#include "m_sched.h"
#include "Compare.h"
int m_sched::cur_run = -1;
m_sched::m_sched ( void )
{
}
void m_sched::run ( strategy algo ) {
event_style event = NOP;
list< PCBs* >::iterator proc;
list< PCBs* >::iterator wproc;
int run_t = 0;
switch ( algo ) {
case FCFS:
printf ( "Process\t\tArri_Time\tBurst_Time\tIO_Gap\tIO_Burst_Time\n" );
for ( list< PCBs* >::iterator itr = m_queue.begin(); itr != m_queue.end(); ++itr ) {
printf("%d\t\t%d\t\t%d\t\t%d\t\t%d\n", (*itr)->pid_t, (*itr)->arri,
(*itr)->burst, (*itr)->io_gap, (*itr)->io_burst );
}
while ( !( m_queue.empty() && w_queue.empty() ) ) {
if ( !w_queue.empty() ) {
for ( list< PCBs* >::iterator itr = w_queue.begin();
itr != w_queue.end(); ++itr ) {
if ( cur_run >= ( ( *itr )->arri + ( *itr )->io_burst ) ) {
( *itr )->io = false;
( *itr )->arri = ( ( *itr )->arri + ( *itr )->io_burst );
printf ( "\nPid %d finish IO operation at CPU time unit %d.\n", ( *itr )->pid_t, ( *itr )->arri );
m_queue.push_back ( *itr );//shift to ready queue
m_queue.sort ( Compare() );//sort by arrive time
w_queue.erase ( itr );//remove from waiting queue
break;
}
}
}
if ( m_queue.empty() ){
++ cur_run;
continue;
}
proc = m_queue.begin();//get the first one of ready queue
while ( cur_run < ( *proc )->arri ) {//waiting the process arrive
Sleep ( 1000 );
++ cur_run;
}
printf ( "\nEvent Process_Start at CPU time unit %d.\n", cur_run );
printf ( "Pid %d execute...", ( *proc )->pid_t );
stat.add_wait ( cur_run - ( ( *proc )->arri ) );//add up the waiting time
while ( run_t < ( *proc )->burst ) {//execute process
Sleep ( 1000 );
printf( "..." );
++ run_t;
++ cur_run;
if ( ( *proc )->io ) {//do io operation
if ( run_t == ( *proc )->io_gap ) {
printf ( "\nPid %d request for IO operation at CPU time unit %d.\n", ( *proc )->pid_t, cur_run );
( *proc )->arri = cur_run;//modify process arrival time
( *proc )->burst -= run_t;//remain burst time
( *proc )->sta = waiting;//ready to waiting
w_queue.push_back( *proc );//shift to waiting queue
printf ( "Have been run %d\n", run_t );
break;
}
}
}
if ( !( *proc )->io ) {
printf ( "\nRunning time is %d\n", run_t );
stat.add_turn ( ( *proc )->pid_t, cur_run );
}
m_queue.pop_front();//pop the the first one of ready queue
run_t = 0;//reset
}
stat.ave_wait ( MAX_PRO );
stat.ave_turn ( MAX_PRO );
break;
case RR:
printf ( "TimeSlice is %d\n", TimeSlice );
printf ( "Process\t\tArri_Time\tBurst_Time\tIO_Gap\tIO_Burst_Time\n" );
for ( list< PCBs* >::iterator itr = m_queue.begin(); itr != m_queue.end(); ++itr ) {
printf("%d\t\t%d\t\t%d\t\t%d\t\t%d\n", (*itr)->pid_t, (*itr)->arri,
(*itr)->burst, (*itr)->io_gap, (*itr)->io_burst );
}
while ( !( m_queue.empty() && w_queue.empty() ) ) {
if ( !w_queue.empty() ) {
for ( list< PCBs* >::iterator itr = w_queue.begin();
itr != w_queue.end(); ++itr ) {
if ( cur_run >= ( ( *itr )->arri + ( *itr )->io_burst ) ) {
( *itr )->io = false;
( *itr )->arri = ( ( *itr )->arri + ( *itr )->io_burst );//back to ready queue time
printf ( "\nPid %d finish IO operation at CPU time unit %d\n", ( *itr )->pid_t, ( *itr )->arri );
m_queue.push_back ( *itr );//shift to ready queue
m_queue.sort();
w_queue.erase ( itr );//remove from waiting queue
break;
}
}
}
if ( m_queue.empty() ){
++ cur_run;
continue;
}
proc = m_queue.begin();
while ( cur_run < ( *proc )->arri ) {//waiting the process arrive
Sleep ( 1000 );
++ cur_run;
}
printf ( "\nEvent Process_Start at CPU time unit %d.\n", cur_run );
printf ( "Pid %d execute...", ( *proc )->pid_t );
stat.add_wait ( cur_run - ( ( *proc )->arri ) );//add up the waiting time
while ( run_t < ( *proc )->burst && run_t < TimeSlice ) {//execute process
Sleep ( 1000 );
printf( "..." );
++ run_t;
++ cur_run;
if ( ( *proc )->io ) {//do io operation
if ( run_t == ( *proc )->io_gap ) {
printf ( "\nPid %d request for IO operation at CPU time unit %d.\n",( *proc )->pid_t , cur_run );
( *proc )->arri = cur_run;//modify process arrival time
( *proc )->burst -= run_t;//remain burst time
( *proc )->sta = waiting;//ready to waiting
w_queue.push_back( *proc );//shift to waiting queue
printf ( "Have been run %d\n", run_t );
break;
}
}
}
if ( run_t == TimeSlice && ( *proc )->burst != TimeSlice ) {
printf ( "\nSwap out Pid %d\n", ( *proc )->pid_t );
( *proc )->arri = cur_run;//modify process arrival time
( *proc )->sta = waiting;//ready to waiting
m_queue.push_back( *proc );//shift to the end of queue
}
if ( !( *proc )->io ) ( *proc )->burst -= run_t;//remain burst time
if ( !( *proc )->burst ) {
printf ( "\nRunning time is %d\n", run_t );
stat.add_turn ( ( *proc )->pid_t, cur_run );
}
m_queue.pop_front();//pop from ready queue
run_t = 0;//reset
}
stat.ave_wait ( MAX_PRO );
stat.ave_turn ( MAX_PRO );
break;
}
}
m_sched::~m_sched ( void ) {
}

lantygaga
- 粉丝: 0
- 资源: 6
最新资源
- 音乐网站(JSP+SERVLET).rar
- 抢购软件:快速复制信息
- oracle错误代码和信息速查手册chm版最新版本
- MATLAB【逆变器二次调频模型】 微电网分布式电源逆变器DROOP控制二次调频模型,加入二次控制实现二次调频控制,及二次调压控制,程序可实现上图功能,工况有所改变 需要matlab2021A版
- 基于python的网页自动化工具项目全套技术资料100%好用.zip
- Oracle数据库命令速查手册doc版最新版本
- 程序名称:转向设计计算程序 开发平台:基于matlab平台 计算内容:阿克曼转角,转弯半径,转向阻力矩,回正力矩,转向主参数,转向传动比,力矩波动,转向梯形,EPS匹配,HPS匹配,齿轮齿条传动比,循
- 基于二阶自抗扰ADRC的轨迹跟踪控制,对车辆的不确定性和外界干扰具有一定抗干扰性,基于carsim和simulink仿真 跟踪轨迹为双移线,效果良好,有对应复现资料,是学习自抗扰技术快速入门很好的资料
- TianleSoftwareOracle学习手册中文pdf格式最新版本
- MATLAB代码:基于分布式ADMM算法的考虑碳排放交易的电力系统优化调度研究 关键词:分布式调度 ADMM算法 交替方向乘子法 碳排放 最优潮流 仿真平台:MATLAB+CPLEX GUROBI
- Oracle安装配置使用WORD文档doc格式最新版本
- 西门子840D HMI ADVANCED FOR PC 也可用于810D,840DSL中文版 1、软件可安装到台式机或笔记本上,可以连接到机床的NCU进行NC与PLC的数据备份与恢复,备份和恢复的数
- OraclePLSQL简单安装指南WORD文档doc格式最新版本
- 网页数据采集软件项目全套技术资料100%好用.zip
- Oracle高级SQL培训与讲解WORD文档doc格式最新版本
- 超智能体写的人工智能深度学习pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


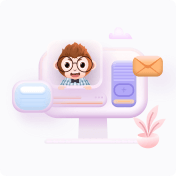