package beecrypt.beeyond;
import java.io.*;
import java.security.*;
import java.security.cert.*;
import java.security.cert.Certificate;
import java.security.spec.*;
import java.util.*;
public class BeeCertificate extends Certificate {
public static final Date FOREVER = new Date(Long.MAX_VALUE);
protected static abstract class Field {
public int type;
public abstract void decode(DataInputStream in) throws IOException;
public abstract void encode(DataOutputStream out) throws IOException;
}
protected static class UnknownField extends Field implements Cloneable {
byte[] encoding;
public UnknownField() {
}
public UnknownField(int type, byte[] encoding) {
this.type = type;
this.encoding = encoding;
}
public Object clone() {
return new UnknownField(type, encoding);
}
public void decode(DataInputStream in) throws IOException {
encoding = new byte[in.available()];
in.readFully(encoding);
}
public void encode(DataOutputStream out) throws IOException {
out.write(encoding);
}
}
protected static class PublicKeyField extends Field implements Cloneable {
public static final int FIELD_TYPE = 0x5055424b;
public PublicKey pub;
public PublicKeyField() {
this.type = FIELD_TYPE;
}
public PublicKeyField(PublicKey pub) {
this.type = FIELD_TYPE;
this.pub = pub;
}
public Object clone() {
return new PublicKeyField(pub);
}
public void decode(DataInputStream in) throws IOException {
String algorithm = in.readUTF();
String format = in.readUTF();
int encsize = in.readInt();
if (encsize <= 0)
throw new IOException("Invalid key encoding size");
byte[] enc = new byte[encsize];
in.readFully(enc);
AnyEncodedKeySpec spec = new AnyEncodedKeySpec(format, enc);
try {
KeyFactory kf = KeyFactory.getInstance(algorithm);
pub = kf.generatePublic(spec);
} catch (InvalidKeySpecException e) {
throw new IOException("Invalid key spec");
} catch (NoSuchAlgorithmException e) {
throw new IOException("Invalid key format");
}
}
public void encode(DataOutputStream out) throws IOException {
out.writeUTF(pub.getAlgorithm());
out.writeUTF(pub.getFormat());
byte[] enc = pub.getEncoded();
if (enc == null)
throw new IOException("Couldn't encode key");
out.writeInt(enc.length);
out.write(enc);
}
}
protected static class ParentCertificateField extends Field {
public static final int FIELD_TYPE = 0x43455254;
public Certificate parent;
public ParentCertificateField() {
this.type = FIELD_TYPE;
}
public ParentCertificateField(Certificate parent) {
this.type = FIELD_TYPE;
this.parent = parent;
}
public Object clone() {
return new ParentCertificateField(parent);
}
public void decode(DataInputStream in) throws IOException {
String type = in.readUTF();
int encsize = in.readInt();
if (encsize <= 0)
throw new IOException("Invalid key encoding size");
byte[] enc = new byte[encsize];
in.readFully(enc);
ByteArrayInputStream bin = new ByteArrayInputStream(enc);
try {
CertificateFactory cf = CertificateFactory.getInstance(type);
parent = cf.generateCertificate(bin);
} catch (CertificateException e) {
throw new IOException("Invalid certificate encoding or type");
}
}
public void encode(DataOutputStream out) throws IOException {
out.writeUTF(parent.getType());
try {
byte[] enc = parent.getEncoded();
out.writeInt(enc.length);
out.write(enc);
} catch (CertificateEncodingException e) {
throw new IOException("Couldn't encoding certificate");
}
}
}
protected static Field instantiateField(int type) {
switch (type) {
case PublicKeyField.FIELD_TYPE:
return new PublicKeyField();
case ParentCertificateField.FIELD_TYPE:
return new ParentCertificateField();
default:
return new UnknownField();
}
}
private byte[] _enc;
protected String issuer = null;
protected String subject = null;
protected Date created = null;
protected Date expires = null;
protected ArrayList fields = new ArrayList();
protected String signatureAlgorithm = null;
protected byte[] signature = null;
protected void encodeTBS(DataOutputStream out) throws IOException {
out.writeUTF(issuer);
out.writeUTF(subject);
out.writeLong(created.getTime());
out.writeLong(expires.getTime());
out.writeInt(fields.size());
for (int i = 0; i < fields.size(); i++) {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
DataOutputStream dos = new DataOutputStream(bos);
Object obj = fields.get(i);
if (obj instanceof Field) {
Field f = (Field) obj;
f.encode(dos);
dos.close();
byte[] fenc = bos.toByteArray();
out.writeInt(f.type);
out.writeInt(fenc.length);
out.write(fenc);
}
}
}
protected byte[] encodeTBS() throws CertificateEncodingException {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
DataOutputStream dos = new DataOutputStream(bos);
try {
encodeTBS(dos);
dos.close();
} catch (IOException e) {
throw new CertificateEncodingException(e.getMessage());
}
return bos.toByteArray();
}
protected BeeCertificate() {
super("BEE");
}
public BeeCertificate(BeeCertificate copy) {
super("BEE");
issuer = copy.issuer;
subject = copy.subject;
created = new Date(copy.created.getTime());
expires = new Date(copy.expires.getTime());
fields.addAll(copy.fields);
signatureAlgorithm = copy.signatureAlgorithm;
if (copy.signature != null)
signature = (byte[]) copy.signature.clone();
}
public BeeCertificate(InputStream in) throws IOException {
super("BEE");
DataInputStream din = new DataInputStream(in);
issuer = din.readUTF();
subject = din.readUTF();
created = new Date(din.readLong());
expires = new Date(din.readLong());
int fieldCount = din.readInt();
if (fieldCount < 0)
throw new IOException("field count < 0");
fields.ensureCapacity(fieldCount);
for (int i = 0; i < fieldCount; i++) {
int type = din.readInt();
int fieldSize = din.readInt();
if (fieldSize < 0)
throw new IOException("field size < 0");
byte[] fenc = new byte[fieldSize];
din.readFully(fenc);
ByteArrayInputStream bis = new ByteArrayInputStream(fenc);
DataInputStream fis = new DataInputStream(bis);
Field f = instantiateField(type);
f.decode(fis);
fields.add(f);
}
signatureAlgorithm = din.readUTF();
int sigSize = din.readInt();
if (sigSize < 0)
throw new IOException("signature size < 0");
signature = new byte[sigSize];
din.readFully(signature);
}
public byte[] getEncoded() throws CertificateEncodingException {
if (_enc == null) {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
DataOutputStream dos = new DataOutputStream(bos);
try {
encodeTBS(dos);
dos.writeUTF(signatureAlgorithm);
dos.writeInt(signature.length);
dos.write(signature);
dos.close();
_enc = bos.toByteArray();
} catch (IOException e) {
throw new CertificateEncodingException(e.getMessage());
}
}
return _enc;
}
public PublicKey getPublicKey() {
for (int i = 0; i < fields.size(); i++) {
Object obj = fields.get(i);
if (obj instanceof PublicKeyField)
return ((PublicKeyField) obj).pub;
}
return null;
}
public Certificate getParentCertificate() {
for (int i = 0; i < fields.size(); i++) {
Object obj = fields.get(i);
if (obj instanceof ParentCertificateField)
return ((ParentCertificateField) obj).parent;
}
return null;
}
public void verify(PublicKey pub) throws CertificateException,
NoSuchAlgorithmException, InvalidKeyException, SignatureException {
Signature sig = Signature.getInstance(signatureAlgorithm);
sig.initVerify(pub);
sig.update(encodeTBS());
if (!sig.verify(signature))
throw new CertificateException("signature doesn't match");
}
public void verify(PublicKey pub, String algorithm)
throws CertificateException, No
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
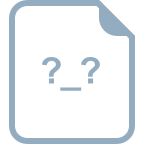
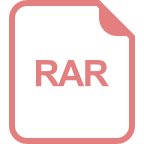
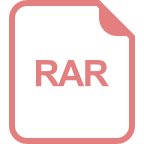
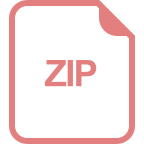
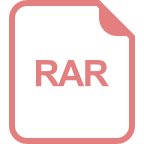
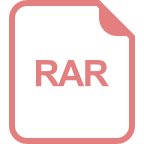
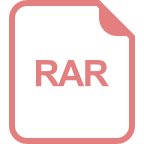
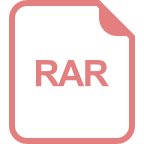
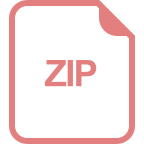
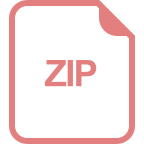
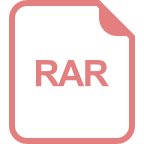
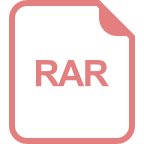
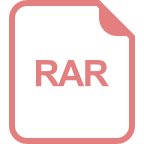
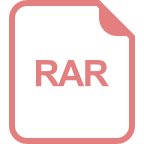
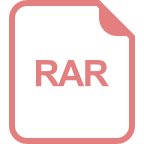
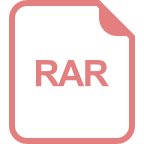
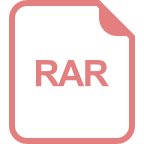
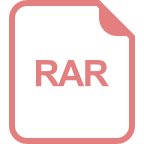
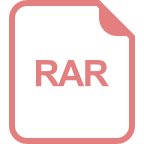
收起资源包目录

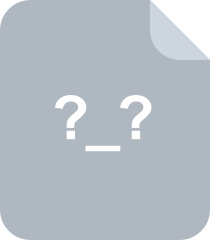
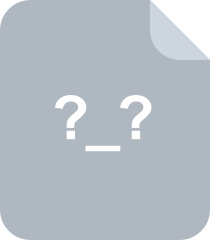
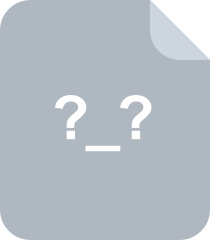
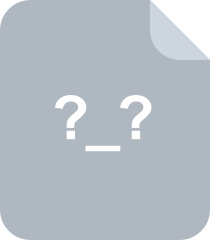
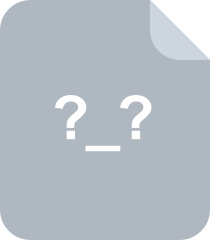
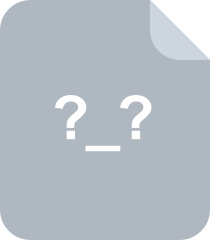
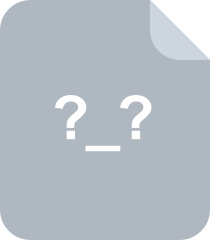
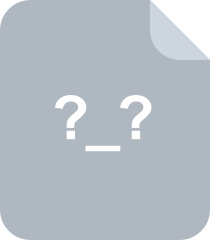
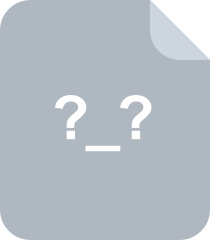
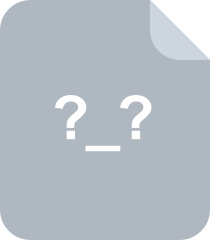
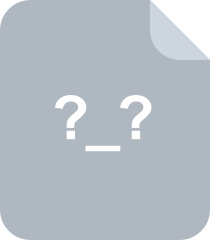
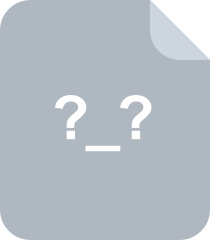
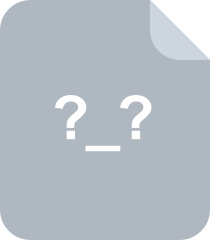
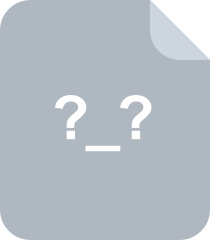
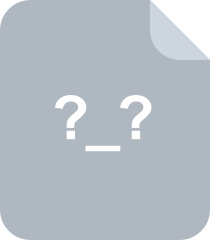
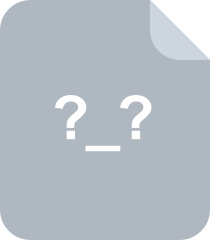
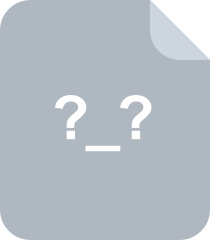
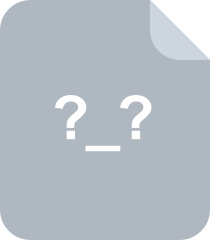
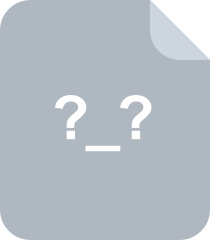
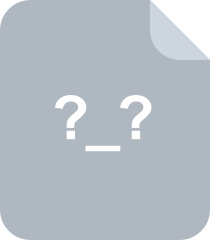
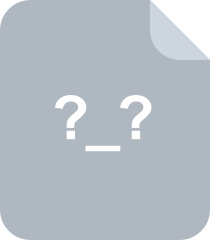
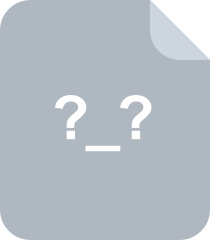
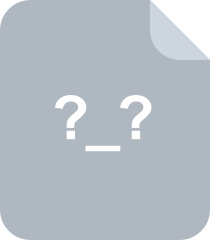
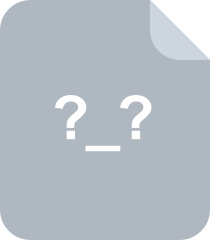
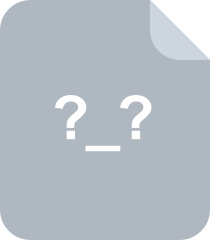
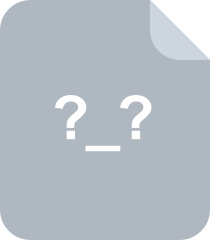
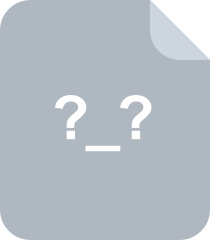
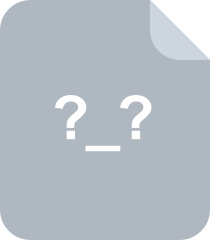
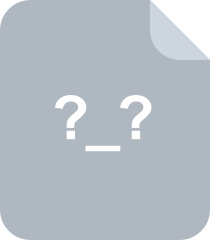
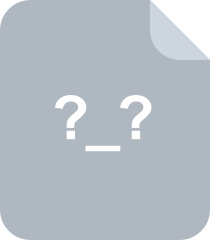
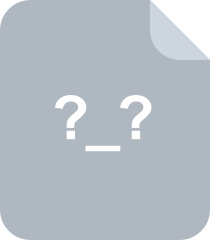
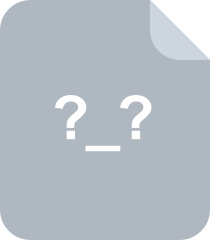
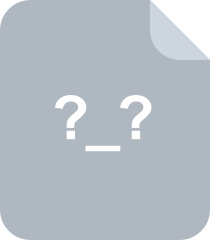
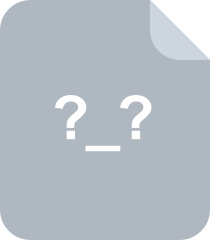
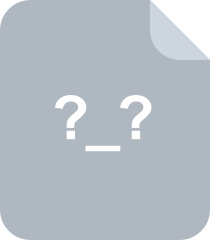
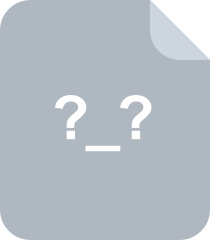
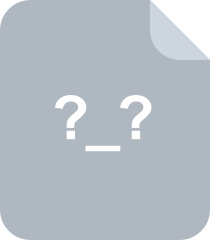
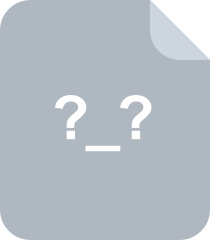
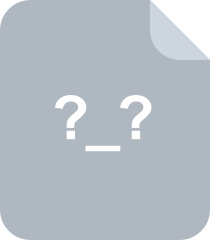
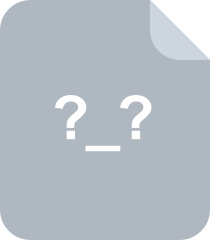
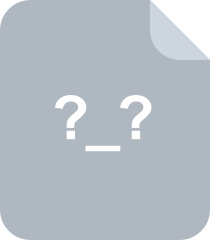
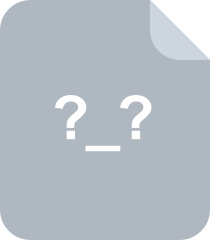
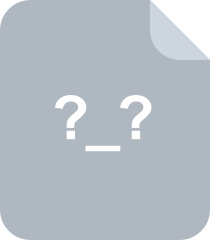
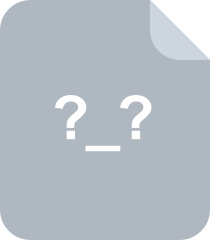
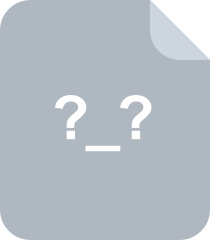
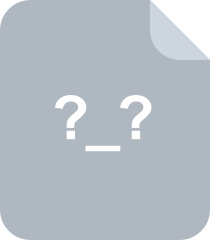
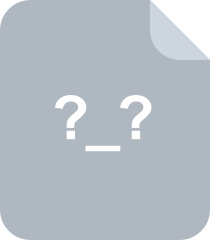
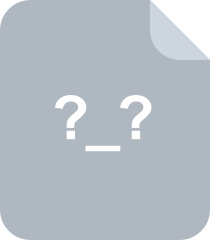
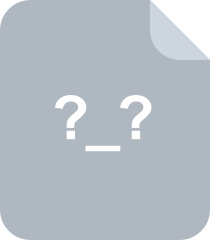
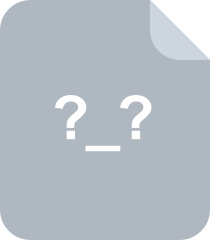
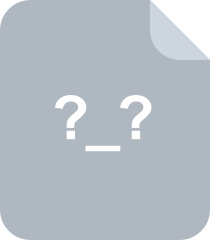
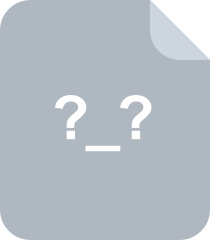
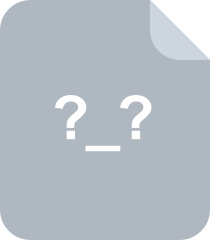
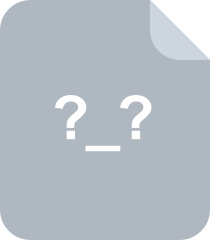
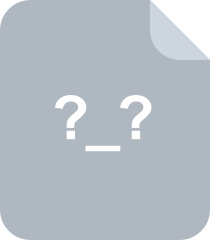
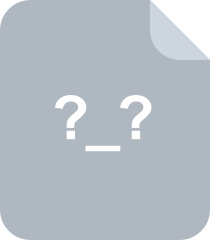
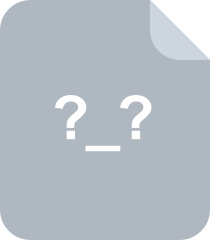
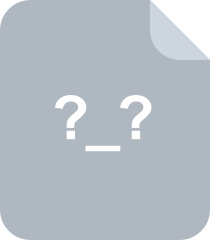
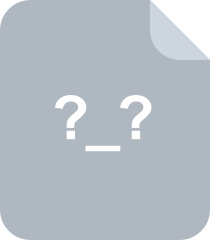
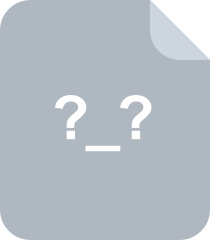
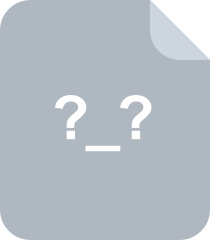
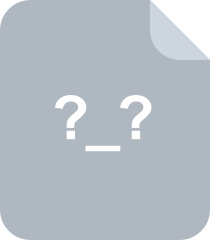
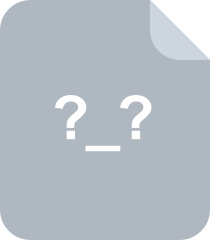
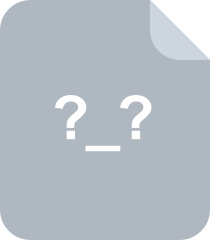
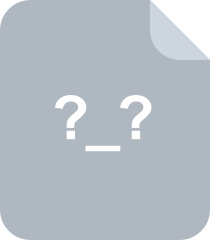
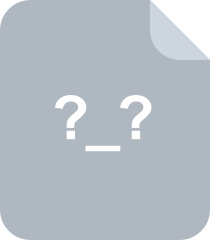
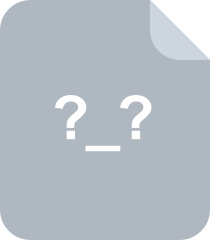
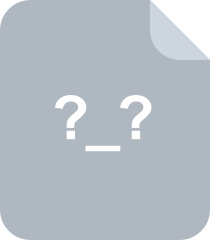
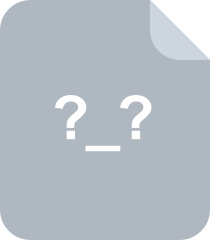
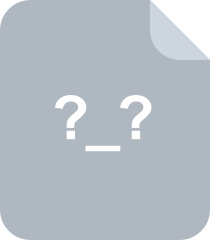
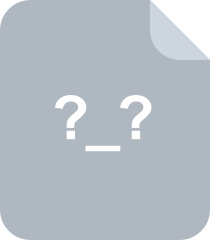
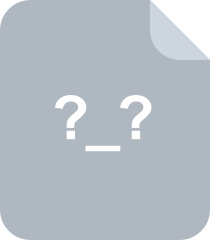
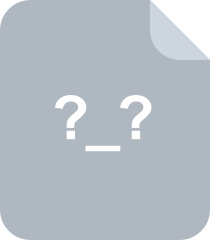
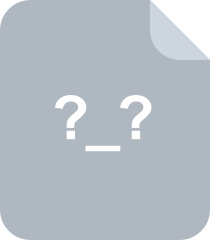
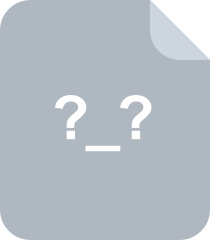
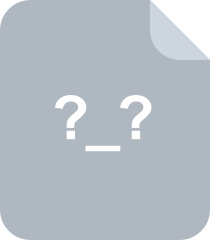
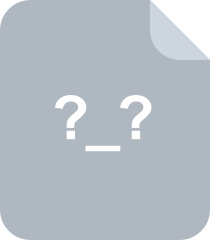
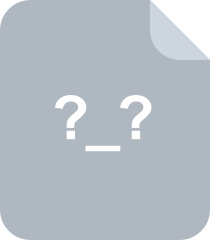
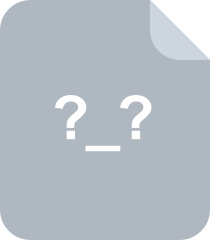
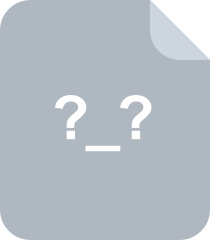
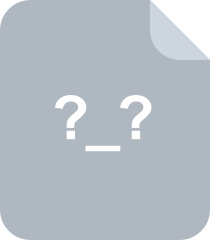
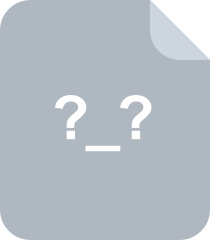
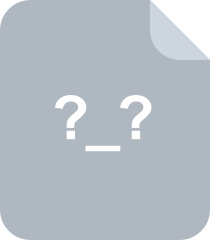
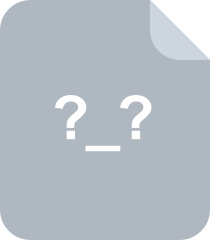
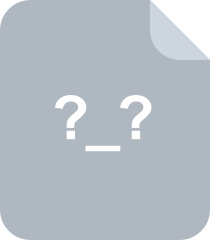
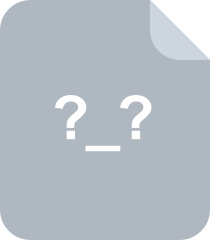
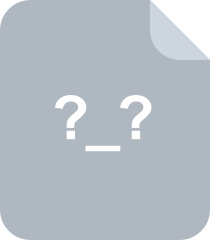
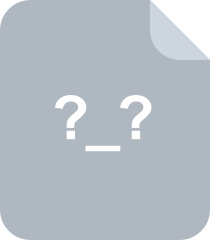
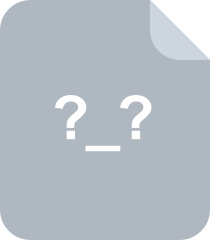
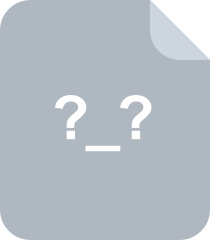
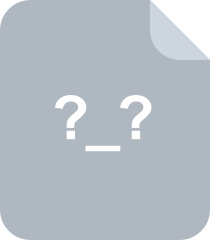
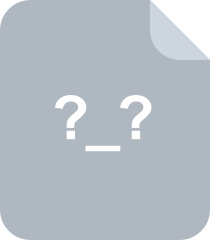
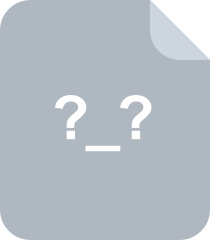
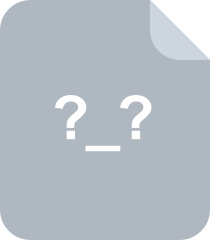
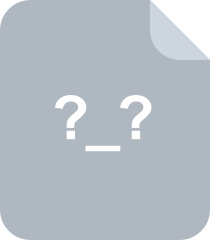
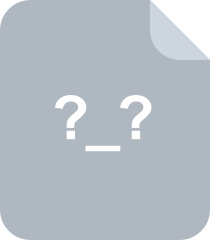
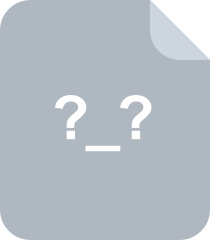
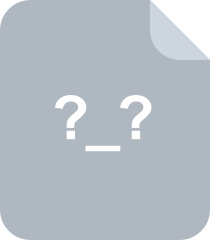
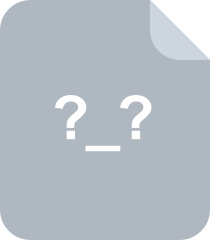
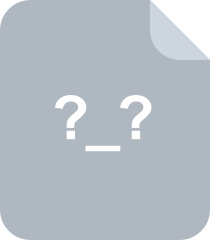
共 708 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
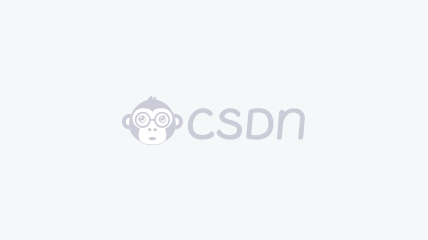
- ferrychen2015-02-09資源還不錯,可以下載!

langr007
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

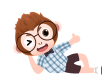
最新资源
- cocos creator 3.8 抖音侧边栏复访功能
- 【重磅,更新!】中国2839个站点逐日降水数据集(0.1°/0.25°/0.5°)(1961-2022年)
- RPC远程调用示例,zeroc入门例程
- 基于python实现的多智能体强化学习(MARL)算法复现,包括QMIX,VDN,QTRAN、MAVEN+源码(毕业设计&课程设计&项目开发)
- 【重磅,更新!】教学成果、一流学科申报书范本、最全教改、课程思政(内附清单)
- mptcp-样包参考协议开发
- 禁止WIN10自动更新
- svg动画示例效果展示
- ndra-dhcpv6样包参考
- 【重磅,更新!】中国31省份全要素生产率(1990-2022年)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


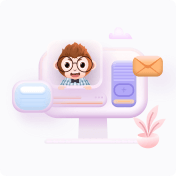
安全验证
文档复制为VIP权益,开通VIP直接复制
