import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.io.FileWriter;
/**
* 文件操作类
*
* @author 9995857
* @version v1.0
*/
public class FileIo {
/**
* 写文本文件内容
*
* @param filePathAndName
* 文本文件完整绝对路径及文件名
* @param fileContent
* 文本文件内容
* @param encoding
* 编码方式 例如 GBK 或者 UTF-8
* @return 文本文件完整绝对路径及文件名
*/
public static String writeText(String filePathAndName, String fileContent,
String encoding) {
if (encoding.equals(""))
encoding = "UTF-8";
filePathAndName = filePathAndName.replace(File.separator, "/");
PrintWriter myFile = null;
try {
File myFilePath = new File(filePathAndName);
if (!myFilePath.exists()) {
createFolders(filePathAndName.substring(0, filePathAndName
.lastIndexOf("/")));
myFilePath.createNewFile();
}
myFile = new PrintWriter(myFilePath, encoding);
myFile.print(fileContent);
myFile.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (myFile != null)
myFile.close();
}
return filePathAndName;
}
/**
* 追加写文本文件内容
*
* @param filePathAndName
* 文本文件完整绝对路径及文件名
* @param fileContent
* 文本文件内容
* @param encoding
* 编码方式 例如 GBK 或者 UTF-8 默认UTF-8
* @return 文本文件完整绝对路径及文件名
*/
public static String appendWriteText(String filePathAndName,
String fileContent, String encoding) {
if (encoding.equals(""))
encoding = "UTF-8";
filePathAndName = filePathAndName.replace(File.separator, "/");
FileWriter myFile = null;
try {
File myFilePath = new File(filePathAndName);
if (!myFilePath.exists()) {
return writeText(filePathAndName, fileContent, encoding);
}
myFile = new FileWriter(filePathAndName, true);
myFile.write(fileContent);
myFile.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (myFile != null)
myFile.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return filePathAndName;
}
/**
* 读取文本文件内容
*
* @param filePathAndName
* 带有完整绝对路径的文件名
* @param encoding
* 文本文件打开的编码方式 GBK UTF-8
* @return 返回文本文件的内容
*/
public static String readText(String filePathAndName, String encoding) {
if (encoding == null)
encoding = "UTF-8";
encoding = encoding.trim();
StringBuffer str = new StringBuffer("");
String st = "";
FileInputStream fs = null;
InputStreamReader isr = null;
try {
fs = new FileInputStream(filePathAndName);
isr = new InputStreamReader(fs, encoding);
BufferedReader br = new BufferedReader(isr);
try {
String data = "";
while ((data = br.readLine()) != null) {
str.append(data + " ");
}
} catch (Exception e) {
str.append(e.toString());
} finally {
if (fs != null)
fs.close();
if (isr != null)
isr.close();
}
st = str.toString();
} catch (IOException e) {
e.printStackTrace();
}
return st;
}
/**
* 写二进制文件
*
* @param inputStream
* 二进制流
* @param newpath
* 保存路径
* @param newfilename
* 保存的文件名
* @return 保存的文件名
*/
public static String writeFile(InputStream inputStream, String newpath,
String newfilename) {
FileOutputStream outStream = null;
try {
newpath = newpath.replace(File.separator, "/");
if (newpath.endsWith("/")) {
newpath = newpath.substring(0, newpath.length() - 1);
}
writeText(newpath + "/" + newfilename, "", "");
outStream = new FileOutputStream(newpath + "/" + newfilename);
byte[] buffer = new byte[1024 * 5];
int byteread = 0;
while ((byteread = inputStream.read(buffer)) != -1) {
outStream.write(buffer, 0, byteread);
outStream.flush();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (outStream != null)
outStream.close();
if (inputStream != null)
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return newfilename;
}
/**
* 写二进制文件
*
* @param byteA
* 二进数组
* @param newpath
* 保存路径
* @param newfilename
* 保存的文件名
* @return 保存的文件名
*/
public static String writeFile(byte[] byteA, String newpath,
String newfilename) {
FileOutputStream outStream = null;
try {
newpath = newpath.replace(File.separator, "/");
if (newpath.endsWith("/")) {
newpath = newpath.substring(0, newpath.length() - 1);
}
writeText(newpath + "/" + newfilename, "", "");
outStream = new FileOutputStream(newpath + "/" + newfilename);
outStream.write(byteA);
outStream.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (outStream != null)
outStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return newfilename;
}
/**
* 读取二进制文件
*
* @param filePathAndName
* 带有完整绝对路径的文件名
* @return 二进制流
*/
public static byte[] readFile(String filePathAndName) {
byte[] byteA = null;
try {
File file = new File(filePathAndName);
if (file.exists()) {
InputStream inputStream = new FileInputStream(file);
inputStream.read(byteA);
inputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
return byteA;
}
/**
* 多级目录创建
*
* @param 要创建的目录路径
* @return 创建好的目录路径
*/
public static String createFolders(String multiFolderPath) {
String cuttentFolder = "";
multiFolderPath = multiFolderPath.replace(File.separator, "/");
String[] multi = multiFolderPath.split("/");
if (multi.length == 0)
return "";
for (int i = 0; i < multi.length; i++) {
cuttentFolder += multi[i] + "/";
createFolder(cuttentFolder);
}
return cuttentFolder;
}
/**
* 新建目录
*
* @param folderPath
* 目录
* @return 返回目录创建后的路径
*/
private static String createFolder(String folderPath) {
File myFilePath = new File(folderPath);
if (!myFilePath.exists())
myFilePath.mkdir();
return folderPath;
}
/**
* 删除文件
*
* @param filePathAndName
* 文本文件完整绝对路径及文件名
* @return Boolean 成功删除返回true 遭遇异常返回false
*/
public static boolean deleteFile(String filePathAndName) {
boolean ret = false;
try {
File myDelFile = new File(filePathAndName);
if (myDelFile.isFile() && myDelFile.exists()) {
myDelFile.delete();
ret = true;
} else {
ret = false;
}
} catch (Exception e) {
ret = false;
}
return ret;
}
/**
* 删除指定文件夹下所有文件
*
* @param filePath
* 文件夹完整绝对路径
* @return 成功删除返回true 遭遇异常返回false
*/
public static boolean deleteAllFile(String filePath) {
boolean ret = false;
filePath = filePath.replace(File.separator, "/");
File file = new File(filePath);
if (!file.exists()) {
return ret;
}
if (!file.isDirectory()) {
return ret;
}
String[] tempList = file.list();
File temp = null;
for (int i = 0; i < tempList.length; i++) {
if (filePath.endsWith("/")) {
filePath = filePath.substring(0, filePath.length() - 1);
}
temp = new File(filePath + File.separator + tempList[i]);
if (temp.isFile()) {
temp.delete();
ret = true;
} els

lc9995857
- 粉丝: 5
- 资源: 22
最新资源
- 机械臂运动仿真与轨迹分析:基于机器人工具箱的MATLAB正逆运动学工作空间探索与示教应用,机械臂运动仿真与轨迹分析:基于MATLAB机器人工具箱的正逆运动学工作空间探索与示教实践,机械臂运动仿真,机器
- 三相VIENNA整流器仿真研究:T型整流器双闭环PI控制及中点电位平衡控制策略,SPWM调制与高效能表现,三相VIENNA整流器仿真研究:T型整流器双闭环PI控制及中点电位平衡控制策略,SPWM调制与
- win32汇编环境,对话框程序使用跟踪条控件示例二
- apollo自动驾驶10.0-感知-lidar-完整注释版
- 五个带隙基准电路展示:包含曲率补偿与高PSRR特性,基于0.18um工艺的基准源电路设计珍藏版,展示五个带隙基准电路:含曲率补偿与高PSRR的BGR,基于0.18um工艺,完整电路及仿真测试成果,可直
- 双馈风机虚拟惯性与下垂控制在系统一次调频中的MATLAB模型:频率二次跌落研究,“双馈风机虚拟惯性与下垂控制在一次调频中的MATLAB应用:转速回复引发频率二次跌落研究”,双馈风机(永磁同步风机)惯性
- 含UPFC电力系统的潮流计算程序:一键设置,轻松复现lunwen,只需调整UPFC安装与控制参数,含UPFC电力系统的潮流计算程序:快速复现Lunwen的实用工具,只需设置安装位置与控制参数,含UPF
- 30天开发操作系统 第 21 天 -保护操作系统
- 富水断层破碎带隧道工程中流固耦合作用下的突水突泥机理及注浆治理技术研究,流固耦合作用下富水断层破碎带隧道突水突泥机理及注浆治理技术实践,富水断层破碎带隧道突水突泥机理及注浆治理技术研究 隧道开挖卸荷
- Notepad_202502151235_47394.png
- go1.23.5.Windows-amd64安装包
- JimuFlow RPA工具Windows版v1.0.0
- 1-1.学生类定义.cpp
- SVG技术在100MW直驱风电场中的应用:五个链路,每链路等值20台2MW直驱风机,配以10Mvar SVG定电压控制,构建10kV电压等级风电系统,基于SVG技术的100MW直驱风电场等值分析:单
- pycharm安装教程和基本配置
- 一个用 c 语言编写的图书管理系统源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


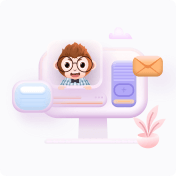