# tough-cookie
[RFC 6265](https://tools.ietf.org/html/rfc6265) Cookies and CookieJar for Node.js
[](https://nodei.co/npm/tough-cookie/)
[](https://travis-ci.org/salesforce/tough-cookie)
## Synopsis
```javascript
var tough = require("tough-cookie");
var Cookie = tough.Cookie;
var cookie = Cookie.parse(header);
cookie.value = "somethingdifferent";
header = cookie.toString();
var cookiejar = new tough.CookieJar();
// Asynchronous!
var cookie = await cookiejar.setCookie(
cookie,
"https://currentdomain.example.com/path"
);
var cookies = await cookiejar.getCookies("https://example.com/otherpath");
// Or with callbacks!
cookiejar.setCookie(
cookie,
"https://currentdomain.example.com/path",
function (err, cookie) {
/* ... */
}
);
cookiejar.getCookies("http://example.com/otherpath", function (err, cookies) {
/* ... */
});
```
Why the name? NPM modules `cookie`, `cookies` and `cookiejar` were already taken.
## Installation
It's _so_ easy! Install with `npm` or your preferred package manager.
```sh
npm install tough-cookie
```
## Node.js Version Support
We follow the [node.js release schedule](https://github.com/nodejs/Release#release-schedule) and support all versions that are in Active LTS or Maintenance. We will always do a major release when dropping support for older versions of node, and we will do so in consultation with our community.
## API
### tough
The top-level exports from `require('tough-cookie')` can all be used as pure functions and don't need to be bound.
#### `parseDate(string)`
Parse a cookie date string into a `Date`. Parses according to [RFC 6265 Section 5.1.1](https://datatracker.ietf.org/doc/html/rfc6265#section-5.1.1), not `Date.parse()`.
#### `formatDate(date)`
Format a `Date` into an [RFC 822](https://datatracker.ietf.org/doc/html/rfc822#section-5) string (the RFC 6265 recommended format).
#### `canonicalDomain(str)`
Transforms a domain name into a canonical domain name. The canonical domain name is a domain name that has been trimmed, lowercased, stripped of leading dot, and optionally punycode-encoded ([Section 5.1.2 of RFC 6265](https://datatracker.ietf.org/doc/html/rfc6265#section-5.1.2)). For the most part, this function is idempotent (calling the function with the output from a previous call returns the same output).
#### `domainMatch(str, domStr[, canonicalize=true])`
Answers "does this real domain match the domain in a cookie?". The `str` is the "current" domain name and the `domStr` is the "cookie" domain name. Matches according to [RFC 6265 Section 5.1.3](https://datatracker.ietf.org/doc/html/rfc6265#section-5.1.3), but it helps to think of it as a "suffix match".
The `canonicalize` parameter toggles whether the domain parameters get normalized with `canonicalDomain` or not.
#### `defaultPath(path)`
Given a current request/response path, gives the path appropriate for storing in a cookie. This is basically the "directory" of a "file" in the path, but is specified by [Section 5.1.4 of the RFC](https://datatracker.ietf.org/doc/html/rfc6265#section-5.1.4).
The `path` parameter MUST be _only_ the pathname part of a URI (excluding the hostname, query, fragment, and so on). This is the `.pathname` property of node's `uri.parse()` output.
#### `pathMatch(reqPath, cookiePath)`
Answers "does the request-path path-match a given cookie-path?" as per [RFC 6265 Section 5.1.4](https://datatracker.ietf.org/doc/html/rfc6265#section-5.1.4). Returns a boolean.
This is essentially a prefix-match where `cookiePath` is a prefix of `reqPath`.
#### `parse(cookieString[, options])`
Alias for [`Cookie.parse(cookieString[, options])`](#cookieparsecookiestring-options).
#### `fromJSON(string)`
Alias for [`Cookie.fromJSON(string)`](#cookiefromjsonstrorobj).
#### `getPublicSuffix(hostname)`
Returns the public suffix of this hostname. The public suffix is the shortest domain name upon which a cookie can be set. Returns `null` if the hostname cannot have cookies set for it.
For example: `www.example.com` and `www.subdomain.example.com` both have public suffix `example.com`.
For further information, see the [Public Suffix List](http://publicsuffix.org/). This module derives its list from that site. This call is a wrapper around [`psl`](https://www.npmjs.com/package/psl)'s [`get` method](https://www.npmjs.com/package/psl##pslgetdomain).
#### `cookieCompare(a, b)`
For use with `.sort()`, sorts a list of cookies into the recommended order given in step 2 of ([RFC 6265 Section 5.4](https://datatracker.ietf.org/doc/html/rfc6265#section-5.4)). The sort algorithm is, in order of precedence:
- Longest `.path`
- oldest `.creation` (which has a 1-ms precision, same as `Date`)
- lowest `.creationIndex` (to get beyond the 1-ms precision)
```javascript
var cookies = [
/* unsorted array of Cookie objects */
];
cookies = cookies.sort(cookieCompare);
```
> **Note**: Since the JavaScript `Date` is limited to a 1-ms precision, cookies within the same millisecond are entirely possible. This is especially true when using the `now` option to `.setCookie()`. The `.creationIndex` property is a per-process global counter, assigned during construction with `new Cookie()`, which preserves the spirit of the RFC sorting: older cookies go first. This works great for `MemoryCookieStore` since `Set-Cookie` headers are parsed in order, but is not so great for distributed systems. Sophisticated `Store`s may wish to set this to some other _logical clock_ so that if cookies A and B are created in the same millisecond, but cookie A is created before cookie B, then `A.creationIndex < B.creationIndex`. If you want to alter the global counter, which you probably _shouldn't_ do, it's stored in `Cookie.cookiesCreated`.
#### `permuteDomain(domain)`
Generates a list of all possible domains that `domainMatch()` the parameter. Can be handy for implementing cookie stores.
#### `permutePath(path)`
Generates a list of all possible paths that `pathMatch()` the parameter. Can be handy for implementing cookie stores.
### Cookie
Exported via `tough.Cookie`.
#### `Cookie.parse(cookieString[, options])`
Parses a single Cookie or Set-Cookie HTTP header into a `Cookie` object. Returns `undefined` if the string can't be parsed.
The options parameter is not required and currently has only one property:
- _loose_ - boolean - if `true` enable parsing of keyless cookies like `=abc` and `=`, which are not RFC-compliant.
If options is not an object it is ignored, which means it can be used with [`Array#map`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map).
To process the Set-Cookie header(s) on a node HTTP/HTTPS response:
```javascript
if (Array.isArray(res.headers["set-cookie"]))
cookies = res.headers["set-cookie"].map(Cookie.parse);
else cookies = [Cookie.parse(res.headers["set-cookie"])];
```
_Note:_ In version 2.3.3, tough-cookie limited the number of spaces before the `=` to 256 characters. This limitation was removed in version 2.3.4.
For more details, see [issue #92](https://github.com/salesforce/tough-cookie/issues/92).
#### Properties
Cookie object properties:
- _key_ - string - the name or key of the cookie (default `""`)
- _value_ - string - the value of the cookie (default `""`)
- _expires_ - `Date` - if set, the `Expires=` attribute of the cookie (defaults to the string `"Infinity"`). See `setExpires()`
- _maxAge_ - seconds - if set, the `Max-Age=` attribute _in seconds_ of the cookie. Can also be set to strings `"Infinity"` and `"-Infinity"` for non-expiry and immediate-expiry, respectively. See `setMaxAge()`
- _domain_ - string - the `Domain=` attribute of the cookie
- _path_ - string - the `Path=` of the cookie
- _secure_ - boolean - the `Secure` cookie flag
- _httpOnly_ - boolean - the `HttpOnly` cookie

萧鼎
- 粉丝: 3w+
- 资源: 157
最新资源
- MATLAB与Processing仿真环境建模Stewart平台,GUI控制及腿部驱动图绘制,确保模拟器腿操作范围安全无偏移,MATLAB 和Processing 的仿真环境用于对Stewart 平台
- 基于斯图尔特机器人Stewart平台的并联机构仿真与逆向运动学控制算法研究,利用SimscapeMultibody进行运动模拟,配合Arduino驱动步进电机与电感传感器实现真实场景应用 ,斯图尔特机
- 基于INFO-KELM回归算法的优化与Matlab实现:时序预测与分类一体化的数据处理程序,INFO-KELM回归,基于向量加权平均算法(INFO)优化核极限学习机(KELM)的数据回归预测(需要时序
- 雷赛DM556步进电机驱动器全套资料:性能卓越的技术文档汇总,性能达到雷赛dm556步进电机驱动器全套资料 ,核心关键词:性能; 雷赛dm556步进电机; 驱动器; 全套资料;,雷赛DM556步进
- 火绒规则:阻止深信服创建EasyConnect
- 自适应迭代无迹卡尔曼滤波算法AIUKF用于锂离子电池SOC估计与参数辨识 采用马里兰大学公开数据集及FUDS工况的鲁棒性分析,自适应迭代无迹卡尔曼滤波算法AIUKF 锂离子电池SOC估计 递推最小二
- soggy:)游戏服务器
- 电池二阶等效电路模型参数辨识与SOC估计:基于最小二乘法和扩展卡尔曼滤波的研究(附参考文献),电池二阶等效电路模型(2RC ECM) 基于最小二乘法的参数辩识代码 基于EKF的SOC估计代码 ps.有
- 西门子S7-1200变频恒压供水系统程序:含触摸屏定时轮询、组态模拟仿真与电气图说明书,西门子s7-1200 变频恒压供水系统程序 带触摸屏恒压供水带定时轮询 包含:说明书+程序+电气图 v16
- C#雷赛运动控制卡框架:适用于多种控制卡,源码开放,中文注释,适合新手入门,功能丰富,物超所值 ,C# 运动控制系统 雷赛运动控制卡控制系统 像高川控制卡、高川控制器、或者固高运动控制卡以及正运动
- 多智能体系统:事件触发控制代码与对应参考文献研究,事件触发控制代码,每个代码有对应参考文献 1.多智能体中基于事件触发的协议 2.多智能体分布式系统的事件触发控制 3.基于观测器的非理想线性多智能体事
- 结合预测模型的动态规划DP在混合动力汽车能量管理策略中的创新应用:实时优化与全局控制,动态规划算法DP在混合动力汽车能量管理策略开发上的运用 可以结合车速预测模型(BP或者RBF神经网络,预测模型资
- 西门子Smart200 PLC空调自控系统恒温恒湿控制系统源代码与MCGSpro触摸屏编程方案,03-空调自控系统恒温恒湿控制系统PLC程序 西门子smart200PLC 源程序,MCGSpro 触摸
- 基于Matlab Simulink的双电机建模:纯电动与混合动力汽车的驱动控制仿真模型图解,基于Matlab simulink的双电机建模驱动控制仿真模型(可以嵌套到整车模型中) -纯电动、混合动力
- 输入:一个整数数组 rewardValues,表示每个奖励的值 排序:对 rewardValues 进行排序,确保选择的奖励是升序的 动态规划: dpim 表示从 rewardValues
- C++实现仓库管理系统
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


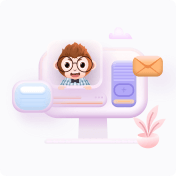