# [python-shell](https://www.npmjs.com/package/python-shell) [](https://ci.appveyor.com/project/Almenon/python-shell/branch/master) [](https://codecov.io/gh/extrabacon/python-shell)
<!-- change above url according to repo -->
A simple way to run Python scripts from Node.js with basic but efficient inter-process communication and better error handling.
## Features
+ Reliably spawn Python scripts in a child process
+ Built-in text, JSON and binary modes
+ Custom parsers and formatters
+ Simple and efficient data transfers through stdin and stdout streams
+ Extended stack traces when an error is thrown
## Requirements
First make sure you are able to run `python3` (Mac/Linux) or `python` (Windows) from the terminal. If you are not then you might need to add it to the PATH. If you want to use a version of python not in the PATH you should specify `options.pythonPath`.
## Installation
```bash
npm install python-shell
```
## Documentation
### Running python code:
```typescript
import {PythonShell} from 'python-shell';
PythonShell.runString('x=1+1;print(x)', null).then(messages=>{
console.log('finished');
});
```
If the script exits with a non-zero code, an error will be thrown.
Note the use of imports! If you're not using typescript ಠ_ಠ you can [still get imports to work with this guide](https://github.com/extrabacon/python-shell/issues/148#issuecomment-419120209).
Or you can use require like so:
```javascript
let {PythonShell} = require('python-shell')
```
### Running a Python script:
```typescript
import {PythonShell} from 'python-shell';
PythonShell.run('my_script.py', null).then(messages=>{
console.log('finished');
});
```
If the script exits with a non-zero code, an error will be thrown.
### Running a Python script with arguments and options:
```typescript
import {PythonShell} from 'python-shell';
let options = {
mode: 'text',
pythonPath: 'path/to/python',
scriptPath: 'path/to/my/scripts',
args: ['value1', 'value2', 'value3']
};
PythonShell.run('my_script.py', options).then(messages=>{
// results is an array consisting of messages collected during execution
console.log('results: %j', messages);
});
```
### Exchanging data between Node and Python:
```typescript
import {PythonShell} from 'python-shell';
let pyshell = new PythonShell('my_script.py');
// sends a message to the Python script via stdin
pyshell.send('hello');
pyshell.on('message', function (message) {
// received a message sent from the Python script (a simple "print" statement)
console.log(message);
});
// end the input stream and allow the process to exit
pyshell.end(function (err,code,signal) {
if (err) throw err;
console.log('The exit code was: ' + code);
console.log('The exit signal was: ' + signal);
console.log('finished');
});
```
Use `.send(message)` to send a message to the Python script. Attach the `message` event to listen to messages emitted from the Python script.
Use `options.mode` to quickly setup how data is sent and received between your Node and Python applications.
* use `text` mode for exchanging lines of text ending with a [newline character](http://hayne.net/MacDev/Notes/unixFAQ.html#endOfLine).
* use `json` mode for exchanging JSON fragments
* use `binary` mode for anything else (data is sent and received as-is)
Stderr always uses text mode.
For more details and examples including Python source code, take a look at the tests.
### Error Handling and extended stack traces
An error will be thrown if the process exits with a non-zero exit code. Additionally, if "stderr" contains a formatted Python traceback, the error is augmented with Python exception details including a concatenated stack trace.
Sample error with traceback (from test/python/error.py):
```
Traceback (most recent call last):
File "test/python/error.py", line 6, in <module>
divide_by_zero()
File "test/python/error.py", line 4, in divide_by_zero
print 1/0
ZeroDivisionError: integer division or modulo by zero
```
would result into the following error:
```typescript
{ [Error: ZeroDivisionError: integer division or modulo by zero]
traceback: 'Traceback (most recent call last):\n File "test/python/error.py", line 6, in <module>\n divide_by_zero()\n File "test/python/error.py", line 4, in divide_by_zero\n print 1/0\nZeroDivisionError: integer division or modulo by zero\n',
executable: 'python',
options: null,
script: 'test/python/error.py',
args: null,
exitCode: 1 }
```
and `err.stack` would look like this:
```
Error: ZeroDivisionError: integer division or modulo by zero
at PythonShell.parseError (python-shell/index.js:131:17)
at ChildProcess.<anonymous> (python-shell/index.js:67:28)
at ChildProcess.EventEmitter.emit (events.js:98:17)
at Process.ChildProcess._handle.onexit (child_process.js:797:12)
----- Python Traceback -----
File "test/python/error.py", line 6, in <module>
divide_by_zero()
File "test/python/error.py", line 4, in divide_by_zero
print 1/0
```
## API Reference
#### `PythonShell(script, options)` constructor
Creates an instance of `PythonShell` and starts the Python process
* `script`: the path of the script to execute
* `options`: the execution options, consisting of:
* `mode`: Configures how data is exchanged when data flows through stdin and stdout. The possible values are:
* `text`: each line of data is emitted as a message (default)
* `json`: each line of data is parsed as JSON and emitted as a message
* `binary`: data is streamed as-is through `stdout` and `stdin`
* `formatter`: each message to send is transformed using this method, then appended with a newline
* `parser`: each line of data is parsed with this function and its result is emitted as a message
* `stderrParser`: each line of logs is parsed with this function and its result is emitted as a message
* `encoding`: the text encoding to apply on the child process streams (default: "utf8")
* `pythonPath`: The path where to locate the "python" executable. Default: "python3" ("python" for Windows)
* `pythonOptions`: Array of option switches to pass to "python"
* `scriptPath`: The default path where to look for scripts. Default is the current working directory.
* `args`: Array of arguments to pass to the script
* `stdoutSplitter`: splits stdout into chunks, defaulting to splitting into newline-seperated lines
* `stderrSplitter`: splits stderr into chunks, defaulting to splitting into newline-seperated lines
Other options are forwarded to `child_process.spawn`.
PythonShell instances have the following properties:
* `script`: the path of the script to execute
* `command`: the full command arguments passed to the Python executable
* `stdin`: the Python stdin stream, used to send data to the child process
* `stdout`: the Python stdout stream, used for receiving data from the child process
* `stderr`: the Python stderr stream, used for communicating logs & errors
* `childProcess`: the process instance created via `child_process.spawn`
* `terminated`: boolean indicating whether the process has exited
* `exitCode`: the process exit code, available after the process has ended
Example:
```typescript
// create a new instance
let shell = new PythonShell('script.py', options);
```
#### `#defaultOptions`
Configures default options for all new instances of PythonShell.
Example:
```typescript
// setup a default "scriptPath"
PythonShell.defaultOptions = { scriptPath: '../scripts' };
```
#### `#run(script, options)`
Runs the Python script and returns a promise. When you handle the promise the argument will be an array of messages emitted from the Python script.
Example:
```typescript
// run a simple script
PythonShell.run('script.py', null).then(results => {
// script finished
}

徐浪老师
- 粉丝: 8492
- 资源: 1万+
最新资源
- 路由与交换技术-第13讲.pptx
- 路由与交换技术-第11讲.pptx
- 路由与交换技术-第14讲.pptx
- 2006-2022年各省农民专业合作社数量数据.xlsx
- SXU-操作系统实验报告
- 价值500元的个人分发源码 带安卓系统+自动识别苹果系统
- 基于springboot的大学生就业服务平台源码(java毕业设计完整源码).zip
- 负荷需求响应模型 基于Logistic函数 采用matlab编程,考虑电价激励下的乐观响应和悲观响应,利用负荷需求响应模型得到峰转平、平转谷的实际负荷转移率,从而得到基于Logistic函数的负荷转移
- 在win32汇编环境中,在对话框里生成richedit控件
- java上传资源-CSDN博客.html上传资源-CSDN博客.html上传资源-CSDN博客.html
- HTML5实现喜庆的新年快乐网页源码.zip
- 永磁同步电机反步(backstepping)控制 1.采用非线性控制策略反步控制法,实现永磁同步电机系统的完全解耦,相比PI控制减少了系统调节参数,抗负载扰动能力明显提高; 2.提供算法对应的参考文献
- 个人存档记录,energy transportation论文实现代码,基于matlab platemo平台
- 基于springboot的电子招投标系统源码(java毕业设计完整源码).zip
- 搭建私域流量十大注意要点
- 基于springboot的高校食堂移动预约点餐系统源码(java毕业设计完整源码).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


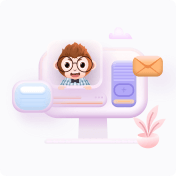