#define OO 1
#define XX 2
#include <afxwin.h>
#include<Windows.h>
#include <iostream>
#include <mmsystem.h>
#include "XXOO.h"
#include "resource.h"
xxooApp theApp;
BOOL xxooApp::InitInstance()
{
m_pMainWnd = new xxooWnd;
m_pMainWnd->ShowWindow(m_nCmdShow);
m_pMainWnd->UpdateWindow();
return TRUE;
}
BEGIN_MESSAGE_MAP(xxooWnd , CFrameWnd)
ON_WM_PAINT()
ON_COMMAND(ID_xExit, &xxooWnd::Onxexit)
ON_COMMAND(ID_xAbout, &xxooWnd::Onxabout)
ON_COMMAND(ID_xNetGame, &xxooWnd::Onxnetgame)
ON_WM_CREATE()
ON_WM_DESTROY()
ON_WM_CLOSE()
ON_WM_LBUTTONDOWN()
ON_COMMAND(ID_xNewGame, &xxooWnd::Onxnewgame)
END_MESSAGE_MAP()
const CRect xxooWnd::m_squre[9] = {
CRect(0 , 0 , 100 , 100) , CRect(110 , 0 , 210, 100), CRect(220 , 0 , 320 , 100),
CRect(0 , 110 , 100 ,210) , CRect(110 , 110 , 210 ,210), CRect(220 , 110 , 320 , 210),
CRect(0 , 220 , 100, 320) , CRect(110 , 220 , 210 ,320), CRect(220 , 220 , 320 , 320),
};
xxooWnd::xxooWnd()
{
CRect rect (0, 0, 330, 365);
Create(0 , _T("XXOO") , WS_CAPTION|WS_SYSMENU, rect , 0 , _T("MENU1") , 0 , 0);
}
void xxooWnd::OnPaint()
{
CPaintDC dc (this);
DrawBoard(&dc);
}
void xxooWnd::DrawBoard(CDC* pDC)
{
CPen pen (PS_SOLID, 10, RGB (0, 0, 0));
CPen* pOldPen = pDC->SelectObject (&pen);
pDC->MoveTo (105, 5);
pDC->LineTo (105, 310);
pDC->MoveTo (215, 5);
pDC->LineTo (215, 310);
pDC->MoveTo (5, 105);
pDC->LineTo (315, 105);
pDC->MoveTo (5, 215);
pDC->LineTo (315, 215);
for (int i=0; i<9; i++)
{
if (m_grid[i] == OO)
DrawO (pDC, i);
else if (m_grid[i] == XX)
DrawX (pDC, i);
}
pDC->SelectObject (pOldPen);
}
void xxooWnd::Onxexit()
{
xxooWnd::OnClose();
// TODO: 在此添加命令处理程序代码
}
void xxooWnd::Onxabout()
{
CDialog dig(IDD_xABOUT,this);
dig.DoModal();
// TODO: 在此添加命令处理程序代码
}
void xxooWnd::Onxnetgame()
{
CDialog dig_net(IDD_xNET,this);
dig_net.DoModal();
// TODO: 在此添加命令处理程序代码
}
int xxooWnd::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CFrameWnd::OnCreate(lpCreateStruct) == -1)
return -1;
ReGame();
PlaySound(_T("Start.wav"),0,0);
// TODO: 在此添加您专用的创建代码
return 0;
}
void xxooWnd::OnClose()
{
// TODO: 在此添加消息处理程序代码和/或调用默认值
CFrameWnd::OnClose();
PlaySound(_T("End.wav") , 0 , 0);
}
void xxooWnd::DrawO(CDC* pDC, int SqNpos)
{
CPen pen(PS_SOLID , 16, RGB(0 , 0, 255));
CPen* OldPen = pDC->SelectObject(&pen);
pDC->SelectStockObject(NULL_BRUSH);
CRect rect = m_squre[SqNpos];
rect.DeflateRect(16 , 16);
pDC->Ellipse(rect);
pDC->SelectObject(OldPen);
}
void xxooWnd::DrawX(CDC* pDC, int SqNpos)
{
CPen pen(PS_SOLID , 16, RGB(0 , 0, 255));
CPen* OldPen = pDC->SelectObject(&pen);
pDC->SelectStockObject(NULL_BRUSH);
CRect rect = m_squre[SqNpos];
rect.DeflateRect (16, 16);
pDC->MoveTo (rect.left, rect.top);
pDC->LineTo (rect.right, rect.bottom);
pDC->MoveTo (rect.left, rect.bottom);
pDC->LineTo (rect.right, rect.top);
pDC->SelectObject(OldPen);
}
int xxooWnd::GetRectID(CPoint point)
{
for (int i=0; i<9; i++)
{
if (m_squre[i].PtInRect (point))
{
return i;
}
}
return -1;
}
void xxooWnd::OnLButtonDown(UINT nFlags, CPoint point)
{
CClientDC dc (this);
if (m_nextone == XX)
{
int npos = GetRectID(point);
if (npos == -1 || (m_grid[npos] != 0))
{
return;
}
DrawO(&dc , npos);
m_grid[npos] = OO;
m_nextone = OO;
GameOver();
return;
}
if (m_nextone == OO)
{
int npos = GetRectID(point);
if (npos == -1 || (m_grid[npos] != 0))
{
return;
}
DrawX(&dc , npos);
m_grid[npos] = XX;
m_nextone = XX;
GameOver();
return;
}
}
int xxooWnd::WhoWin(void)
{
static int nPattern[8][3] = {
0, 1, 2,
3, 4, 5,
6, 7, 8,
0, 3, 6,
1, 4, 7,
2, 5, 8,
0, 4, 8,
2, 4, 6
};
for (int i=0; i<8; i++) {
if ((m_grid[nPattern[i][0]] == OO) && // OO win
(m_grid[nPattern[i][1]] == OO) &&
(m_grid[nPattern[i][2]] == OO))
return OO;
if ((m_grid[nPattern[i][0]] == XX) && //XX win
(m_grid[nPattern[i][1]] == XX) &&
(m_grid[nPattern[i][2]] == XX))
return XX;
}
return 0;
}
void xxooWnd::GameOver(void)
{
int nWinner;
if (nWinner =WhoWin())
{
CString str = (nWinner==OO ) ? _T("OO WIN O(∩_∩)O") : _T("XX WIN (*^__^*) ");
AfxMessageBox(str , MB_OK);
ReGame();
}
else if (IsDraw())
{
AfxMessageBox(_T("平了~") , MB_OK);
ReGame();
}
}
void xxooWnd::ReGame(void)
{
m_nextone = XX;
::ZeroMemory (m_grid, 9 * sizeof (int));
Invalidate ();
}
void xxooWnd::Onxnewgame()
{
ReGame();
// TODO: 在此添加命令处理程序代码
}
bool xxooWnd::IsDraw(void)
{
for (int i=0; i<9; i++) {
if (m_grid[i] == 0)
return FALSE;
}
return TRUE;
}

lehomedai
- 粉丝: 0
- 资源: 1
最新资源
- (源码)基于Spring Boot和Vue的后台管理系统.zip
- 用于将 Power BI 嵌入到您的应用中的 JavaScript 库 查看文档网站和 Wiki 了解更多信息 .zip
- (源码)基于Arduino、Python和Web技术的太阳能监控数据管理系统.zip
- (源码)基于Arduino的CAN总线传感器与执行器通信系统.zip
- (源码)基于C++的智能电力系统通信协议实现.zip
- 用于 Java 的 JSON-RPC.zip
- 用 JavaScript 重新实现计算机科学.zip
- (源码)基于PythonOpenCVYOLOv5DeepSort的猕猴桃自动计数系统.zip
- 用 JavaScript 编写的贪吃蛇游戏 .zip
- (源码)基于ASP.NET Core的美术课程管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


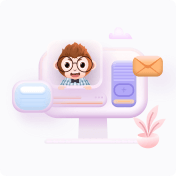