# Dubbo Spring Boot Production-Ready
`dubbo-spring-boot-actuator` provides production-ready features (e.g. [health checks](#health-checks), [endpoints](#endpoints), and [externalized configuration](#externalized-configuration)).
## Content
1. [Main Content](https://github.com/apache/dubbo-spring-boot-project)
2. [Integrate with Maven](#integrate-with-maven)
3. [Health Checks](#health-checks)
4. [Endpoints](#endpoints)
5. [Externalized Configuration](#externalized-configuration)
## Integrate with Maven
You can introduce the latest `dubbo-spring-boot-actuator` to your project by adding the following dependency to your pom.xml
```xml
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-spring-boot-actuator</artifactId>
<version>2.7.4.1</version>
</dependency>
```
If your project failed to resolve the dependency, try to add the following repository:
```xml
<repositories>
<repository>
<id>apache.snapshots.https</id>
<name>Apache Development Snapshot Repository</name>
<url>https://repository.apache.org/content/repositories/snapshots</url>
<releases>
<enabled>false</enabled>
</releases>
<snapshots>
<enabled>true</enabled>
</snapshots>
</repository>
</repositories>
```
## Health Checks
`dubbo-spring-boot-actuator` supports the standard Spring Boot `HealthIndicator` as a production-ready feature , which will be aggregated into Spring Boot's `Health` and exported on `HealthEndpoint` that works MVC (Spring Web MVC) and JMX (Java Management Extensions) both if they are available.
### Web Endpoint : `/health`
Suppose a Spring Boot Web application did not specify `management.server.port`, you can access http://localhost:8080/actuator/health via Web Client and will get a response with JSON format is like below :
```json
{
"status": "UP",
"dubbo": {
"status": "UP",
"memory": {
"source": "management.health.dubbo.status.defaults",
"status": {
"level": "OK",
"message": "max:3641M,total:383M,used:92M,free:291M",
"description": null
}
},
"load": {
"source": "management.health.dubbo.status.extras",
"status": {
"level": "OK",
"message": "load:1.73583984375,cpu:8",
"description": null
}
},
"threadpool": {
"source": "management.health.dubbo.status.extras",
"status": {
"level": "OK",
"message": "Pool status:OK, max:200, core:200, largest:0, active:0, task:0, service port: 12345",
"description": null
}
},
"server": {
"source": "dubbo@ProtocolConfig.getStatus()",
"status": {
"level": "OK",
"message": "/192.168.1.103:12345(clients:0)",
"description": null
}
}
}
// ignore others
}
```
`memory`, `load`, `threadpool` and `server` are Dubbo's build-in `StatusChecker`s in above example.
Dubbo allows the application to extend `StatusChecker`'s SPI.
Default , `memory` and `load` will be added into Dubbo's `HealthIndicator` , it could be overridden by
externalized configuration [`StatusChecker`'s defaults](#statuschecker-defaults).
### JMX Endpoint : `Health`
`Health` is a JMX (Java Management Extensions) Endpoint with ObjectName `org.springframework.boot:type=Endpoint,name=Health` , it can be managed by JMX agent ,e.g. JDK tools : `jconsole` and so on.

### Build-in `StatusChecker`s
`META-INF/dubbo/internal/org.apache.dubbo.common.status.StatusChecker` declares Build-in `StatusChecker`s as follow :
```properties
registry=org.apache.dubbo.registry.status.RegistryStatusChecker
spring=org.apache.dubbo.config.spring.status.SpringStatusChecker
datasource=org.apache.dubbo.config.spring.status.DataSourceStatusChecker
memory=org.apache.dubbo.common.status.support.MemoryStatusChecker
load=org.apache.dubbo.common.status.support.LoadStatusChecker
server=org.apache.dubbo.rpc.protocol.dubbo.status.ServerStatusChecker
threadpool=org.apache.dubbo.rpc.protocol.dubbo.status.ThreadPoolStatusChecker
```
The property key that is name of `StatusChecker` can be a valid value of `management.health.dubbo.status.*` in externalized configuration.
## Endpoints
Actuator endpoint `dubbo` supports Actuator Endpoints :
| ID | Enabled | HTTP URI | HTTP Method | Description | Content Type |
| ------------------- | ----------- | ----------------------------------- | ------------------ | ------------------ | ------------------ |
| `dubbo` | `true` | `/actuator/dubbo` | `GET` | Exposes Dubbo's meta data | `application/json` |
| `dubboproperties` | `true` | `/actuator/dubbo/properties` | `GET` | Exposes all Dubbo's Properties | `application/json` |
| `dubboservices` | `false` | `/dubbo/services` | `GET` | Exposes all Dubbo's `ServiceBean` | `application/json` |
| `dubboreferences` | `false` | `/actuator/dubbo/references` | `GET` | Exposes all Dubbo's `ReferenceBean` | `application/json` |
| `dubboconfigs` | `true` | `/actuator/dubbo/configs` | `GET` | Exposes all Dubbo's `*Config` | `application/json` |
| `dubboshutdown` | `false` | `/actuator/dubbo/shutdown` | `POST` | Shutdown Dubbo services | `application/json` |
### Web Endpoints
#### `/actuator/dubbo`
`/dubbo` exposes Dubbo's meta data :
```json
{
"timestamp": 1516623290166,
"versions": {
"dubbo-spring-boot": "2.7.5",
"dubbo": "2.7.5"
},
"urls": {
"dubbo": "https://github.com/apache/dubbo/",
"google-group": "dev@dubbo.apache.org",
"github": "https://github.com/apache/dubbo-spring-boot-project",
"issues": "https://github.com/apache/dubbo-spring-boot-project/issues",
"git": "https://github.com/apache/dubbo-spring-boot-project.git"
}
}
```
###
#### `/actuator/dubbo/properties`
`/actuator/dubbo/properties` exposes all Dubbo's Properties from Spring Boot Externalized Configuration (a.k.a `PropertySources`) :
```json
{
"dubbo.application.id": "dubbo-provider-demo",
"dubbo.application.name": "dubbo-provider-demo",
"dubbo.application.qos-enable": "false",
"dubbo.application.qos-port": "33333",
"dubbo.protocol.id": "dubbo",
"dubbo.protocol.name": "dubbo",
"dubbo.protocol.port": "12345",
"dubbo.registry.address": "N/A",
"dubbo.registry.id": "my-registry",
"dubbo.scan.basePackages": "org.apache.dubbo.spring.boot.sample.provider.service"
}
```
The structure of JSON is simple Key-Value format , the key is property name as and the value is property value.
#### `/actuator/dubbo/services`
`/actuator/dubbo/services` exposes all Dubbo's `ServiceBean` that are declared via `<dubbo:service/>` or `@Service` present in Spring `ApplicationContext` :
```json
{
"ServiceBean@org.apache.dubbo.spring.boot.sample.api.DemoService#defaultDemoService": {
"accesslog": null,
"actives": null,
"cache": null,
"callbacks": null,
"class": "org.apache.dubbo.config.spring.ServiceBean",
"cluster": null,
"connections": null,
"delay": null,
"document": null,
"executes": null,
"export": null,
"exported": true,
"filter": "",
"generic": "false",
"group": null,
"id": "org.apache.dubbo.spring.boot.sample.api.DemoService",
"interface": "org.apache.dubbo.spring.boot.sample.api.DemoService",
"interfaceClass": "org.apache.dubbo.spring.boot.sample.api.DemoService",
"layer": null,
"listener": "",
"loadbalance": null,
"local": null,
"merger": null,
"mock": null,
"onconnect": null,
"ondisconnect": null,
"owner": null,
"path": "org.apache.dubbo.spring.boot.sample.api.DemoService",
"proxy": null,
"retries": null,
"scope": null,
"sent": null,
"stub": null,
"timeout": null,
"token": null,
"unexported": false,
"uniqueServiceNam
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
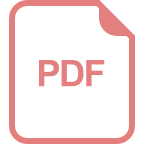
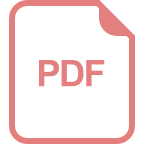
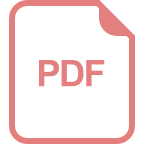
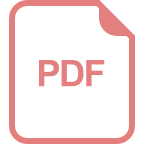
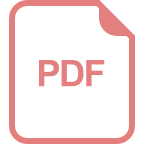
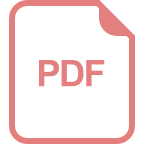
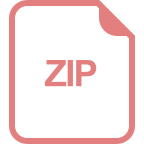
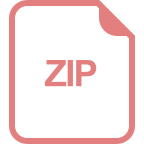
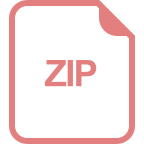
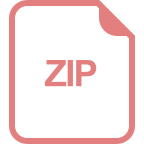
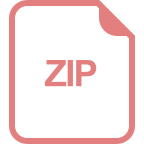
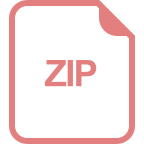
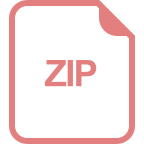
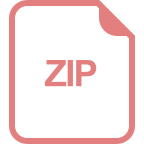
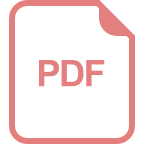
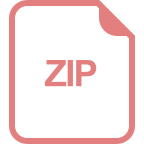
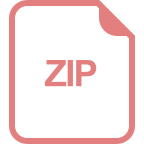
收起资源包目录

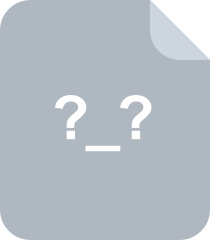
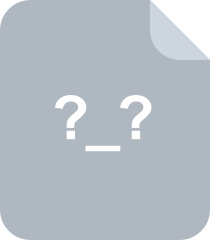
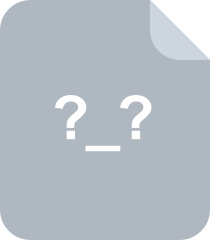
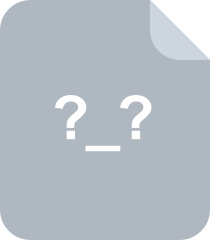
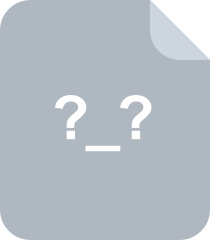
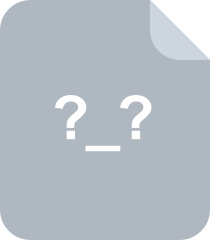
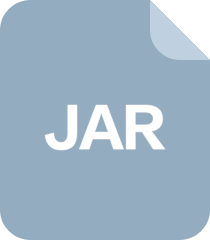
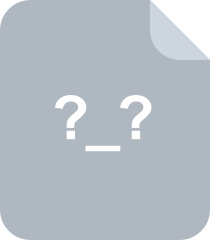
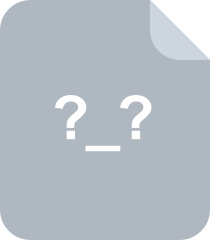
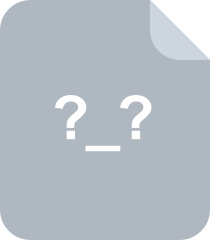
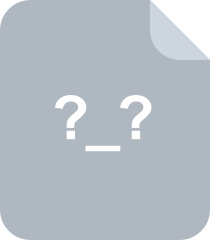
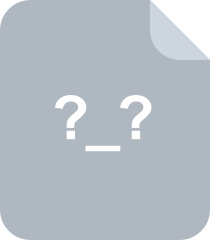
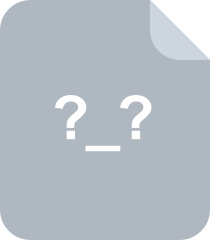
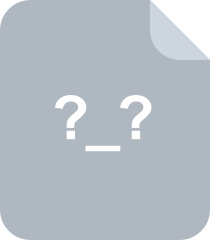
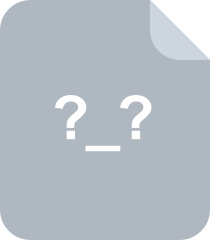
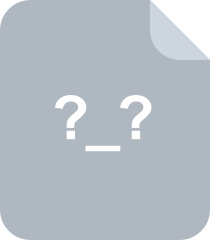
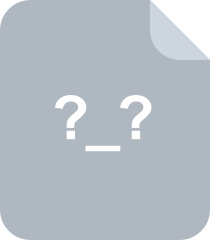
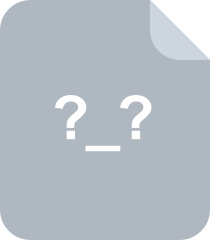
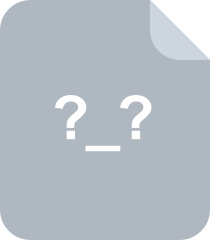
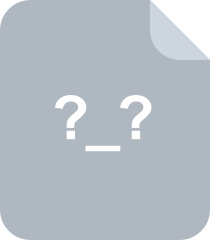
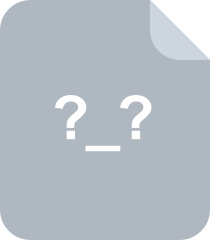
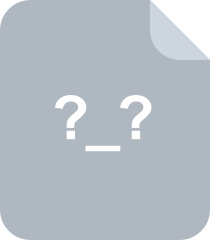
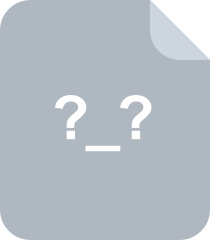
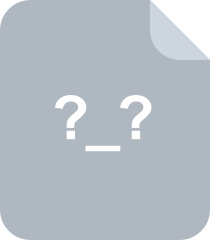
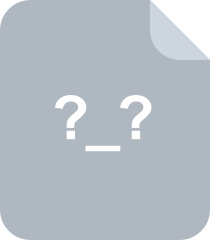
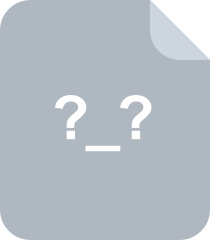
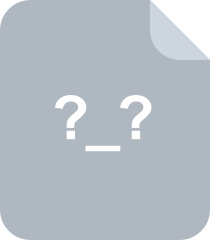
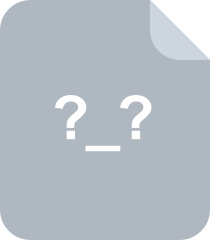
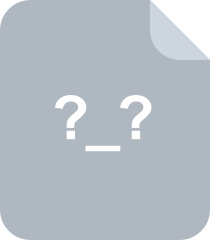
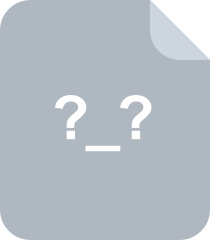
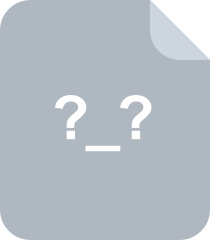
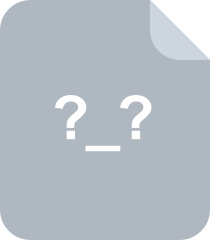
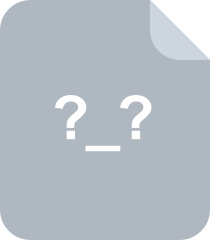
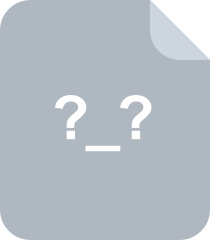
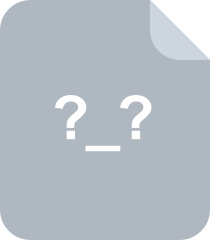
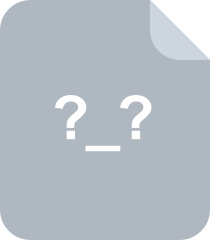
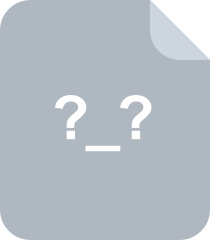
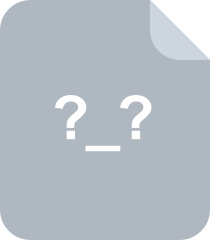
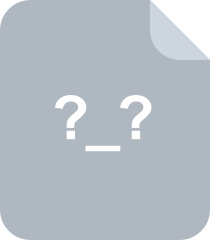
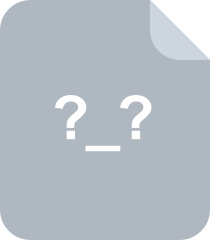
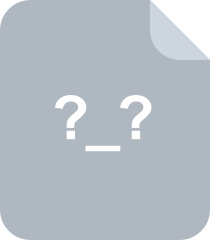
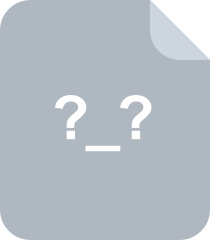
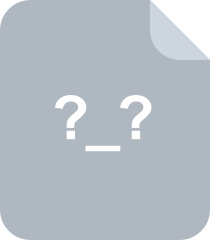
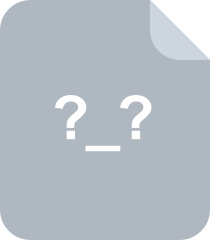
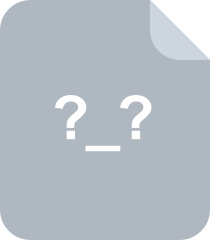
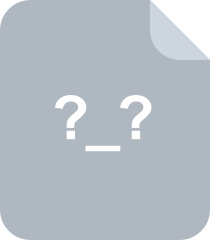
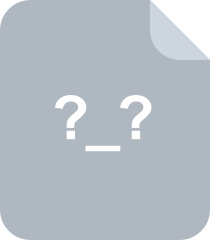
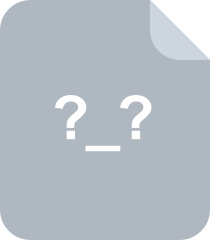
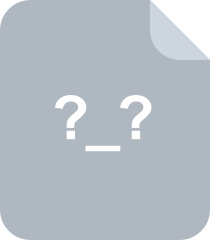
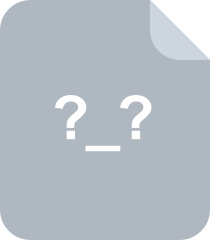
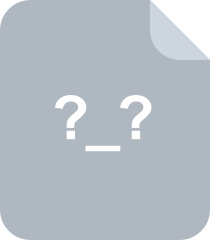
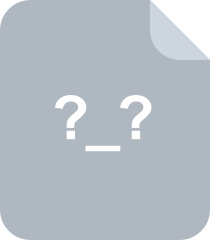
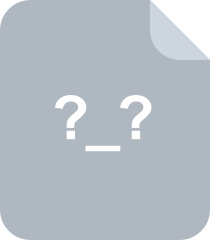
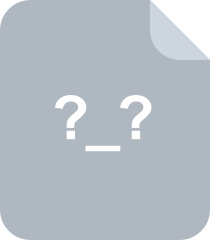
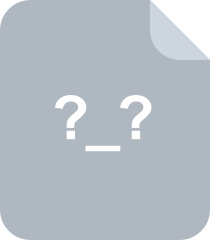
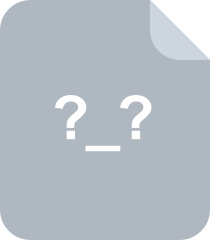
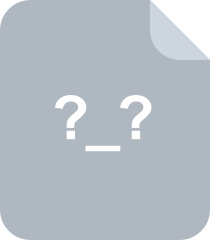
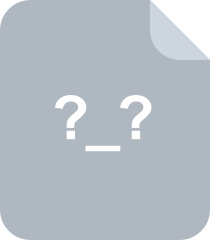
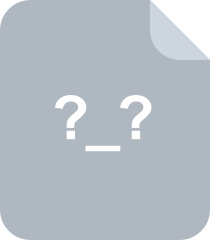
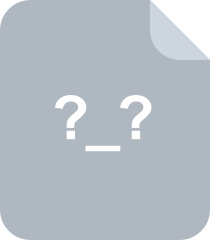
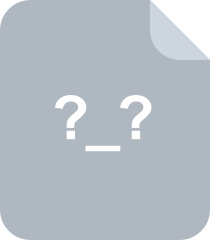
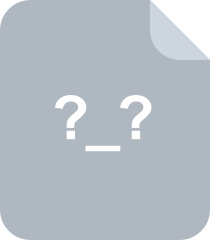
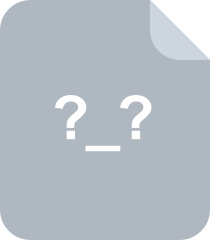
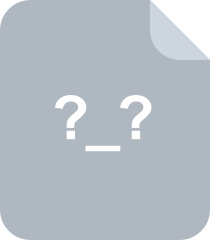
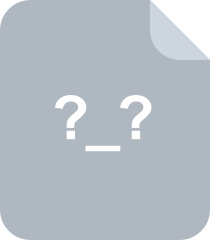
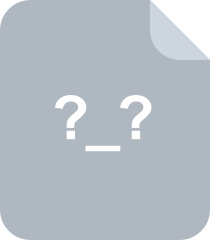
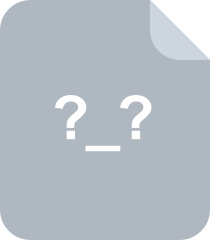
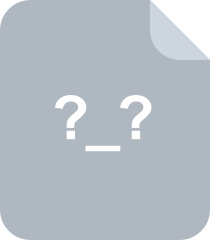
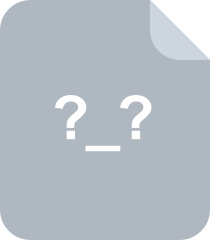
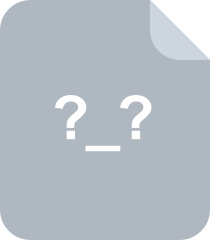
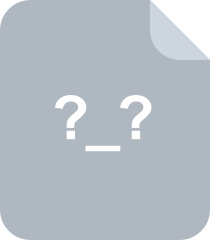
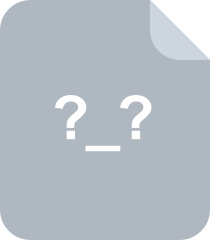
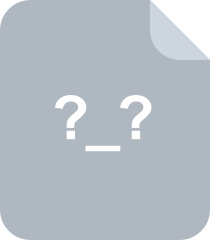
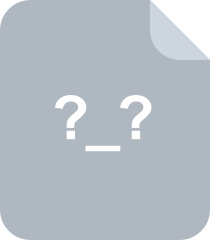
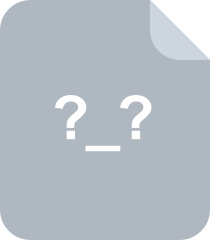
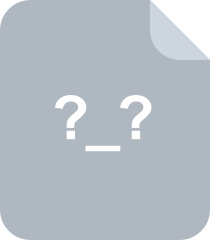
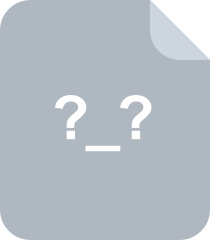
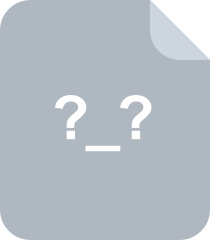
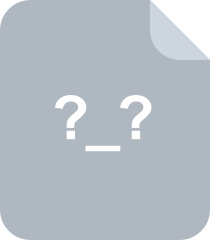
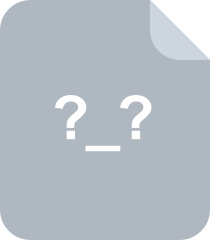
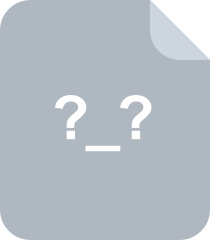
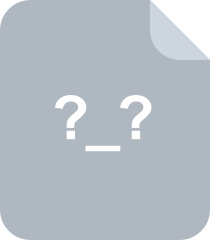
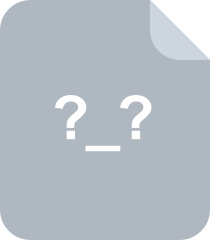
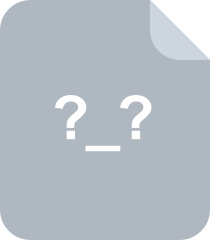
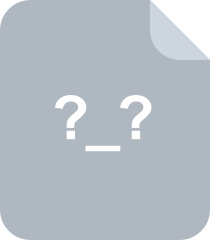
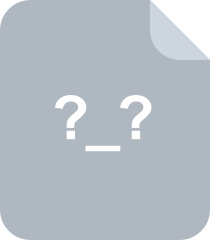
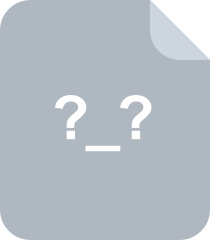
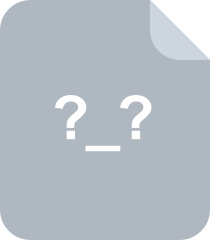
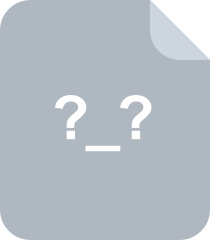
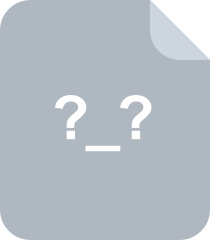
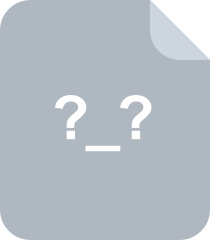
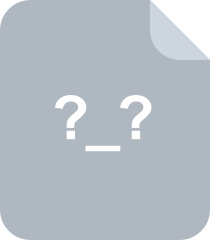
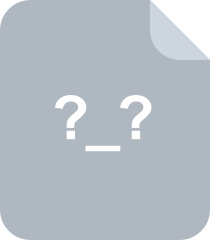
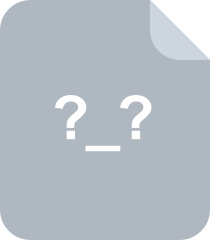
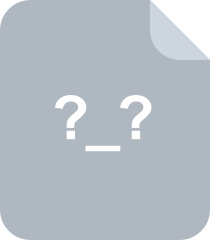
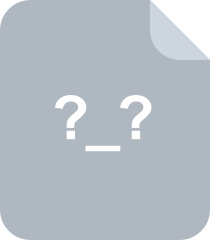
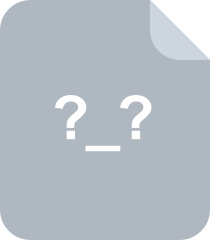
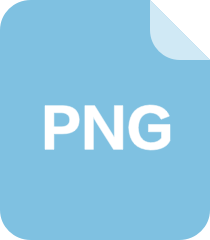
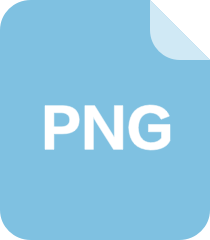
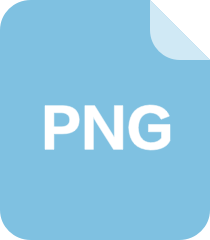
共 156 条
- 1
- 2
资源评论
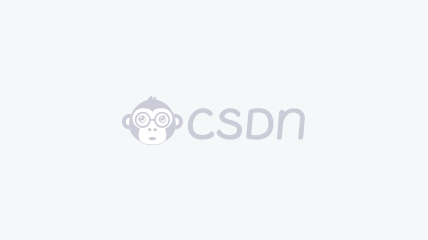

hello_中年人
- 粉丝: 7
- 资源: 324
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

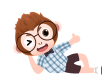
最新资源
- 基于WeChaty与多AI服务的微信机器人设计源码
- vic水文模型 VIC水文模型 全程视频教学指导,讲解详细,从基础内容处理讲解到模型参数率定全程教学 零基础可学 自用模型,从零到实践,历时两周左右
- PCB表面缺陷检测数据集 含有xml标签文件 可用于yolov3 yolov4 yolov5 yolov6 yolov7 yolov8目标检测
- 基于家庭陪伴式教育的0-12岁儿童游泳俱乐部网站设计源码
- 基于Java Web技术的电商购物系统设计与实现-涵盖前后端技术及安全高效的在线购物解决方案
- C#编写CIP通讯源码,欧姆龙NX1P通讯DEMO
- 基于Vue3、TypeScript、Vite、Pinia、Vue Router、Axios、Element Plus和Mock的现代化前端设计源码
- MATLAB代码:基于纳什谈判理论的风–光–氢多主体能源系统合作运行方法 关键词:合作博弈 纳什谈判 风–光–氢系统 综合能源 参考文档:《基于纳什谈判理论的风–光–氢多主体能源系统合作运行方法》
- apache-tomcat-9.0.88.7z
- nvm-setup.zip安装包
- 基于matlab的指纹图像预处理系统代码
- apache-maven-3.6.3-bin.rar
- comsol18650.21700锂电池热失控仿真,26650.温度和电压等结果
- 配网两阶段鲁棒优化调度模型 关键词:两阶段鲁棒优化,CCG算法,储能 仿真算例采用33节点,采用matlab+yalmip+cplex编写,两阶段模型采用CCG算法求解 模型中一阶段变量主要包括01
- node-modules 前端依赖比较完整的依赖 node需要14的
- 纯汽蒸汽发生器程pro序 组态系统 PID程序,液位控制,阀门控制,趋势图 硬件:1200触摸屏和西门子1500,源程序 适合过程控制学习,博图入门学习~
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


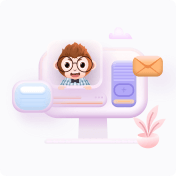
安全验证
文档复制为VIP权益,开通VIP直接复制
