<!doctype html>
<title>CodeMirror: Scala mode</title>
<meta charset="utf-8"/>
<link rel=stylesheet href="../../doc/docs.css">
<link rel="stylesheet" href="../../lib/codemirror.css">
<link rel="stylesheet" href="../../theme/ambiance.css">
<script src="../../lib/codemirror.js"></script>
<script src="../../addon/edit/matchbrackets.js"></script>
<script src="clike.js"></script>
<div id=nav>
<a href="http://codemirror.net"><h1>CodeMirror</h1><img id=logo src="../../doc/logo.png"></a>
<ul>
<li><a href="../../index.html">Home</a>
<li><a href="../../doc/manual.html">Manual</a>
<li><a href="https://github.com/codemirror/codemirror">Code</a>
</ul>
<ul>
<li><a href="../index.html">Language modes</a>
<li><a class=active href="#">Scala</a>
</ul>
</div>
<article>
<h2>Scala mode</h2>
<form>
<textarea id="code" name="code">
/* __ *\
** ________ ___ / / ___ Scala API **
** / __/ __// _ | / / / _ | (c) 2003-2011, LAMP/EPFL **
** __\ \/ /__/ __ |/ /__/ __ | http://scala-lang.org/ **
** /____/\___/_/ |_/____/_/ | | **
** |/ **
\* */
package scala.collection
import generic._
import mutable.{ Builder, ListBuffer }
import annotation.{tailrec, migration, bridge}
import annotation.unchecked.{ uncheckedVariance => uV }
import parallel.ParIterable
/** A template trait for traversable collections of type `Traversable[A]`.
*
* $traversableInfo
* @define mutability
* @define traversableInfo
* This is a base trait of all kinds of $mutability Scala collections. It
* implements the behavior common to all collections, in terms of a method
* `foreach` with signature:
* {{{
* def foreach[U](f: Elem => U): Unit
* }}}
* Collection classes mixing in this trait provide a concrete
* `foreach` method which traverses all the
* elements contained in the collection, applying a given function to each.
* They also need to provide a method `newBuilder`
* which creates a builder for collections of the same kind.
*
* A traversable class might or might not have two properties: strictness
* and orderedness. Neither is represented as a type.
*
* The instances of a strict collection class have all their elements
* computed before they can be used as values. By contrast, instances of
* a non-strict collection class may defer computation of some of their
* elements until after the instance is available as a value.
* A typical example of a non-strict collection class is a
* <a href="../immutable/Stream.html" target="ContentFrame">
* `scala.collection.immutable.Stream`</a>.
* A more general class of examples are `TraversableViews`.
*
* If a collection is an instance of an ordered collection class, traversing
* its elements with `foreach` will always visit elements in the
* same order, even for different runs of the program. If the class is not
* ordered, `foreach` can visit elements in different orders for
* different runs (but it will keep the same order in the same run).'
*
* A typical example of a collection class which is not ordered is a
* `HashMap` of objects. The traversal order for hash maps will
* depend on the hash codes of its elements, and these hash codes might
* differ from one run to the next. By contrast, a `LinkedHashMap`
* is ordered because it's `foreach` method visits elements in the
* order they were inserted into the `HashMap`.
*
* @author Martin Odersky
* @version 2.8
* @since 2.8
* @tparam A the element type of the collection
* @tparam Repr the type of the actual collection containing the elements.
*
* @define Coll Traversable
* @define coll traversable collection
*/
trait TraversableLike[+A, +Repr] extends HasNewBuilder[A, Repr]
with FilterMonadic[A, Repr]
with TraversableOnce[A]
with GenTraversableLike[A, Repr]
with Parallelizable[A, ParIterable[A]]
{
self =>
import Traversable.breaks._
/** The type implementing this traversable */
protected type Self = Repr
/** The collection of type $coll underlying this `TraversableLike` object.
* By default this is implemented as the `TraversableLike` object itself,
* but this can be overridden.
*/
def repr: Repr = this.asInstanceOf[Repr]
/** The underlying collection seen as an instance of `$Coll`.
* By default this is implemented as the current collection object itself,
* but this can be overridden.
*/
protected[this] def thisCollection: Traversable[A] = this.asInstanceOf[Traversable[A]]
/** A conversion from collections of type `Repr` to `$Coll` objects.
* By default this is implemented as just a cast, but this can be overridden.
*/
protected[this] def toCollection(repr: Repr): Traversable[A] = repr.asInstanceOf[Traversable[A]]
/** Creates a new builder for this collection type.
*/
protected[this] def newBuilder: Builder[A, Repr]
protected[this] def parCombiner = ParIterable.newCombiner[A]
/** Applies a function `f` to all elements of this $coll.
*
* Note: this method underlies the implementation of most other bulk operations.
* It's important to implement this method in an efficient way.
*
*
* @param f the function that is applied for its side-effect to every element.
* The result of function `f` is discarded.
*
* @tparam U the type parameter describing the result of function `f`.
* This result will always be ignored. Typically `U` is `Unit`,
* but this is not necessary.
*
* @usecase def foreach(f: A => Unit): Unit
*/
def foreach[U](f: A => U): Unit
/** Tests whether this $coll is empty.
*
* @return `true` if the $coll contain no elements, `false` otherwise.
*/
def isEmpty: Boolean = {
var result = true
breakable {
for (x <- this) {
result = false
break
}
}
result
}
/** Tests whether this $coll is known to have a finite size.
* All strict collections are known to have finite size. For a non-strict collection
* such as `Stream`, the predicate returns `true` if all elements have been computed.
* It returns `false` if the stream is not yet evaluated to the end.
*
* Note: many collection methods will not work on collections of infinite sizes.
*
* @return `true` if this collection is known to have finite size, `false` otherwise.
*/
def hasDefiniteSize = true
def ++[B >: A, That](that: GenTraversableOnce[B])(implicit bf: CanBuildFrom[Repr, B, That]): That = {
val b = bf(repr)
if (that.isInstanceOf[IndexedSeqLike[_, _]]) b.sizeHint(this, that.seq.size)
b ++= thisCollection
b ++= that.seq
b.result
}
@bridge
def ++[B >: A, That](that: TraversableOnce[B])(implicit bf: CanBuildFrom[Repr, B, That]): That =
++(that: GenTraversableOnce[B])(bf)
/** Concatenates this $coll with the elements of a traversable collection.
* It differs from ++ in that the right operand determines the type of the
* resulting collection rather than the left one.
*
* @param that the traversable to append.
* @tparam B the element type of the returned collection.
* @tparam That $thatinfo
* @param bf $bfinfo
* @return a new collection of type `That` which con
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
<项目介绍> 基于JavaWeb的医院挂号及管理系统,使用SSM框架+MySQL实现 - 不懂运行,下载完可以私聊问,可远程教学 该资源内项目源码是个人的毕设,代码都测试ok,都是运行成功后才上传资源,答辩评审平均分达到96分,放心下载使用! 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。 --------
资源推荐
资源详情
资源评论
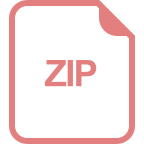
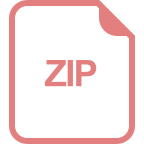
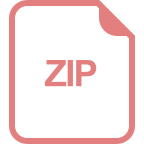
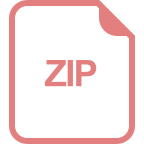
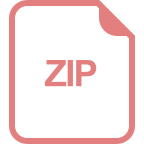
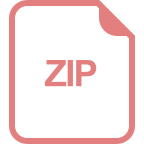
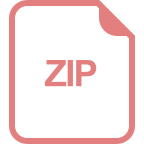
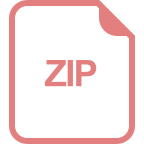
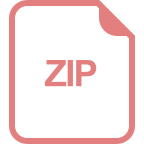
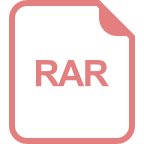
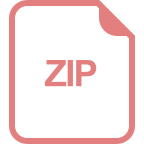
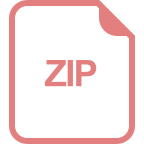
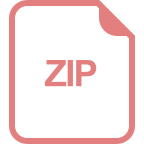
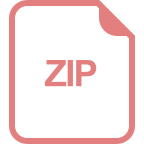
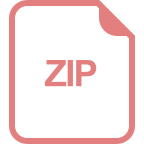
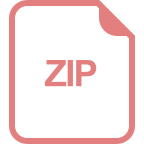
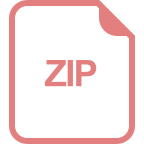
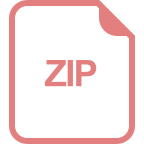
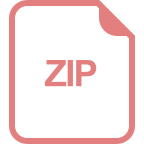
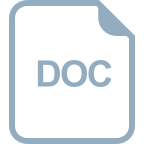
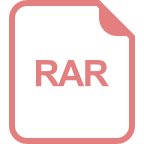
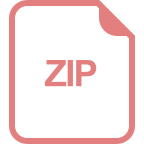
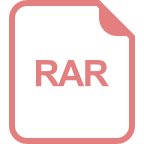
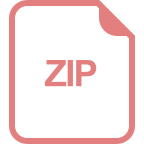
收起资源包目录

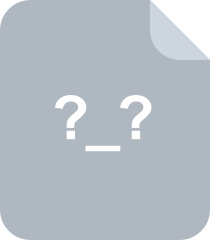
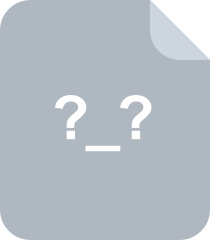
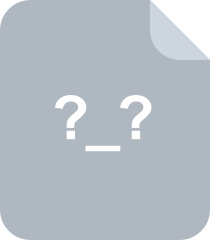
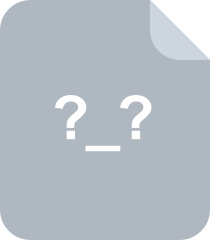
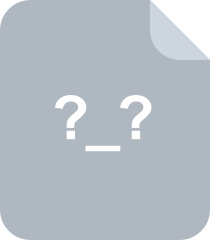
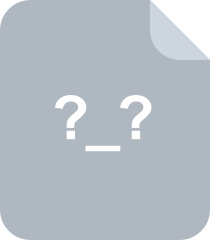
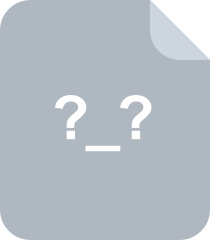
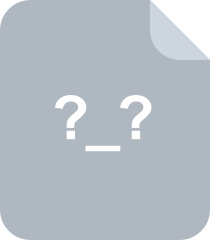
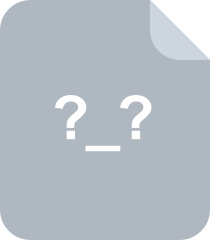
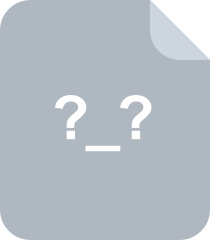
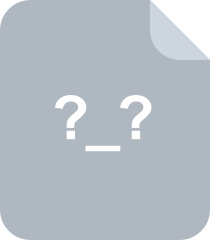
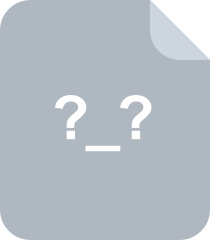
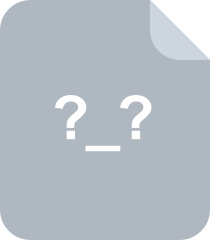
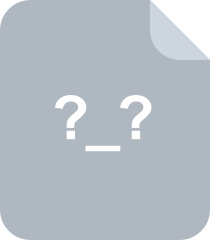
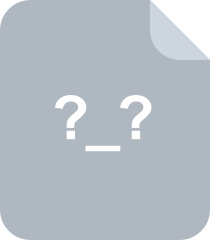
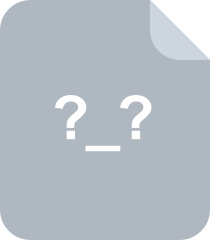
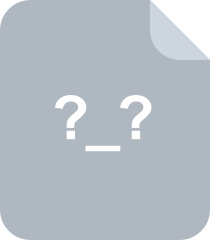
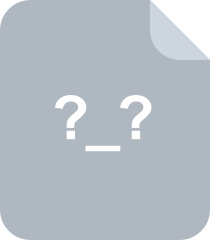
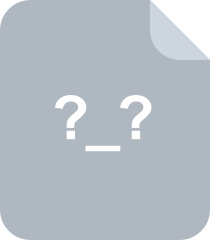
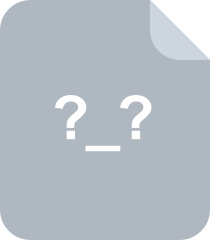
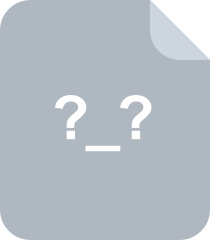
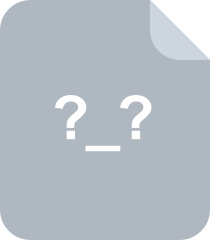
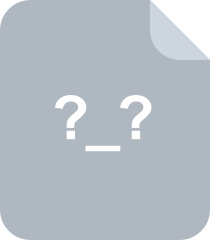
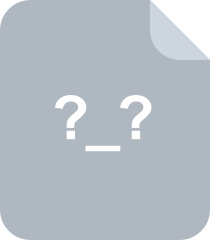
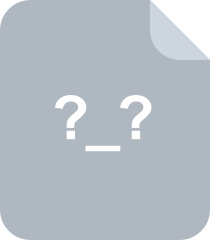
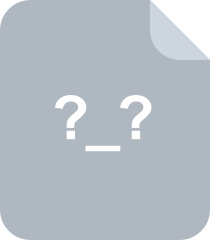
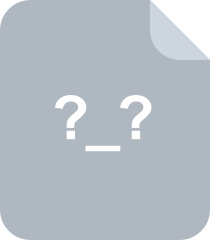
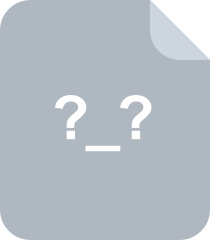
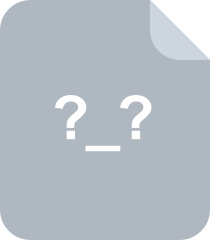
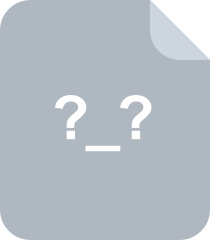
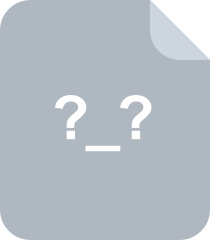
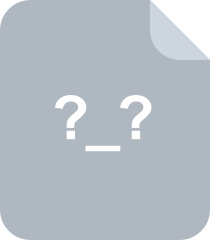
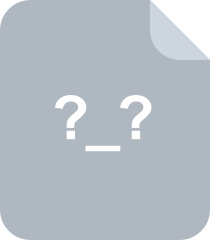
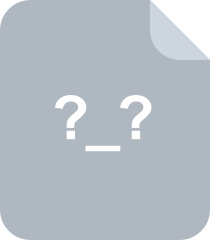
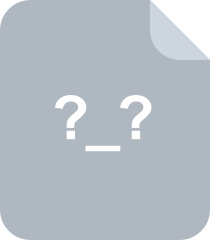
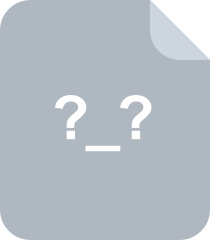
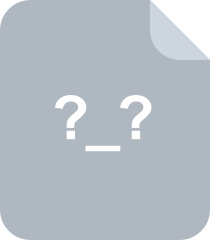
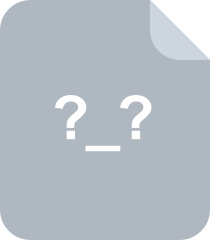
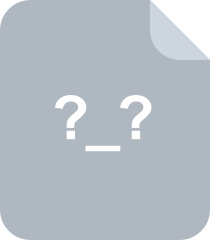
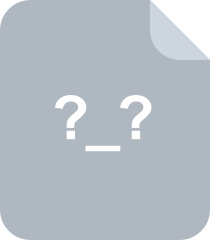
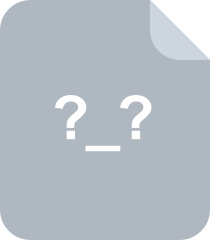
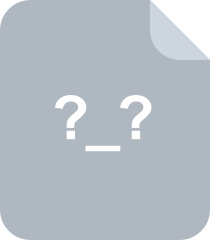
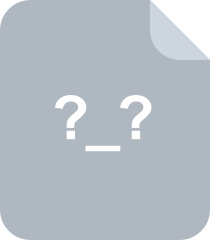
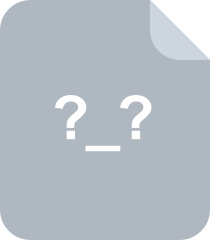
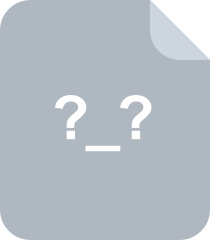
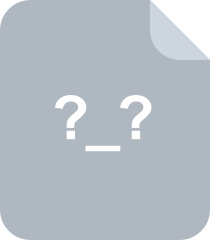
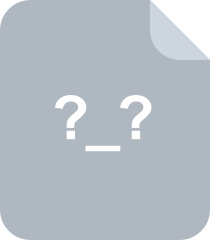
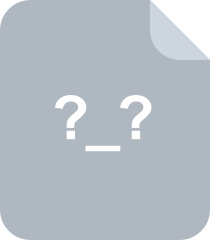
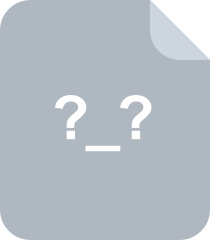
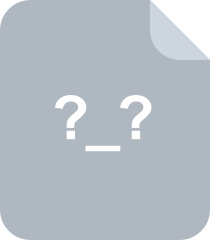
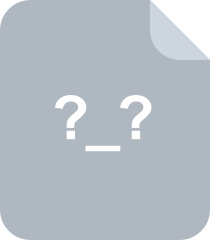
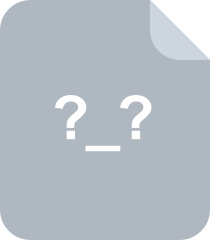
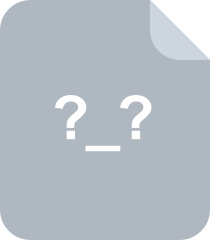
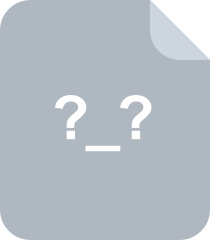
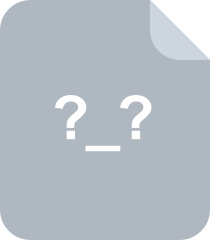
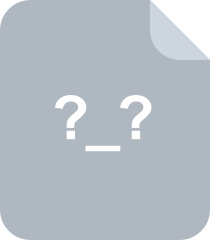
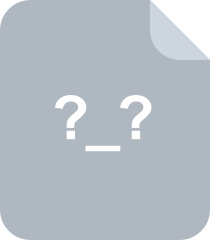
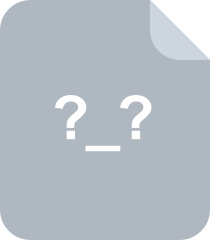
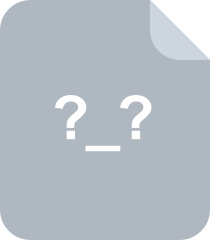
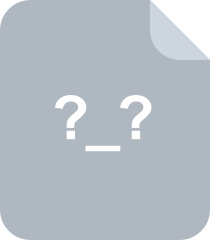
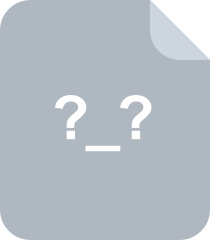
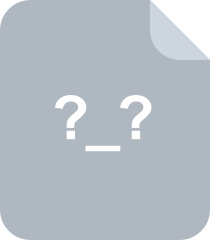
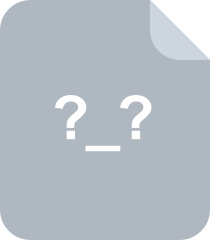
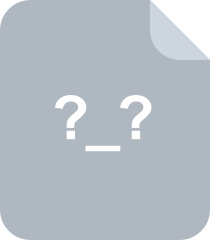
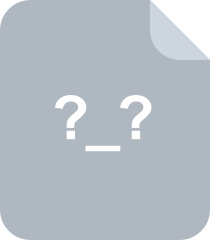
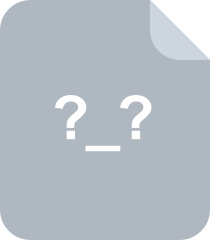
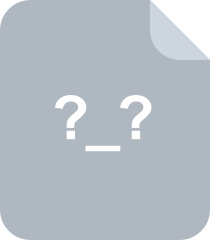
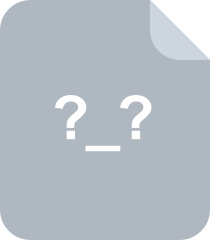
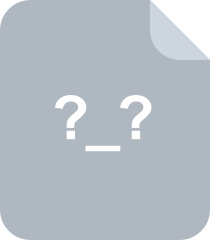
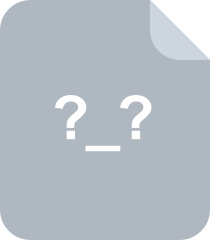
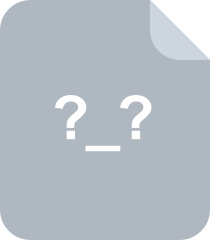
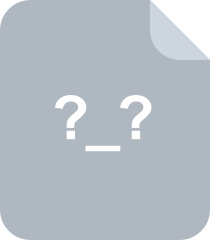
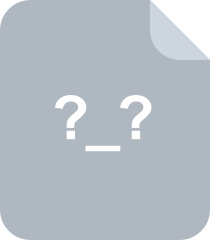
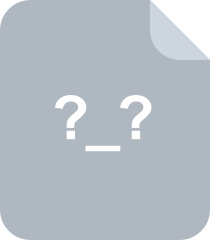
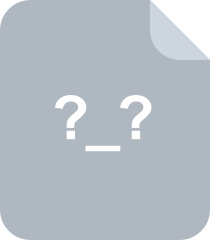
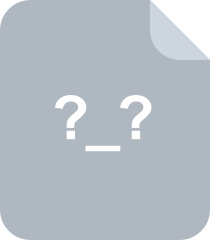
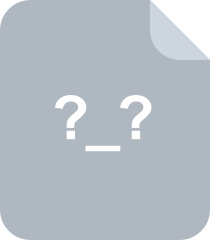
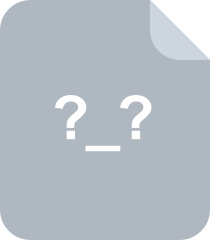
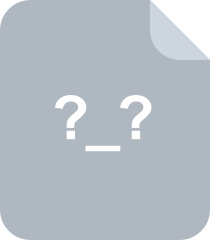
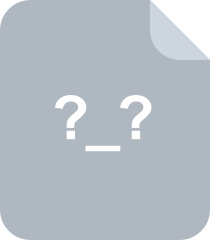
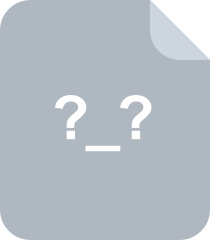
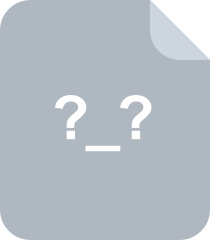
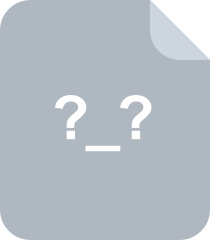
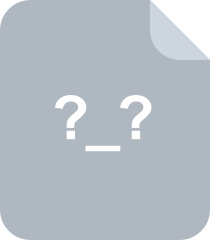
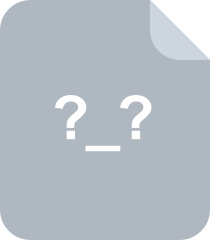
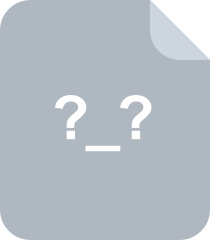
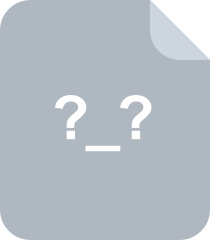
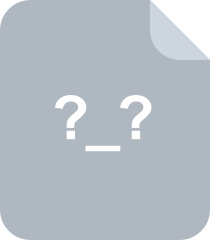
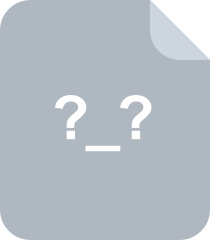
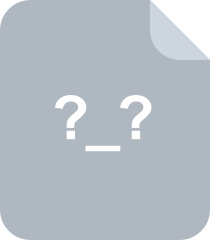
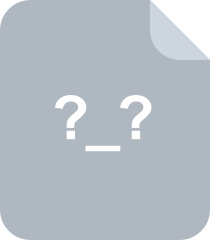
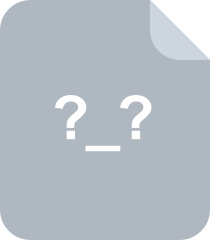
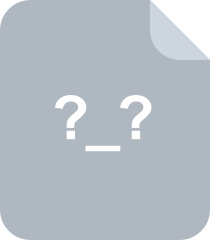
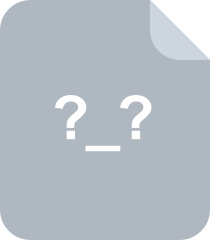
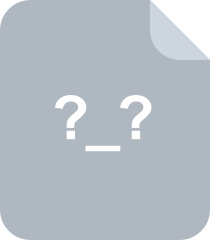
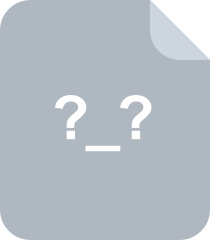
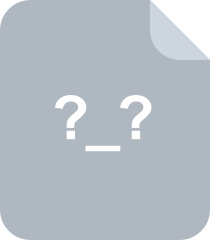
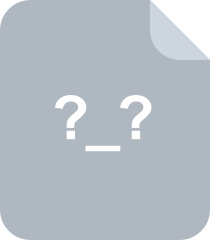
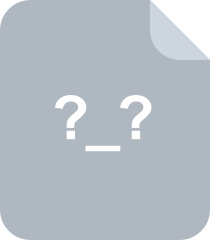
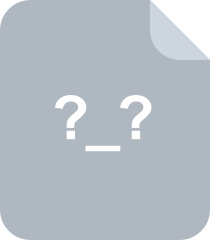
共 1287 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
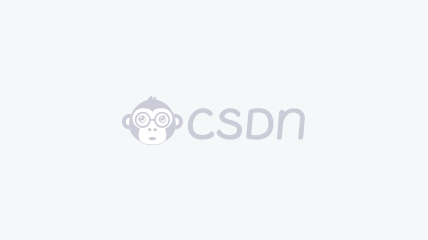

程序员无锋
- 粉丝: 3708
- 资源: 2573

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

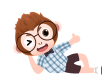
最新资源
- 华为HCIE网络工程师面试题目详解:覆盖二层技术、IGP/BGP路由协议、组播及其他关键技术
- 深度解析Transformer架构:核心技术及其广泛应用与未来展望
- node-v22.13.0-x64.msi
- PDF 早期版本,实用性高
- HCIE网络工程面试精讲之OSPF协议详解及其应用
- 基于java+ssm+mysql的农产品线上销售网站开题报告.docx
- 基于java+ssm+mysql的私人定制旅游系统开题报告.docx
- 电子电路分析与设计 数字电子技术 ((美)尼曼(Donald A. Neamen), 尼曼 (Neaman, Donald A.))
- win11远程连接花屏,需要替换的文件
- 基于java+ssm+mysql的体育用品交易网站开题报告.docx
- Python-3.9.0-amd64.zip
- 基于Matlab实现FSK仿真包括信号产生,调制,加噪声,解调(源码+数据).rar
- docker minio镜像arm64架构
- HCIE网工面试常见问题解析-ISIS协议、BGP协议与组播技术详解及其应用场景
- 3b032大学生心理服务系统_springboot+vue0.zip
- 3b033大学校园防疫与服务系统_springboot+vue.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


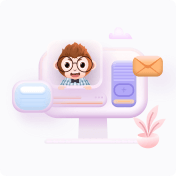
安全验证
文档复制为VIP权益,开通VIP直接复制
