package examination.controller;
import examination.entity.ChoiceQuestion;
import examination.entity.JudgeQuestion;
import examination.entity.Paper;
import examination.entity.Question.Choicedba;
import examination.entity.Question.Judgedba;
import examination.entity.SubjectQuestion;
import examination.service.ChartService;
import examination.entity.Question.Subdba;
import examination.service.ExamService;
import examination.service.ExerciseService;
import examination.service.PaperService;
import examination.service.TeacherService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.util.FileCopyUtils;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/teacher")
public class TeacherController {
final static String path = "teacher/";
@Autowired
TeacherService teacherService;
@Autowired
PaperService paperService;
@Autowired
ChartService chartService;
@Autowired
ExerciseService exerciseService;
@Autowired
ExamService examService;
@RequestMapping(value = "")
String index(Model model, HttpSession httpSession) {
return "redirect:" + path + "teacherpage";
}
@RequestMapping(value = "teacherpage")
String adminpage(Model model, HttpSession httpSession) {
model.addAttribute("name", httpSession.getAttribute("name"));
model.addAttribute("permission", httpSession.getAttribute("permission"));
return path + "teacherpage";
}
@RequestMapping(value = "question_list")
String questionList(Model model, HttpServletRequest request) {
model.addAttribute("page", paperService.getPage(request.getParameter("currentPage"),"choice"));
model.addAttribute("choiceQuestuon", paperService.getChoiceQuestion());
return path + "question_list";
}
@RequestMapping(value = "truefalse_list")
String truefalseList(Model model, HttpServletRequest request) {
model.addAttribute("page", paperService.getPage(request.getParameter("currentPage"),"judge"));
model.addAttribute("judgeQuestuon", paperService.getJudgeQuestion());
return path + "truefalse_list";
}
@RequestMapping(value = "sub_list")
String subList(Model model, HttpServletRequest request) {
model.addAttribute("page", paperService.getPage(request.getParameter("currentPage"),"sub"));
model.addAttribute("subjectQuestuon", paperService.getSubjectQuestion());
return path + "sub_list";
}
@RequestMapping(value = "get_paper")
String getPaper(Model model) {
model.addAttribute("choiceQuestuon", paperService.getAllChoiceQuestion());
return path + "choice_combine";
}
@RequestMapping(value = "/{type}/combine")
public String question(@PathVariable String type, HttpServletRequest request,Model model) {
HttpSession session = request.getSession();
if ("choice".equals(type)) {
session.setAttribute("choi", request.getParameter("choice"));
model.addAttribute("judgeQuestuon", paperService.getAllJudgeQuestion());
return path + "judge_combine";
} else if ("judge".equals(type)) {
session.setAttribute("judg", request.getParameter("judge"));
model.addAttribute("subjectQuestuon", paperService.getAllSubjectQuestion());
return path + "sub_combine";
} else if ("sub".equals(type)) {
session.setAttribute("sub", request.getParameter("sub"));
return path + "other_config";
}
return "error";
}
@RequestMapping(value = "{type}/delete")
@ResponseBody()
boolean deleteQuestion(@PathVariable String type, long id) {
if ("choice".equals(type)) {
return teacherService.deleteChoiceQuestion(id) != 0;
} else if ("judge".equals(type)) {
return teacherService.deleteJudgeQuestion(id) != 0;
} else if ("sub".equals(type)) {
return teacherService.deleteSubjectQuestion(id) != 0;
}
return false;
}
@RequestMapping(value = "{type}/delete_batch")
@ResponseBody()
boolean deleteQuestionBatch(@PathVariable String type, @RequestParam("list[]") List<Long> list) {
if ("choice".equals(type)) {
return teacherService.deleteChoiceQuestionBatch(list) != 0;
} else if ("judge".equals(type)) {
return teacherService.deleteJudgeQuestionBatch(list) != 0;
} else if ("sub".equals(type)) {
return teacherService.deleteSubjectQuestionBatch(list) != 0;
}
return false;
}
//update之所以不按照上面套路写作{type}/update,是因为springmvc需要把传入的参数映射为
// ChoiceQuestion等对象,分开写才能对于不同的对象映射。
@RequestMapping(value = "choice/update")
@ResponseBody()
boolean updateChoiceQuestion(ChoiceQuestion choiceQuestion) {
return teacherService.updateChoiceQuestion(choiceQuestion) != 0;
}
@RequestMapping(value = "judge/update")
@ResponseBody()
boolean updateJudgeQuestion(JudgeQuestion judgeQuestion) {
return teacherService.updateJudgeQuestion(judgeQuestion) != 0;
}
@RequestMapping(value = "sub/update")
@ResponseBody()
boolean updateSubjectQuestion(SubjectQuestion subjectQuestion) {
return teacherService.updateSubjectQuestion(subjectQuestion) != 0;
}
@RequestMapping(value = "paper_finish")
@ResponseBody
boolean finishPaper(HttpServletRequest request) {
HttpSession session = request.getSession();
String name = request.getParameter("name");
String begintime = request.getParameter("begintime");
String finishtime = request.getParameter("finishtime");
String choi = (String) session.getAttribute("choi");
String judg = (String) session.getAttribute("judg");
String sub = (String) session.getAttribute("sub");
long tid = (long) session.getAttribute("userid");
String classid = request.getParameter("classid");
String choiscore="";
for (int i = 0; i < choi.split(",").length; i++) {
choiscore+="4,";
}
choiscore=choiscore.substring(0,choiscore.length()-1);
String judgscore="";
for (int i = 0; i < judg.split(",").length; i++) {
judgscore +="4,";
}
judgscore=judgscore.substring(0,judgscore.length()-1);
String subscore="";
for (int i = 0; i < sub.split(",").length; i++) {
subscore+="4,";
}
subscore=subscore.substring(0,subscore.length()-1);
System.out.println("name:" + name);
System.out.println("begintime:" + begintime);
System.out.println("finishtime:" + finishtime);
System.out.println("choi:" + choi);
System.out.println("judg:" + judg);
System.out.println("sub:" + sub);
System.out.println("tid:" + tid);
System.out.println("classid:" + classid);
return paperService.addPaper(new Paper(name, begintime, finishtime, choi,
judg, sub,choiscore,judgscore,subscore, tid, classid)) != 0;
}
@RequestMapping(value = "student_grade")
String student_grade(Model model, HttpSession session) {
long tid = (long) session.getAttribute("userid");
model.addAttribute("paper", teacherService.listExamById(tid));
return path + "student_grade";
}
@RequestMapping(value = "student_grade_list")
String studentGradeList(Model model, HttpSessio
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
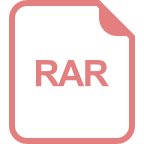
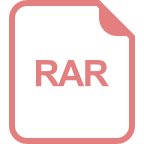
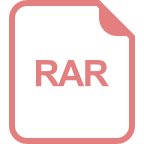
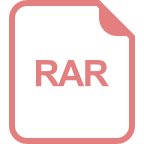
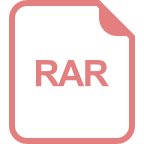
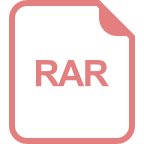
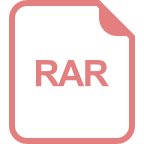
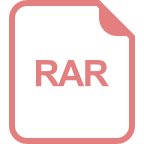
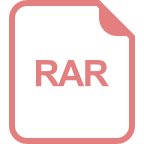
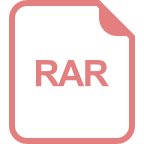
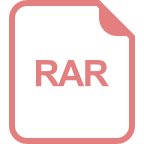
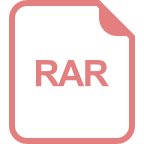
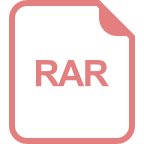
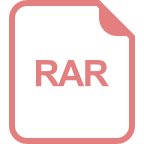
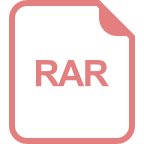
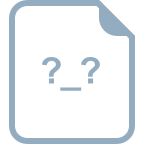
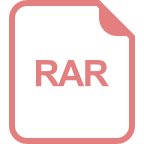
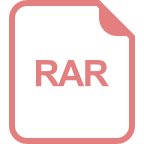
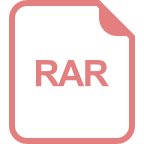
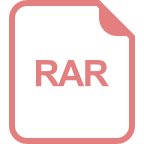
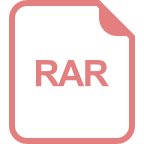
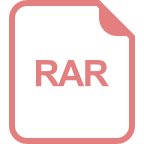
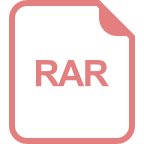
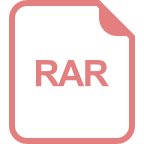
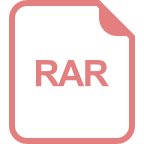
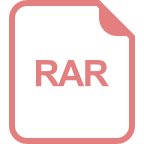
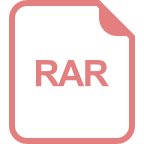
收起资源包目录

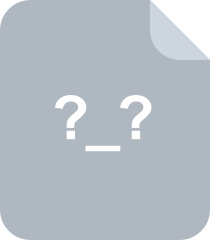
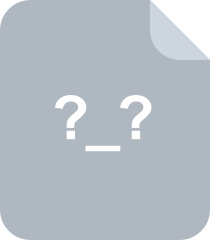
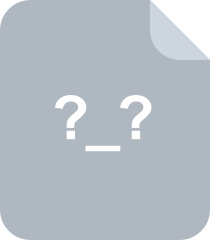
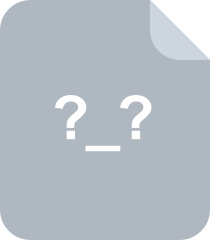
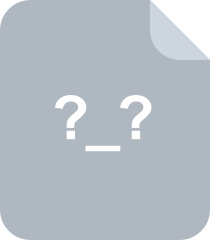
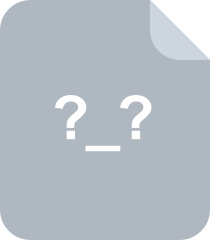
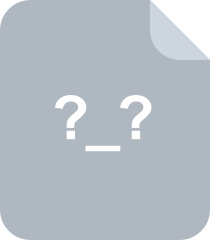
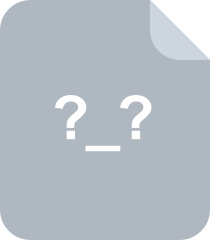
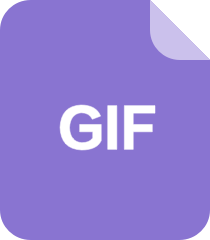
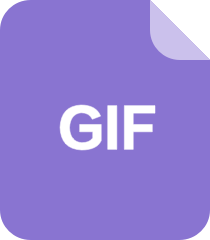
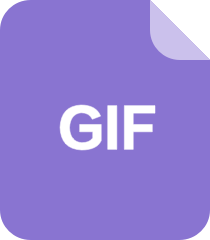
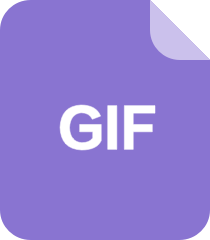
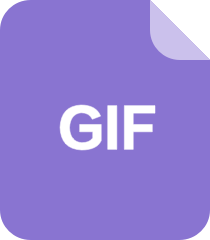
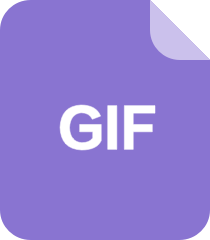
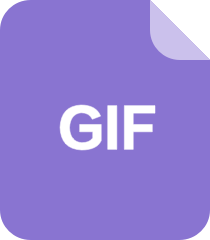
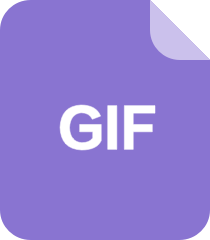
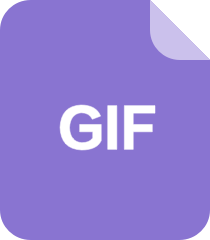
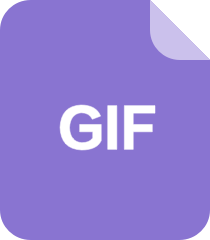
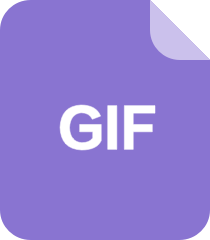
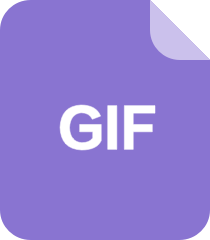
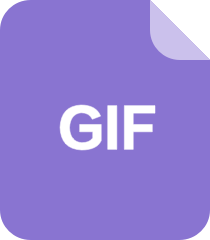
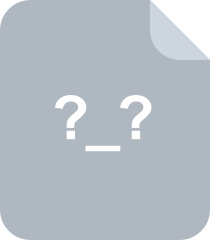
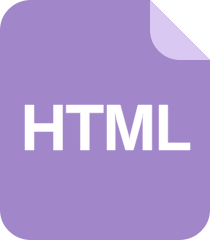
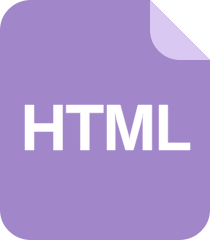
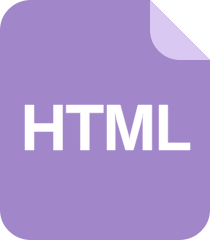
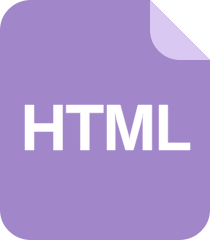
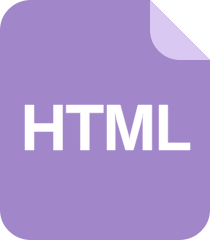
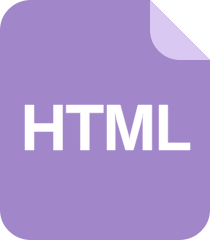
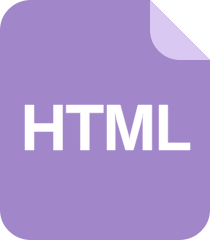
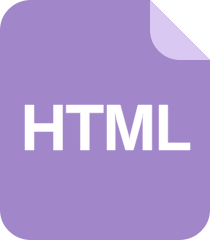
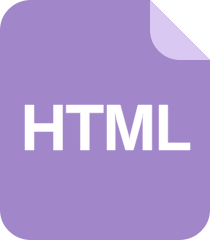
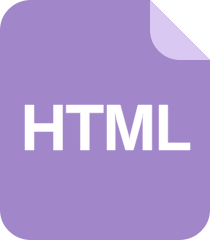
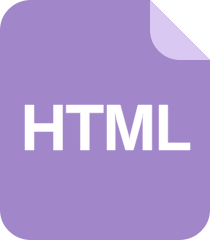
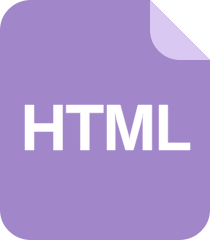
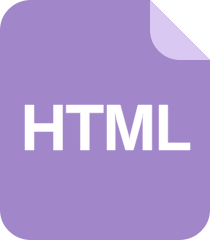
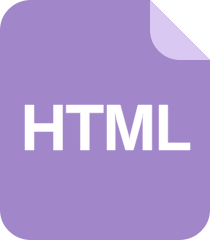
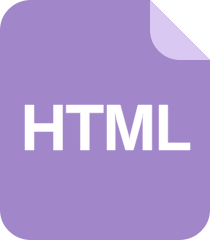
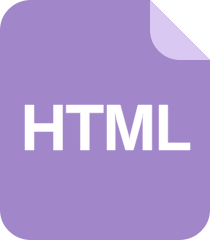
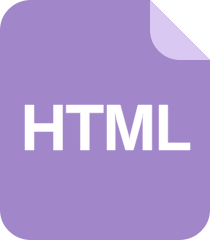
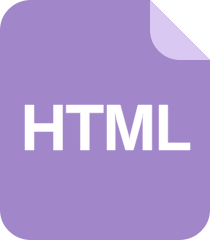
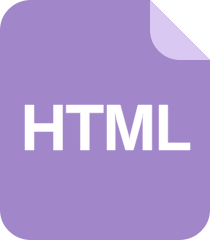
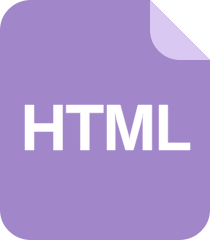
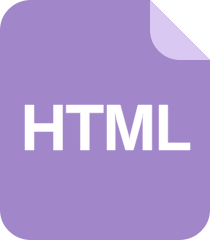
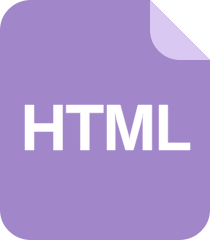
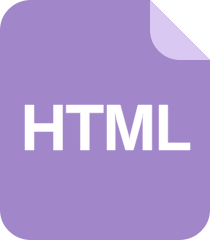
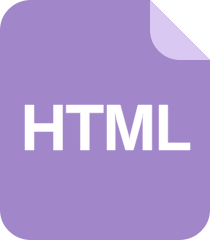
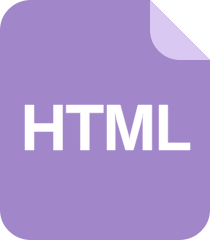
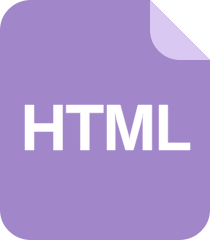
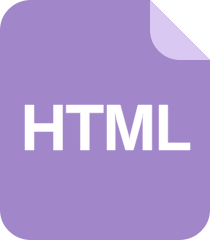
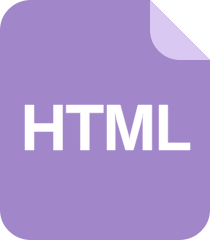
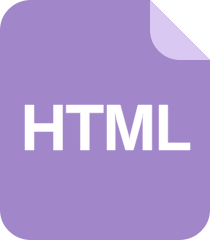
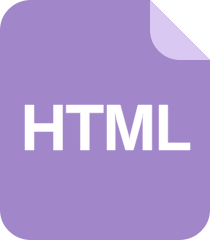
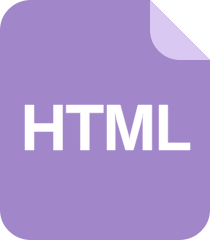
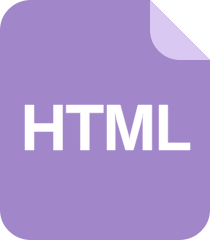
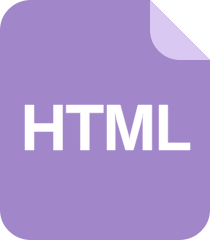
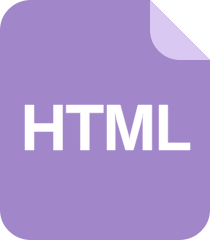
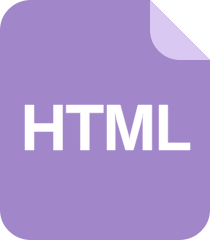
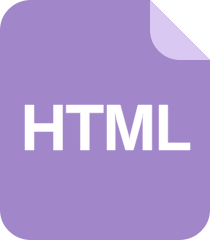
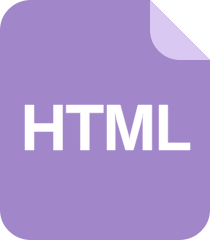
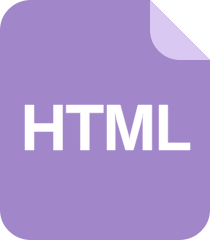
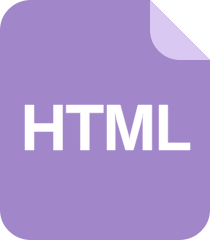
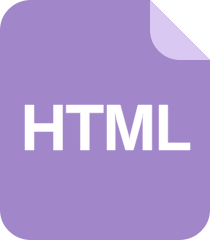
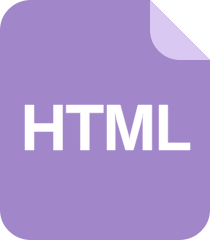
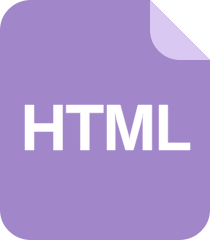
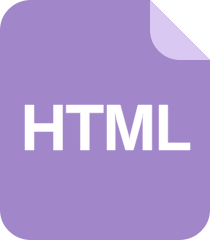
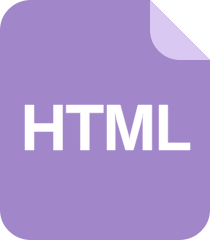
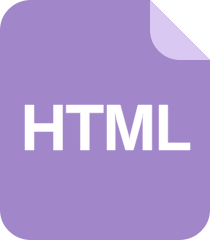
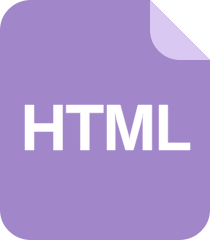
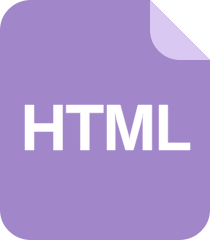
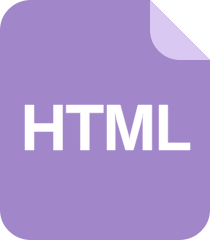
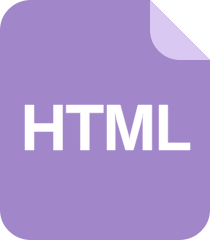
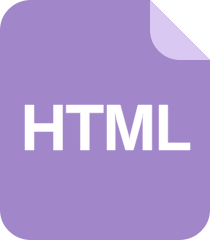
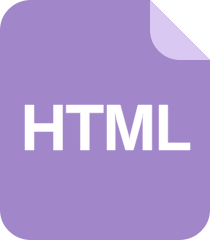
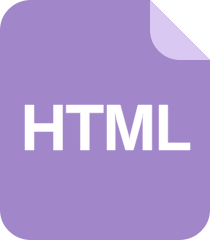
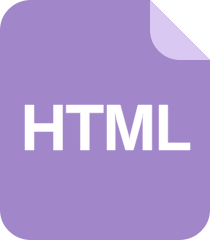
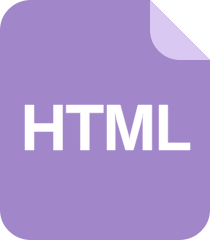
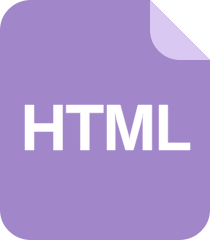
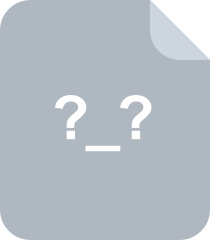
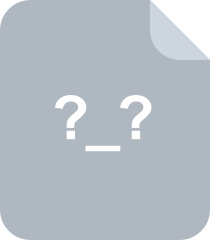
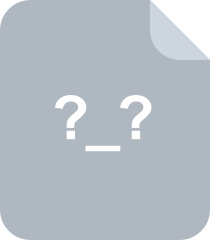
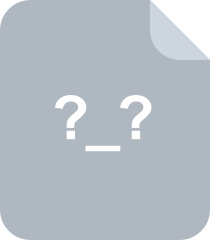
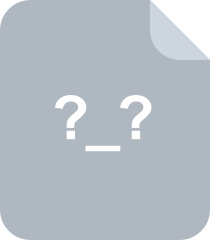
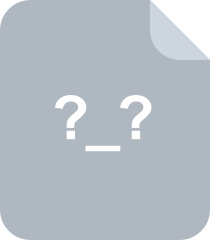
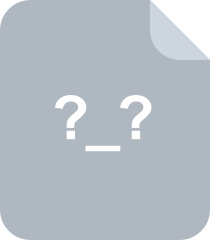
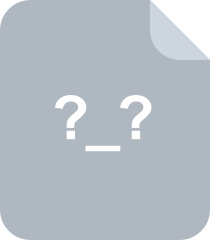
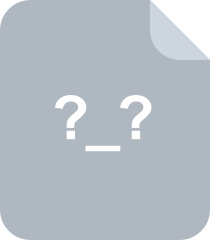
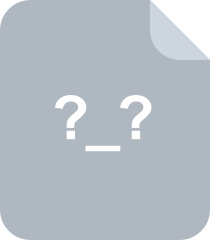
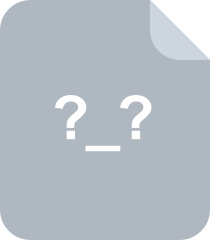
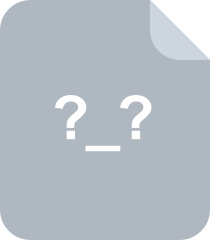
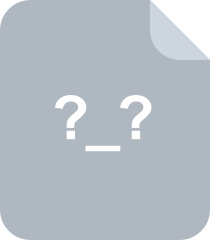
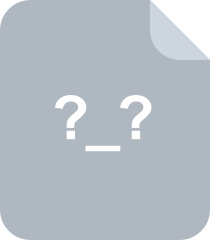
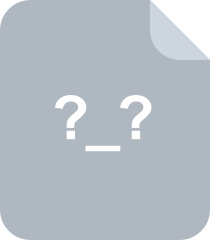
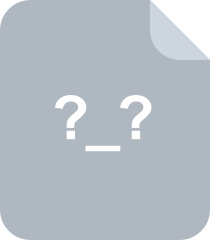
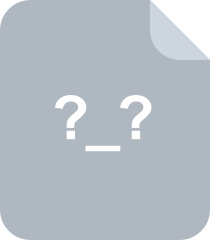
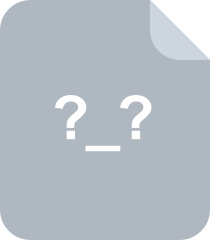
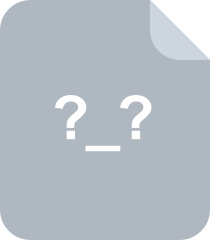
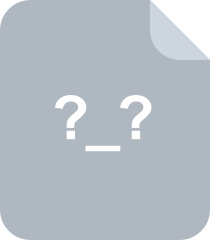
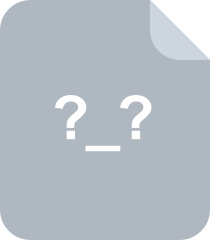
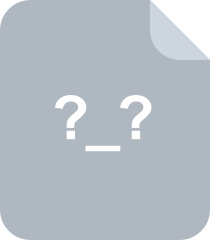
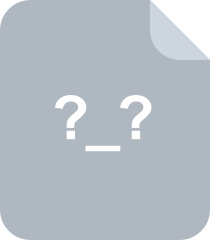
共 242 条
- 1
- 2
- 3
资源评论
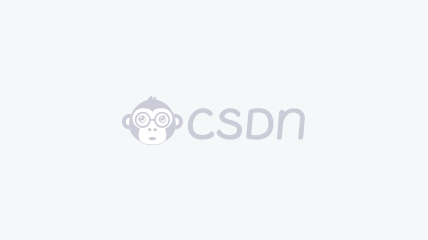

徐浪老师
- 粉丝: 8250
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

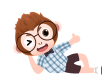
最新资源
- 基于MySQL的嵌入式Linux智慧农业采集控制系统+c语言源码+文档说明(高分作品)
- 在线商城系统-需求规格说明书
- 城市大脑-泸州市城市大脑项目(智能化系统).pdf
- AI(Adobe Illustrator)从入门到精通系统视频教程【84节完整版】-10G网盘下载.txt
- 城市大脑-泸州市“城市大脑”项目(数字底座及应用场景).pdf
- style05.css
- 嵌入式项目-Linux多线程方式实现嵌入式网关Server( 包括参数数据解析、协议转换、Socket收发、Sqlite、Uart、Camera等操作&UI界面)
- 计算机操作系统 - 实验二 - 进程调度算法的实现 - FCFS & SJF
- java权限工作流管理系统源码带本地搭建教程数据库 MySQL源码类型 WebForm
- 智慧景区信息化解决方案
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


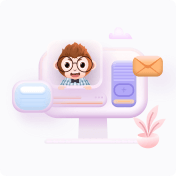
安全验证
文档复制为VIP权益,开通VIP直接复制
