# DirectHook
**DirectHook** is a library designed to intercept and modify DirectX API function calls, making it ideal for tasks like implementing ImGui overlays or custom graphics monitoring within applications.
### Supported APIs
DirectHook currently supports the following DirectX APIs:
- Direct3D 9, 10, 11, 12
- DirectDraw 7
### Planned Features
- **Cross-API Support**: Future versions may add support for non-DirectX graphics APIs such as OpenGL and Vulkan.
## Getting Started
### Samples
The **Samples** folder provides basic DLLs for each supported API, demonstrating DirectHook’s capabilities to intercept API function calls. Each sample includes:
- **Function Hooking**: Basic hooks for common DirectX functions.
- **ImGui Integration**: Samples show how to use DirectHook to implement an ImGui overlay, enabling custom in-game interfaces.
#### Sample Usage
To test a sample, you have two options:
1. Use the provided `DLLInjector` tool. This requires specifying the target process and the DLL path as command-line arguments:
```sh
DLLInjector.exe D3D11App.exe D3D11Hook.dll
```
2. For quick testing, directly add the DLL load call in your application code:
```cpp
LoadLibrary(L"D3D11Hook.dll");
```
### API Utilities
DirectHook provides two utility headers for each supported API:
- **Hook Indices**: Enumerations that help you specify which function to intercept.
- **Function Type Definitions**: Convenient typedefs for function pointers, making it easy to define custom functions that match DirectX’s API function signatures.
## Example: D3D11 Hook
The following example demonstrates how to use DirectHook to intercept the `Present` function in Direct3D 11, allowing for custom behavior on each frame render. This example could be extended to implement an ImGui overlay.
1. **Hook Indices**: Enumerated constants to specify the function to intercept:
```cpp
namespace directhook::d3d11
{
enum {
// Other indices...
SwapChain_Present = 8, // Index for Present call
// Other indices...
};
}
```
2. **Function Typedefs**: Aliases to simplify function pointer declarations:
```cpp
namespace directhook::d3d11 {
// Other typedefs...
using PFN_DXGISwapChain_Present = HRESULT(STDMETHODCALLTYPE*)(IDXGISwapChain*, UINT, UINT);
// Other typedefs...
}
```
3. **Hook Implementation**: Intercept `Present` call to add custom behavior.
```cpp
#include "directhook.h"
using namespace directhook;
static d3d11::PFN_DXGISwapChain_Present dxgiPresent = nullptr;
HRESULT STDMETHODCALLTYPE MyPresent(IDXGISwapChain* SwapChain, UINT SyncInterval, UINT Flags) {
static BOOL called = FALSE;
if (!called) {
MessageBoxA(0, "Called MyPresent!", "DirectHook", MB_OK);
called = TRUE;
}
return dxgiPresent(SwapChain, SyncInterval, Flags);
}
INT D3D11HookThread() {
if (DH_Status dh = DH_Initialize(); dh == Status::Success) {
Hook(d3d11::SwapChain_Present, dxgiPresent, MyPresent);
}
return 0;
}
BOOL WINAPI DllMain(HINSTANCE hInstance, DWORD fdwReason, LPVOID) {
DisableThreadLibraryCalls(hInstance);
if (fdwReason == DLL_PROCESS_ATTACH) {
CreateThread(NULL, 0, (LPTHREAD_START_ROUTINE)D3D11HookThread, NULL, 0, NULL);
}
return TRUE;
}
```
## Usage Guide
1. **Integrate DirectHook**: Add the DirectHook source folder to your DLL project.
2. **MinHook Dependency**: Link to MinHook libraries or add MinHook source code directly to your project.
3. **Select Graphics API**: Define the graphics API you’re targeting with the relevant `DH_USE_*` macro:
- **In Visual Studio**: Go to `Project -> Properties -> C/C++ -> Preprocessor -> Preprocessor Definitions` and add the desired macro.
- **In CMake**: Use `target_compile_definitions(MyDLL PRIVATE DH_USE_*)`.
- **Direct Declaration**: Alternatively, add the macro at the top of `directhook.h`.
## Screenshots
### The Talos Principle (D3D11)

### S.T.A.L.K.E.R. 2: Heart of Chornobyl (D3D12 UE 5.1)

没有合适的资源?快使用搜索试试~ 我知道了~
用于挂接 DirectX API 调用的库.zip
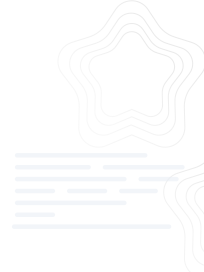
共152个文件
h:64个
cpp:45个
vcxproj:11个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 6 浏览量
2024-11-27
22:29:07
上传
评论
收藏 7.34MB ZIP 举报
温馨提示
直接挂钩DirectHook是一个用于拦截和修改 DirectX API 函数调用的库,非常适合在应用程序内实现 ImGui 覆盖或自定义图形监控等任务。支持的 APIDirectHook 当前支持以下 DirectX APIDirect3D 9、10、11、12直接绘图 7计划功能跨 API 支持未来版本可能会增加对非 DirectX 图形 API(例如 OpenGL 和 Vulkan)的支持。入门示例Samples文件夹为每个受支持的 API 提供了基本 DLL,展示了 DirectHook 拦截 API 函数调用的能力。每个示例包括函数挂钩常见 DirectX 函数的基本挂钩。ImGui 集成示例展示如何使用 DirectHook 实现 ImGui 覆盖,从而实现自定义游戏内界面。示例用法要测试样本,您有两种选择使用提供的DLLInjector工具。这需要指定目标进程和 DLL 路径作为命令行参数DLLInjector.exe D3D11App.exe D3D11Hook.dll为了快速测试,直接在应用程序代码中添加 DLL
资源推荐
资源详情
资源评论
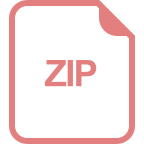
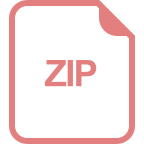
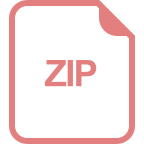
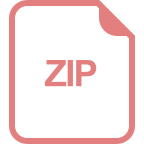
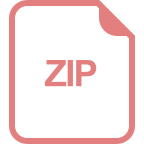
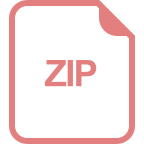
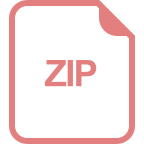
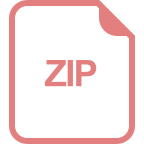
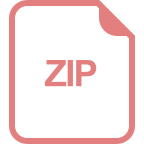
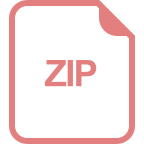
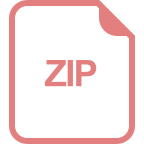
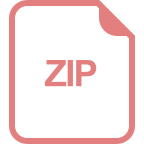
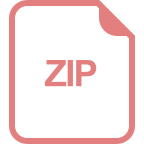
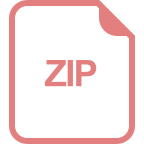
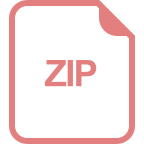
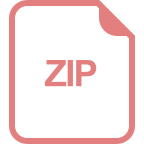
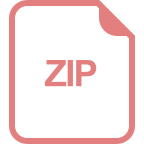
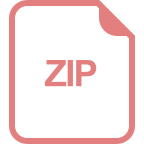
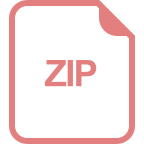
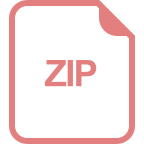
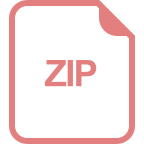
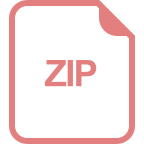
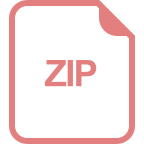
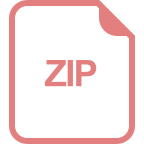
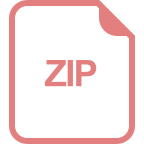
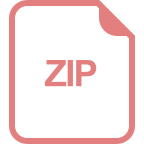
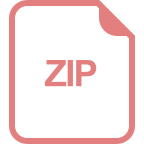
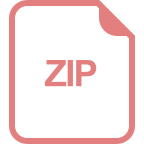
收起资源包目录

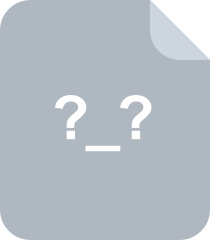
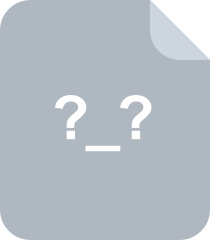
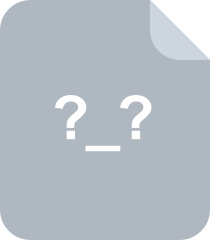
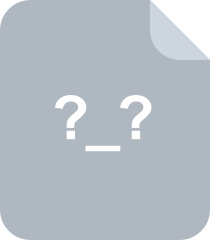
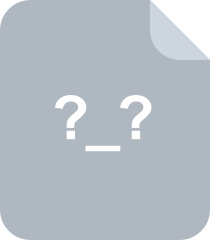
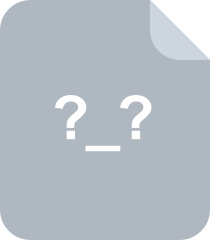
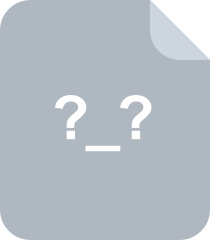
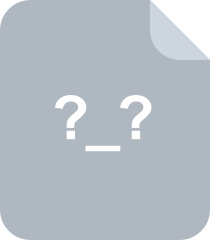
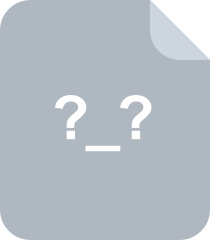
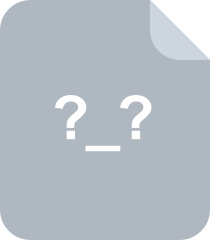
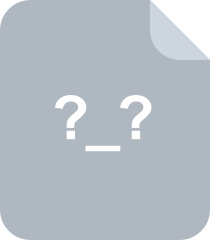
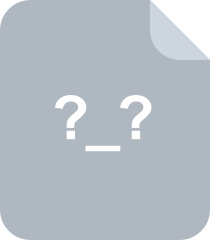
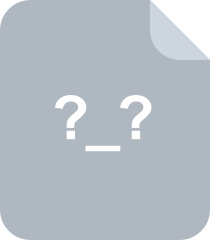
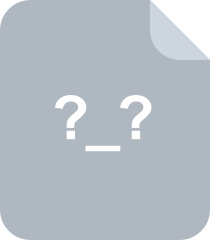
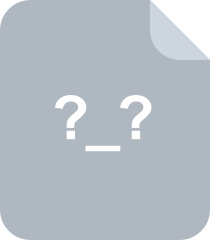
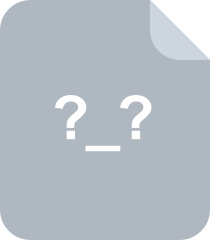
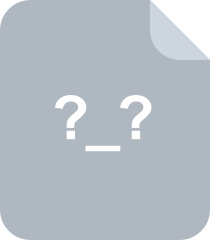
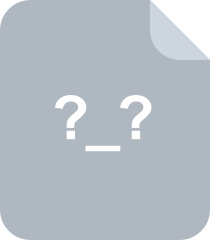
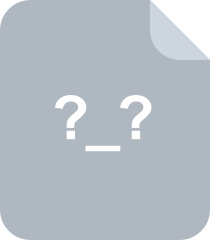
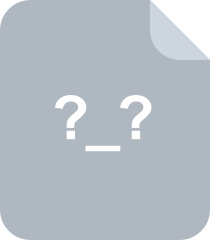
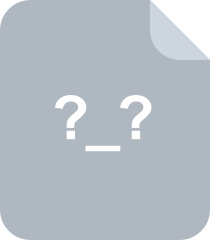
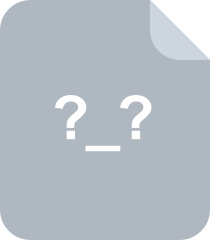
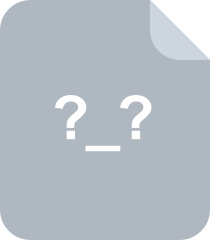
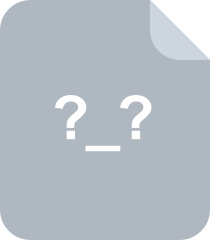
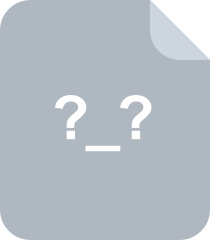
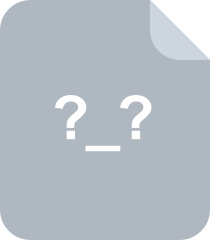
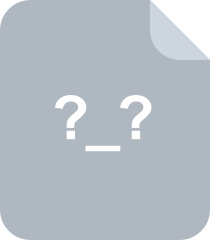
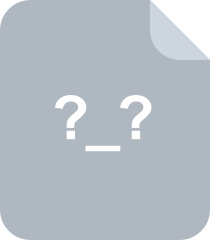
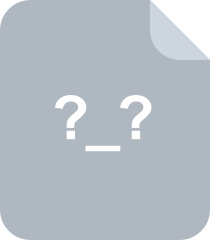
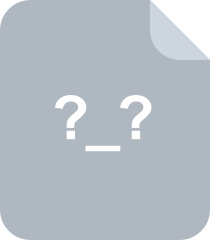
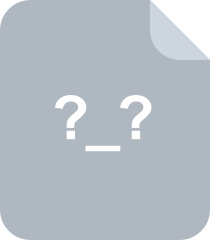
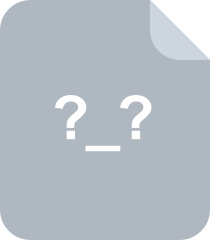
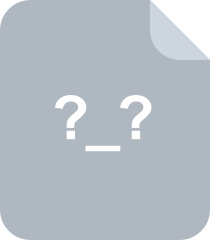
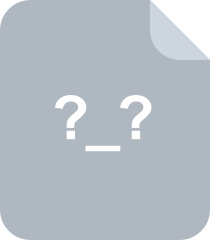
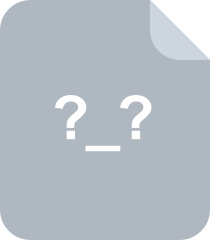
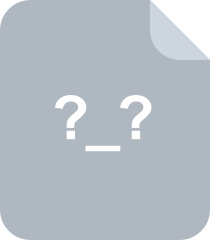
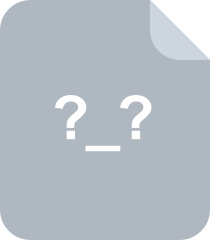
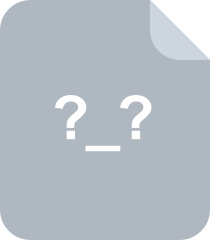
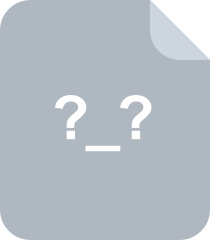
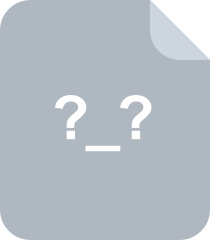
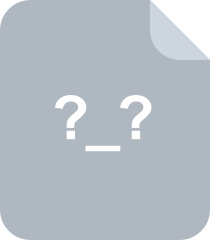
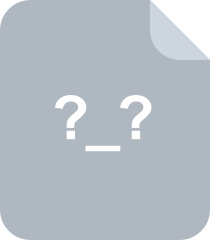
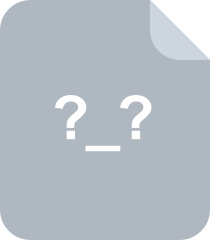
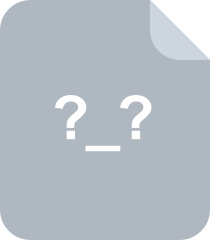
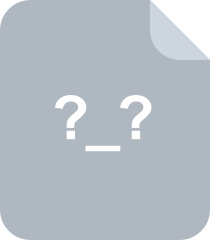
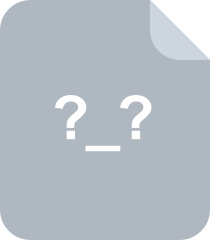
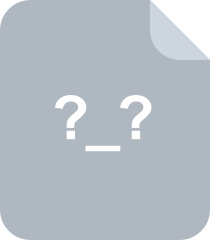
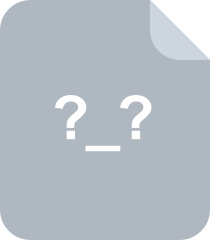
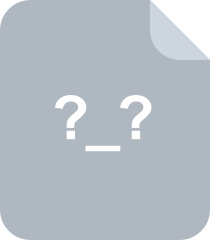
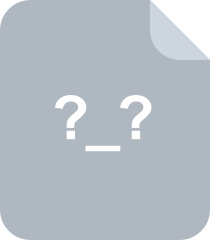
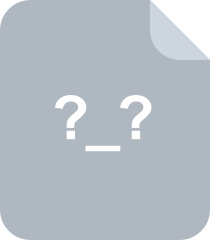
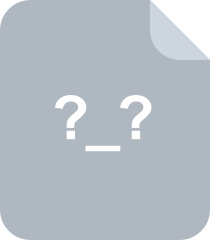
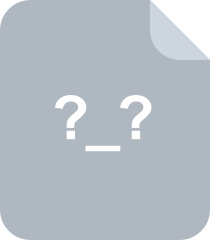
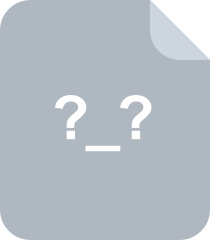
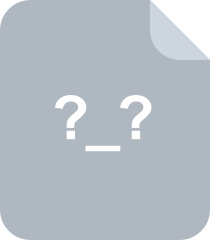
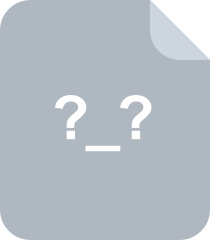
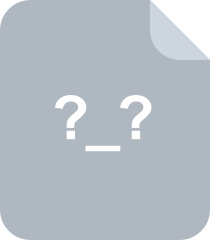
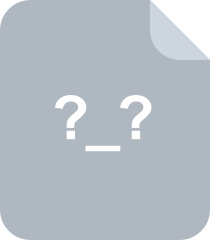
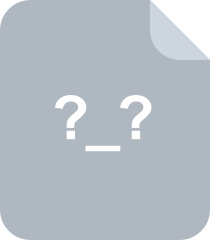
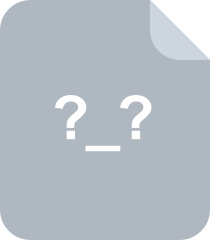
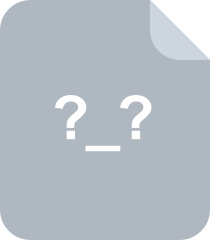
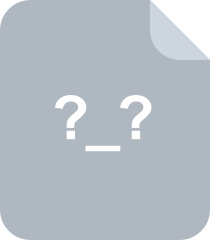
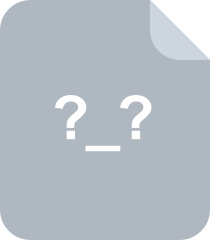
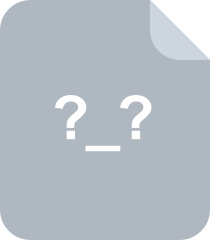
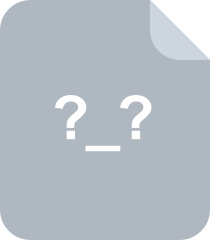
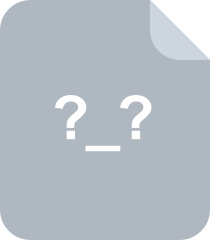
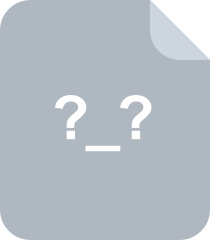
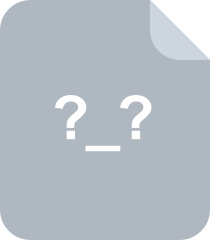
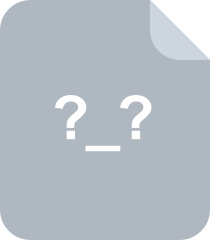
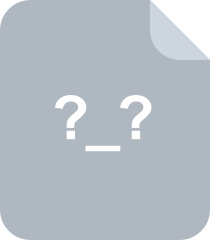
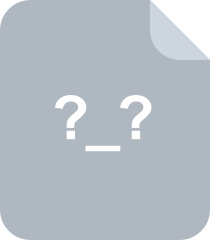
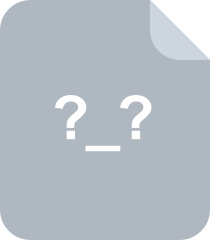
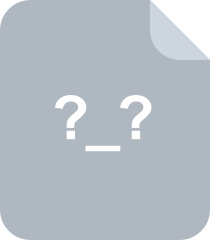
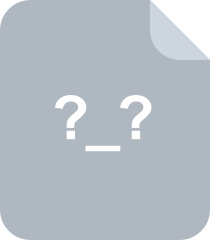
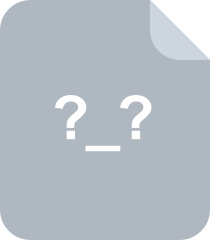
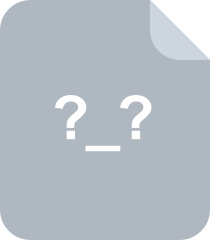
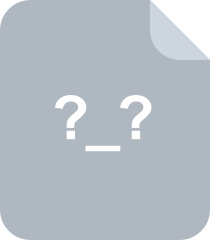
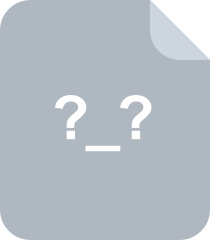
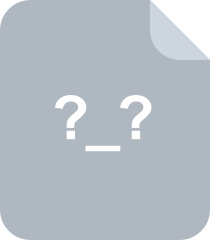
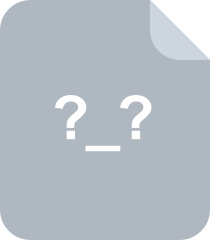
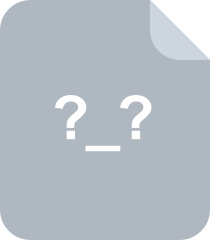
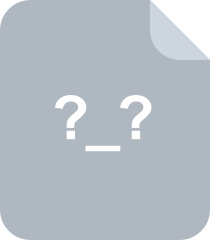
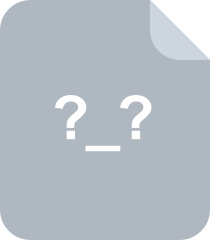
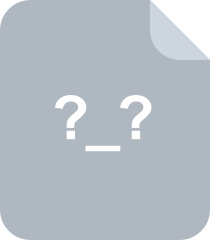
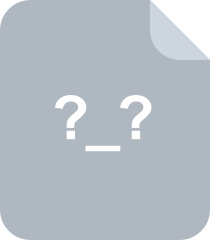
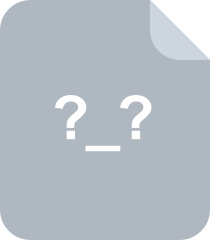
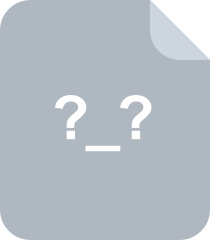
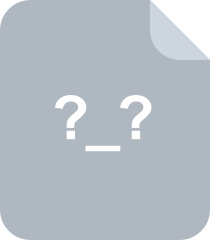
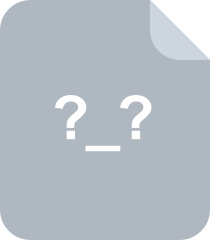
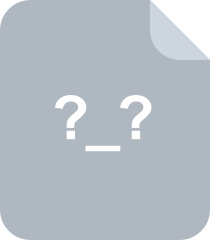
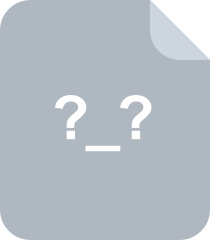
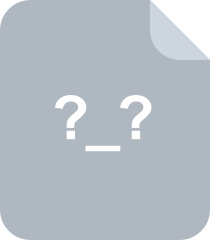
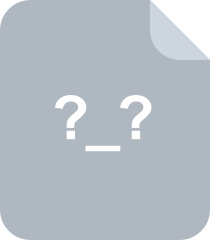
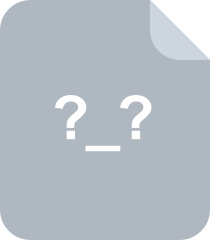
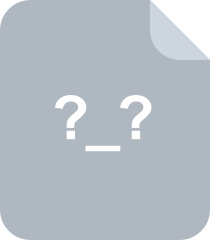
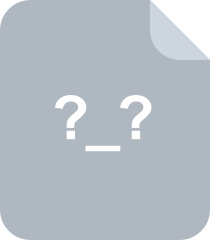
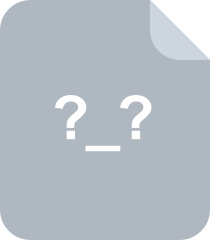
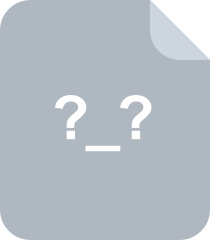
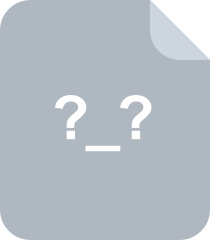
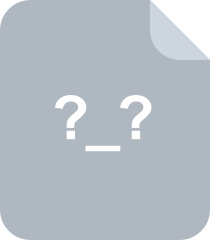
共 152 条
- 1
- 2
资源评论
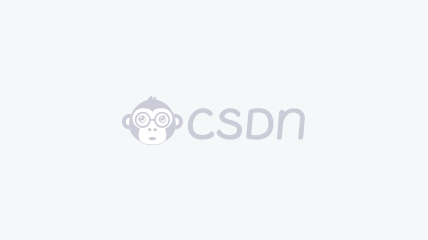

徐浪老师
- 粉丝: 8125
- 资源: 8383
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

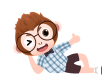
最新资源
- bdwptqmxgj11.zip
- onnxruntime-win-x86
- onnxruntime-win-x64-gpu-1.20.1.zip
- vs2019 c++20 语法规范 头文件 <ratio> 的源码阅读与注释,处理分数的存储,加减乘除,以及大小比较等运算
- 首次尝试使用 Win,DirectX C++ 中的形状渲染套件.zip
- 预乘混合模式是一种用途广泛的三合一混合模式 它已经存在很长时间了,但似乎每隔几年就会被重新发现 该项目包括使用预乘 alpha 的描述,示例和工具 .zip
- 项目描述 DirectX 引擎支持版本 9、10、11 库 Microsoft SDK 功能相机视图、照明、加载网格、动画、蒙皮、层次结构界面、动画控制器、网格容器、碰撞系统 .zip
- 项目 wiki 文档中使用的代码教程的源代码库.zip
- 面向对象的通用GUI框架.zip
- 基于Java语言的PlayerBase游戏角色设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


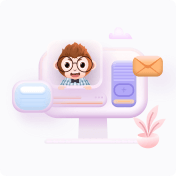
安全验证
文档复制为VIP权益,开通VIP直接复制
