/* eslint-disable */
/**
* jQuery Hiprint 2.5.4
*
* Copyright (c) 2016-2021 www.hinnn.com. All rights reserved.
*
* Licensed under the LGPL or commercial licenses
* To use it on other terms please contact us: hinnn.com@gmail.com
*
*/
"use strict";
function _instanceof(left, right) {
if (right != null && typeof Symbol !== "undefined" && right[Symbol.hasInstance]) {
return !!right[Symbol.hasInstance](left);
} else {
return left instanceof right;
}
}
function _typeof(obj) {
if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") {
_typeof = function _typeof(obj) {
return typeof obj;
};
} else {
_typeof = function _typeof(obj) {
return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj;
};
}
return _typeof(obj);
}
/**
* import 相关资源
*/
import $ from "jquery";
// js颜色选择
import "@claviska/jquery-minicolors/jquery.minicolors.min";
// 条形码
import JsBarcode from "jsbarcode";
// 二维码
import "./plugins/qrcode.js";
import bwipjs from "bwip-js"
// 水印
import watermark from "./plugins/watermark.js";
// 直接打印需要
import {io} from "socket.io-client";
//引入标尺
import lImg from "./css/image/l_img.svg";
import vImg from "./css/image/v_img.svg";
// pdf
import {jsPDF} from "jspdf";
import html2canvas from "@wtto00/html2canvas";
// 数字转中文,大写,金额
import Nzh from "nzh/dist/nzh.min.js";
// 解析svg 到 canvas, 二维码条形码需要
import Canvg from 'canvg';
// 默认自定义拖拽列表
import defaultTypeProvider from "./etypes/default-etyps-provider";
window.$ = window.jQuery = $;
window.autoConnect = true;
window.io = io;
var languages = {}
const ctx = require.context("../i18n", true, /\.json$/);
ctx.keys().forEach(key => {
languages[key.match(/\.\/([^.]+)/)[1]] = ctx(key)
})
var i18n = {
lang: 'cn',
languages,
__: function(key, params) {
var str = this.languages[this.lang][key] || key
if (params && params instanceof Object) {
Object.keys(params).forEach(key => {
str = str.replace(new RegExp(`{{${key}}}`, 'g'), params[key])
})
return str
} else if (params) {
str= str.replace(/%s/g, params)
return str
} else {
return str
}
},
__n: function(key, val) {
var str = this.languages[this.lang][key]
str = str.replace(/%s/g, val)
return str
},
}
var hiprint = function (t) {
var e = {};
function n(i) {
if (e[i]) return e[i].exports;
var o = e[i] = {
i: i,
l: !1,
exports: {}
};
return t[i].call(o.exports, o, o.exports, n), o.l = !0, o.exports;
}
return n.m = t, n.c = e, n.d = function (t, e, i) {
n.o(t, e) || Object.defineProperty(t, e, {
enumerable: !0,
get: i
});
}, n.r = function (t) {
"undefined" != typeof Symbol && Symbol.toStringTag && Object.defineProperty(t, Symbol.toStringTag, {
value: "Module"
}), Object.defineProperty(t, "__esModule", {
value: !0
});
}, n.t = function (t, e) {
if (1 & e && (t = n(t)), 8 & e) return t;
if (4 & e && "object" == _typeof(t) && t && t.__esModule) return t;
var i = Object.create(null);
if (n.r(i), Object.defineProperty(i, "default", {
enumerable: !0,
value: t
}), 2 & e && "string" != typeof t) for (var o in t) {
n.d(i, o, function (e) {
return t[e];
}.bind(null, o));
}
return i;
}, n.n = function (t) {
var e = t && t.__esModule ? function () {
return t.default;
} : function () {
return t;
};
return n.d(e, "a", e), e;
}, n.o = function (t, e) {
return Object.prototype.hasOwnProperty.call(t, e);
}, n.p = "/", n(n.s = 21);
}([function (t, e, n) {/**/
"use strict";
var i;
n.d(e, "a", function () {
return hinnn;
}), window.hinnn = {}, hinnn.event = (i = {}, {
on: function on(t, e) {
i[t] || (i[t] = []), i[t].push(e);
},
id: 0,
off: function off(t, e) {
var n = i[t];
if (n) {
for (var o = -1, r = 0; r < n.length; r++) {
if (n[r] === e) {
o = r;
break;
}
}
o < 0 || i[t].splice(o, 1);
}
},
trigger: function trigger(t) {
var e = i[t];
if (e && e.length) for (var n = Array.prototype.slice.call(arguments, 1), o = 0; o < e.length; o++) {
e[o].apply(this, n);
}
},
clear: function clear(t) {
i[t] = [];
},
getId: function getId() {
return this.id += 1, this.id;
},
getNameWithId: function getNameWithId(t) {
return t + "-" + this.getId();
}
}), hinnn.form = {
serialize: function serialize(t) {
var e = $(t).serializeArray(),
n = {};
return $.each(e, function () {
n[this.name] ? "[object Array]" == Object.prototype.toString.call(n[this.name]) ? n[this.name].push(this.value) : n[this.name] = [n[this.name], this.value] : n[this.name] = this.value;
}), n;
}
}, hinnn.pt = {
toPx: function toPx(t) {
return t * (this.getDpi() / 72);
},
toMm: function toMm(t) {
return hinnn.px.toMm(hinnn.pt.toPx(t));
},
dpi: 0,
getDpi: function getDpi() {
if (!this.dpi) {
var _t2 = document.createElement("DIV");
_t2.style.cssText = "width:1in;height:1in;position:absolute;left:0px;top:0px;z-index:99;visibility:hidden", document.body.appendChild(_t2), this.dpi = _t2.offsetHeight;
}
return this.dpi;
}
}, hinnn.px = {
toPt: function toPt(t) {
return t * (72 / this.getDpi());
},
toMm: function toMm(t) {
return Math.round((t / this.getDpi() * 25.4) * 100) / 100;
},
dpi: 0,
getDpi: function getDpi() {
if (!this.dpi) {
var _t3 = document.createElement("DIV");
_t3.style.cssText = "width:1in;height:1in;position:absolute;left:0px;top:0px;z-index:99;visibility:hidden", document.body.appendChild(_t3), this.dpi = _t3.offsetHeight;
}
return this.dpi;
}
}, hinnn.mm = {
toPt: function toPt(t) {
return 72 / 25.4 * t;
},
toPx: function toPx(t) {
return hinnn.pt.toPx(hinnn.mm.toPt(t));
}
}, hinnn.throttle = function (t, e, n) {
var i,
o,
r,
a = null,
p = 0;
n || (n = {});
var s = function s() {
p = !1 === n.leading ? 0 : _.now(), a = null, r = t.apply(i, o), a || (i = o = null);
};
return function () {
var l = _.now();
p || !1 !== n.leading || (p = l);
var u = e - (l - p);
return i = this, o = arguments, u <= 0 || u > e ? (a && (clearTimeout(a), a = null), p = l, r = t.apply(i, o), a || (i = o = null)) : a || !1 === n.trailing || (a = setTimeout(s, u)), r;
};
}, hinnn.debounce = function (t, e, n) {
var i,
o,
r,
a,
p,
s = function s() {
var l = _.now() - a;
l < e && l >= 0 ? i = setTimeout(s, e - l) : (i = null, n || (p = t.apply(r, o), i || (r = o = null)));
};
return function () {
r = this, o = arguments, a = _.now();
var l = n && !i;
return i || (i = setTimeout(s, e)), l && (p = t.apply(r, o), r = o = null), p;
};
}, hinnn.toUtf8 = function (t) {
var e, n, i, o;
for (e = "", i = t.length, n = 0; n < i; n++) {
(o = t.charCodeAt(n)) >= 1 && o <= 127 ? e += t.charAt(n) : o > 2047 ? (e += String.fromCharCode(224 | o >> 12 & 15), e += String.fromCharCode(128 | o >> 6 & 63), e += String.fromCharCode(128 | o >> 0 & 63)) : (e += String.fromCharCode(192 | o >> 6 & 31), e += String.fromCharCode(128 | o >> 0 & 63));
}
return e;
}, hinnn.groupBy
没有合适的资源?快使用搜索试试~ 我知道了~
统一平台 mes 管理系统 vue
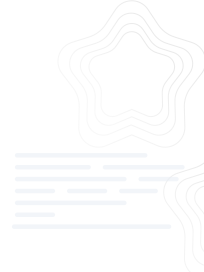
共1384个文件
vue:682个
js:296个
svg:212个

需积分: 5 0 下载量 20 浏览量
2024-11-14
10:49:16
上传
评论
收藏 30.5MB ZIP 举报
温馨提示
mes 管理系统
资源推荐
资源详情
资源评论
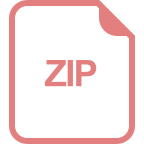
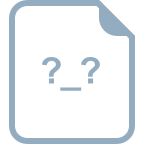
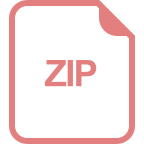
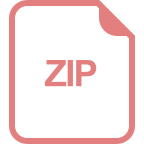
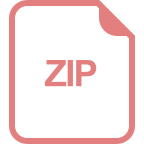
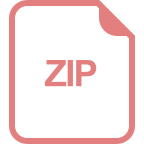
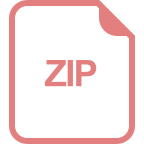
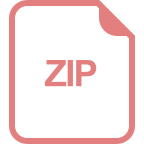
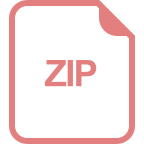
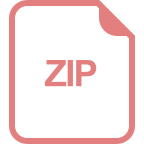
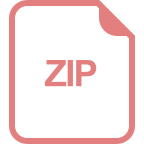
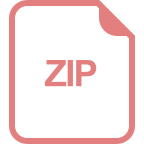
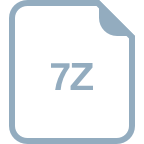
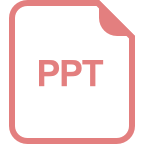
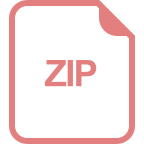
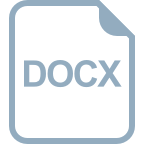
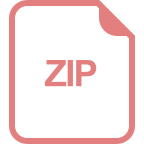
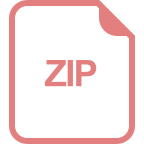
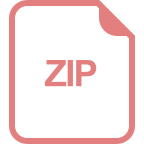
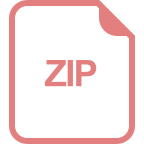
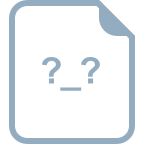
收起资源包目录

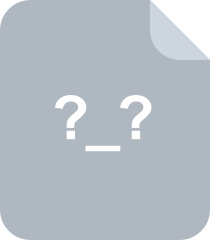
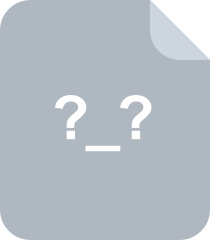
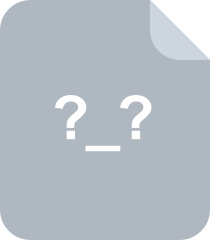
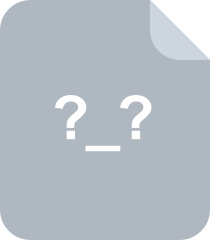
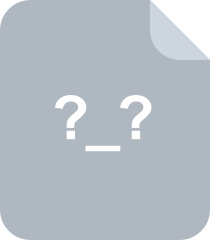
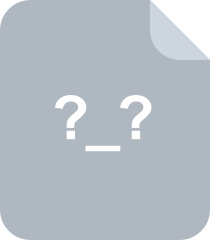
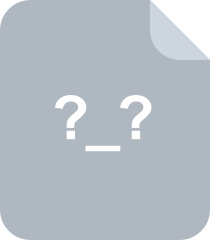
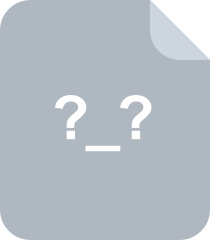
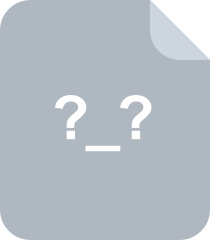
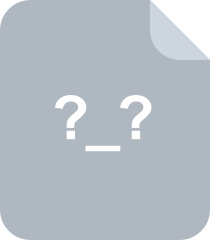
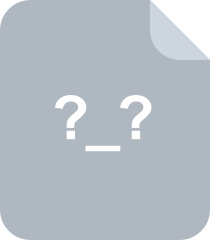
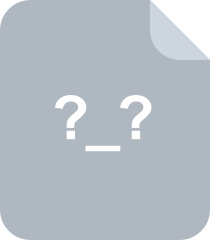
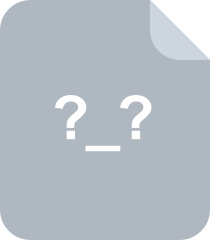
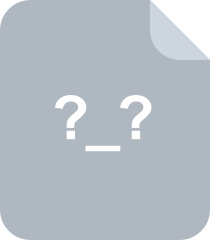
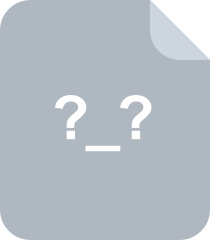
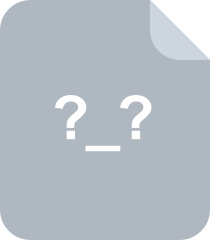
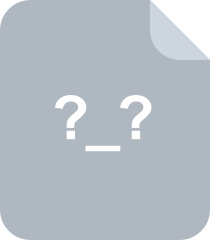
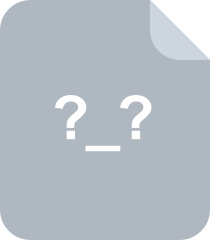
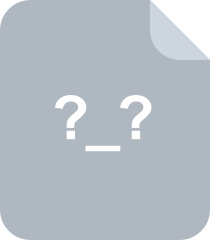
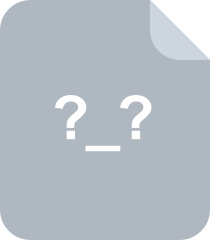
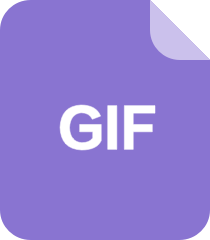
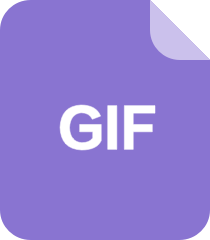
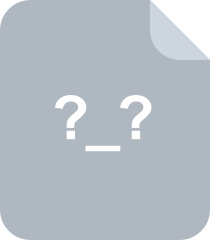
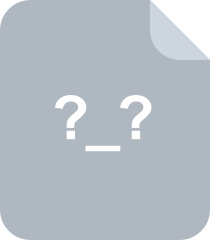
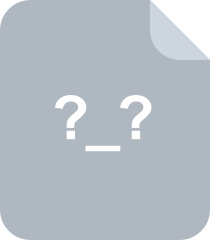
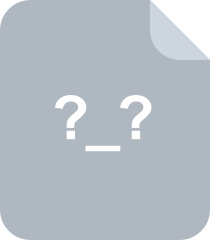
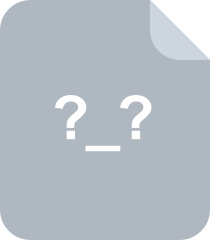
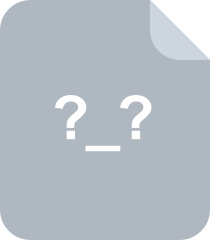
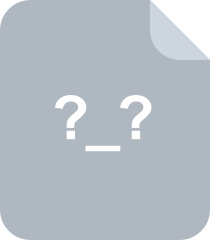
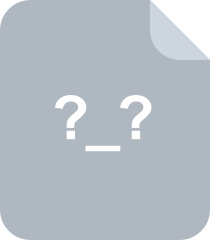
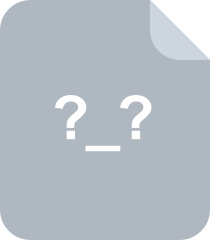
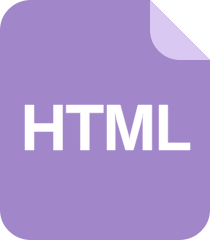
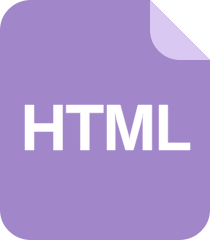
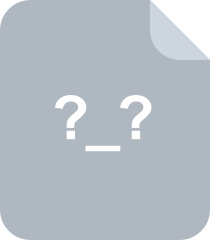
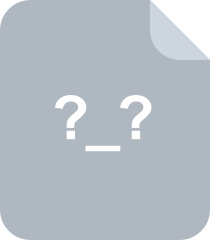
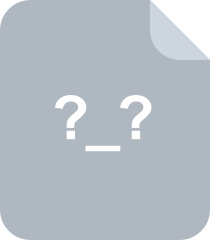
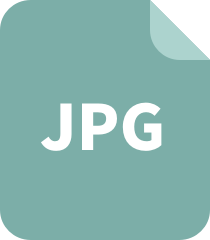
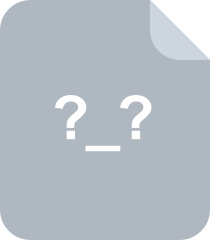
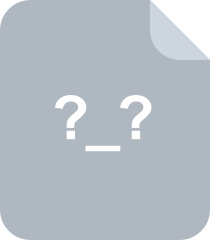
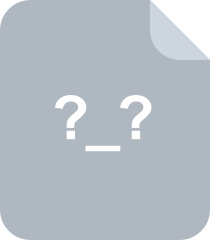
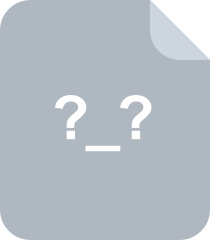
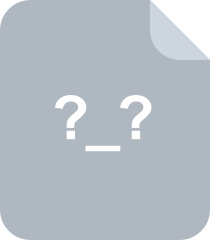
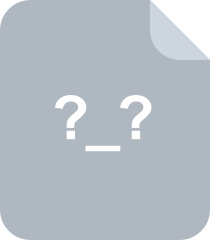
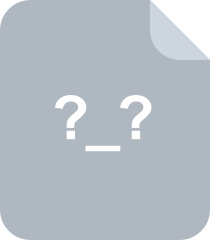
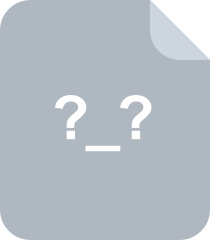
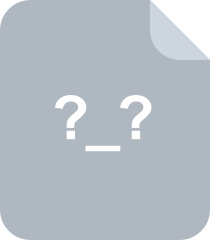
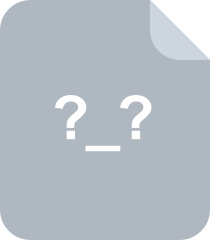
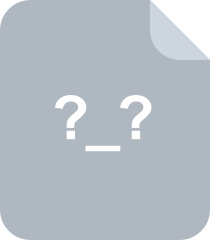
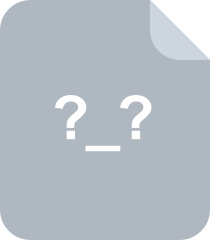
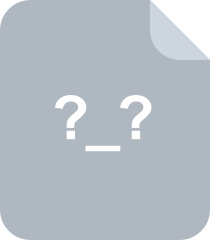
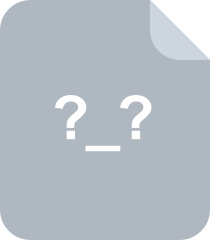
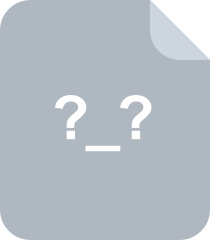
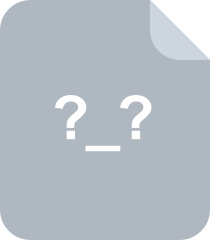
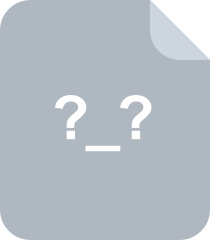
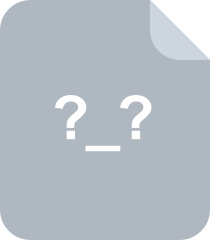
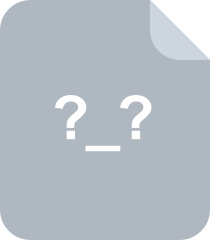
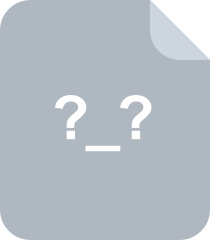
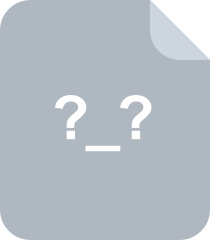
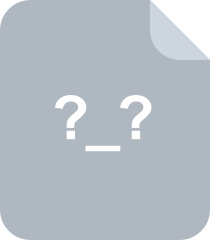
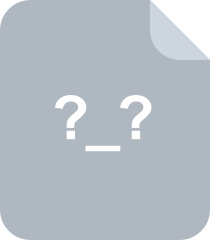
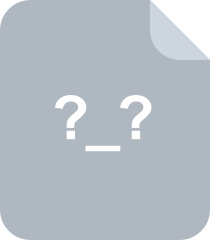
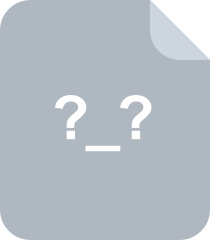
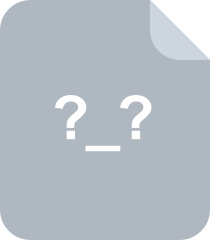
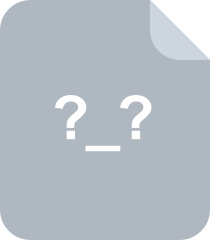
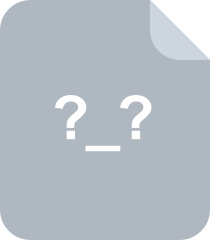
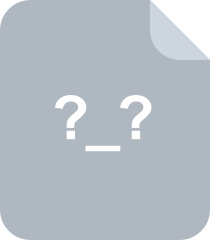
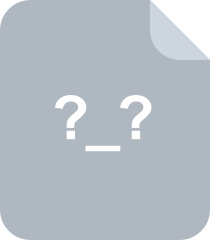
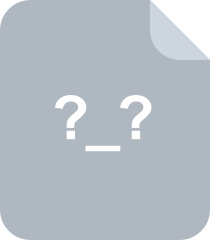
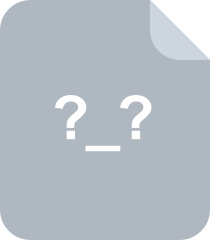
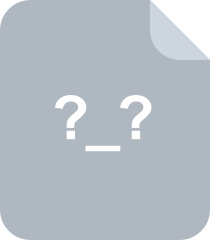
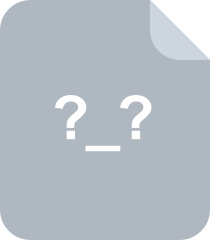
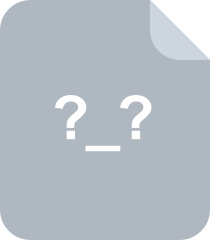
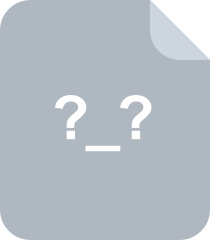
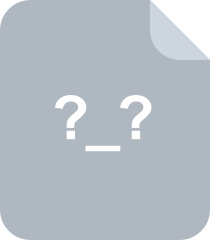
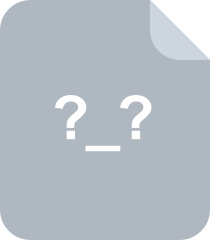
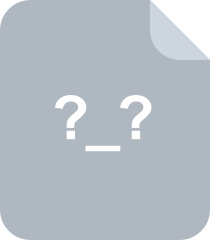
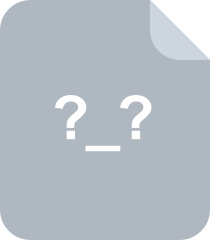
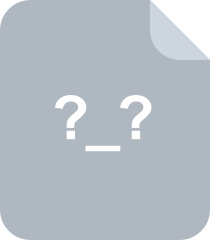
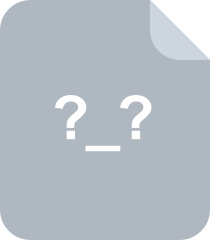
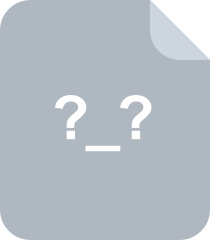
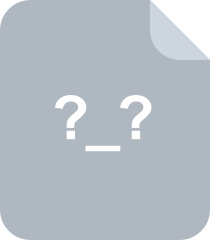
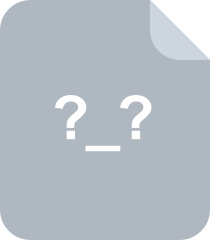
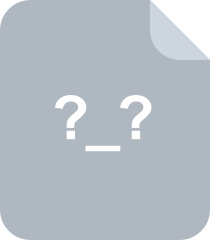
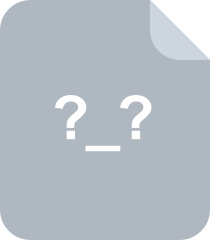
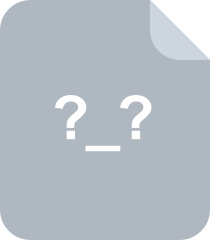
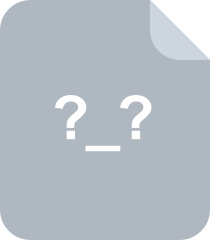
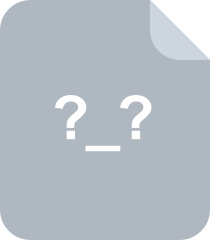
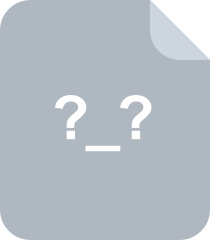
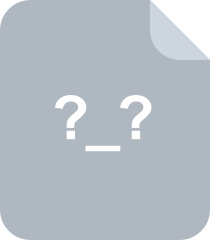
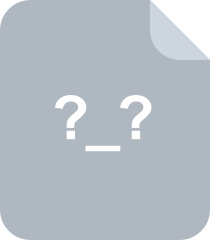
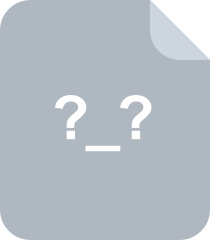
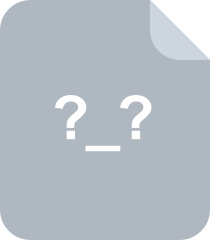
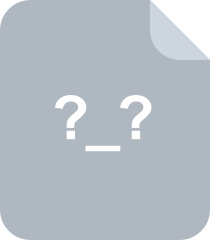
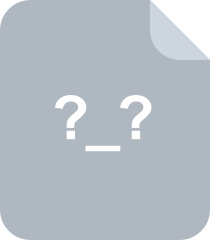
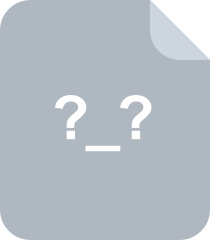
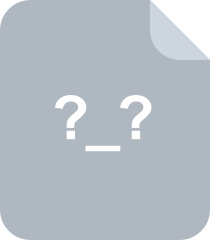
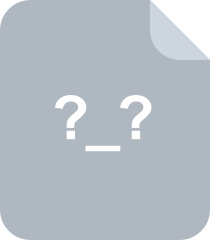
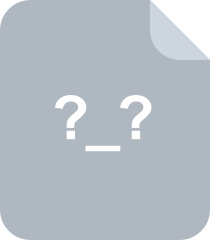
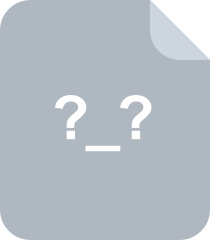
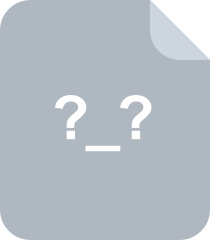
共 1384 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
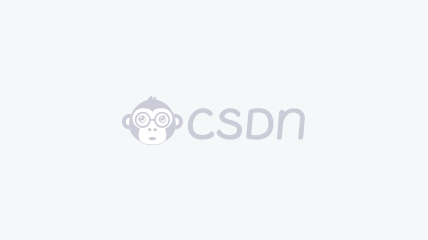

kowalsik
- 粉丝: 5
- 资源: 19
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

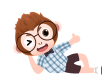
最新资源
- 基于ARIMA-LSTM-transformer等模型进行流感时间序列预测Python源码(高分项目)
- 基于景观生态风险评价的流域景观格局优化,教学视频和资料,喜欢的就下载吧,保证受用
- java设计模式-建造者模式(Builder Pattern)
- C语言刷题-lesson5_1731564764305.pdf
- JavaScript开发指南PDG版最新版本
- JavaScript程序员参考(JavaScriptProgrammer'sReference)pdf文字版最新版本
- jQuery1.4参考指南的实例源代码实例代码最新版本
- CUMCM-2018-D.pdf
- 私钥+助记词碰撞器 概括了BTC ETH BNB TRX SOL各链 最新版
- jQueryapi技术文档chm含jQuery选择器使用最新版本
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


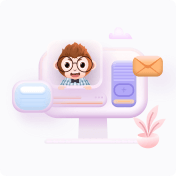
安全验证
文档复制为VIP权益,开通VIP直接复制
