package com.zbw.domain;
import java.util.ArrayList;
import java.util.List;
public class BookExample {
protected String orderByClause;
protected boolean distinct;
protected List<Criteria> oredCriteria;
public BookExample() {
oredCriteria = new ArrayList<Criteria>();
}
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
public String getOrderByClause() {
return orderByClause;
}
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
public boolean isDistinct() {
return distinct;
}
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andBookIdIsNull() {
addCriterion("book_id is null");
return (Criteria) this;
}
public Criteria andBookIdIsNotNull() {
addCriterion("book_id is not null");
return (Criteria) this;
}
public Criteria andBookIdEqualTo(Integer value) {
addCriterion("book_id =", value, "bookId");
return (Criteria) this;
}
public Criteria andBookIdNotEqualTo(Integer value) {
addCriterion("book_id <>", value, "bookId");
return (Criteria) this;
}
public Criteria andBookIdGreaterThan(Integer value) {
addCriterion("book_id >", value, "bookId");
return (Criteria) this;
}
public Criteria andBookIdGreaterThanOrEqualTo(Integer value) {
addCriterion("book_id >=", value, "bookId");
return (Criteria) this;
}
public Criteria andBookIdLessThan(Integer value) {
addCriterion("book_id <", value, "bookId");
return (Criteria) this;
}
public Criteria andBookIdLessThanOrEqualTo(Integer value) {
addCriterion("book_id <=", value, "bookId");
return (Criteria) this;
}
public Criteria andBookIdIn(List<Integer> values) {
addCriterion("book_id in", values, "bookId");
return (Criteria) this;
}
public Criteria andBookIdNotIn(List<Integer> values) {
addCriterion("book_id not in", values, "bookId");
return (Criteria) this;
}
public Criteria andBookIdBetween(Integer value1, Integer value2) {
addCriterion("book_id between", value1, value2, "bookId");
return (Criteria) this;
}
public Criteria andBookIdNotBetween(Integer value1, Integer value2) {
addCriterion("book_id not between", value1, value2, "bookId");
return (Criteria) this;
}
public Criteria andBookNameIsNull() {
addCriterion("book_name is null");
return (Criteria) this;
}
public Criteria andBookNameIsNotNull() {
addCriterion("book_name is not null");
return (Criteria) this;
}
public Criteria andBookNameEqualTo(String value) {
addCriterion("book_name =", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameNotEqualTo(String value) {
addCriterion("book_name <>", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameGreaterThan(String value) {
addCriterion("book_name >", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameGreaterThanOrEqualTo(String value) {
addCriterion("book_name >=", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameLessThan(String value) {
addCriterion("book_name <", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameLessThanOrEqualTo(String value) {
addCriterion("book_name <=", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameLike(String value) {
addCriterion("book_name like", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameNotLike(String value) {
addCriterion("book_name not like", value, "bookName");
return (Criteria) this;
}
public Criteria andBookNameIn(List<String> values) {
addCriterion("book_name in", values, "bookName");
return (Criteria) this;
}
public Criteria andBookNameNotIn(List<String> values) {
addCriterion("book_name not in", values, "bookName");
return (Criteria) this;
}
public Criteria andBookNameBetween(String value1, String value2) {
addCriterion("book_name between", value1, value2, "bookName");
return (Criteria) this;
}
public Criteria andBookNameNotBetween(String value1, String value2) {
addCriterion("book_name not between", value1, value2, "bookName");
return (Criteria) this;
}
public Criteria andBookAuthorIsNull() {
addCriterion("book_author is null");
return (Criteria) this;
}
public Criteria andBookAuthorIsNotNull() {
addCriterion("book_author is not null");
return (Criteria) this;
}
public Criteria andBookAuthorEqualTo(String value) {
addCriterion("book_author =", value, "bookAuthor");
return (Criteria) this;
}
public Criteria andBookAuthorNotEqualTo(String value) {
addCriterion("book_author <>", value, "bookAuthor");
return (Criteria) this;
}
public Criteria andBookAuthorGreaterThan(String value) {
addCriterion("book_author >", value, "bookAuthor");
return (Cri

mYlEaVeiSmVp
- 粉丝: 2212
- 资源: 19万+
最新资源
- 毕业设计-基于树莓派的寝室小监控系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于树莓派的人脸识别系统(调用百度云api)全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于微服务架构实现的智能招聘系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于微服务的商城秒杀系统全部资料+详细文档+高分项目+源码.zip
- 毕业设计-基于微信小程序的共享雨伞租借系统全部资料+详细文档+高分项目+源码.zip
- Delphi 12 控件之DevExpressUniversalTrialCompleteSetup-20241212-Downloadly.ir.rar
- 自动驾驶,AutoWareAuto框架全框架梳理思维导图及代码注释 授人以鱼不如授人以渔,涵盖:融合感知模块,定位模块,决策规划模块,控制模块,预测模块等较为详细的注释(并非每行都有注释)及框架梳理
- cb.zip
- 银行数字化转型程度-根据年报词频计算(2012-2021年).zip
- 基于labview的OneNET云平台数据写入与读取 可通过labview往云台设备写入 读取数据 也可通过手机app查看labview写入的数据,实现实时监控
- 动手学深度学习,沐神版配套代码,所有代码均可在jupyter中运行,内附有极为详尽的代码注释
- abp使用微服务代码示例
- 地热模拟软件OGS手册的中文翻译中英对照版
- python读取西门子s7-300 plc数据,通过调用微信发送给微信联系人
- IMG_20241223_084327.jpg
- IMG_20241223_084327.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


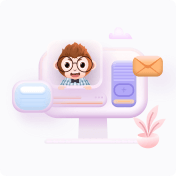