"""
"""
from tkinter import *
from tkinter import filedialog,messagebox
from tkinter.ttk import *
from typing import Dict
import os
import pandas as pd
from docxtpl import DocxTemplate
class WinGUI(Tk):
widget_dic: Dict[str, Widget] = {}
def __init__(self):
super().__init__()
self.__win()
self.widget_dic["tk_label_arrange"] = self.__tk_label_arrange(self)
self.widget_dic["tk_input_arrange"] = self.__tk_input_arrange(self)
self.widget_dic["tk_label_excel"] = self.__tk_label_excel(self)
self.widget_dic["tk_input_excel"] = self.__tk_input_excel(self)
self.widget_dic["tk_button_openexcel"] = self.__tk_button_openexcel(self)
self.widget_dic["tk_input_word"] = self.__tk_input_word(self)
self.widget_dic["tk_label_word"] = self.__tk_label_word(self)
# self.widget_dic["tk_button_openword"] = self.__tk_button_openword(self)
self.widget_dic["tk_label_info"] = self.__tk_label_info(self)
self.widget_dic["tk_button_lhld0n3u"] = self.__tk_button_lhld0n3u(self)
self.widget_dic["tk_select_box_lhldb7sn"] = self.__tk_select_box_lhldb7sn(self)
self.widget_dic["tk_label_lhldbccg"] = self.__tk_label_lhldbccg(self)
self.widget_dic["tk_label_lhle5h7h"] = self.__tk_label_lhle5h7h(self)
self.widget_dic["tk_label_lhlgfjup"] = self.__tk_label_lhlgfjup(self)
self.widget_dic["tk_input_name"] = self.__tk_input_name(self)
self.widget_dic["tk_label_name"] = self.__tk_label_name(self)
self.widget_dic["tk_label_lhlnz4v6"] = self.__tk_label_lhlnz4v6(self)
def __win(self):
self.title("excel生成word")
# 设置窗口大小、居中
width = 612
height = 400
screenwidth = self.winfo_screenwidth()
screenheight = self.winfo_screenheight()
geometry = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
self.geometry(geometry)
self.resizable(width=False, height=False)
# 自动隐藏滚动条
def scrollbar_autohide(self,bar,widget):
self.__scrollbar_hide(bar,widget)
widget.bind("<Enter>", lambda e: self.__scrollbar_show(bar,widget))
bar.bind("<Enter>", lambda e: self.__scrollbar_show(bar,widget))
widget.bind("<Leave>", lambda e: self.__scrollbar_hide(bar,widget))
bar.bind("<Leave>", lambda e: self.__scrollbar_hide(bar,widget))
def __scrollbar_show(self,bar,widget):
bar.lift(widget)
def __scrollbar_hide(self,bar,widget):
bar.lower(widget)
def __tk_label_arrange(self,parent):
label = Label(parent,text="excel中列名",anchor="center")
label.place(x=32, y=20, width=87, height=30)
return label
def __tk_input_arrange(self,parent):
ipt = Entry(parent)
ipt.place(x=130, y=20, width=449, height=30)
return ipt
def __tk_label_excel(self,parent):
label = Label(parent,text="excel文件",anchor="center")
label.place(x=30, y=70, width=85, height=30)
return label
def __tk_input_excel(self,parent):
ipt = Entry(parent)
ipt.place(x=130, y=70, width=388, height=30)
return ipt
def __tk_button_openexcel(self,parent):
btn = Button(parent, text="选择")
btn.place(x=530, y=70, width=50, height=30)
return btn
def __tk_input_word(self,parent):
ipt = Entry(parent)
ipt.place(x=130, y=170, width=388, height=30)
return ipt
def __tk_label_word(self,parent):
label = Label(parent,text="点此打开word模版文件",anchor="center")
label.place(x=320, y=120, width=153, height=30)
return label
# def __tk_button_openword(self,parent):
# btn = Button(parent, text="按钮")
# btn.place(x=530, y=170, width=50, height=30)
# return btn
def __tk_label_info(self,parent):
label = Label(parent,text="状态",anchor="center")
label.place(x=30, y=350, width=548, height=30)
return label
def __tk_button_lhld0n3u(self,parent):
btn = Button(parent, text="生成")
btn.place(x=280, y=300, width=50, height=30)
return btn
def __tk_select_box_lhldb7sn(self,parent):
cb = Combobox(parent, state="readonly")
cb['values'] = ("一行一word","一行一页")
cb.place(x=130, y=120, width=150, height=30)
return cb
def __tk_label_lhldbccg(self,parent):
label = Label(parent,text="生成方式",anchor="center")
label.place(x=30, y=120, width=70, height=30)
return label
def __tk_label_lhle5h7h(self, parent):
label = Label(parent, text="举例:序号,姓名,年龄,学分(英文逗号分割)", anchor="center")
label.place(x=130, y=0, width=262, height=24)
return label
def __tk_label_lhlgfjup(self, parent):
label = Label(parent, text="生成word文件名", anchor="center")
label.place(x=30, y=170, width=100, height=30)
return label
def __tk_input_name(self, parent):
ipt = Entry(parent)
ipt.place(x=156, y=220, width=362, height=30)
return ipt
def __tk_label_name(self, parent):
label = Label(parent, text="以列命名word", anchor="center")
label.place(x=30, y=220, width=109, height=30)
return label
def __tk_label_lhlnz4v6(self, parent):
label = Label(parent, text="举例:姓名(非必填),列内容不能有回车或特殊符号", anchor="center")
label.place(x=150, y=260, width=304, height=24)
return label
class Win(WinGUI):
def __init__(self):
super().__init__()
self.__event_bind()
def openexcel(self,evt):
strpath = filedialog.StringVar()
selected_file_path = filedialog.askopenfilename() # 使用askopenfilename函数选择单个文件
self.widget_dic["tk_input_excel"].delete(0, "end")
self.widget_dic["tk_input_excel"].insert(0, selected_file_path)
global dbpath
dbpath = selected_file_path
def openexample(self,evt):
createway=self.widget_dic["tk_select_box_lhldb7sn"].get()
print(createway)
if createway=="一行一页": #根据生成方式打开不同的模版
base_path = self.base_path('')
os.system('start ' + base_path + 'moban2.docx')
else:
base_path = self.base_path('')
os.system('start ' + base_path + 'moban1.docx')
# def openword(self,evt):
# strpath = filedialog.StringVar()
# selected_file_path = filedialog.askopenfilename() # 使用askopenfilename函数选择单个文件
# self.widget_dic["tk_input_word"].delete(0, "end")
# self.widget_dic["tk_input_word"].insert(0, selected_file_path)
# global dbpath
# dbpath = selected_file_path
def create(self,evt):
excelarr = len(self.widget_dic["tk_input_arrange"].get())
excellen = len(self.widget_dic["tk_input_excel"].get())
waylen = len(self.widget_dic["tk_select_box_lhldb7sn"].get())
wordlen = len(self.widget_dic["tk_input_word"].get())
namelen = len(self.widget_dic["tk_input_name"].get())
if excelarr<1 or excellen<1 or waylen<1 or wordlen<1:
messagebox.showinfo('错误','excel列名或excel文件或生成方式或生成名未填!')
return False
# if wordlen+namelen<1:
# messagebox.showinfo('生成名或列命名最少填一项!')
createway = self.widget_dic["tk_select_box_lhldb7sn"].get()
print(createway)
mobanpath = ""
if createway=="一行一页": #根据生成方式打开不同的模版
base

li642041156
- 粉丝: 5
- 资源: 31
最新资源
- 【java毕业设计】springboot医学电子技术线上课堂系统(springboot+vue+mysql+说明文档).zip
- java 输入任意字符串找回文
- NewModel_3.2.2(1).zip
- 上海交通大学版 asp.NET第152页-运用ADO.NET访问数据库(注册账号并在网站中查询)
- 【源码+数据库】利用Java Swing框架与Socket技术开发的即时通讯系统,系统分为客户端和服务端,类似于qq聊天
- 计算机科学与技术数据结构实践考核要求.ppt
- 【java毕业设计】springboot中医院问诊系统的设计与实现(springboot+vue+mysql+说明文档).zip
- MATLAB大数计算工具箱及其用法
- 基于 python 实现的微博的数据挖掘与社交舆情分析
- Screenshot_20241105_140450.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


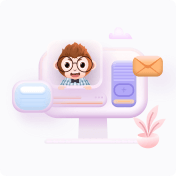