div id="app"></div>
<script src="{{ .Loader }}"></script>
</body>
</html>
```
*Note, the example above is compatible with [wasmgo](https://github.com/dave/wasmgo).*
## Serve Examples
Install `wasmgo` to serve examples.
```bash
go get -u github.com/dave/wasmgo
```
Serve an example [locally](http://localhost:8080/).
```bash
cd examples/01-declarative-rendering
wasmgo serve
```
## Status
Alpha - The state of this project is experimental until the common features of Vue are implemented.
The plan is to follow the Vue API closely except for areas of major simplification, which may lead to a subset of the Vue API.
During this stage, the API is expected to encounter minor breaking changes but increase in stability as the project progresses.
## F.A.Q.
#### Why Vue?
One of the common themes of existing frameworks is to combine component application logic with frontend rendering.
This can lead to a confusing mental model to reason about because both concerns may be mixed together in the same logic.
By design, Vue renders components with templates which ensures application logic is developed separately from frontend rending.
Another commonality of existing frameworks is to unnecessarily expose the relation of state management to rendering in the API.
By design, Vue binds data in both directions which ensures automatic updating and rendering when state changes.
This project aims to combine the simplicity of Vue with the power of Go WebAssembly.
License
-------
* [MIT License](LICENSE)
没有合适的资源?快使用搜索试试~ 我知道了~
WebAssembly 应用程序的渐进式框架 .zip
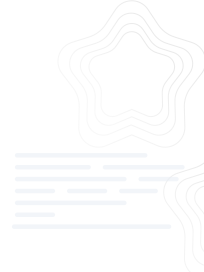
共40个文件
go:22个
html:12个
txt:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 115 浏览量
2024-12-01
16:23:45
上传
评论
收藏 24KB ZIP 举报
温馨提示
Vue 的Package是WebAssemblyvue应用程序的渐进式框架。安装GOARCH=wasm GOOS=js go get github.com/norunners/vue需要 Go 1.12 或更高版本。目标为前端空间的框架、状态管理器和路由器提供统一的解决方案。利用模板将应用程序逻辑与前端渲染分开。简化数据绑定以简化状态管理与渲染的关系。鼓励组件重用以提高开发效率。遵循熟悉的 Vue API 的惯用 Go 翻译。你好世界!该main.go文件被编译为.wasmWebAssembly 文件。package mainimport "github.com/norunners/vue"type Data struct { Message string}func main() { vue.New( vue.El("#app"), vue.Template("<p>{{ Message }}</p>"), vue.Data(Data{Message: "Hello WebAssembly!"}), ) sele
资源推荐
资源详情
资源评论
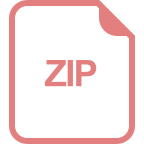
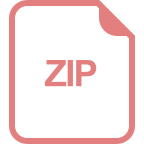
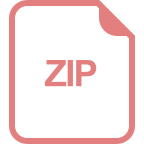
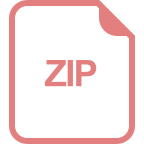
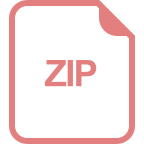
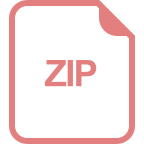
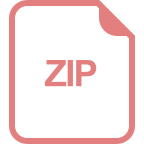
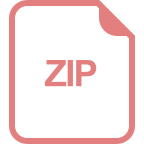
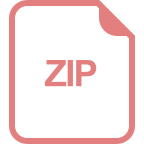
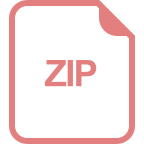
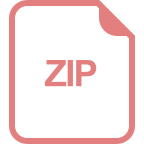
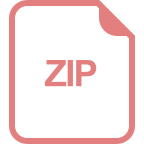
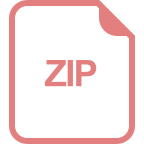
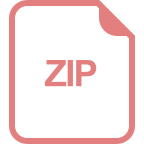
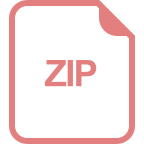
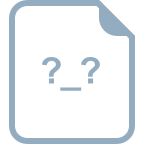
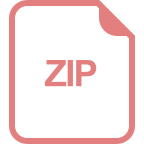
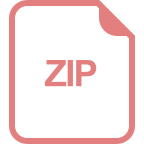
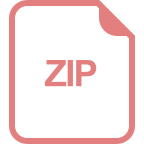
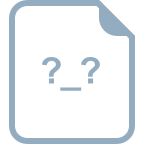
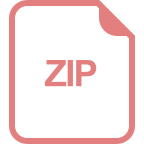
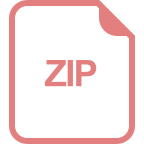
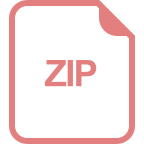
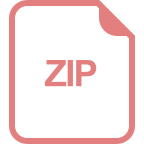
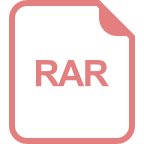
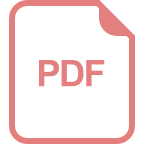
收起资源包目录

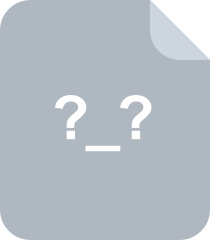
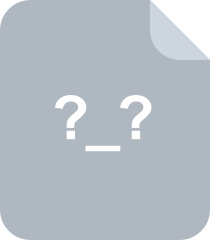
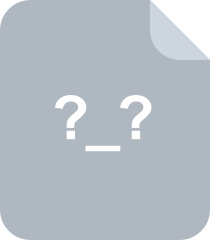
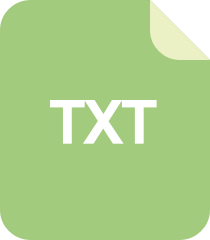
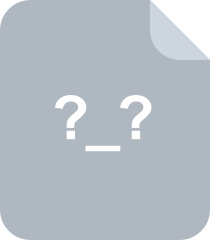
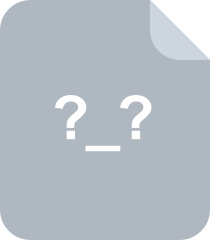
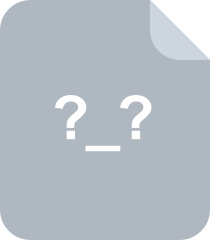


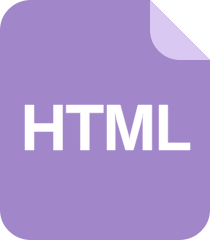
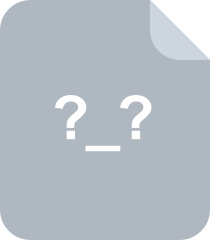

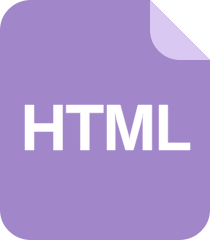
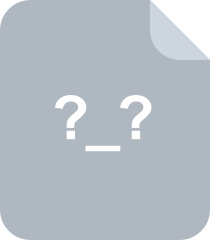

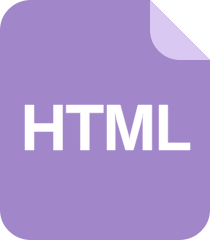
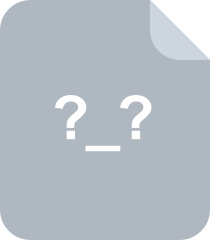

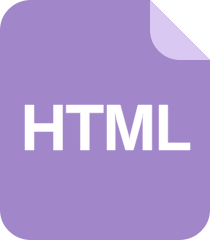
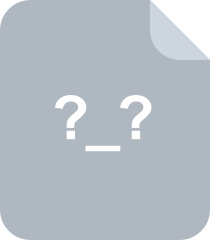

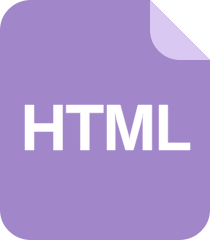
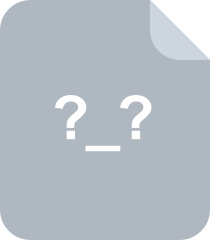

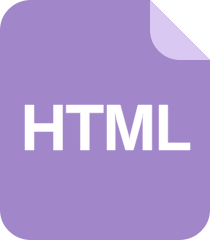
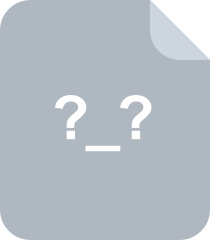

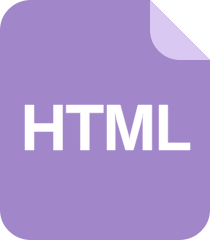
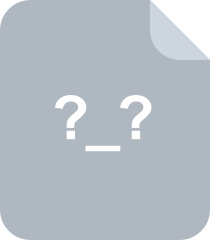

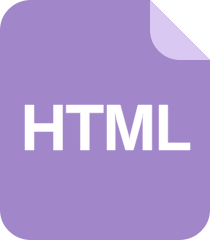
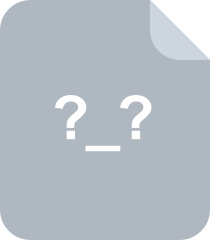

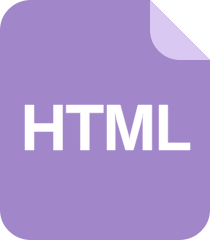
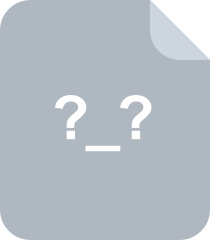

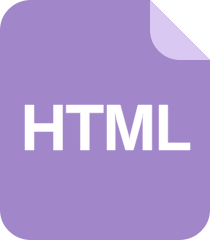
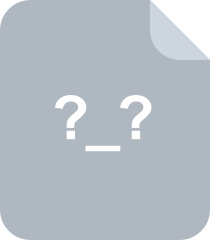

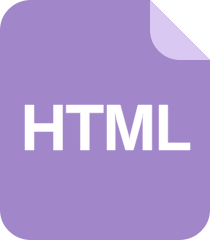
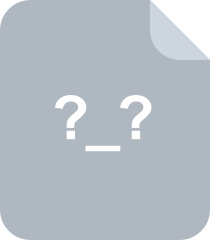

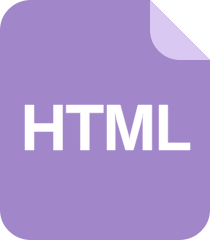
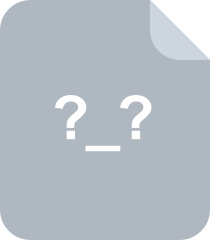
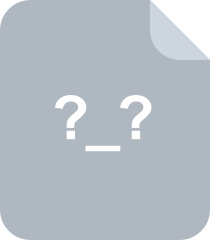
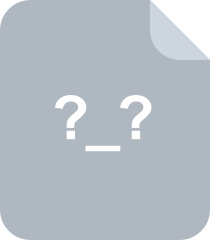
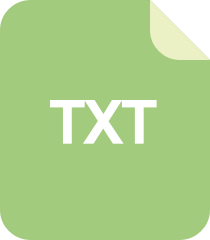
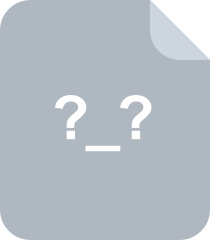
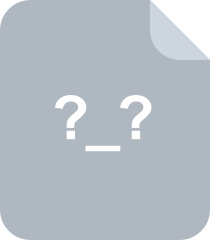
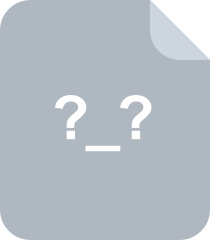
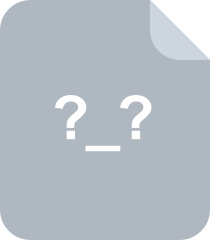
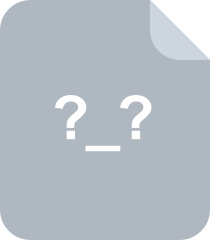
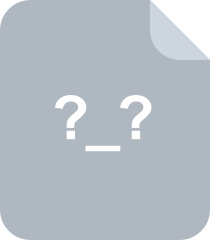
共 40 条
- 1
资源评论
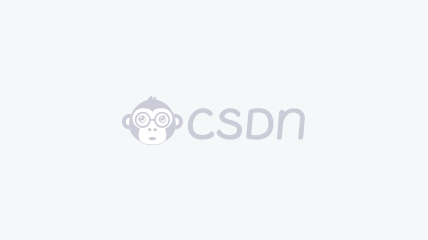

徐浪老师
- 粉丝: 8249
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

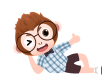
最新资源
- C语言核心知识点详解-数据类型、运算符、数组与指针综合应用
- 基于 SpringBoot 开发 BS 架构宠物健康咨询系统:从需求调研到上线运营全纪实
- 快乐星球0000000
- C#ASP.NET企业年终员工抽奖系统源码数据库 Access源码类型 WinForm
- 用 Fluent 进行飞机模拟
- C#ASP.NET程序软件销售网站源码数据库 SQL2008源码类型 WebForm
- 浅谈食盐与人体健康.docx
- jsppspsppspspspspspsps
- Python毕业设计基于知识图谱和生成式AI的智能食谱推荐系统源码.zip
- 基于 SpringBoot 开发网上蛋糕售卖店管理系统:从需求剖析到上线运营全解析
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


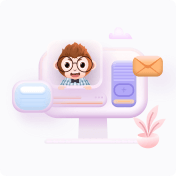
安全验证
文档复制为VIP权益,开通VIP直接复制
