/*
* WARNING: DO NOT EDIT THIS FILE. This is a generated file that is synchronized
* by MyEclipse Hibernate tool integration.
*
* Created Sun Feb 17 17:05:30 CST 2008 by MyEclipse Hibernate Tool.
*/
package com.xinda.dao;
import java.io.Serializable;
/**
* A class that represents a row in the order table.
* You can customize the behavior of this class by editing the class, {@link Order()}.
* WARNING: DO NOT EDIT THIS FILE. This is a generated file that is synchronized
* by MyEclipse Hibernate tool integration.
*/
public abstract class AbstractOrder
implements Serializable
{
/** The cached hash code value for this instance. Settting to 0 triggers re-calculation. */
private int hashValue = 0;
/** The composite primary key value. */
private java.lang.Integer orderno;
/** The value of the simple orderdate property. */
private java.util.Date orderdate;
/** The value of the simple customcode property. */
private java.lang.String customcode;
/** The value of the simple customname property. */
private java.lang.String customname;
/** The value of the simple contactname property. */
private java.lang.String contactname;
/** The value of the simple contactphone property. */
private java.lang.String contactphone;
/** The value of the simple saleman property. */
private java.lang.String saleman;
/** The value of the simple ordertype property. */
private java.lang.String ordertype;
/** The value of the simple orderbrief property. */
private java.lang.String orderbrief;
/** The value of the simple sampleyn property. */
private java.lang.String sampleyn;
/** The value of the simple deadlinedatetime property. */
private java.util.Date deadlinedatetime;
/** The value of the simple feeinstall property. */
private java.lang.Double feeinstall;
/** The value of the simple feeother property. */
private java.lang.Double feeother;
/** The value of the simple feereceivable property. */
private java.lang.Double feereceivable;
/** The value of the simple feeincome property. */
private java.lang.Double feeincome;
/** The value of the simple feeoffrate property. */
private java.lang.Float feeoffrate;
/** The value of the simple accounttype property. */
private java.lang.String accounttype;
/** The value of the simple incomeyn property. */
private java.lang.String incomeyn;
/** The value of the simple incomedate property. */
private java.util.Date incomedate;
/** The value of the simple invoiceyn property. */
private java.lang.String invoiceyn;
/** The value of the simple invoicenum property. */
private java.lang.String invoicenum;
/** The value of the simple deliverman property. */
private java.lang.String deliverman;
/** The value of the simple receiveman property. */
private java.lang.String receiveman;
/** The value of the simple receivedatetime property. */
private java.util.Date receivedatetime;
/** The value of the simple notes property. */
private java.lang.String notes;
/** The value of the simple isdelete property. */
private java.lang.Integer isdelete;
/**
* Simple constructor of AbstractOrder instances.
*/
public AbstractOrder()
{
}
/**
* Constructor of AbstractOrder instances given a simple primary key.
* @param orderno
*/
public AbstractOrder(java.lang.Integer orderno)
{
this.setOrderno(orderno);
}
/**
* Return the simple primary key value that identifies this object.
* @return java.lang.Integer
*/
public java.lang.Integer getOrderno()
{
return orderno;
}
/**
* Set the simple primary key value that identifies this object.
* @param orderno
*/
public void setOrderno(java.lang.Integer orderno)
{
this.hashValue = 0;
this.orderno = orderno;
}
/**
* Return the value of the orderDate column.
* @return java.util.Date
*/
public java.util.Date getOrderdate()
{
return this.orderdate;
}
/**
* Set the value of the orderDate column.
* @param orderdate
*/
public void setOrderdate(java.util.Date orderdate)
{
this.orderdate = orderdate;
}
/**
* Return the value of the customCode column.
* @return java.lang.String
*/
public java.lang.String getCustomcode()
{
return this.customcode;
}
/**
* Set the value of the customCode column.
* @param customcode
*/
public void setCustomcode(java.lang.String customcode)
{
this.customcode = customcode;
}
/**
* Return the value of the customName column.
* @return java.lang.String
*/
public java.lang.String getCustomname()
{
return this.customname;
}
/**
* Set the value of the customName column.
* @param customname
*/
public void setCustomname(java.lang.String customname)
{
this.customname = customname;
}
/**
* Return the value of the contactName column.
* @return java.lang.String
*/
public java.lang.String getContactname()
{
return this.contactname;
}
/**
* Set the value of the contactName column.
* @param contactname
*/
public void setContactname(java.lang.String contactname)
{
this.contactname = contactname;
}
/**
* Return the value of the contactPhone column.
* @return java.lang.String
*/
public java.lang.String getContactphone()
{
return this.contactphone;
}
/**
* Set the value of the contactPhone column.
* @param contactphone
*/
public void setContactphone(java.lang.String contactphone)
{
this.contactphone = contactphone;
}
/**
* Return the value of the saleMan column.
* @return java.lang.String
*/
public java.lang.String getSaleman()
{
return this.saleman;
}
/**
* Set the value of the saleMan column.
* @param saleman
*/
public void setSaleman(java.lang.String saleman)
{
this.saleman = saleman;
}
/**
* Return the value of the orderType column.
* @return java.lang.String
*/
public java.lang.String getOrdertype()
{
return this.ordertype;
}
/**
* Set the value of the orderType column.
* @param ordertype
*/
public void setOrdertype(java.lang.String ordertype)
{
this.ordertype = ordertype;
}
/**
* Return the value of the orderBrief column.
* @return java.lang.String
*/
public java.lang.String getOrderbrief()
{
return this.orderbrief;
}
/**
* Set the value of the orderBrief column.
* @param orderbrief
*/
public void setOrderbrief(java.lang.String orderbrief)
{
this.orderbrief = orderbrief;
}
/**
* Return the value of the sampleYN column.
* @return java.lang.String
*/
public java.lang.String getSampleyn()
{
return this.sampleyn;
}
/**
* Set the value of the sampleYN column.
* @param sampleyn
*/
public void setSampleyn(java.lang.String sampleyn)
{
this.sampleyn = sampleyn;
}
/**
* Return the value of the deadlineDateTime column.
* @return java.util.Date
*/
public java.util.Date getDeadlinedatetime()
{
return this.deadlinedatetime;
}
/**
* Set the value of the deadlineDateTime column.
Struts和Hibernate是Java Web开发中的两个重要框架,它们在构建高效、可维护的Web应用程序时发挥着关键作用。这个“Struts+Hibernate项目”旨在为开发者提供一个学习和实践的平台,帮助他们快速理解这两种框架的集成使用,并应用于实际项目开发。 Struts是一个基于MVC(Model-View-Controller)设计模式的开源框架,主要用于控制应用的流程和处理HTTP请求。它通过Action类来处理业务逻辑,与视图层进行交互,使得开发者能够更清晰地分离表现层和业务逻辑层。Struts的核心组件包括ActionForm、Action、ActionServlet以及配置文件struts-config.xml,这些都为创建可扩展和模块化的应用程序提供了便利。 Hibernate则是一个对象关系映射(ORM)框架,它简化了数据库操作,将Java对象和数据库表之间的映射关系自动化处理。开发者可以通过定义实体类和映射文件(hibernate.cfg.xml和.hbm.xml)来管理数据,无需编写大量的SQL语句。Hibernate支持事务处理、缓存机制和复杂的查询,提高了开发效率和数据一致性。 在这个“Struts+Hibernate项目”中,用户登录功能是基础,这通常涉及到用户验证和权限控制。开发者可能使用Struts的ActionForm收集用户输入,然后通过Hibernate查询数据库进行身份验证。数据的显示可能涉及到多个页面,如用户信息展示、数据列表等,这需要Struts的转发和包含机制来组织视图。 增删改查(CRUD)操作是任何数据库驱动的应用程序都会遇到的基本功能。在Struts和Hibernate的结合下,开发者可以轻松实现这些操作。例如,创建新记录时,可以通过Hibernate的Session接口保存实体对象;更新记录则对应于Session的update方法;删除操作使用Session的delete方法;查询通常借助Criteria、HQL或SQL语句,通过SessionFactory的getCurrentSession()获取的Session执行。 项目的具体实现细节,例如业务逻辑处理、数据访问对象(DAO)的设计、异常处理以及安全性考虑,都需要参考项目中的源代码。文件“xinda”可能是该项目的主入口或者某种配置文件,具体内容需解压后查看。通过研究这个项目,开发者不仅可以学习到如何整合Struts和Hibernate,还能了解到如何在MyEclipse环境中进行开发,这对提升Java Web开发技能大有裨益。








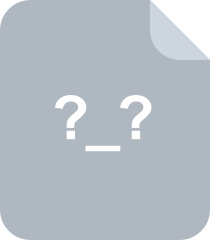
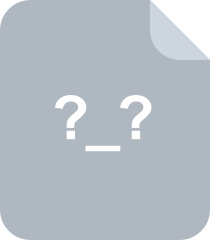
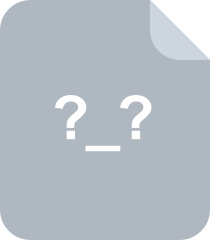
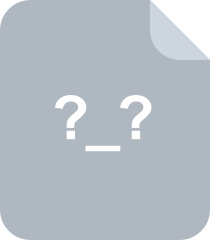
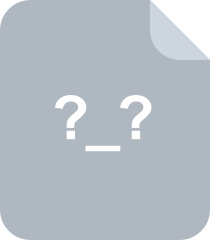
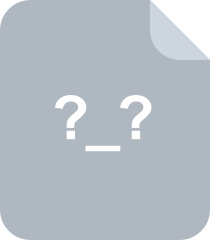
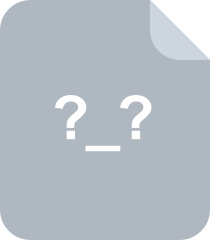
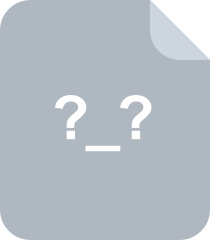
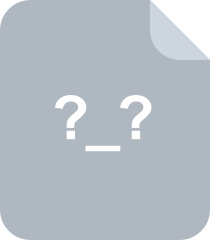
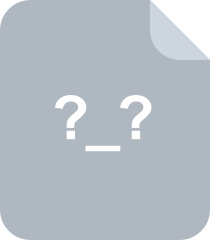
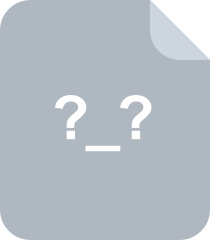
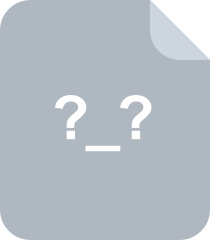
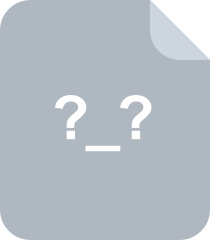
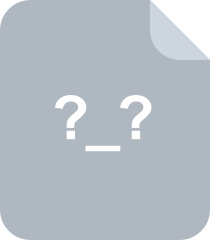
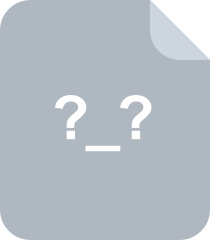
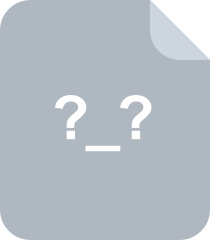
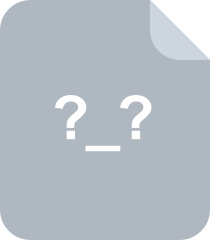
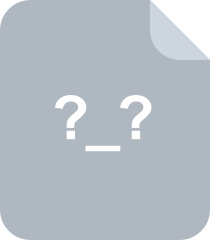
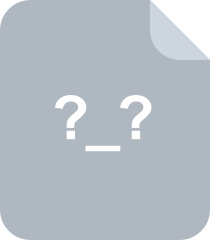
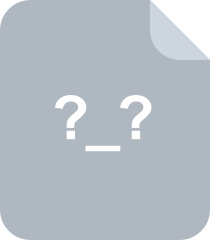
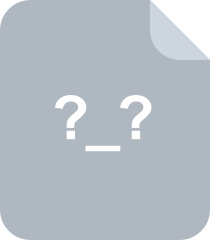
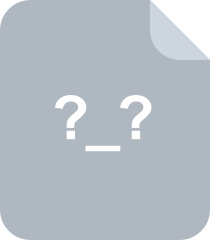
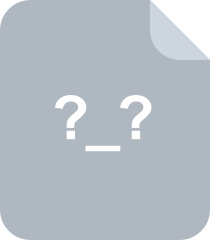
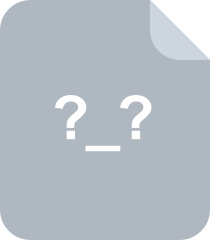
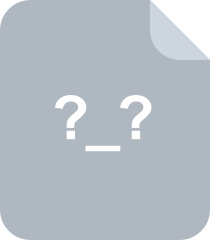
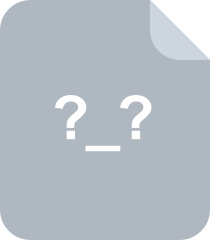
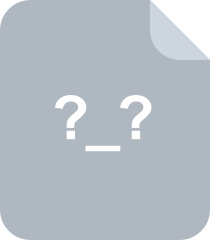
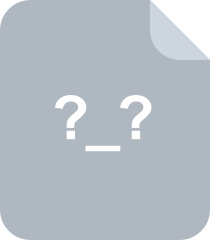
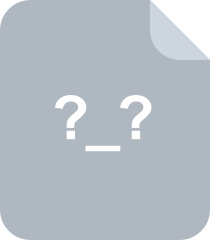
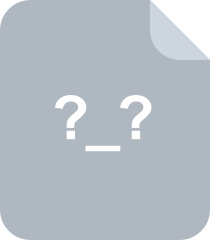
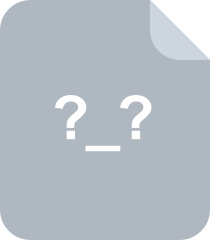
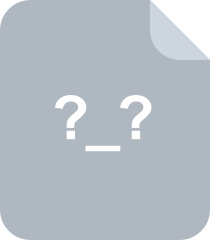
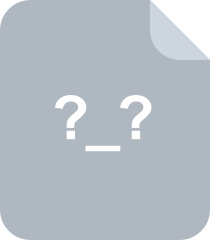
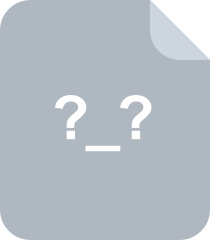
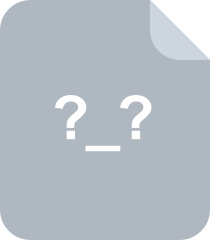
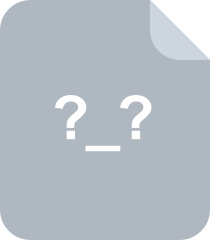
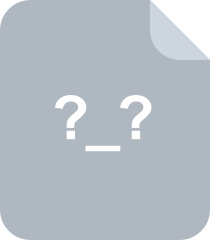
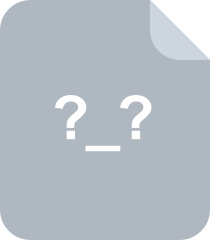
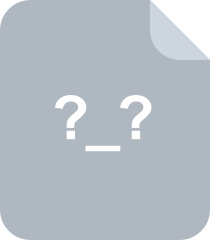
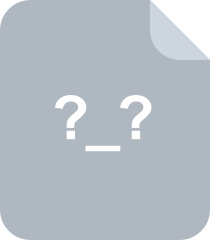
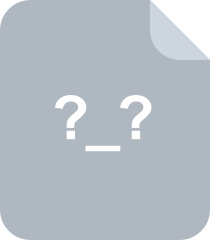
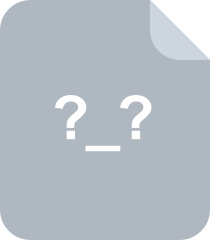
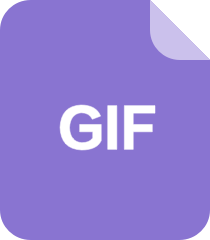
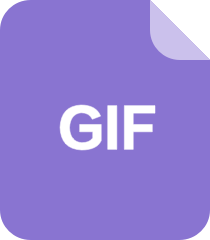
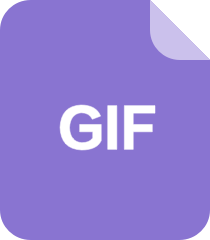
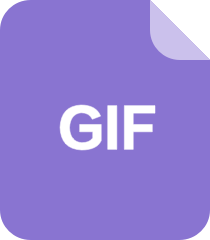
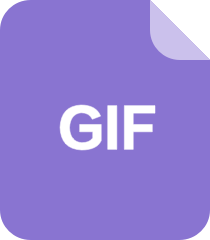
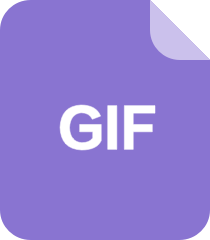
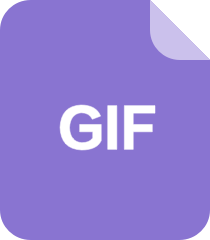
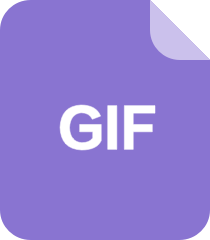
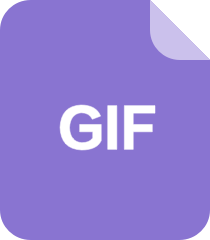
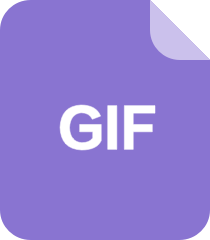
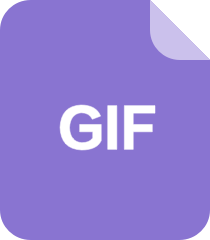
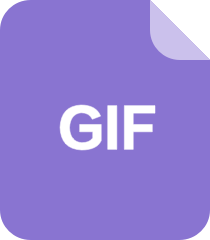
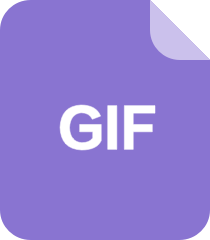
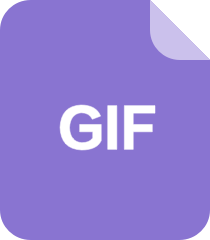
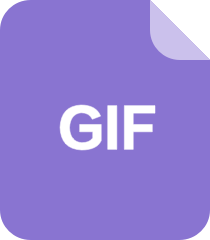
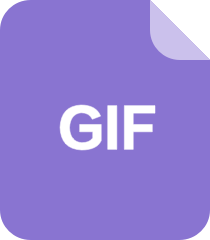
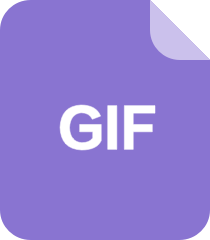
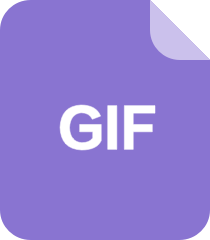
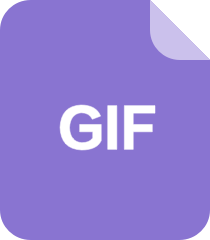
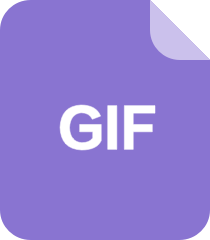
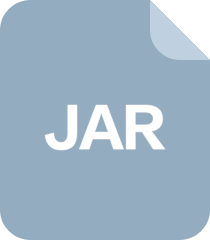
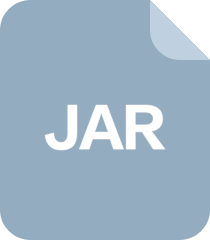
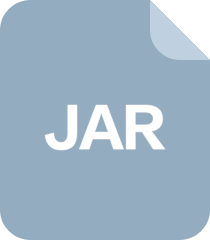
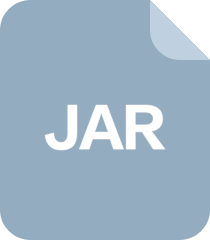
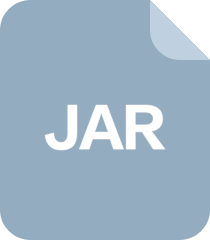
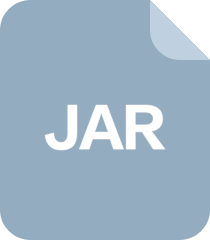
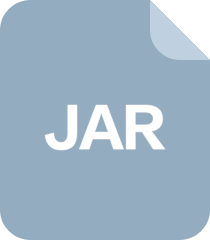
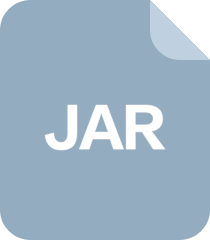
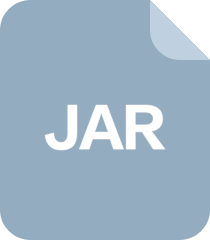
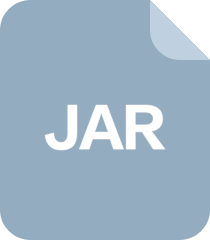
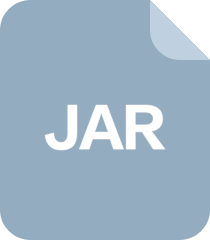
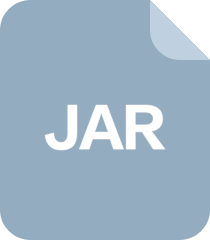
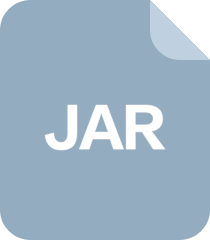
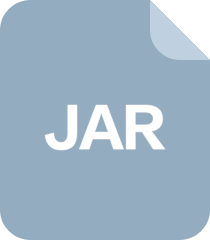
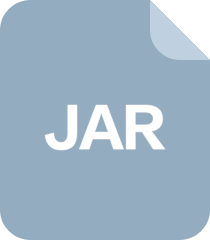
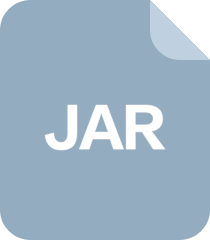
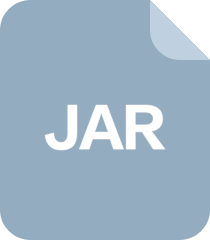
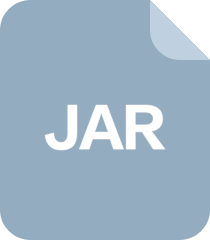
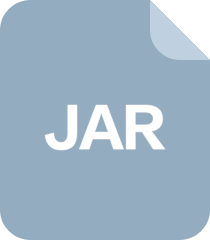
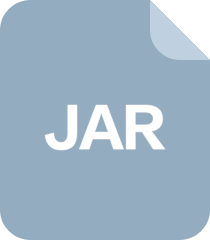
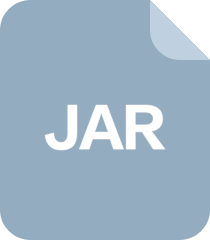
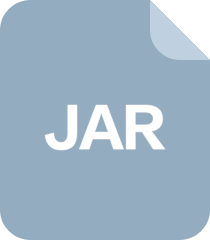
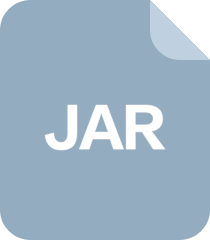
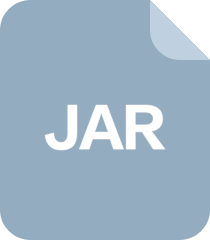
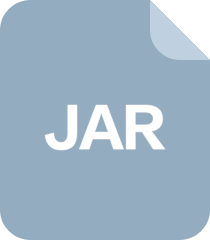
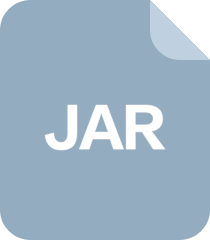
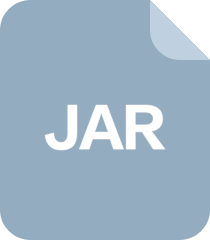
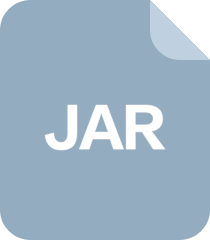
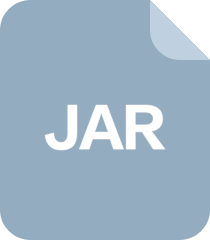
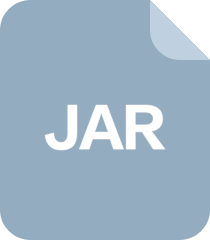
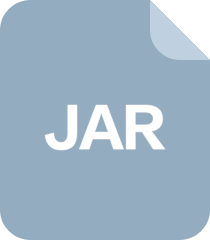
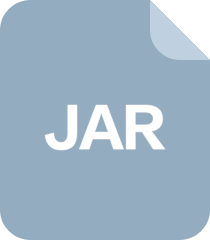
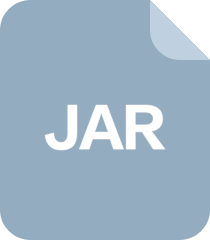
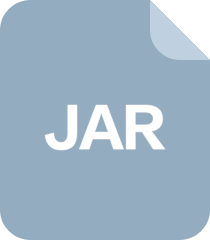
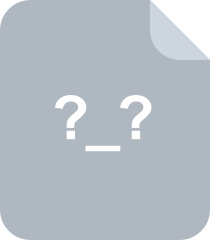
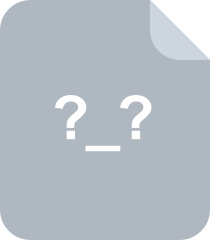
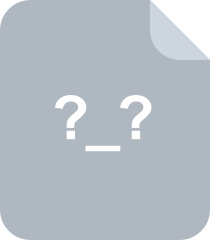
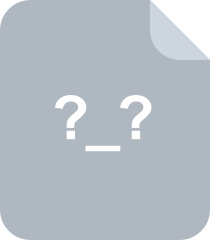
- 1
- 2
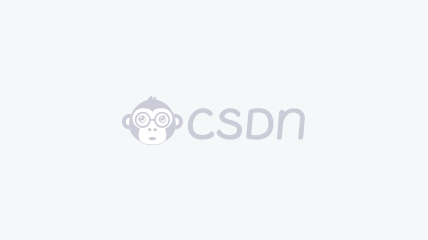
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

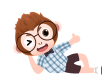
最新资源
- 基于POA算法优化BP神经网络的分类预测模型-包含多种优化算法对比的代码实现,基于POA算法优化BP神经网络的分类预测模型-包含多种优化算法对比的代码实现,鹈鹕优化算法(POA)优化BP神经网络(
- 瑞丽2011服装cad带超排
- FPGA PCIe软核解决方案:应对硬核资源不足,实现高效NVME大容量存储扩展,FPGA PCIe软核助力硬核扩展,高效应对NVMe大容量存储挑战,fpga pcie软核,用于扩展硬核不足的场景,例
- 图像保边滤波之Anisotropic滤波Demo
- 纯电动汽车Matlab Simulink软件模型:电池电机动力性经济性仿真,开源可编辑,与Cruise一致性达95%以上,纯电动汽车Matlab Simulink模型:开放源码,高效仿真,动力性与经济
- C51/STM32仿真软件,主要通过三极管、电阻、电容、单片机等等元器件进行搭载电路,软件程序调试的过程,完成项目功能
- 基于随机配置网络SCN的单输入单输出时间序列预测建模:Matlab程序化与注释详解,Matlab时间序列拟合预测建模:随机配置网络SCN单输入单输出建模方法详解,随机配置网络SCN做单输入单输出的时间
- 永磁同步电机PMSM全速域无速度传感器控制技术研究:高频注入与参考模型法的结合应用,永磁同步电机PMSM全速域无速度传感器控制技术研究:高频注入与参考模型法滑模观测结合应用,永磁同步电机PMSM全速域
- Arch Linux.zip
- 模块化多电平换流器(MMC)在孤岛模式下保护电网免受瞬态电压骤降影响的电能质量调节系统,模拟背靠背HVDC模块化多电平换流器(MMC)孤岛模式运行下的电能质量调节系统:瞬态电压保护、无功补偿与低谐波失
- 三菱PLC焊接机智能控制参考方案:含触摸屏程序、PLC程序、伺服定位与通信控制等全套解决方案,专为精准内外径圆环物料处理设计 ,三菱PLC焊接机智能控制参考方案:集成触摸屏程序、PLC编程、伺服控制与
- 伤寒钤法-draft草稿版-028
- 汇川AM系列程序及全自动N95口罩机:专业编程解决方案,涵盖高级功能如凸轮同步控制、超声波焊接及多种控制模式,ST编写,注释齐全 ,汇川AM系列程序与全自动N95口罩机:专业轴控制及触摸屏编程解决方案
- 五运六气批量处理=字体缩放+窗体缩放+xlsm格式=ok3(第13版)-exe-2024-6-11-用户烦不顺
- 超表面指导:宽频带多峰吸波器,效率与频率双调,实现多功能干涉模型应用 ,超表面指导下的多功能吸波器:宽频带、多峰吸波与干涉模型的高效结合,超表面指导 宽频带吸波器 多峰吸波器 效率可调,频率可调,多功
- 奇门遁甲草稿版-draft-SRC-D1023-033-OOKK

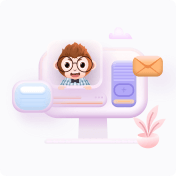
