# JavaReedSolomon
This is a simple and efficient Reed-Solomon implementation in Java,
which was originally built at [Backblaze](https://www.backblaze.com).
There is an overview of how the algorithm works in my [blog
post](https://www.backblaze.com/blog/reed-solomon/).
The ReedSolomon class does the encoding and decoding, and is supported
by Matrix, which does matrix arithmetic, and Galois, which is a finite
field over 8-bit values.
For examples of how to use ReedSolomon, take a look at SampleEncoder
and SampleDecoder. They show, in a very simple way, how to break a
file into shards and encode parity, and then how to take a subset of
the shards and reconstruct the original file.
There is a Gradle build file to make a jar and run the tests. Running
it is simple. Just type: `gradle build`
We would like to send out a special thanks to James Plank at the
University of Tennessee at Knoxville for his useful papers on erasure
coding. If you'd like an intro into how it all works, take a look at
[this introductory paper](http://web.eecs.utk.edu/~plank/plank/papers/SPE-9-97.html).
This project is limited to a pure Java implementation. If you need
more speed, and can handle some assembly-language programming,
you may be interested in using the Intel SIMD instructions to speed
up the Galois field multiplication. You can read more about that
in the paper on [Screaming Fast Galois Field Arithmetic](http://www.kaymgee.com/Kevin_Greenan/Publications_files/plank-fast2013.pdf).
## Performance Notes
The performance of the inner loop depends on the specific processor
you're running on. There are twelve different permutations of the
loop in this library, and the ReedSolomonBenchmark class will tell
you which one is faster for your particular application. The number
of parity and data shards in the benchmark, as well as the buffer
sizes, match the usage at Backblaze. You can set the parameters of
the benchmark to match your specific use before choosing a loop
implementation.
These are the speeds I got running the benchmark on a Backblaze
storage pod:
```
ByteInputOutputExpCodingLoop 95.2 MB/s
ByteInputOutputTableCodingLoop 107.0 MB/s
ByteOutputInputExpCodingLoop 130.3 MB/s
ByteOutputInputTableCodingLoop 181.4 MB/s
InputByteOutputExpCodingLoop 94.4 MB/s
InputByteOutputTableCodingLoop 138.3 MB/s
InputOutputByteExpCodingLoop 200.4 MB/s
InputOutputByteTableCodingLoop 525.7 MB/s
OutputByteInputExpCodingLoop 143.7 MB/s
OutputByteInputTableCodingLoop 209.5 MB/s
OutputInputByteExpCodingLoop 217.6 MB/s
OutputInputByteTableCodingLoop 515.7 MB/s
```


徐浪老师
- 粉丝: 8507
- 资源: 1万+
最新资源
- 电子学习资料设计作品全资料红外遥控电路设计资料
- 基于Python爬虫、Echarts与情感分析的电影票房及评分数据采集与可视化项目源码.zip
- 基于 NodeJS Express 框架开发的动态网站项目(含源码与说明,首页功能已实现).zip
- 基于python+turtle生日快乐弹框满屏(完整代码)
- 基于 Snort、Barnyard 和 Basenetwork 的入侵检测系统部署与应用(含源码与说明).zip
- 基于PyTorch深度学习的加密图像检索系统源码+设计文档资料(含图像分类标记).zip
- 基于C语言的人工智能与自动化照片管理编辑系统+设计报告及资料(课程设计).zip
- 2019“创青春・交子杯”新网银行高校金融科技挑战赛AI算法赛道语义识别项目python源码+全部资料.zip
- 毕业设计基于Flume_kafka_spark设计与实现的电商网站日志分析系统-最新开发(含全新源码+设计报告及资料).zip
- 基于机器学习随机森林分类提取gram特征对android恶意代码检测-最新开发(含全新源码+设计报告及资料).zip
- 2020“创青春・交子杯”新网银行金融科技挑战赛AI算法赛道pytorch版项目代码及完整资料.zip
- 前后端分离项目-基于vue+Springboot+MongoDB构建的教学排课系统-最新开发(含全新源码+设计报告及资料).zip
- 课程作业基于MATLAB、SIMULINK的互耦水槽液位控制的PID整定方法比较-最新开发(含全新源码+设计报告).zip
- 课程设计基于JAVA设计实现的中国象棋游戏-可局域网联机对战-最近开发(全新源码+设计报告及资料).zip
- 人工智能课设基于机器学习-随机森林的GNSS欺骗检测系统-最近开发(含全新源码+完整资料+设计报告).zip
- python基于BERT+BiLSTM+CRF算法实现法律文书命名实体识别系统-最新开发(全新源码+设计报告及资料).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


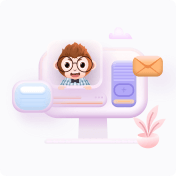