/*
* Generated by MyEclipse Struts
* Template path: templates/java/JavaClass.vtl
*/
package com.lb.struts.form;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts.action.ActionErrors;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionMapping;
/**
* MyEclipse Struts
* Creation date: 03-26-2009
*
* XDoclet definition:
* @struts.form name="insertForm"
*/
public class InsertForm extends ActionForm {
/*
* Generated fields
*/
/** linkman property */
private String linkman;
/** serverfax property */
private String serverfax;
/** remark property */
private String remark;
/** mainGive property */
private String mainGive;
/** fax property */
private String fax;
/** need property */
private String need;
/** outlay property */
private String outlay;
/** linktel property */
private String linktel;
/** serverLinkman property */
private String serverLinkman;
/** data property */
private String data;
/** serverTel property */
private String serverTel;
/** email property */
private String email;
/** serverDealer property */
private String serverDealer;
/** name property */
private String name;
/*
* Generated Methods
*/
/**
* Method validate
* @param mapping
* @param request
* @return ActionErrors
*/
public ActionErrors validate(ActionMapping mapping,
HttpServletRequest request) {
// TODO Auto-generated method stub
return null;
}
/**
* Method reset
* @param mapping
* @param request
*/
public void reset(ActionMapping mapping, HttpServletRequest request) {
// TODO Auto-generated method stub
}
/**
* Returns the linkman.
* @return String
*/
public String getLinkman() {
return linkman;
}
/**
* Set the linkman.
* @param linkman The linkman to set
*/
public void setLinkman(String linkman) {
this.linkman = linkman;
}
/**
* Returns the serverfax.
* @return String
*/
public String getServerfax() {
return serverfax;
}
/**
* Set the serverfax.
* @param serverfax The serverfax to set
*/
public void setServerfax(String serverfax) {
this.serverfax = serverfax;
}
/**
* Returns the remark.
* @return String
*/
public String getRemark() {
return remark;
}
/**
* Set the remark.
* @param remark The remark to set
*/
public void setRemark(String remark) {
this.remark = remark;
}
/**
* Returns the mainGive.
* @return String
*/
public String getMainGive() {
return mainGive;
}
/**
* Set the mainGive.
* @param mainGive The mainGive to set
*/
public void setMainGive(String mainGive) {
this.mainGive = mainGive;
}
/**
* Returns the fax.
* @return String
*/
public String getFax() {
return fax;
}
/**
* Set the fax.
* @param fax The fax to set
*/
public void setFax(String fax) {
this.fax = fax;
}
/**
* Returns the need.
* @return String
*/
public String getNeed() {
return need;
}
/**
* Set the need.
* @param need The need to set
*/
public void setNeed(String need) {
this.need = need;
}
/**
* Returns the outlay.
* @return String
*/
public String getOutlay() {
return outlay;
}
/**
* Set the outlay.
* @param outlay The outlay to set
*/
public void setOutlay(String outlay) {
this.outlay = outlay;
}
/**
* Returns the linktel.
* @return String
*/
public String getLinktel() {
return linktel;
}
/**
* Set the linktel.
* @param linktel The linktel to set
*/
public void setLinktel(String linktel) {
this.linktel = linktel;
}
/**
* Returns the serverLinkman.
* @return String
*/
public String getServerLinkman() {
return serverLinkman;
}
/**
* Set the serverLinkman.
* @param serverLinkman The serverLinkman to set
*/
public void setServerLinkman(String serverLinkman) {
this.serverLinkman = serverLinkman;
}
/**
* Returns the data.
* @return String
*/
public String getData() {
return data;
}
/**
* Set the data.
* @param data The data to set
*/
public void setData(String data) {
this.data = data;
}
/**
* Returns the serverTel.
* @return String
*/
public String getServerTel() {
return serverTel;
}
/**
* Set the serverTel.
* @param serverTel The serverTel to set
*/
public void setServerTel(String serverTel) {
this.serverTel = serverTel;
}
/**
* Returns the email.
* @return String
*/
public String getEmail() {
return email;
}
/**
* Set the email.
* @param email The email to set
*/
public void setEmail(String email) {
this.email = email;
}
/**
* Returns the serverDealer.
* @return String
*/
public String getServerDealer() {
return serverDealer;
}
/**
* Set the serverDealer.
* @param serverDealer The serverDealer to set
*/
public void setServerDealer(String serverDealer) {
this.serverDealer = serverDealer;
}
/**
* Returns the name.
* @return String
*/
public String getName() {
return name;
}
/**
* Set the name.
* @param name The name to set
*/
public void setName(String name) {
this.name = name;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
word+excel+jfreechart例子
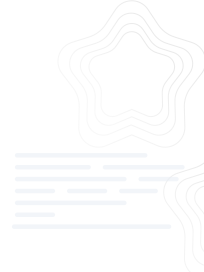
共110个文件
tld:23个
java:19个
class:19个


温馨提示
这个压缩包中包含了用java操作word,Excel,JFreeChart的三个例子,做的不是很完善,但是应该能体现一点内容的
资源推荐
资源详情
资源评论
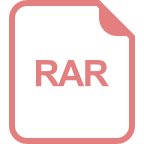
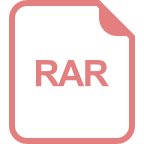
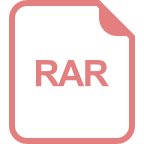
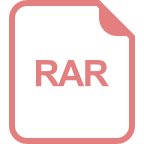
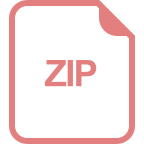
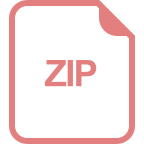
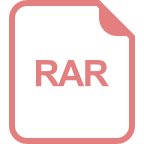
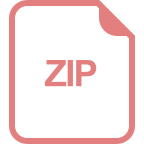
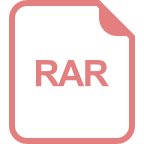
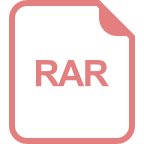
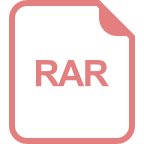
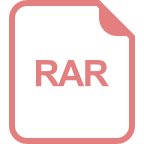
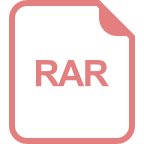
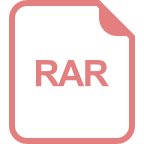
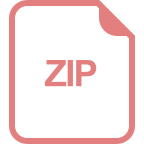
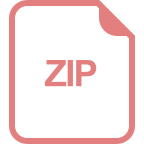
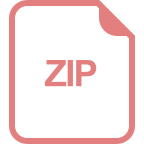
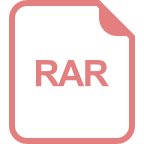
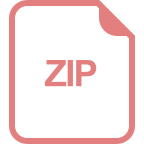
收起资源包目录

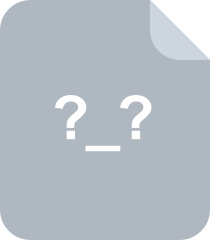
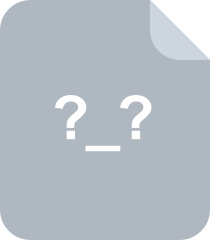
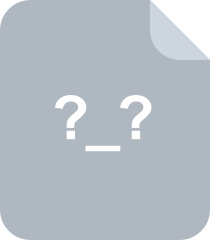
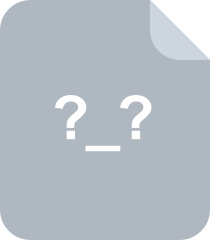
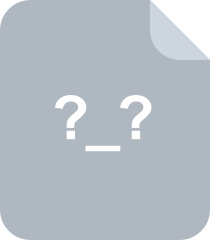
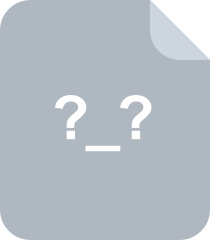
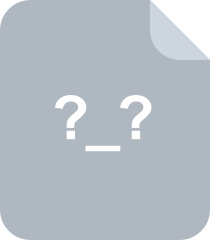
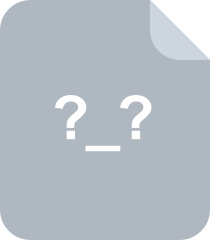
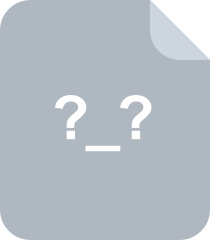
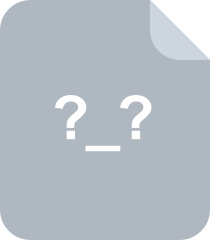
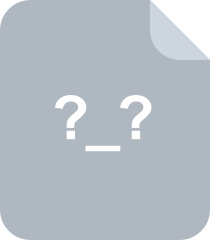
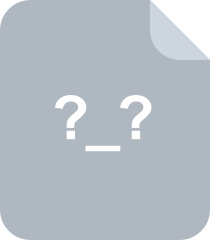
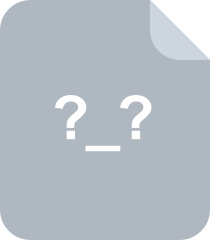
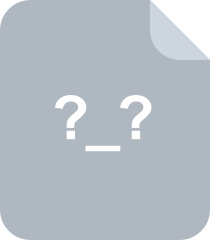
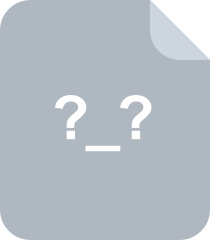
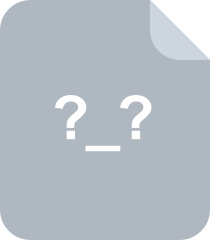
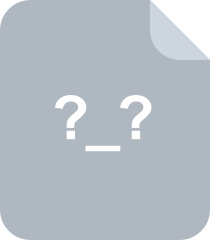
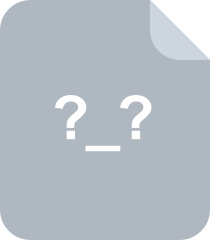
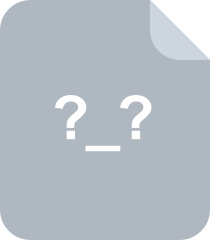
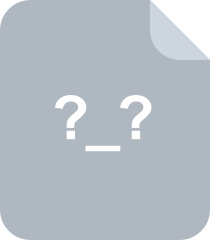
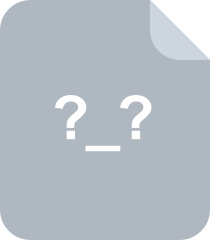
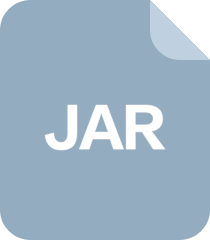
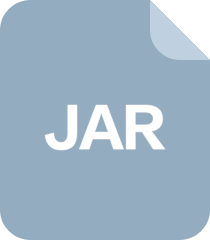
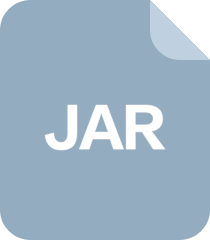
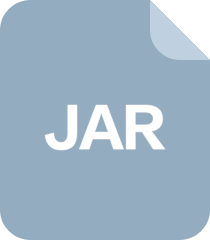
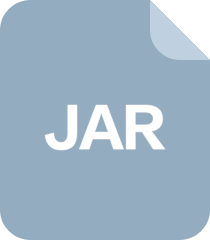
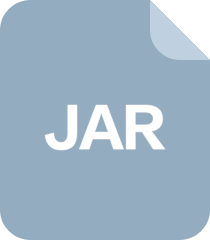
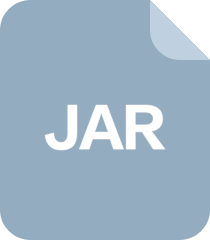
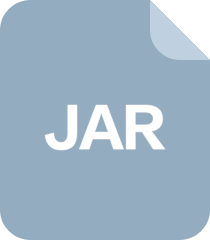
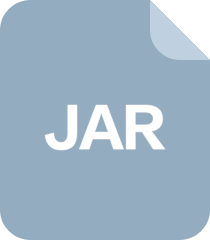
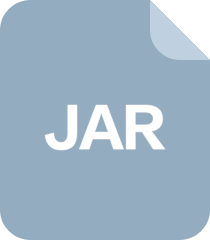
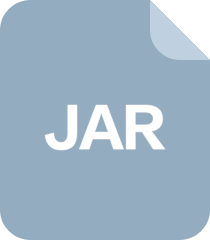
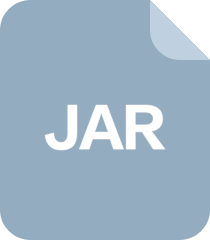
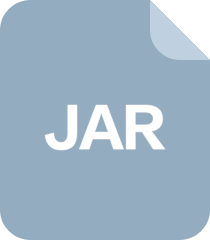
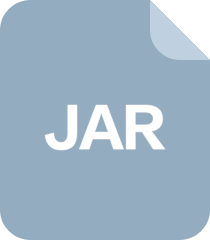
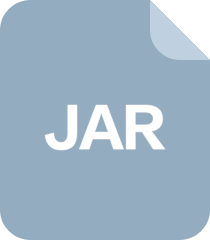
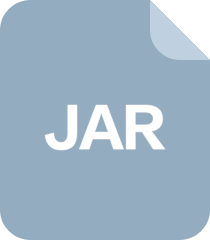
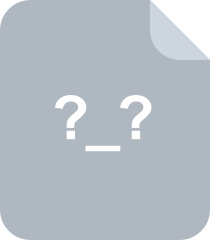
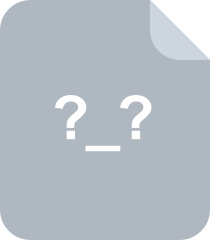
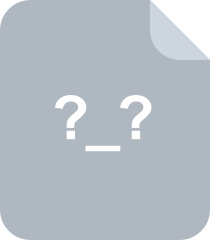
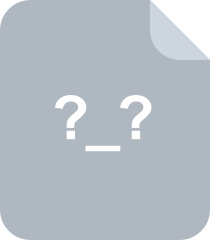
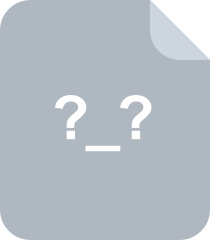
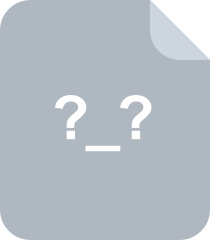
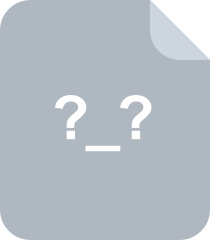
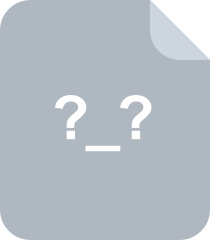
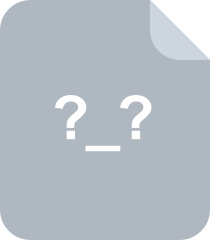
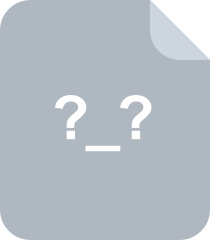
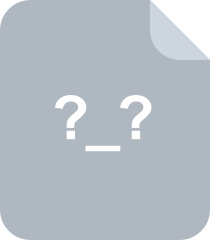
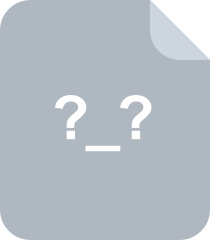
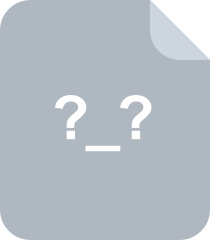
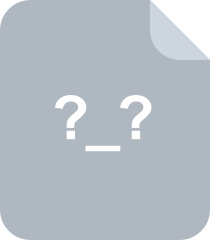
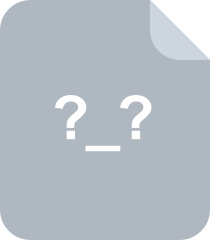
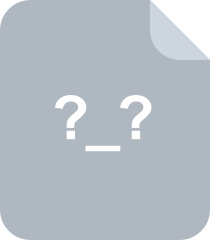
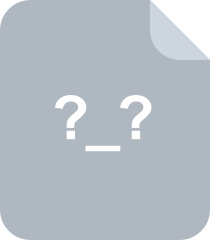
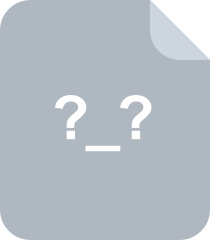
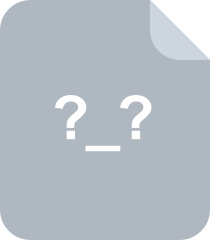
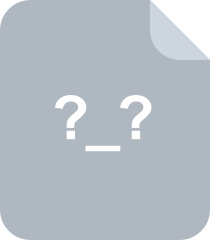
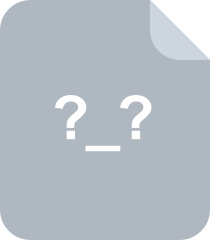
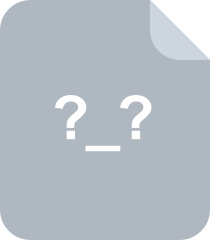
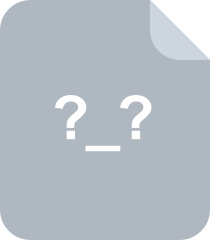
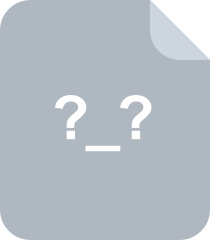
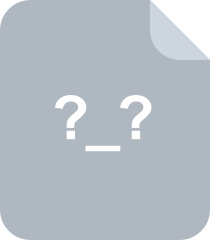
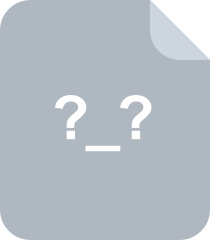
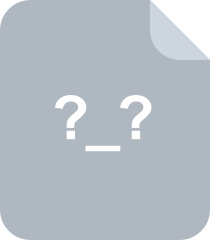
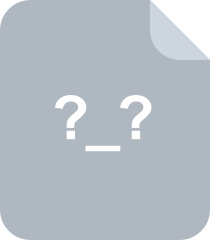
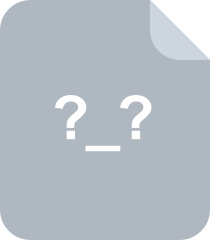
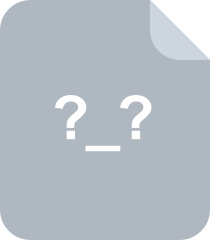
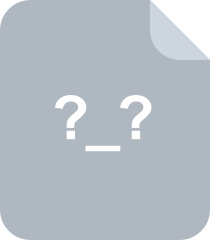
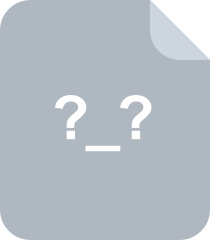
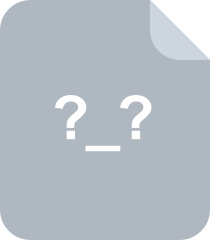
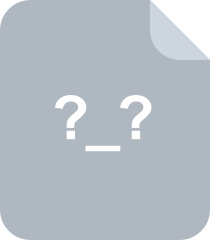
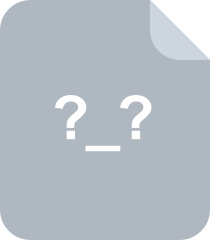
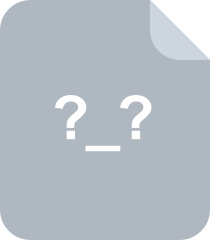
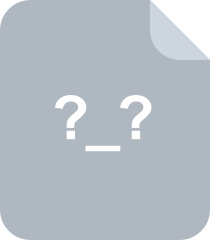
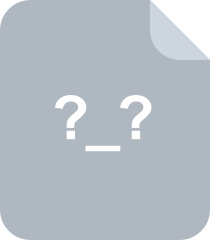
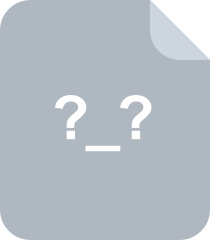
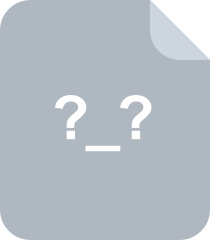
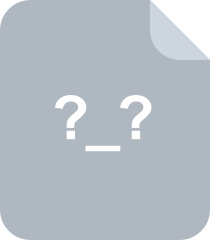
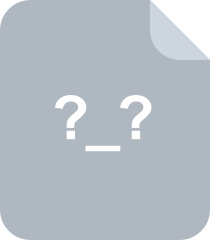
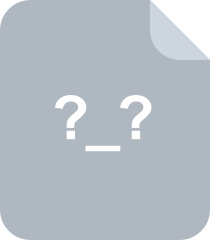
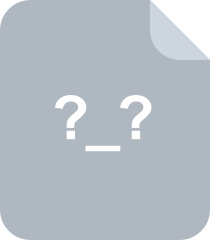
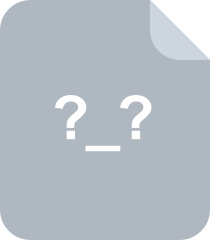
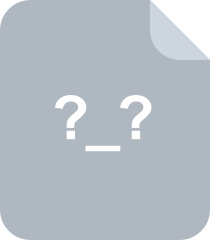
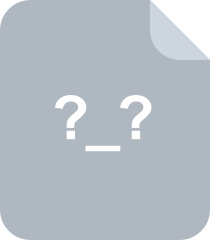
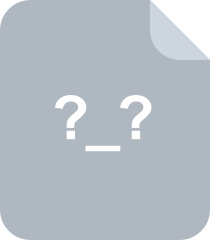
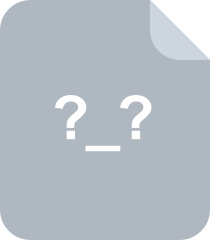
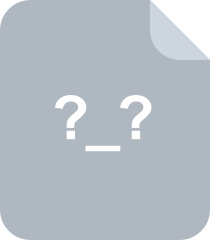
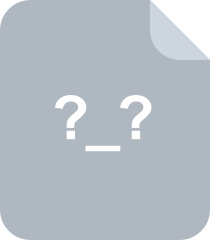
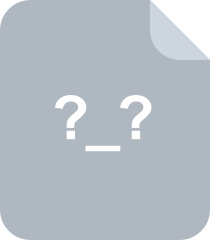
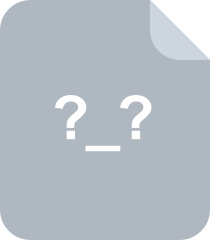
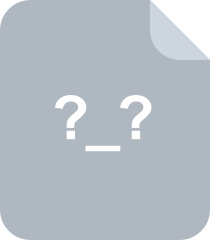
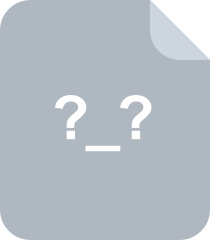
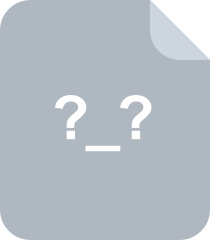
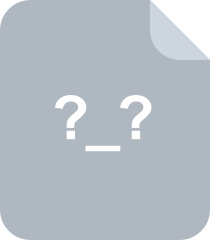
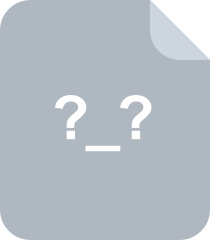
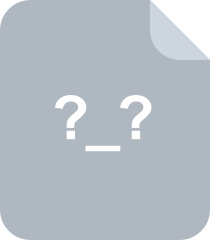
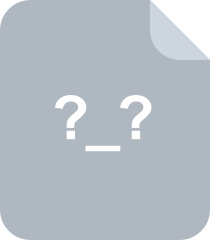
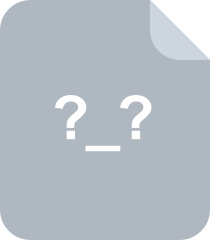
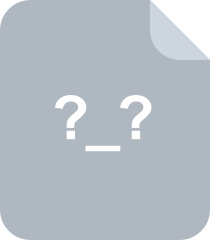
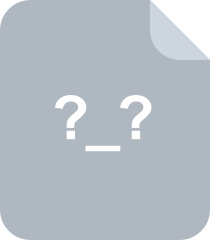
共 110 条
- 1
- 2
资源评论
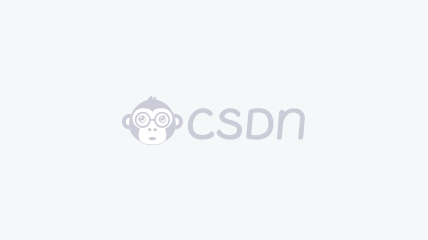
- yfx0082013-05-10还算不错,但是不太全
- pchelp20082015-03-11还算不错吧。分太高了

lbloveoop
- 粉丝: 18
- 资源: 14
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

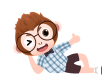
安全验证
文档复制为VIP权益,开通VIP直接复制
