ExpandableListView 展开列表控件
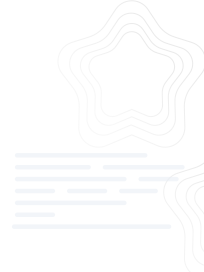


`ExpandableListView`是Android平台中一个非常实用的列表控件,它允许用户展示层次结构的数据,例如,一个父项可以展开显示多个子项。在Android应用开发中,当需要展示具有嵌套关系的数据时,`ExpandableListView`是一个理想的选择。本篇将详细介绍`ExpandableListView`的基本用法、数据绑定、自定义适配器以及优化技巧。 ### 1. `ExpandableListView`基础 `ExpandableListView`继承自`ListView`,它提供了扩展和折叠的功能。每个可展开的组(group)都有一个或多个子项(child)。用户可以通过点击父项来展开或折叠相应的子项。 ```xml <ExpandableListView android:id="@+id/expandable_list" android:layout_width="match_parent" android:layout_height="match_parent" /> ``` ### 2. 数据模型 在`ExpandableListView`中,数据模型通常由两个类表示:一个是代表父项的类,另一个是代表子项的类。例如,`Group`类用于存储父项数据,`Child`类用于存储子项数据。 ```java public class Group { public String groupName; public List<Child> children; } public class Child { public String childName; } ``` ### 3. 自定义适配器 为了将数据模型与`ExpandableListView`关联起来,我们需要创建一个自定义的适配器,继承自`BaseExpandableListAdapter`。这个适配器负责填充数据并处理视图的创建和复用。 ```java public class MyExpandableListAdapter extends BaseExpandableListAdapter { private List<Group> groups; // 构造函数,初始化数据 public MyExpandableListAdapter(List<Group> groups) { this.groups = groups; } @Override public int getGroupCount() { return groups.size(); } @Override public int getChildrenCount(int groupPosition) { return groups.get(groupPosition).children.size(); } @Override public Object getGroup(int groupPosition) { return groups.get(groupPosition); } @Override public Object getChild(int groupPosition, int childPosition) { return groups.get(groupPosition).children.get(childPosition); } // 其他重写方法... } ``` ### 4. 设置适配器 在活动中,我们需要设置自定义适配器到`ExpandableListView`上: ```java ExpandableListView expandableListView = findViewById(R.id.expandable_list); MyExpandableListAdapter adapter = new MyExpandableListAdapter(groups); expandableListView.setAdapter(adapter); ``` ### 5. 事件监听 我们可以监听`ExpandableListView`的展开和折叠事件,通过设置`OnGroupClickListener`和`OnChildClickListener`: ```java expandableListView.setOnGroupClickListener(new OnGroupClickListener() { @Override public boolean onGroupClick(ExpandableListView parent, View v, int groupPosition, long id) { // 处理父项点击事件 return false; // 返回false允许默认展开/折叠行为 } }); expandableListView.setOnChildClickListener(new OnChildClickListener() { @Override public boolean onChildClick(ExpandableListView parent, View v, int groupPosition, int childPosition, long id) { // 处理子项点击事件 return true; // 返回true阻止其他点击事件传播 } }); ``` ### 6. 自定义样式 `ExpandableListView`允许我们通过设置头布局和子布局来自定义视图。可以在XML中定义布局文件,并在适配器中使用它们。 ```xml <!-- header.xml --> <TextView android:id="@+id/group_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="18sp" /> <!-- child.xml --> <TextView android:id="@+id/child_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="16sp" /> ``` 在适配器中: ```java @Override public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) { Child child = (Child) getChild(groupPosition, childPosition); if (convertView == null) { LayoutInflater inflater = LayoutInflater.from(context); convertView = inflater.inflate(R.layout.child, parent, false); } TextView childTextView = convertView.findViewById(R.id.child_text); childTextView.setText(child.childName); return convertView; } @Override public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) { Group group = (Group) getGroup(groupPosition); if (convertView == null) { LayoutInflater inflater = LayoutInflater.from(context); convertView = inflater.inflate(R.layout.header, parent, false); } TextView groupTextView = convertView.findViewById(R.id.group_text); groupTextView.setText(group.groupName); return convertView; } ``` ### 7. 性能优化 由于`ExpandableListView`的复用机制,我们应该注意减少不必要的对象创建,尤其是在`getChildView`和`getGroupView`方法中。此外,如果数据量大,可以考虑使用异步加载和分页策略来提高性能。 ### 8. 扩展功能 除了基本功能,`ExpandableListView`还支持一些高级特性,如动画效果、多选模式等。开发者可以根据需求利用这些特性来丰富用户体验。 `ExpandableListView`是Android开发中处理层级数据的有效工具。理解其工作原理,熟练掌握自定义适配器和事件监听,结合适当的优化策略,能够帮助我们创建出功能丰富的界面。
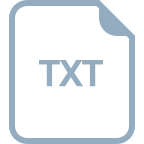
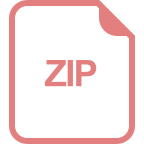
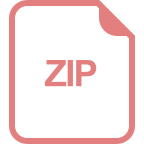
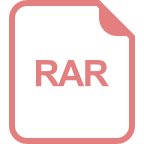
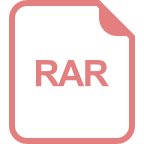
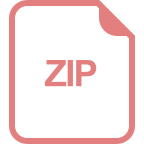
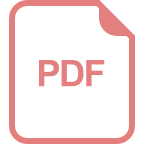
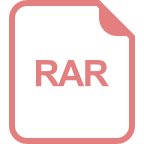
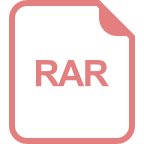
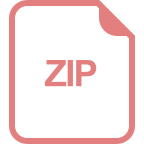
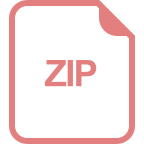
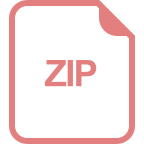
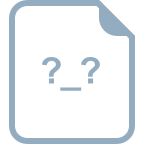
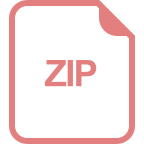
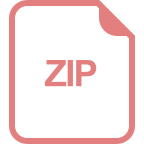
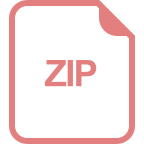
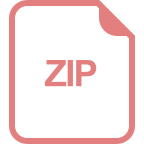
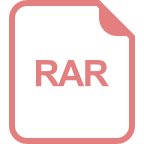
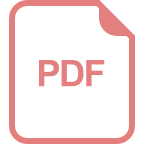



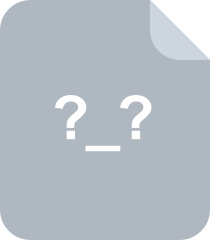


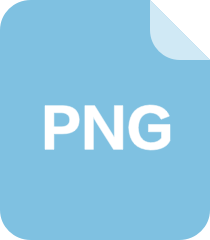

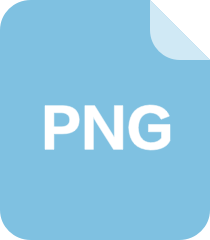

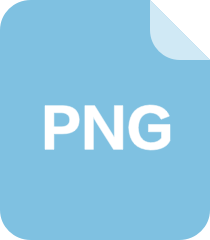
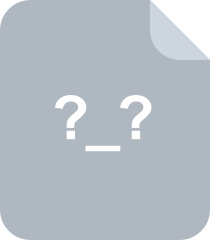




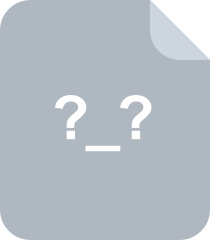
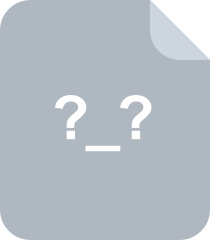
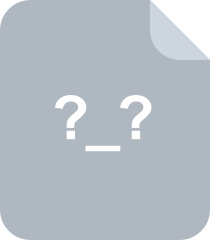
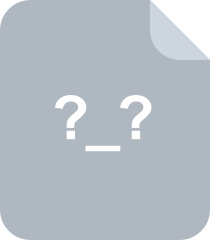
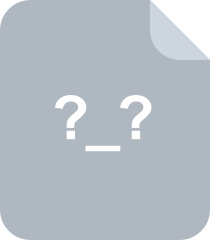
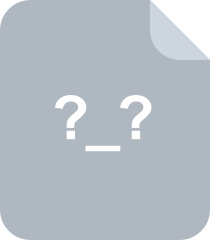
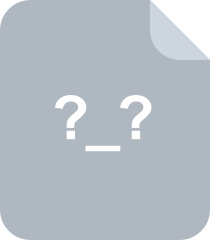
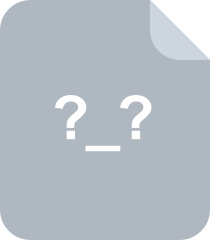
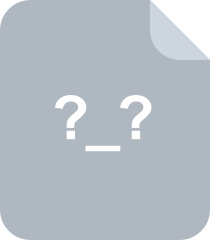


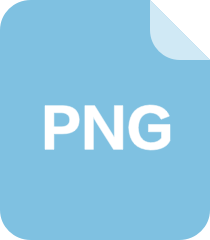

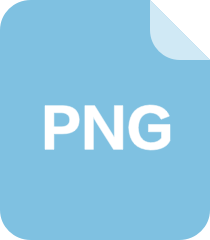
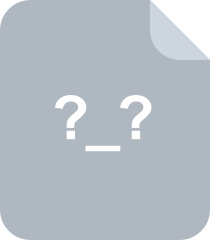
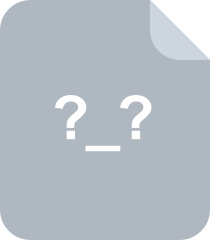

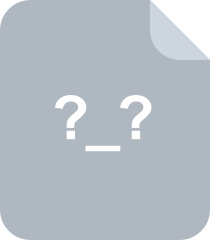

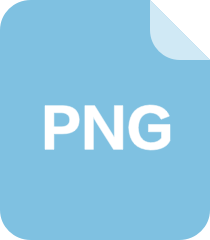

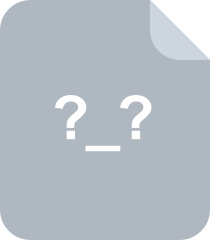
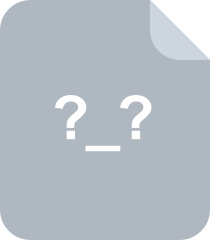





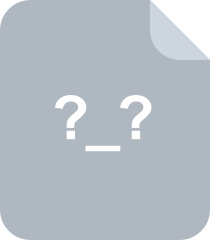
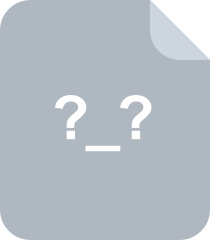




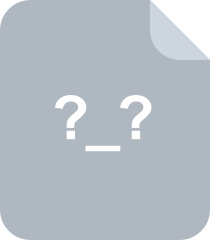
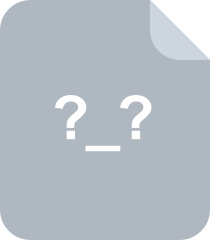
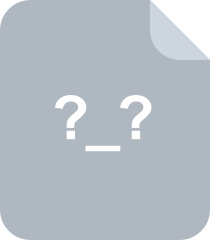
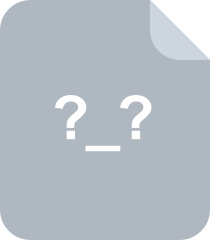
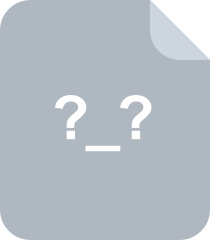
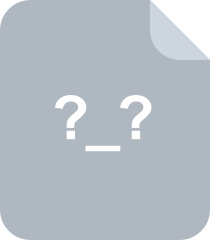
- 1

- 粉丝: 36
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

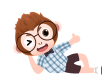
最新资源
- 概率论笔记概率论笔记自用
- 电子学习资料课程设计放大器电路的
- ConsoleApplication2.0控制台应用空白源码
- 电子学习资料课程设计计数器电路
- 电子学习资料课程设计交通灯控制器设计
- Python+Django+Vue在线图书借阅网站、图书管理系统 - 毕业设计 - 课程设计
- 电子学习资料课程设计模拟电路插件电路
- 《Chapter 8 ERP项目之JeecgBoot》《Chapter 9 ERP项目之Activiti引擎》对应资源一
- 电子学习资料课程设计模拟电路插接电路
- 电子学习资料课程设计世界十大设计团队的设计策略
- 《Chapter 8 ERP项目之JeecgBoot》《Chapter 9 ERP项目之Activiti引擎》对应资源二
- 深度学习飞机大战智能体训练代码(V1.0)
- 电子学习资料课程设计数字电路插接,焊接电路部分
- selenium+google浏览器
- 电子学习资料课程设计数字逻辑电路设计课题
- 计算机C语言程序设计实验

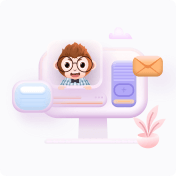

- 1
- 2
- 3
- 4
- 5
- 6
前往页