#!/usr/bin/env python
#
# Hi There!
#
# You may be wondering what this giant blob of binary data here is, you might
# even be worried that we're up to something nefarious (good for you for being
# paranoid!). This is a base85 encoding of a zip file, this zip file contains
# an entire copy of pip (version 23.1.2).
#
# Pip is a thing that installs packages, pip itself is a package that someone
# might want to install, especially if they're looking to run this get-pip.py
# script. Pip has a lot of code to deal with the security of installing
# packages, various edge cases on various platforms, and other such sort of
# "tribal knowledge" that has been encoded in its code base. Because of this
# we basically include an entire copy of pip inside this blob. We do this
# because the alternatives are attempt to implement a "minipip" that probably
# doesn't do things correctly and has weird edge cases, or compress pip itself
# down into a single file.
#
# If you're wondering how this is created, it is generated using
# `scripts/generate.py` in https://github.com/pypa/get-pip.
import sys
this_python = sys.version_info[:2]
min_version = (3, 7)
if this_python < min_version:
message_parts = [
"This script does not work on Python {}.{}".format(*this_python),
"The minimum supported Python version is {}.{}.".format(*min_version),
"Please use https://bootstrap.pypa.io/pip/{}.{}/get-pip.py instead.".format(*this_python),
]
print("ERROR: " + " ".join(message_parts))
sys.exit(1)
import os.path
import pkgutil
import shutil
import tempfile
import argparse
import importlib
from base64 import b85decode
def include_setuptools(args):
"""
Install setuptools only if absent and not excluded.
"""
cli = not args.no_setuptools
env = not os.environ.get("PIP_NO_SETUPTOOLS")
absent = not importlib.util.find_spec("setuptools")
return cli and env and absent
def include_wheel(args):
"""
Install wheel only if absent and not excluded.
"""
cli = not args.no_wheel
env = not os.environ.get("PIP_NO_WHEEL")
absent = not importlib.util.find_spec("wheel")
return cli and env and absent
def determine_pip_install_arguments():
pre_parser = argparse.ArgumentParser()
pre_parser.add_argument("--no-setuptools", action="store_true")
pre_parser.add_argument("--no-wheel", action="store_true")
pre, args = pre_parser.parse_known_args()
args.append("pip")
if include_setuptools(pre):
args.append("setuptools")
if include_wheel(pre):
args.append("wheel")
return ["install", "--upgrade", "--force-reinstall"] + args
def monkeypatch_for_cert(tmpdir):
"""Patches `pip install` to provide default certificate with the lowest priority.
This ensures that the bundled certificates are used unless the user specifies a
custom cert via any of pip's option passing mechanisms (config, env-var, CLI).
A monkeypatch is the easiest way to achieve this, without messing too much with
the rest of pip's internals.
"""
from pip._internal.commands.install import InstallCommand
# We want to be using the internal certificates.
cert_path = os.path.join(tmpdir, "cacert.pem")
with open(cert_path, "wb") as cert:
cert.write(pkgutil.get_data("pip._vendor.certifi", "cacert.pem"))
install_parse_args = InstallCommand.parse_args
def cert_parse_args(self, args):
if not self.parser.get_default_values().cert:
# There are no user provided cert -- force use of bundled cert
self.parser.defaults["cert"] = cert_path # calculated above
return install_parse_args(self, args)
InstallCommand.parse_args = cert_parse_args
def bootstrap(tmpdir):
monkeypatch_for_cert(tmpdir)
# Execute the included pip and use it to install the latest pip and
# setuptools from PyPI
from pip._internal.cli.main import main as pip_entry_point
args = determine_pip_install_arguments()
sys.exit(pip_entry_point(args))
def main():
tmpdir = None
try:
# Create a temporary working directory
tmpdir = tempfile.mkdtemp()
# Unpack the zipfile into the temporary directory
pip_zip = os.path.join(tmpdir, "pip.zip")
with open(pip_zip, "wb") as fp:
fp.write(b85decode(DATA.replace(b"\n", b"")))
# Add the zipfile to sys.path so that we can import it
sys.path.insert(0, pip_zip)
# Run the bootstrap
bootstrap(tmpdir=tmpdir)
finally:
# Clean up our temporary working directory
if tmpdir:
shutil.rmtree(tmpdir, ignore_errors=True)
DATA = b"""
P)h>@6aWAK2ms(pnpOdJ9~Srk003nH000jF003}la4%n9X>MtBUtcb8c|B0UO2j}6z0X&KUUXrd;wrc
n6ubz6s0VM$QfAw<4YV^ulDhQoop$MlK*;0e<?$L01LzdVw?IP-tnf*qTlkJj!Mom=viw7qw3H>hK(>
3Z_jZ>VV`^+*aO7_tw^Cd$4zs{Pl#j>6{|X*AaQ6!2wJ?w>%d+2&1X4Rc!^r6h-hMtH_<n)`omXfA!z
c)+2_nTCfpGRv1uvmTkcug)ShEPeC#tJ!y1a)P)ln~75Jc!yqZE1Gl6K?CR$<8F6kVP)a}pU*@~6k=y
<MFxvzbFl3|p@5?5Ii7qF0_`NT{r7m1lM_B44a9>d5{IF3D`nKTt~p1QY-O00;o!N}5(98{@xi0ssK6
1ONaJ0001RX>c!JUu|J&ZeL$6aCu!*!ET%|5WVviBXU@FMMw_qp;5O|rCxIBA*z%^Qy~|I#aghDZI*1
mzHbcdCgFtbH*em&nbG}VT_EcdJ^%Uh<#$rfXmjvMazjtt+Y{4fL(0@tjn1(F!nz|6RBOjou<lHavpt
2DsnN~{0?3^aZW|#k1{K<zbVGw<F9gAoI$2%Q=!IwHz3?Ga8yfULmF;_^_Efc89djgN{>LCQKB%tCsn
f_O;(TkT9D!5I2G1vZ<eHSH;T&3P=(dl1Ul+n}iN0$4eg8-DWoeqjlH$Ojn(A!3eMku3iYf*>WcORK<
*}iONjWAr8Zm1&KuL0jC{@?djd+x5R}RGfYPBawx08>U(W?WmDk1T9S4?epCt{Z(ueTz)EC*E`5mT15
-&2~-DsS-6=uU3I|BmObEPJI*Sr)^2!Om@h-$wOJl_c@O>A_3OHg5wqIeD(E7`y@m0ou*N^~8Scf|wu
`N_HtL5`*k&gASg%W(oQp9a7<~IpnR_S}F8z9z|q{`1rb)-o!>My0eex)q(ByedFLGyO7=Ikq8}(HcH
6i;acy-%V$hD`fEosH<F#RjvR|Bj1`9E=F8_#Ldd3;(iLXg4(#CUYq600w1FS!F^DDXW$?A?W<%x`1G
o!_!Mo=`yT9C6$GfF^02ZS8gBiIDv=G#cRjO3bn3+}$P=T2Wt8GE|SP^4`jHeuCMeAZ0bK3QoB})QI^
}#>@wgA+8z#{H{ToXOd_?&uMj~(yRVmD7BE?-`X6FU!78rkLs#HE1jqSOWnjp~Z3(}j4wN{#<0DmEaw
w2fbN$l@K=F!>KqO9KQH000080N_fRR(=O1mCynJ0Hg%~02KfL0B~t=FJE79X>cuab#88Da$jFAaCv=
H!EW0y488j+IQK9caJ`}(Fd$fl0Y!@S(qu_`7`j4GY`W2AORgkmF^c~CNIOp2pqmbfB$JPikIxRnaI(
d$@d&t;nJ-)LYvmv_bql6|TGa{sQFNz4LavGeEou*_H_94a(LN1=C8rdsM4*2yE6hPUP@awnctg>yu}
H|$_wbd;8;Z`Pe(zyLX;p2kr?icdfz-P*I4?c+HNr3qf)n`g?k9BBHfWtPnuu1l^lGI_<y*+snEeKan
dfI!<2v+N>(_KV-v^hNGExu>by~;Zm!?+4p|GYR4Bymg-3GHC%Wu;gF`s<Dy`E1NFox(NflqM|1UVK1
58=FxcKdh)a00%qaRll?8;b$ZvV>?gglU-mGD=5XMhu0qumO^H$R=P_Fr{?BR=O~Eqw{<C3`cB6J+f2
|VbMAgYqC{7>TY?3ue9pt3AsU<lA*Snx@*7g^?7=R$l_z8EIt1+etM3HD$%Rt3q)Qodwz>&oV*G-;vD
a>`d!S@U$N$E@)j6PhU=^WjoZ)e0t%F*ACjR~4Z8?QBJJh2pR&bDDosFd&3Zp)+i0>i4M&?tIfBW@v-
6;vcK^IotF)3Cc^z##cyK2D`by~>?OTb)rBr1-1n0`K{f{Dp+4W2;rnmFU$wJJh(<PZ`7a0xzLh$<|M
9lNgO6eTTRDIpS&XJ<n)B2%j(vSUX7gixPoOc)O$ASWct1*ilmPG<ZQ<hvH0p>ZrF`Bks+6VOA;U8w@
MJ^n&;oTTQ@=iE<WrUrj87J63;NsNaLAUh4>A>dhSd;sK*1;dt9rD{rnF&P435VVe89PFpK{B#8NggS
<^;Bs_i_Vh&2ph5dyRt$swh%C;-i2U)2@xg`UP{ex51EN#NR(6Bkz<6Q&jo+?B+Xu*a{TT)7Y3d2Qu-
DBP1K2*Fh*Bp{IY;7J)sf#@zOTb++R>j0|XQR000O8;7XcS&BwqszyJUM9svLV3;+NCaA|NaaCt6td2
nT9C62L9#V`y-_kP9QSf2V13=C{<Kw>tnX)PsolsJX_Jx&=d9p7_`6i5SMvz$qHBvD4Gc2vqMK2J#u@
ySRoi8HJ74pBUZpQaDYr)B{xbde<biidBj6SwLQ4C~0fIn*4z#kiE0Sc{#il<@j|pBMZL#}ADaAESsK
i)hSbaxtCyXu4z%H~r`8#VV{D!!(UMBd94M9GxnKfLFZz7T$d6N~+ca-?5#f2RFEdlxM*G?W6ErQaLd
-ZtL;~P)h>@6aWAK2ms(pnpT#zaG_BF002D#000>P003}la4%nJZggdGZeeUMUtei%X>?y-E^v8ukug
uiFbswF{0i$>t`ejR5^xfXOea{_5ITj{ZH>|-*e<C59=C8HB*+r$$$9?r+;JX3=R&Cm8cSw{J&B&eeN
oCOMCZQbLd72_DYB`4Qi|d!y<npU!DeDq4oTKlIDwR3gX<RaKi(ZD9b)dCI{`|hOWhmAwt{gIg=d5&#
E7j`U1o%k=2ZdB@YU;k)V-C++sbU-2WkcwLMfO8f*!}@4#sSjV{WI2;@vXK{~qd`Yti}wrETC|cCbAr
@VEr>D9TSy6<otzPFTU&jZy2)ft}4}^DvP8N}w<b@|#f`GvwGplau6#APrMd0UZo%3^Rx&5tnZ=cF33
-<5=xTy<3Z0vj}ZVpBT`h1`F>L1Q7<+BD=coNr&m#H+ihfTtaPW*CaBb)EDPhm;MO2-v8~xV^W?=HuY
yW@4N)bpD4E7iPN{ZMpU^EP)h>@6aWAK2ms(pnpO-Rh+|s}0009h000^Q003}la4%nJZggdGZeeUMVs
&Y3WM5@&b}n#v)mlq)<G2yN>sMeohb0q|`#7akqa^dt?6@-VxMn<?Y^hXSiiAdtDUu72wzV$*_jES^5
+Et>Y$})4!4knnqaR;C0NC@qmt9fjY+c<JqOOj-YFQ_F&i1ung{;f8E$WKpohYi$Oy-4b*$!vG<HGa2
#iBSiwP;ycfBuYP`mvVgSCh7fve=nNS+u<Wed?)Ne&61Sv{~#$ePmf(mOR6iCDR1&Ma~7!Ul&=cXxdZ
5`?uF3&G;_OZmwape~%&?f}gI|$*Z^PSO2)U{2^
没有合适的资源?快使用搜索试试~ 我知道了~
ChatGPT小型机器人
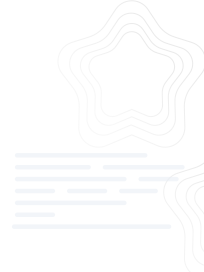
共11150个文件
png:3693个
py:3161个
pyc:3148个

需积分: 0 42 下载量 71 浏览量
2023-05-30
17:59:06
上传
评论 4
收藏 145.36MB ZIP 举报
温馨提示
利用ChatGPT,如何创建一个属于自己的专属QQ机器人?
资源推荐
资源详情
资源评论
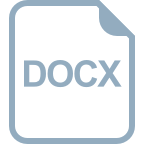
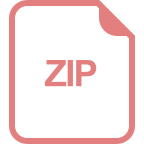
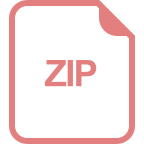
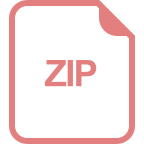
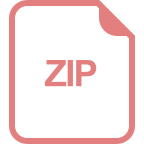
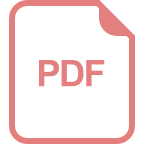
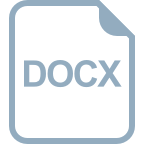
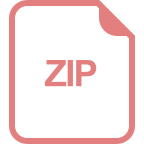
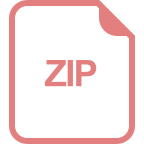
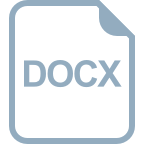
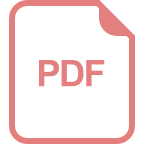
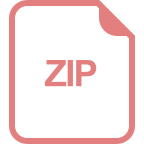
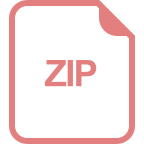
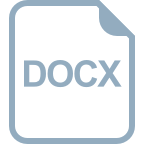
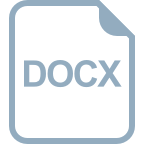
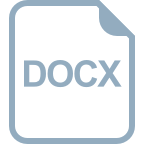
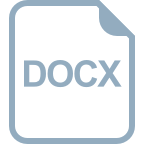
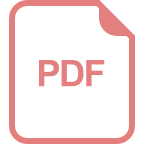
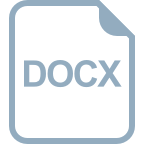
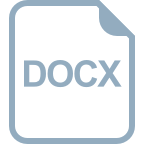
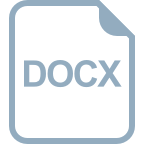
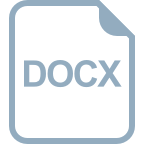
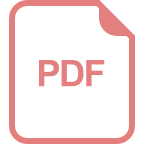
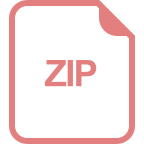
收起资源包目录

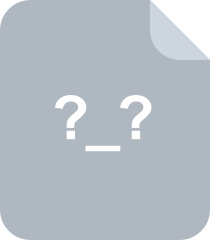
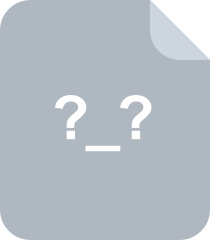
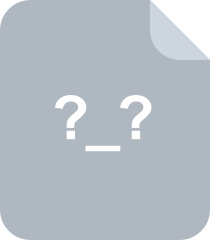
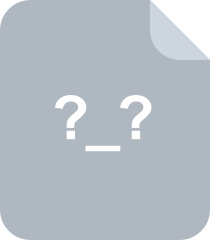
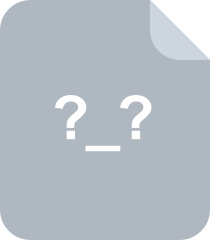
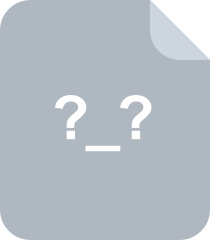
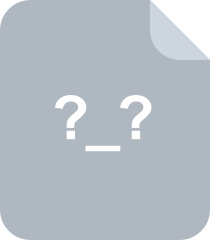
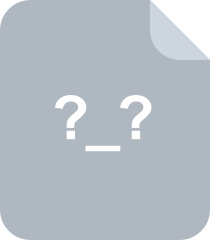
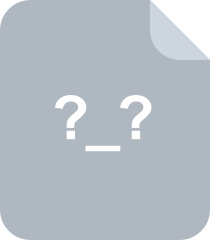
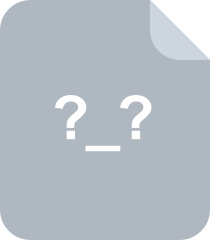
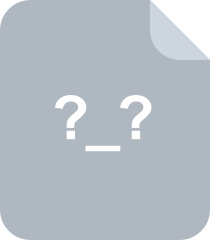
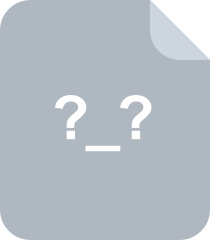
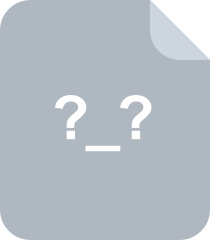
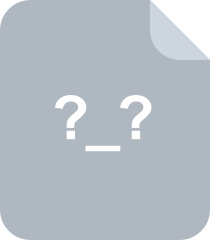
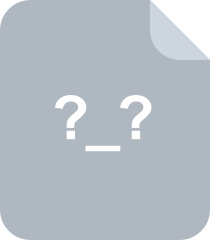
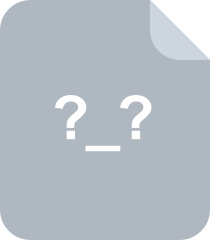
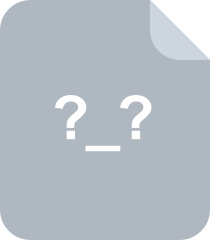
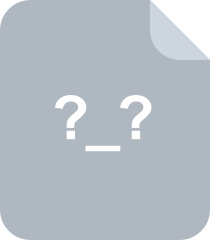
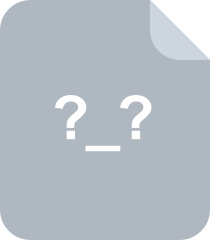
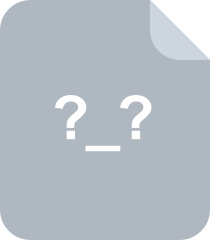
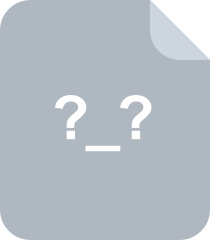
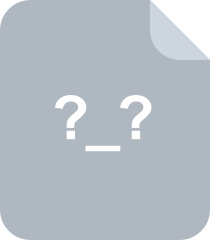
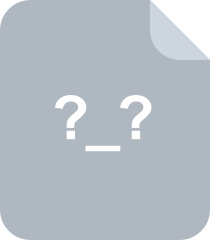
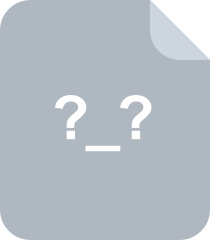
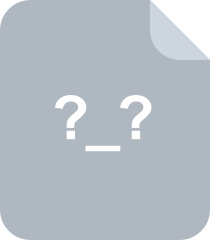
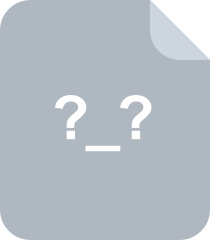
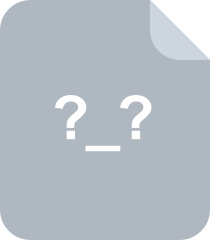
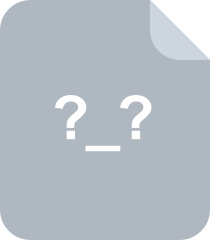
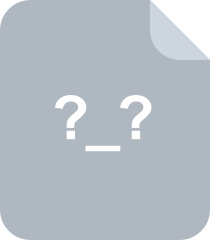
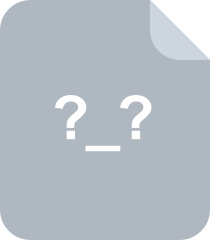
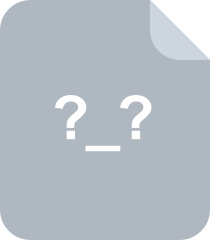
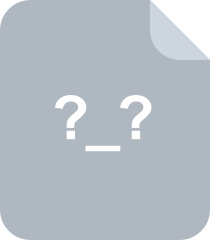
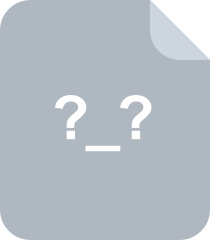
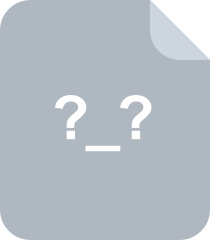
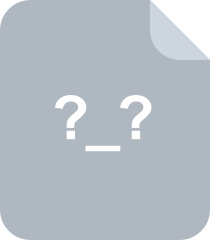
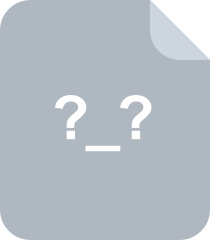
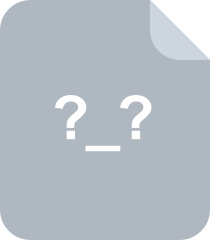
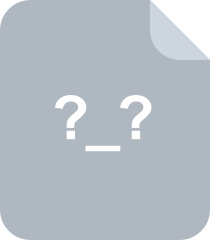
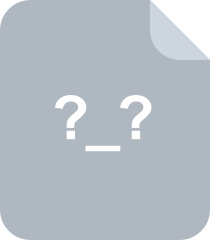
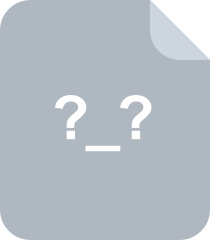
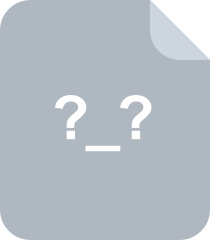
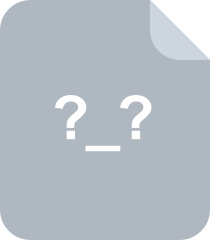
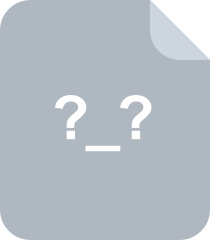
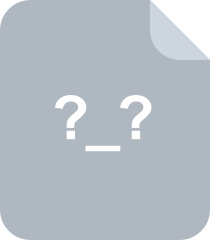
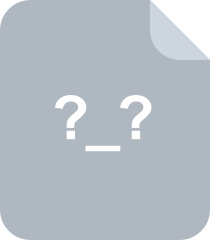
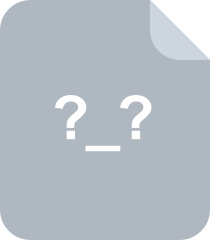
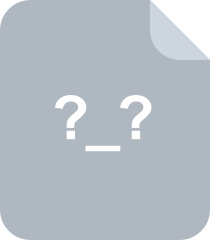
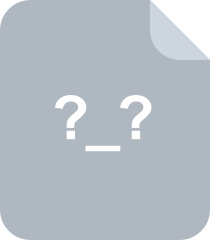
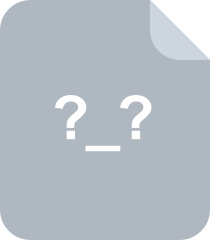
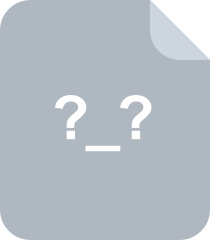
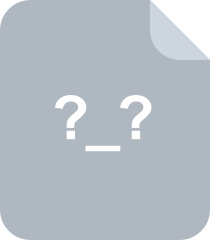
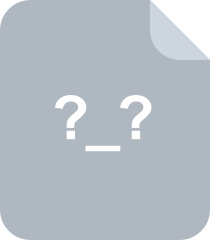
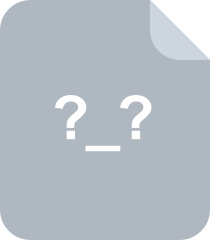
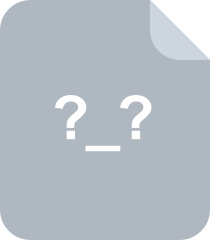
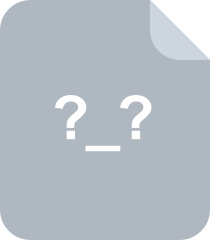
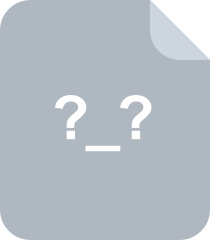
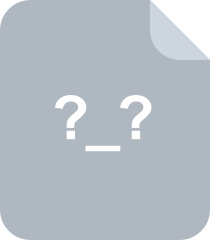
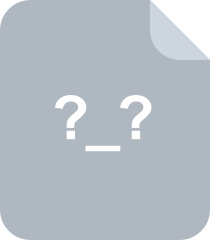
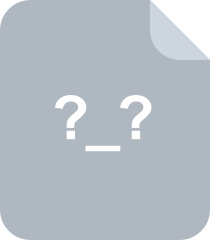
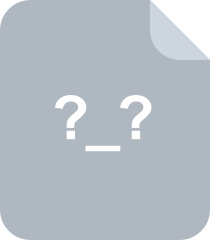
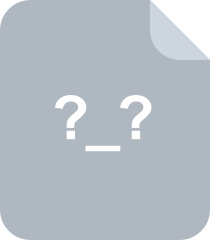
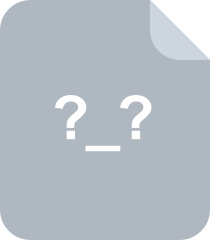
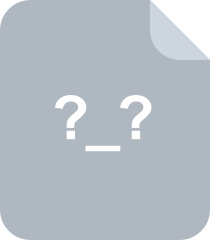
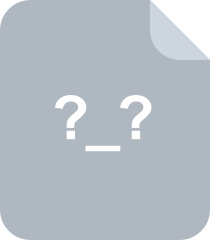
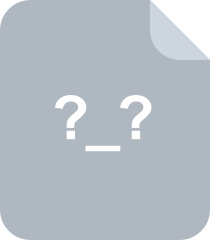
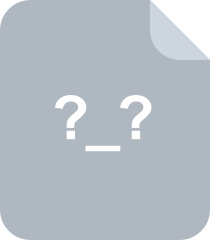
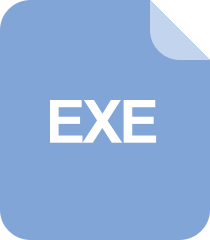
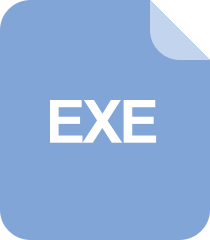
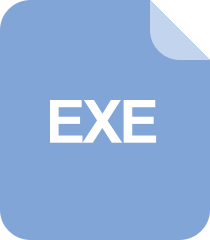
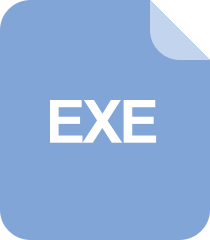
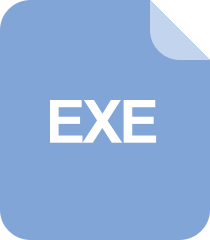
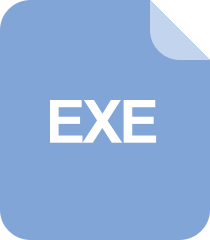
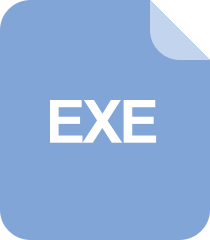
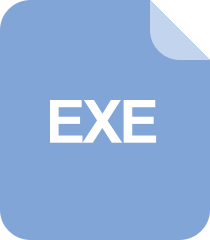
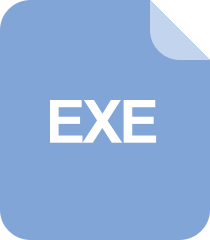
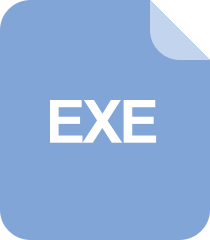
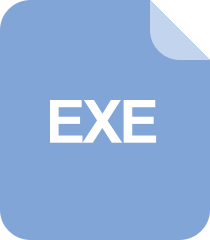
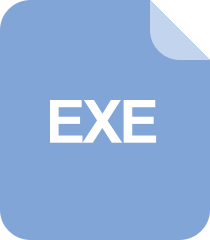
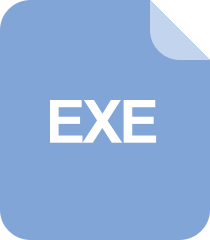
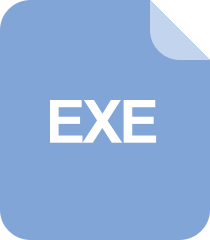
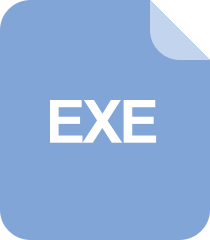
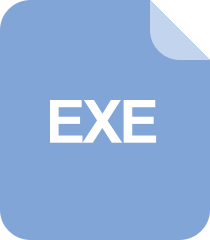
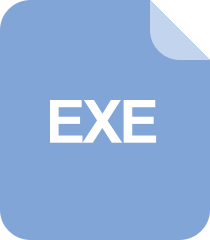
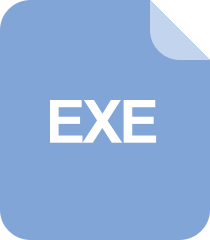
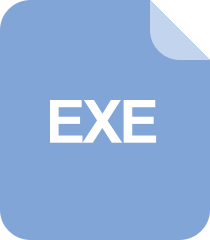
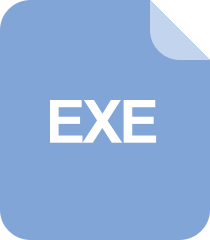
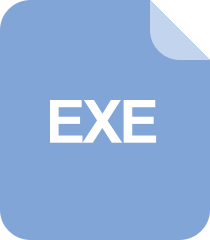
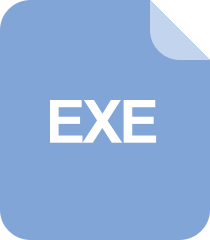
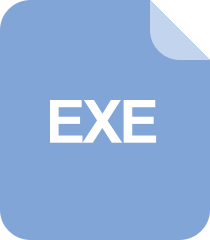
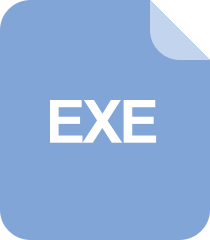
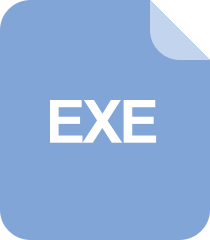
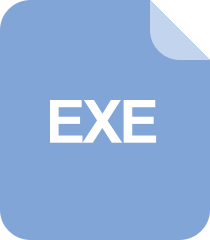
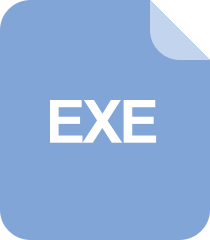
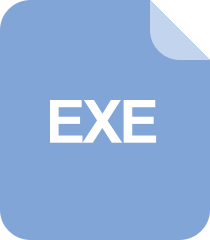
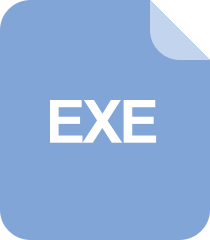
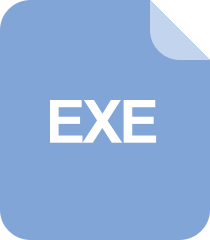
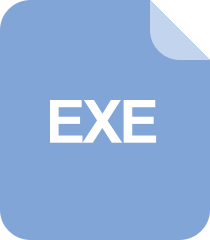
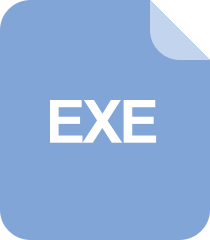
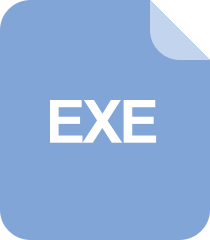
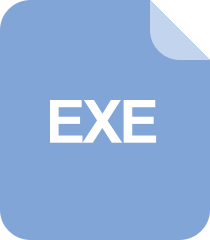
共 11150 条
- 1
- 2
- 3
- 4
- 5
- 6
- 112
资源评论
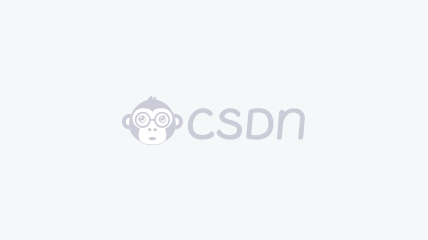

库库新
- 粉丝: 326
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

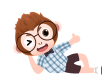
最新资源
- 【岗位说明】公司营销部职位说明书(共7个职位).doc
- 【岗位说明】某公司市场部岗位说明书.doc
- 【岗位说明】某销售总公司各岗位的职责标准.doc
- 【岗位说明】市场部研展工作流程图及具体流程.docx
- 【岗位说明】市场部校园助理职责.doc
- 【岗位说明】市场部职能说明书.doc
- 【岗位说明】市场人员岗位职责.doc
- 【岗位说明】市场营销部部门职责.doc
- 【岗位说明】市场营销部岗位职责.doc
- 【岗位说明】市场营销部各岗位说明书.doc
- 【岗位说明】售后经理岗位职责.doc
- 【岗位说明】市场营销类职位说明书.doc
- 【岗位说明】市场营销部总经理职位说明书.doc
- 【岗位说明】市场与销售类岗位说明书.doc
- 【岗位说明】项目部职能说明书.doc
- 【岗位说明】销售部岗位职责01.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


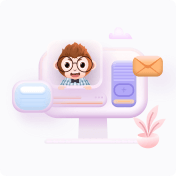
安全验证
文档复制为VIP权益,开通VIP直接复制
