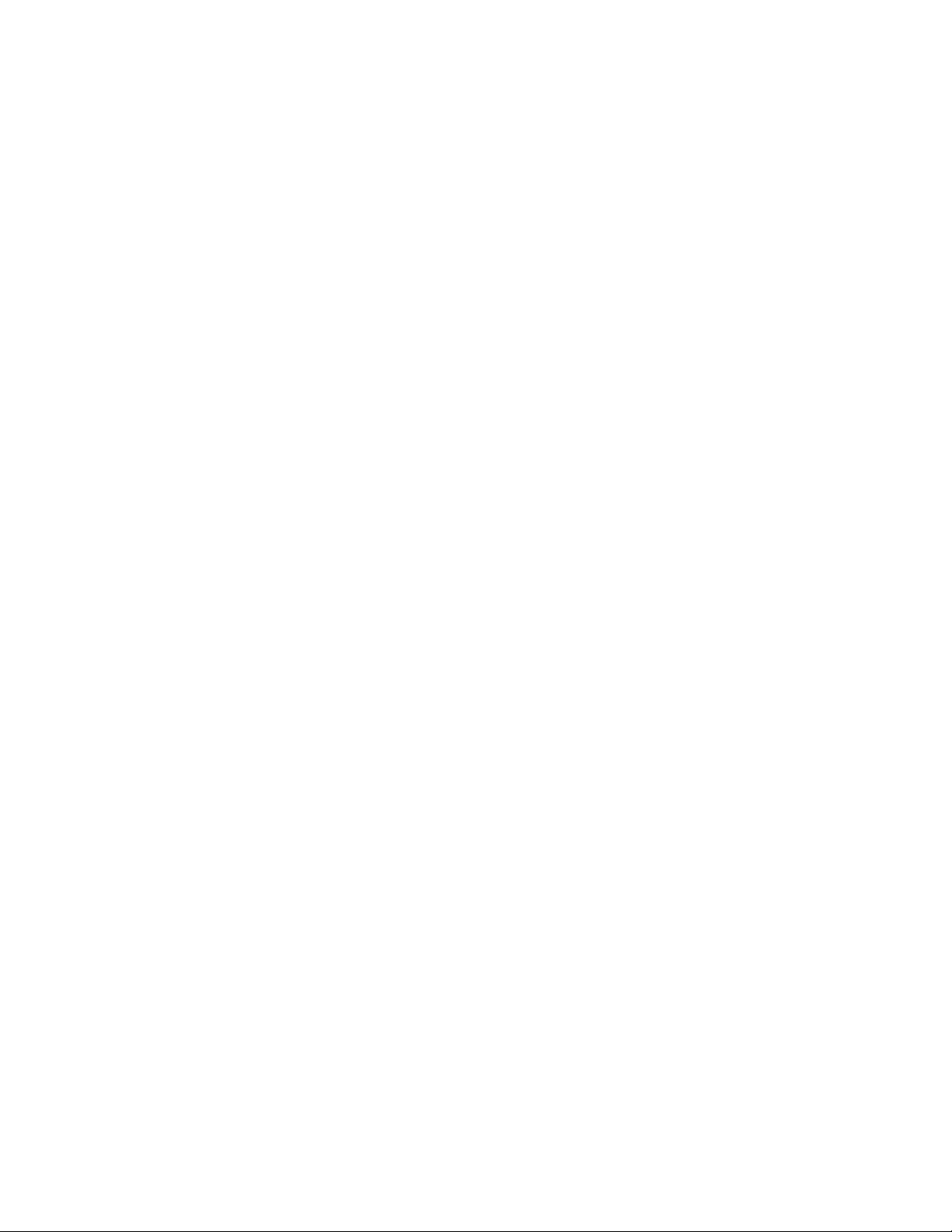
1
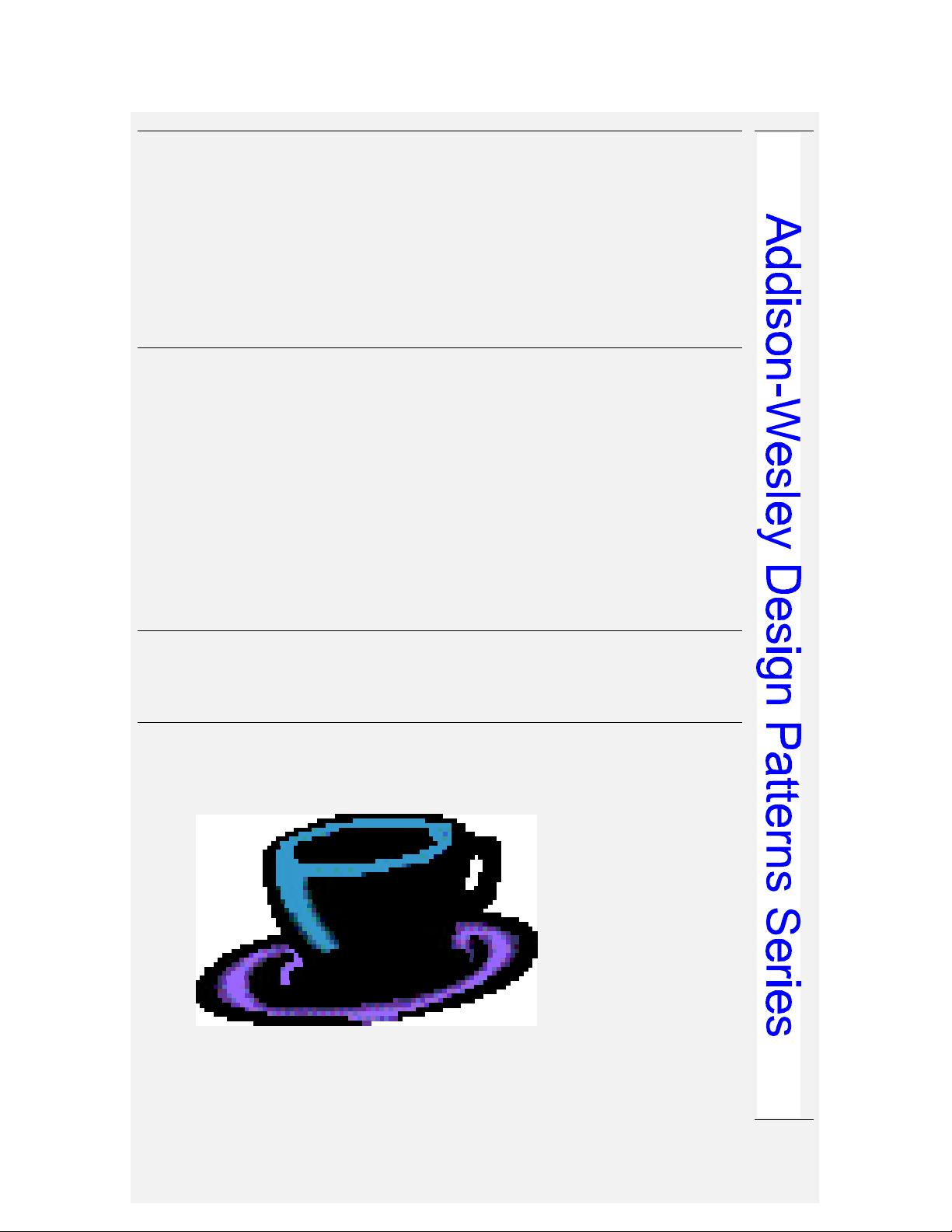
2
THE
DESIGN PATTERNS
JAVA COMPANION
JAMES W. COOPER
October 2, 1998
Copyright © 1998, by James W. Cooper
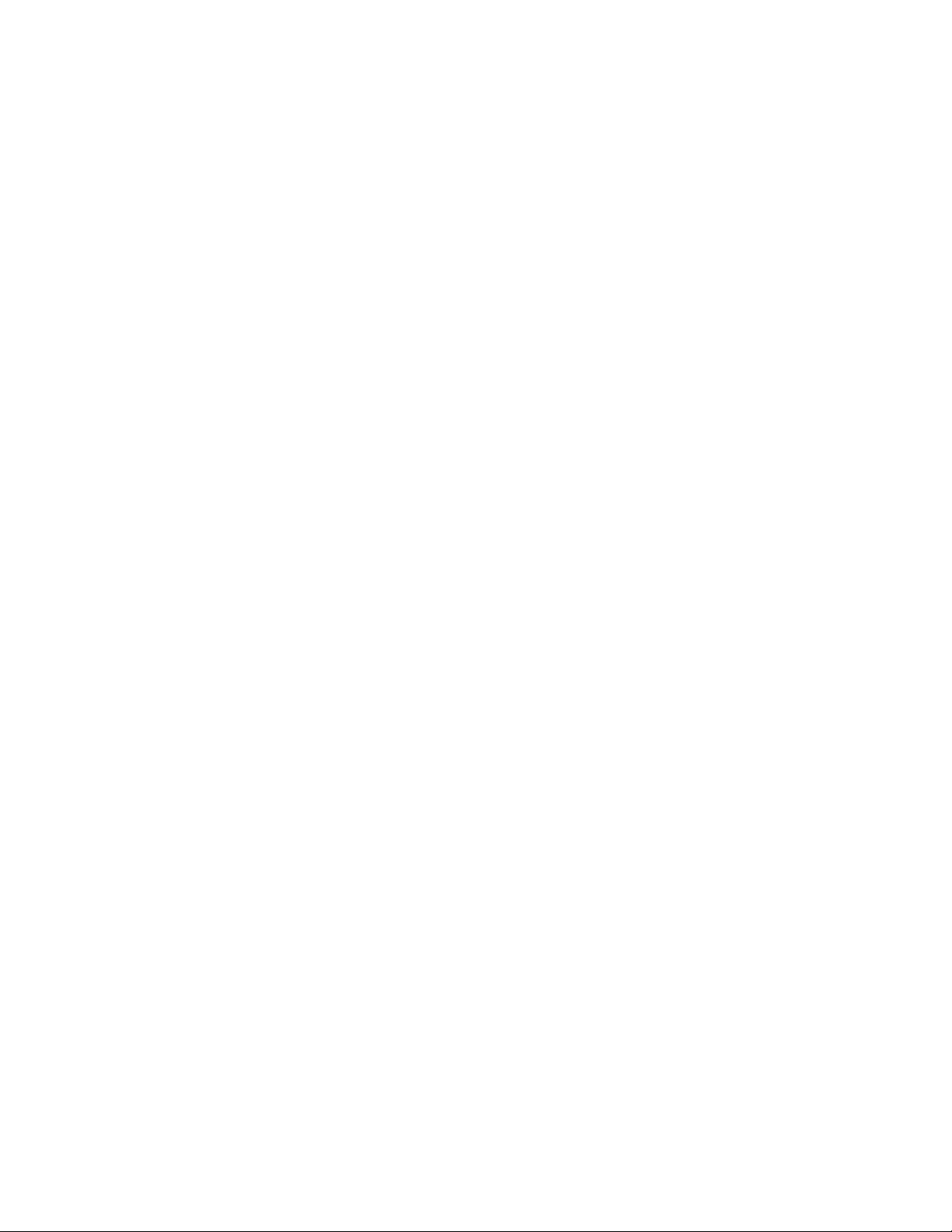
3
Some Background on Design Patterns 10
Defining Design Patterns 11
This Book and its Parentage 13
The Learning Process 13
Studying Design Patterns 14
Notes on Object Oriented Approaches 14
The Java Foundation Classes 15
Java Design Patterns 15
1. Creational Patterns 17
The Factory Pattern 18
How a Factory Works 18
Sample Code 18
The Two Derived Classes 19
Building the Factory 20
Factory Patterns in Math Computation 22
When to Use a Factory Pattern 24
Thought Questions 25
The Abstract Factory Pattern 26
A GardenMaker Factory 26
How the User Interface Works 28
Consequences of Abstract Factory 30
Thought Questions 30
The Singleton Pattern 31
Throwing the Exception 32
Creating an Instance of the Class 32
Static Classes as Singleton Patterns 33
Creating Singleton Using a Static Method 34
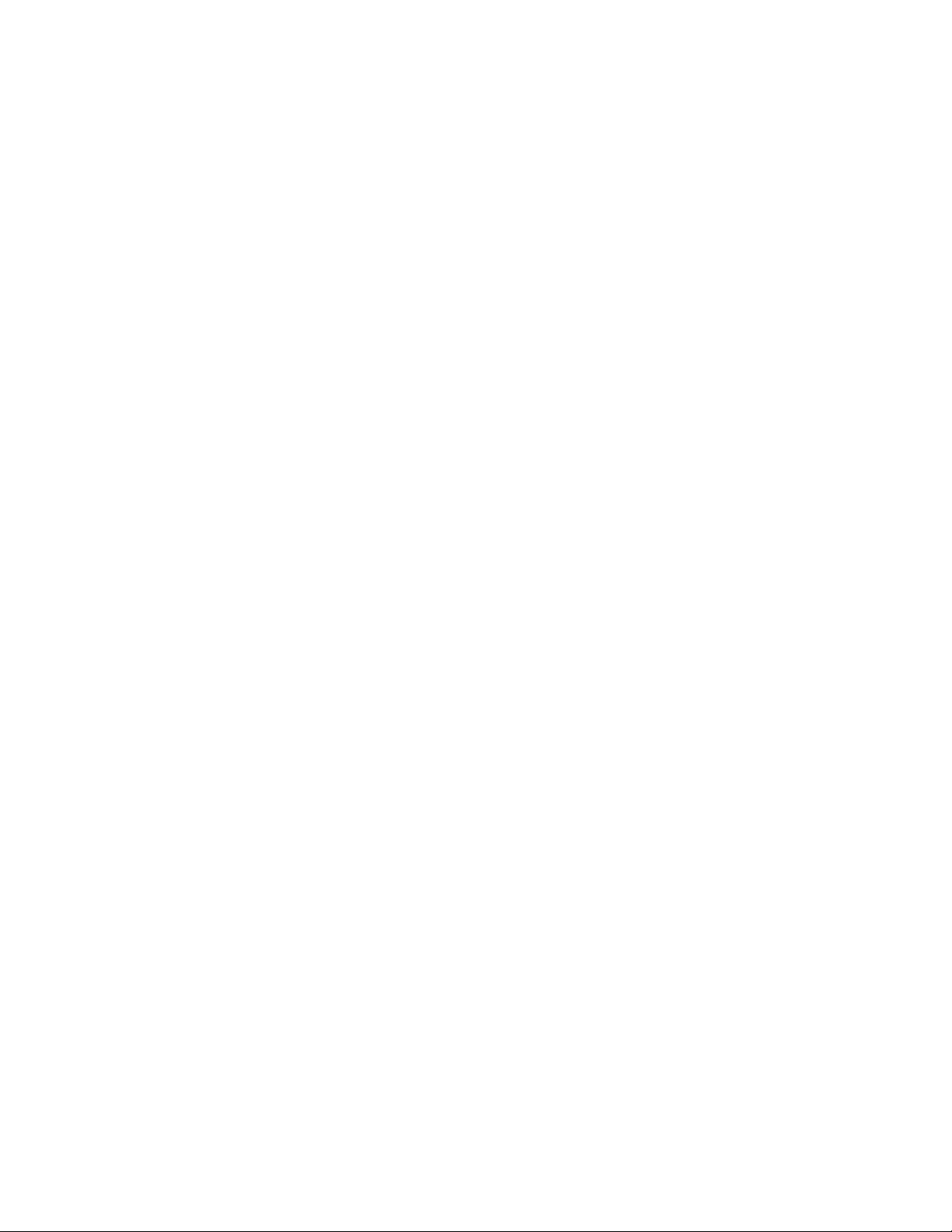
4
Finding the Singletons in a Large Program 35
Other Consequences of the Singleton Pattern 35
The Builder Pattern 37
An Investment Tracker 38
Calling the Builders 40
The List Box Builder 42
The Checkbox Builder 43
Consequences of the Builder Pattern 44
Thought Questions 44
The Prototype Pattern 45
Cloning in Java 45
Using the Prototype 47
Consequences of the Prototype Pattern 50
Summary of Creational Patterns 51
2. The Java Foundation Classes 52
Installing and Using the JFC 52
Ideas Behind Swing 53
The Swing Class Hierarchy 53
Writing a Simple JFC Program 54
Setting the Look and Feel 54
Setting the Window Close Box 55
Making a JxFrame Class 55
A Simple Two Button Program 56
More on JButtons 57
Buttons and Toolbars 59
Radio Buttons 59
The JToolBar 59
Toggle Buttons 60
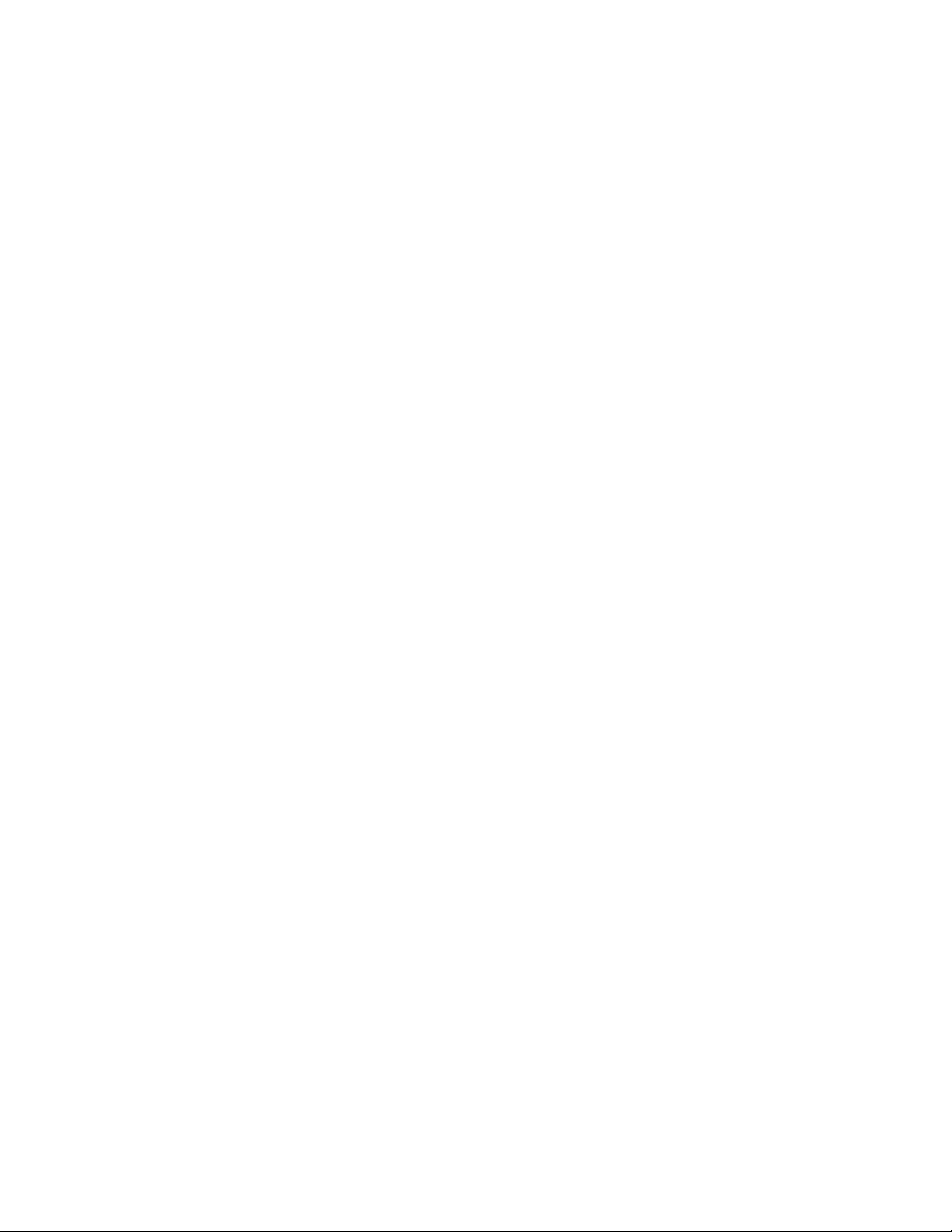
5
Sample Code 61
Menus and Actions 62
Action Objects 62
Design Patterns in the Action Object 65
The JList Class 67
List Selections and Events 68
Changing a List Display Dynamically 69
The JTable Class 71
A Simple JTable Program 71
Cell Renderers 74
The JTree Class 77
The TreeModel Interface 78
Summary 79
3. Structural Patterns 80
The Adapter Pattern 81
Moving Data between Lists 81
Using the JFC JList Class 83
Two Way Adapters 87
Pluggable Adapters 87
Adapters in Java 88
The Bridge Pattern 90
Building a Bridge 91
Consequences of the Bridge Pattern 93
The Composite Pattern 95
An Implementation of a Composite 96
Building the Employee Tree 98
Restrictions on Employee Classes 100