<?php
/**
* CodeIgniter
*
* An open source application development framework for PHP
*
* This content is released under the MIT License (MIT)
*
* Copyright (c) 2014 - 2016, British Columbia Institute of Technology
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
* @package CodeIgniter
* @author EllisLab Dev Team
* @copyright Copyright (c) 2008 - 2014, EllisLab, Inc. (https://ellislab.com/)
* @copyright Copyright (c) 2014 - 2016, British Columbia Institute of Technology (http://bcit.ca/)
* @license http://opensource.org/licenses/MIT MIT License
* @link https://codeigniter.com
* @since Version 1.0.0
* @filesource
*/
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* Query Builder Class
*
* This is the platform-independent base Query Builder implementation class.
*
* @package CodeIgniter
* @subpackage Drivers
* @category Database
* @author EllisLab Dev Team
* @link https://codeigniter.com/user_guide/database/
*/
abstract class CI_DB_query_builder extends CI_DB_driver {
/**
* Return DELETE SQL flag
*
* @var bool
*/
protected $return_delete_sql = FALSE;
/**
* Reset DELETE data flag
*
* @var bool
*/
protected $reset_delete_data = FALSE;
/**
* QB SELECT data
*
* @var array
*/
protected $qb_select = array();
/**
* QB DISTINCT flag
*
* @var bool
*/
protected $qb_distinct = FALSE;
/**
* QB FROM data
*
* @var array
*/
protected $qb_from = array();
/**
* QB JOIN data
*
* @var array
*/
protected $qb_join = array();
/**
* QB WHERE data
*
* @var array
*/
protected $qb_where = array();
/**
* QB GROUP BY data
*
* @var array
*/
protected $qb_groupby = array();
/**
* QB HAVING data
*
* @var array
*/
protected $qb_having = array();
/**
* QB keys
*
* @var array
*/
protected $qb_keys = array();
/**
* QB LIMIT data
*
* @var int
*/
protected $qb_limit = FALSE;
/**
* QB OFFSET data
*
* @var int
*/
protected $qb_offset = FALSE;
/**
* QB ORDER BY data
*
* @var array
*/
protected $qb_orderby = array();
/**
* QB data sets
*
* @var array
*/
protected $qb_set = array();
/**
* QB aliased tables list
*
* @var array
*/
protected $qb_aliased_tables = array();
/**
* QB WHERE group started flag
*
* @var bool
*/
protected $qb_where_group_started = FALSE;
/**
* QB WHERE group count
*
* @var int
*/
protected $qb_where_group_count = 0;
// Query Builder Caching variables
/**
* QB Caching flag
*
* @var bool
*/
protected $qb_caching = FALSE;
/**
* QB Cache exists list
*
* @var array
*/
protected $qb_cache_exists = array();
/**
* QB Cache SELECT data
*
* @var array
*/
protected $qb_cache_select = array();
/**
* QB Cache FROM data
*
* @var array
*/
protected $qb_cache_from = array();
/**
* QB Cache JOIN data
*
* @var array
*/
protected $qb_cache_join = array();
/**
* QB Cache WHERE data
*
* @var array
*/
protected $qb_cache_where = array();
/**
* QB Cache GROUP BY data
*
* @var array
*/
protected $qb_cache_groupby = array();
/**
* QB Cache HAVING data
*
* @var array
*/
protected $qb_cache_having = array();
/**
* QB Cache ORDER BY data
*
* @var array
*/
protected $qb_cache_orderby = array();
/**
* QB Cache data sets
*
* @var array
*/
protected $qb_cache_set = array();
/**
* QB No Escape data
*
* @var array
*/
protected $qb_no_escape = array();
/**
* QB Cache No Escape data
*
* @var array
*/
protected $qb_cache_no_escape = array();
// --------------------------------------------------------------------
/**
* Select
*
* Generates the SELECT portion of the query
*
* @param string
* @param mixed
* @return CI_DB_query_builder
*/
public function select($select = '*', $escape = NULL)
{
if (is_string($select))
{
$select = explode(',', $select);
}
// If the escape value was not set, we will base it on the global setting
is_bool($escape) OR $escape = $this->_protect_identifiers;
foreach ($select as $val)
{
$val = trim($val);
if ($val !== '')
{
$this->qb_select[] = $val;
$this->qb_no_escape[] = $escape;
if ($this->qb_caching === TRUE)
{
$this->qb_cache_select[] = $val;
$this->qb_cache_exists[] = 'select';
$this->qb_cache_no_escape[] = $escape;
}
}
}
return $this;
}
// --------------------------------------------------------------------
/**
* Select Max
*
* Generates a SELECT MAX(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_max($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'MAX');
}
// --------------------------------------------------------------------
/**
* Select Min
*
* Generates a SELECT MIN(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_min($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'MIN');
}
// --------------------------------------------------------------------
/**
* Select Average
*
* Generates a SELECT AVG(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_avg($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'AVG');
}
// --------------------------------------------------------------------
/**
* Select Sum
*
* Generates a SELECT SUM(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_sum($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'SUM');
}
// --------------------------------------------------------------------
/**
* SELECT [MAX|MIN|AVG|SUM]()
*
* @used-by select_max()
* @used-by select_min()
* @used-by select_avg()
* @used-by select_sum()
*
* @param string $select Field name
* @param string $alias
* @param string $type
* @return CI_DB_query_builder
*/
protected function _max_min_avg_sum($select = '', $alias = '', $type = 'MAX')
{
if ( ! is_string($select) OR $select === '')
{
$this->display_error('db_invalid_query');
}
$type = strtoupper($type);
if ( ! in_array($type, array('MAX', 'MIN', 'AVG', 'SUM')))
{
show_error('Invalid function type: '.$type);
}
if ($alias === '')
{
$alias = $this->_create_alias_from_table(trim($select));
}
$sql = $type.'('.$this->protect_identifiers(trim($select)).') AS '.$this->escape_identifiers(trim($alias));
$this->qb_select[] = $sql;
$this->qb_no_escape[] = NULL;
if ($this->qb_caching === TRUE)
{
$this->qb_cache
没有合适的资源?快使用搜索试试~ 我知道了~
《使用vue全家桶+express+php ci+Mysql实现的电影图书查询APP(使用豆瓣API) 》+项目源码+文档说明
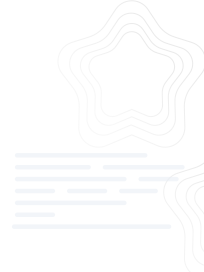
共476个文件
php:207个
jpg:116个
html:47个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 69 浏览量
2024-11-11
22:39:13
上传
评论
收藏 43.79MB ZIP 举报
温馨提示
<项目介绍> - 使用vue全家桶+express+php ci+Mysql实现的电影图书查询APP,使用豆瓣API - 不懂运行,下载完可以私聊问,可远程教学 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。 --------
资源推荐
资源详情
资源评论
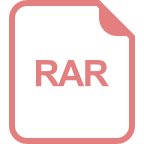
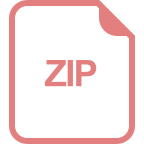
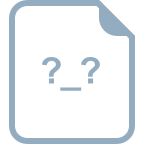
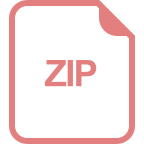
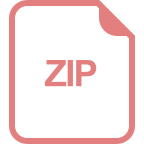
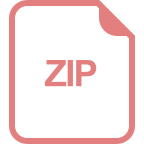
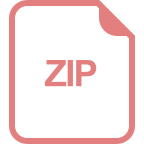
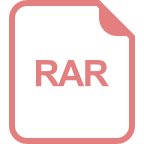
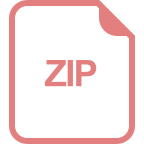
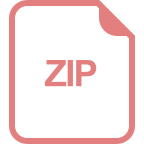
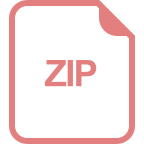
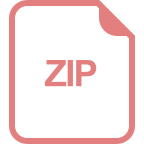
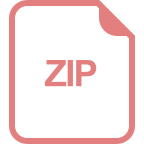
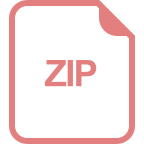
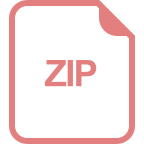
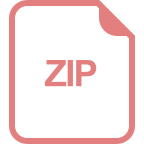
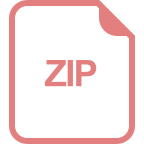
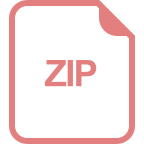
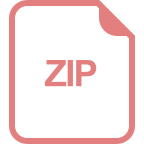
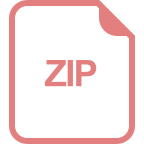
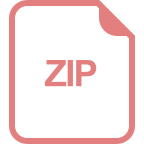
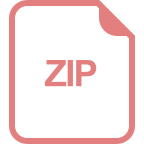
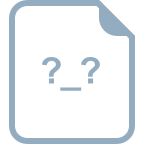
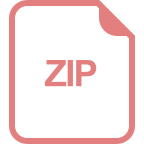
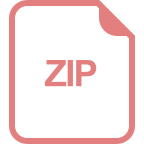
收起资源包目录

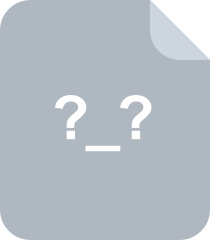
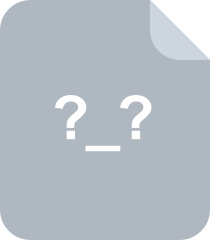
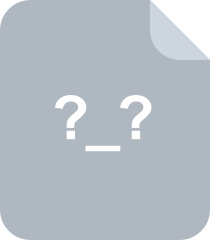
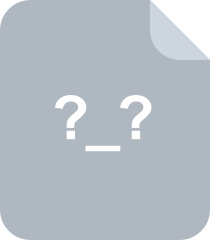
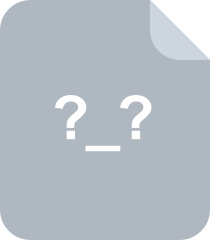
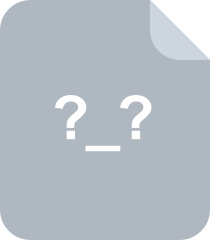
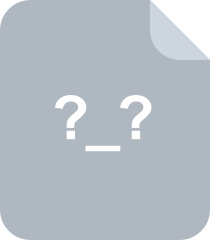
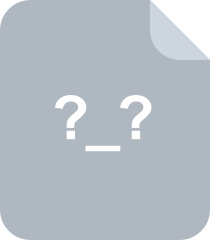
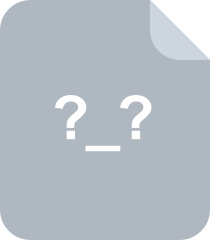
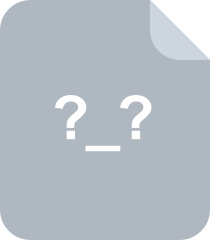
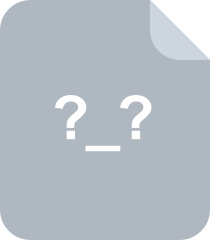
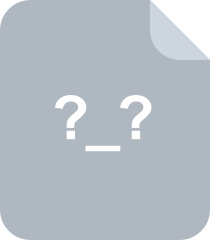
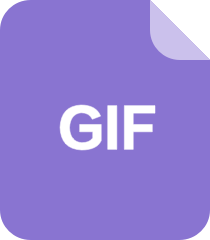
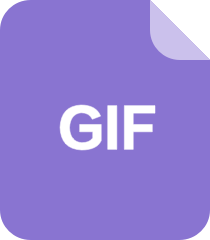
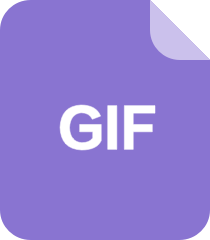
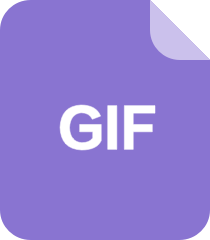
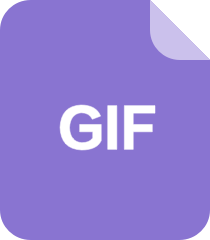
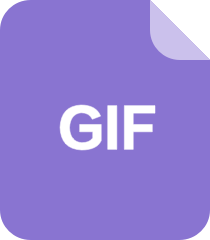
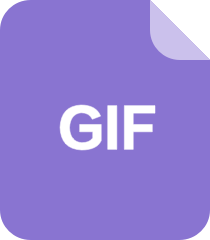
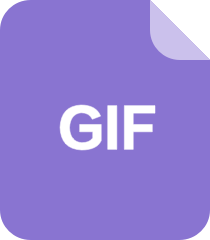
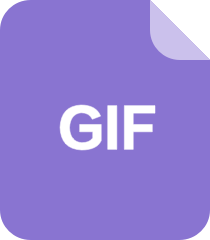
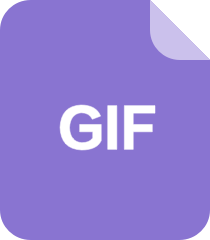
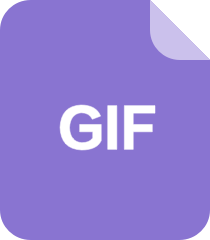
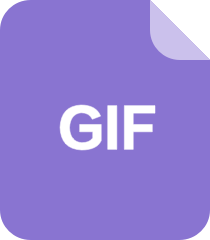
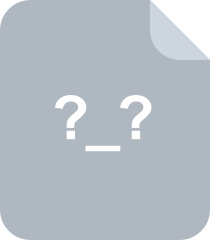
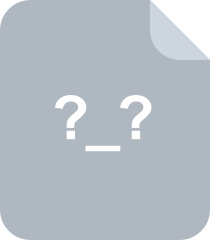
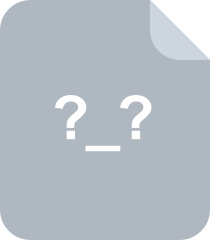
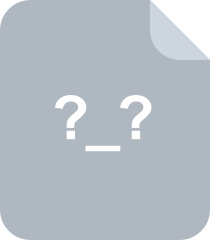
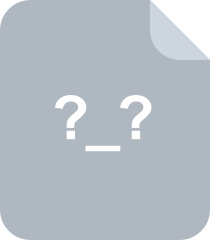
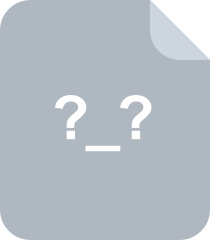
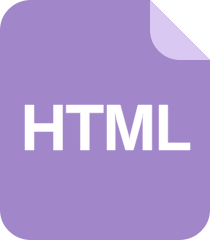
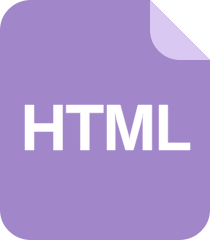
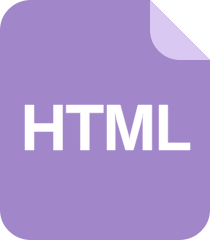
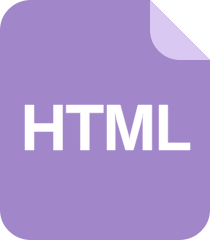
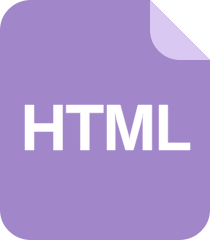
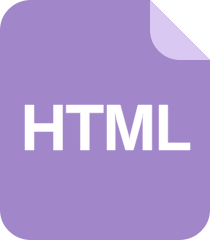
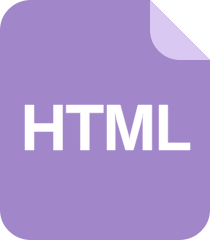
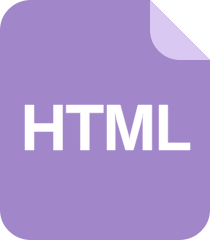
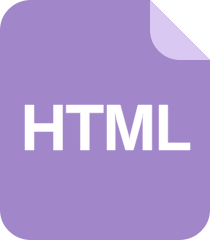
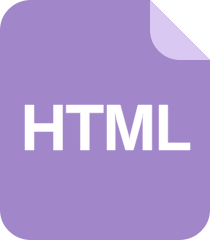
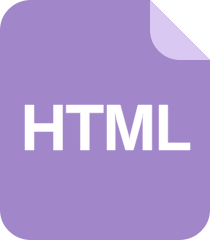
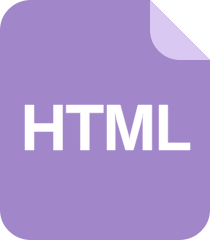
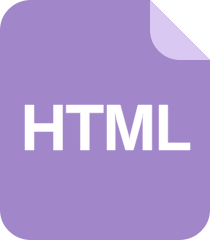
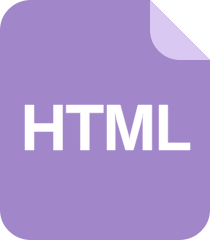
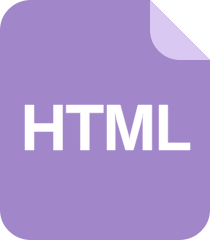
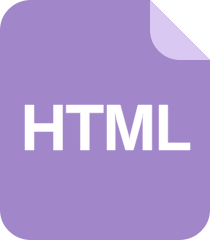
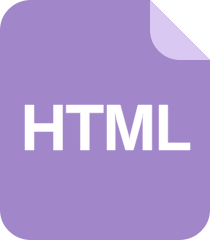
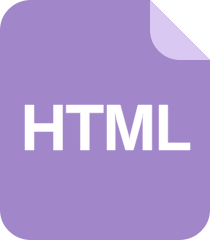
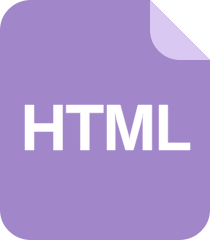
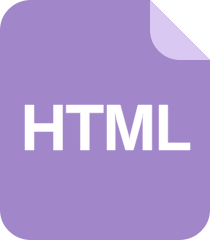
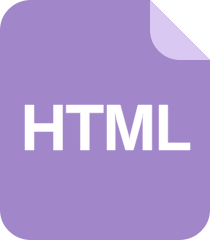
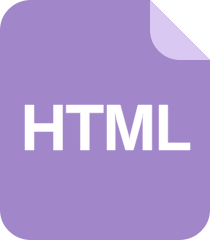
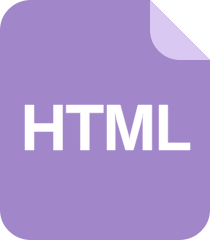
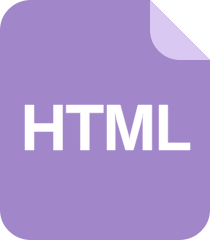
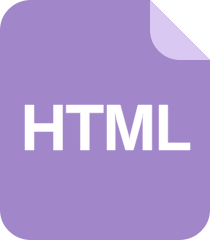
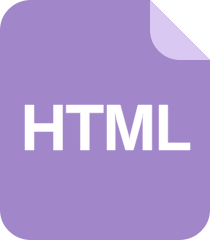
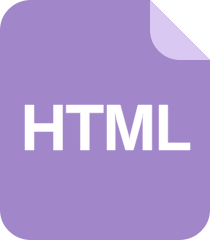
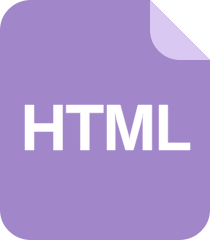
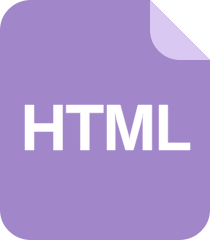
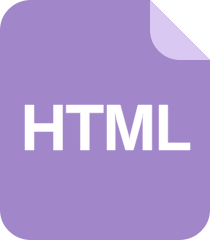
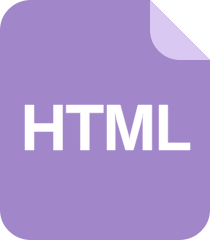
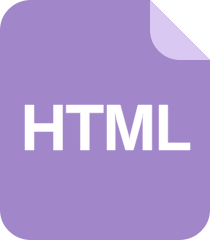
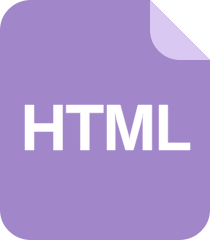
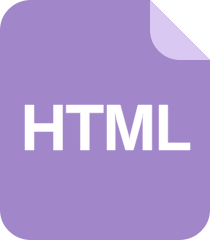
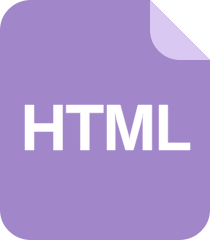
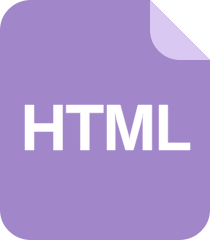
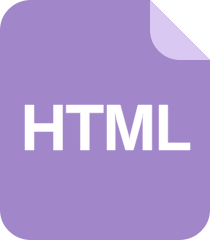
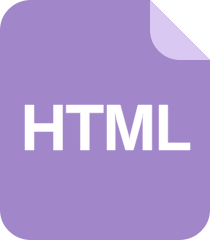
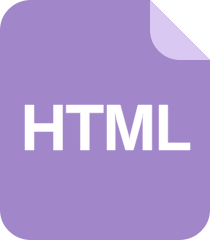
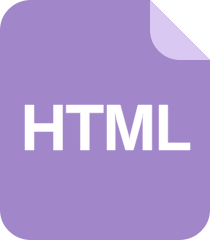
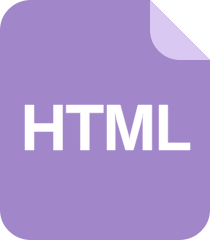
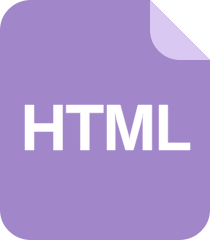
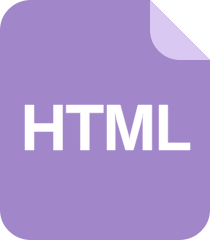
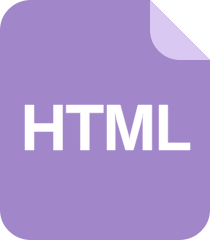
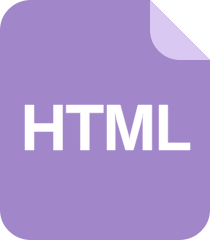
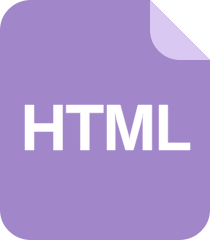
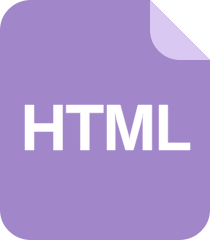
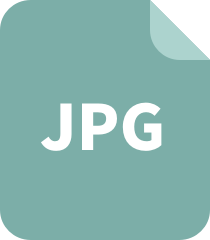
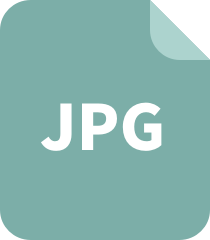
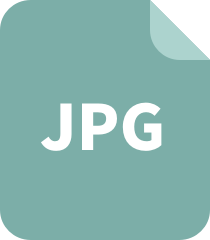
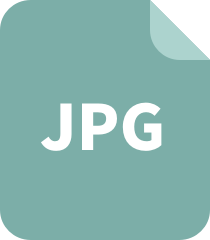
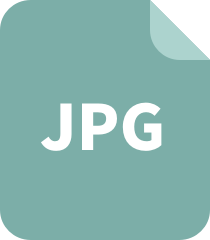
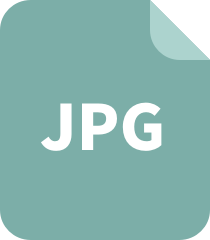
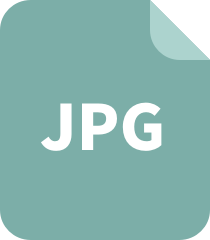
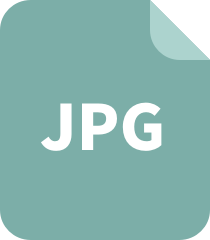
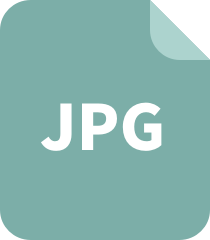
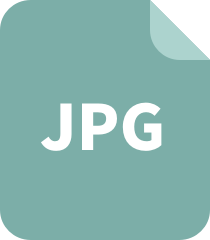
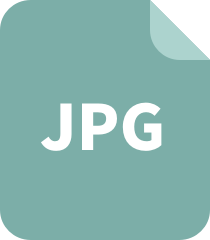
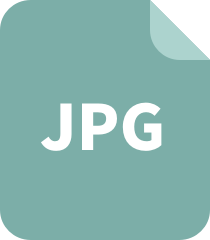
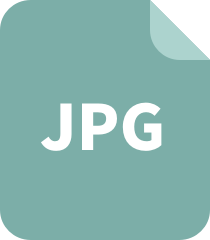
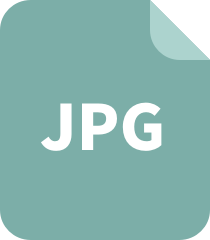
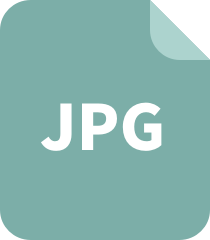
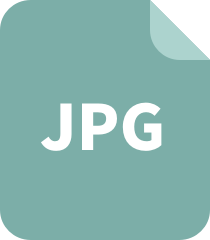
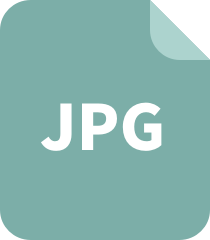
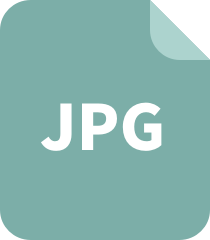
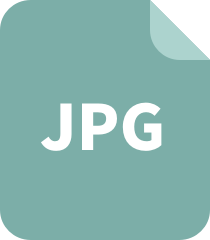
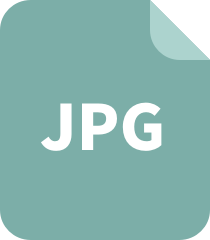
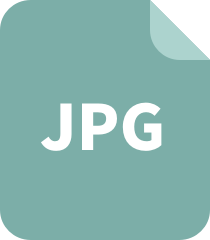
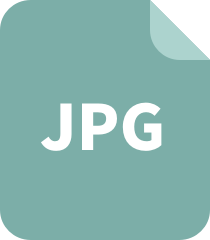
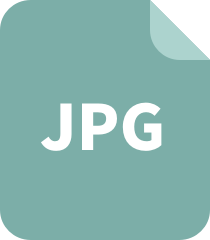
共 476 条
- 1
- 2
- 3
- 4
- 5
资源评论
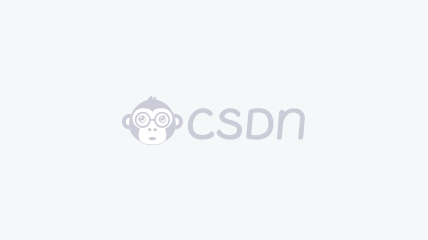

程序员无锋
- 粉丝: 3674
- 资源: 1799

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

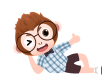
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


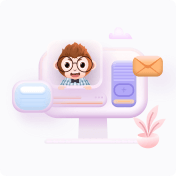
安全验证
文档复制为VIP权益,开通VIP直接复制
