#include <reg52.h>
#include <intrins.h>
#include <string.h>
#define uchar unsigned char //宏代换,方便程序修改,提高运行效率
#define uint unsigned int //定义unit为无符号整型
bit bdata flag_key; //分配一个地址控制,存一个标志位的数据
#include "main.h"
#include "LCD12864.h"
#include "HX711.h"
#include "keyboard.h"
#include "eeprom52.h"
#include "FM24W256.h"
#include "uart1.h"
//#include "wannianli.h"
//#include "yyxp.h"
unsigned long HX711_Buffer = 0;
unsigned long Weight_Maopi = 0;
unsigned long Weight_Maopi_0 = 0;
int qupi=0;
unsigned char a=0;
long Weight_Shiwu = 0;
//键盘处理变量
unsigned char keycode;
unsigned char DotPos; //小数点标志及位置
uint GapValue,GapValue1;
unsigned long idata price; //单价,长整型值,单位为分
unsigned long idata danjia[8]={11,22,33,44,100,200,300,400};
unsigned char count_danjia;
unsigned long idata money,total_money; //总价,长整型值,单位为分
//定义标识
volatile bit FlagTest = 0; //定时测试标志,每0.5秒置位,测完清0
volatile bit FlagKeyPress = 0; //有键按下标志,处理完毕清0
//校准参数
//因为不同的传感器特性曲线不是很一致,因此,每一个传感器需要矫正这里这个参数才能使测量值很更准确。
//当发现测试出来的重量偏大时,增加该数值。
//如果测试出来的重量偏小时,减小改数值。
//该值可以为小数
//#define GapValue 349
sbit LED=P3^6;
sbit SC=P3^5;
sbit Buzzer = P3^7;
volatile bit ClearWeighFlag = 0; //传感器调零标志位,清除0漂
unsigned int xdata Record_Num=1;//用来储存已经储存了多少组的打卡数据 储存在地址299
unsigned char xdata Record_Data[16]={'D',':','0','0','.','0',' ','Z',':','0','0','0','0','.','0','\0'};
unsigned char xdata Record_tab[16]={' ',' ','D','J',' ',' ',' ',' ',' ',' ',' ','Z','J',' ',' ',' '};
/******************把数据保存到单片机内部eeprom中******************/
void write_eeprom()
{
SectorErase(0x2000);
GapValue1=GapValue&0x00ff;
byte_write(0x2000, GapValue1);
GapValue1=(GapValue&0xff00)>>8;
byte_write(0x2001, GapValue1);
byte_write(0x2060, a_a);
}
/******************把数据从单片机内部eeprom中读出来*****************/
void read_eeprom()
{
GapValue = byte_read(0x2001);
GapValue = (GapValue<<8)|byte_read(0x2000);
a_a = byte_read(0x2060);
}
/**************开机自检eeprom初始化*****************/
void init_eeprom()
{
read_eeprom(); //先读
if(a_a != 1) //新的单片机初始单片机内问eeprom
{
GapValue = 3500;
a_a = 1;
write_eeprom(); //保存数据
}
}
//显示单价,单位为元,四位整数,两位小数
void Display_Price()
{
LCD12864_write_com(0x88);
LCD12864_write_word("单价: ");
LCD12864_write_data(price/100 + 0x30);
LCD12864_write_data(price%100/10 + 0x30);
LCD12864_write_data('.');
LCD12864_write_data(price%10 + 0x30);
LCD12864_write_word("元");
}
//显示重量,单位kg,两位整数,三位小数
void Display_Weight()
{
LCD12864_write_com(0x90);
LCD12864_write_word("重量: ");
LCD12864_write_data(' ');
LCD12864_write_data(Weight_Shiwu/1000 + 0x30);
LCD12864_write_data('.');
LCD12864_write_data(Weight_Shiwu%1000/100 + 0x30);
LCD12864_write_data(Weight_Shiwu%100/10 + 0x30);
LCD12864_write_data(Weight_Shiwu%10 + 0x30);
LCD12864_write_word("Kg");
}
//显示总价,单位为元,四位整数,两位小数
void Display_Money()
{
// unsigned int i,j;
LCD12864_write_com(0x98); //指针设置
LCD12864_write_word("总价: ");
LCD12864_write_com(0x9f); //指针设置
LCD12864_write_word("元");
if (money>=1000)
{
LCD12864_write_com(0x9c);
LCD12864_write_data(' ');
LCD12864_write_data(money/1000 + 0x30);
LCD12864_write_data(money%1000/100 + 0x30);
LCD12864_write_data(money%100/10 + 0x30);
LCD12864_write_data('.');
LCD12864_write_data(money%10 + 0x30);
}
else if (money>=100)
{
LCD12864_write_com(0x9c);
LCD12864_write_data(' ');
LCD12864_write_data(0x20);
LCD12864_write_data(money%1000/100 + 0x30);
LCD12864_write_data(money%100/10 + 0x30);
LCD12864_write_data('.');
LCD12864_write_data(money%10 + 0x30);
}
else if(money>=10)
{
LCD12864_write_com(0x9c);
LCD12864_write_data(' ');
LCD12864_write_data(0x20);
LCD12864_write_data(0x20);
LCD12864_write_data(money%100/10 + 0x30);
LCD12864_write_data('.');
LCD12864_write_data(money%10+ 0x30);
}
else
{
LCD12864_write_com(0x9c);
LCD12864_write_data(' ');
LCD12864_write_data(0x20);
LCD12864_write_data(0x20);
LCD12864_write_data(0 + 0x30);
LCD12864_write_data('.');
LCD12864_write_data(money%10 + 0x30);
}
}
//数据初始化
void Data_Init()
{
price = 0;//////////////////////////////////
DotPos = 0;
}
//定时器0初始化
void Timer0_Init()
{
ET0 = 1; //允许定时器0中断
TMOD = 1; //定时器工作方式选择
TL0 = 0xb0;
TH0 = 0x3c; //定时器赋予初值
TR0 = 1; //启动定时器
}
//定时器0中断
void Timer0_ISR (void) interrupt 1 using 0
{
uchar Counter;
TL0 = 0xb0;
TH0 = 0x3c; //定时器赋予初值
//每0.5秒钟刷新重量
Counter ++;
if (Counter >= 10)
{
FlagTest = 1;
Counter = 0;
}
}
//按键响应程序,参数是键值
//返回键值:
// 7 8 9 10(清0)
// 4 5 6 11(删除)
// 1 2 3 12(未定义)
// 14(未定义) 0 15(.) 13(确定价格)
void KeyPress(uchar keycode)
{
switch (keycode)
{
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
case 6:
case 7:
case 8:
case 9: //目前在设置整数位,要注意price是整型,存储单位为分
if (DotPos == 0)
{ //最多只能设置到千位
if (price<100)
{
price=price*10+keycode*10;
}
}//目前在设置小数位
else if (DotPos==1) //小数点后第一位
{
price=price+keycode;
DotPos=2;
}
Display_Price();
break;
case 10: //清零键
// speak(41);
if(qupi==0)
qupi=Weight_Shiwu;
else
qupi=0;
Display_Price();
// FlagSetPrice = 0;
DotPos = 0;
break;
case 11: //删除键,按一次删除最右一个数字
price=0;
DotPos=0;
Display_Price();
break;
case 12: //加
if(GapValue<10000)
GapValue++;
// Get_Weight();
break;
case 13: //减
if(GapValue>1)
GapValue--;
// Get_Weight();
break;
case 14:
if(Record_Num<100)
{//////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////
Record_Data[2]= price/100 +0x30;
Record_Data[3]= price%100/10 +0x30;
Record_Data[4]='.';
Record_Data[5]= price%10+0x30;
Record_Data[9]=' ';
Record_Data[10]= money/1000 + 0x30;
Record_Data[11]= money%1000/100 + 0x30;
Record_Data[12]=money%100/10 + 0x30;
Record_Data[13]='.';
Record_Data[14]= money%10 + 0x30;
// Record_Data[2]= 0x36;
// Record_Data[3]= 0x35;
// Record_Data[4]='.';
// Record_Data[5]= 0x35;
// Record_Data[9]=' ';
// Record_Data[10]= 0x35;
// Record_Data[11]= 0x35;
// Record_Data[12]= 0x35;
// Record_Data[13]='.';
// Record_Data[14]= 0x35;
SectorErase(0x8000);
Delay_ms(100);
byte_write(0x8000,Record_Num);
Delay_ms(10);
Delay_ms(10);
// a=byte_read(0x8100);
if(Record_Num==1)
{SectorErase(0x8200);
}
Delay_ms(10);
FM24W_WrToROM(Record_Num*16+0x8200,Record_Data,16);//储存打卡信息
Delay_ms(100);
Record_Num++;
SectorErase(0x8000);
Delay_ms(100);
byte_write(0x8000,Record_Num);
Delay_ms(10);
}
break;
case 15: //小数点按下
DotPos = 1;
没有合适的资源?快使用搜索试试~ 我知道了~
基于51单片机的多功能电子秤设计.zip
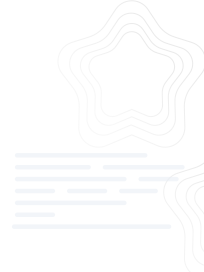
共45个文件
h:8个
obj:6个
lst:6个

需积分: 5 2 下载量 128 浏览量
2024-03-22
11:21:06
上传
评论 1
收藏 174KB ZIP 举报
温馨提示
上位机显示数据
资源推荐
资源详情
资源评论
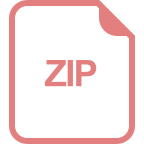
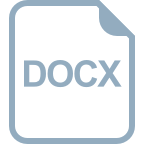
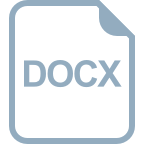
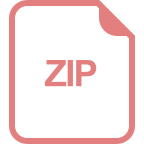
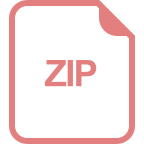
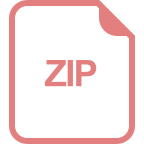
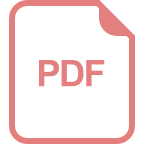
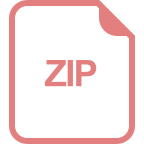
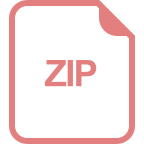
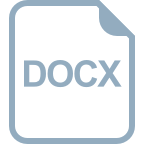
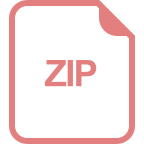
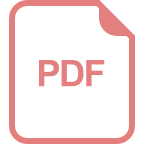
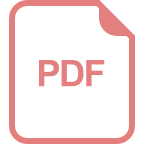
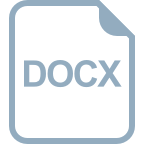
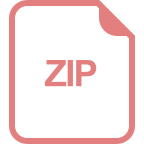
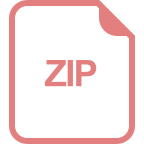
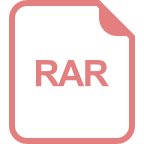
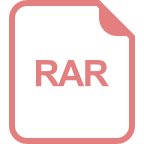
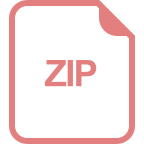
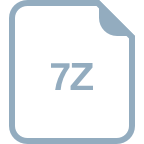
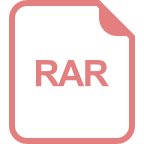
收起资源包目录


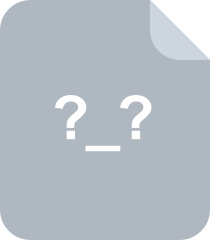
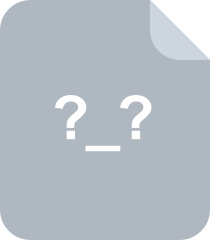
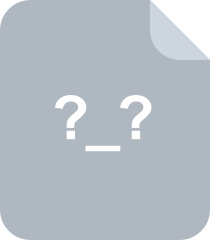
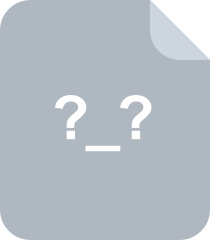
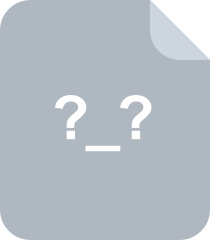
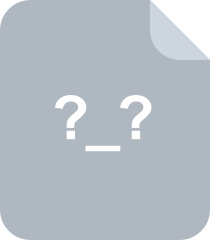
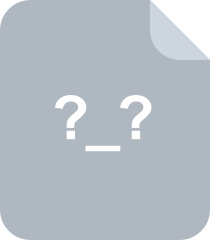
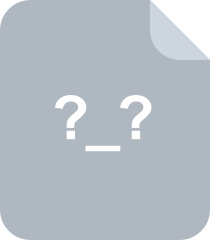
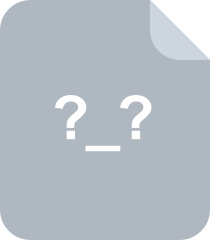
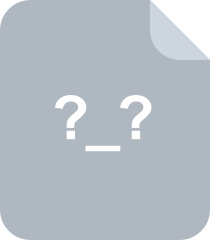
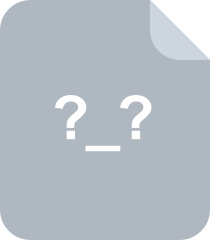
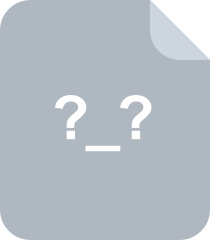
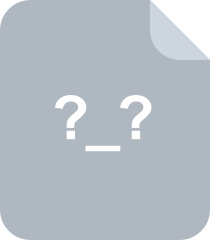
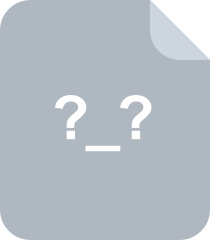
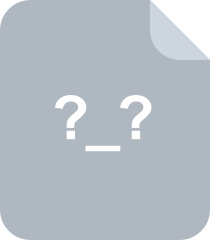
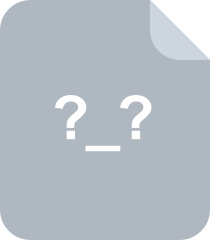
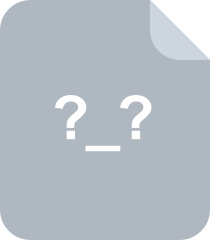
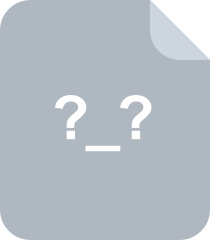
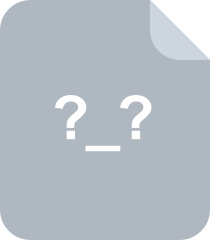
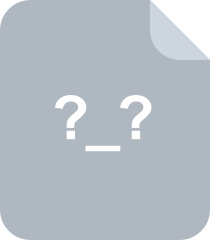
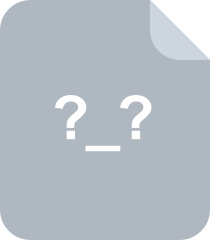
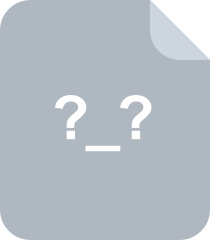
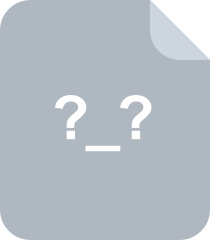
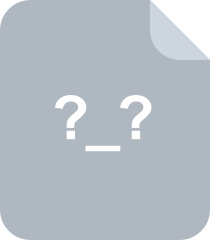
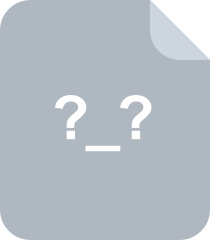
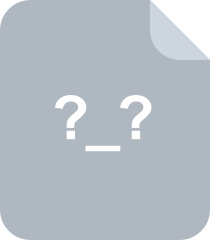
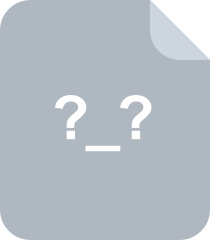
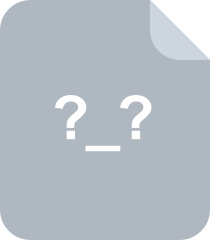
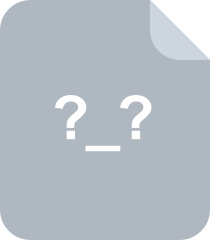
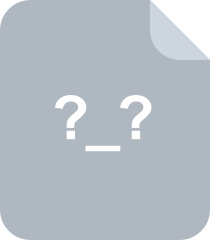
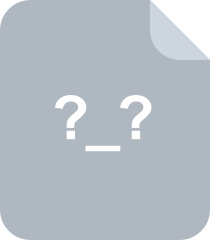
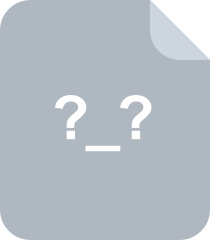
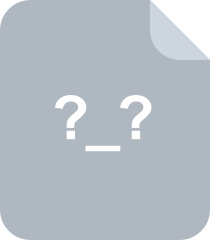
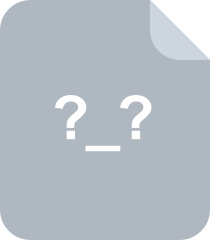
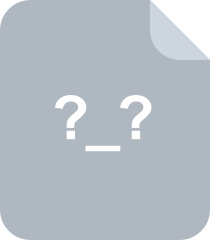
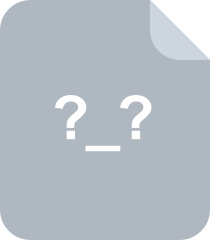
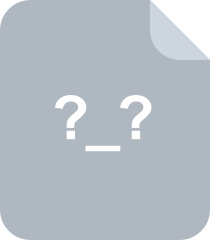
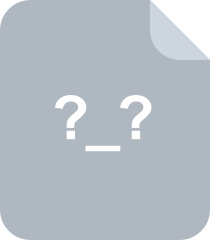
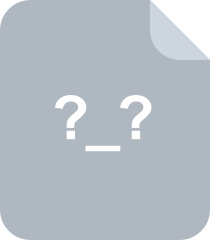
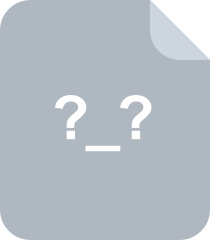
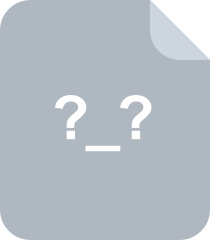
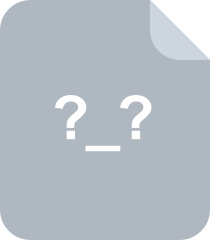
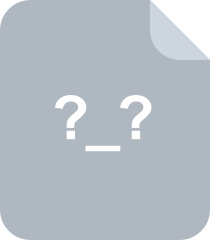
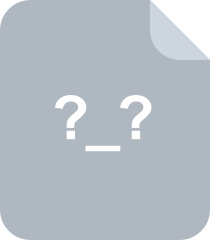
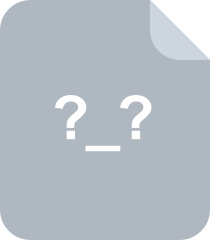
共 45 条
- 1
资源评论
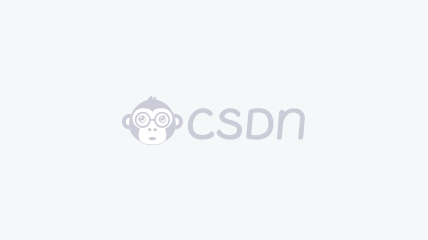

lantiandianzi
- 粉丝: 41
- 资源: 101
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

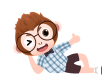
最新资源
- 创新MOM培训文档_物料主数据之包材_240625.pptx
- 医学图像分类数据集:CT胸部扫描癌症分类(4分类)【包括划分好的数据、类别字典文件、python数据可视化脚本 】
- 基于C51单片机设计四位数字频率计数码管显示实验Proteus仿真及软件实例源码.zip
- 基于C51单片机设计MAX7221数码管动态显示程序Proteus仿真及软件实例源码.zip
- DS18B20温度传感器实战应用与源码解析.zip
- python-leetcode面试题解之第384题打乱数组.zip
- python-leetcode面试题解之第383题赎金信.zip
- python-leetcode面试题解之第380题O1插入删除和获取随机元素.zip
- python-leetcode面试题解之第375题猜数字大小II.zip
- python-leetcode面试题解之第374题猜数字大小.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


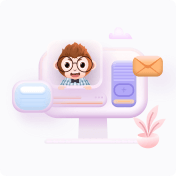
安全验证
文档复制为VIP权益,开通VIP直接复制
