/*
* Copyright (C) 2004-2008 Jive Software, 2021-2022 Ignite Realtime Foundation. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jivesoftware.openfire.muc;
import org.dom4j.DocumentHelper;
import org.dom4j.Element;
import org.dom4j.QName;
import org.jivesoftware.database.JiveID;
import org.jivesoftware.database.SequenceManager;
import org.jivesoftware.openfire.XMPPServer;
import org.jivesoftware.openfire.auth.UnauthorizedException;
import org.jivesoftware.openfire.event.GroupEventListener;
import org.jivesoftware.openfire.group.*;
import org.jivesoftware.openfire.muc.spi.*;
import org.jivesoftware.openfire.user.UserAlreadyExistsException;
import org.jivesoftware.openfire.user.UserNotFoundException;
import org.jivesoftware.util.*;
import org.jivesoftware.util.cache.CacheSizes;
import org.jivesoftware.util.cache.Cacheable;
import org.jivesoftware.util.cache.CannotCalculateSizeException;
import org.jivesoftware.util.cache.ExternalizableUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmpp.packet.*;
import org.xmpp.resultsetmanagement.Result;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.time.Duration;
import java.time.temporal.ChronoUnit;
import java.util.*;
import java.util.concurrent.*;
import java.util.stream.Collectors;
/**
* A chat room on the chat server manages its users, and enforces its own security rules.
*
* A MUCRoom could represent a persistent room which means that its configuration will be maintained in sync with its
* representation in the database, or it represents a non-persistent room. These rooms have no representation in the
* database.
*
* @author Gaston Dombiak
* @author Guus der Kinderen, guus@goodbytes.nl
*/
@JiveID(JiveConstants.MUC_ROOM)
public class MUCRoom implements GroupEventListener, Externalizable, Result, Cacheable {
private static final Logger Log = LoggerFactory.getLogger(MUCRoom.class);
public static final SystemProperty<Boolean> JOIN_PRESENCE_ENABLE = SystemProperty.Builder.ofType(Boolean.class)
.setKey("xmpp.muc.join.presence")
.setDynamic(true)
.setDefaultValue(true)
.build();
private static final SystemProperty<Duration> SELF_PRESENCE_TIMEOUT = SystemProperty.Builder.ofType(Duration.class)
.setKey("xmpp.muc.join.self-presence-timeout")
.setDynamic(true)
.setDefaultValue(Duration.ofSeconds( 2 ))
.setChronoUnit(ChronoUnit.MILLIS)
.build();
public static final SystemProperty<Boolean> ALLOWPM_BLOCKALL = SystemProperty.Builder.ofType( Boolean.class )
.setKey("xmpp.muc.allowpm.blockall")
.setDefaultValue(false)
.setDynamic(true)
.build();
/**
* The service hosting the room.
*/
private MultiUserChatService mucService;
/**
* All occupants that are associated with this room.
*/
public final ArrayList<MUCRole> occupants = new ArrayList<>();
/**
* The name of the room.
*/
private String name;
/**
* The role of the room itself.
*/
private MUCRole role;
/**
* The start time of the chat.
*/
long startTime;
/**
* The end time of the chat.
*/
long endTime;
/**
* After a room has been destroyed it may remain in memory, but it won't be possible to use it.
* When a room is destroyed it is immediately removed from the MultiUserChatService, but it's
* possible that while the room was being destroyed it was being used by another thread, so we
* need to protect the room under these rare circumstances.
*/
public boolean isDestroyed = false;
/**
* ChatRoomHistory object.
*/
private MUCRoomHistory roomHistory;
/**
* Time when the room was locked. A value of zero means that the room is unlocked.
*/
private long lockedTime;
/**
* List of chatroom's owner. The list contains only bare jid.
*/
GroupAwareList<JID> owners = new ConcurrentGroupList<>();
/**
* List of chatroom's admin. The list contains only bare jid.
*/
GroupAwareList<JID> admins = new ConcurrentGroupList<>();
/**
* List of chatroom's members. The list contains only bare jid, mapped to a nickname.
*/
GroupAwareMap<JID, String> members = new ConcurrentGroupMap<>();
/**
* List of chatroom's outcast. The list contains only bare jid of not allowed users.
*/
private GroupAwareList<JID> outcasts = new ConcurrentGroupList<>();
/**
* The natural language name of the room.
*/
private String naturalLanguageName;
/**
* Description of the room. The owner can change the description using the room configuration
* form.
*/
private String description;
/**
* Indicates if occupants are allowed to change the subject of the room.
*/
private boolean canOccupantsChangeSubject;
/**
* Maximum number of occupants that could be present in the room. If the limit's been reached
* and a user tries to join, a not-allowed error will be returned.
*/
private int maxUsers;
/**
* List of roles of which presence will be broadcast to the rest of the occupants. This
* feature is useful for implementing "invisible" occupants.
*/
private List<MUCRole.Role> rolesToBroadcastPresence = new ArrayList<>();
/**
* A public room means that the room is searchable and visible. This means that the room can be
* located using service discovery requests.
*/
private boolean publicRoom;
/**
* Persistent rooms are saved to the database to make sure that rooms configurations can be
* restored in case the server goes down.
*/
private boolean persistent;
/**
* Moderated rooms enable only participants to speak. Users that join the room and aren't
* participants can't speak (they are just visitors).
*/
private boolean moderated;
/**
* A room is considered members-only if an invitation is required in order to enter the room.
* Any user that is not a member of the room won't be able to join the room unless the user
* decides to register with the room (thus becoming a member).
*/
private boolean membersOnly;
/**
* Some rooms may restrict the occupants that are able to send invitations. Sending an
* invitation in a members-only room adds the invitee to the members list.
*/
private boolean canOccupantsInvite;
/**
* The password that every occupant should provide in order to enter the room.
*/
private String password = null;
/**
* Every presence packet can include the JID of every occupant unless the owner deactivates this configuration.
*/
private boolean canAnyoneDiscoverJID;
/**
* The minimal role of persons that are allowed to send private messages in the room.
*/
private String canSendPrivateMessage;
/**
* Enables the logging of the conversation. The conversation in the room will be saved to the
* database.
*/
private boolean logEnabled;
/**
* Enables the logging of the conversation. The conversation in the room will be saved to the
* database.
*/
private boolean loginRestrictedToNickname;
/**
*
没有合适的资源?快使用搜索试试~ 我知道了~
Openfire.zip
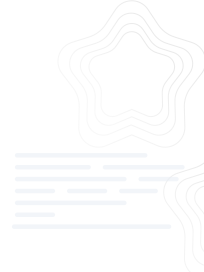
共1584个文件
java:821个
sql:236个
jsp:128个

需积分: 5 0 下载量 56 浏览量
2023-08-19
12:59:21
上传
评论
收藏 8.82MB ZIP 举报
温馨提示
Openfire.zip
资源推荐
资源详情
资源评论
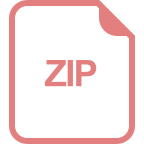
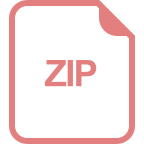
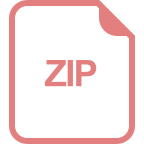
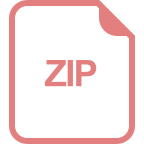
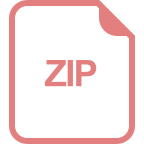
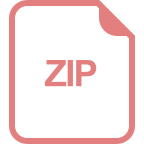
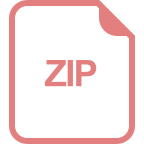
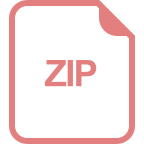
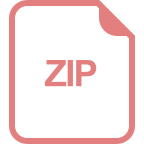
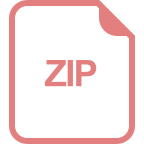
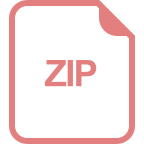
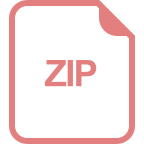
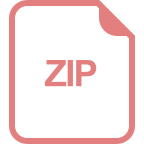
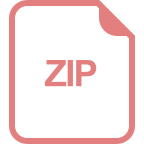
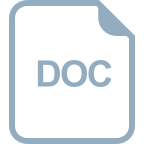
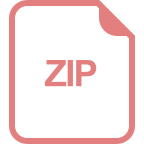
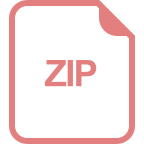
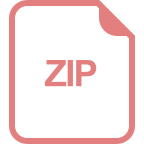
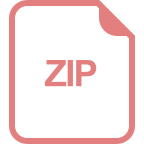
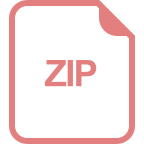
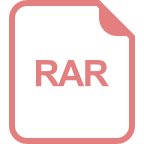
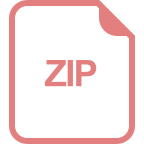
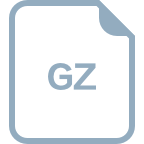
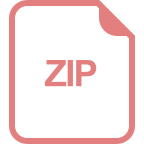
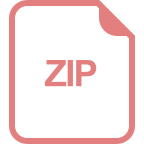
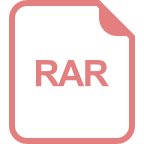
收起资源包目录

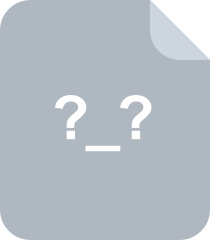
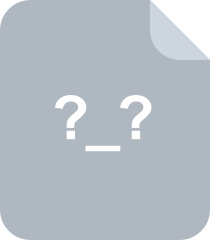
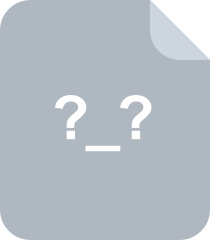
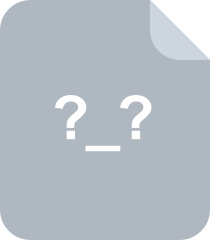
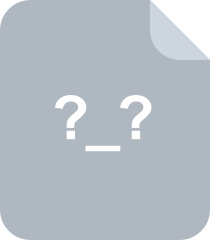
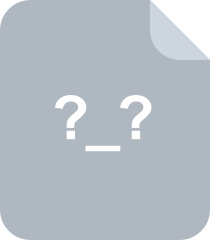
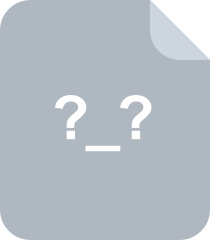
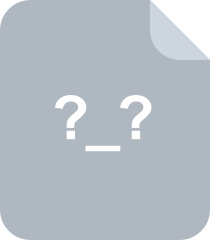
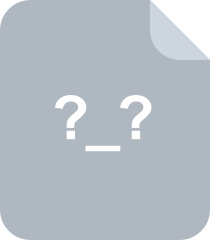
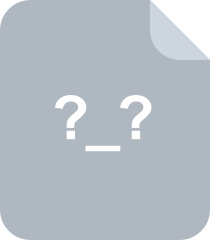
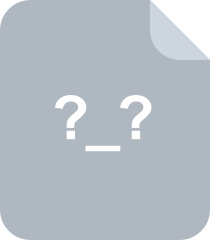
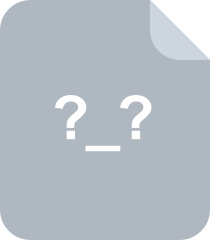
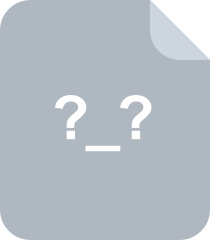
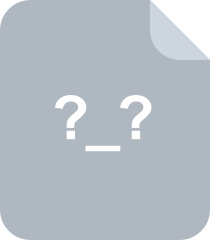
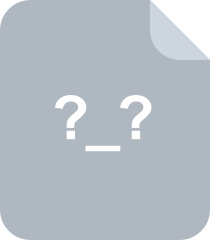
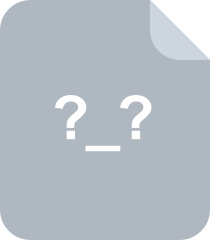
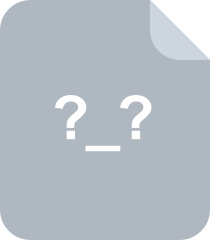
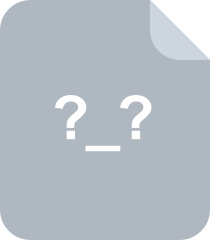
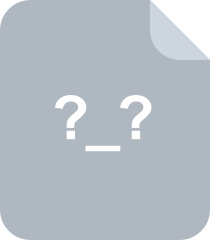
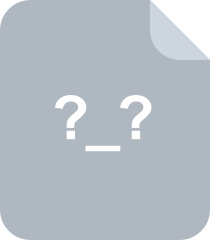
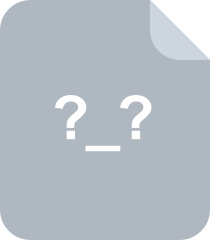
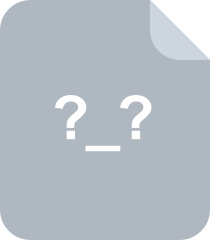
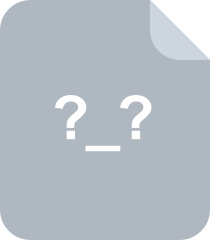
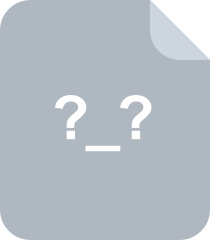
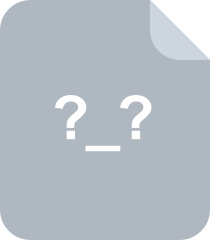
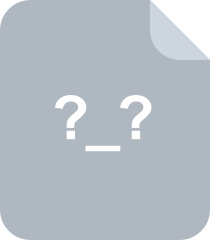
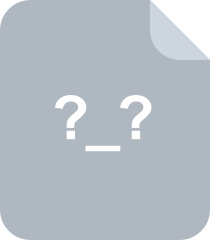
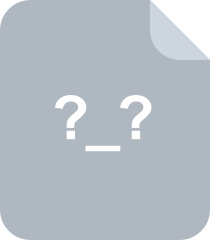
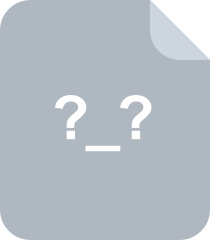
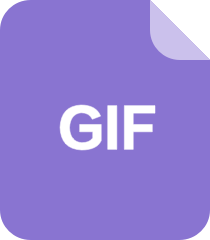
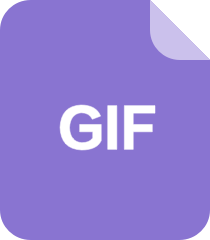
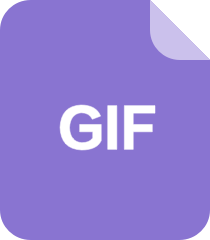
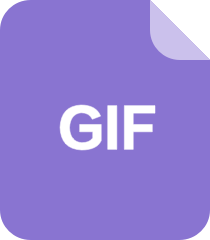
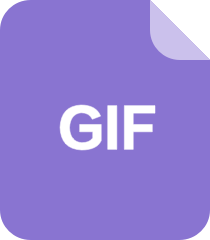
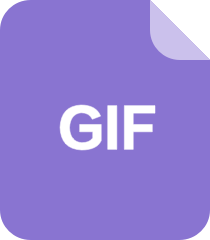
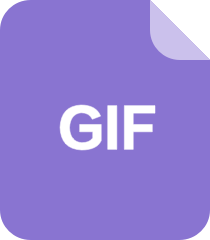
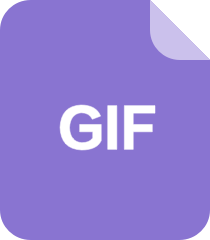
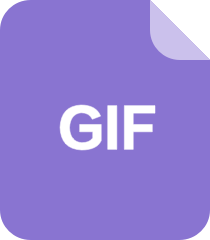
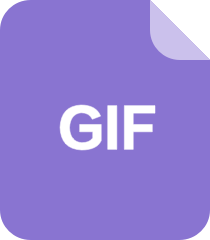
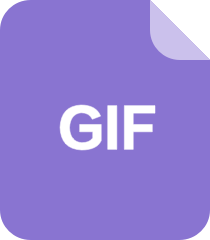
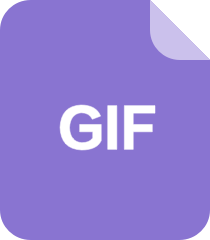
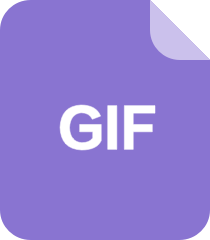
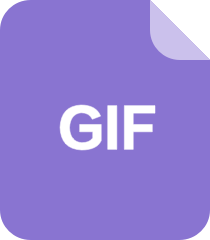
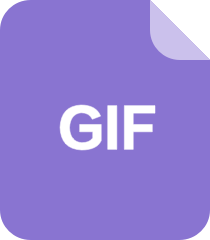
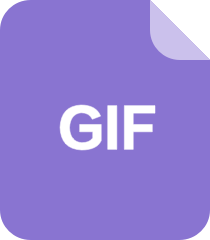
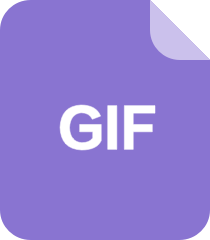
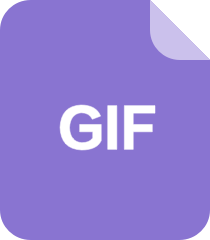
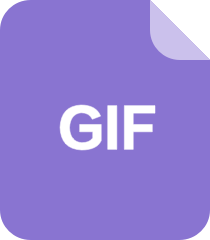
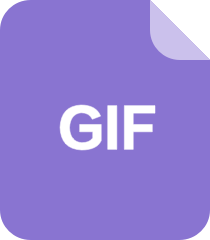
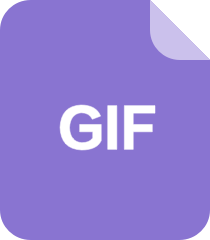
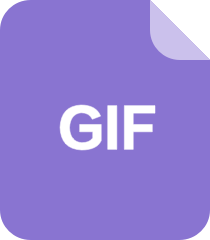
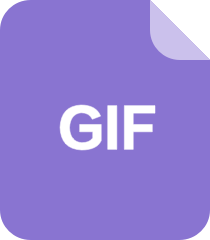
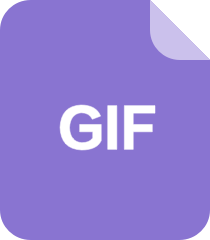
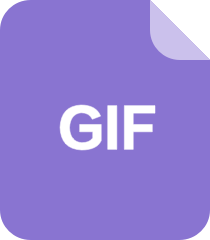
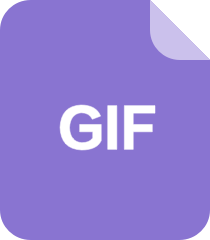
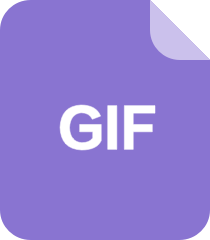
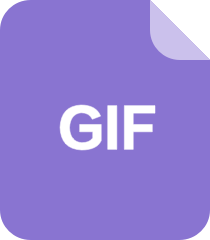
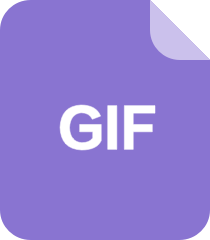
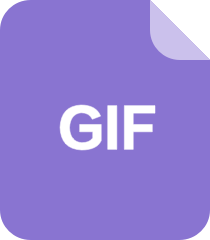
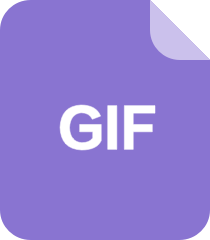
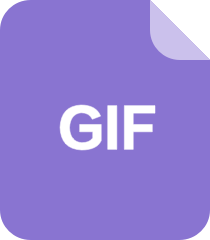
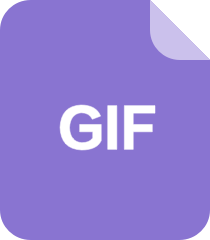
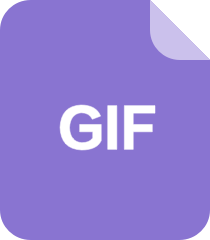
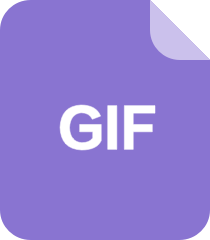
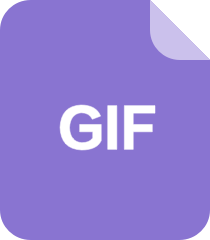
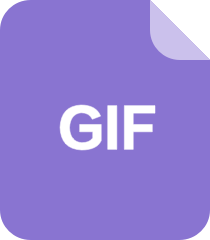
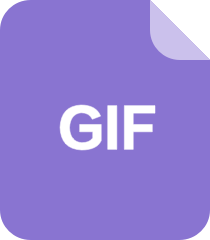
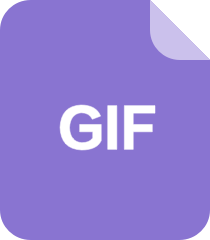
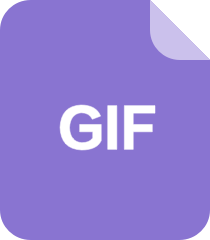
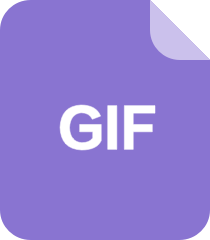
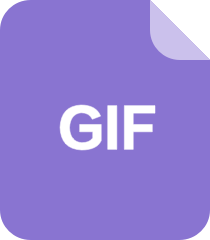
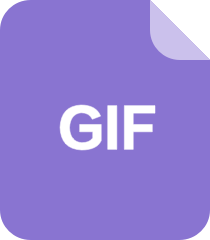
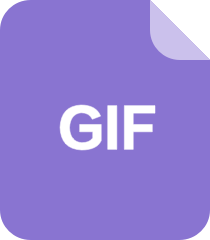
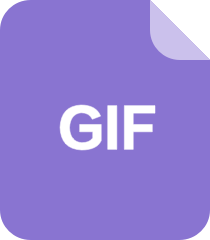
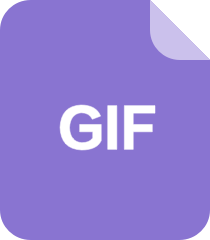
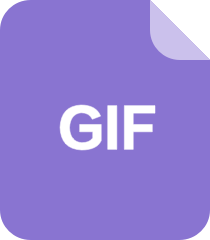
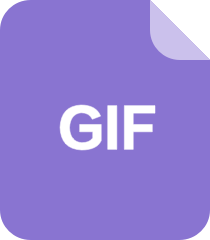
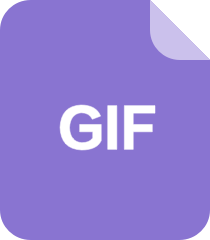
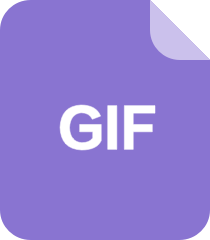
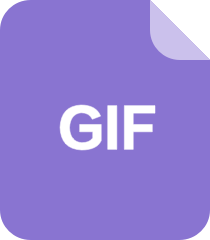
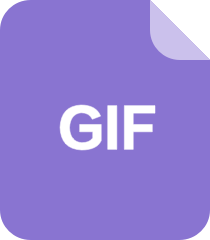
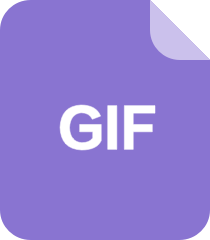
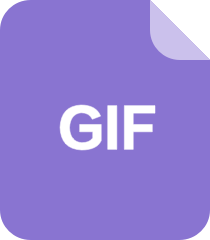
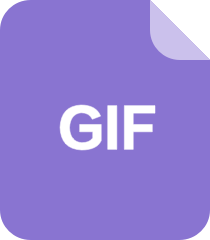
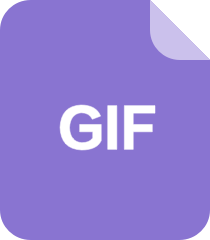
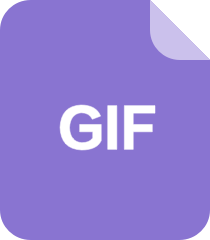
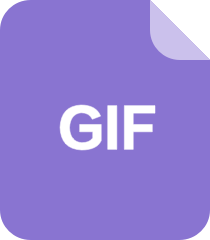
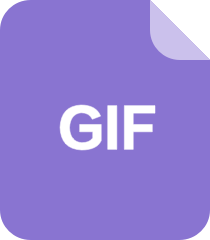
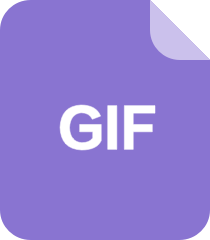
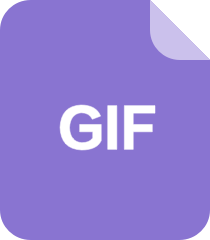
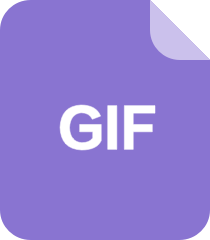
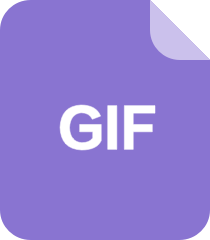
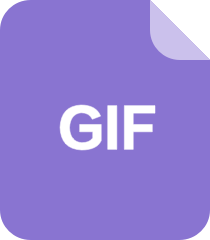
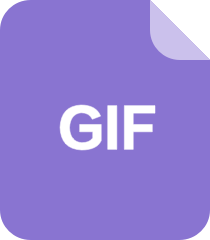
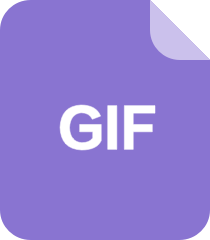
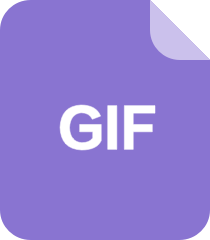
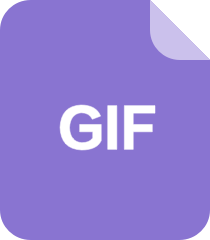
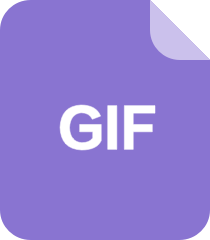
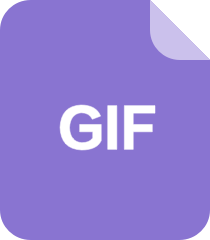
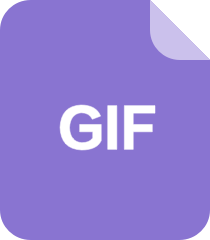
共 1584 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
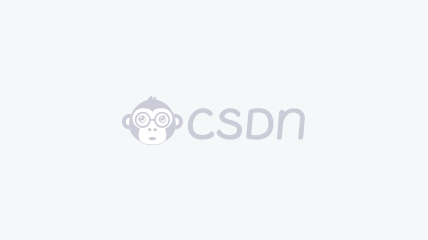

hello_中年人
- 粉丝: 7
- 资源: 324
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

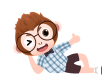
最新资源
- 文字生产视频-可灵1.6
- 特易通 TYTMD-760 V2版 MD-760 V2版固件
- 玄奥八字合婚注册版,,很实用的一个软件
- TYT 特易通 MD-760 V2版升级软件
- 2025年北京幼儿园家长会模板.pptx
- 2025年新学期幼儿园家长会卡通模板.pptx
- 2025年上海幼儿园新学期家长会模板.pptx
- 地球仪电灯炮儿童读书素材班会家长会模板.pptx
- TYTMD-760 V2版写频软件
- 春天柳树风筝素材小学班会家长会模板.pptx
- 成都幼儿园2025年新学期家长会模板.pptx
- 深圳小学一年级家长会通用模板.pptx
- 上海小学三年级卡通班会家长会模板.pptx
- 手绘彩虹元素小学家长会班会模板.pptx
- 向日葵背景元素小学班会家长会模板.pptx
- 长沙卡通2025年幼儿园家长会模板.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


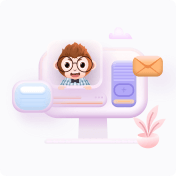
安全验证
文档复制为VIP权益,开通VIP直接复制
