// demoDlg.cpp : implementation file
//
#include "stdafx.h"
#include "demo.h"
#include "demoDlg.h"
#include "SkinScrollBar/SkinScrollBar.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// CdemoDlg dialog
CdemoDlg::CdemoDlg(CWnd* pParent /*=NULL*/)
: CDialog(CdemoDlg::IDD, pParent)
{
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
void CdemoDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
}
BEGIN_MESSAGE_MAP(CdemoDlg, CDialog)
ON_WM_PAINT()
ON_WM_DESTROY()
ON_WM_QUERYDRAGICON()
//}}AFX_MSG_MAP
ON_BN_CLICKED(IDC_ATTACH, &CdemoDlg::OnBnClickedAttach)
ON_BN_CLICKED(IDC_DETACH, &CdemoDlg::OnBnClickedDetach)
END_MESSAGE_MAP()
// CdemoDlg message handlers
BOOL CdemoDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
SubclassUI_ScrollBar_Hook();
SubclassUI_ScrollBar_SetBitmap(::LoadBitmap(::AfxGetResourceHandle(), MAKEINTRESOURCE(IDB_SCROLLBAR)), RGB(255, 0, 255));
HWND h = ::GetDlgItem(m_hWnd, IDC_COMBO1);
WCHAR sz[50];
for(int i = 0; i < 50; ++i)
{
swprintf_s(sz, 50, L"item%d", i);
::SendMessage(h, CB_ADDSTRING, 0, (LPARAM)sz);
}
::SendMessage(h, CB_SETCURSEL, 0, 0L);
h = ::GetDlgItem(m_hWnd, IDC_COMBOBOXEX2);
COMBOBOXEXITEM cbex = {0};
cbex.mask = CBEIF_TEXT;
cbex.pszText = sz;
cbex.iIndent = 0;
for(int i = 0; i < 50; ++i)
{
cbex.iItem = i;
swprintf_s(sz, 50, L"item%d", i);
::SendMessage(h, CBEM_INSERTITEM, 0, (LPARAM)&cbex);
}
::SendMessage(h, CB_SETCURSEL, 0, 0L);
h = ::GetDlgItem(m_hWnd, IDC_LIST1);
for(int i = 0; i < 50; ++i)
{
swprintf_s(sz, 50, L"ListItem%d", i);
::SendMessage(h, LB_ADDSTRING, 0, (LPARAM)sz);
}
HWND hTree = ::GetDlgItem(m_hWnd, IDC_TREE1);
HTREEITEM hItem = NULL, hParent = NULL;
TVINSERTSTRUCT tvis = {0};
tvis.hInsertAfter = TVI_LAST;
tvis.item.mask = TVIF_TEXT;
tvis.item.cchTextMax = 50;
for(int i = 0; i < 15; ++i)
{
swprintf_s(sz, 50, L"item%d", i);
tvis.hParent = hParent;
tvis.item.pszText = sz;
hItem = (HTREEITEM)::SendMessage(hTree, TVM_INSERTITEM, 0, (LPARAM)&tvis);
::SendMessage(hTree, TVM_EXPAND, TVE_EXPAND, (LPARAM)hParent);
hParent = hItem;
}
HWND hListView = ::GetDlgItem(m_hWnd, IDC_LIST2);
LVCOLUMN lvl = {0};
lvl.cchTextMax = 50;
lvl.cx = 100;
lvl.mask = LVCF_TEXT|LVCF_WIDTH;
for(int i = 0; i < 5; ++i)
{
swprintf_s(sz, 50, L"Item%d", i);
lvl.pszText = sz;
::SendMessage(hListView, LVM_INSERTCOLUMN, (WPARAM)i, (LPARAM)&lvl);
}
LVITEM li = {0};
li.cchTextMax = 50;
li.mask = LVIF_TEXT;
for(int i = 0; i < 100; ++i)
{
swprintf_s(sz, 50, L"row%d", i);
li.pszText = sz;
li.iItem = i;
::SendMessage(hListView, LVM_INSERTITEM, 0, (LPARAM)&li);
}
return TRUE; // return TRUE unless you set the focus to a control
}
// If you add a minimize button to your dialog, you will need the code below
// to draw the icon. For MFC applications using the document/view model,
// this is automatically done for you by the framework.
void CdemoDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, reinterpret_cast<WPARAM>(dc.GetSafeHdc()), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
// The system calls this function to obtain the cursor to display while the user drags
// the minimized window.
HCURSOR CdemoDlg::OnQueryDragIcon()
{
return static_cast<HCURSOR>(m_hIcon);
}
void CdemoDlg::OnBnClickedAttach()
{
HWND h = NULL;
h = ::GetDlgItem(m_hWnd, IDC_COMBO1);
COMBOBOXINFO cbi;
cbi.cbSize = sizeof(COMBOBOXINFO);
::GetComboBoxInfo(h, &cbi);
SubclassUI_ScrollBar_Attach(cbi.hwndList);
h = ::GetDlgItem(m_hWnd, IDC_COMBOBOXEX2);
h = (HWND)::SendMessage(h, CBEM_GETCOMBOCONTROL, 0, 0L);
::GetComboBoxInfo(h, &cbi);
SubclassUI_ScrollBar_Attach(cbi.hwndList);
h = ::GetDlgItem(m_hWnd, IDC_EDIT1);
SubclassUI_ScrollBar_Attach(h);
h = ::GetDlgItem(m_hWnd, IDC_RICHEDIT21);
SubclassUI_ScrollBar_Attach(h);
h = ::GetDlgItem(m_hWnd, IDC_LIST1);
SubclassUI_ScrollBar_Attach(h);
h = ::GetDlgItem(m_hWnd, IDC_LIST2);
SubclassUI_ScrollBar_Attach(h);
h = ::GetDlgItem(m_hWnd, IDC_TREE1);
SubclassUI_ScrollBar_Attach(h);
}
void CdemoDlg::OnBnClickedDetach()
{
HWND h = ::GetDlgItem(m_hWnd, IDC_COMBO1);
COMBOBOXINFO cbi;
cbi.cbSize = sizeof(COMBOBOXINFO);
::GetComboBoxInfo(h, &cbi);
SubclassUI_ScrollBar_Detach(cbi.hwndList);
h = ::GetDlgItem(m_hWnd, IDC_COMBOBOXEX2);
h = (HWND)::SendMessage(h, CBEM_GETCOMBOCONTROL, 0, 0L);
::GetComboBoxInfo(h, &cbi);
SubclassUI_ScrollBar_Detach(cbi.hwndList);
h = ::GetDlgItem(m_hWnd, IDC_EDIT1);
SubclassUI_ScrollBar_Detach(h);
h = ::GetDlgItem(m_hWnd, IDC_RICHEDIT21);
SubclassUI_ScrollBar_Detach(h);
h = ::GetDlgItem(m_hWnd, IDC_LIST1);
SubclassUI_ScrollBar_Detach(h);
h = ::GetDlgItem(m_hWnd, IDC_LIST2);
SubclassUI_ScrollBar_Detach(h);
h = ::GetDlgItem(m_hWnd, IDC_TREE1);
SubclassUI_ScrollBar_Detach(h);
}
void CdemoDlg::OnDestroy()
{
SubclassUI_ScrollBar_Unhook();
}

liaoguobao
- 粉丝: 1
- 资源: 3
最新资源
- C# winform -类火车头采集器、采集工具、任务新建和编辑、网址采集、 标签编辑、数据采集、数据发布、发布配置的修改,编辑和测试、发布模块的修改和
- 全国铁路线路数据.rar
- Vue开源项目Pure Admin二次开发:实现前后端柱状图
- 2000-2023年全国各市CPI数据集.xlsx
- 2000-2023年全国+各省通货膨胀率数据集.xlsx
- 纯电动汽车电池系统HIL测试库
- Java+JSP+Mysql实现Web学生图书管理系统源码+数据库
- 基于SSM框架的农业信息管理系统的实现
- 自己毕业论文配套代码,B站有讲解 和运行效果
- Java+JSP+Mysql实现Web学生图书管理系统源码
- 可靠有效springboot使用netty搭建TCP服务器
- Firefox-latest.exe
- Modbus测试与仿真.rar
- PCIE参考时钟架构详解:同源与非同源的区别
- Java+JSP+Mysql实现Web学生图书管理系统
- 新年海报,讲稿,文案封面
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


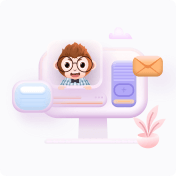