# NGFX Graphics Framework
NGFX is a low level graphics framework, providing an abstraction API on
top of Vulkan, DirectX12, and Metal. It exposes the benefits of
next-generation graphics technology via a common platform abstraction
API, with minimal programming complexity. It also supports optional
access to the backend data structures, enabling platform specific
optimizations.
---
## Source Code
<https://github.com/gopro/ngfx>
---
## High Level Architecture

---
## Low Level Architecture

The ngfx graphics classes provide high-level abstractions on top of
various graphics objects, including Buffer, Texture, Renderpass,
Swapchain, Surface, CommandBuffer, etc. Each backend (Vulkan, Metal,
DirectX12) is responsible for implementing the high-level abstraction
interface via a subclass.
The user can construct 3D graphics commands using these high-level
abstractions. Optionally, the user can access the backend-specific
data by casting the high-level object to a backend-specific subclass.
For example:
```
Buffer *buffer = Buffer::create(...);
VKBuffer *vkBuffer = (VKBuffer*)buffer;
```
From there, the user can access platform specific data, including the
underlying Vulkan buffer object, etc. This provides a high degree of
flexibility, where the user can primarily use platform-agnostic code,
while still being able to use platform-specific code for
high-performance optimizations, and for achieving optimal interaction
with the platform.
## Modules

| Module | Description |
| ------ | ----------- |
| ComputePipeline | Programs the modules on the GPU related to compute operations. In particular, defines the compute shader input and various portions of the GPU pipeline layout.
| ComputeShader | Defines a programmable shader that can perform arbitrary computation.
| ComputeOp | Defines the base interface for compute operations. Provides support for performing one or more compute operations. Describes the inputs, outputs, intermediate buffers, and layout transitions.
| ComputeApplication | A helper class for creating an application that performs compute operations.
| GraphicsPipeline | Defines the input to the shader stages (vertex, fragment, geometry, tessellation, etc), and GPU pipeline layout.
| DrawOp | Defines the base class for draw operations.
| RenderPass | Provides support for performing one or more draw operations. Describes the inputs, outputs, intermediate buffers, and layout transitions.
| GraphicsCore | Defines various graphics data types such as BlendOp, BlendFactor, etc.
| FilterOp | Defines the base interface for image filters.
| MeshData | Defines the data for a 3D mesh.
| MeshUtil | Provides various mesh utility functions.
| Graphics | Defines various graphics commands via a high level abstraction API. Also provides various profiling functions.
| Surface | Defines a high-level abstraction for a platform surface. A surface is essentially a buffer of memory along with an associated pixel format.
| Texture | Defines a high-level abstraction for a GPU texture. A texture consists of an organized array of texels along with the texture format. In addition, a texture supports random sampling on the GPU. Supports various types of textures including 2D, 3D, 2D arrays, cubemaps, etc. Also supports mipmapping.
| Pipeline | Programs the GPU pipeline modules, including the input assembler, shader stages, rasterizer, blend parameters, etc.
| Fence | Supports GPU-CPU synchronization. Notifies the CPU when some operation on the GPU is completed.
| Camera | Provides an orbital camera that supports pan / tilt / zoom. Supports keyboard, mouse, and touch input
| ShaderModule | Defines the base class for programmable shader modules. A programmable shader allows the user to write code in a high-level shading language that performs arbitrary graphics or computation on the GPU. Supports precompiled shaders for optimal performance. Also supports runtime shader compilation although this usage is discouraged. Supports GLSL (in Vulkan backend), HLSL (in DirectX12 backend) and MSL (in Metal backend), along with the associated precompiled formats (spirv, dxc, metallib). The user can either program in a platform-specific shading language, or program in GLSL and the code can be auto-converted to HLSL / MSL for other platforms (see ShaderTools).
| ShaderTools | Provides support for auto-converting GLSL to HLSL / MSL. Uses spirv-cross internally to convert the shaders. Supports offline shader conversion for optimal performance and more importantly, validation. Also supports runtime shader conversion although this usage is discouraged.
| VertexShaderModule | Defines the shader input to the vertex shader module.
| FragmentShaderModule | Defines the shader input to the fragment shader module.
| ComputeShaderModule | Defines the shader input to the compute shader module
| Semaphore | Support GPU-GPU synchronization. Synchronizes GPU operations within or across command queues. The main difference between fences and semaphores is that fences can be accessed from your program (on the CPU) while semaphores are only accessible within the GPU.
| Barrier |
| GraphicsContext |
| Device | Defines a logical abstraction for a GPU device. Also has logic to query the devices in the system and choose the device based on capabilities, although this logic will probably be moved.
| Queue | Defines a logical abstraction for a GPU queue. Commands are recorded to CommandBuffers and submitted to the GPU via queues. Queues can support up to 3 uses: compute, transfer, and graphics.
| Swapchain | Manages a set of images (typically 2 or 3) that are presented to the display. Supports various present modes.
| Framebuffer | Supports rendering to one or more textures via attachments.
| Window | Provides a high-level window abstraction, and has logic for creating the window and handling resizing, input events, etc. NGFX currently supports various window backends: glfw, Windows, appkit, etc. NGFX also supports rendering directly to a surface (which can be existing window surface or an offscreen surface).
| CommandBuffer | Supports recording multiple commands and submitting them to the GPU. Supports both primary and secondary command buffers. Secondary command buffers can be recorded in parallel, using multiple threads, and added to a primary command buffer.
| Buffer | Supports GPU buffers. A buffer is an unorganized block of data. The same buffer can support multiple usage scenarios, including storing uniform data, vertex buffer data, index buffer data, etc. On shared memory architectures, supports shared CPU/GPU access. For discrete GPUs, supports uploading / downloading to dedicated GPU memory.
| BufferUtil | Provides various helper functions for creating specialized buffers.
| Config | Defines various configuration options.
| Application | Defines the base class for an application interface, which is an optional module that simplifies creating an application.
| File | Supports file operations.
| StringUtil | Supports various string operations.
| RegexUtil | Provides various regex utility functions.
| Timer | Provides a basic timer that measures elapsed time.
| FPSCounter | Supports measing FPS (frames per second).
| Util | Various utility functions.
| InputListener | Defines the base interface for an object that can respond to input events.
| InputMap | Provides a common abstraction for input codes (such as key codes).
---
## Build Instructions
**1) Install Dependencies**
1.1) Install Platform-Specific Dependencies
*On Windows 10 (or newer):*
Install Microsoft Visual Studio 2019 (or newer)
Install PowerShell Core version 7.1.3 (or newer)
*On Linux (Ubuntu 20.04.1 or newer, other Linux dist

徐浪老师
- 粉丝: 8525
- 资源: 1万+
最新资源
- 基于全阶磁链观测器的异步电机矢量控制 全阶磁链观测器的主要思想是将异步电机模型作为参考,把状态估计的方程作为可调节部分 这两部分具有相同物理意义的输出量,利用两个部分的输出量误差再经过反馈校
- 事件触发控制代码+对应参考文献 1.2023IEEE TRANS 顶刊基于事件触发的深度强化学习自动驾驶决策(CCF-A) 2.多智能体分布式系统的事件触发控制 3.基于观测器的非理想线性多智能体事件
- 序列相关同步检测电路,电子科技大学电子设计自动化实验代码
- 微调 MySQL 全文搜索.pdf
- 直接扩频序列通信技术仿真与设计实验 实验设计m序列和gold序列在不同信道当中进行仿真,最后得出关于信噪比和误码率的规律 扩频序列实验、码序列、通信工程课程设计、matlab,附带报告一份
- Money Pro for Mac v2.11.3
- 光伏储能同步发电机VSG并网仿真模型C 光伏阵列搭建的光伏电池模型 光伏:采用扰动观察法最大功率点MPPT跟踪控制 储能:蓄电池充放电控制,双向Buck Boost变器,采用直流母线电压外环控制稳定直
- Visual Studio Code 中的配置文件.pdf
- 遥感目标检测 TAS遥感目标检测数据集.zip
- STM32三相电压型SVPWM整流器仿真,以电压外环和电流内环控制,双闭环PID控制,输出电压600V 三相电压型SVPWM整流器仿真,以电压外环和电流内环控制,双闭环PID控制,输出电压600V
- 在本地计算机上开发并行 MATLAB代码并扩展到集群.pdf
- Mellel 6 for Mac v6.2.1
- 电力系统11个节点无功补偿仿真,功率因数和谐波可观察,线路阻抗参数可改,matlab2018b及以上(可改版)
- Ls-dyna 聚能爆破.研究聚能射流击穿钢板,本模型为二维模型,研究聚能射流对钢板的破坏形式.可查看聚能射流的应力变化情况
- 改进的紧凑拉伸试样的疲劳裂纹扩展分析 - ANSYS Workbench
- 永磁同步电机的MTPA最大转矩电流比控制算法+弱磁控制的仿真模型 (附带一份建模及说明文档) 1. 永磁同步电机的数学模型; 2. 永磁同步电机的矢量控制原理; 3. 最大转矩电流比控制; 4.
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


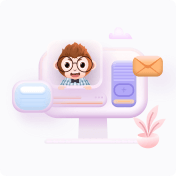