"""
The MIT License (MIT)
Copyright (c) 2014-2015 Dave Parsons & Sam Bingner
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the 'Software'), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
vSMC Header Structure
Offset Length struct Type Description
----------------------------------------
0x00/00 0x08/08 Q ptr Offset to key table
0x08/08 0x04/4 I int Number of private keys
0x0C/12 0x04/4 I int Number of public keys
vSMC Key Data Structure
Offset Length struct Type Description
----------------------------------------
0x00/00 0x04/04 4s int Key name (byte reversed e.g. #KEY is YEK#)
0x04/04 0x01/01 B byte Length of returned data
0x05/05 0x04/04 4s int Data type (byte reversed e.g. ui32 is 23iu)
0x09/09 0x01/01 B byte Flag R/W
0x0A/10 0x06/06 6x byte Padding
0x10/16 0x08/08 Q ptr Internal VMware routine
0x18/24 0x30/48 48B byte Data
"""
import os
import sys
import struct
import subprocess
if sys.version_info < (2, 7):
sys.stderr.write('You need Python 2.7 or later\n')
sys.exit(1)
# Setup imports depending on whether IronPython or CPython
if sys.platform == 'win32' \
or sys.platform == 'cli':
from _winreg import *
def rot13(s):
chars = 'AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz'
trans = chars[26:] + chars[:26]
rot_char = lambda c: trans[chars.find(c)] if chars.find(c) > -1 else c
return ''.join(rot_char(c) for c in s)
def bytetohex(bytestr):
return ''.join(['%02X ' % ord(x) for x in bytestr]).strip()
def printkey(i, offset, smc_key, smc_data):
print str(i+1).zfill(3) \
+ ' ' + hex(offset) \
+ ' ' + smc_key[0][::-1] \
+ ' ' + str(smc_key[1]).zfill(2) \
+ ' ' + smc_key[2][::-1].replace('\x00', ' ') \
+ ' ' + '{0:#0{1}x}'.format(smc_key[3], 4) \
+ ' ' + hex(smc_key[4]) \
+ ' ' + bytetohex(smc_data)
E_CLASS64 = 2;
E_SHT_RELA = 4;
def patchELF(f, oldOffset, newOffset):
f.seek(0)
magic = f.read(4)
if not magic == b'\x7fELF':
raise Exception('Magic number does not match')
ei_class = struct.unpack('=B', f.read(1))[0]
if ei_class != E_CLASS64:
raise Exception('Not 64bit elf header: ' + ei_class)
f.seek(40)
e_shoff = struct.unpack('=Q', f.read(8))[0]
f.seek(58)
e_shentsize = struct.unpack('=H', f.read(2))[0]
e_shnum = struct.unpack('=H', f.read(2))[0]
e_shstrndx = struct.unpack('=H', f.read(2))[0]
#print 'e_shoff: 0x{:x} e_shentsize: 0x{:x} e_shnum:0x{:x} e_shstrndx:0x{:x}'.format(e_shoff, e_shentsize, e_shnum, e_shstrndx)
for i in range(0, e_shnum):
f.seek(e_shoff + i * e_shentsize)
e_sh = struct.unpack('=LLQQQQLLQQ', f.read(e_shentsize))
e_sh_name = e_sh[0]
e_sh_type = e_sh[1]
e_sh_offset = e_sh[4]
e_sh_size = e_sh[5]
e_sh_entsize = e_sh[9]
if e_sh_type == E_SHT_RELA:
e_sh_nument = e_sh_size / e_sh_entsize
#print 'RELA at 0x{:x} with {:d} entries'.format(e_sh_offset, e_sh_nument)
for j in range(0, e_sh_nument):
f.seek(e_sh_offset + e_sh_entsize * j)
rela = struct.unpack('=QQq', f.read(e_sh_entsize))
r_offset = rela[0]
r_info = rela[1]
r_addend = rela[2]
if r_addend == oldOffset:
r_addend = newOffset;
f.seek(e_sh_offset + e_sh_entsize * j)
f.write(struct.pack('=QQq', r_offset, r_info, r_addend))
print 'Relocation modified at: ' + hex(e_sh_offset + e_sh_entsize * j)
def patchkeys(f, vmx, key, osname):
# Setup struct pack string
key_pack = '=4sB4sB6xQ'
# Do Until OSK1 read
i = 0
while True:
# Read key into struct str and data byte str
offset = key + (i * 72)
f.seek(offset)
smc_key = struct.unpack(key_pack, f.read(24))
smc_data = f.read(smc_key[1])
# Reset pointer to beginning of key entry
f.seek(offset)
if smc_key[0] == 'SKL+':
# Use the +LKS data routine for OSK0/1
smc_new_memptr = smc_key[4]
print '+LKS Key: '
printkey(i, offset, smc_key, smc_data)
elif smc_key[0] == '0KSO':
# Write new data routine pointer from +LKS
print 'OSK0 Key Before:'
printkey(i, offset, smc_key, smc_data)
smc_old_memptr = smc_key[4]
f.seek(offset)
f.write(struct.pack(key_pack, smc_key[0], smc_key[1], smc_key[2], smc_key[3], smc_new_memptr))
f.flush()
# Write new data for key
f.seek(offset + 24)
smc_new_data = rot13('bheuneqjbexolgurfrjbeqfthneqrqcy')
f.write(smc_new_data)
f.flush()
# Re-read and print key
f.seek(offset)
smc_key = struct.unpack(key_pack, f.read(24))
smc_data = f.read(smc_key[1])
print 'OSK0 Key After:'
printkey(i, offset, smc_key, smc_data)
elif smc_key[0] == '1KSO':
# Write new data routine pointer from +LKS
print 'OSK1 Key Before:'
printkey(i, offset, smc_key, smc_data)
smc_old_memptr = smc_key[4]
f.seek(offset)
f.write(struct.pack(key_pack, smc_key[0], smc_key[1], smc_key[2], smc_key[3], smc_new_memptr))
f.flush()
# Write new data for key
f.seek(offset + 24)
smc_new_data = rot13('rnfrqbagfgrny(p)NccyrPbzchgreVap')
f.write(smc_new_data)
f.flush()
# Re-read and print key
f.seek(offset)
smc_key = struct.unpack(key_pack, f.read(24))
smc_data = f.read(smc_key[1])
print 'OSK1 Key After:'
printkey(i, offset, smc_key, smc_data)
# Finished so get out of loop
break
else:
pass
i += 1
return smc_old_memptr, smc_new_memptr
def patchsmc(name, osname, sharedobj):
with open(name, 'r+b') as f:
# Read file into string variable
vmx = f.read()
print 'File: ' + name
# Setup hex string for vSMC headers
# These are the private and public key counts
smc_header_v0 = '\xF2\x00\x00\x00\xF0\x00\x00\x00'
smc_header_v1 = '\xB4\x01\x00\x00\xB0\x01\x00\x00'
# Setup hex string for #KEY key
key_key = '\x59\x45\x4B\x23\x04\x32\x33\x69\x75'
# Setup hex string for $Adr key
adr_key = '\x72\x64\x41\x24\x04\x32\x33\x69\x75'
# Find the vSMC headers
smc_header_v0_offset = vmx.find(smc_header_v0) - 8
smc_header_v1_offset = vmx.find(smc_header_v1) - 8
# Find '#KEY' keys
smc_key0 = vmx.find(key_key)
sm
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Unlock All 是 VMware虚拟机安装MAC OS X系统时所需要安装的补丁,需要运行这个补丁之后,才能看到Apple Mac OS X 的安装选项。否则无法加载ISO(DMG)镜像进行安装。所以,如果各位要安装黑苹果的话这个补丁是必须有的,赶紧下载收藏吧。 Unlock-All-v2.1.1 10/10/17 2.1.0 - New version to support ESXi 6.5, Workstation/Player 14 and Fusion 10 - Removed support for ESXi 6.0 - Added ESXi boot option to disable unlocker (nounlocker)
资源推荐
资源详情
资源评论
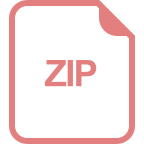
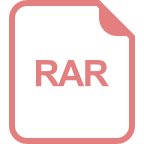
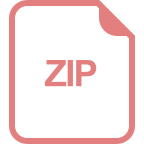
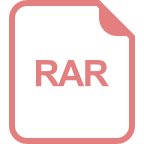
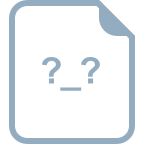
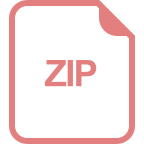
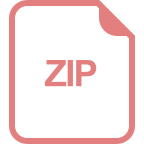
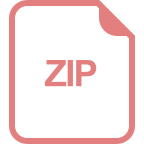
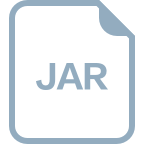
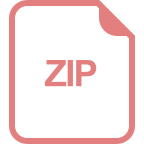
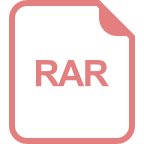
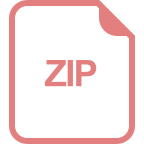
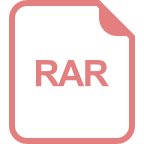
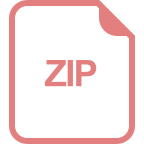
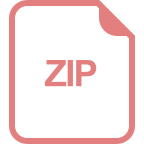
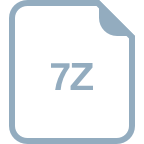
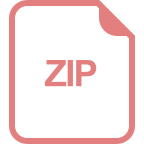
收起资源包目录


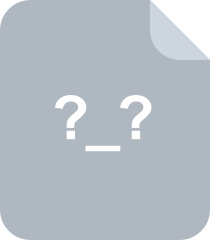
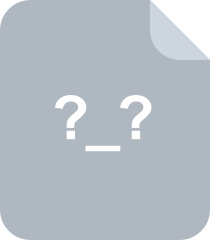
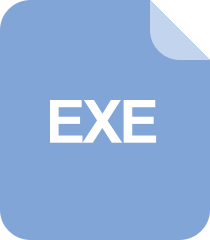
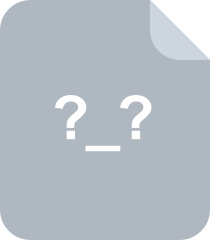

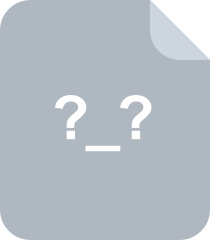
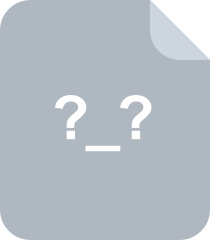
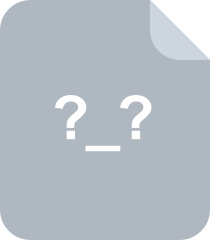
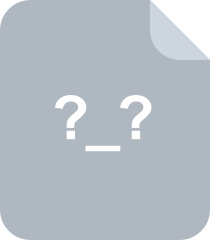

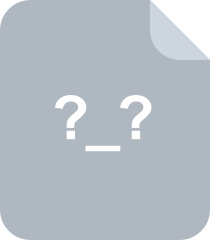
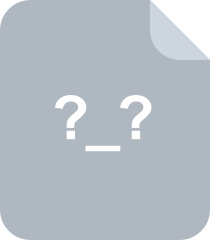
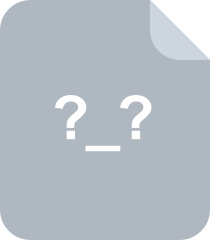
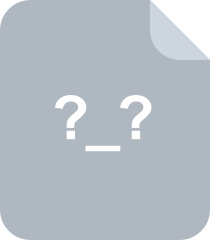
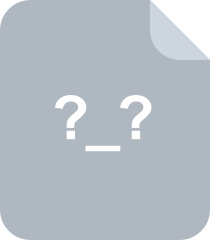
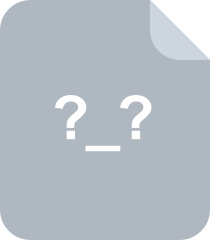
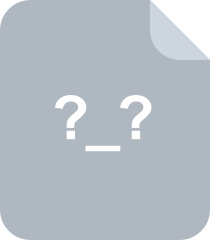
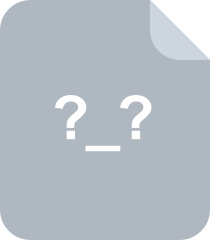
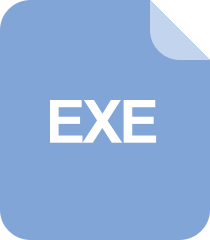
共 17 条
- 1
资源评论
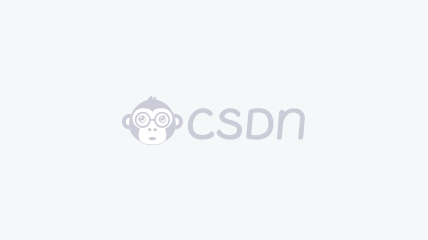
- gzykhm2018-02-10试用中,没成功

lenyo
- 粉丝: 3
- 资源: 15
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

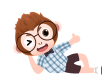
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


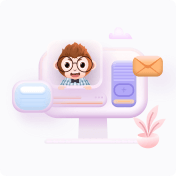
安全验证
文档复制为VIP权益,开通VIP直接复制
