<p align="center">
<img src="logo.png" alt="logo"/>
</p>
# Learn Python 3
## Introduction
This repository contains a collection of materials for teaching/learning Python 3 (3.10+).
#### Requirements
* Have Python 3.10 or newer installed. You can check the version by typing `python3 --version` in your command line. You can download the latest Python version from [here](https://www.python.org/downloads/).
* Have [Jupyter Notebook installed](http://jupyter.readthedocs.io/en/latest/install.html). `pip install jupyter` is sufficient in most cases.
If you can not access Python and/or Jupyter Notebook on your machine, you can still follow the web based materials. However, you should be able to use Jupyter Notebook in order to complete the exercises.
#### Usage (locally)
1. Clone or download this repository.
2. Run `jupyter notebook` command in your command line in the repository directory.
3. Jupyter Notebook session will open in the browser and you can start navigating through the materials.
#### Usage (Binder in the cloud)
You can also just use [Binder:](https://mybinder.org/) By clicking of this [](https://mybinder.org/v2/gh/jerry-git/learn-python3/master) badge, the project is opened in a Jupyter instance in the cloud and you can then navigate to the folders containing the notebooks and start them each and interactively explore them!
#### Contributing
See [contributing](https://github.com/jerry-git/learn-python3/blob/master/CONTRIBUTING.md) guide.
## Beginner
1. [Strings](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/01_strings.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/01_strings.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/01_strings_exercise.ipynb)
2. [Numbers](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/02_numbers.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/02_numbers.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/02_numbers_exercise.ipynb)
3. [Conditionals](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/03_conditionals.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/03_conditionals.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/03_conditionals_exercise.ipynb)
4. [Lists](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/04_lists.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/04_lists.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/04_lists_exercise.ipynb)
5. [Dictionaries](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/05_dictionaries.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/05_dictionaries.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/05_dictionaries_exercise.ipynb)
6. [For loops](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/06_for_loops.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/06_for_loops.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/06_for_loops_exercise.ipynb)
7. [Functions](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/07_functions.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/07_functions.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/07_functions_exercise.ipynb)
8. [Testing with pytest - part 1](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/08_testing1.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/08_testing1.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/08_testing1_exercise.ipynb)
9. Recap exercise 1 [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/09_recap1_exercise.ipynb)
10. [File I\O](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/10_file_io.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/10_file_io.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/10_file_io_exercise.ipynb)
11. [Classes](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/11_classes.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/11_classes.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/11_classes_exercise.ipynb)
12. [Exceptions](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/12_exceptions.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/12_exceptions.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/12_exceptions_exercise.ipynb)
13. [Modules and packages](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/13_modules_and_packages.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/13_modules_and_packages.ipynb)
14. [Debugging](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/14_debugging.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/14_debugging.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/14_debugging_exercise.ipynb)
15. [Goodies of the Standard Library - part 1](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/15_std_lib.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/15_std_lib.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/15_std_lib1_exercise.ipynb)
16. [Testing with pytest - part 2](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/16_testing2.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/16_testing2.ipynb) [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/16_testing2_exercise.ipynb)
17. [Virtual environment](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/17_venv.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/17_venv.ipynb)
18. [Project structure](https://jerry-git.github.io/learn-python3/notebooks/beginner/html/18_project_structure.html) [[notebook]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/notebooks/18_project_structure.ipynb)
19. Recap exercise 2 [[exercise]](http://nbviewer.jupyter.org/github/jerry-git/learn-python3/blob/master/notebooks/beginner/exercises/19_recap2_exercise.ipynb)
## Intermediate
#### Idiomatic Python
Python is a powerful language which contains
没有合适的资源?快使用搜索试试~ 我知道了~
用于教学,学习 Python 3 的 Jupyter 笔记本.zip
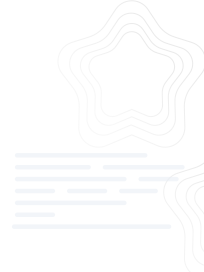
共98个文件
ipynb:42个
html:24个
txt:11个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 17 浏览量
2024-11-23
21:32:47
上传
评论
收藏 3.08MB ZIP 举报
温馨提示
学习 Python 3介绍该存储库包含用于教授/学习 Python 3 (3.10+) 的材料集合。要求python3 --version已安装 Python 3.10 或更新版本。您可以通过在命令行中输入来检查版本。您可以从此处下载最新的 Python 版本。安装Jupyter Notebookpip install jupyter在大多数情况下就足够了。如果您无法在计算机上访问 Python 和/或 Jupyter Notebook,您仍然可以按照基于网络的资料进行操作。但是,您应该能够使用 Jupyter Notebook 来完成练习。使用情况(本地)克隆或下载此存储库。jupyter notebook在存储库目录中的命令行中运行命令。Jupyter Notebook 会话将在浏览器中打开,您可以开始浏览材料。使用情况(云端 Binder)您也可以使用Binder通过单击此徽章,项目将在云中的 Jupyter 实例中打开,然后您可以导航到包含笔记本的文件夹并启动它们并以交互方式探索它们!贡献参见贡献指南。初学者字符串 [笔记本] [
资源推荐
资源详情
资源评论























收起资源包目录

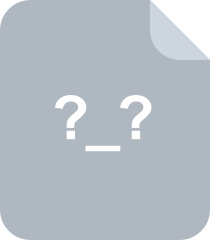


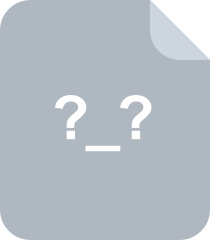
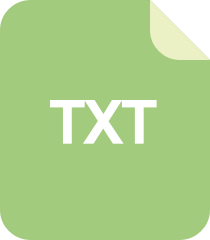
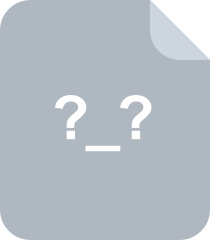
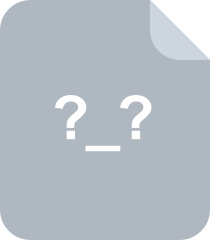
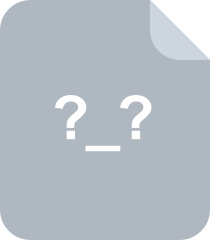
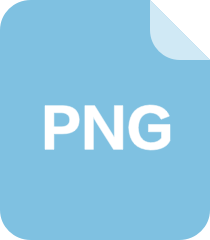
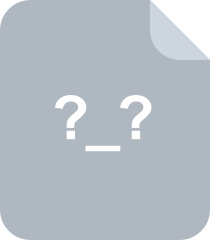
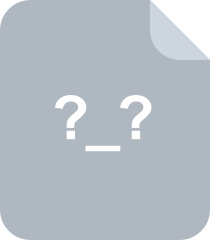
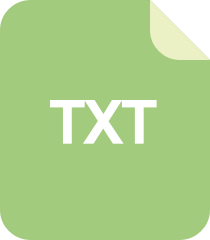
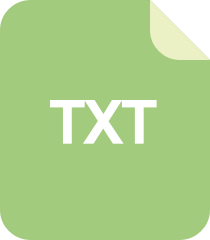
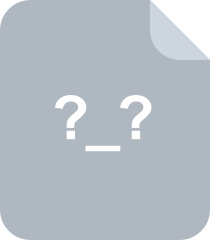
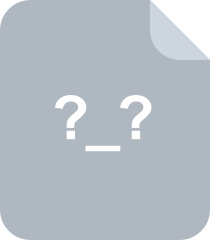



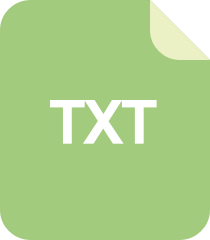
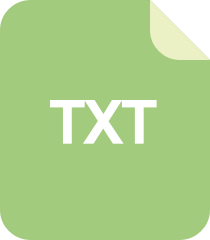
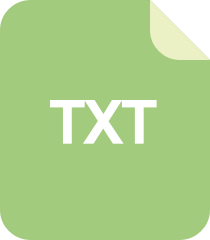

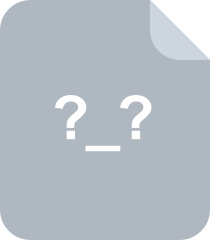
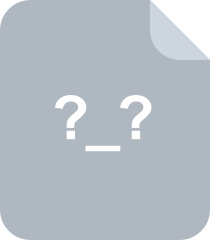

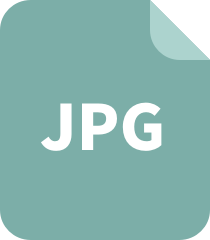
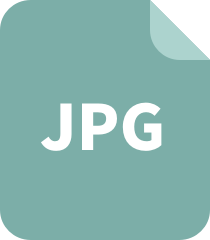
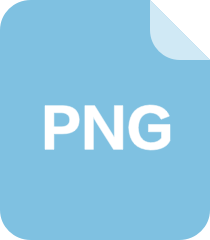
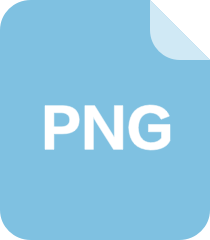
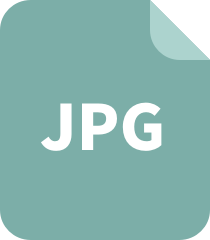
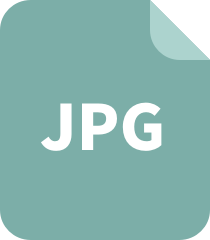
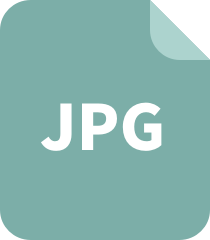
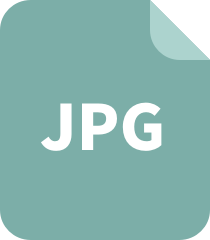
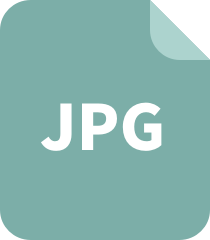
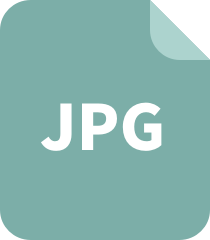

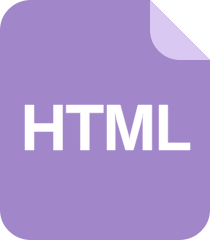
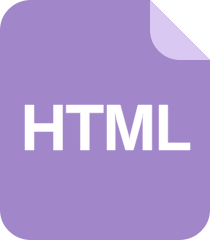
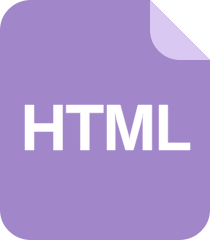
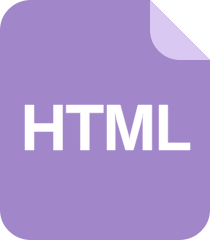
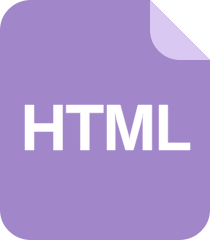
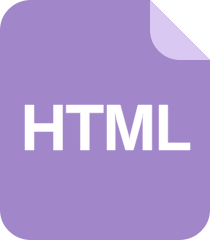
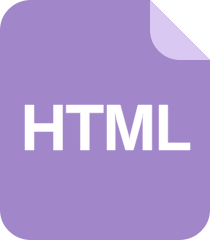

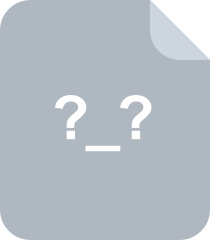
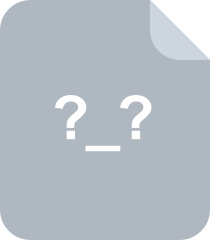
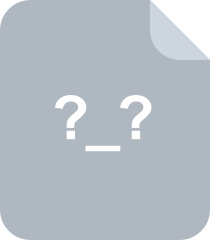
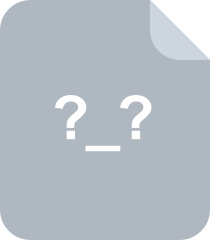
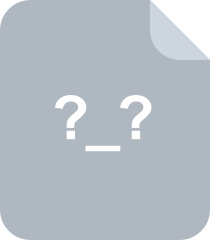
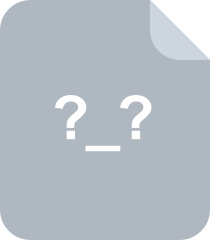
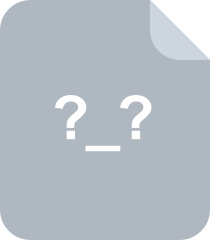


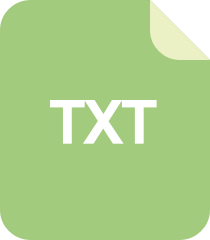
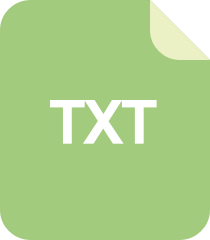
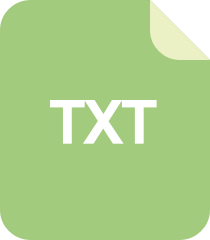
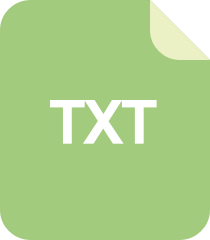
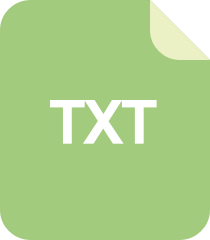

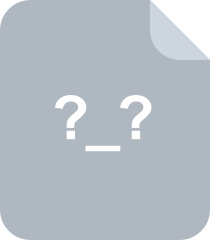
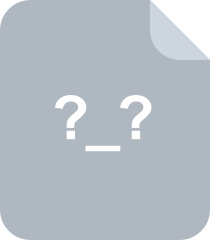
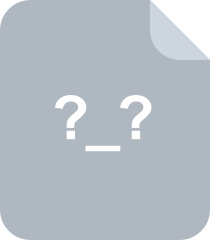
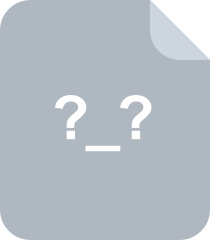
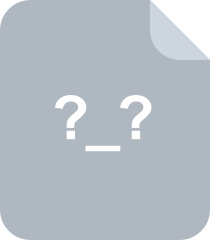
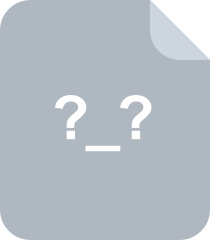
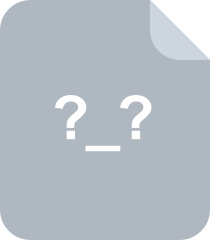
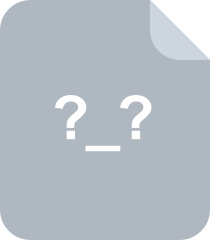
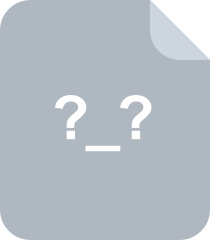
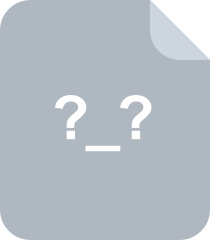
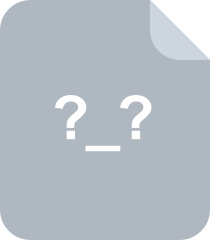
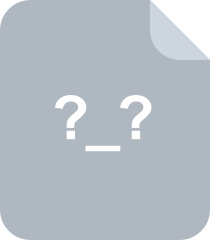
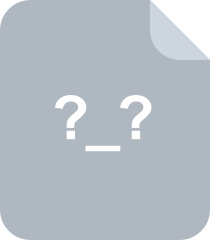
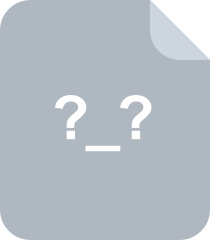
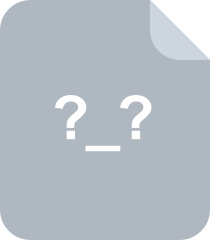
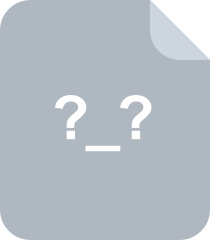

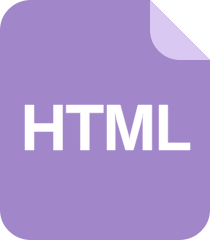
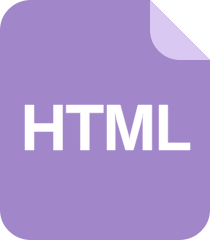
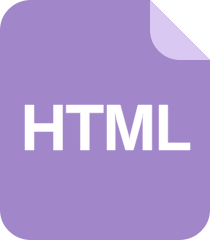
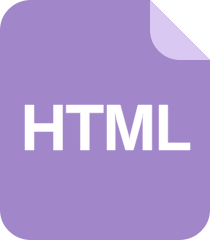
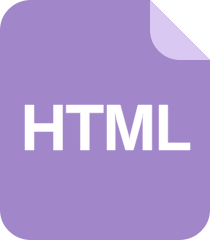
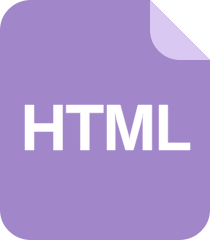
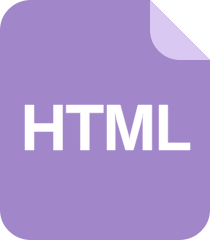
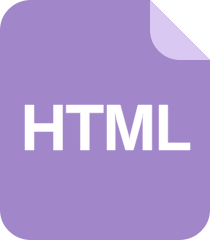
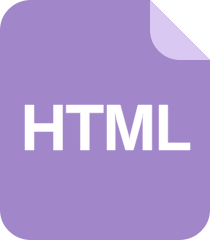
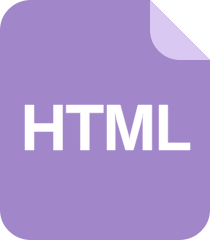
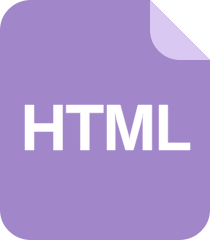
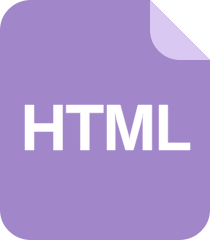
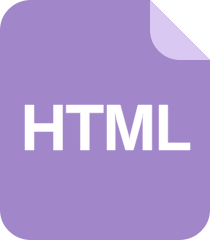
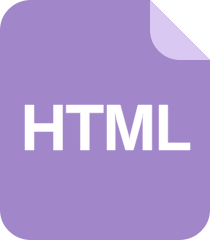
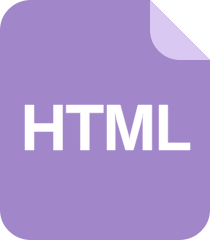
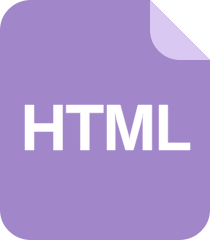
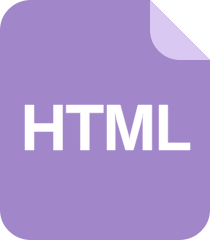

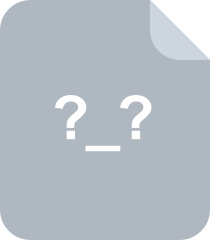
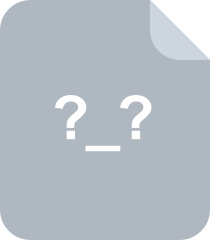
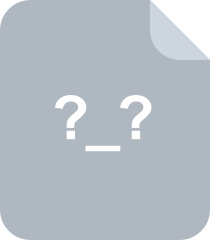
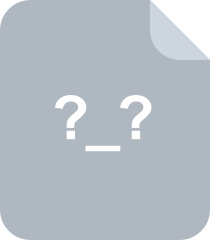
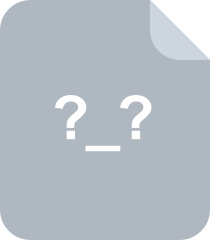
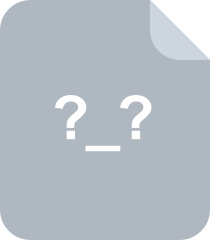
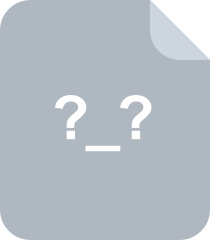
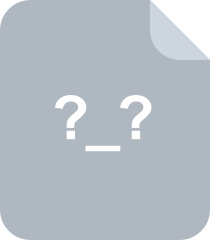
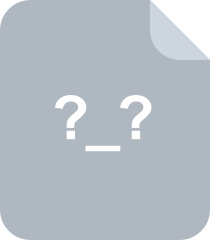
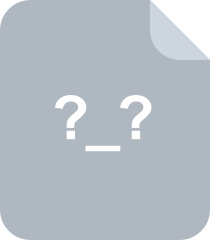
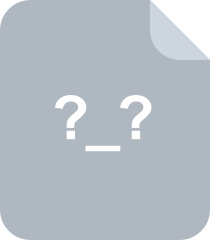
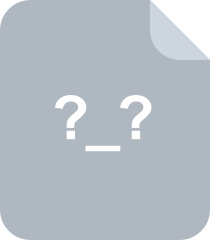
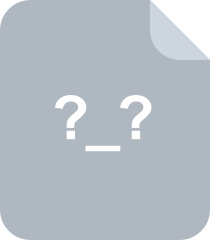
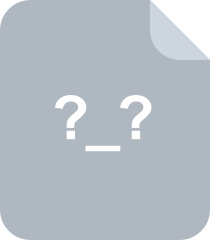
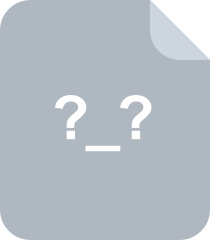
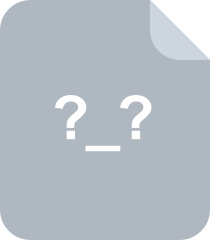
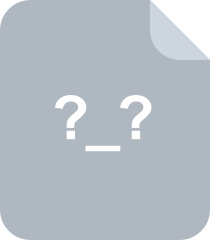

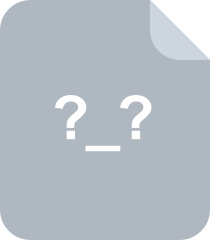
共 98 条
- 1
资源评论
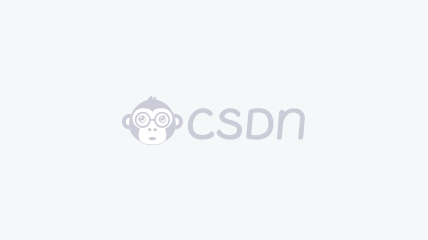

徐浪老师
- 粉丝: 8689
- 资源: 1万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

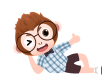
最新资源
- 《ACM-ICPC 的参考资料》(毕业设计,源码,教程)简单部署即可运行 功能完善、操作简单,适合毕设或课程设计.zip
- CO2驱替甲烷的深度探讨与研究基于Comsol数值模拟分析,基于COMSOL软件的二氧化碳驱替甲烷技术研究,comsol 二氧化碳驱替甲烷 ,COMSOL模拟; 二氧化碳驱替; 甲烷浓度变化; 数值模
- 基于stm32和proteus的家居环境采集仿真设计,采集家居环境的信温湿度、光照值以及气体检测(源码+报告+演示视频)
- 《2021 数模美赛O奖论文及代码公开》(毕业设计,源码,教程)简单部署即可运行 功能完善、操作简单,适合毕设或课程设计.zip
- dvanced archive password recovery pro v4.54
- 《2024蓝桥杯嵌入式学习资料》(毕业设计,源码,教程)简单部署即可运行 功能完善、操作简单,适合毕设或课程设计.zip
- JavaEE框架及关键技术的设计与实现指南:面向企业级应用
- MATLAB Simulink仿真研究:永磁同步电机FOC矢量控制与DTC矢量控制的动静态性能对比分析,MATLAB Simulink仿真研究:永磁同步电机FOC矢量控制与DTC矢量控制的动静态性能对
- 液压.zip
- 一体空间站 1.zip
- STM32学习标准库实现STM32 ADC采集1路、2路、多路
- STEP7-MicroWIN-SMART-V2.8库包括模拟量比例换算指令库
- 银河系加昼夜地球.zip
- 银河系扩展包2.81.3—1.5移植版(1.4移植1.5).zip
- 银河系扩展包2.6by航天星辰.zip
- 宇航员.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


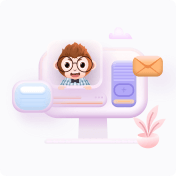
安全验证
文档复制为VIP权益,开通VIP直接复制
