没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
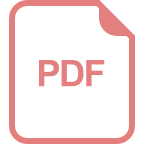
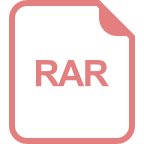
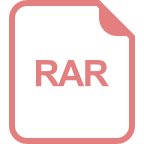
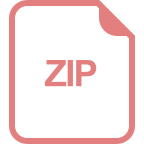
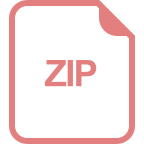
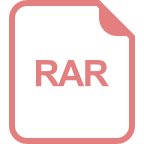
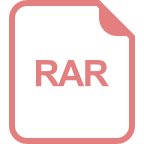
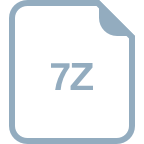
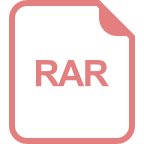
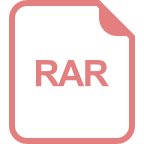
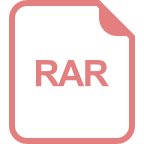
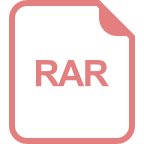
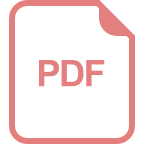
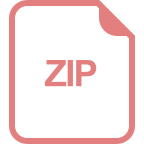
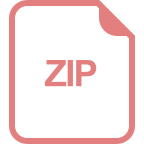
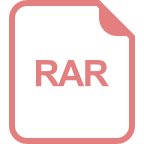
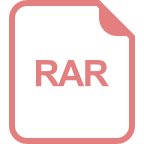
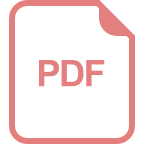
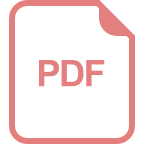
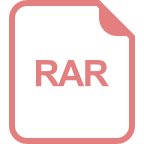
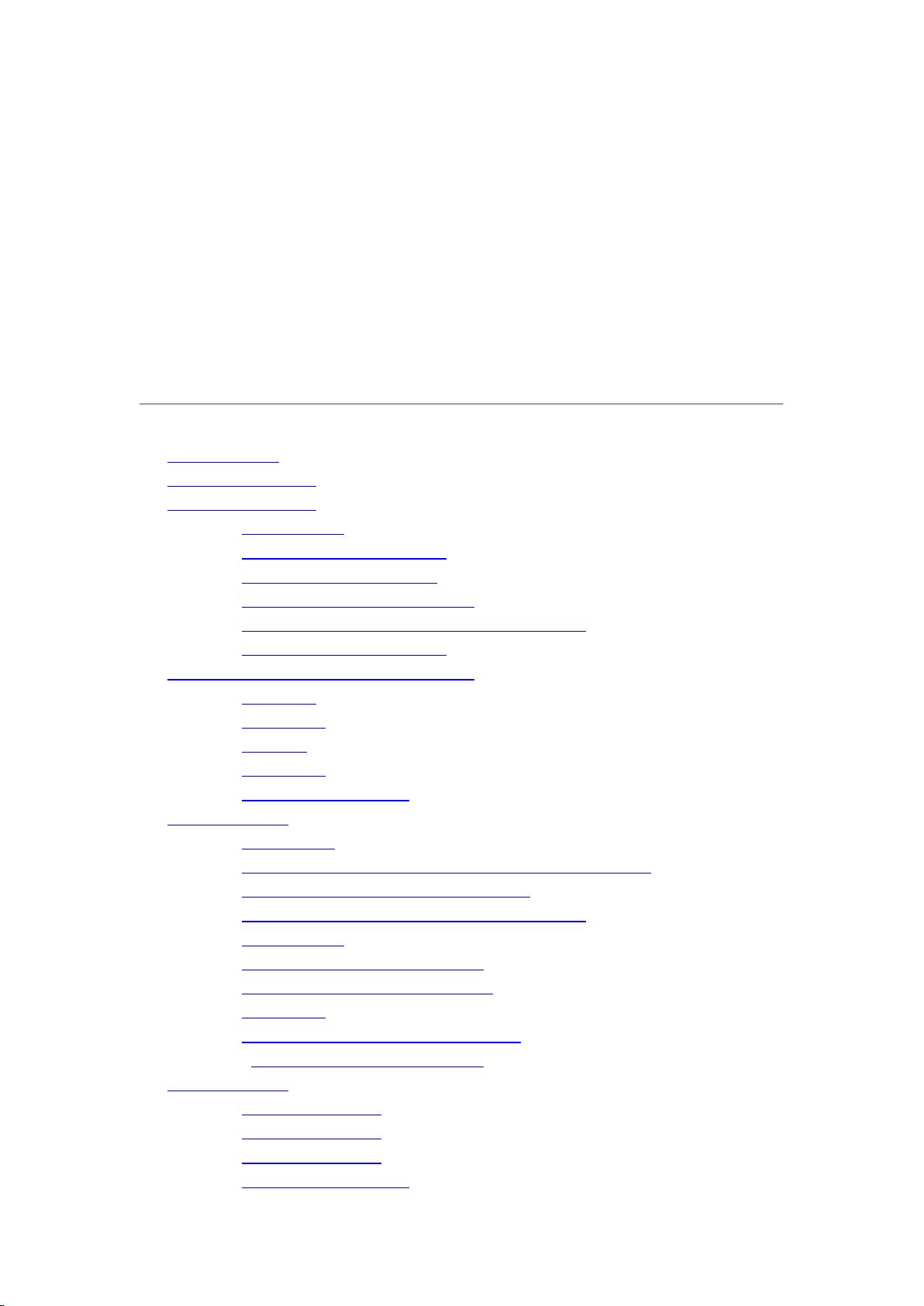
Program Library HOWTO
David A. Wheeler
version 1.20, 11 April 2003
This HOWTO for programmers discusses how to create and use program
libraries on Linux. This includes static libraries, shared libraries, and
dynamically loaded libraries.
Table of Contents
1. Introduction
2. Static Libraries
3. Shared Libraries
3.1. Conventions
3.2. How Libraries are Used
3.3. Environment Variables
3.4. Creating a Shared Library
3.5. Installing and Using a Shared Library
3.6. Incompatible Libraries
4. Dynamically Loaded (DL) Libraries
4.1. dlopen()
4.2. dlerror()
4.3. dlsym()
4.4. dlclose()
4.5. DL Library Example
5. Miscellaneous
5.1. nm command
5.2. Library constructor and destructor functions
5.3. Shared Libraries Can Be Scripts
5.4. Symbol Versioning and Version Scripts
5.5. GNU libtool
5.6. Removing symbols for space
5.7. Extremely small executables
5.8. C++ vs. C
5.9. Speeding up C++ initialization
5.10. Linux Standard Base (LSB)
6. More Examples
6.1. File libhello.c
6.2. File libhello.h
6.3. File demo_use.c
6.4. File script_static
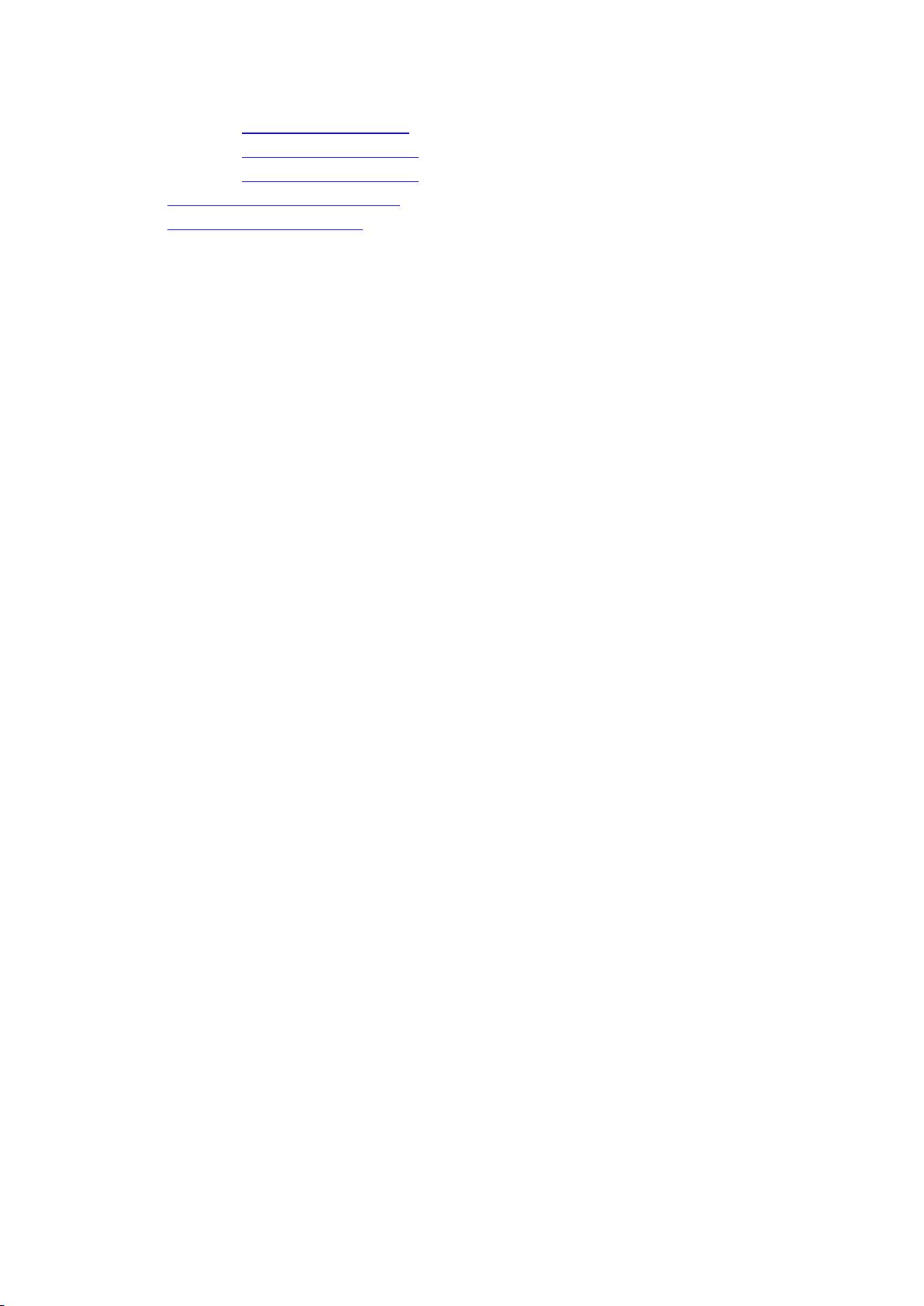
6.5. File script_shared
6.6. File demo_dynamic.c
6.7. File script_dynamic
7. Other Information Sources
8. Copyright and License
1. Introduction
This HOWTO for programmers discusses how to create and use program
libraries on Linux using the GNU toolset. A ``program library'' is simply
a file containing compiled code (and data) that is to be incorporated later
into a program; program libraries allow programs to be more modular,
faster to recompile, and easier to update. Program libraries can be
divided into three types: static libraries, shared libraries, and
dynamically loaded (DL) libraries.
This paper first discusses static libraries, which are installed into a
program executable before the program can be run. It then discusses shared
libraries, which are loaded at program start-up and shared between
programs. Finally, it discusses dynamically loaded (DL) libraries, which
can be loaded and used at any time while a program is running. DL libraries
aren't really a different kind of library format (both static and shared
libraries can be used as DL libraries); instead, the difference is in how
DL libraries are used by programmers. The HOWTO wraps up with a section
with more examples and a section with references to other sources of
information
Most developers who are developing libraries should create shared
libraries, since these allow users to update their libraries separately
from the applications that use the libraries. Dynamically loaded (DL)
libraries are useful, but they require a little more work to use and many
programs don't need the flexibility they offer. Conversely, static
libraries make upgrading libraries far more troublesome, so for
general-purpose use they're hard to recommend. Still, each have their
advantages, and the advantages of each type are described in the section
discussing that type. Developers using C++ and dynamically loaded (DL)
libraries should also consult the ``C++ dlopen mini-HOWTO''.
It's worth noting that some people use the term dynamically
linked
libraries (DLLs) to refer to shared libraries, some use the term DLL to
mean any library that is used as a DL library, and some use the term DLL
to mean a library meeting either condition. No matter which meaning you
pick, this HOWTO covers DLLs on Linux.
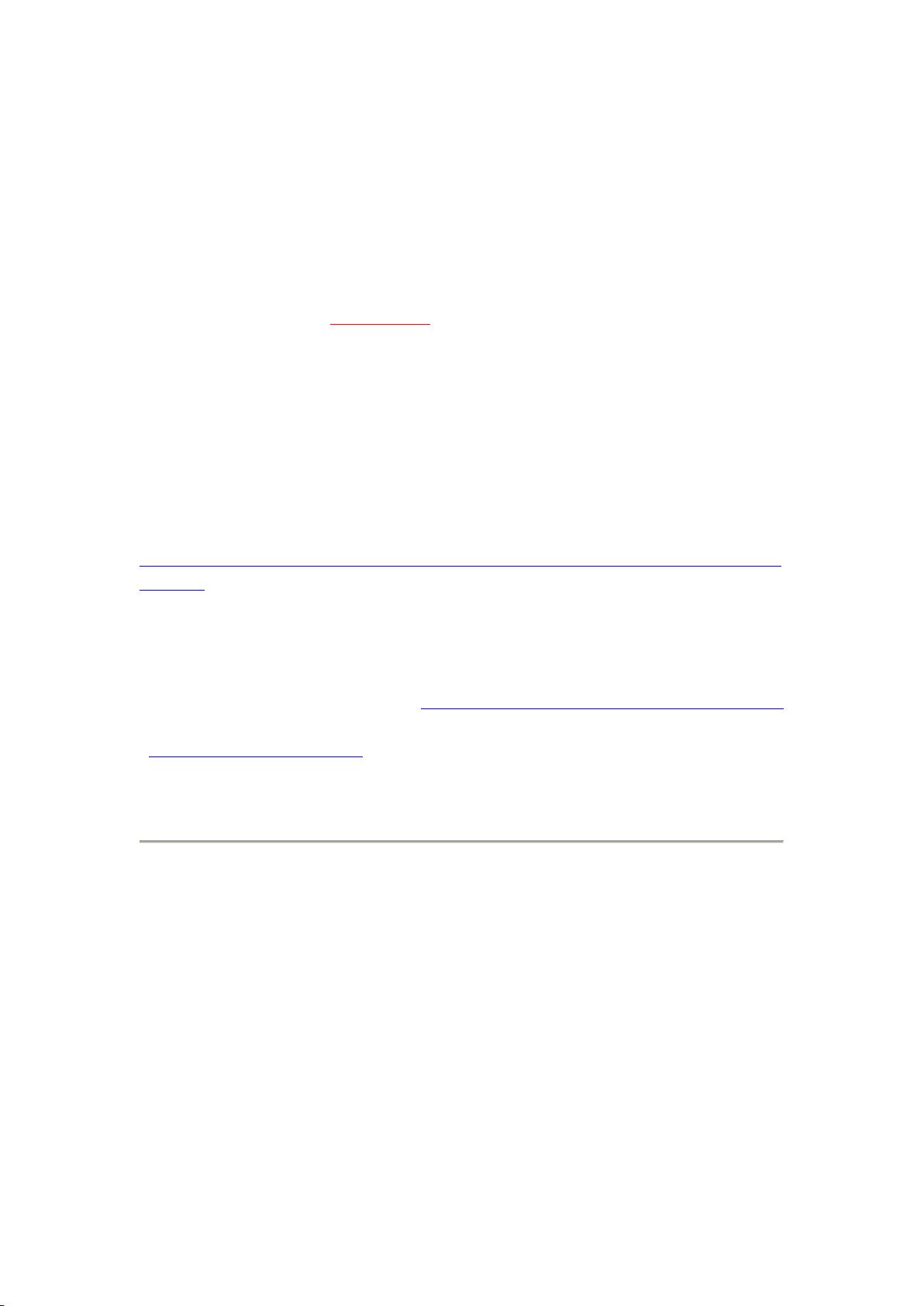
This HOWTO discusses only the Executable and Linking Format (ELF) format
for executables and libraries, the format used by nearly all Linux
distributions today. The GNU gcc toolset can actually handle library
formats other than ELF; in particular, most Linux distributions can still
use the obsolete a.out format. However, these formats are outside the
scope of this paper.
If you're building an application that should port to many systems, you
might consider using GNU libtool to build and install libraries instead
of using the Linux tools directly. GNU libtool is a generic library support
script that hides the complexity of using shared libraries (e.g., creating
and installing them) behind a consistent, portable interface. On Linux,
GNU libtool is built on top of the tools and conventions described in this
HOWTO. For a portable interface to dynamically loaded libraries, you can
use various portability wrappers. GNU libtool includes such a wrapper,
called ``libltdl''. Alternatively, you could use the glib library (not
to be confused with glibc) with its portable support for Dynamic Loading
of Modules. You can learn more about glib at
http://developer.gnome.org/doc/API/glib/glib-dynamic-loading-of-modul
es.html. Again, on Linux this functionality is implemented using the
constructs described in this HOWTO. If you're actually developing or
debugging the code on Linux, you'll probably still want the information
in this HOWTO.
This HOWTO's master location is http://www.dwheeler.com/program-library,
and it has been contributed to the Linux Documentation Project
(http://www.linuxdoc.org). It is Copyright (C) 2000 David A. Wheeler and
is licensed through the General Public License (GPL); see the last section
for more information.
2. Static Libraries
Static libraries are simply a collection of ordinary object files;
conventionally, static libraries end with the ``.a'' suffix. This
collection is created using the ar (archiver) program. Static libraries
aren't used as often as they once were, because of the advantages of shared
libraries (described below). Still, they're sometimes created, they
existed first historically, and they're simpler to explain.
Static libraries permit users to link to programs without having to
recompile its code, saving recompilation time. Note that recompilation
time is less important given today's faster compilers, so this reason is
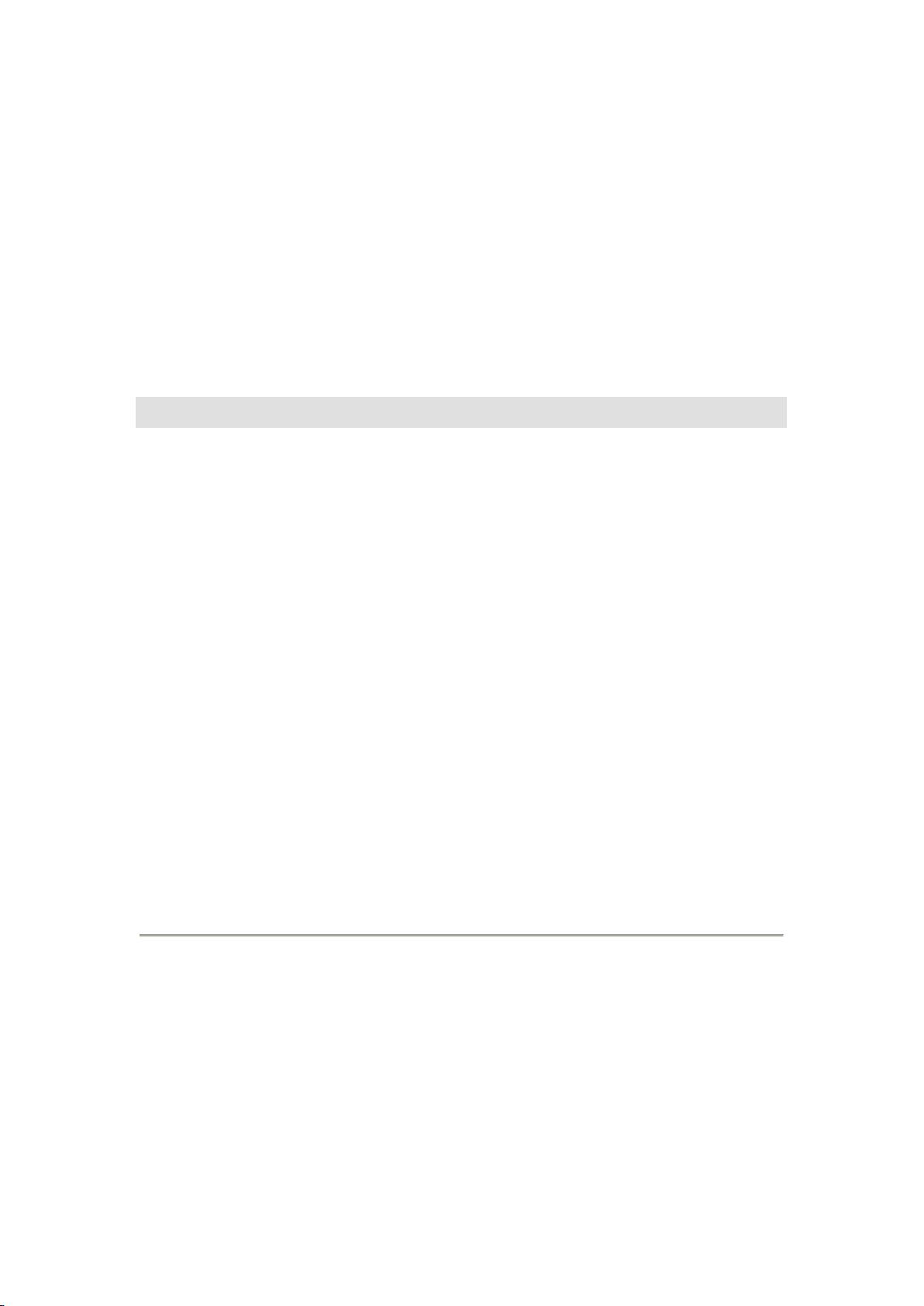
not as strong as it once was. Static libraries are often useful for
developers if they wish to permit programmers to link to their library,
but don't want to give the library source code (which is an advantage to
the library vendor, but obviously not an advantage to the programmer
trying to use the library). In theory, code in static ELF libraries that
is linked into an executable should run slightly faster (by 1-5%) than
a shared library or a dynamically loaded library, but in practice this
rarely seems to be the case due to other confounding factors.
To create a static library, or to add additional object files to an
existing static library, use a command like this:
ar rcs my_library.a file1.o file2.o
This sample command adds the object files file1.o and file2.o to the static
library my_library.a, creating my_library.a if it doesn't already exist.
For more information on creating static libraries, see ar(1).
Once you've created a static library, you'll want to use it. You can use
a static library by invoking it as part of the compilation and linking
process when creating a program executable. If you're using gcc(1) to
generate your executable, you can use the -l option to specify the library;
see info:gcc for more information.
Be careful about the order of the parameters when using gcc; the -l option
is a linker option, and thus needs to be placed AFTER the name of the file
to be compiled. This is quite different from the normal option syntax.
If you place the -l option before the filename, it may fail to link at
all, and you can end up with mysterious errors.
You can also use the linker ld(1) directly, using its -l and -L options;
however, in most cases it's better to use gcc(1) since the interface of
ld(1) is more likely to change.
3. Shared Libraries
Shared libraries are libraries that are loaded by programs when they start.
When a shared library is installed properly, all programs that start
afterwards automatically use the new shared library. It's actually much
more flexible and sophisticated than this, because the approach used by
Linux permits you to:
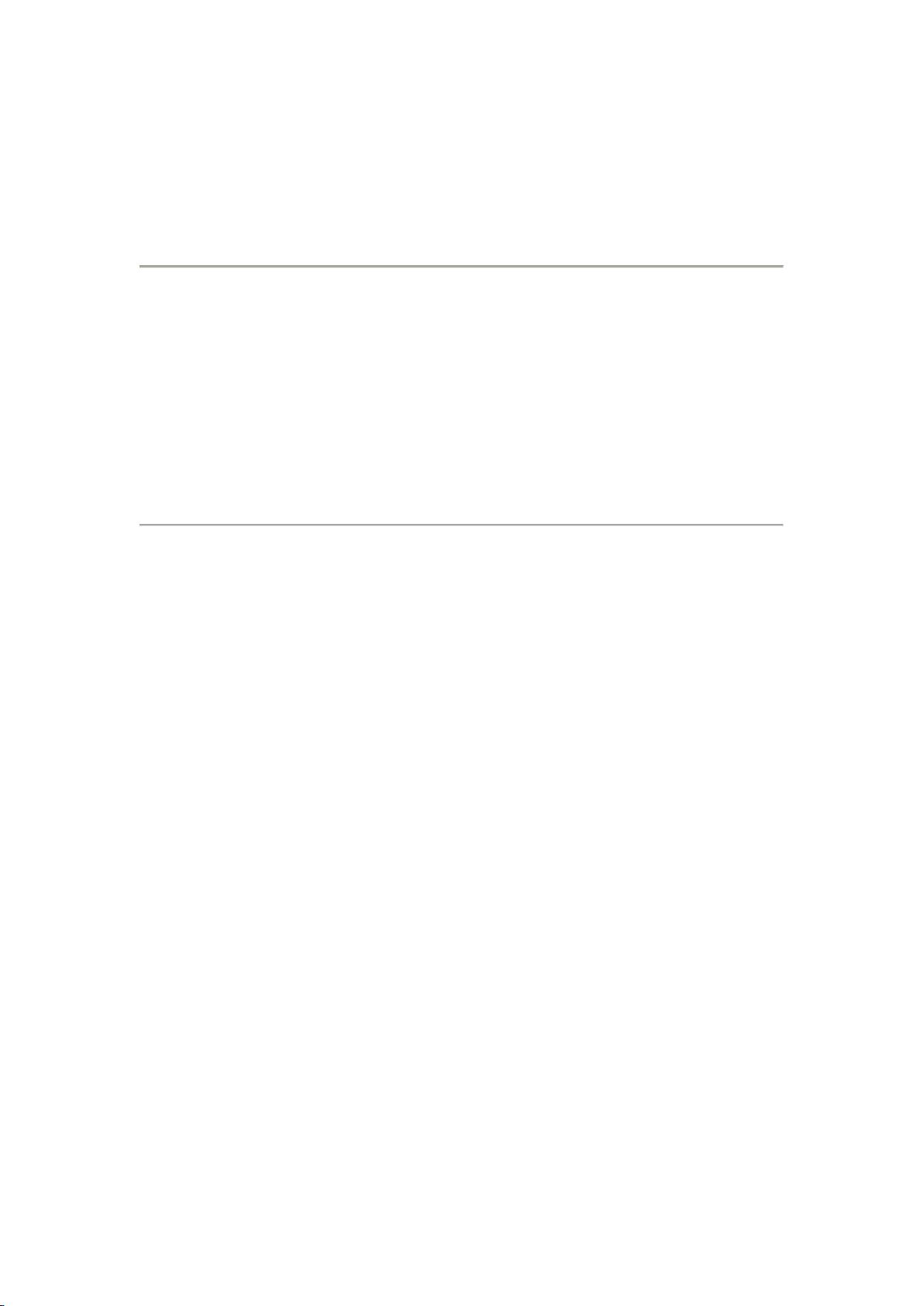
update libraries and still support programs that want to use older,
non-backward-compatible versions of those libraries;
override specific libraries or even specific functions in a library
when executing a particular program.
do all this while programs are running using existing libraries.
3.1. Conventions
For shared libraries to support all of these desired properties, a number
of conventions and guidelines must be followed. You need to understand
the difference between a library's names, in particular its ``soname''
and ``real name'' (and how they interact). You also need to understand
where they should be placed in the filesystem.
3.1.1. Shared Library Names
Every shared library has a special name called the ``soname''. The soname
has the prefix ``lib'', the name of the library, the phrase ``.so'',
followed by a period and a version number that is incremented whenever
the interface changes (as a special exception, the lowest-level C
libraries don't start with ``lib''). A fully-qualified soname includes
as a prefix the directory it's in; on a working system a fully-qualified
soname is simply a symbolic link to the shared library's ``real name''.
Every shared library also has a ``real name'', which is the filename
containing the actual library code. The real name adds to the soname a
period, a minor number, another period, and the release number. The last
period and release number are optional. The minor number and release
number support configuration control by letting you know exactly what
version(s) of the library are installed. Note that these numbers might
not be the same as the numbers used to describe the library in
documentation, although that does make things easier.
In addition, there's the name that the compiler uses when requesting a
library, (I'll call it the ``linker name''), which is simply the soname
without any version number.
The key to managing shared libraries is the separation of these names.
Programs, when they internally list the shared libraries they need, should
剩余31页未读,继续阅读
资源评论
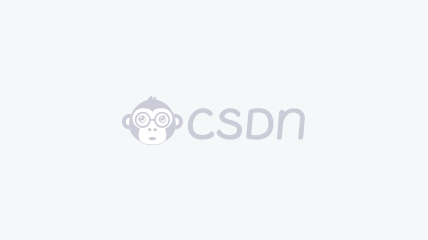

LGD_2008
- 粉丝: 3
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

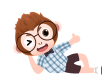
最新资源
- 时间复杂度与数据结构:算法效率的双重奏
- QT 简易项目 网络调试器(未实现连接唯一性) QT5.12.3环境 C++实现
- YOLOv3网络架构深度解析:关键特性与代码实现
- 2024 CISSP考试大纲(2024年4月15日生效)
- ACOUSTICECHO CANCELLATION WITH THE DUAL-SIGNAL TRANSFORMATION LSTM NETWORK
- 深入解析:动态数据结构与静态数据结构的差异
- YOLOv2:在YOLOv1基础上的飞跃
- imgview图片浏览工具v1.0
- Toony Colors Pro 2 2.2.5的资源
- Java项目:基于SSM框架+Mysql+Jsp实现的药品管理系统(ssm+B/S架构+源码+数据库)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


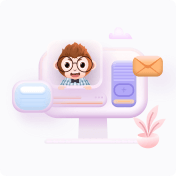
安全验证
文档复制为VIP权益,开通VIP直接复制
