// serverDlg.cpp : implementation file
//
#include "stdafx.h"
#include "server.h"
#include "serverDlg.h"
#include "ace/Auto_Ptr.h"
#include "ace/FILE_IO.h"
#include "ace/Log_Msg.h"
#include "ace/Signal.h"
#include "ace/Thread_Manager.h"
#include "Thread_Per_Connection_Logging_Server.h"
#include "Logging_Handler.h"
#include "Logging_Client.h"
#include <errno.h>
namespace {
extern "C" void sigterm_handler (int /* signum */) { /* No-op. */ }
}
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
#define MAX_DATA_BUFF 1024*1024
/////////////////////////////////////////////////////////////////////////////
// CAboutDlg dialog used for App About
class CAboutDlg : public CDialog
{
public:
CAboutDlg();
// Dialog Data
//{{AFX_DATA(CAboutDlg)
enum { IDD = IDD_ABOUTBOX };
//}}AFX_DATA
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(CAboutDlg)
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
//}}AFX_VIRTUAL
// Implementation
protected:
//{{AFX_MSG(CAboutDlg)
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD)
{
//{{AFX_DATA_INIT(CAboutDlg)
//}}AFX_DATA_INIT
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CAboutDlg)
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)
//{{AFX_MSG_MAP(CAboutDlg)
// No message handlers
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CServerDlg dialog
CServerDlg::CServerDlg(CWnd* pParent /*=NULL*/)
: CDialog(CServerDlg::IDD, pParent)
{
//{{AFX_DATA_INIT(CServerDlg)
m_cIP = _T("127.0.0.1");
m_cServerPort = 8080;
m_cClientPort = 8080;
//}}AFX_DATA_INIT
// Note that LoadIcon does not require a subsequent DestroyIcon in Win32
m_hIcon = AfxGetApp()->LoadIcon(IDI_ICON_File);
}
void CServerDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CServerDlg)
DDX_Control(pDX, IDC_LIST_File, m_List);
DDX_Control(pDX, IDC_EDIT_SEND, m_cSEND);
DDX_Control(pDX, IDC_LIST_Msg, m_cMsg);
DDX_Text(pDX, IDC_EDIT_IP, m_cIP);
DDX_Text(pDX, IDC_EDIT_ServerPort, m_cServerPort);
DDX_Text(pDX, IDC_EDIT_ClientPort, m_cClientPort);
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CServerDlg, CDialog)
//{{AFX_MSG_MAP(CServerDlg)
ON_WM_SYSCOMMAND()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_BN_CLICKED(ID_SEND, OnSend)
ON_BN_CLICKED(IDC_BUTTON_Connect, OnConnect)
ON_BN_CLICKED(IDC_BUTTON_Select, OnSelect)
ON_BN_CLICKED(IDC_BUTTON_TRANS, OnButtonTrans)
ON_BN_CLICKED(IDC_BUTTON_Listen, OnBUTTONListen)
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CServerDlg message handlers
BOOL CServerDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Add "About..." menu item to system menu.
// IDM_ABOUTBOX must be in the system command range.
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
m_ImageList.Create(32,32,ILC_COLOR24|ILC_MASK,0,0);
m_ImageList.Add(LoadIcon(AfxGetInstanceHandle(), MAKEINTRESOURCE(IDI_ICON_File)));
m_List.SetImageList(&m_ImageList,LVSIL_NORMAL);
return TRUE; // return TRUE unless you set the focus to a control
}
void CServerDlg::OnSysCommand(UINT nID, LPARAM lParam)
{
if ((nID & 0xFFF0) == IDM_ABOUTBOX)
{
CAboutDlg dlgAbout;
dlgAbout.DoModal();
}
else
{
CDialog::OnSysCommand(nID, lParam);
}
}
// If you add a minimize button to your dialog, you will need the code below
// to draw the icon. For MFC applications using the document/view model,
// this is automatically done for you by the framework.
void CServerDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, (WPARAM) dc.GetSafeHdc(), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
// The system calls this to obtain the cursor to display while the user drags
// the minimized window.
HCURSOR CServerDlg::OnQueryDragIcon()
{
return (HCURSOR) m_hIcon;
}
void CServerDlg::DefaultConnect()
{
logging_client.~Logging_Client();
UpdateData(TRUE);
// TODO: Add your control notification handler code here
const char *logger_host=m_cIP;
if(m_cIP==_T(""))
{
MessageBox("请写入对方主机名!");
return;
}
CString m_Msg;
ACE_INET_Addr server_addr;
if (server_addr.set (m_cClientPort, logger_host)==-1)
{
MessageBox("主机名输入错误!");
return;
}
ACE_SOCK_Connector connector;
if(connector.connect(logging_client.peer(),server_addr)==-1)
{
MessageBox("无法连接主机!");
return;
}
UpdateData(FALSE);
}
void CServerDlg::OnBUTTONListen()
{
// TODO: Add your control notification handler code here
UpdateData(TRUE);
server_port=m_cServerPort;
UpdateData(FALSE);
m_hThread_server=CreateThread(NULL,100,Thread_server,(void*)this,NULL,NULL);
}
void CServerDlg::OnConnect()
{
logging_client.~Logging_Client();
UpdateData(TRUE);
// TODO: Add your control notification handler code here
const char *logger_host=m_cIP;
if(m_cIP==_T(""))
{
MessageBox("请写入对方主机名!");
return;
}
CString m_Msg;
ACE_INET_Addr server_addr;
if (server_addr.set (m_cClientPort, logger_host)==-1)
{
MessageBox("主机名输入错误!");
return;
}
ACE_SOCK_Connector connector;
if(connector.connect(logging_client.peer(),server_addr)==-1)
{
MessageBox("无法连接主机!");
return;
}
UpdateData(FALSE);
MessageBox("成功连接!");
}
void CServerDlg::OnSend()
{
// TODO: Add your control notification handler code here
CString m_Msg;
m_cSEND.GetWindowText(m_Msg);
if(m_Msg==_T(""))
{
MessageBox("信息不能为空!");
return;
}
m_hThread_client_msg=CreateThread(NULL,100,Thread_client_msg,(void*)this,NULL,NULL);
}
void CServerDlg::OnSelect()
{
CFileDialog fileDlg (TRUE,NULL,NULL,OFN_HIDEREADONLY | OFN_OVERWRITEPROMPT,NULL,this);
if (fileDlg.DoModal()==IDOK)
{
m_List.DeleteAllItems();
m_FileName = fileDlg.GetFileName();
m_List.InsertItem(0,m_FileName,0);
}
}
void CServerDlg::OnButtonTrans()
{
DefaultConnect();
if (m_List.GetItemCount()<1)
{
MessageBox("请选择发送的文件!");
return;
}
if (m_cIP.IsEmpty())
{
MessageBox("请设置主机名称或IP!");
return;
}
m_SendFile.Abort();
m_SendFile.Open(m_FileName,CFile::modeRead);
m_SendFileLen = m_SendFile.GetLength();
if(m_SendFileLen<MAX_DATA_BUFF)MessageBox("OK");
else MessageBox("Too Large");
char* membuf= (char*)LocalAlloc(LMEM_FIXED,m_SendFileLen);
memset(membuf,0,m_SendFileLen);
m_SendFile.ReadHuge(membuf,m_SendFileLen);
m_SendFile.Close();
if(logging_client.send_file(m_FileName,membuf,m_SendFileLen)==-1)
{
LocalFree(membuf);
return;
}
//else MessageBox("已发送!");
LocalFree(membuf);
}
//////////////////////

leogift_5815
- 粉丝: 0
- 资源: 2
最新资源
- 手手势检测3-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 学生成绩链表处理-C语言实现学生成绩链表处理技术解析与应用
- 手套手势检测7-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- CentOS bridge 工具包 bridge-utils-1.6-1.33.x86-64.rpm
- 手势检测7-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于python flask实现某瓣数据可视化数据分析平台
- awewq1132323
- 手写流程图检测31-YOLO(v5至v8)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- frida拦截微信小程序云托管API
- 肝脏及其肿瘤分割的 CT 数据集,已经切片成jpg数据,约2w张数据和mask
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


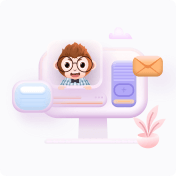
- 1
- 2
前往页