# JavaScriptBridge 
[](https://cocoapod-badges.herokuapp.com/v/JavaScriptBridge/badge.png)
[](https://cocoapod-badges.herokuapp.com/p/JavaScriptBridge/badge.png)
[](https://travis-ci.org/kishikawakatsumi/JavaScriptBridge)
[](https://github.com/igrigorik/ga-beacon)
Write iOS apps in Javascript! JavaScriptBridge provides the way to write iOS apps with JavaScript.
JavaScriptBridge bridges Cocoa touch to JavaScriptCore (JavaScriptCore.framework is introduced in iOS 7).
You get the power of dynamics of scripting language for your apps.
*It is still in development, obviously. You're welcomed to contribute if you find the project interesting!*
## Usage
```objc
#import <JavaScriptBridge/JavaScriptBridge.h>
...
// Retrieve the prepared context
JSContext *context = [JSBScriptingSupport globalContext];
// Add framework support if needed.
// ('Foundation', 'UIKit', 'QuartzCore' enabled by default.)
[context addScriptingSupport:@"MapKit"];
[context addScriptingSupport:@"MessageUI"];
// Evaluate script
[context evaluateScript:
@"var window = UIWindow.new();"
@"window.frame = UIScreen.mainScreen().bounds;"
@"window.backgroundColor = UIColor.whiteColor();"
@"window.makeKeyAndVisible();"
];
```
1. Retrieve the `JSContext` instance from `JSBScriptingSupport`.
The context includes a lot of system classes that has been `JSExports` adopted.
```objc
JSContext *context = [JSBScriptingSupport globalContext];
```
2. Add `JSExports` adopted classes each framework if needed.
By default, `Foundation`, `UIKit`, `QuartzCore` frameworks are included.
```objc
[context addScriptingSupport:@"MapKit"];
[context addScriptingSupport:@"MessageUI"];
```
3. It is ready to use, writing appliction code and evaluate in JavaScript.
```objc
[context evaluateScript:
@"var window = UIWindow.new();"
@"window.frame = UIScreen.mainScreen().bounds;"
@"window.backgroundColor = UIColor.whiteColor();"
@"window.makeKeyAndVisible();"
];
```
#### Manually setting up a new JSContext instance
1. Create new `JSContext` instance instead using `globalContext`.
You can separate JavaScript environments to use multiple contexts.
```objc
JSContext *context = [[JSContext alloc] init];
```
2. Add `JSExports` adopted classes each framework if needed.
`Foundation`, `UIKit` and `QuartzCore` frameworks **MUST** be added.
```objc
[context addScriptingSupport:@"Foundation"];
[context addScriptingSupport:@"UIKit"];
[context addScriptingSupport:@"QuartzCore"];
[context addScriptingSupport:@"Accounts"];
[context addScriptingSupport:@"Social"];
```
### Syntax / Naming conventions
**Class name**
Same as Objective-C
**Variable declaration**
Get rid of `Type name` instead use `var`
```objc
UILabel *label;
```
```javascript
var label;
```
**Properties**
Use dot syntax
```objc
UISlider *slider = [[UISlider alloc] initWithFrame:frame];
slider.backgroundColor = [UIColor clearColor];
slider.minimumValue = 0.0;
slider.maximumValue = 100.0;
slider.continuous = YES;
slider.value = 50.0;
```
```javascript
var slider = UISlider.alloc().initWithFrame(frame);
slider.backgroundColor = UIColor.clearColor();
slider.minimumValue = 0.0;
slider.maximumValue = 100.0;
slider.continuous = true;
slider.value = 50.0;
```
**Invoking method**
Use dot syntax
All colons are removed from the selector
Any lowercase letter that had followed a colon will be capitalized
```objc
UIWindow *window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
```
```javascript
var window = UIWindow.alloc().initWithFrame(UIScreen.mainScreen().bounds);
```
**Struct (CGRect, NSRange, etc.)**
Use Hashes
```objc
UIView *view = [UIView new];
view.frame = CGRectMake(20, 80, 280, 80);
CGFloat x = view.frame.origin.x;
CGFloat width = view.frame.size.width;
```
```javascript
var view = UIView.new();
view.frame = {x: 20, y: 80, width: 280, height: 80};
var x = view.frame.x; // => 20
var width = view.frame.width; // => 280
```
## Examples
###Hello world on JavaScriptBridge
This is the most simplest way.
```objc
@implementation JSBAppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
JSContext *context = [JSBScriptingSupport globalContext];
[context evaluateScript:
@"var window = UIWindow.new();"
@"window.frame = UIScreen.mainScreen().bounds;"
@"window.backgroundColor = UIColor.whiteColor();"
@""
@"var navigationController = UINavigationController.new();"
@"var viewController = UIViewController.new();"
@"viewController.navigationItem.title = 'Make UI with JavaScript';"
@""
@"var view = UIView.new();"
@"view.backgroundColor = UIColor.redColor();"
@"view.frame = {x: 20, y: 80, width: 280, height: 80};"
@""
@"var label = UILabel.new();"
@"label.backgroundColor = UIColor.blueColor();"
@"label.textColor = UIColor.whiteColor();"
@"label.text = 'Hello World.';"
@"label.font = UIFont.boldSystemFontOfSize(24);"
@"label.sizeToFit();"
@""
@"var frame = label.frame;"
@"frame.x = 10;"
@"frame.y = 10;"
@"label.frame = frame;"
@""
@"view.addSubview(label);"
@"viewController.view.addSubview(view);"
@""
@"navigationController.viewControllers = [viewController];"
@""
@"window.rootViewController = navigationController;"
@"window.makeKeyAndVisible();"
];
return YES;
}
@end
```
Of course, the script is able to be loaded from external file.
```objc
@implementation JSBAppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
NSBundle *mainBundle = [NSBundle mainBundle];
NSString *path = [mainBundle pathForResource:@"main" ofType:@"js"];
NSString *script = [NSString stringWithContentsOfFile:path encoding:NSUTF8StringEncoding error:nil];
JSContext *context = [JSBScriptingSupport globalContext];
[context evaluateScript:script];
return YES;
}
@end
```
###Writing apps with only JavaScript
See the [UICatalog](https://github.com/kishikawakatsumi/JavaScriptBridge/tree/master/Examples/UICatalog/UICatalog) example.
## Enhancements
###Define custom classes
You can define custom class in JavaScript.
It is needs to interact system provided framework.
`JSB.defineClass(declaration, instanceMembers, staticMembers)` function defines Objective-C class in JavaScript.
Pass the class declaration string to first argument.
Second argument is instance method definitions as hash.
The hash object inclueds function object, each keys are to be used as method name.
**Example**
```javascript
var MainViewController = JSB.defineClass('MainViewController : UITableViewController', {
// Instance Method Definitions
viewDidLoad: function() {
self.navigationItem.title = 'UICatalog';
},
viewWillAppear: function(animated) {
self.tableView.reloadData();
}
}, {
// Class Method Definitions
attemptRotationToDeviceOrientation: function() {
...
}
});
```
**Example**
```javascript
var MainViewController = JSB.defineClass('MainViewController : UITableViewController <UITableviewDataSource, UITableviewDelegate>', // Declaration
// Instance Method Definitions
{
viewDidLoad: function() {
self.navigationItem.title = 'UICatalog';
},
tableViewNumberOfRowsInSection: function(tableView, section) {
return self.menuList.length;
},
tableViewCellForRowAtIndexPath: function(tableView, indexPath) {
var cell = UITableViewCell.alloc().initWithStyleReuseIdentifier(3, 'Cell');
cell.ac

徐浪老师
- 粉丝: 8683
- 资源: 1万+
最新资源
- 高阶AI指令大合集!.zip
- DeepSeek零基础到精通手册(保姆级教程).zip
- DeepSeek使用攻略.zip
- 【官网提示库】探索 DeepSeek 提示词样例,挖掘更多可能.zip
- 《7天精通DeepSeek实操手册》.zip
- 教大家如何使用Deepseek AI进行超级降维知识输出V1.0版.zip
- DeepSeek 15天指导手册-从入门到精通.zip
- 10天精通+DeepSeek+实操手册.zip
- 112页!DeepSeek 7大场景+50大案例+全套提示词 从入门到精通干货-202502.zip
- Deepseek+V3从零基础到精通学习手册(1).zip
- OMO2203class3面向对象.mp4
- 三相逆变器下垂控制参数调整与波形质量分析报告:直流侧电压800V,交流侧电压220V,开关频率达10kHz,模拟调频工况下性能表现优越,三相逆变器下垂控制参数调整与波形质量分析报告:直流侧电压800V
- 工具变量-上市公司企业绿色创新泡沫数据(1995-2023年).txt
- Simulink Simscape中的UR5机械臂三次多项式轨迹规划仿真:动态动画展示角度、力矩与运动参数图,六自由度UR5机械臂Simulink Simscape三次多项式轨迹规划仿真动画及数据图表
- 基于FPGA与Matlab算法的超声多普勒频移解调系统:DDS生成信号、混合与滤波处理、FFT运算及峰值搜索比对,基于FPGA和MATLAB的超声多普勒频移解调技术:DDS生成信号、混频处理、滤波、F
- 松下FP-XH双PLC 10轴摆盘程序范例:清晰分输出与调试、报警通信、启动复位,维纶通触摸屏操作,一年平稳运行经验分享,松下FP-XH双PLC 10轴摆盘程序范例:清晰思路,易学易懂,带触摸屏与通信
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


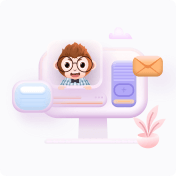